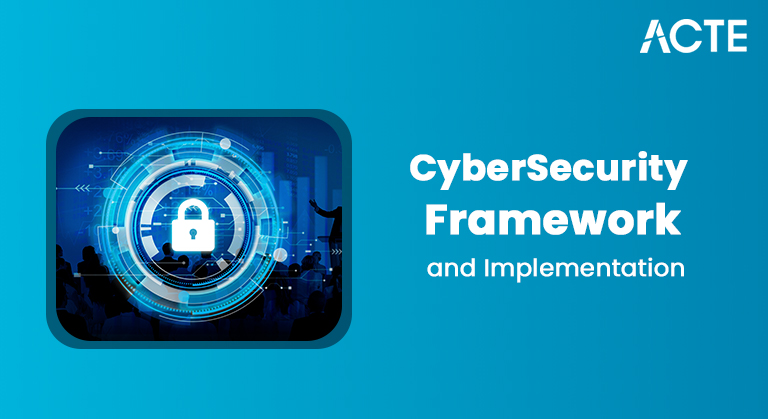
- Introduction to Docker Compose
- Setting Up Docker Compose
- Docker Compose File Structure
- Docker Compose Commands
- Docker Compose Use Cases
- Multi-Container Applications with Docker Compose
- Debugging Docker Compose Issues
- Conclusion
Introduction to Docker Compose
Docker Compose is a tool that allows developers to define and run multi-container Docker applications. It enables the easy management of multi-container environments by using a single configuration file to define all services, networks, and volumes required for a project. Instead of managing containers individually, Docker Compose simplifies the process by managing everything from a single YAML configuration file. Docker Compose is particularly useful for applications that require multiple services (e.g., a web server, database, caching layer) to work together. It allows you to manage dependencies, scale services, and deploy complex environments easily. Docker Training Compose also facilitates the process of testing applications locally, mimicking production environments with ease. It supports multiple environments and configurations, making it easy to switch between different setups for development, testing, and production. With the ability to define volumes and networks, it ensures that services can communicate and store data persistently. Docker Compose can be integrated into CI/CD pipelines to streamline the deployment process. Additionally, it allows developers to define service dependencies, ensuring that each service starts in the correct order.
Setting Up Docker Compose
To set up Docker Compose, you first need to install Docker and Docker Compose on your system.
- Install Docker:Follow the installation guide on the Docker website to install Docker based on your operating system (Linux, macOS, or Windows).
- Install Docker Compose:
On Linux:
- #sudo curl-L”https://github.com/docker/compose/releases/download/$(curl -s https://api.github.com/repos/docker/compose/releases/latest | jq -r .tag_name)/docker-compose-$(uname -s)-$(uname -m)” -o /usr/local/bin/docker-compose
- # sudo chmod +x /usr/local/bin/docker-compose
On macOS and Windows, Docker Compose comes bundled with the Docker Desktop installation.
- Verify Installation: After installation, verify Docker Compose is installed by running:
- # docker-compose –version
- Version:The version specified in the Docker Compose file determines the syntax and features available for use. Docker Swarm Architecture important to choose the correct version to ensure compatibility with the features your application requires.
- # version: ‘3’
- Services:Each service in Docker Compose represents a single container and can be customized with specific settings like environment variables, networks, and dependencies. By defining services, you can manage the entire application’s infrastructure in a single file.
- services:
- web:
- image: nginx
- ports:
- – “80:80”
- db:
- image: mysql
- environment:
- MYSQL_ROOT_PASSWORD: exampl
- Networks: Networks allow services to discover and communicate with each other by name, ensuring they can interact within a defined environment. You can also control the scope of communication by creating multiple networks for isolation or segmentation.
- networks:
- default:
- driver: bridge
- Volumes:Volumes allow data to be stored outside of a container, ensuring that data persists even if the container is removed or recreated. This is especially useful for databases or other services where data needs to be retained across restarts.
- volumes:
- db_data:
- Build:The build context defines the directory where Docker will look for the Dockerfile and other necessary files to build the image. You can also specify additional build arguments or configurations within this context for more customized builds.
- services:
- web:
- build:
- context: ./app
- dockerfile: Dockerfile
- Example Docker Compose File:
- version: ‘3’
- services:
- web:
- image: nginx
- ports:
- – “80:80”
- networks:
- – frontend
- db:
- image: mysql:5.7
- environment:
- MYSQL_ROOT_PASSWORD: example
- networks:
- – frontend
- – backend
- redis:
- image: redis
- networks:
- – backend
- networks:
- frontend:
- backend:
- docker-compose up: Starts all services defined in the docker-compose.yml file
- # docker-compose up
- -d (detached mode) runs containers in the background.
