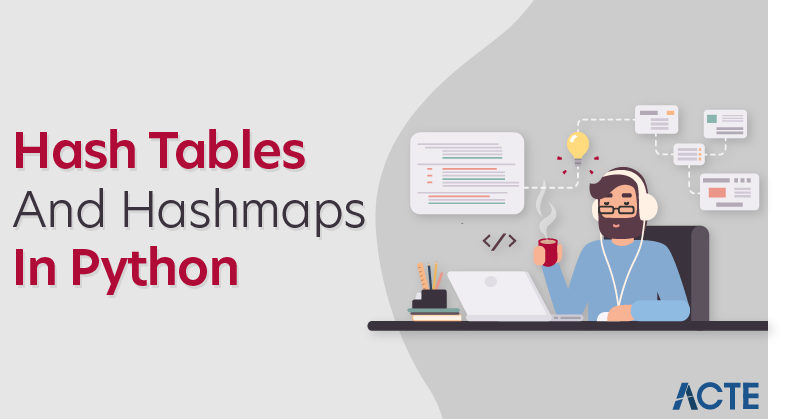
Data requires a number of ways in which it can be stored and accessed. One of the most important implementations includes Hash Tables. In Python, these Hash tables are implemented through the built-in data type i.e, dictionary. In this article, you will learn what are Hash Tables and Hashmaps in Python and how you can implement them using dictionaries.
What is a Hash table or a Hashmap in Python?
- In computer science, a Hash table or a Hashmap is a type of data structure that maps keys to its value pairs (implement abstract array data types). It basically makes use of a function that computes an index value that in turn holds the elements to be searched, inserted, removed, etc. This makes it easy and fast to access data. In general, hash tables store key-value pairs and the key is generated using a hash function.
- Hash tables or has maps in Python are implemented through the built-in dictionary data type. The keys of a dictionary in Python are generated by a hashing function. The elements of a dictionary are not ordered and they can be changed.
- An example of a dictionary can be a mapping of employee names and their employee IDs or the names of students along with their student IDs.
- Moving ahead, let’s see the difference between the hash table and hashmap in Python.
Creating Dictionaries:
Dictionaries can be created in two ways:
- Using curly braces ({})
- Using the dict() function
Using curly braces:
Dictionaries in Python can be created using curly braces as follows:
EXAMPLE:
- my_dict={‘Dave’ : ‘001’ , ‘Ava’: ‘002’ , ‘Joe’: ‘003’}
- print(my_dict)
- type(my_dict)
OUTPUT:
{‘Dave’: ‘001’, ‘Ava’: ‘002’, ‘Joe’: ‘003’}
Performing Operations on Hash tables using Dictionaries:
There are a number of operations that can be performed on has tables in Python through dictionaries such as:
- Accessing Values
- Updating Values
- Deleting Element
Accessing Values:
The values of a dictionary can be accessed in many ways such as:
- Using key values
- Using functions
- Implementing the for loop
Using key values:
Dictionary values can be accessed using the key values as follows:
EXAMPLE:
- my_dict={‘Dave’ : ‘001’ , ‘Ava’: ‘002’ , ‘Joe’: ‘003’}
- my_dict[‘Dave’]
- print(my_dict)
- type(my_dict)
OUTPUT:
‘001′
Using functions:
There are a number of built-in functions that can be used such as get(), keys(), values(), etc.
EXAMPLE:
- my_dict={‘Dave’ : ‘001’ , ‘Ava’: ‘002’ , ‘Joe’: ‘003’}
- print(my_dict.keys())
- print(my_dict.values())
- print(my_dict.get(‘Dave’))
OUTPUT:
dict_keys([‘Dave’, ‘Ava’, ‘Joe’])
dict_values([‘001’, ‘002’, ‘003’])
001
Implementing the for loop:
The for loop allows you to access the key-value pairs of a dictionary easily by iterating over them.
For example
- my_dict={‘Dave’ : ‘001’ , ‘Ava’: ‘002’ , ‘Joe’: ‘003’}
- print(“All keys”)
- for x in my_dict:
- print(x) #prints the keys
- print(“All values”)
- for x in my_dict.values():
- print(x) #prints values
- print(“All keys and values”)
- for x,y in my_dict.items():
- print(x, “:” , y) #prints keys and values
OUTPUT:
All keys
Dave
Ava
Joe
All values
001
002
003
All keys and values
Dave : 001
Ava : 002
Joe : 003
Updating Values:
Dictionaries are mutable data types and therefore, you can update them as and when required. For example, if I want to change the ID of the employee named Dave from ‘001’ to ‘004’ and if I want to add another key-value pair to my dictionary, I can do as follows:
EXAMPLE:
- my_dict={‘Dave’ : ‘001’ , ‘Ava’: ‘002’ , ‘Joe’: ‘003’}
- my_dict[‘Dave’] = ‘004’ #Updating the value of Dave
- my_dict[‘Chris’] = ‘005’ #adding a key-value pair
- print(my_dict)
OUTPUT:
{‘Dave’: ‘004’, ‘Ava’: ‘002’, ‘Joe’: ‘003’, ‘Chris’: ‘005’}
Deleting items from a dictionary:
There a number of functions that allow you to delete items from a dictionary such as del(), pop(), popitem(), clear(), etc. For example:
EXAMPLE:
- my_dict={‘Dave’: ‘004’, ‘Ava’: ‘002’, ‘Joe’: ‘003’, ‘Chris’: ‘005’}
- del my_dict[‘Dave’] #removes key-value pair of ‘Dave’
- my_dict.pop(‘Ava’) #removes the value of ‘Ava’
- my_dict.popitem() #removes the last inserted item
- print(my_dict)
OUTPUT:
{‘Joe’: ‘003’}
Converting Dictionary into a dataframe:
As you have seen previously, I have created a nested dictionary containing employee names and their details mapped to it. Now to make a clear table out of that, I will make use of the pandas library in order to put everything as a dataframe.
EXAMPLE:
- import pandas as pd
- emp_details = {‘Employee’: {‘Dave’: {‘ID’: ‘001’,’Salary’: 2000,’Designation’:’Python Developer’},
- ‘Ava’: {‘ID’:’002′,’Salary’: 2300,’Designation’: ‘Java Developer’},
- ‘Joe’: {‘ID’: ‘003’,’Salary’: 1843,’Designation’: ‘Hadoop Developer’}}}
- df=pd.DataFrame(emp_details[‘Employee’])
- print(df)
OUTPUT:

.