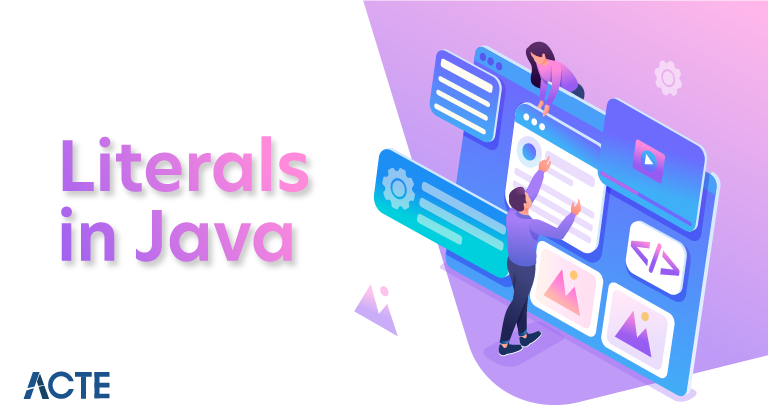
Literals in Java: A Complete Guide with Best Practices
Last updated on 04th Jun 2020, Blog, General
What are Literals in Java?
They are used to represent the constant value. Literals are source code representation of a fixed value or the sequence of characters which represents the constant value that is to be stored in a variable.
There are five types of literals in Java.
- Integer Literals
- Boolean Literals
- Character Literals
- String Literals
- Floating Point Literals
Integer Literals in Java
There are four primitive data types present in integer literals, they are a byte, short, long, int and we can represent these in 4 ways:
Decimal literals:
Any number from 0-9 are allowed here.
Ex: int a=100;
Octal literals:
Any number from 0-7 is allowed here, but the value should start from 0 only.
Ex: int b=035;
Hexa-decimal literals:
Here the digit from 0-9 and characters from a-f are allowed. The value can be a combination of characters and digit. We can use both uppercase and lower-case characters here, for this it is not case-sensitive. This value should start from 0X or 0x.
Ex: int c=0X238ce;
Binary literals:
It consists of only two-digit 0 and 1, but the value should start from 0b or 0B.
Ex: int d=0B1101;
See the following program.
- public class Integer {
- public static void main(String args[]) {
- int a = 100; // decimal literals
- int b = 035; // octal literals
- int c = 0X238ce; // hexadecimal literals
- int d = 0B1101; // binary literals
- System.out.println(a);
- System.out.println(b);
- System.out.println(c);
- System.out.println(d);
- }
- }
See the following output.
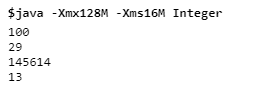
Floating-point literals
Floating-point literals only contain decimal values with a fractional component. It consists of a double and floats data type.
Ex:
- double a=3.24343;
- Float b=45.34f;
See the following program.
- public class Floating {
- public static void main(String[] args) {
- double a = 3.24343;
- float b = 45.34f;
- System.out.println(a);
- System.out.println(b);
- }
- }
See the following output.
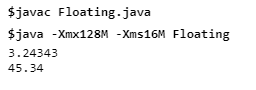
By default, every floating-point literal is of double type, and hence we cant assign directly to float variable. But we can specify floating-point literal as float type by suffixed with f or F. We can specify explicitly floating-point literal as double type by suffixed with d or D., and Of course, this convention is not required.
Character Literals
It is a 16-bit Unicode, and it is enclosed in single quotes whereas in the string is enclosed in double quote.
Ex:
- Char ch=’a’;
- Char ch1=’#’;
- Char ch2=’4’;
For the char data types, we can define the literals in 4 ways:
Single quote
We can specify literal to a char data type as a single character within a single quote.
- char ch = ‘a’;
Char literal as Integral literal
We can define the char literal as an integral literal which represents the Unicode value of a character and those integral literals can be defined either in the Decimal, Octal, and Hexadecimal forms.
- But the final allowed range is 0 to 65535.
- char ch = 062;
Unicode Representation
We can define the char literals in Unicode representation ‘\uxxxx’. Here xxxx represents 4 hexadecimal numbers.
- char ch = ‘\u0061’;
- Here /u0061 represent a.
Escape Sequence
Every escape character can be specified as char literals.
- char ch = ‘\n’;
See the following program.
- public class Test {
- public static void main(String[] args) {
- char ch1 = ‘a’;
- char ch2 = ‘#’;
- char ch3 = ‘4’;
- System.out.println(ch3);
- System.out.println(ch2);
- System.out.println(ch3);
- }
- }
See the output.
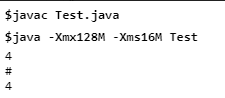
String Literals
They are the sequence of characters that are enclosed in double-quotes, and it can be a sentence too.
Any sequence of the characters within the double quotes is treated as String literals.
- String str1=” how are you?”;
- String str2=” I’m good thanks!”;
See the following program.
- public class Test {
- public static void main(String[] args) {
- String str1 = “how are you?”;
- String str2 = ” I’m good thanks!”;
- System.out.println(str1);
- System.out.println(str2);
- }
- }
See the output:
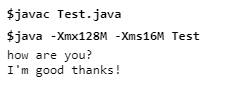
String literals may not contain unescaped newline or linefeed characters. However, the Java compiler will evaluate compile-time expressions.
Boolean literals
Only two values can be represented in Boolean literals, i.e. either true or false. These two values can be assigned to a variable. These true and false are case sensitive in java.
Ex:
- boolean flg1=” true”;
- boolean flg2=” false”;
See the program of Boolean literals.
- public class Boolean {
- public static void main(String args[]) {
- boolean flg1 = true;
- boolean flg2 = false;
- System.out.println(flg1);
- System.out.println(flg2);
- }
- }
See the output:
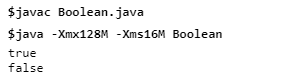
- When we are performing concatenation operations, then the values in brackets are concatenated first. Then the values are concatenated from left to right.
- We should be careful when we are mixing character literals and integers in String concatenation operations, and this type of operation is known as Mixed Mode operation.