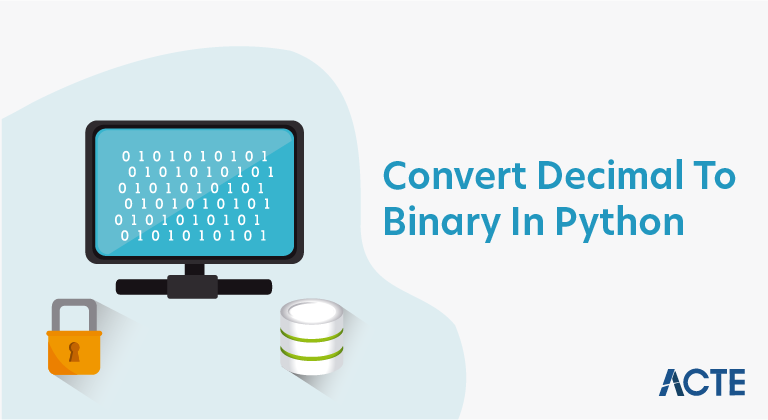
Must-Know Convert Decimal To Binary In Python & How to Master It
Last updated on 04th Jun 2020, Blog, General
Python is a highly versatile and capable programming language. Among the many things one can do, converting from decimal to binary and vice versa is one that stands out the most. Thus in this article, we will speak more about how to convert decimal to binary in Python and vice versa.
Following pointers will be covered in this article,
- Converting Decimal To Binary In Python
- Sample Program
- Making Use Of Bin Function
- Binary To Decimal In Python
Let’s begin!
To understand what this operation means, check out the example below.
From decimal to binary
Input : 8
Output : 1000
From binary to decimal
Input : 100
Output : 4
Let us see how to convert Decimal to Binary in Python,
Converting Decimal To Binary In Python
In order to convert decimal to binary, take a look at the example below.
Keep calling conversion function with n/2 till n > 1,
later perform n % 1 to get MSB of converted binary number.
Example: 7
- 7/2 = Quotient = 3(greater than 1), Remainder = 1.
- 3/2 = Quotient = 1(not greater than 1), Remainder = 1.
- 1%2 = Remainder = 1.
Therefore, the answer is 111.
Let us see a sample program,
Sample Program
- # Function to print binary number for the
- # input decimal using recursion
- def decimalToBinary(n):
- if(n > 1):
- # divide with integral result
- # (discard remainder)
- decimalToBinary(n//2)
- print(n%2, end=’ ‘)
- # Driver code
- if __name__ == ‘__main__’:
- decimalToBinary(8)
- print(“n”)
- decimalToBinary(18)
- print(“n”)
- decimalToBinary(7)
- print(“n”)
The output of the above program will look something like this.
1000
10010
111
We can convert Decimal To Binary in Python using bin function as well, let us see how,
Making Use Of Bin Function
- #Function to convert Decimal number
- # to Binary number
- def decimalToBinary(n):
- return bin(n).replace(“0b”,””)
- # Driver code
- if __name__ == ‘__main__’:
- print(decimalToBinary(8))
- print(decimalToBinary(18))
- print(decimalToBinary(7))
The output of the above program will look something like this
1000
10010
111
Now that you know how to convert from decimal to binary in Python, let’s see how to do the reverse that is binary to decimal.
Binary To Decimal In Python
To understand this better, refer to the example below.
Example: 1011
- Take the modulo of given binary number with 10.
(1011 % 10 = 1)
- Multiply rem with 2 raised to the power.
Next it’s position from right end : (1 * 2^0)
Note that we start counting positions with 0.
- Add result with previously generated result.
decimal = decimal + (1 * 2^0)
- Update binary number by dividing it by 10.
(1011 / 10 = 101)
- Keep repeating upper steps till binary > 0.
Final Conversion -: (1 * 2^3) + (0 * 2^2) + (1 * 2^1) + (1 * 2^0) = 11
Let us take a look at a sample program,
Sample Program
When the above program is executed, the output will look like this.
4
5
9
Let us move to the last bit of this Decimal to Binary in Python article.
Sample Program
- # Function to convert Binary number
- # to Decimal number
- def binaryToDecimal(n):
- return int(n,2)
- # Driver code
- if __name__ == ‘__main__’:
- print(binaryToDecimal(‘100’))
- print(binaryToDecimal(‘101’))
- print(binaryToDecimal(‘1001’))
The output of the above program will be
4
5
9
To find a binary equivalent, the decimal number has to be recursively divided by the value 2 until the decimal number reaches the value zero. After each and every division, the remainder has to be noted. The reverse of the remainder values gives the binary equivalent of the decimal number.
