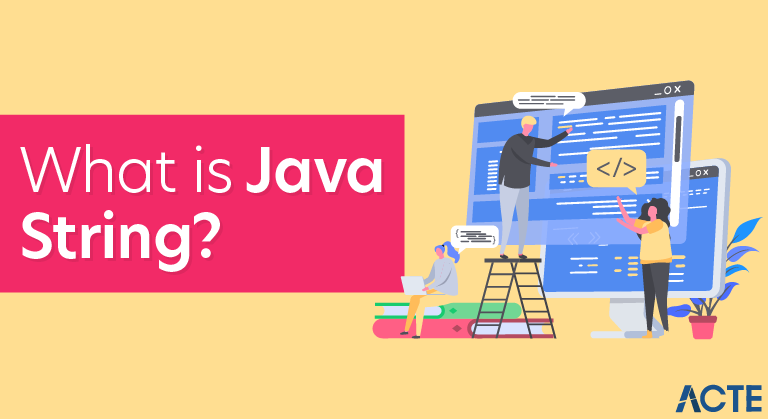
In Java, string is basically an object that represents sequence of char values. An array of characters works same as Java string. For example:
- char[] ch={‘j’,’a’,’v’,’a’,’t’,’p’,’o’,’i’,’n’,’t’};
- String s=new String(ch);
is same as:
- String s=”javatpoint”;
Java String class provides a lot of methods to perform operations on strings such as compare(), concat(), equals(), split(), length(), replace(), compareTo(), intern(), substring() etc.
The java.lang.String class implements Serializable, Comparable and CharSequence interfaces.
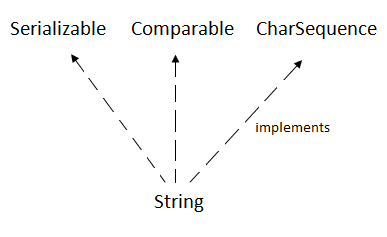
Char Sequence Interface
The CharSequence interface is used to represent the sequence of characters. String, StringBuffer and StringBuilder classes implement it. It means, we can create strings in java by using these three classes.
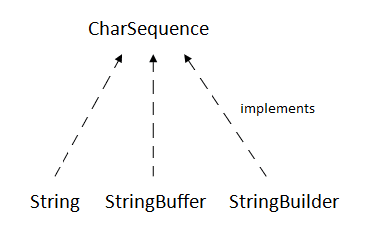
The Java String is immutable which means it cannot be changed. Whenever we change any string, a new instance is created. For mutable strings, you can use StringBuffer and StringBuilder classes.
We will discuss immutable string later. Let’s first understand what is String in Java and how to create the String object.
Different Ways to Create String
There are many ways to create a string object in java, some of the popular ones are given below.
- Using string literal
This is the most common way of creating string. In this case a string literal is enclosed with double quotes. - String str = “abc”;
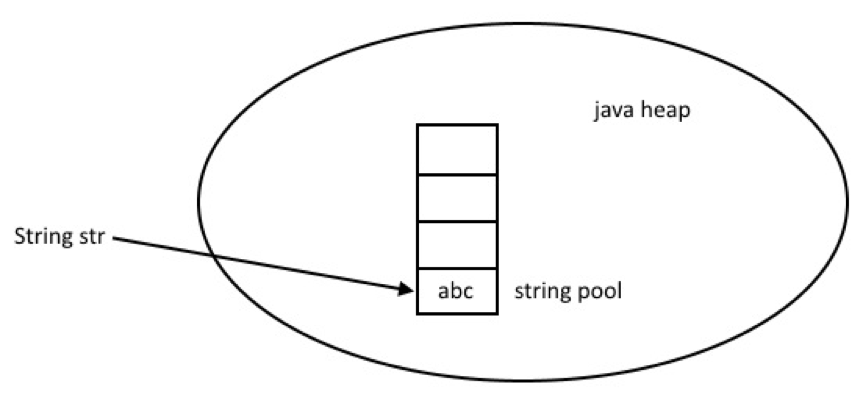
- When we create a String using double quotes, JVM looks in the String pool to find if any other String is stored with same value. If found, it just returns the reference to that String object else it creates a new String object with given value and stores it in the String pool.
- Using new keyword
We can create String object using new operator, just like any normal java class. There are several constructors available in String class to get String from char array, byte array, StringBuffer and StringBuilder. - String str = new String(“abc”); char[] a = {‘a’, ‘b’, ‘c’}; String str2 = new String(a);
- Java String compare
String class provides equals() and equalsIgnoreCase() methods to compare two strings. These methods compare the value of string to check if two strings are equal or not. It returns true if two strings are equal and false if not.
package com.journaldev.string.examples; /** * Java String Example * * @author pankaj * */ public class StringEqualExample { public static void main(String[] args) { //creating two string object String s1 = “abc”; String s2 = “abc”; String s3 = “def”; String s4 = “ABC”; System.out.println(s1.equals(s2));//true System.out.println(s2.equals(s3));//false System.out.println(s1.equals(s4));//false; System.out.println(s1.equalsIgnoreCase(s4));//true } }
Output of above program is:
true false false true
String class implements Comparable interface, which provides compareTo() and compareToIgnoreCase() methods and it compares two strings lexicographically.
