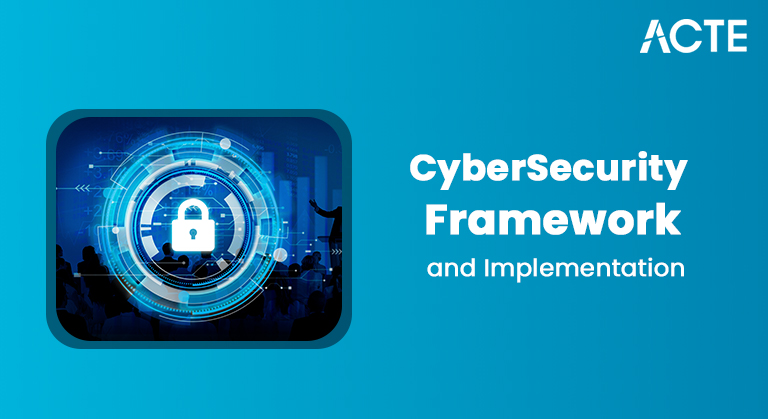
- Introduction
- Understanding State Management in Web Development
- Why State Management Matters in ASP.NET
- Types of State Management in ASP.NET
- Client-Side State Management in ASP.NET
- Server-Side State Management in ASP.NET
- Best Practices for Effective State Management
- Conclusion
Introduction
State management is a vital aspect of web development, especially in ASP.NET applications. Unlike desktop applications, web applications operate in a stateless environment, meaning each HTTP request from a client is processed independently, with no memory of prior interactions. This poses a challenge when building dynamic, data-driven websites that require persistence of data across multiple user requests. Managing state effectively ensures a seamless user experience and functional consistency. ASP.NET provides several mechanisms for handling state, both on the client and server sides. To build a strong foundation in these areas, consider exploring our Web Designing & Development Courses This blog will explore the various state management techniques available in ASP.NET, such as view state, session state, cookies, and application state. It will also highlight best practices and offer practical guidance on selecting the appropriate method based on your application’s needs. Whether you’re handling user sessions, storing temporary data, or optimizing performance, understanding state management is essential for building efficient and scalable ASP.NET web applications.
Understanding State Management in Web Development
In web applications, “state” refers to the data or information that persists across different requests and sessions. Since HTTP is a stateless protocol, state management is necessary to maintain the continuity of a user’s experience. Effective state management ensures that users do not lose their progress or context as they interact with a website. If you’re starting out in the field, exploring the Best Web Development Languages To Learn can provide a solid foundation for mastering broader concepts like state management. State management techniques in ASP.NET can be broadly categorized into client-side and server-side methods. Understanding the differences between these two approaches is key to mastering ASP.NET state management.
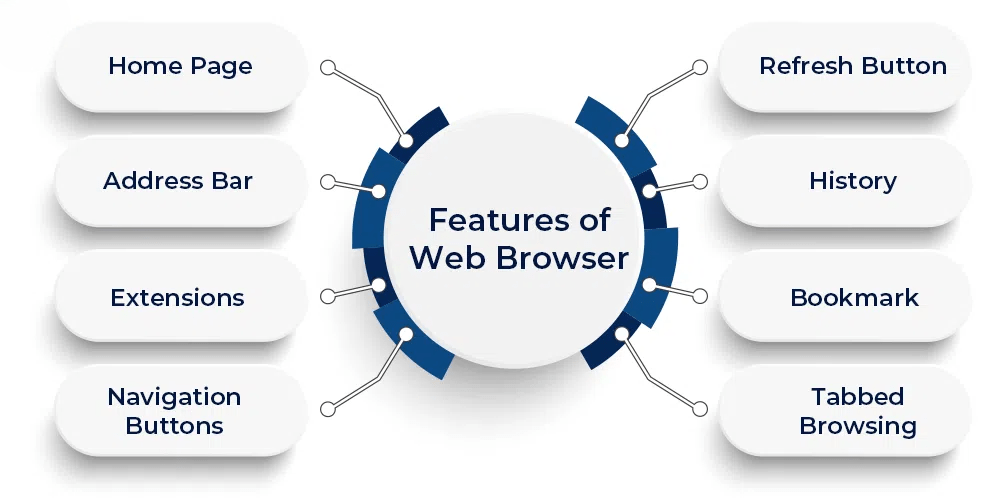
Why State Management Matters in ASP.NET
ASP.NET applications handle user interactions by processing HTTP requests. Without maintaining state, applications would not be able to track user inputs, preferences, authentication status, or session data. For developers looking to strengthen their programming fundamentals, understanding Control Statements in Java can help build logical thinking essential for backend development. Thus, it is imperative to manage the state effectively to ensure the application delivers a smooth, continuous experience to users. Effective state management:
- Enhances user experience by retaining form data, preferences, and login states.
- Reduces server load by leveraging client-side state when appropriate.
- Provides better scalability and performance by choosing the optimal storage location for state data.
Types of State Management in ASP.NET
ASP.NET provides two broad categories for state management: Client-Side State Management and Server-Side State Management. The right choice depends on factors like data security, performance requirements, and scalability.
4.1 Client-Side State ManagementClient-side state management involves storing state data directly on the client’s machine. This reduces the load on the server but also introduces security and data integrity concerns.
4.2 Server-Side State ManagementServer-side state management involves storing state data on the server. While this is more secure, it can increase the load on the server and may impact scalability if not handled properly.
