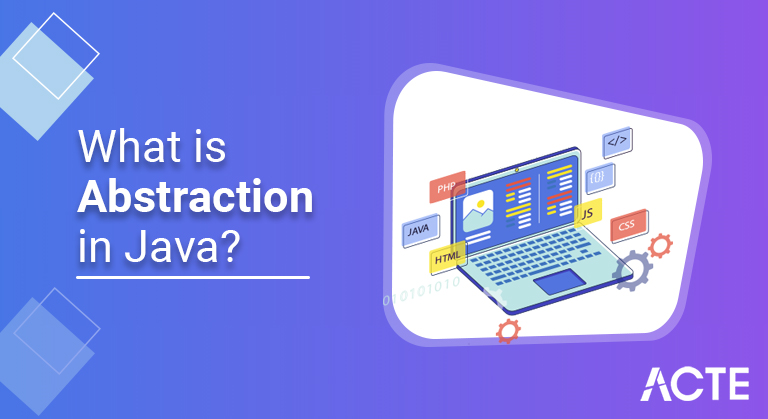
What is Abstraction in Java | Implementations of Abstraction in Java | A Definitive Guide with Best Practices
Last updated on 30th Dec 2021, Blog, General
In Java, Data Abstraction is defined as the process of reducing the object to its essence so that only the necessary characteristics are exposed to the users. Abstraction defines an object in terms of its properties (attributes), behavior (methods), and interfaces (means of communicating with other objects).
- Abstraction in Java
- Real-life Example for Java Abstraction
- How to Achieve Abstraction in Java
- Syntax of declaring an abstract class
- Abstract Methods in Java
- Why do we need Abstract Classes in Java?
- Why can’t we create an object of an Abstract Class?
- Types of Abstraction in Java
- Conclusion
Abstraction in Java:
Abstraction is a process of highlighting all the necessary details and hiding the rest. In Java, data abstraction is defined as the process of reducing an object to its essence so as to expose only the essential features to users. Abstraction defines an object in terms of its properties (attributes), behaviour (methods), and interfaces (means of communication with other objects).
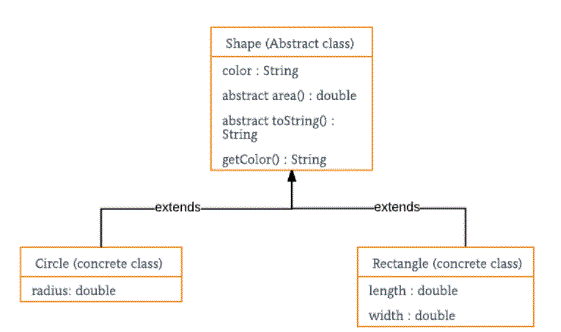
- If we want to process something from the real world, we have to extract the essential characteristics of that object. Consider the example shown in the figure below.
- Here, you can see if an owner is interested in details like car details, service history, etc.; Garage personnel are interested in details like licence, job description, bill, owner, etc.; And the registration office is interested in the details like vehicle identification number, current owner, licence plate etc. This means that each application identifies the details that are important to it.
- Abstraction can be seen as a technique of filtering out unnecessary details of an object so that only the useful features that define it remain. Focuses on the perceived behaviour of the abstract entity. It provides an outside view of the unit.
Real-life Example for Java Abstraction:
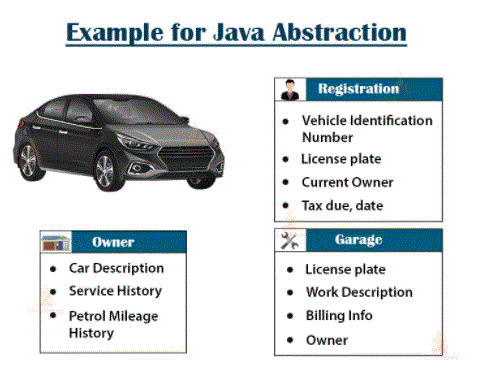
- If a class has at least one abstract method, it must be declared abstract.
- An abstract class does not allow you to create objects of its type. In this case, we can only access the objects of its subclasses.
- By using an abstract class, we can achieve 0 to 100% abstraction.
- An abstract class always has a default constructor, it can also have a parameterized constructor.
- Abstract class can also have final and static methods.
How to Achieve Abstraction in Java:
In Java, we can achieve data abstraction using abstract classes and interfaces. Interfaces allow 100% abstraction (full abstraction). Interfaces allow you to completely abstract the implementation.
Abstract classes allow 0 to 100% abstraction (partial to full abstraction) because abstract classes can have concrete methods that have an implementation that results in partial abstraction.
