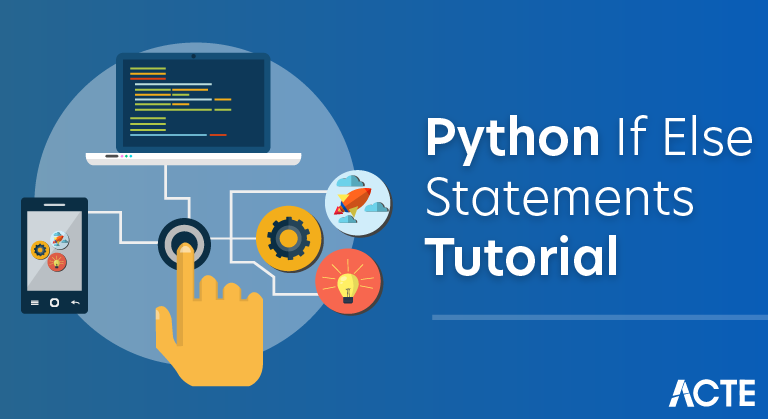
An else statement can be combined with an if statement. An else statement contains the block of code that executes if the conditional expression in the if statement resolves to 0 or a FALSE value.The else statement is an optional statement and there could be at most only one else statement following if.
Syntax:
- The syntax of the if…else statement is:
- if expression:
- statement(s)
- else:
- statement(s)
Flow Diagram:
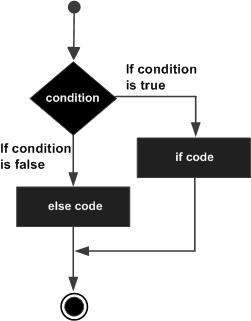
Example:
- #!/usr/bin/python
- var1 = 100
- if var1:
- print “1 – Got a true expression value”
- print var1
- else:
- print “1 – Got a false expression value”
- print var1
- var2 = 0
- if var2:
- print “2 – Got a true expression value”
- print var2
- else:
- print “2 – Got a false expression value”
- print var2
- print “Good bye!”
When the above code is executed, it produces the following result:
- 1 – Got a true expression value
- 100
- 2 – Got a false expression value
- 0
- Good bye!
The elif Statement:
The elif statement allows you to check multiple expressions for TRUE and execute a block of code as soon as one of the conditions evaluates to TRUE.Similar to the else, the elif statement is optional. However, unlike else, for which there can be at most one statement, there can be an arbitrary number of elif statements following an if.
syntax:
- if expression1: statement(s)
- elif expression2: statement(s)
- elif expression3: statement(s)
- else: statement(s)
Core Python does not provide switch or case statements as in other languages, but we can use if..elif…statements to simulate switch case as follows:
Example:
- #!/usr/bin/python
- var = 100
- if var == 200:
- print “1 – Got a true expression value”
- print var
- elif var == 150:
- print “2 – Got a true expression value”
- print var
- elif var == 100:
- print “3 – Got a true expression value”
- print var
- else:
- print “4 – Got a false expression value”
- print var
- print “Good bye!”
When the above code is executed, it produces the following result:
- 3 – Got a true expression value
- 100
- Good bye!
Python – Decision Making
Decision making is anticipation of conditions occurring while execution of the program and specifying actions taken according to the conditions.Decision structures evaluate multiple expressions which produce TRUE or FALSE as outcome. You need to determine which action to take and which statements to execute if the outcome is TRUE or FALSE otherwise.Following is the general form of a typical decision making structure found in most of the programming languages
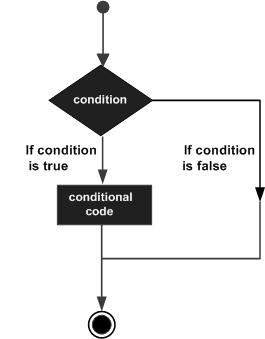
Python programming language assumes any non-zero and non-null values as TRUE, and if it is either zero or null, then it is assumed as FALSE value.Python programming language provides following types of decision making statements. Click the following links to check their detail.
Statement | Description |
---|---|
if statements | An if statement consists of a boolean expression followed by one or more statements. |
if…else | statements An if statement can be followed by an optional else statement, which executes when the boolean expression is FALSE. |
Let us go through each decision making briefly
Single Statement Suites:
If the suite of an if clause consists only of a single line, it may go on the same line as the header statement.
Here is an example of a one-line if clause:
- #!/usr/bin/python
- var = 100
- if ( var == 100 ) : print “Value of expression is 100”
- print “Good bye!”
When the above code is executed, it produces the following result:
- Value of expression is 100
- Good bye!
Python – Loops
In general, statements are executed sequentially: The first statement in a function is executed first, followed by the second, and so on. There may be a situation when you need to execute a block of code several number of times.Programming languages provide various control structures that allow for more complicated execution paths.A loop statement allows us to execute a statement or group of statements multiple times. The following diagram illustrates a loop statement −
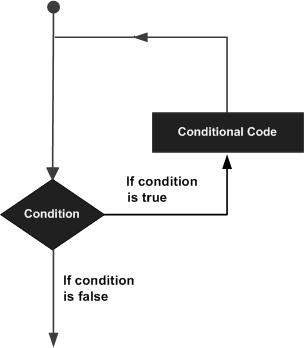
Python programming language provides the following types of loops to handle looping requirements.
Loop Type | Description |
---|---|
while loop | Repeats a statement or group of statements while a given condition is TRUE. It tests the condition before executing the loop body. |
for loop | Executes a sequence of statements multiple times and abbreviates the code that manages the loop variable. |
nested loops | You can use one or more loops inside any another while, for or do..while loop. |
Loop Control Statements:
Loop control statements change execution from its normal sequence. When execution leaves a scope, all automatic objects that were created in that scope are destroyed.Python supports the following control statements. Click the following links to check their detail.
Let us go through the loop control statements briefly
Control Statement | Description |
---|---|
break statement | Terminates the loop statement and transfers execution to the statement immediately following the loop. |
continue statement | Causes the loop to skip the remainder of its body and immediately retest its condition prior to reiterating. |
pass statement | The pass statement in Python is used when a statement is required syntactically but you do not want any command or code to execute. |
Python – Numbers
Number data types store numeric values. They are immutable data types, meaning that changing the value of a number data type results in a newly allocated object.
Number objects are created when you assign a value to them. For example:
- var1 = 1
- var2 = 10
You can also delete the reference to a number object by using the del statement. The syntax of the del statement is −del var1[,var2[,var3[….,varN]]]]
You can delete a single object or multiple objects by using the del statement. For example:
- del var
- del var_a, var_b
Python supports four different numerical types :
int (signed integers) − They are often called just integers or ints, are positive or negative whole numbers with no decimal point.
- long (long integers ) − Also called longs, they are integers of unlimited size, written like integers and followed by an uppercase or lowercase L.
- float (floating point real values) − Also called floats, they represent real numbers and are written with a decimal point dividing the integer and fractional parts. Floats may also be in scientific notation, with E or e indicating the power of 10 (2.5e2 = 2.5 x 102 = 250).
- complex (complex numbers) − are of the form a + bJ, where a and b are floats and J (or j) represents the square root of -1 (which is an imaginary number). The real part of the number is a, and the imaginary part is b. Complex numbers are not used much in Python programming.
Examples
Here are some examples of numbers
int | long | floa | complex |
---|---|---|---|
10 | 51924361L | 0.0 | 3.14j |
100 | -0x19323L | 15.20 | 45.j |
-786 | 0122L | -21.9 | 9.322e-36j |
080 | 0xDEFABCECBDAECBFBAEL | 32.3+e18 | .876j |
-0490 | 535633629843L | -90. | -.6545+0J |
Python allows you to use a lowercase L with long, but it is recommended that you use only an uppercase L to avoid confusion with the number 1. Python displays long integers with an uppercase L.
- A complex number consists of an ordered pair of real floating point numbers denoted by a + bj, where a is the real part and b is the imaginary part of the complex number.
Number Type Conversion:
Python converts numbers internally in an expression containing mixed types to a common type for evaluation. But sometimes, you need to coerce a number explicitly from one type to another to satisfy the requirements of an operator or function parameter.
- Type int(x) to convert x to a plain integer.
- Type long(x) to convert x to a long integer.
- Type float(x) to convert x to a floating-point number.
- Type complex(x) to convert x to a complex number with real part x and imaginary part zero.
- Type complex(x, y) to convert x and y to a complex number with real part x and imaginary part y. x and y are numeric expressions
Mathematical Functions:
Python includes the following functions that perform mathematical calculation
Function | return description |
---|---|
abs(x) | The absolute value of x: the (positive) distance between x and zero. |
ceil(x) | The ceiling of x: the smallest integer not less than x |
Random Number Functions:
Random numbers are used for games, simulations, testing, security, and privacy applications. Python includes the following functions that are commonly used.
Function | Description |
---|---|
choice(seq) | A random item from a list, tuple, or string. |
randrange ([start,] stop [,step]) | A randomly selected element from range(start, stop, step) |
random() | A random float r, such that 0 is less than or equal to r and r is less than 1 |
seed([x]) |
Sets the integer starting value used in generating random numbers. Call this function before calling any other random module function. Returns None. |
shuffle(lst) | Randomizes the items of a list in place. Returns None. |
Trigonometric Functions:
Python includes the following functions that perform trigonometric calculations.
Function | Description |
---|---|
acos(x) | Return the arc cosine of x, in radians. |
asin(x) | Return the arc sine of x, in radians. |
atan(x) | Return the arc tangent of x, in radians. |
atan2(y, x) | Return atan(y / x), in radians. |
cos(x) | Return the cosine of x radians. |
hypot(x, y) | Return the Euclidean norm, sqrt(x*x + y*y). |
Mathematical Constants:
The module also defines two mathematical constants:
Constants | Description |
---|---|
pi | The mathematical constant pi. |
E | The mathematical constant e. |
Python – Strings
Strings are amongst the most popular types in Python. We can create them simply by enclosing characters in quotes. Python treats single quotes the same as double quotes. Creating strings is as simple as assigning a value to a variable. For example:
- var1 = ‘Hello World!’
- >var2 = “Python Programming”
Accessing Values in Strings:
- Python does not support a character type; these are treated as strings of length one, thus also considered a substring.
- To access substrings, use the square brackets for slicing along with the index or indices to obtain your substring. For example:
- #!/usr/bin/python
- var1 = ‘Hello World!’
- var2 = “Python Programming”
- print “var1[0]: “, var1[0]
- print “var2[1:5]: “, var2[1:5]
When the above code is executed, it produces the following result
- var1[0]: H
- var2[1:5]: ytho
Conclusion:
Hypotheses followed by a conclusion are called an If-then statement or a conditional statement.A conditional statement is false if the hypothesis is true and the conclusion is false. The example above would be false if it said “if you get good grades then you will not get into a good college”.