Key methods of server-side state management:- Session State
- Application State
- Cache
- Reduces server load.
- Persistent across sessions (unless deleted by the user). Disadvantages:
- Size limitations (usually 4KB).
- Security concerns (can be tampered with if not encrypted). Example:
- HttpCookie userCookie = new HttpCookie(“UserPreferences”);
- userCookie[“Theme”] = “Dark”;
- userCookie.Expires = DateTime.Now.AddDays(30);
- Response.Cookies.Add(userCookie);
- Easy to implement and use.
- Data remains hidden from the user. Disadvantages:
- Limited in size.
- Can be modified by the client if not validated properly.
- Simple to implement.
- Visible in the URL, which can be useful for bookmarking or sharing URLs with specific states. Disadvantages:
- Exposed to users (can be modified).
- Limited length. Example:
- Response.Redirect(“Profile.aspx?theme=Dark”);
- Data is not exposed to the client.
- Supports complex data types. Disadvantages:
- Consumes server memory.
- Session state may expire, requiring users to log in again. Example:
- Session[“UserTheme”] = “Dark”;
- Data is shared across all users.
- Ideal for storing application-wide settings. Disadvantages:
- Not suitable for user-specific data.
- Consumes server memory and can lead to concurrency issues in multi-threaded environments. Example:
- Application[“AppTheme”] = “Light”;
- Improves application performance.
- Can store complex data (e.g., objects, datasets). Disadvantages:
- Cache expiration can lead to stale data.
- Requires careful management to avoid memory issues. Example:
- Cache[“RecentPosts”] = GetRecentPosts();
Excited to Obtaining Your web developer Certificate? View The web developer course Offered By ACTE Right Now!
Client-Side State Management in ASP.NET
In client-side state management, the state is stored in the client’s browser, reducing the need to interact with the server for every request. To better understand the underlying communication processes, learning about HTTP Request Methods is essential. Below are some of the most common methods of managing state on the client side:
5.1 CookiesCookies are small pieces of data stored in a user’s browser. They are sent to the server with each HTTP request and can be used to store user preferences, authentication tokens, or tracking information.
Advantages:Getting Ready for a Web Developer Job Interview? Check Out Our Blog on Web Developer Interview Questions & Answer
5.2 Hidden FieldsHidden fields are HTML input elements that are not visible to the user but can store data that needs to be passed between requests.
Advantages:Excited to Achieve Your Web developer Certification? View The Web developer course Offered By ACTE Right Now!
5.3 Query StringsQuery strings are part of the URL, and they pass state information from one page to another.
Advantages:Interested in Pursuing Web Developer Master’s Program? Enroll For Web developer course Today!
Server-Side State Management in ASP.NET
Server-side state management involves storing state data on the server, which enhances security but introduces performance and scalability concerns. To deepen your understanding of server-side techniques and best practices, consider enrolling in our Web Developer Certification. There are various methods to manage state on the server.
6.1 Session StateSession state allows you to store data that is specific to a user’s session. Data is stored on the server and is available across multiple requests from the same user.
Advantages:6.2 Application State
Application state is shared across all users of the application. It is typically used for global data that does not change often (e.g., application settings, lookup data).
Advantages:6.3 Cache
Caching allows data to be stored temporarily for faster access. Cache is typically used to store expensive data that doesn’t change frequently, reducing database calls and improving application performance.
Advantages: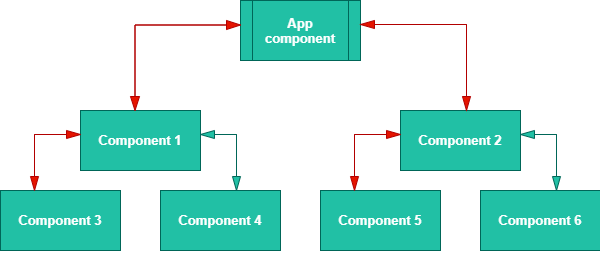
Best Practices for Effective State Management
Effective state management is essential for building scalable and maintainable web applications. To begin with, it’s important to choose the right state management technique. Client-side methods such as cookies and query strings are suitable for scenarios requiring low overhead and non-sensitive data. In contrast, server-side techniques like session or application state are better suited for handling sensitive or large volumes of data. It’s also advisable to minimize the amount of state stored on the server, as excessive storage can hinder scalability. In such cases, using a distributed cache or a database is a more efficient approach. Security is another critical aspect; sensitive data stored in cookies or query strings should always be encrypted, and HTTPS should be used to secure communication. Additionally, session state should be used sparingly, as over-reliance on it can cause performance issues, especially if large objects or collections are stored. If you’re interested in learning more about the field, check out our guide on How to Become a Web Developer. Finally, optimizing cache usage is key to maintaining performance; this includes setting proper cache expiration and being mindful of memory limitations.
Conclusion: Future of Data Integration and Its Impact
Mastering state management in ASP.NET is essential for building efficient, secure, and scalable web applications. Understanding the various state management techniques, both client-side and server-side, enables you to make informed decisions based on your application’s needs. To strengthen your front-end skills alongside state management, consider exploring our Web Designing Training. Whether using cookies for lightweight, client-side storage or session and application states for server-side persistence, effective state management ensures a seamless user experience and optimized performance. By adhering to best practices, such as securing sensitive data and minimizing server-side state storage, you can build robust ASP.NET applications that provide consistent, scalable experiences for your users.