Abstract classes in java:
An abstract class is a class whose objects cannot be created. An abstract class is created using the abstract keyword. It is used to represent a concept. An abstract class can contain abstract methods (methods without body) as well as non-abstract methods or concrete methods (methods with body). A non-abstract class cannot have abstract methods.
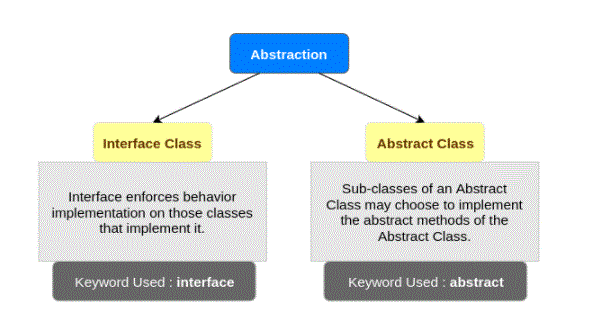
- abstract class ClassName
- ,
- // class body
- ,
Syntax of declaring an abstract class:
Syntax to declare an abstract class:
To declare an abstract class we use the keyword abstract. The syntax is given below:
- Abstract methods are methods that have no implementation and are without a method body. There is no method statement in them.
- An abstract method is declared with the abstract keyword.
- An abstract method declaration must end with a semicolon;
- The child classes that inherit the abstract class must provide the implementation of these inherited abstract methods.
- access-specifier abstract return-type method-name();
- Example of abstract class and abstract methods
- package com.techvidvan.abstraction;
- // parent class
- abstract class base class
- ,
- // abstract method
- abstract public void show1();
- // concrete method
- public void show 2()
- ,
- System.out.println(“Concrete method of parent class”);
- ,
- ,
- // child class
- Class ChildClass extends BaseClass
- ,
- // This method must be overridden when extending the parent class
- public void show 1()
- ,
- System.out.println(“Overriding abstract method of parent class”);
- ,
- // It is not mandatory to override the concrete method
- public voidshow2()
- ,
- System.out.println(“Overriding concrete method of parent class”);
- ,
- ,
- public class abstraction demo
- ,
- public static void main (String [] args)
- ,
- /* We cannot create object of parent class so we are creating object of child class */
- ChildClass obj = new ChildClass();
- obj.show1();
- obj.show2();
- ,
- ,
- Overriding abstract method of parent class
- Overriding concrete method of parent class
Abstract Methods in Java:
Syntax for declaring abstract methods:
Output:
- To answer this question, let’s take a situation where we want to create a class that declares a general form or structure or guidelines without any special considerations, without giving a complete implementation of each method.
- And, we want this generalised form or structure of the class to be used by all its child classes, and the child classes will enforce these guidelines by fulfilling all the implementation details as per the requirement.
- There is no need to implement the method in the parent class, so we can declare these methods as abstract in the parent class. That is, we will not provide any method bodies or implementations of these abstract methods.
- Making these methods abstract will force all derived classes to implement these abstract methods otherwise, there will be a compilation error in your code.
Why do we need Abstract Classes in Java?
If we cannot create or use any object from abstract classes, then what is the need of abstract classes?
- package com.techvidvan.abstraction;
- abstract square geometric shape
- String nameOfShape;
- // abstract methods
- abstract double enumeration field();
- public abstract String toString();
- // constructor
- public Geometric Shape(String nameOfShape)
- ,
- System.out.println(“Inside constructor of Geometric Shape class”);
- this.nameOfShape = nameOfShape;
- ,
- // non-abstract method
- public String getNameOfShape()
- ,
- return nameOfShape;
- ,
- ,
- class circle extends geometric shape
- ,
- double radius;
- public Circle(String nameOfShape, double radius)
- ,
- super (nameOfShape);
- System.out.println(“Inside constructor of Circle class”);
- this radius = radius;
- ,
- // implement methods
- @ override
- double calculate area()
- ,
- return Math.PI * Math.pow(radius, 2);
- ,
- @ override
- public String toString()
- ,
- return “shape name is ” + super.nameOfShape +
- “and its area is: ” + calculateArea();
- ,
- ,
- square square increases geometric shape
- ,
- double length;
- public class(String nameOfShape, double length)
- ,
- //calling shape constructor
- super (nameOfShape);
- System.out.println(“Inside constructor of Square class”);
- this length = length;
- ,
- // implement methods
- @ override
- double calculate area()
- ,
- return length * length;
- ,
- @ override
- public String toString()
- ,
- return “shape name is ” + super.nameOfShape +
- “and its area is: ” + calculateArea();
- ,
- ,
- public class abstraction demo
- ,
- public static void main (String [] args)
- ,
- Geometric Shapes shapeObject1 = new Circle(“Circle”, 6.5);
- System.out.println(shapeObject1.toString());