- –build forces a rebuild of images before starting the containers.
- #docker-compose up -d
- docker-compose down:Stops and removes containers, networks, and volumes defined in the Compose file.
- # docker-compose down
- docker-compose ps:Lists the containers that are running in the Compose application.
- # docker-compose ps
- docker-compose build: Builds the images for the services defined in the Compose file.
- #docker-compose build
- docker-compose logs: Displays the logs from all services or a specific service.
- # docker-compose logs
- docker-compose exec: Run a command in a running container.
- # docker-compose exec
- # docker-compose exec web bash
- docker-compose stop:Stop the services without removing them.
- #docker-compose stop
- docker-compose restart: Restarts services after a change in the configuration or environment.
- #docker-compose restart
- Microservices Architectures: Docker Compose simplifies the orchestration of microservices by managing the various services (e.g., web servers, databases, caching services) in one configuration file. It enables easy scaling and management of multiple containers running together.
- Local Development Environments:Developers can use Docker Compose to quickly set up and replicate production-like environments locally. For example, you Ansible Kubernetes and Docker set up a web application with a database, caching layer, and message broker all running together on a local machine.
- Testing:Docker Compose is ideal for setting up test environments that replicate production environments. It allows teams to run integration and functional tests with a consistent environment for every test run.
- CI/CD Pipelines:In a CI/CD pipeline, Docker Compose can be used to run multiple containers for building, testing, and deploying applications. It ensures that all services (such as databases and APIs) are running in a controlled environment before deployment.
- Multi-Container Applications: Docker Compose is perfect for applications that require multiple containers to work together, such as applications with a front-end and back-end that are split into separate services. Instead of manually managing each container, Compose lets you define how these containers interact and manage dependencies between them.
- Continuous Integration and Delivery (CI/CD) Automation: In CI/CD pipelines, Docker Compose is often used to create environments where developers can run tests or deploy applications automatically. It can set up environments that are easily reproducible in different stages of the pipeline (e.g., staging, production), helping to avoid discrepancies between environments.
- Monitoring and Logging: Docker Compose can be utilized for running monitoring tools (e.g., Prometheus, Grafana) and log aggregation systems (e.g., ELK Stack) as part of the application stack. Ansible Tower allows developers to easily monitor the health and performance of the various services running within the containers in a unified way.
- version: ‘3’
- services:
- web:
- image: nginx
- ports:
- – “80:80”
- networks:
- – frontend
- app:
- image: node:14
- environment:
- – DB_HOST=db
- depends_on:
- – db
- networks:
- – frontend
- – backend
- db:
- image: postgres:latest
- environment:
- – POSTGRES_PASSWORD=secret
- networks:
- – backend
- redis:
- image: redis
- networks:
- backend
- networks:
- frontend:
- backend:
- version: ‘3.8’
- services:
- web:
- image: nginx:latest
- ports:
- – “8080:80”
- volumes:
- – ./html:/usr/share/nginx/html
- networks:
- – app-network
- db:
- image: mysql:5.7
- environment:
- MYSQL_ROOT_PASSWORD: example
- MYSQL_DATABASE: testdb
- volumes:
- – db-data:/var/lib/mysql
- networks:
- – app-network
- networks:
- app-network:
- driver: bridge
- volumes:
- db-data:
Once Docker Compose is installed, you can begin creating docker-compose.yml files to define your multi-container application environments.
Docker Compose File Structure
The docker-compose.yml file defines how the containers for your application should be built and connected. It uses YAML syntax and includes several key sections:
Become a Docker expert by enrolling in this Docker Online Course today.
