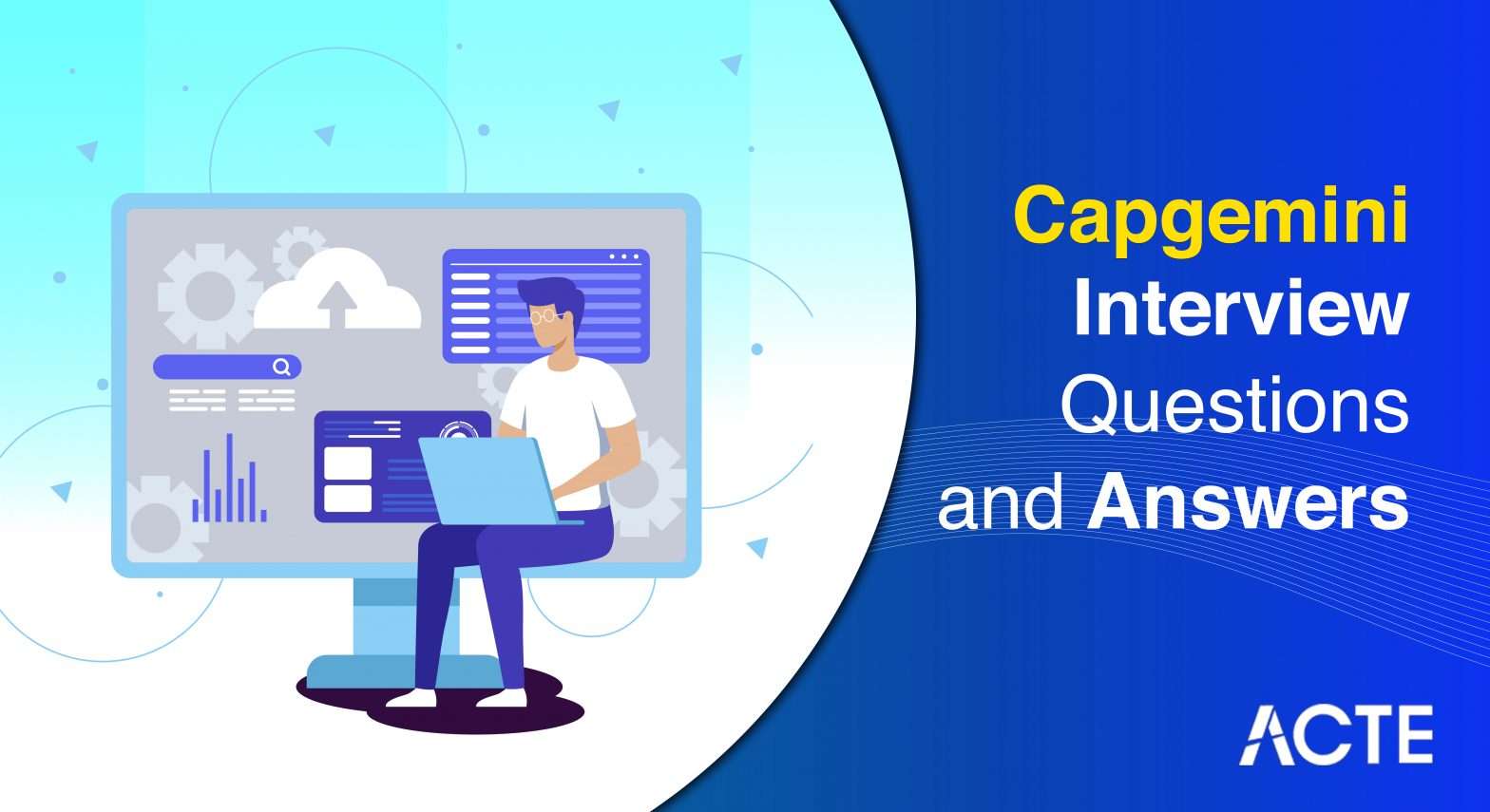
Capgemini is a worldwide leader in consulting, technology services, and digital transformation. Emphasizing innovation and sustainability, the company collaborates with clients to provide customized solutions that enhance efficiency and foster growth. With a broad array of services, Capgemini assists organizations in navigating the challenges of the digital landscape to achieve their strategic goals.
1. Describe object-oriented programming (OOP) concepts.
Ans:
Object-oriented programming (OOP) is a software design and structuring paradigm with a program written as a collection of objects interacting. The four fundamental concepts of OOP are encapsulation, inheritance, polymorphism, and abstraction. Encapsulation This is bundling data with methods that operate on that data within a single unit or object and controlling access to the internal state. Inheritance A new class inherits properties and methods from an existing class; this thus promotes code reuse.
2. What is polymorphism? How is it implemented in Java/C++?
Ans:
- Polymorphism is the ability of different objects to be treated as instances of the same class through a common interface, enabling a single function or method to operate on various data types.
- The main methods used in Java to implement polymorphism are interfaces and method overriding. For example, one can define a method in the superclass, leaving its implementation to subclasses.
- In this case, one can call the same method for objects of different classes. Polymorphism can also be achieved in C++ by function overloading, where one can declare multiple functions with the same name but with parameters that are different in number or type.
3. What are the differences between overloading and overriding?
Ans:
- Overloading and overriding are two techniques used in object-oriented programming. We will compare overloading and overriding based on their distinct purposes.
- Overloading occurs whenever two or more methods in the same class have the same name but differ in the type or number of parameters.
- This method enables methods to be called in different contexts based on the provided arguments, enhancing flexibility. Overriding involves a subclass redefinition of a superclass method, supplying a specific implementation tailored for the subclass.