For example, the binary conversion of the decimal number 15 is shown in the below image.
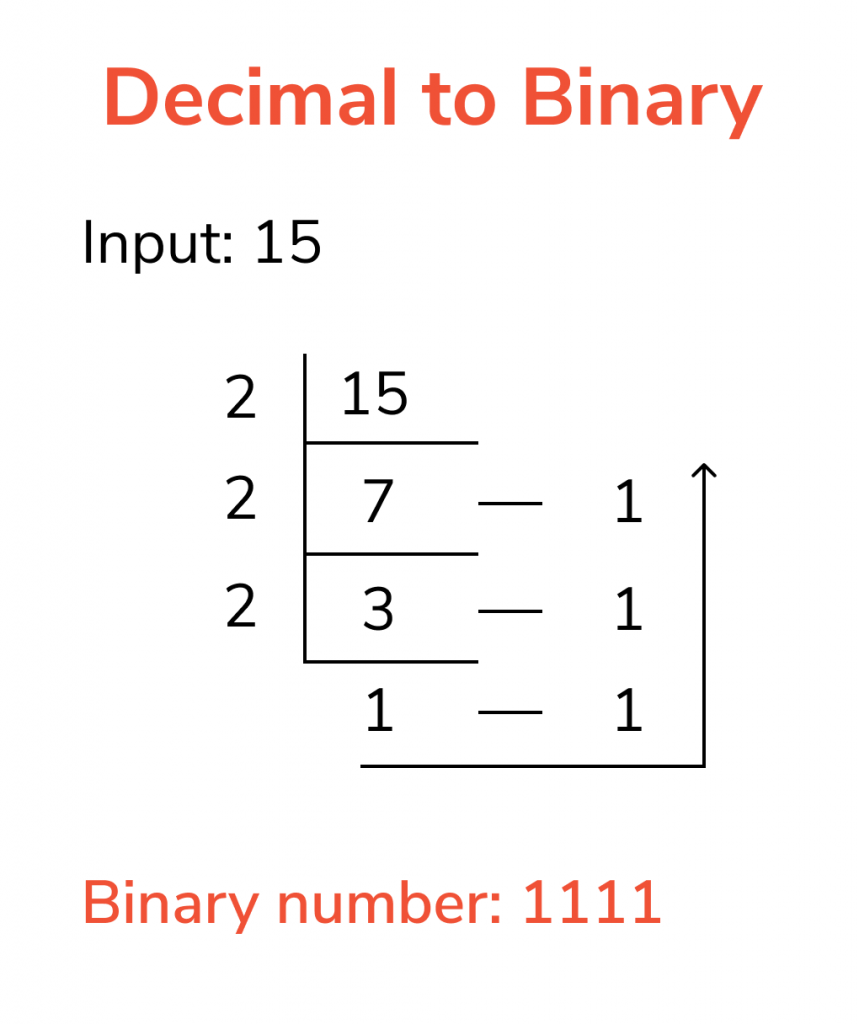
INPUT FORMAT:
Input consists of an integer
OUTPUT FORMAT:
Binary equivalent of the given input decimal number
SAMPLE INPUT:
14
SAMPLE OUTPUT:
1110
Algorithm for converting Decimal to Binary
Step 1: Get an input value from the user
Step 2: If the input is greater than zero, divide the number by the value 2 and note the remainder
Step 3: Repeat Step 2 until the decimal number reaches zero
Step 4: Print the remainder values
Step 5: End
Method1: Using user-defined function
Python program to convert the given decimal number to binary using a user-defined function
- def decimalToBinary(num):if num > 1:
- decimalToBinary(num // 2)
- print(num % 2, end = ”)
- number = int(input(“Enter the decimal number: “))
- #main() function
- decimalToBinary(number)
Input:
Enter the decimal number: 25
Output:
11001
PREREQUISITE KNOWLEDGE: Built-In Functions in Python
Method 2: Using built-in function
When we use the built-in function, the output will be obtained with the prefix ‘ob’.
Python program to convert the given decimal number to binary using a built-in function
- number = int(input(“Enter the decimal number: “))
- print(bin(number))
Input:
Enter the decimal number: 56
Output:
Binary Number: 0b111000
This brings us to the end of this article on Decimal To Binary In Python.