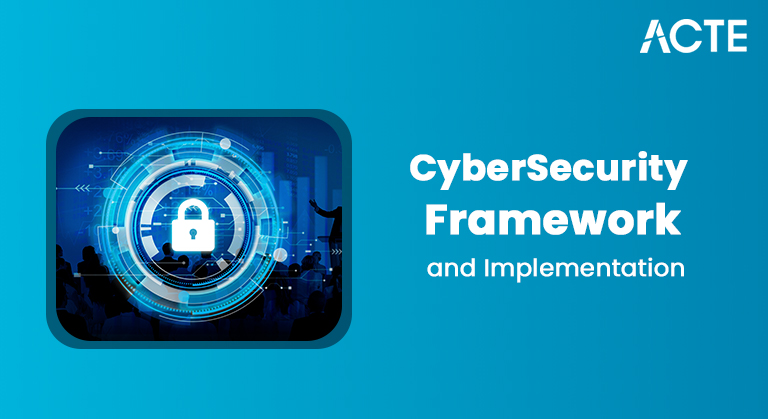
- Introduction to Git and Version Control
- History and Evolution of Git
- Key Features of Git
- Basic Git Commands and Their Uses
- Git Branching and Merging
- Working with Remote Repositories
- Git Workflow: Centralized vs Distributed
- Best Practices for Using Git
- Git vs. Other Version Control Systems
- Advanced Git Features and Customization
- Conclusion
Introduction to Git and Version Control
In today’s software development world, version control systems (VCS) are essential for managing the development process. GitLab is one of the most widely used distributed version control systems, enabling developers to track changes in their codebase, collaborate effectively, and maintain the integrity of their project over time. Git’s popularity stems from its robustness, flexibility, and ability to manage complex software development projects. Version control allows developers to track changes in their code, revert to previous versions when necessary, and collaborate with others in a team environment. There are two primary types of version control systems: centralized and distributed. Git is a distributed version control system, meaning every developer has a complete copy of the project history on their local machine, enabling faster and more efficient workflows. In this article, we will explore the history, features, and key functionalities of Git and provide practical tips and best practices for using Git in software development projects.
History and Evolution of Git
Linus Torvalds created Git in 2005 to support the development of the Linux kernel. Before Git, the Linux kernel project used a version control system called BitKeeper. However, due to licensing issues with BitKeeper, Torvalds decided to create a new version control system that would better suit the needs of large-scale development projects. The main goals of Git were to be fast and reliable and allow decentralized development. Torvalds designed Git focusing on performance and the ability to handle large repositories efficiently. Over the years, Git has evolved from a tool designed for a single project (the Linux kernel) to a widely used version control system in software development. Since its creation, Git has undergone continuous development, with contributions from developers worldwide. In 2008, Git was open-sourced under the General Public License (GPL), which allowed it to gain widespread adoption and become the tool of choice for version control in many software development environments. Today, Git powers some of the most significant open-source projects, and it is also integrated into major platforms like GitHub, GitLab, and Bitbucket, making collaboration more straightforward and efficient.
Key Features of Git
Git is a powerful version control system with many features to help developers manage their code. Some of the key features of Git include:
- Distributed Version Control: In Git, every developer has a local copy of the entire repository, including its history. This allows developers to work offline, make changes, and commit them locally before pushing them to a central repository. This decentralized model makes Git more resilient and faster for certain tasks than centralized version control systems.
- Branching and Merging: Git allows developers to create multiple branches within a repository, enabling them to work on different features or bug fixes simultaneously. Once development is complete, branches can be easily merged back into the main codebase. Git’s branching model is lightweight and efficient, encouraging frequent use of branches to isolate work and improve code management.
- Commit History: Every change made in Git is recorded in a commit, including a snapshot of the code at a particular time. Git’s commit history is immutable, meaning it cannot be changed once a commit is made. This provides a reliable record of all changes made to a project.
