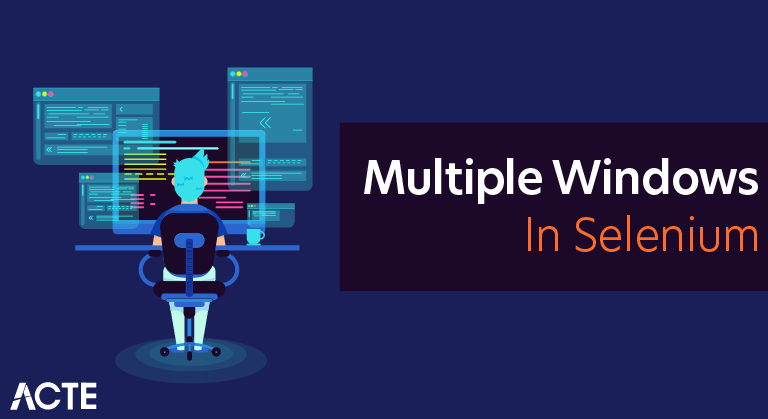
In this rapidly developing world of technology, one must always find ways to keep up with the time. As the world is evolving towards software development, testing plays a vital role in making the process defect free. Selenium is one such tool that helps in finding bugs and resolving them. This article on How to handle multiple windows in Selenium gives you an idea about how you can handle multiple windows while testing an application.
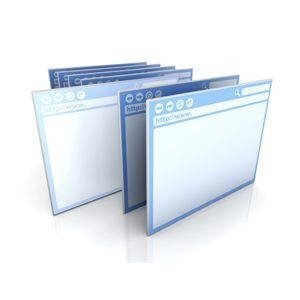
Let’s take the example of Login page of Facebook Application.
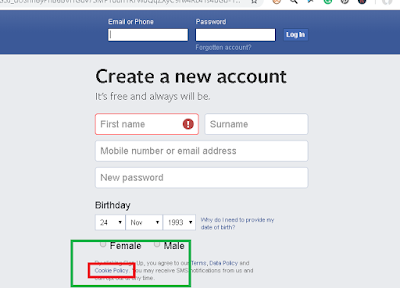
There is one link ‘Cookie Policy’ as highlighted in the above image.
If we click on the link, what will happen?
It will open in the new window. That’s mean we have two opened windows now.
This is the concept of multiple windows.
Now the question is, how to go to that second window and perform actions as required while automating the script?
Solution – We need to switch to that second window like we do while handling alert/frames and then we can perform required actions.
There are two methods in Selenium WebDriver to handle multiple windows –
- driver.getWindowHandle()
- It returns the control of current opened window.
Return type – String
- driver.getWindowHandles()
- It returns the control of all the opened windows so that we can iterate over the all the windows.
Return Type – Set<String>
Selenium WebDriver provides one command in order to switch to the expected window
- switchTo().window(“window_name”);
Scenario –
- Open the Login Page of the Facebook application (Main Window).
- Get the control of the currently opened window.
- Click on the “Cookie Policy” link.
- Now get the control of all the opened windows.
- Switch to the second window (Second Window).
- Get the title of the page.
- Switch back to the main window.
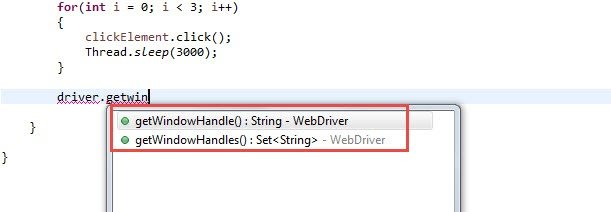
To uniquely identify an opened browser Selenium WebDriver keeps a map of Opened windows VS Window Handle. Window handle is a unique string value that uniquely identifies a Browser window on desktop. It is guaranteed that each browser will have a unique window handle. To get Window handle WebDriver interface provides two methods
- getWindowHandle() – getWindowHandles()
getWindowHandle() method return a string value and it returns the Window handle of current focused browser window. getWindowHandles() method returns a set of all Window handles of all the browsers that were opened in the session. In this case it will return 4 windows handles because we have 4 windows open. Here is the code to print out window handles on console of eclipse.
