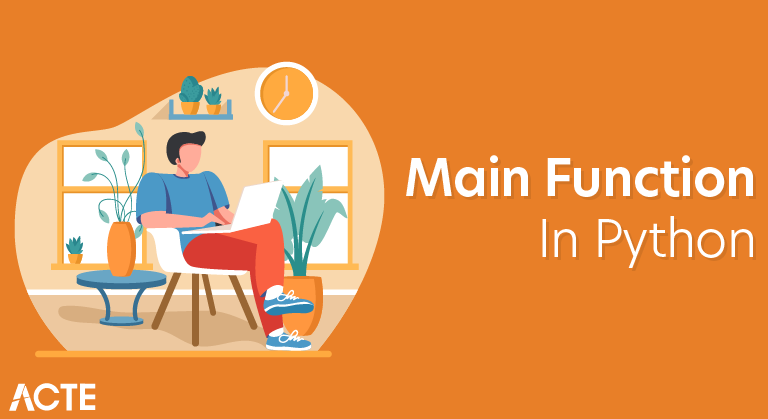
Main function is like the entry point of a program. However, the Python interpreter runs the code right from the first line. The execution of the code starts from the starting line and goes line by line. It does not matter where the main function is present or it is present or not.
Since there is no main() function in Python, when the command to run a Python program is given to the interpreter, the code that is at level 0 indentation is to be executed. However, before doing that, it will define a few special variables. __name__ is one such special variable.
If the source file is executed as the main program, the interpreter sets the __name__ variable to have a value __main__.
If this file is being imported from another module, __name__ will be set to the module’s name.
__name__ is a built-in variable which evaluates to the name of the current module.
Example:
filter_none
edit
play_arrow
brightness_4
- # Python program to demonstrate
- # main() function
- print(“Hello”)
- # Defining main function
- def main():
- print(“hey there”)
- # Using the special variable
- # __name__
- if __name__==”__main__”:
- main()
Output:
Hello
hey there
When the above program is executed, the interpreter declares the initial value of name as “main”. When the interpreter reaches the if statement it checks for the value of name and when the value of if is true it runs the main function else the main function is not executed.
Main function as Module
Now when we import a Python script as a module the __name__ variable gets the value same as the name of the python script imported.
Example: Let’s consider there are two Files(File1.py and File2.py). File1 is as follows.
filter_none
edit
play_arrow
brightness_4
# File1.py
- print(“File1 __name__ = %s” %__name__)
- if __name__ == “__main__”:
- print(“File1 is being run directly”)
- else:
- print(“File1 is being imported”)
Output:
File1 __name__ = __main__
File1 is being run directly
Now, when the File1.py is imported into File2.py, the value of __name__ changes.
filter_none
brightness_4
# File2.py
- import File1
- print(“File2 __name__ = %s” %__name__)
- if __name__ == “__main__”:
- print(“File2 is being run directly”)
- else:
- print(“File2 is being imported”)
Output:
File1 __name__ = File1
File1 is being imported
File2 __name__ = __main__
File2 is being run directly
As seen above, when File1.py is run directly, the interpreter sets the __name__ variable as __main__ and when it is run through File2.py by importing, the __name__ variable is set as the name of the python script, i.e. File1. Thus, it can be said that if __name__ == “__main__” is the part of the program that runs when the script is run from the command line using a command like Python File1.py.
Many programming languages have a special function that is automatically executed when an operating system starts to run a program. This function is usually called main() and must have a specific return type and arguments according to the language standard. On the other hand, the Python interpreter executes scripts starting at the top of the file, and there is no specific function that Python automatically executes.
Nevertheless, having a defined starting point for the execution of a program is useful for understanding how a program works. Python programmers have come up with several conventions to define this starting point.
By the end of this article, you’ll understand:
- What the special __name__ variable is and how Python defines it
- Why you would want to use a main() in Python
- What conventions there are for defining main() in Python
- What the best-practices are for what code to put into your main()
A Basic Python main()
In some Python scripts, you may see a function definition and a conditional statement that looks like the example below:
- def main():
- print(“Hello World!”)
- if __name__ == “__main__”:
- main()
In this code, there is a function called main() that prints the phrase Hello World! when the Python interpreter executes it. There is also a conditional (or if) statement that checks the value of __name__ and compares it to the string “__main__”. When the if statement evaluates to True, the Python interpreter executes main(). This code pattern is quite common in Python files that you want to be executed as a script and imported in another module. To help understand how this code will execute, you should first understand how the Python interpreter sets __name__ depending on how the code is being executed.
Execution Modes in Python
There are two primary ways that you can instruct the Python interpreter to execute or use code:
- You can execute the Python file as a script using the command line.
- You can import the code from one Python file into another file or into the interactive interpreter.
You can read a lot more about these approaches in How to Run Your Python Scripts. No matter which way of running your code you’re using, Python defines a special variable called __name__ that contains a string whose value depends on how the code is being used.
We’ll use this example file, saved as execution_methods.py, to explore how the behavior of the code changes depending on the context:
- print(“This is my file to test Python’s execution methods.”)
- print(“The variable __name__ tells me which context this file is running in.”)
- print(“The value of __name__ is:”, repr(__name__))
In this file, there are three calls to print() defined. The first two print some introductory phrases. The third print() will first print the phrase The value of __name__ is, and then it will print the representation of the __name__ variable using Python’s built-in repr().
In Python, repr() displays the printable representation of an object. This example uses repr() to emphasize that the value of __name__ is a string. You can read more about repr() in the Python documentation.
You’ll see the words file, module, and script used throughout this article. Practically, there isn’t much difference between them. However, there are slight differences in meaning that emphasize the purpose of a piece of code:
- File: Typically, a Python file is any file that contains code. Most Python files have the extension .py.
- Script: A Python script is a file that you intend to execute from the command line to accomplish a task.
- Module: A Python module is a file that you intend to import from within another module or a script, or from the interactive interpreter. You can read more about modules in the Python documentation.
This distinction is also discussed in How to Run Your Python Scripts.
Executing From the Command Line
In this approach, you want to execute your Python script from the command line.
When you execute a script, you will not be able to interactively define the code that the Python interpreter is executing. The details of how you can execute Python from your command line are not that important for the purpose of this article, but you can expand the box below to read more about the differences between the command line on Windows, Linux, and macOS.
Importing Into a Module or the Interactive Interpreter
Now let’s take a look at the second way that the Python interpreter will execute your code: imports. When you are developing a module or script, you will most likely want to take advantage of modules that someone else has already built, which you can do with the import keyword.
During the import process, Python executes the statements defined in the specified module (but only the first time you import a module). To demonstrate the results of importing your execution_methods.py file, start the interactive Python interpreter and then import your execution_methods.py file:
- >>> import execution_methods
This is my file to test Python’s execution methods.
The variable __name__ tells me which context this file is running in.
The value of __name__ is: ‘execution_methods’
In this code output, you can see that the Python interpreter executes the three calls to print(). The first two lines of output are exactly the same as when you executed the file as a script on the command line because there are no variables in either of the first two lines. However, there is a difference in the output from the third print().
When the Python interpreter imports code, the value of __name__ is set to be the same as the name of the module that is being imported. You can see this in the third line of output above. __name__ has the value ‘execution_methods’, which is the name of the .py file that Python is importing from.
Note that if you import the module again without quitting Python, there will be no output.
Best Practices for Python Main Functions
Now that you can see the differences in how Python handles its different execution modes, it’s useful for you to know some best practices to use. These will apply whenever you want to write code that you can run as a script and import in another module or an interactive session.
You will learn about four best practices to make sure that your code can serve a dual purpose:
- Put most code into a function or class.
- Use __name__ to control execution of your code.
- Create a function called main() to contain the code you want to run.
- Call other functions from main().