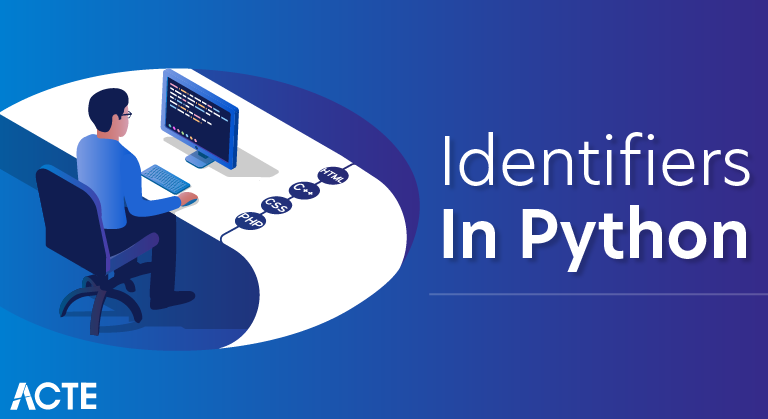
An identifier is a name given to entities like class, functions, variables, etc. It helps to differentiate one entity from another.
Rules for writing identifiers
- Identifiers can be a combination of letters in lowercase (a to z) or uppercase (A to Z) or digits (0 to 9) or an underscore _. Names like myClass, var_1 and print_this_to_screen, all are valid example.
- An identifier cannot start with a digit. 1variable is invalid, but variable1 is a valid name.
Keywords cannot be used as identifiers.
- global = 1
- Output
File “<interactive input>”, line 1 - global = 1
- ^
SyntaxError: invalid syntax
We cannot use special symbols like !, @, #, $, % etc. in our identifier.
a@ = 0
- Output
File “<interactive input>”, line 1 - a@ = 0
- ^
- SyntaxError: invalid syntax
- An identifier can be of any length.
Ways to Define Identifiers in Python
We can define identifiers in Python in a few ways:
“An identifier is a user-defined name to represent a variable, a function, a class, a module, or any other object.”
“It is a programmable entity in Python- one with a name.”
“It is a name given to the fundamental building blocks in a program.”
Python Identifier Naming Rules
Rules in Identifiers in Python
So we know what a Python Identifier is. But can we name it anything? Or do certain rules apply? Well, we do have five rules to follow when naming identifiers in Python:
A Python identifier can be a combination of lowercase/ uppercase letters, digits, or an underscore. The following characters are valid:
- Lowercase letters (a to z)
- Uppercase letters (A to Z)
- Digits (0 to 9)
- Underscore (_)
Some valid names are:
- myVar
- var_3
- this_works_too
- An identifier cannot begin with a digit.
Some valid names:
- _9lives
- lives9
- We cannot use special symbols in the identifier name. Some of these are:
- @
- !
- #
- $
- %
We cannot use a keyword as an identifier. Keywords are reserved names in Python and using one of those as a name for an identifier will result in a SyntaxError.
An identifier can be as long as you want. According to the docs, you can have an identifier of infinite length. However, the PEP-8 standard sets a rule that you should limit all lines to a maximum of 79 characters.
Lexical Definitions in Python Identifiers
To sum those rules up lexically, we can say:
- identifier ::= (letter | “_”) (letter | digit | “_”)* #It has to begin with a letter or an underscore; letters, digits, or/and underscores may follow
- letter ::= lowercase | uppercase #Anything from a-z and from A-Z
- lowercase ::= “a” … “z” #Lowercase letters a to z
- uppercase ::= “A” … “Z” #Uppercase letters A to Z
- digit ::= “0” … “9” #Integers 0 to 9
Best Practices in Identifiers in Python
While it’s mandatory to follow the rules, it is also good to follow some recommended practices:
- Begin class names with an uppercase letter, begin all other identifiers with a lowercase letter
- Begin private identifiers with an underscore (_); Note that this doesn’t make a variable private, but discourages the user from attempting to access it
- Put __ around names of magic methods (use leading and trailing double underscores), avoid doing this to anything else. Also, built-in types already use this notation.
- Use leading double underscores only when dealing with mangling.
- Prefer using names longer than one character- index=1 is better than i=1
- Use underscores to combine words in an identifier, like in this_is_an_identifier
- Since Python is case-sensitive, name and Name are two different identifiers.
- Use camel case for naming. Let’s just clear the air here by saying camelcase is myVarOne and Pascal case is MyVarOne.
Testing the Validity of Identifiers in Python
While it is great to follow the rules and guidelines, we can test an identifier’s validity just to be sure. For this, we make use of the keyword.iskeyword() function.
The keyword module lets us determine whether a string is a keyword. It has two functions:
- keyword.iskeyword(s)- If s is a Python keyword, return true
- Keyword.kwlist- Return a sequence holding all keywords the interpreter understands. This includes even those that are active only when certain __future__ statements are in effect.
Coming back to iskeyword(s), it returns True if the string s is a reserved keyword. Else, it returns False. Let’s import this module.
- import keyword
- keyword.iskeyword(‘_$$_’)
False
- keyword.iskeyword(‘return’)
True.
Also, the str.isidentifier() function will tell us if a string is a valid identifier. This is available since Python 3.0.
Reserved Classes of Python Identifiers
Finally, let us talk about classes of identifiers. Some classes have special meanings and to identify them, we use patterns of leading and trailing underscores.
Single Leading Underscore (_*)
We use this identifier to store the result of the last evaluation in the interactive interpreter. This result is stored in the __builtin__ module. Importing a module as from module import * does not import such private variables.
Leading and Trailing Double Underscores (__*__)
These are system-defined names (by the interpreter). A class can implement operations to be invoked by special syntax using methods with special names. Consider this an attempt at operator overloading in a Pythonic fashion. One such special/ magic method is __getitem__(). Then, x[i] is equivalent to x.__getitem__(i). In the near future, the set of names of this class by Python may be extended.
Leading Double Underscores (__*)
These are class-private names. Within a class definition, the interpreter rewrites (mangles) such a name to avoid name clashes between the private attributes of base and derived classes.So, this was all in Identifiers in Python tutorial. Hope you like our explanation.
Things to Remember
Python is a case-sensitive language. This means, Variable and variable are not the same.
Always give the identifiers a name that makes sense. While c = 10 is a valid name, writing count = 10 would make more sense, and it would be easier to figure out what it represents when you look at your code after a long gap.
Multiple words can be separated using an underscore, like this_is_a_long_variable.
Variable name is known as identifier. There are few rules that you have to follow while naming the variables in Python.
For example here the variable is of integer type that holds the value 10. The name of the variable, which is num is called identifier.
- num = 10
- The name of the variable must always start with either a letter or an underscore (_). For example: _str, str, num, _num are all valid name for the variables.
- The name of the variable cannot start with a number. For example: 9num is not a valid variable name.
- The name of the variable cannot have special characters such as %, $, # etc, they can only have alphanumeric characters and underscore (A to Z, a to z, 0-9 or _ ).
Variable name is case sensitive in Python which means num and NUM are two different variables in python.
Python identifier example
In the following example we have three variables. The name of the variables num, _x and a_b are the identifiers.
- # few examples of identifiers
- num = 10
- print(num)
- _x = 100
- print(_x)
- a_b = 99
- print(a_b)
Output:
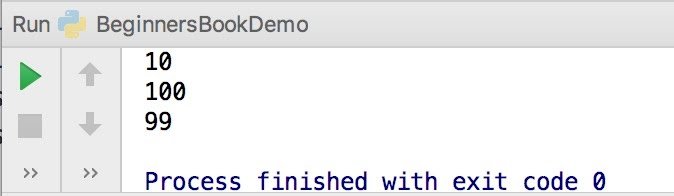
Best Practices for Identifier Naming.
- Better have class names starting with a capital letter. All other identifiers should begin with a lowercase letter.
- Declare private identifiers by using the (‘_’) underscore as their first letter.
- Don’t use ‘_’ as the leading and trailing character in an identifier. As Python built-in types already use this notation.
- Avoid using names with only one character. Instead, make meaningful names.
- For example – While i = 1 is valid, but writing iter = 1 or index = 1 would make more sense.
- You can use underscore to combine multiple words to form a sensible name.
- For example – count_no_of_letters.
Conclusion
Hence, in this Python Identifiers, we discussed the meaning of Identifiers in Python. Moreover, we learned naming rules and best practices in Python Identifiers. Also, we discussed reserved classes in Python Identifier. Still, if you have any doubt, ask in the comment tab.