- Staging Area: Git uses a staging area (also known as the index) where changes are temporarily stored before they are committed to the repository. This allows developers to review and organize their changes before finalizing them.
- Remote Repositories: Git allows developers to work with remote repositories, enabling collaboration with other team members. Git developers can push their changes to a shared remote repository and pull updates from others, ensuring everyone is working with the project’s latest version.
- Fast and Efficient: Git is designed for speed and efficiency, even with large repositories. Git’s data storage model and indexing allow quick access to previous commits, making it well-suited for large-scale projects with many files and contributors.
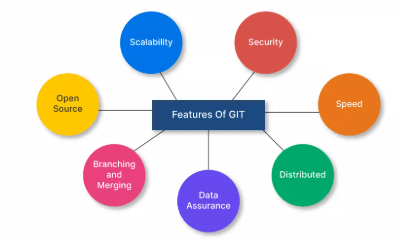
Basic Git Commands and Their Uses
Git provides a set of powerful commands for managing repositories, staging changes, and interacting with remote repositories. Here are some of the most commonly used Git commands:
- Git init : Initializes a new Git powers repository in the current directory. This command is used when starting a new project and setting up a repository for version control.
- Git clone [url]: Clones an existing repository from a remote source, such as GitHub or GitLab, to your local machine. This command copies the entire repository, including its history.
- Git status : shows the repository’s current state, including any changes made to files, files staged for commit, and untracked files.
- Git add [file]: adds files to the staging area, preparing them for commit. For example, Git adds index.html stages to the file index.html for commit.
- Git commit -m “[message]”: Commits the staged changes with a descriptive message. The commit message should describe the changes made to the project.
- Git log: This command displays the repository’s commit history, showing a list of commits along with their timestamps and commit messages.
- Git push [remote] [branch]: This command pushes local changes to a remote repository. For example, Git push origin master pushes the changes from the local master branch to the origin remote repository.
- Git pull [remote] [branch]: This command pulls changes from a remote repository and merges them into the local repository. It is commonly used to fetch and integrate changes made by other contributors.
- Git branch: Lists all the branches in the repository. It also highlights the current branch you are working on.
- Git checkout [branch]: This command switches to a different branch in the repository. For example, git checkout feature-branch switches to the feature-branch
- Branch git merge [branch]: Merges the specified branch into the current branch.
Git Branching and Merging
Git branching and merging are essential features that enable developers to manage different lines of development within a project. A branch in Git represents an independent version of the project, allowing developers to work on new features, bug fixes, or experiments without impacting the main codebase, typically referred to as the master or main branch. To create a new branch, you use the Git powers branch command followed by the branch name (e.g., git branch feature-branch). However, creating a branch alone does not switch to it; you would need to run git checkout feature-branch to start working on it. Alternatively, you can combine both creating and switching to a new branch in a single command with git checkout -b feature-branch. After working on the changes in the new branch, the next step is to merge it back into the main branch. To do this, first switch to the branch you want to merge changes into, such as git checkout master, and then use the GitLab merge command to combine the changes, for example, git merge feature-branch. This process ensures that new developments are integrated into the main codebase efficiently while keeping changes isolated and manageable.
Working with Remote Repositories
In collaborative development, multiple developers often work on the same project. Git powers allows developers to work with remote repositories hosted on platforms like GitHub, GitLab, or Bitbucket. A remote repository is typically a shared repository from which Git developers push and pull.
Adding a Remote Repository
To link your local repository to a remote repository, use the Git remote add command:
- git remote add origin https://github.com/username/repository.git
This associates your local repository with the remote repository located at the specified URL.
Pushing and Pulling Changes
Once the remote repository is configured, you can push changes to the remote repository and pull changes from it. Use the git push command to upload changes:
- git push origin master
Use the git pull command to fetch and integrate changes from the remote repository:
- git pull origin master
Git Workflow: Centralized vs Distributed
Git’s distributed nature offers flexibility in team collaboration. There are two primary Git workflows centralized and distributed.
