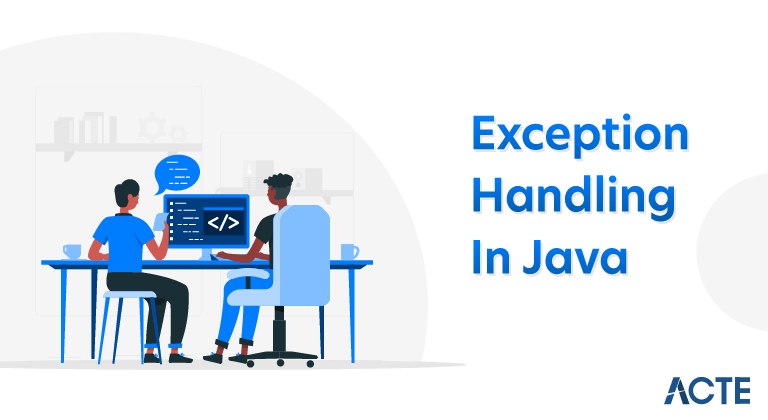
Exception Handling in Java: A Complete Guide with Best Practices
Last updated on 07th Jun 2020, Blog, General
Exception handling is one of the most important feature of java programming that allows us to handle the runtime errors caused by exceptions. In this guide, we will learn what is an exception, types of it, exception classes and how to handle exceptions in java with examples.
An Exception is an unwanted event that interrupts the normal flow of the program. When an exception occurs program execution gets terminated. In such cases we get a system generated error message. The good thing about exceptions is that they can be handled in Java. By handling the exceptions we can provide a meaningful message to the user about the issue rather than a system generated message, which may not be understandable to a user.
Exception Handling in Java
- Exception Handling
- Advantage of Exception Handling
- Hierarchy of Exception classes
- Types of Exception
- Exception Example
- Scenarios where an exception may occur
The Exception Handling in Java is one of the powerful mechanisms to handle the runtime errors so that normal flow of the application can be maintained.
In this page, we will learn about Java exceptions, its type and the difference between checked and unchecked exceptions.
What is Exception in Java
Dictionary Meaning: Exception is an abnormal condition.
In Java, an exception is an event that disrupts the normal flow of the program. It is an object which is thrown at runtime.
Types of exceptions
There are two types of exceptions in Java:
1)Checked exceptions
2)Unchecked exceptions
Checked exceptions
All exceptions other than Runtime Exceptions are known as Checked exceptions as the compiler checks them during compilation to see whether the programmer has handled them or not. If these exceptions are not handled/declared in the program, you will get compilation error. For example, SQLException, IOException, ClassNotFoundException etc.
Unchecked Exceptions
Runtime Exceptions are also known as Unchecked Exceptions. These exceptions are not checked at compile-time so compiler does not check whether the programmer has handled them or not but it’s the responsibility of the programmer to handle these exceptions and provide a safe exit. For example, ArithmeticException, NullPointerException, ArrayIndexOutOfBoundsException etc.
Advantage of Exception Handling
The core advantage of exception handling is to maintain the normal flow of the application. An exception normally disrupts the normal flow of the application that is why we use exception handling. Let’s take a scenario:
- statement 1;
- statement 2;
- statement 3;
- statement 4;
- statement 5;//exception occurs
- statement 6;
- statement 7;
- statement 8;
- statement 9;
- statement 10;
Suppose there are 10 statements in your program and there occurs an exception at statement 5, the rest of the code will not be executed i.e. statement 6 to 10 will not be executed. If we perform exception handling, the rest of the statement will be executed. That is why we use exception handling in Java.
Do You Know?
- What is the difference between checked and unchecked exceptions?
- What happens behind the code int data=50/0;?
- Why use multiple catch blocks?
- Is there any possibility when finally the block is not executed?
- What is exception propagation?
- What is the difference between throw and throw keyword?
- What are the 4 rules for using exception handling with method overriding?
Hierarchy of Java Exception classes
The java.lang.Throwable class is the root class of Java Exception hierarchy which is inherited by two subclasses: Exception and Error. A hierarchy of Java Exception classes are given below:
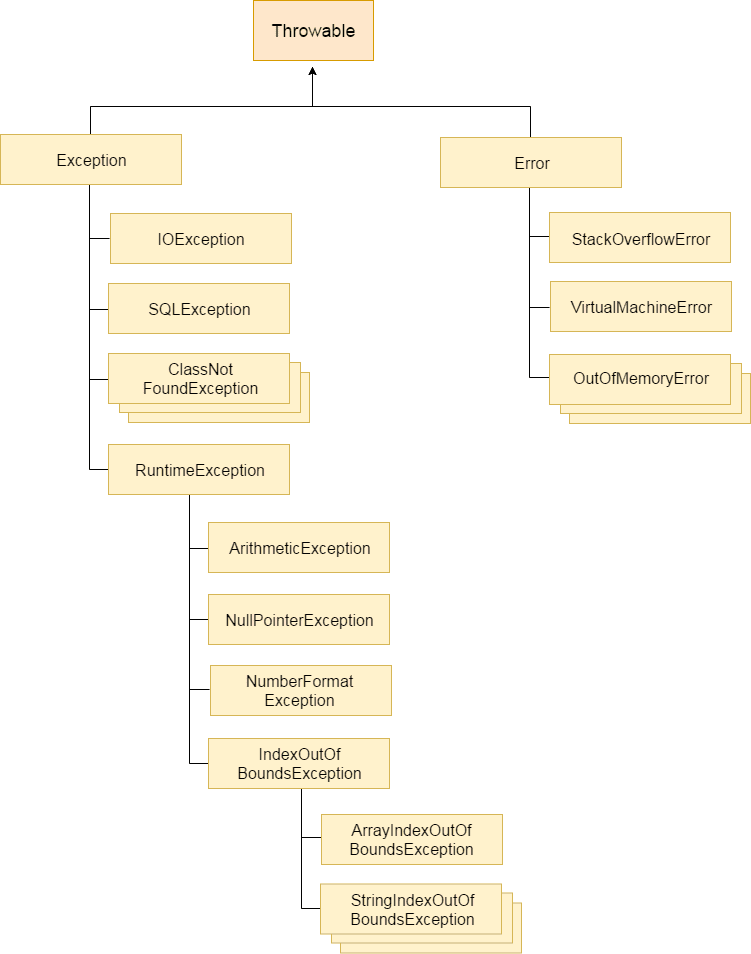
Types of Java Exceptions
There are mainly two types of exceptions: checked and unchecked. Here, an error is considered as the unchecked exception. According to Oracle, there are three types of exceptions:
- Checked Exception
- Unchecked Exception
- Error
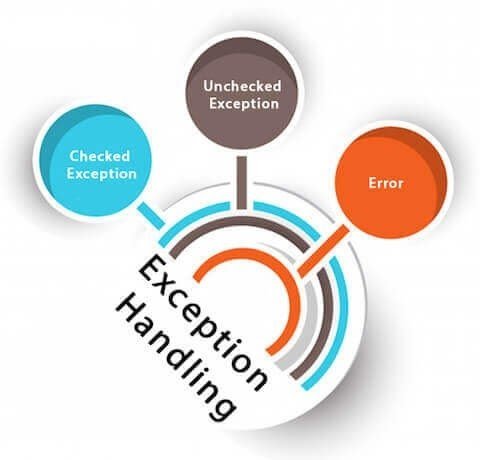
Difference between Checked and Unchecked Exceptions
1) Checked Exception
The classes which directly inherit Throwable classes except RuntimeException and Error are known as checked exceptions e.g. IOException, SQLException etc. Checked exceptions are checked at compile-time.
2) Unchecked Exception
The classes which inherit RuntimeException are known as unchecked exceptions e.g. ArithmeticException, NullPointerException, ArrayIndexOutOfBoundsException etc. Unchecked exceptions are not checked at compile-time, but they are checked at runtime.
3) Error
Error is irrecoverable e.g. OutOfMemoryError, VirtualMachineError, AssertionError etc.
Java Exception Hierarchy
As stated earlier, when any exception is raised an exception object is getting created. Java Exceptions are hierarchical and inheritance is used to categorize different types of exceptions. Throwable is the parent class of Java Exceptions Hierarchy and it has two child objects – Error and Exception. Exceptions are further divided into checked exceptions and runtime exception.
- Errors: Errors are exceptional scenarios that are out of scope of application and it’s not possible to anticipate and recover from them, for example hardware failure, JVM crash or out of memory error. That’s why we have a separate hierarchy of errors and we should not try to handle these situations. Some of the common Errors are OutOfMemoryError and StackOverflowError.
- Checked Exceptions: Checked Exceptions are exceptional scenarios that we can anticipate in a program and try to recover from it, for example FileNotFoundException. We should catch this exception and provide useful message to user and log it properly for debugging purpose. Exception is the parent class of all Checked Exceptions and if we are throwing a checked exception, we must catch it in the same method or we have to propagate it to the caller using throws keyword.
- Runtime Exception: Runtime Exceptions are cause by bad programming, for example trying to retrieve an element from the Array. We should check the length of array first before trying to retrieve the element otherwise it might throw ArrayIndexOutOfBoundException at runtime. RuntimeException is the parent class of all runtime exceptions. If we are throwing any runtime exception in a method, it’s not required to specify them in the method signature throws clause. Runtime exceptions can be avoided with better programming.
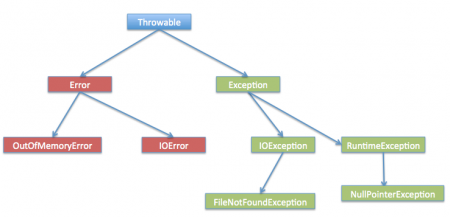
Exception Handling in Java – Useful Methods
Java Exception and all of it’s subclasses doesn’t provide any specific methods and all of the methods are defined in the base class Throwable. The exception classes are created to specify different kind of exception scenarios so that we can easily identify the root cause and handle the exception according to it’s type. Throwable class implements Serializable interface for interoperability.
Some of the useful methods of Throwable class are;
- public String getMessage() – This method returns the message String of Throwable and the message can be provided while creating the exception through it’s constructor.
- public String getLocalizedMessage() – This method is provided so that subclasses can override it to provide locale specific message to the calling program. Throwable class implementation of this method simply use getMessage() method to return the exception message.
- public synchronized Throwable getCause() – This method returns the cause of the exception or null id the cause is unknown.
- public String toString() – This method returns the information about Throwable in String format, the returned String contains the name of Throwable class and localized message.
- public void printStackTrace() – This method prints the stack trace information to the standard error stream, this method is overloaded and we can pass PrintStream or PrintWriter as argument to write the stack trace information to the file or stream.
Java Exception Keywords
There are 5 keywords which are used in handling exceptions in Java.
False | await |
---|---|
try | The “try” keyword is used to specify a block where we should place exception code. The try block must be followed by either catch or finally. It means, we can’t use try blocks alone. |
catch | The “catch” block is used to handle the exception. It must be preceded by try block which means we can’t use catch block alone. It can be followed by finally block later. |
finally | The “finally” block is used to execute the important code of the program. It is executed whether an exception is handled or not. |
throw | The “throw” keyword is used to throw an exception |
throws | The “throws” keyword is used to declare exceptions. It doesn’t throw an exception. It specifies that there may occur an exception in the method. It is always used with method signatures. |
Java Exception Handling Example
Let’s see an example of Java Exception Handling where we use a try-catch statement to handle the exception.
- public class JavaExceptionExample{
- public static void main(String args[]){
- try{
- //code that may raise exception
- int data=100/0;
- }catch(ArithmeticException e){System.out.println(e);}
- //rest code of the program
- System.out.println(“rest of the code…”);
- }
- }
Output:
Exception in thread main java.lang.ArithmeticException:/ by zero
rest of the code…
In the above example, 100/0 raises an ArithmeticException which is handled by a try-catch block.
Common Scenarios of Java Exceptions
There are given some scenarios where unchecked exceptions may occur. They are as follows:
1) A scenario where ArithmeticException occurs
If we divide any number by zero, there occurs an ArithmeticException.
- int a 50/0;// ArithmeticException
2) A scenario where NullPointerException occurs
If we have a null value in any variable, performing any operation on the variable throws a NullPointerException.
- string s= null;
- System.out.println(s.length());//NullPointerException
3) A scenario where NumberFormatException occurs
The wrong formatting of any value may occur NumberFormatException. Suppose I have a string variable that has characters, converting this variable into digits will occur NumberFormatException.
- String S=”abc”;
- int i=integer.parseint(s);//NumberFormatException
4) A scenario where ArrayIndexOutOfBoundsException occurs
If you are inserting any value in the wrong index, it would result in ArrayIndexOutOfBoundsException as shown below:
- int a[]=new int[5];
- a[10]=50;//ArrayIndexOutOfBoundsException
Java Exceptions Index
- Java Try-Catch Block
- Java Multiple Catch Block
- Java Nested Try
- Java Finally Block
- Java Throw Keyword
- Java Exception Propagation
- Java Throws Keyword
- Java Throw vs Throws
- Java Final vs Finally vs Finalize
- Java Exception Handling with Method Overriding
- Java Custom Exceptions