- Geometric Shape ShapeObject2 = new Square(“Rectangle”, 8);
- System.out.println(shapeObject2.toString());
- ,
- ,
- Inside the constructor of the Geometric Shapes class
- Inside the Circle class’s constructor
- The name of the figure is circle and its area is: 132.73228961416876
- Inside the constructor of the Geometric Shapes class
- Inside the constructor of the Square class
- The name of the figure is rectangle and its area is: 64.0
Code to understand the concept of Abstraction in Java:
Output:
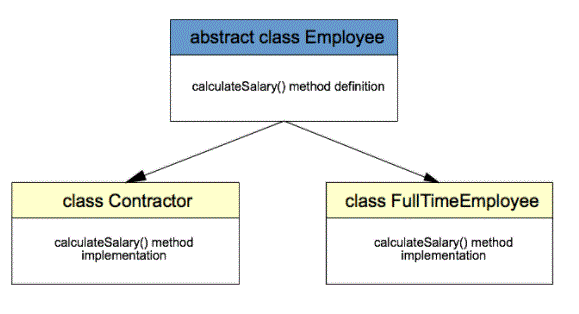
- Code to tell that object creation of an abstract class is invalid:
- As discussed above, we cannot instantiate an abstract class. This program throws a compilation error.
- package com.techvidvan.abstraction;
- // parent class
- abstract class
- ,
- // abstract method
- abstract public void show Details();
- ,
- // child class
- Class ChildClass extends ParentClass
- ,
- public void showDetails()
- ,
- System.out.print(“Overriding abstract method of parent class”);
- ,
- ,
- public class abstraction demo
- ,
- public static void main (String [] args[])
- ,
- // Error: You cannot create an object of an abstract class
- ParentClass obj = new ParentClass();
- obj.showDetails();
- ,
- ,
Why can’t we create an object of an Abstract Class?
You must be thinking that why can’t we instantiate an abstract class or create an object from it? Suppose, if java allows you to create an abstract class and use this object. Now what if someone calls the abstract method through an object of the abstract class? There will be no actual implementation of the called method.
Also, an abstract class is like a guideline or template which has to be extended by its child classes for implementation.
Output:
Exception in thread “main” java.lang.Error: Unresolved compilation problem:Cannot instantiate type ParentClass
- It reduces the complexity of looking things up.
- Increases software reuse and avoids code duplication: loosely-coupled classes often prove useful in other contexts.
- It helps in enhancing the security and privacy of an application as only essential details are exposed to the user.
- Reduces maintenance burden – classes can be understood and debugged more quickly without fear of harming other modules.
- Data Encapsulation vs Data Abstraction in Java
- Encapsulation is one step ahead of abstraction.
- Encapsulation binds data members and methods together whereas data abstraction deals with showing external details of an entity to the user and hiding its implementation details.
- Abstraction provides access to a specific part of the data while encapsulation hides the data.
Types of Abstraction in Java:
1. Data Abstraction
Data abstraction is the most common type of abstraction in which we create complex data types such as HashMap or HashSet, and hide its implementation details from users and display only meaningful operations to interact with the data type. The advantage of this approach is to address performance issues and improve implementation over time. Any changes that occur while improving performance are not reflected on the code that is on the client-side.
2. Control Abstraction
Control abstraction is the process of determining all such statements that are similar and repeated multiple times and exposing them as a single unit of work. We generally use this type of abstraction when we want to create a function to perform a given task.
Advantages of Abstraction in Java:
- In Java, you cannot create an object from an abstract class using the new operator. If you try to instantiate an abstract class, it will give a compilation error.
- An abstract class can have a constructor.
- This can include both abstract and concrete methods. An abstract method has only the declaration but no method body.
- In Java, only classes or methods can be declared as abstract, we cannot declare a variable as abstract.
- We use abstract keywords to declare both class and method as abstract.
- If we declare any method as abstract, the class automatically needs to become an abstract class.
- Through data abstraction we can reduce the real world entity to its essential defined features and show only what is required by the users. The concept of data abstraction is completely based on abstract classes in Java.
- Here we come to the end of our Java article. Now let’s see what we have learned. In this article, we have discussed the importance of abstraction in Java with real life examples.
- We have covered the detailed description of abstract classes and abstract methods in Java along with their syntax and examples. Now, you must have understood the importance of abstraction in OOP and also understand how to implement it in your code.
Conclusion: