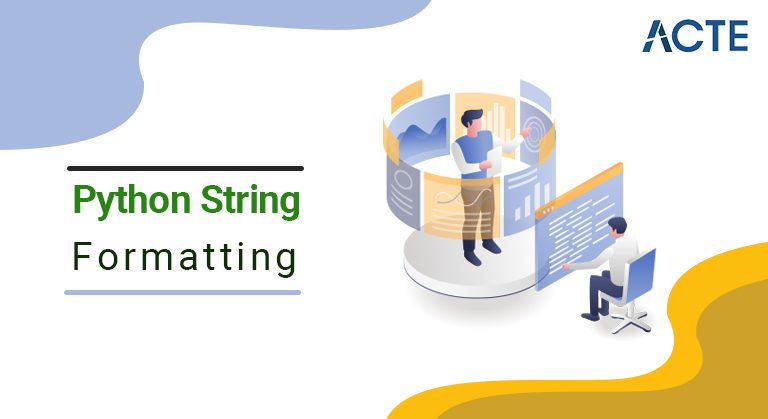
- I am using the %s format specifier to tell Python where to replace the value of name, which is represented as a string.
- There are other format specifiers available that let you control the output format. For example, it is possible to convert numbers to hexadecimal notation or add whitespace padding to produce well-formatted tables and reports. (See the Python docs: “printf-style string formatting”.)
- Here, you can use the %x format specifier to convert an int value to a string and represent it as a hexadecimal number:
- >>> ‘%x’% error
- ‘Bad Coffee’
- >>> ‘Hey %s, 0x%x is an error!’ % (name, error)
- ‘Hey Bob, there’s an error 0xc0ffee!’
- If you pass a mapping to the % operator, it is also possible to refer to the variable replacement by name in your format string:
- >>> ‘Hey %(name)s, 0x%(errno)x is an error!’ ,
- … “name”: name, “err”: error}
“Old Style” String Formatting (% Operator):
Strings in Python have a unique built-in operation that can be accessed with the % operator. It lets you do simple positional formatting very easily. If you’ve ever worked with a printf-style function in C, you’ll recognize how it works immediately. Here’s a simple example:
>>> ‘Hello, %s’% name
“Hello, Bob”
The “old-style” string formatting syntax changes a bit if you want to do multiple replacements in a single string. Since the % operator only takes one argument, you need to wrap the right hand side in a tuple, like this:
‘Hey Bob, there’s an error 0xc0ffee!’
This makes your format strings easier to maintain and easier to modify in the future. You don’t have to worry about making sure that the order you’re passing in the values matches the order in which the values are referenced in the format string. Of course, the downside is that this technique requires a bit more typing.
I’m sure you must be wondering why this printf-style formatting is called “old-style” string formatting. This was technically superseded by the “new style” formatting in Python 3, which we’re going to talk about next.
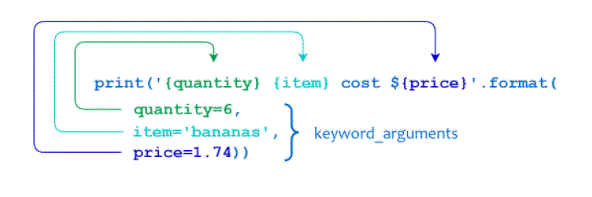
- >>> ‘Hello, {}’. format (name)
- ‘Hello, Bob’
- Or, you can refer to your variable replacements by name and use them in the order you want. This is quite a powerful feature as it allows to rearrange the order of the display without changing the arguments passed to format():
- >>> ‘Hey {name}, there is a 0x{errno:x} error!’.format(
- … name = name, error = error)
- ‘Hey Bob, there’s an error 0xc0ffee!’
- This also suggests that the syntax for formatting an int variable as a hexadecimal string has changed. Now you have to pass a format spec by adding the 😡 suffix. Format string syntax has become more powerful without complicating simple use cases. It pays to read this string formatting mini-language in the Python documentation.
- In Python 3, this “new-style” string formatting is to take precedence over %-style formatting. While “old-style” formatting is not emphasised, it is not deprecated. It is still supported in the latest versions of Python. According to this discussion on the Python Dev Email List and this issue on the Python Dev Bug Tracker, %-formatting is going to be around for a long time to come.
- Still, the official Python 3 documentation doesn’t recommend “old-style” formatting at all or speak very lovingly of it: “The formatting operations described here exhibit a variety of quirks that lead to a number of common errors (such as failing to represent tuples and dictionaries correctly).” helps to avoid these errors.These options also provide more powerful, flexible and extensible.
“New Style” String Formatting (str.format):
Python 3 introduced a new way of formatting strings that was later ported back to Python 2.7. This “new style” string formatting gets rid of the %-operator special syntax and makes the syntax for string formatting more regular. Formatting is now controlled by calling .format() on a String object. You can use format() to do simple positional formatting, just like you can with “old-style” formatting:
Approach to text formatting. ” (Source)
This is why I personally try to stick with str.format for new code going forward. Starting with Python 3.6, there is another way to format your strings. I’ll tell you about it in the next section.- >>> Hello, {name}!’
- ‘Hello, Bob!’
- As you can see, it prefixes string constants with the letter “f” – hence the name “off-strings”. This new formatting syntax is powerful. Since you can embed arbitrary Python expressions, you can also do inline arithmetic with it. Look at this example:
- >>> a = 5
- >>> b = 10
- >>> f’five plus ten is {a + b} and not {2 * (a + b)}.’
- ‘Five plus ten is 15 and not 30.’
- Formatted string literals is a Python parser utility that converts f-strings into a series of string constants and expressions. They are then joined to form the final string.
- >>> define salutation (name, question):
- … return f”Hello, {name}! How is it {question}?”
- >>> Greeting(‘Bob’, ‘Going’)
- “Hello, Bob! How’s it going?”
- When you disassemble the function and observe what’s going on behind the scenes, you’ll see that the f-string in the function turns into something similar to the following:
- >>> def salutation (name, question):
- … return “Hello,” + name + “! How is it” + question + “?”
- The actual implementation is slightly faster than that because it uses the BUILD_STRING opcode as an optimization. But functionally they are the same:
- >>> Import Department
- >>> dis.dis(hello)
- 2 0 LOAD_CONST 1 (‘Hello,’)
- 2 LOAD_FAST 0 (name)
- 4 FORMAT_VALUE 0
- 6 LOAD_CONST 2(“!How is it”)
- 8 LOAD_FAST 1 (QUESTION)
- 10 FORMAT_VALUE 0
- 12 LOAD_CONST 3 (‘?’)
- 14 BUILD_STRING 5
- 16 RETURN_VALUE
- >>> f”Hey {name}, there is an {error:#x} error!”
- “Hey Bob, there’s an error 0xc0ffee!”
3 String Interpolation / f-Strings (Python 3.6+):
Python 3.6 added a new string formatting approach called formatted string literals or “f-strings”. This new way of formatting strings lets you use Python expressions embedded inside string constants. Here’s a simple example to give you a feel for the convenience:
Imagine you have the following greet() function that contains an f-string:
String literals also support the current format string syntax of the str.format() method. This allows you to solve the same formatting problems we discussed in the last two sections.
Python’s newly formatted string literals are similar to JavaScript’s template literals added in ES2015. I think they are good enough for Python, and I have started using them in my day to day (Python 3) work. You can learn more about formatted string literals in our in-depth Python f-string tutorial.
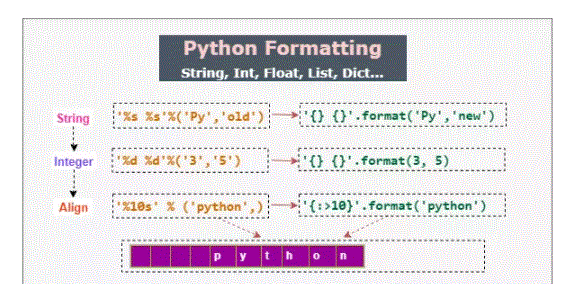
- Let’s look at a simple greeting example:
- >>> from string import template
- >>> t = template(‘Hey, $name!’)
- >>> t.substitute(name = name)
- ‘Hey, Bob!’
- >>> templ_string = ‘Hey $name, there is a $error error!’
- >>> template(templ_string). the substitute (
- … name = name, error = hex(err))
- ‘Hey Bob, there’s an error 0xc0ffee!’
- It worked great.
- >>> # This is our super secret key:
- >>> secret = ‘it’s a secret’
- >>> class error:
- … def __init__(self):
- … passed
- >>> # A malicious user can generate a format string that
- >>> # Can read data from global namespace:
- >>> user_input = ‘{error.__init__.__globals__[secret]}’
- >>> # This allows them to take out sensitive information,
- >>> # like secret key:
- >>> error = error()
- >>> user_input.format(error = error)
- ‘This is a secret’
- See how a hypothetical attacker was able to extract our secret string from the malicious format string by accessing the __globals__ dictionary? scary, huh? Template strings set off this attack vector. This makes them a safer option if you’re handling format strings generated from user input:
- >>> user_input = ‘${error.__init__.__globals__[SECRET]}’
- >>> template(user_input). substitute(error = error)
- value error:
- “Invalid placeholder in string: line 1, column 1”
Template Strings (Standard Library):
Here’s another tool for string formatting in Python: Template Strings. It’s a simpler and less powerful mechanism, but in some cases it might be what you’re looking for.
You see here that we need to import the template class from Python’s built-in string module. Template strings are not a core language feature, but they are supplied by the String module in the standard library. Another difference is that template strings do not allow format specifiers. So to get the previous error string example to work, you’ll need to manually convert the int error number to a hex-string:
So when should you use template strings in your Python programs? In my opinion, the best time to use template strings is when you are handling formatted strings generated by users of your program. Because of their low complexity, template strings are a safer choice.
The more complex formatting mini-languages of other string formatting techniques can introduce security vulnerabilities to your programs. For example, it is possible to use arbitrary variables in your program for format strings. This means, if a malicious user can supply the format string, they can potentially leak the secret key and other sensitive information! Here’s a simple proof of concept of how this attack can be used against your code:
Which String Formatting Method Should You Use:
I totally think having so many options to format your strings in Python can feel very confusing. This handy flowchart is an excellent pointers to bust infographic that I’ve put together for you:
Python string formatting flowchart:
Python String Formatting Rule of Thumb This flowchart is based on a rule of thumb that I apply when writing Python:
Python String Formatting Rule of Thumb: If your format strings are user-supplied, use template strings (#4) to avoid security issues. Otherwise, if you’re on Python 3.6+, use literal string interpolation/f-strings (#3), and the “new-style” str.format (#2) if you’re not.
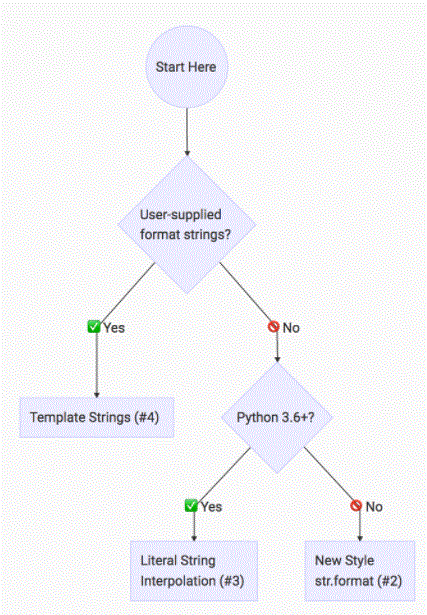
- syntax
- string.format(value1, value2…)
- parameter value
- parameter description
- Value1, Value2… Required. One or more values that must be formatted and inserted into the string.
- The value can be of any data type.
- placeholder
- Placeholders can be identified using named index {price}, numbered index {0}, or even an empty placeholder {}.
- Example
- Using different placeholder values:
- txt1 = “My name is {fname}, I am {age}”. format(fname = “john”, age = 36)
- txt2 = “My name is {0}, I am {1}”. format(“John”, 36)
- txt3 = “My name is {}, I am {}”. format(“John”, 36)
Definition and Usage:
The format() method formats the specified value(s) and inserts them inside a string’s placeholder. Placeholders are defined using curly brackets: {}. Read more about placeholders in the Placeholders section below. The format() method returns a formatted string.