Docker Compose Commands
Here are some essential Introduction to Docker Networking Compose commands for managing your application lifecycle:
Command:
Options:
Example:
Command:
Command:
Command:
Command:
Command:
Example:
Command:
Docker Compose Use Cases
Docker Compose is commonly used in a variety of scenarios, Docker Compose is commonly used in a variety of scenarios.Docker Compose simplifies the management of multi-container applications, allowing users to define and run multiple services in a single configuration file (`docker-compose.yml`).It helps in deploying and managing microservices, where each service runs in its container and interacts with others.Developers use Docker Compose to replicate complex production environments locally for easier testing and debugging.Docker Compose can be integrated into CI/CD pipelines to automatically set up and tear down environments during automated testing.It makes scaling individual services within an application easy with a simple command to increase the number of containers running a service.Compose automatically sets up a private network for the services, enabling secure communication between containers.Docker Compose allows you to manage environment variables and configuration settings for different services.It handles the orchestration of starting, stopping, and managing containerized services in the correct order.By defining services and configurations in a version-controlled `docker-compose.yml`, Docker Compose ensures that environments are consistent across teams.Easy service updates With a single command (`docker-compose up`), users can update services to newer versions and apply changes to containers effortlessly.
Dive into Docker by enrolling in this Docker Online Course today.
Take charge of your Cloud Computing career by enrolling in ACTE’s Cloud Computing Master Program Training Course today!
Multi-Container Applications with Docker Compose
Docker Compose is specifically designed to manage multi-container applications. For example, a web application might need a front-end service (e.g., Nginx), a back-end service (e.g., Node.js), a database (e.g., PostgreSQL), and a caching service (e.g., Redis). With Docker Compose, you can define all these services in one configuration file, making Docker Training easier to launch, stop, and scale the application.
Example: Multi-Container Application
In this example, you have a web server (Nginx), an application (Node.js), a database (PostgreSQL), and a caching service (Redis) running as separate containers, all defined in the docker-compose.yml file. The services communicate with each other over separate networks (frontend, backend).
Docker Sample Resumes! Download & Edit, Get Noticed by Top Employers! DownloadDebugging Docker Compose Issues
While Docker Compose simplifies managing multi-container applications, troubleshooting can still be challenging. To debug issues, start by checking the logs with docker-compose logs to identify errors or How to Install Docker on Ubuntu. If containers aren’t starting, running the application in the foreground (docker-compose up without -d) can provide more detailed output. Verify that all containers are healthy and running with docker-compose ps, and check individual container logs for more insights. If a container is running but not functioning as expected, use docker-compose exec to enter the container’s shell for further investigation. It’s also essential to validate your docker-compose.yml file for syntax issues, missing dependencies, or incorrect configurations. Network configuration can cause communication issues between services, so ensure proper network setup in your docker-compose.yml. Additionally, check Docker and Docker Compose version compatibility, as some features may not work with older versions. Be mindful of resource allocation, as containers may face issues due to memory or CPU limits. Verify that volumes and mounts are properly configured to ensure data persistence, and check that port bindings don’t conflict with other services. If there are communication issues, inspect the Docker networks and ensure services are correctly linked to the appropriate network. If you’re still experiencing issues, consider rebuilding your containers using docker-compose build to ensure that the latest changes to the Dockerfile or dependencies are applied. Additionally, check for permission issues with mounted volumes, as incorrect permissions can cause containers to fail to read or write data. It’s also helpful to test containers independently using docker run to isolate specific service problems before using Docker Compose. Ensure that environment variables and configuration files are correctly loaded, especially if Improve Code Quality with CI CD Automation differ across environments. Lastly, consult the Docker and Docker Compose documentation, as new features or deprecated options may provide solutions or cause unexpected behavior. Example of a docker-compose.yml file that defines a simple multi-container application with a web service (using Nginx) and a database service (using MySQL). This example also includes troubleshooting techniques mentioned earlier, such as logging and checking service status.
docker-compose.yml
Preparing for Docker interviews? Visit our blog for the best Docker Interview Questions and Answers!
Conclusion
Docker Compose is an invaluable tool for developers and DevOps teams managing multi-container applications. It simplifies the orchestration of various services, ensuring that complex environments can be easily defined, configured, and managed using a single YAML file. Whether you’re building microservices, replicating production environments locally, setting up testing environments, or automating deployment through CI/CD pipelines, Docker Training streamlines the process and reduces configuration errors. By using Docker Compose, you can ensure that your application components—such as web servers, databases, and caching systems are consistently deployed and scaled. Additionally, it facilitates collaboration by making it easy to share environment configurations and manage dependencies between services. With robust debugging capabilities and clear service definitions, Docker Compose helps to resolve issues faster, ensuring that you can focus more on development and less on managing individual containers. Overall, Docker Compose is a powerful tool that enhances productivity, collaboration, and efficiency, making it an essential component for managing modern containerized applications.