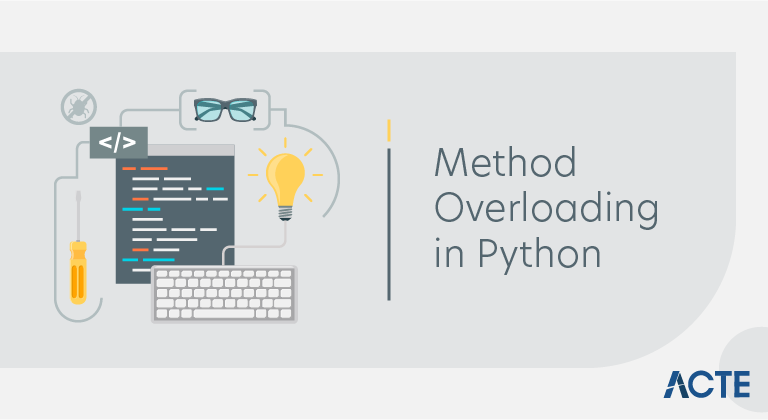
Must-Know Method Overloading in Python & How to Master It
Last updated on 05th Jun 2020, Blog, General
Method overloading is a unique methodology offered by Python. Using this feature, a method can be defined in such a manner that it can be called in multiple ways. Every time, a method is called, it depends on the user as to how to call that method i.e. how many and what parameters to pass in the method. So, the method can be called with no or single or multiple parameters. Such a functionality offered by Python is termed as Method Overloading in Python. The feature allows the use of methods to be regulated in a user-defined manner.
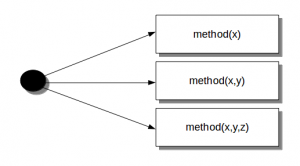
Several ways to call a method (method overloading)
- In Python you can define a method in such a way that there are multiple ways to call it.
- Given a single method or function, we can specify the number of parameters yourself.
- Depending on the function definition, it can be called with zero, one, two or more parameters.
- This is known as method overloading. Not all programming languages support method overloading, but Python does.
What is Overloading?
Overloading is the ability of a function or an operator to behave in different ways based on the parameters that are passed to the function, or the operands that the operator acts on.
Some of the advantages of using overload are:
- Overloading a method fosters reusability. For example, instead of writing multiple methods that differ only slightly, we can write one method and overload it.
- Overloading also improves code clarity and eliminates complexity.
- Overloading is a very useful concept. However, it has a number of disadvantages associated with it.
- Overloading can create confusion when used across inheritance boundaries. When used excessively, it becomes cumbersome to manage overloaded functions.
Method Overloading in Python
In Python, you can create a method that can be called in different ways. So, you can have a method that has zero, one or more number of parameters. Depending on the method definition, we can call it with zero, one or more arguments.
Given a single method or function, the number of parameters can be specified by you. This process of calling the same method in different ways is called method overloading.
Examples of Method Overloading in Python
Given below are the examples of Method Overloading in Python:
Example 1:
Code:
- # Define the class
- class multiply:
- # Define the method
- def mult_num(self, n1 = None, n2 = None, n3 = None):
- if n1 != None and n2 != None and n3 != None:
- print(n1 * n2 * n3)
- elif n1 != None and n2 != None:
- print(n1 * n2)
- else:
- print(n1)
- # Creating object as an instance of class
- object_multiply = multiply()
- # Sinlge variable method execution
- object_multiply.mult_num(20)
- # Two variable method execution
- object_multiply.mult_num(20, 30)
- # Three variable method execution
- object_multiply.mult_num(20, 30, 40)
Output:
In the above program, we have class multiply. The class has a method called mult_num. This method takes three numbers as parameters. Using the if-elif-else statement it is checked any of the numbers equals to None. When only a single number is passed as a parameter, the program simply prints that number. When two numbers are passed as parameters, the program returns the product of these two numbers, and when three numbers are passed as parameters the program returns the product of the three numbers. So, we find a program being used in a manner as required by the user. In every situation the method does its job correctly.