A value is either a comma-separated list of values, a key=value list, or a combination of both.
- :< Left aligns the result (within the available space)
- :> Right aligns the result (within the available space)
- :^ Centre aligns the result (within the available space)
- := puts the symbol on the far left
- :+ Use a plus sign to indicate whether the result is positive or negative
- :- For negative values use negative sign only
- : Use a space to put an extra space before positive numbers (and a minus sign before negative numbers)
- :, Use a comma as a thousand separator
- :_ Use an underscore as a thousand separator
- :b binary format
- :c Converts the value to the corresponding Unicode character
- :d decimal format
- :e Scientific format, lowercase e . with
- :e scientific format, uppercase e . with
- :f fix point number format
- :F Fixed point number format, in uppercase format (show inf and nan as INF and NAN)
- :g normal format
- :G Common format (using capital letter E for scientific notation)
- 😮 octal format
- 😡 hex format, lower case
- :X hex format, upper case
- :n number format
- :% percent format
Formatting Types:
Inside placeholders you can add a formatting type to format the result:
- Here, instead of just a parameter, we have used a key-value for the parameter. Namely, name = “Adam” and blc = 230.2346.
- Since these parameters are referred to by their keys as {name} and {blc:9.3f}, they are known as keywords or named arguments. internally,
- The placeholder {name} is replaced by the value of the name – “Adam”. Since it does not contain any other format codes, “Adam” is placed.
- For the argument blc=230.2346, the placeholder {blc:9.3f} is replaced with the value 230.2346. But before replacing it, as in the previous example, 9.3f operates on it.
- It outputs 230.235. The decimal part is truncated after 3 places and the remaining digits are rounded off. Similarly, leaving two spaces on the left is assigned a total width of 9.
- number formatting type
- type meaning
- d decimal integer
- C compliant unicode characters
- B. Binary Format
- o octal format
- x hexadecimal format (lower case)
- X hexadecimal format (upper case)
- Similar to n’ d’. except that it uses the current locale setting for the number separator
- E exponential notation. (lowercase e)
- e exponential notation (uppercase e)
- f Displays the fixed point number (default: 6)
- Similar to f’f’. In addition to displaying ‘inf’ as ‘INF’ and ‘nan’ as ‘NAN’
- G general format. Integer number to p significant digit. (default precision: 6)
- Similar to ‘G’. except switch to ‘e’ when the number is large.
- % Percent. Multiply by 100 and put % at the end.
For keyword arguments:
We have used the same example from above to show the difference between keywords and positional arguments.
Formatting numbers with format()
You can format numbers using the below format specifiers:
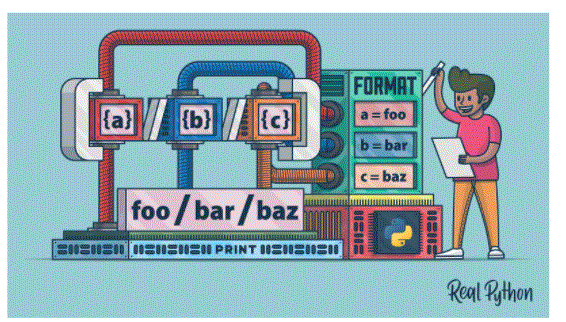
- Use operators with strings
- Access and remove parts of strings
- Use built-in Python functions with characters and strings
- Use methods to manipulate and modify string data
- You were also introduced to two other Python objects used to represent raw byte data: bytes and bytearray types.
- String formatting is a very important function of any type of programming language. It helps the user to understand the output of the script properly. String formatting in Python can be done in various ways, such as using the ‘%’ symbol, format() method, string interpolation, etc.
- This article describes Python’s string formatting methods. New Python programmers will be able to perform string formatting tasks with ease after reading this article
Conclusion:
Learned about working with strings, which are objects that contain sequences of character data. Processing character data is an integral part of programming. It’s a rare application that doesn’t require manipulating strings, at least to some extent. Python provides a rich set of operators, functions, and methods for working with strings. Now you know how to: