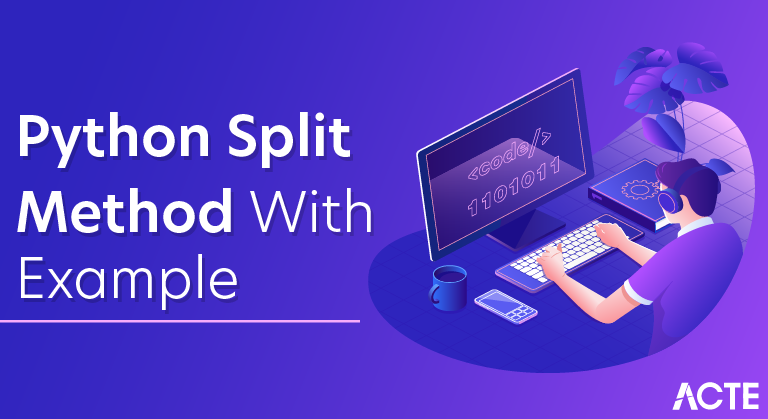
Description
Python string method split() returns a list of all the words in the string, using str as the separator (splits on all whitespace if left unspecified), optionally limiting the number of splits to num.
Syntax
Following is the syntax for split() method −
- str.split(str=””, num=string.count(str)).
Parameters
- str − This is any delimiter, by default it is space.
- num − this is number of lines minus one
Return Value
This method returns a list of lines.
Example
The following example shows the usage of split() method.
- #!/usr/bin/python
- str = “Line1-abcdef \nLine2-abc \nLine4-abcd”;
- print str.split( )
- print str.split(‘ ‘, 1 )
- When we run above program, it produces following result −
- [‘Line1-abcdef’, ‘Line2-abc’, ‘Line4-abcd’]
- [‘Line1-abcdef’, ‘\nLine2-abc \nLine4-abcd’]
How Python split() works
String variables are useful tools for any Python programmer. They can contain numeric or alphanumeric information and are commonly used to store data directories or print messages.
- The .split() Python function is a commonly-used string manipulation tool.
- If you’ve already tried joining two strings in Python by concatenation, then split() does the exact opposite of that.
- It scans through a string and separates it whenever the script comes across a pre-specified separator. It operates as follows using two parameters:
Example Copy
- str.split(separator, maxsplit)
- The separator parameter tells Python where to break the string. You don’t, in fact, always need to use it. If no delimiter is set, Python will use any whitespace in the string as a separation point instead.
- maxsplit defines how many times the string can be broken up. If you don’t add any value here, Python will scan the entire string length and separate it whenever a delimiter is found.
Examples of using split() in Python
One of the easiest examples of how to divide Python strings with split() is to assign no parameters and break down a string of text into individual words:
Example Copy
- text = ‘The quick brown fox jumps over the lazy dog’
- # Split the text wherever there’s a space.
- words = text.split()
- print(words)
- The code above produces the following output:
- Example Copy
- [‘The’, ‘quick’, ‘brown’, ‘fox’, ‘jumps’, ‘over’, ‘the’, ‘lazy’, ‘dog’]
The Python split() function can extract multiple pieces of information from an individual string and assign each to a separate variable. The following example presents a paragraph and turns each sentence into a variable:
Example Copy
paragraph = ‘The quick brown fox jumps over the lazy dog. The quick brown dog jumps over the lazy fox’
- # Split the text wherever there’s a full stop.
- a,b = paragraph.split(‘.’)
- # Display the results.
- print(a)
- print(b)
Theory is great, but we recommend digging deeper!
Example:
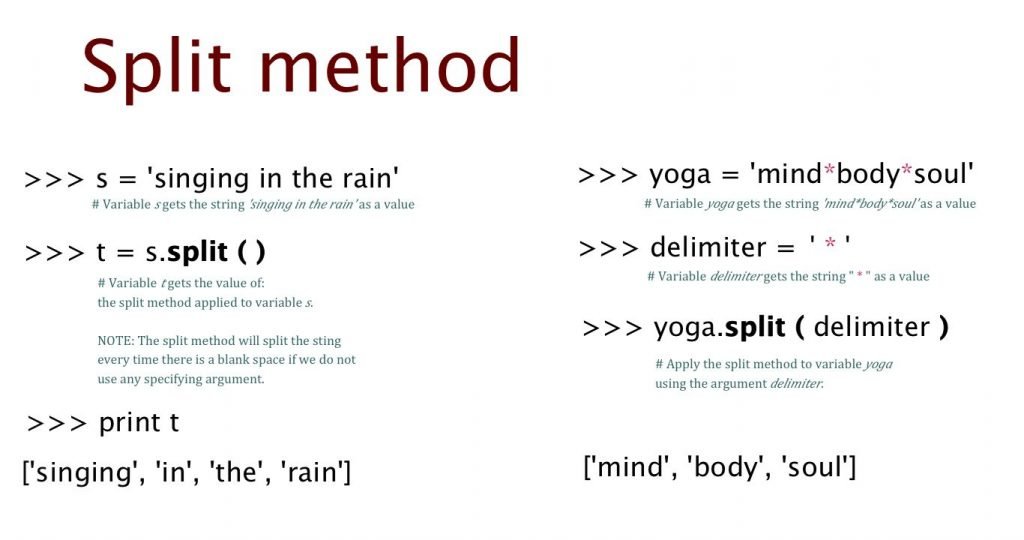
AI in Stock Market Predictions: Learn How to Use TensorFlow and Python
How maxsplit affects string splitting in Python
Don’t forget to use the maxsplit parameter if you only want to break up the first part of a string with the split() Python operator:
Example Copy
- cars= ‘Audi and Kia and BMV and Volvo and Opel’
- # maxsplit of 1
- print(cars.split(‘ and ‘,1))
- # maxsplit of 2
- print(cars.split(‘ and ‘,2))
- # maxsplit of 1
- print(cars.split(‘ and ‘,3))
- # maxsplit of 1
- print(cars.split(‘ and ‘,4))
The output of the code snippet above will be:
Example Copy
- [‘Audi’, ‘Kia and BMV and Volvo and Opel’]
- [‘Audi’, ‘Kia’, ‘BMV and Volvo and Opel’]
- [‘Audi’, ‘Kia’, ‘BMV’, ‘Volvo and Opel’]
- [‘Audi’, ‘Kia’, ‘BMV’, ‘Volvo’, ‘Opel’]
Python split(): useful tips
- If you do specify maxsplit and there are an adequate number of delimiting pieces of text in the string, the output will have a length of maxsplit+1.
- Recombining a string that has already been split in Python can be done via string concatenation.
- Python split() only works on string variables. If you encounter problems with split(), it may be because you are trying to call it upon a non-string object. If this happens, you can force Python to treat the variable as a string with str(x).