Both strings are converted into Unicode value for comparison and return an integer value which can be greater than, less than or equal to zero. If strings are equal then it returns zero or else it returns either greater or less than zero.
package com.journaldev.examples; /** * Java String compareTo Example * * @author pankaj * */ public class StringCompareToExample { public static void main(String[] args) { String a1 = “abc”; String a2 = “abc”; String a3 = “def”; String a4 = “ABC”; System.out.println(a1.compareTo(a2));//0 System.out.println(a2.compareTo(a3));//less than 0 System.out.println(a1.compareTo(a4));//greater than 0 System.out.println(a1.compareToIgnoreCase(a4));//0 } }
Output of above program is:
0 -3 32 0
Java String Methods
Let’s have a look at some of the popular String class methods with an example program.
- split()
Java String split() method is used to split the string using given expression. There are two variants of split() method.- split(String regex): This method splits the string using given regex expression and returns array of string.
- split(String regex, int limit): This method splits the string using given regex expression and return array of string but the element of array is limited by the specified limit. If the specified limit is 2 then the method return an array of size 2.
- package com.journaldev.examples; /** * Java String split example * * @author pankaj * */ public class StringSplitExample { public static void main(String[] args) { String s = “a/b/c/d”; String[] a1 = s.split(“/”); System.out.println(“split string using only regex:”); for (String string : a1) { System.out.println(string); } System.out.println(“split string using regex with limit:”); String[] a2 = s.split(“/”, 2); for (String string : a2) { System.out.println(string); } } }
- Output of above program is:
- split string using only regex: a b c d split string using regex with limit: a b/c/d
- contains(CharSequence s)
Java String contains() methods checks if string contains specified sequence of character or not. This method returns true if string contains specified sequence of character, else returns false. - package com.journaldev.examples; /** * Java String contains() Example * * @author pankaj * */ public class StringContainsExample { public static void main(String[] args) { String s = “Hello World”; System.out.println(s.contains(“W”));//true System.out.println(s.contains(“X”));//false } }
- Output of above program is:
- true false
- length()
Java String length() method returns the length of string. - package com.journaldev.examples; /** * Java String length * * @author pankaj * */ public class StringLengthExample { public static void main(String[] args) { String s1 = “abc”; String s2 = “abcdef”; String s3 = “abcdefghi”; System.out.println(s1.length());//3 System.out.println(s2.length());//6 System.out.println(s3.length());//9 } }
- replace()
Java String replace() method is used to replace a specific part of string with other string. There are four variants of replace() method.- replace(char oldChar, char newChar): This method replace all the occurrence of oldChar with newChar in string.
- replace(CharSequence target, CharSequence replacement): This method replace each target literals with replacement literals in string.
- replaceAll(String regex, String replacement): This method replace all the occurrence of substring matches with specified regex with specified replacement in string.
- replaceFirst(String regex, String replacement): This method replace first occurrence of substring that matches with specified regex with specified replacement in string.
- package com.journaldev.examples; /** * Java String replace * * @author pankaj * */ public class StringReplaceExample { public static void main(String[] args) { //replace(char oldChar, char newChar) String s = “Hello World”; s = s.replace(‘l’, ‘m’); System.out.println(“After Replacing l with m :”); System.out.println(s); //replaceAll(String regex, String replacement) String s1 = “Hello journaldev, Hello pankaj”; s1 = s1.replaceAll(“Hello”, “Hi”); System.out.println(“After Replacing :”); System.out.println(s1); //replaceFirst(String regex, String replacement) String s2 = “Hello guys, Hello world”; s2 = s2.replaceFirst(“Hello”, “Hi”); System.out.println(“After Replacing :”); System.out.println(s2); } }
- The output of above program is:
- After Replacing l with m : Hemmo Wormd After Replacing : Hi journaldev, Hi pankaj After Replacing : Hi guys, Hello world
- format()
Java Sting format() method is used to format the string. There is two variants of java String format() method.- format(Locale l, String format, Object… args): This method formats the string using specified locale, string format and arguments.
- format(String format, Object… args): This method formats the string using specified string format and arguments.
- package com.journaldev.examples; import java.util.Locale; /** * Java String format * * @author pankaj * */ public class StringFormatExample { public static void main(String[] args) { String s = “journaldev.com”; // %s is used to append the string System.out.println(String.format(“This is %s”, s)); //using locale as Locale.US System.out.println(String.format(Locale.US, “%f”, 3.14)); } }
- Output of above program is:
- This is journaldev.com 3.140000
- substring()
This method returns a part of the string based on specified indexes. - package com.journaldev.examples; /** * Java String substring * */ public class StringSubStringExample { public static void main(String[] args) { String s = “This is journaldev.com”; s = s.substring(8,18); System.out.println(s); } }
Java String Methods
Here are the list of the methods available in the Java String class. These methods are explained in the separate tutorials with the help of examples. Links to the tutorials are provided below:
char charAt(int index): It returns the character at the specified index. Specified index value should be between 0 to length() -1 both inclusive. It throws IndexOutOfBoundsException if index<0||>= length of String.
boolean equals(Object obj): Compares the string with the specified string and returns true if both matches else false.
boolean equalsIgnoreCase(String string): It works same as equals method but it doesn’t consider the case while comparing strings. It does a case insensitive comparison.
int compareTo(String string): This method compares the two strings based on the Unicode value of each character in the strings.
int compareToIgnoreCase(String string): Same as CompareTo method however it ignores the case during comparison.
boolean startsWith(String prefix, int offset): It checks whether the substring (starting from the specified offset index) is having the specified prefix or not.
boolean startsWith(String prefix): It tests whether the string is having specified prefix, if yes then it returns true else false.
boolean endsWith(String suffix): Checks whether the string ends with the specified suffix.
int hashCode(): It returns the hash code of the string.
int indexOf(int ch): Returns the index of first occurrence of the specified character ch in the string.
int indexOf(int ch, int fromIndex): Same as indexOf method however it starts searching in the string from the specified fromIndex.
int lastIndexOf(int ch): It returns the last occurrence of the character ch in the string.
int lastIndexOf(int ch, int fromIndex): Same as lastIndexOf(int ch) method, it starts search from fromIndex.
int indexOf(String str): This method returns the index of first occurrence of specified substring str.
int lastindexOf(String str): Returns the index of last occurrence of string str.
String substring(int beginIndex): It returns the substring of the string. The substring starts with the character at the specified index.
String substring(int beginIndex, int endIndex): Returns the substring. The substring starts with character at beginIndex and ends with the character at endIndex.
String concat(String str): Concatenates the specified string “str” at the end of the string.
String replace(char oldChar, char newChar): It returns the new updated string after changing all the occurrences of oldChar with the newChar.
boolean contains(CharSequence s): It checks whether the string contains the specified sequence of char values. If yes then it returns true else false. It throws NullPointerException of ‘s’ is null.
String toUpperCase(Locale locale): Converts the string to upper case string using the rules defined by specified locale.
String toUpperCase(): Equivalent to toUpperCase(Locale.getDefault()).
public String intern(): This method searches the specified string in the memory pool and if it is found then it returns the reference of it, else it allocates the memory space to the specified string and assign the reference to it.
public boolean isEmpty(): This method returns true if the given string has 0 length. If the length of the specified Java String is non-zero then it returns false.
public static String join(): This method joins the given strings using the specified delimiter and returns the concatenated Java String
String replaceFirst(String regex, String replacement): It replaces the first occurrence of substring that fits the given regular expression “regex” with the specified replacement string.
String replaceAll(String regex, String replacement): It replaces all the occurrences of substrings that fits the regular expression regex with the replacement string.
String[] split(String regex, int limit): It splits the string and returns the array of substrings that matches the given regular expression. limit is a result threshold here.
String[] split(String regex): Same as split(String regex, int limit) method however it does not have any threshold limit.
String toLowerCase(Locale locale): It converts the string to lower case string using the rules defined by given locale.
public static String format(): This method returns a formatted java String
String toLowerCase(): Equivalent to toLowerCase(Locale. getDefault()).
String trim(): Returns the substring after omitting leading and trailing white spaces from the original string.
char[] toCharArray(): Converts the string to a character array.
static String copyValueOf(char[] data): It returns a string that contains the characters of the specified character array.
static String copyValueOf(char[] data, int offset, int count): Same as above method with two extra arguments – initial offset of subarray and length of subarray.
void getChars(int srcBegin, int srcEnd, char[] dest, int destBegin): It copies the characters of src array to the dest array. Only the specified range is being copied(srcBegin to srcEnd) to the dest subarray(starting fromdestBegin).
static String valueOf(): This method returns a string representation of passed arguments such as int, long, float, double, char and char array.
boolean contentEquals(StringBuffer sb): It compares the string to the specified string buffer.
boolean regionMatches(int srcoffset, String dest, int destoffset, int len): It compares the substring of input to the substring of specified string.
boolean regionMatches(boolean ignoreCase, int srcoffset, String dest, int destoffset, int len): Another variation of regionMatches method with the extra boolean argument to specify whether the comparison is case sensitive or case insensitive.
byte[] getBytes(String charsetName): It converts the String into sequence of bytes using the specified charset encoding and returns the array of resulted bytes.
byte[] getBytes(): This method is similar to the above method it just uses the default charset encoding for converting the string into sequence of bytes.
int length(): It returns the length of a String.
boolean matches(String regex): It checks whether the String is matching with the specified regular expression regex.
int codePointAt(int index):It is similar to the charAt method however it returns the Unicode code point value of specified index rather than the character itself.