- Centralized Workflow: In this workflow, developers clone the central repository and commit their changes locally before pushing them back to the central repository. This is similar to how centralized version control systems like Subversion (SVN) work. The centralized workflow is simple and well-suited for smaller teams.
- Distributed Workflow: In the distributed workflow, Git developers work on their copies of the repository and can push changes to a shared repository when ready. This allows for more flexibility and decentralized collaboration, as developers can work independently without affecting others.
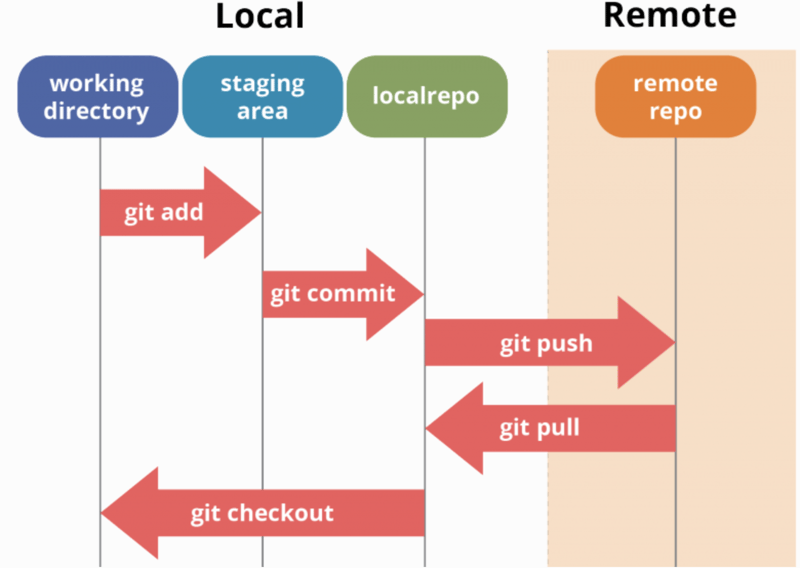
Best Practices for Using Git
To maximize the benefits of Git and avoid common pitfalls, developers should follow some best practices. Commit Early and Often Make small, incremental commits to ensure your changes are tracked in the repository and easy to revert if necessary. Write Meaningful Commit Messages Use clear, concise commit messages explaining the changes’ purpose. This makes it easier for others to understand your work. Branch for Features Always create a new branch for each feature or bug fix to keep the main branch clean and stable. Pull Frequently pull changes from the remote repository to stay up-to-date with the latest changes from your team members. Avoid Committing Sensitive Data Never commit sensitive information such as passwords or API keys to a Git repository. Use a .gitignore file to exclude sensitive files.
Git vs Other Version Control Systems
Git stands out among other version control systems like Subversion (SVN), Mercurial (Hg), and CVS due to its distributed nature, speed, and flexibility. While centralized systems like Subversion store the repository in a single location, Git’s distributed architecture allows every developer to have a full copy of the repository, enabling offline work and faster operations. Git also provides better branching and merging support, making it the go-to choice for modern software development projects.
Advanced Git Features and Customization
Git offers a variety of advanced features for power users and organizations with specific needs:
- Rebasing: Rebase is an advanced way of integrating changes from one branch into another. It rewrites commit history to provide a cleaner, linear project history.
- Git Hooks: Git hooks are scripts triggered by various Git events, such as commits or pushes, to automate tasks like testing or deployment.
- Submodules: Git allows you to embed other Git repositories inside a repository as submodules. This is useful for managing dependencies or large projects.
Conclusion
Git is an essential tool for modern software development, providing powerful features for tracking changes, collaborating with Subversion , and managing complex projects. Understanding the basics of Git commands, branching, merging, and working with remote repositories is crucial for developers. As you gain more experience with Git, you can use advanced features like rebasing and hooks to customize your Git Workflow and improve your development process. By following best practices and leveraging Git’s full capabilities, Git developers can ensure efficient, streamlined development and maintain a clean and reliable codebase.