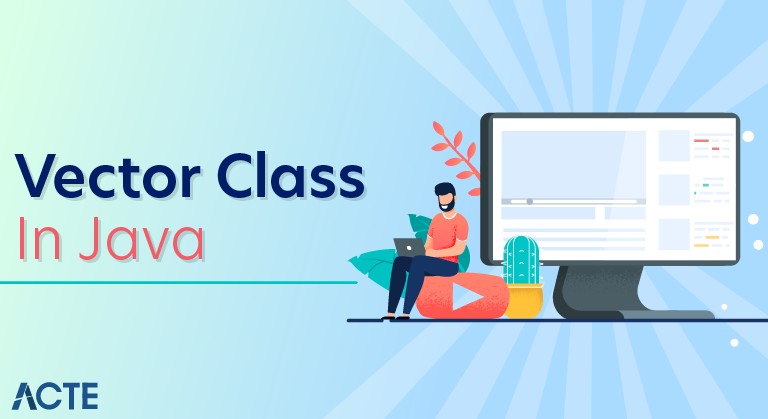
A vector can be defined as a dynamic array that can grow or shrink on its own i.e. vector will grow when more elements are added to it and will shrink when elements are removed from it.
This behavior is unlike that of arrays which are static. But similar to arrays, vector elements can be accessed using integer indices.
A vector can be viewed as similar to another dynamic array data structure, ArrayList except for the two below differences:
- The vector is synchronized i.e. all the methods in Vector are marked ‘synchronized’ and thus once a method is invoked, the same method cannot be invoked unless the previous call has ended.
- The vector class has many methods that are not a part of the collections framework but its legacy methods.
Java Vector Class
A Vector class is apart of the “java.util” package and implements List interface. A Vector is an array of objects or vector of objects.
A class declaration of Vector class is given below:
- public class Vector<E> extends Object<E> implements List<E>, Cloneable, Serializable
As shown above, a Vector class extends “java.lang.object” and implements List, Cloneable and Serializable interfaces.
How To Create A Vector In Java?
You can create a Vector object using any of the following Vector constructor methods.
Constructor Prototype | Description |
---|---|
vector() | This is the default constructor of the Vector class. It creates an empty vector with size 10. |
vector(int initialCapacity) | This overloaded constructor constructs an empty Vector object with the capacity = initialCapacity. |
vector(int initialCapacity, int capacityIncrement) | This constructor method creates an empty Vector object with specified initialCapacity and capacityIncrement. |
Vector( Collection< ? extends E> c) | A Vector object is created with the initial elements from specified collection c. |
Let’s look at each of the constructors to initialize Vector objects.
Initialize Vector
(i) Vector()
This is the default constructor of the Vector class. When you invoke this constructor, a Vector object of default size 10 is created.
The general syntax of this method is:
- Vector object = new Vector();
For Example,
- Vector vec1 = new Vector ();
The above statement creates a new Vector ‘vec1’ with size 10.
(ii) Vector(int initialCapacity)
The overloaded constructor of the Vector class accepts ‘initialCapacity’ as the argument. This constructor creates a Vector object with the specified capacity.
The general syntax of the method is:
- Vector object = new Vector (initialCapacity);
For Example,
- Vector vec1 = new Vector (10);
The above programming statement will create a Vector object ‘vec1’ with the capacity of 10 i.e. this Vector can store up to 10 elements.
(iii) Vector(int initialCapacity, int capacityIncrement)
This is yet another overloaded constructor of Vector class and it creates a Vector object with the specified initial capacity and increment for the capacity.
The general syntax for this method is:
- Vector object = new Vector (initialCapacity, capacityIncrement);
For Example,
- Vector vec1 = new Vector(5,10);
In the above statement, the initial capacity of the Vector is 5 and increment is 10. This means when the 6th element is inserted into the vector, the capacity of the vector will be incremented to 15 (5 + 10). Similarly, when the 16th element is inserted, the vector capacity of the Vector will be extended to 25 (15 +10).
(iv) Vector(Collection<? extends E> c)
The last overloaded constructor of the Vector class takes in a predefined collection as an argument and creates a Vector with all the elements from this collection as its elements.
The general syntax is:
- Vector object = new Vector (Collection<? extends E> c);
For Example,
- Vector vec1 = new Vector(aList); where aList = {1,2,3,4,5};
The above statement will create a Vector ‘vec1’ with initial elements as {1,2,3,4, 5}.
Keeping all these descriptions in mind will let us implement a Vector program to understand these constructors better.
Vector proves to be very useful if you don’t know the size of the array in advance or you just need one that can change sizes over the lifetime of a program.
Following is the list of constructors provided by the vector class.