4. What is inheritance? Give an example.
Ans:
Inheritance is an OOP concept where a subclass (derived class) inherits characteristics and methods from a superclass (base class). This mechanism promotes code reuse and establishes a class hierarchy. For example, a base class called ‘Animal’ includes methods like ‘eat()’ and ‘sleep()’. A derived class ‘Dog’ can inherit these methods and add specific behavior, such as ‘bark()’, thus illustrating inheritance by extending the functionality defined in the ‘Animal’ class.
5. What are design patterns?
Ans:
A design pattern is a reusable solution to common problems in software design. It proposes a generic solution to a widely faced set of design problems and encourages best practices in software development. The commonly used patterns are the Singleton, which limits the instantiation of a class to just one instance; the Observer, defined as one-to-many dependency between objects so that a change to one object may notify others; and Factory, which creates objects without specifying the concrete class of object to be made.
6. Explain the differences between an interface and an abstract class.
Ans:
Interfaces and abstract classes define contracts for courses in object-oriented programming but have distinct characteristics. An interface defines a contract with only abstract methods (before Java 8) and constants, requiring implementing classes to provide concrete implementations for all methods. Also, an abstract class can have both abstract and concrete methods to provide common functionality.
7. What are the key features of the Java Virtual Machine (JVM)?
Ans:
- The JVM is the heart of the Java platform. It executes Java bytecode and ensures that applications can run without platform dependency.
- Key features of a JVM include garbage collection, which automatically manages memory allocations and deallocations; JIT compilation, whereby the JVM translates bytecode into native machine code for better performance; and exception handling, wherein the JVM hosts runtime errors cleanly and nicely.
- Finally, the JVM also gives a safe execution environment through its classloader and security manager that should not allow access to system resources without permission.
8. How does garbage collection work in Java?
Ans:
- Garbage collection in Java is the automatic memory management process that identifies and frees up memory occupied by objects no longer in use. It prevents memory leaks and optimizes resource utilization.
- The JVM periodically performs garbage collection by scanning the heap for unreachable objects, meaning they are no longer referenced by any part of the application.
- These identified objects are possible candidates for garbage collection and their memory is recovered to be reused in new allocations.
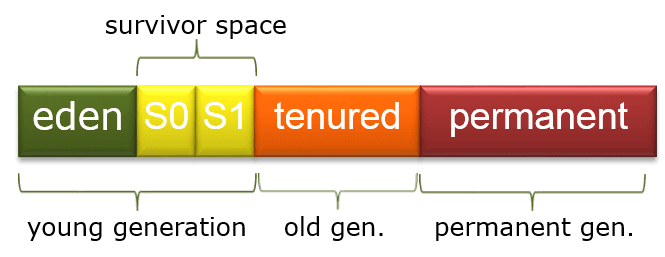
9. What are SOLID principles in software design?
Ans:
SOLID principles are five key design guidelines that improve software development by enhancing maintainability and flexibility. The Single Responsibility Principle asserts that a class should focus on a single task and have one reason to change. The Open/Closed Principle promotes the idea that software entities should be open to extension but closed to modification. The Liskov Substitution Principle ensures that a superclass’s objects can be substituted with subclass objects without affecting program behavior.
10. What distinguishes Stack memory from Heap memory?
Ans:
Feature | Stack Memory | Heap Memory |
---|---|---|
Allocation | Automatically allocated and deallocated | Manually allocated and deallocated |
Size | Limited and fixed size | Larger and dynamically sized |
Access Speed | Faster access due to local storage | Slower access due to fragmentation |
Lifespan | Limited to the scope of the function | Persists until explicitly freed |
Data Structure | LIFO (Last In, First Out) | Can store any type of data structure |
11. How to reverse a linked list?
Ans:
Reversing a linked list means reversing the pointers’ direction between the nodes, moving the last node to the front. The operation has three references: ‘previous, current, and’ next. First, ‘previous’ is assigned ‘null, and ‘current’ refers to the head of the linked list. When iterating over the linked list, ‘next’ holds a pointer to the next node temporarily. The ‘current’s next’ reference is changed to ‘previous’, reversing the link. Then, the pointers are updated by replacing ‘previous’ with ‘current’ and ‘current’ with ‘next’.
12. What is the time complexity of Binary Search?
Ans:
- Binary search has a time complexity of O(log n). It is an efficient algorithm for finding an element in a sorted array or list.
- The idea of binary search is to split the interval being searched repeatedly. First, it compares the target value with the middle element of the sorted array.
- The search is complete when the target value is equal to the middle element. If the middle element is lesser, it searches in the array’s lower half; otherwise, it searches in the upper half.
- Therefore, the number of elements that would have to be searched greatly decreases with each iteration, and the performance is logarithmic.
13. What are the differences between a queue and a stack?
Ans:
- A queue and a stack are the same thing; they are abstract data types used to store collections of elements, with the difference being the way elements are added or removed.
- A stack adheres to the Last In, First Out (LIFO) principle, in which the last element added to the stack is the first to be removed.
- Operations on a stack include ‘push’ (to add an element) and ‘pop’ (to remove the top element).
- On the other hand, a queue is a data structure in the design to which the principle of FIFO (First In, First Out) applies. Thus, the first element added to the queue is also the first to be removed.
14. How would you determine if there is a cycle in a linked list?
Ans:
A cycle in a linked list can be detected using Floyd’s Cycle-Finding Algorithm, also commonly referred to as the Tortoise and Hare algorithm. This technique employs two pointers that move through the list at different speeds: a slow pointer or tortoise that moves one step at a time and a fast pointer or hare that moves two steps at a time. If the linked list contains a cycle, the fast pointer will eventually collide with the slow one; if it is a non-cyclic list, the fast one will ultimately reach the end.
15. How does a binary search tree compare to a binary heap?
Ans:
A binary search tree and a binary heap are both kinds of binary trees, but their purposes and properties differ. On the other hand, a binary tree is a tree in which a node has at most two children, with the left child having lower values and the right child having higher values than the parent. The binary search tree property is efficient in the search, insertions, and deletions of operations, which usually take O(log n) time.
16. Explain Hash Tables and how they Work.
Ans:
- Hash tables are basic data structures in which an associative array is implemented. This allows for fast data retrieval through key-value pairs.
- They employ a hash function that computes an index, or hash code, from the key to determine where the associated value is stored within the table.
- This enables average-case constant-time complexity (O(1)) for lookups, insertions, and deletions. Nonetheless, collisions in hash tables may happen in which two keys hash into the same index.
17. What is dynamic programming, and where is it commonly used?
Ans:
- Dynamic programming is an optimization technique that solves complicated problems by breaking them into simpler sub-problems and storing the results so they need not be recomputed in other program areas where the same sub-problems occur again.
- Dynamic programming is highly suitable for problems with overlapping subproblems and optimal substructure properties.
- Some common things computed using dynamic programming are Fibonacci numbers, solutions to Knapsack, and shortest paths from any given vertex to others in a graph; this is known as Dijkstra’s algorithm.
18. What is a balanced binary tree? How can balance be maintained?
Ans:
Balanced binary trees are binary trees where any node’s heights of its left and right subtrees can differ by no more than one, ensuring optimal search, insertion, and deletion operations. AVL trees and Red-Black trees are examples of balanced binary trees involving certain balancing criteria and rotations to maintain equilibrium after operations. For an AVL tree, the balance factor is declared to be greater than some threshold during insertion or deletion.
19. Describe the depth-first search algorithm procedure.
Ans:
A depth-first search (DFS) is a traversal or search algorithm for tree or graph data structures, exploring as much as possible along each branch before reconditioning in on itself. It can be done using recursion or an explicit stack. Then, the algorithm analyses all of its adjacent unvisited nodes iteratively. Then, if every adjacent node to a node has already been visited, it backs up and backtracks on that node, continuing the search until all accessible nodes have been explored at least once.
20. What is the most efficient way to find the shortest path in a graph?
Ans:
- The most efficient algorithm for finding the shortest path depends on the characteristics of the graph, such as whether it contains weighted edges.
- Dijkstra’s algorithm is the most widely used algorithm for finding the shortest path from a source to all other nodes in graphs with nonnegative weights. It picks the closest unvisited node iteratively and updates the distances.
- The most used algorithm for graphs with negative weights is the Bellman-Ford algorithm, which can handle negative weights and detect negative cycles.
21. What is normalization? Give an example.
Ans:
- Normalization is a database design process that organizes data to reduce redundancy and improve integrity. It breaks up a database into tables by referencing the relationship between two tables so that the data will be stored efficiently.
- The relations in the general process follow several normal forms, each with specific rules to eliminate various kinds of duplicate data and dependency.
- Suppose a customer database maintains information about the customers and details regarding their orders. Normalization removes all this information from the same table.
22. How do JOIN operations work in SQL?
Ans:
SQL JOIN operations combine records from two or more tables based on related columns, and this is how users can retrieve meaningful data from more than one source. The four most basic types of JOINs include INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL OUTER JOIN. INNER JOIN uses only those rows with corresponding values in the two tables. LEFT JOIN returns all the rows from the table on the left and the matched rows from the right table. It fills NULLs for non-matches.
23. What is a stored procedure?
Ans:
A stored procedure is a precompiled collection of SQL statements and optionally control-flow statements stored in a database; it lets users encapsulate complex logic and operations that can be executed as a single unit. Stored procedures improve code reusability, maintainability, and performance since the network traffic and execution time are decreased because the database engine will directly process it. They can be parameterized to take input dynamically and might be executed differently.
24. What is the difference between SQL and NoSQL databases?
Ans:
- Most importantly, they differ in their data models and design philosophies. A SQL database is relational; it uses structured query language (SQL) to manage and manipulate structured data organized into tables with specific schemas.
- SQL databases are largely characterized by their focus on using ACID properties to guarantee data integrity and consistency. NoSQL, on the other hand, is non-relational.
- NoSQL databases were designed to deal with unstructured or semi-structured data, which allows them flexibility and scalability. They are categorized as document-based, key-value stores, wide-column stores, and graph databases, and their usage is optimized for specific use cases.
25. How can a slow SQL query be optimized?
Ans:
- Various techniques, including improving performance and reducing execution time, can be used to optimize a slow SQL query. First, the execution plan of queries should be analyzed to identify bottlenecks and time-consuming operations.
- In this technique, several optimization techniques, like indexing, are used. This means the database can quickly locate the necessary data instead of scanning whole tables.
- Others are rewriting the query so that expensive joins are not called, using COUNT instead of COUNT, and reducing the number of rows returned by using LIMIT and WHERE clauses.
26. What is indexing, and how does it improve query performance?
Ans:
Indexing is an optimization technique used in a database. An index accelerates the retrieval of rows from a table by creating a data structure called an index. Much like the table of contents for a book, an index allows the database to find the data without having to scan every row. A database can quickly access the necessary data when it has indexes on columns that frequently appear in search queries, join conditions, or sorting operations.