In the above program code, the parameters were passed as hard-coded values, and so, we got the output as shown below.
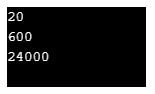
Example 2:
We shall modify the above program code slightly in the sense that we take the inputs from the user.
Code:
- # Define the class
- class multiply:
- # Define the method
- def mult_num(self, n1 = None, n2 = None, n3 = None):
- if n1 != None and n2 != None and n3 != None:
- print(n1 * n2 * n3)
- elif n1 != None and n2 != None:
- print(n1 * n2)
- else:
- print(n1)
- # Creating object as an instance of class
- object_multiply = multiply()
- # Sinlge variable method execution
- print(“Single variable operation”)
- object_multiply.mult_num(float(input(“Enter the number: “)))
- # Two variable method execution
- print(“Two variable operation”)
- object_multiply.mult_num(float(input(“Enter the first number: “)), float(input(“Enter the second number: “)))
- # Three variable method execution
- print(“Three variable operation”)
- object_multiply.mult_num(float(input(“Enter the first number: “)), float(input(“Enter the second number: “)), float(input(“Enter the third number: “)))
Output:
We can see those input parameters are no more hard-coded. They can be passed dynamically. The default data type of the input variables is a string, so, we need to convert it into a number first. As a result of this, we used the float function for type conversion. Let’s see how the program code works when executed.
Initially, the single variable operation gets performed. We passed 12.5 as the input and got the same number as output. Then we are moved to “Two variable operations” as shown in the below screenshot.
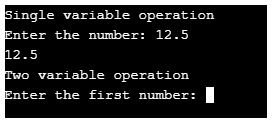
In “Two variable operations”, we specified the first number as 13.5 and the second number as 19.7 as can be seen in the below screenshot.
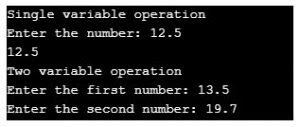
Just go through the screenshot below, and see that this time we have got the product of two decimal numbers which we have passed as input parameters. The product is 265.95. As soon as the two variable operation gets performed, we are moved to “Three variable operations” as shown in the below screenshot.
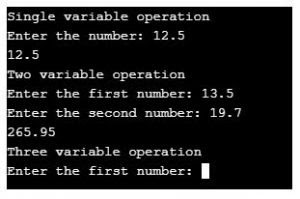
Finally, we can see that the program code returns the product of three variables as can be seen in the bottom section of the output.
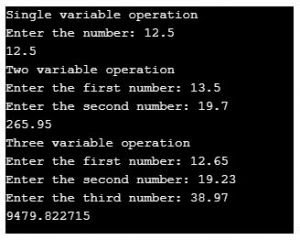
Through the above programs, we saw how the concept of method overloading works in Python. What basically, we do is that we create an object which we assign to the class. The class object as an operator operates over the method defined in the class. The object regulates the use of function based on the requirement.
Through the above two examples, we worked on numeric data. Now, we go through a program that demonstrates the concept of method overloading through string variable examples.
Example 3:
The program code is as written below. Go through the code and see how each of its components work.
Code:
- class Name:
- def name_declare(self, name1 = None, name2 = None):
- if name1 is not None and name2 is not None:
- print(“Hello, I am ” + name1 + ” ” + name2+”.”)
- elif name1 is not None:
- print(“Hello, I am ” + name1 + “.”)
- else:
- print(“Hi, How are you doing?”)
- object_name = Name()
- # method execution by passing both name and surname.
- object_name.name_declare(“John”, “Maurice”)
- # method execution by passing just name.
- object_name.name_declare(“Matthews”)
- # method execution by not passing any parameter.
- object_name.name_declare()
When the above program code in Python is executed, we get the output as shown by the below screenshot.
Conclusion
Method overloading is a very crucial methodology offered by Python. The methodology is quite useful in complex situations, in which condition-based use and execution of certain parameters are needed. The methodology must be used appropriately, so as to avoid wrong use of any parameter.