Sr.No. | Constructor & Description |
---|---|
1 | Vector( )This constructor creates a default vector, which has an initial size of 10. |
2 | Vector(int size)This constructor accepts an argument that equals to the required size, and creates a vector whose initial capacity is specified by size. |
3 | Vector(int size, int incr)This constructor creates a vector whose initial capacity is specified by size and whose increment is specified by incr. The increment specifies the number of elements to allocate each time that a vector is resized upward. |
4 | Vector(Collection c)This constructor creates a vector that contains the elements of collection c. |
Apart from the methods inherited from its parent classes, Vector defines the following methods −
Sr.No. | Method & Description |
---|---|
1 | void add(int index, Object element)Inserts the specified element at the specified position in this Vector. |
2 | boolean add(Object o)Appends the specified element to the end of this Vector. |
3 | boolean addAll(Collection c)Appends all of the elements in the specified Collection to the end of this Vector, in the order that they are returned by the specified Collection’s Iterator. |
4 | boolean addAll(int index, Collection c)Inserts all of the elements in in the specified Collection into this Vector at the specified position. |
5 | void addElement(Object obj)Adds the specified component to the end of this vector, increasing its size by one. |
6 | int capacity()Returns the current capacity of this vector. |
7 | void clear()Removes all of the elements from this vector. |
8 | Object clone()Returns a clone of this vector. |
9 | boolean contains(Object elem)Tests if the specified object is a component in this vector. |
10 | boolean containsAll(Collection c)Returns true if this vector contains all of the elements in the specified Collection. |
11 | void copyInto(Object[] anArray)Copies the components of this vector into the specified array. |
12 | Object elementAt(int index)Returns the component at the specified index. |
13 | Enumeration elements()Returns an enumeration of the components of this vector. |
14 | void ensureCapacity(int minCapacity)Increases the capacity of this vector, if necessary, to ensure that it can hold at least the number of components specified by the minimum capacity argument. |
15 | boolean equals(Object o)Compares the specified Object with this vector for equality. |
16 | Object firstElement()Returns the first component (the item at index 0) of this vector. |
17 | Object get(int index)Returns the element at the specified position in this vector. |
18 | int hashCode()Returns the hash code value for this vector. |
19 | int indexOf(Object elem)Searches for the first occurence of the given argument, testing for equality using the equals method. |
20 | int indexOf(Object elem, int index)Searches for the first occurence of the given argument, beginning the search at index, and testing for equality using the equals method. |
21 | void insertElementAt(Object obj, int index)Inserts the specified object as a component in this vector at the specified index. |
22 | boolean isEmpty()Tests if this vector has no components. |
23 | Object lastElement()Returns the last component of the vector. |
24 | int lastIndexOf(Object elem)Returns the index of the last occurrence of the specified object in this vector. |
25 | int lastIndexOf(Object elem, int index)Searches backwards for the specified object, starting from the specified index, and returns an index to it. |
26 | Object remove(int index)Removes the element at the specified position in this vector. |
27 | boolean remove(Object o)Removes the first occurrence of the specified element in this vector, If the vector does not contain the element, it is unchanged. |
28 | boolean removeAll(Collection c)Removes from this vector all of its elements that are contained in the specified Collection. |
29 | void removeAllElements()Removes all components from this vector and sets its size to zero. |
30 | boolean removeElement(Object obj)Removes the first (lowest-indexed) occurrence of the argument from this vector. |
31 | void removeElementAt(int index)removeElementAt(int index). |
32 | protected void removeRange(int fromIndex, int toIndex)Removes from this List all of the elements whose index is between fromIndex, inclusive and toIndex, exclusive. |
33 | boolean retainAll(Collection c)Retains only the elements in this vector that are contained in the specified Collection. |
34 | Object set(int index, Object element)Replaces the element at the specified position in this vector with the specified element. |
35 | void setElementAt(Object obj, int index)Sets the component at the specified index of this vector to be the specified object. |
36 | void setSize(int newSize)Sets the size of this vector. |
37 | int size()Returns the number of components in this vector. |
38 | List subList(int fromIndex, int toIndex)Returns a view of the portion of this List between fromIndex, inclusive, and toIndex, exclusive. |
39 | Object[] toArray()Returns an array containing all of the elements in this vector in the correct order. |
40 | Object[] toArray(Object[] a)Returns an array containing all of the elements in this vector in the correct order; the runtime type of the returned array is that of the specified array. |
41 | String toString()Returns a string representation of this vector, containing the String representation of each element. |
42 | void trimToSize()Trims the capacity of this vector to be the vector’s current size. |
Example of Vector in Java:
- import java.util.*;
- public class VectorExample {
- public static void main(String args[]) {
- /* Vector of initial capacity(size) of 2 */
- Vector<String> vec = new Vector<String>(2);
- /* Adding elements to a vector*/
- vec.addElement(“Apple”);
- vec.addElement(“Orange”);
- vec.addElement(“Mango”);
- vec.addElement(“Fig”);
- /* check size and capacityIncrement*/
- System.out.println(“Size is: “+vec.size());
- System.out.println(“Default capacity increment is: “+vec.capacity());
- vec.addElement(“fruit1”);
- vec.addElement(“fruit2”);
- vec.addElement(“fruit3”);
- /*size and capacityIncrement after two insertions*/
- System.out.println(“Size after addition: “+vec.size());
- System.out.println(“Capacity after increment is: “+vec.capacity());
- /*Display Vector elements*/
- Enumeration en = vec.elements();
- System.out.println(“\nElements are:”);
- while(en.hasMoreElements())
- System.out.print(en.nextElement() + ” “);
- }
- }
Output:
- Size is: 4
- Default capacity increment is: 4
- Size after addition: 7
- Capacity after increment is: 8
- Elements are:
- Apple Orange Mango Fig fruit1 fruit2 fruit3
Commonly used methods of Vector Class:
- void addElement(Object element): It inserts the element at the end of the Vector.
- int capacity(): This method returns the current capacity of the vector.
- int size(): It returns the current size of the vector.
- void setSize(int size): It changes the existing size with the specified size.
- boolean contains(Object element): This method checks whether the specified element is present in the Vector. If the element is been found it returns true else false.
- boolean containsAll(Collection c): It returns true if all the elements of collection c are present in the Vector.
- Object elementAt(int index): It returns the element present at the specified location in Vector.
- Object firstElement(): It is used for getting the first element of the vector.
- Object lastElement(): Returns the last element of the array.
- Object get(int index): Returns the element at the specified index.
- boolean isEmpty(): This method returns true if Vector doesn’t have any element.
- boolean removeElement(Object element): Removes the specifed element from vector.
- boolean removeAll(Collection c): It Removes all those elements from vector which are present in the Collection c.
- void setElementAt(Object element, int index): It updates the element of specifed index with the given element.