- public static void main(String[] args) throws InterruptedException
- {
- WebDriver driver = new FirefoxDriver();
- driver.get(“https://toolsqa.com/automation-practice-switch-windows/”);
- String parentWindowHandle = driver.getWindowHandle();
- System.out.println(“Parent window’s handle -> ” + parentWindowHandle);
- WebElement clickElement = driver.findElement(By.id(“button1”));
- for(int i = 0; i < 3; i++)
- {
- clickElement.click();
- Thread.sleep(3000);
- }
- Set<String> allWindowHandles = driver.getWindowHandles();
- for(String handle : allWindowHandles)
- {
- System.out.println(“Window handle – > ” + handle);
- }
- }
- }
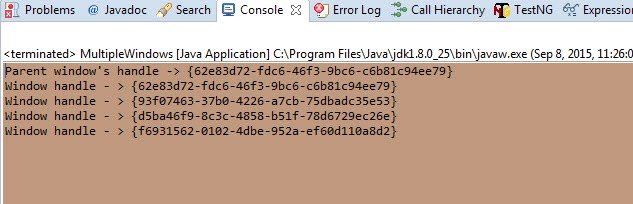
Console Output : This is the output that you will get on console. Pay attention to the Window handle values, they are unique to each other
Handling Multiple Windows in Selenium WebDriver
There is a concept of current focused window which means that all selenium webdriver commands will go to the focused window. By default the focus is always on the Parent window, please see the screenshot above. In order to shift focus from Parent Window to any child window we have to use the following command on WebDriver – WebDriver.SwitchTo().window(String windowHandle); This command takes in a window handle and switches the driver context on that window. Once the Switch happens all the driver commands will go to the newly focused window. This is very important to understand, without switching to the desired window we wil not be able to perform any action on that window. Now lets see some code which iteratively moves across all the open windows and navigates to a particular page in all the open windows one by one.
- public static void main(String[] args) throws InterruptedException
- {
- WebDriver driver = new FirefoxDriver();
- driver.get(“https://toolsqa.com/automation-practice-switch-windows/”);
- String parentWindowHandle = driver.getWindowHandle();
- System.out.println(“Parent window’s handle -> ” + parentWindowHandle);
- WebElement clickElement = driver.findElement(By.id(“button1”));
- for(int i = 0; i < 3; i++)
- {
- clickElement.click();
- Thread.sleep(3000);
- }
- Set<String> allWindowHandles = driver.getWindowHandles();
- for(String handle : allWindowHandles)
- {
- System.out.println(“Switching to window – > ” + handle);
- System.out.println(“Navigating to google.com”);
- driver.switchTo().window(handle); //Switch to the desired window first and then execute commands using driver
- driver.get(“https://google.com”);
- }
- }
Closing all the Windows
There are basically two commands that we can use to close the opened browser windows. – WebDriver.close() – WebDriver.quit() WebDriver.Close() command will close the current window on which the focus is present. This can be used to close windows selectively. Just switch to the window that you want to close by using the correct Window handle and the call the WebDriver.close command. That will close the current browser window. Note: After closing a window you have to explicity switch to another valid window before sending in any WebDriver commands. if you fail to do this you wil get following exception. org.openqa.selenium.NoSuchWindowException: Window not found. The browser window may have been closed. In the code below we will close the parent window and then explicitly move focus to the last window in the list.
- public static void main(String[] args) throws InterruptedException
- {
- WebDriver driver = new FirefoxDriver();
- driver.get(“https://toolsqa.com/automation-practice-switch-windows/”);
- String parentWindowHandle = driver.getWindowHandle();
- System.out.println(“Parent window’s handle -> ” + parentWindowHandle);
- WebElement clickElement = driver.findElement(By.id(“button1”));
- for(int i = 0; i < 3; i++)
- {
- clickElement.click();
- Thread.sleep(3000);
- }
- Set<String> allWindowHandles = driver.getWindowHandles();
- String lastWindowHandle = “”;
- for(String handle : allWindowHandles)
- {
- System.out.println(“Switching to window – > ” + handle);
- System.out.println(“Navigating to google.com”);
- driver.switchTo().window(handle); //Switch to the desired window first and then execute commands using driver
- driver.get(“https://google.com”);
- lastWindowHandle = handle;
- }
- //Switch to the parent window
- driver.switchTo().window(parentWindowHandle);
- //close the parent window
- driver.close();
- //at this point there is no focused window, we have to explicitly switch back to some window.
- driver.switchTo().window(lastWindowHandle);
- driver.get(“https://toolsqa.com”);
- }
WebDriver.quit() will close all the windows opened in the session. This command basically shuts down the driver instance and any further commands to WebDriver results in exception.That’s pretty much it that we have on handling multiple windows. Do let your comments flow in.
Now with this, we come to an end to this “How to handle multiple windows in Selenium” blog. I Hope you guys enjoyed this article and understood what is Selenium Webdriver and also understood how the window handle function helps in switching between the windows.