27. What are the ACID properties of a database? Explain.
Ans:
ACID properties refer to rules that ensure the reliable processing of database transactions. The properties are Atomicity, Consistency, Isolation, and Durability. It provides that all the transaction operations are either performed or not performed at all, and there will be no partial updates with a chance of causing data inconsistency. Consistency ensures that a transaction moves the database from one valid state to another in which all defined rules and constraints are satisfied.
28. What are the differences between DELETE and TRUNCATE commands?
Ans:
- The DELETE and TRUNCATE commands both remove records from a database. However, they operate differently on each command. DELETE deletes rows from the table based on some specified condition determined by a WHERE clause.
- If the DELETE command is wrapped in a transaction, it can be rolled back, allowing users to recover deleted data in case they need it.
- On the other hand, TRUNCATE completely delete all rows in a table without having any conditions and cannot be rolled back, so it is a quicker operation, since it doesn’t log individual row deletion.
29. What is sharding in NoSQL databases?
Ans:
- Sharding is a database architecture pattern in which data is distributed across multiple servers or nodes in NoSQL databases to improve performance and scalability.
- Every shard of the data contains a subset of the actual data, making it ideal for parallel query processing and reducing the load on a single server.
- It allows excellent distribution of large volumes of datasets and high-traffic applications since every shard can be accessed independently.
- Sharding also increases the level of fault tolerance since one failing shard has no effect on others. It also calls for considering data-distribution strategies to achieve optimal performance across the system.
30. What are database transactions, and how are they managed?
Ans:
Database transactions are sequences of operations performed as a single logical unit of work, such that those operations can be either entirely completed or leave no effects in case those operations cannot be completed. Transactions may often entail read and write operations on the database. Transaction control is necessary for maintaining the proper state of the database. It prescribes how a database transaction should be defined regarding its boundaries, such as when it commences, commits, or rolls back.
31. What are RESTful web services?
Ans:
RESTful web services are a type of web service that follows the principles of Representational State Transfer (REST), an architectural style for designing networked applications. The services use standard HTTP methods like GET, POST, PUT, and DELETE for creating actions on resources described through URLs. Web services referred to as RESTful are stateless. Meaning that each request coming from the client contains all the information the server needs to provide that request in such a way that no server-side session state is kept.
32. Define how HTTP methods like GET, POST, PUT, and DELETE work.
Ans:
- HTTP methods define the actions which may be performed on the resources in RESTful web services.
- The GET retrieves resources held on a server. The client could use the GET to request and receive a representation of a resource and not expect anything on its part to change.
- The POST is used when data is submitted to the server to create new resources, in which case the server may create a new resource.
- The PUT updates an existing resource, in which case it sends the entire resource representation to the server and replaces the data currently held there.
33. What is AJAX in web development?
Ans:
- AJAX stands for Asynchronous JavaScript and XML, and it refers to a way of developing web applications that employ dynamic, interactive web pages.
- AJAX enables web pages to exchange some data with the server asynchronously. It assists in partial updates of pages without loading the entire page.
- This results in an efficient user experience because users can interact with the application as they want while background processing is underway.
- AJAX typically uses JavaScript for asynchronous HTTP requests and can support a wide variety of formatted data, including XML, JSON, or HTML.
34. Which security elements should every web application always employ?
Ans:
Web application security is an absolute must because data is sensitive, and user confidence is highly dependent on it. Input validation is one of the most important security practices that prevent SQL injection and cross-site scripting attacks because user input undergoes proper sanitizing. Proper authentication and authorization practices, including strong password policies and proper role-based access control, ensure safe user accounts.
35. What is the Document Object Model (DOM)?
Ans:
A Document Object Model, or DOM, is a programming interface that reflects the structure of an HTML or XML document as a tree of objects, thereby allowing scripts to access and manipulate the content, structure, or style of the document. A node in such a tree structure represents an attribute and piece of text within the document. The DOM has made dynamic interaction with web pages possible, and developers can create, update or delete elements or respond to any user events in real-time.
36. Explain Cross-Origin Resource Sharing (CORS).
Ans:
Cross-Origin Resource Sharing (CORS) is the browser’s security-related feature that controls web applications’ capability to make requests on servers other than their domain. As mentioned earlier, by default, web browsers enforce the same-origin policy, that is, prevent scripts from making cross-origin requests and access resources from one origin to another to reduce exposure to security risks. CORS allows a server to explicitly grant or deny certain origins the right to access those resources via HTTP.
37. What are the differences between a session and a cookie?
Ans:
- Cookies and sessions are two client-side storage tools, but they store data for different purposes and are characterized differently.
- A session is one of the server-side storage mechanisms used by web applications to keep data associated with a user over several requests.
- The client usually identifies it using a unique session ID stored in a cookie. Sessions can hold much bigger chunks of data and expire after a certain period or when the user logs out. Conversely, cookies are small pieces of data held directly on the client’s browser.
38. How can the loading time of web pages be improved?
Ans:
- Optimizing web page loading times encompasses several methods for optimizing performance and enhancing user experience.
- Initial strategies include reducing HTTP requests by joining multiple files, such as CSS and JavaScript, so the browser needs to load fewer resources.
- Compressing files like Gzip reduces their size, meaning people can download the file much faster. Browsers cache static resources locally so that subsequent visits to the site do not have to re-fetch them.
- Finally, optimizing images using formats such as WebP and applying lazy loading can make the page load much faster.
39. What is a Content Delivery Network (CDN)?
Ans:
A Content Delivery Network is a distributed network of servers placed strategically across various geographic locations to deliver web content more efficiently to the user. By caching copies of static resources such as image stylesheets and scripts, CDNs reduce the physical distance between the user and the server, reducing latency and improving loading times. Whenever a user requests the content, a CDN deflects the request to the nearest server for faster data delivery.
40. Explain client-side and server-side rendering.
Ans:
Client-side rendering (CSR) and server-side rendering (SSR) are two techniques used in rendering web pages, with their pros and cons and respective applications. CSR downloads a minimal HTML document and then uses JavaScript to generate the page content dynamically on the client side. This approach makes navigation between pages faster after the initial load due to fetching only the required data, which may reduce user interaction. However, the initial loads are going to be slower.
41. What are some benefits of cloud computing for organizations?
Ans:
- Cloud computing offers cost-effectiveness, flexibility, and scalability to business organizations. By using cloud services, companies reduce their need for on-premises hardware, lowering capital expenditures and maintenance costs.
- Since the organization can scale down or up to meet demand, its response to changing its business’s market position will be quicker and ensure the timely utilization of resources.
- Cloud computing enhances collaboration by making applications and data accessible from anywhere through remote access, engaging an agile workforce.
42. Describe the different types of cloud services (IaaS, PaaS, SaaS).
Ans:
- The three significant models of cloud services are usually divided into Infrastructure as a Service (IaaS), Platform as a Service (PaaS), and Software as a Service (SaaS).
- IaaS would offer virtualized computing resources over a private network, so firms do not need to buy, manage, or maintain physical or virtualized hardware to support applications.
- PaaS gives developers a platform to develop, deploy, and manage their applications without any major infrastructure overhang in the development process.
43. What key security considerations exist in cloud environments?
Ans:
Data protection, access control, compliance, and incident response are important security considerations in cloud environments. Data encryption in transit and at rest are key aspects to protect against unauthorized access and breaches of confidentiality. Good IAM practices help regulate who can access cloud resources and what actions they may perform. Organizations should be aware of regulations binding them to their respective industries. Cloud services should ensure that all services employed support set standards and requirements.
44. What are the differences between vertical and horizontal scaling in the cloud?
Ans:
Scaling up” or vertical scaling, increases resources such as CPU, RAM, or storage on an existing server to raise the workload level. It is fairly simple but comes with caps on the maximum number of resources and might require some downtime to upgrade. “Scaling out” or horizontal scaling adds more servers or instances to distribute the load across many machines. This method is flexible and redundant because more traffic will be handled without one point of failure.
45. What is containerization, and how do Docker and Kubernetes work?
Ans:
- Containerization is a software technology that enables applications and their dependencies to run encapsulated in isolated containers, ensuring consistency across different environments.
- Docker is a popular platform on which developers can easily create, deploy, and manage containers. It offers an integrated platform for developing applications without worrying about underlying infrastructural differences.
- Kubernetes is an orchestration tool for automating the deployment, scaling, and management of containerized applications across clusters of machines.
46. What is the microservices architecture?
Ans:
- Microservices architecture is an approach to application development in which an application is designed as a system of loosely coupled services. In this architecture, each service is responsible for a business function.
- This architecture boosts modularity, allowing teams to develop, deploy, and scale individual services independently. Most importantly, each microservice will communicate with other microservices via APIs, but never in a manner that blurs concerns.
- The design also facilitates flexibility regarding individual service changes, as changes will not affect the whole application.
47. How does a serverless architecture operate?
Ans:
Serverless architecture is the design and development of applications in such a manner that they run without the developer caring about Infrastructure, so the developers may solely focus on their code and deliver features in the application. In this model, application code is executed in response to events, such as HTTP requests or database changes. All provisioning and scaling are taken care of automatically by the cloud providers. The cost and time consumed are saved as the developers pay only for the compute time consumed during execution.
48. What is a key feature of AWS/Azure/Google Cloud?
Ans:
Amazon Web Services (AWS), Azure, and Google Cloud provide several features that meet various business needs. In this regard, it is what makes the offering of AWS stand out is an extensive list of services, from compute-EC2-to storage-S3-and further on to database services. These services make the environment highly scalable and flexible. Microsoft Azure also leads the competition by integrating other products and services from Microsoft.
49. How do applications migrate to the cloud?
Ans:
- Application migration to the cloud involves various steps to help it happen smoothly. First, a comprehensive analysis of the existing applications and dependencies is needed to determine which workloads can be migrated.
- The migration strategy can be either lift-and-shift (rehosting), refactoring (modifying for cloud optimization), or rearchitecting (redesigning an application for cloud-native benefits).
- A good plan should also entail data migration. This should be done so that data integrity is not lost and that minimum downtime is experienced during the transition.
50. Define load balancing in the context of cloud computing.
Ans:
- Load balancing is the term for the process of distributing incoming network traffic or workloads across multiple servers or resources to achieve optimum performance and availability in cloud computing.
- The load balancers will be efficient in regulating traffic so that no specific server becomes saturated, which could cause poor performance or even downtime.
- Cloud load balancers are essential because they can automatically respond to a shift in traffic patterns; they can start scaling up their resources to cater to changing workloads.
51. What management strategies were used in a difficult project?
Ans:
A challenging project was migrating large-scale legacy applications to cloud-based architecture. The main management practices utilized include planning extensively and also engaging stakeholders on their needs, and handling any issues that may come along. Agile methodologies have been applied to allow opportunities for iterative development and room to change the requirements. There are frequent check-ins and progress presentations in transparency so that people within the team can be engaged in working on the project.
52. What is the prioritization strategy when working on tight deadlines?
Ans:
Effective prioritization strategies are key to well-timed management of our tasks when dealing with tight deadlines. The Eisenhower Matrix would be a tool known for categorizing the functions based on two variables, urgency and importance, so end up working on what matters first. Also break down bigger tasks into smaller ones to help the track completion better and speed up in making progress. Time blocking techniques enable dedicated focus on high-priority tasks while creating minimum distraction.
53. How can teamwork be used for a goal?
Ans:
- Teamwork can achieve a goal through team collaboration, using a diverse set of skills, and enhancing one’s problem-solving capabilities.
- Open communication with team members encourages the flow of ideas and the reception of feedback, which might even lead to more innovative solutions.
- The definition of roles and responsibilities thus ensures a clear understanding of each person’s contribution to the team’s objectives.
- Collaborative tools like project management software streamline coordination and keep people aligned with progress.
54. Which response is provided when constructive criticism is given?
Ans:
- Receiving constructive criticism requires an open mind and taking the feedback as a way to better oneself. Being more open to criticism and not becoming defensive is essential to understanding one’s viewpoint in giving criticism.
- Asking a clarifying question will provide deeper insight into the suggestions made by showing a willingness to improve. Based on feedback, changing as suggested might enhance our skills and performance.
- A word of gratitude for the feedback establishes a sound relationship and opens channels of continuous communication for further growth.
55. Describe an experience that called for initiative.
Ans:
An experience that called for initiative was during a project when, a critical team member fell ill unexpectedly,l, which resulted in delaying the project. Knowing this, began to scrutinize the remaining pieces left to do and where I could intervene to fill the gaps. Aligned with fellow team members and scheduled regular checks to ensure all was well and on course, further staying on top of roadblocks that came through. Not communicating proactively about the situation with stakeholders helped to set up managing expectations and trust.
56. Describe how disagreements between fellow employees are resolved.
Ans:
Open and collaborative problem-solving resolves disagreements between fellow employees. If a discussion is initiated in a neutral setting, each party can voice viewpoints and concerns without interruptions. Active listening is pertinent because it incorporates the real causes of the disagreement and locates commonalities. Running a brainstorming session to identify solutions encourages teamwork and creativity toward agreeing.
57. How does one stay updated on the latest technology trends?
Ans:
- Staying updated on the latest technology trends involves continuous learning and engagement with the broader industry communities. To this end, subscribing to
- Newsletters, blogs, and podcasts track new technologies, insights, and developments. Conferences, webinars, and workshops present an opportunity to network and learn from industry leaders firsthand.
- Online forums and social media groups create a platform for like-minded people to discuss and collaborate on sharing their know-how. Lastly, relevant certifications and other training courses keep one abreast of the latest technologies.
58. How changes to the project scope that were unexpected are managed?
Ans:
- Change Management requires structured execution to determine the impact of such changes and make necessary adjustments to project change management.
- The nature of the change would first be assessed, followed by its implications for the scope, time, or resources that may be affected. It is also important to seek the opinion of relevant stakeholders so that realignment can occur and address the already existing concerns.
- If feasible, reprioritizing tasks and refreshing the project plan will ensure these changes are assimilated in a non-disruptive way.
59. How are stress and pressure managed in the workplace?
Ans:
Managing stress and pressure in the workplace involves proactive strategies that promote well-being and productivity in workers. Techniques such as time management can relieve stress, including prioritizing tasks and realistic deadlines. The ability to break up the routine with frequent breaks and some physical activity can contribute to success in reducing stress and mental clarity. This may involve practising mindfulness through deep breathing or another method.
60. What tools or techniques improve personal productivity?
Ans:
There are a myriad of tools and techniques that empower personal productivity depending on the individual work style. These include, for example, task management tools, such as Trello or Asana, by which one can organize and prioritize tasks so as not to let anything fall through the cracks. Time management techniques continue sustaining long periods of concentration with short breaks to avoid burnout. Thirdly, using calendar applications for activity scheduling and blocking dedicated time for deep work keeps things structured.
61. Which strategies work for managing teamwork conflicts?
Ans:
- Maintaining open communication, motivating team members to express their views, and actively listening to all sides of the opinion are effective ways to manage team conflicts.
- A neutral facilitator may moderate the discussion to identify common objectives. Clear team norms and guidelines are set to prevent conflict escalation.
- Moreover, concentrating on collaborative problem-solving techniques can transform disagreements into constructive outcomes.
62. What are the successful steps in leading a project?
Ans:
- In leading a project to successful completion, the following must be considered: first, define clear goals and objectives so that all people who will be assigned to it are on the same page.
- Develop a comprehensive project plan that accounts for both tasks to be performed, as well as timeline and resource allocation. Assemble a competent team.
- Train/coordinate stakeholders and effectively delegate responsibilities to create an environment that encourages collaboration. Track progress and adjust plans if necessary while maintaining communication with team members and all stakeholders.
63. How can the team’s motivation be enhanced to meet its targets?
Ans:
The team’s motivation to meet targets can be enhanced through recognition and rewards for achievements, providing opportunities for professional growth, and a positive team culture. A sense of accomplishment can be achieved by setting clear, attainable goals and celebrating achievements. Empowerment of individual team members through involvement in decision-making and encouragement of autonomy increases members’ value and ownership sentiment in the project’s success.
64. Which management style fits best with different team dynamics types?
Ans:
Each management style is required for different team dynamics. With highly experienced and independent teams, for example, a change leader or laissez-faire leader would encourage creativity and independence. A directive or autocratic management style may be needed for teams with more structure or extremely tight deadlines. Situational leadership then allows managers to determine their approach based on the team’s maturity and the specific situation needs.
65. What should the project learn from those that did not go as planned?
Ans:
- There are lessons learned in projects that go differently than planned, particularly in planning, risk management, and communication.
- The cause of failure could be broken down for lessons to be learned on why the scope is not as it ought to be, why the time allocated is unrealistic, or why the stakeholders were not done enough.
- These lessons need documentation for information on other projects for future direction and to avoid making similar mistakes.
- Moreover, creating an environment that celebrates learning from mistakes should result in more resilient teams. Flexibility and continuous improvement are key to long-term success.
66. What methods are used to offer feedback to team members?
Ans:
- Team members receive informal feedback through formal performance reviews, one-on-one sessions, and ad-hoc casual meetings. Constructive feedback focuses on the specifics of behaviours and outcomes rather than personality traits.
- Using the ‘sandwich method,’ which commences by discussing some positive aspects, then targets areas where improvements are needed, and concludes by having discourses that encourage, makes it effective.
- Open dialogue encouragement opens avenues for individual team members to ask questions and clear expectations but creates a culture of continuous feedback and improvement.
67. What are the best solutions for poor-performing team members?
Ans:
In situations where team members are not performing well, managing this situation would call for ascertaining the causes of such performance through open communication. Well-defined and correct performance expectations and goals can form a platform for improvement. Periodic scheduled check-in times can provide corrective guidance, support, and constructive feedback to get them back on track. The ability to access training or resources can also help build related skills.
68. How to balance long-term objectives with short-term tasks?
Ans:
Fine balancing between long-term and short-term tasks is only possible when appropriately prioritized and planned. Divide long-term goals into small goals, which, in some measure, keep up with short-term work and allow gradual, incremental accomplishment. Review a priority regularly, adapt to new situations, and focus on the long term. Time management skills like the Eisenhower Matrix can help managers distinguish between urgent and critical tasks.
69. What metrics are used to measure team performance?
Ans:
- The metrics that can be used to measure team performance are such that there are quantifiable measures, including productivity rates, project completion times, and quality of deliverables.
- Some of the qualitative metrics include team engagement through surveys and peer feedback on team dynamics and collaboration.
- Monitoring KPIs aligned with project goals will also help judge their success. Moreover, tracking the number of people who attend and participate in team meetings will indicate commitment levels.
70. How does an organization or team set and communicate project timelines?
Ans:
- Project timelines are developed by carefully planning and estimating task durations, resource availability, and dependencies. Project management tools facilitate the visualization of timelines and Gantt charts while helping to understand and clarify them.
- Once a timeline is developed, distribute it to all the team members and familiarize them with the roles of their mates and the deadlines.
- To sustain alignment and accountability, all project members must be informed about possible changes and updates at any stage of the project lifecycle.
71. What is the importance of technology consulting in driving business transformation?
Ans:
Technology consulting acts to align technology solutions with organizational goals and strategies. The consultant measures current systems, determines points that need to be improved and suggests new technologies that help increase operative efficiency and innovation. The consultant guides a business through the adaptation of digital solutions in such a way that changes are executed transparently and effectively.
72. How does the software solution align with business needs?
Ans:
To align software solutions with business needs, a proper analysis of the organization’s requirements, goals, and processes in the current organization needs to be done. This requires stakeholder input to know what some of the problems and their objectives are. According to this analysis, consultants can compare alternative software choices with different criteria about their ability to scale, being friendly to the users, and integration of both in-house and third-party ones.
73. What is digital transformation, and why is it important for organizations?
Ans:
- Digital transformation involves embedding digital technologies into every aspect of business and fundamentally changing the way in which value is delivered to customers. This includes new technologies, rethinking one’s business model, and enhancing customer experience.
- Digital transformation is an important factor in enhancing efficiency, encouraging innovation, and maintaining competitiveness in a rapidly changing digital landscape.
- Organizations embracing digital transformation will be best positioned to respond rapidly to market changes; they can improve the agility of their operations and respond faster to their customers’ needs.
74. How do IT strategies align with more general business objectives?
Ans:
That involves prioritizing the business and determining how technology may be leveraged to fuel growth, efficiency, and innovation. A detailed IT roadmap outlining specific projects and initiatives is developed, which helps ensure resources and investments are aligned with business needs. Communication and feedback loops between IT and business units ensure that technology is responsive to changing organizational objectives and market dynamics.
75. How can the return on investment for a software solution be determined?
Ans:
Determining the ROI of a software solution involves setting the financial benefits accruable from the solution against its complete costs within the implementation, licensing, maintenance, and training. KPIs like increasing productivity, decreasing operational costs, and creating more revenue provide the quantitative yardsticks against which to evaluate. Time can then be calculated based on the cost-benefit analysis to check the solution’s effectiveness.
76. What are the benefits of big data analytics for businesses?
Ans:
- Big data analytics allows businesses to avail a wide range of benefits, including increasing the efficiency with which better decisions are made, improving business operations, and providing more personalized customer experiences.
- It goes without saying that organizations can extract insights and trends from this enormous volume of data to feed strategic decisions and innovation.
- Big data analytics also allows for predictive modelling, which enables businesses to predict customer behaviour and market trends.
77. What common problems occur when implementing enterprise software solutions?
Ans:
- The most common issues in implementing enterprise software solutions include employees’ resistance to change, the problem of integrating data, and failure to prepare for the training in advance.
- Organizations face new systems that are difficult to align with existing processes, causing significant disruption to workflows. Integrating data quality across the systems is another challenge.
- Even in time and budget, resource constraints can hinder change management.
- Effective strategies, planning, and support at every stage will guard against many challenges that crop up in implementation. All these aspects are crucial for the successful adoption of enterprise software.
78. How is change management executed during a project deployment?
Ans:
Change management in project deployment is preparing, supporting, and equipping people to cope with new processes and systems. This would involve impact assessments that indicate changes as likely to affect stakeholders, which must be communicated. Training and support resources are also key issues during this transition process. Facilitating the transition process by engaging stakeholders would mean that concerns could be listened to and buy in facilitated.
79. What are the key ingredients of smooth client project communication?
Ans:
Clear communication channels, set expectations from updates, and active listening to the client’s feedback are key factors that ensure smooth client communication. Regular meetings and status reports keep all the stakeholders well-informed about progress and issues. The collaboration can easily be established through real-time communication and document sharing for transparency. Building relationships and trust with clients is vital because it provides open communication channels, which promotes cooperation.
80. How are technology solutions built to be future-proof for business?
Ans:
- Technology solutions are built to be future-proof through scalability, flexibility, and interoperability.
- Solutions should be based on open standards and modular architectures, making it thus easy to interface with new technologies that surface.
- Updates and support from vendors enhance solutions over time in terms of relevance and security. Further, mechanisms for incorporating feedback enable businesses to adapt their technology solutions to meet their changing needs and market conditions.
- If an organization considers all these elements, it will be able to invest in technology that will foster growth and innovation in the long term.
81. What are the core services and market positioning for Capgemini?
Ans:
- Capgemini is a global consulting, technology services, and digital transformation firm. Its solutions span entire industries. Its core services include strategy and transformation, application services, technology and engineering services, and managed services.
- Capgemini emphasizes cloud services, data and analytics, and digital customer experience solutions. Its robust focus on innovation and collaboration serves as the foundation for its market positioning, leveraging partnerships with best-in-class technology leaders.
82. How can Capgemini support clients with their digital transformation endeavours?
Ans:
Capgemini helps clients on their path to digital transformation by providing an integrated solution that covers strategy, technology, and change management. Capgemini assesses clients’ capabilities and shortcomings. The company then works with clients to create individualized transformation roadmaps, integrating modern technologies like cloud computing, artificial intelligence, and data analytics. It also offers change management capabilities to employees during the onboarding process of newer processes and technologies, making the transition easier.
83. What are the main reasons that make Capgemini a strong consulting partner?
Ans:
Several main reasons make Capgemini a strong consulting partner. First, its decades-long reputation speaks for reliability and expertise in the field. Capgemini’s global reach benefits multinational clients that can be serviced locally with deep knowledge and insights. Third, the company thrives on innovation and provides innovative solutions through constant updates in emerging technologies.
84. How do technological solutions from Capgemini support businesses in staying competitive?
Ans:
- Capgemini supports businesses to stay competitive through innovative technological solutions that enhance efficiency and agility.
- By assessing clients’ existing processes and identifying those that can be automated and improved, Capgemini uses leading-edge technologies like AI, machine learning, and cloud computing.
- These solutions enable businesses to optimize operations, cut costs, and enhance customer experiences.
- In addition, it also allows organizations to make the best decisions with clear insights from data analytics. Thus, it enables an organization to make decisions on time amidst rapid fluxes in the market.
85. What is the employee development and training plan of Capgemini?
Ans:
- Capgemini prioritizes employee development and training through a number of initiatives that seek to enhance career advancement and skilling.
- The company provides broad training and development programs ranging from technical workshops and leadership courses to mentorship opportunities.
- Capgemini also emphasizes continuous learning, which implies that employees are motivated to become certified in the latest technologies and methods through their respective roles.
86. What distinguishes Capgemini’s service approach to sustainability and innovation?
Ans:
At Capgemini, sustainability is incorporated into its business model and service offerings by embedding sustainable practices into every part of the business. The company has always focused on the environment to conserve it and manage all its resources responsibly. Through innovative technologies, Capgemini empowers clients with the efficiencies to build sustainable strategies with less waste.
87. What will automation and AI’s role in future services Capgemini offers?
Ans:
Automation and AI will be the significant drivers of the service offerings of Capgemini’s future, adding more efficiency and allowing customers to make better decisions. Capgemini will integrate AI-driven tools into its automation technologies to keep operations lean and relatively free from manual processes to improve service delivery. Incorporating these technologies will enable it to provide smarter solutions, such as predictive analytics towards better forecasting and personal interactions, leading to better customer experiences.
88. How are international clients and global projects managed effectively?
Ans:
- Capgemini will address international clients and global projects through a well-planned approach that brings cooperation, communication, and domestic expertise.
- It creates global dedicated teams whose members are from all regions, offering varied perceptions and understanding of local markets.
- The company applies project management methodologies, such as Agile, to allow responsiveness and adaptability to client-changing needs.
- Last but not least, the company also uses advanced collaboration tools to enhance interaction among its team members across time zones.
89. How does the organization measure client satisfaction?
Ans:
Capgemini utilizes several strategies for client satisfaction. The firm has focused primarily on relationship-building through quality services. The company emphasizes understanding the client’s needs through comprehensive assessments and the involvement of various stakeholders. In this respect, solutions are tailored to deal with particular issues for a specific client. Regular interaction and openness in all project life cycles will manage expectations and establish trust.
90. What trends define the future of consulting in the technology sector?
Ans:
Some of the various trends that define the future of consulting in the technology sector echo the changing business environment and the advancement of technology. One of the important trends in this process is the development of consulting with more focus on digital transformation, where an organization recognizes that harnessing technology can create operational efficiencies and meaningful customer engagement.