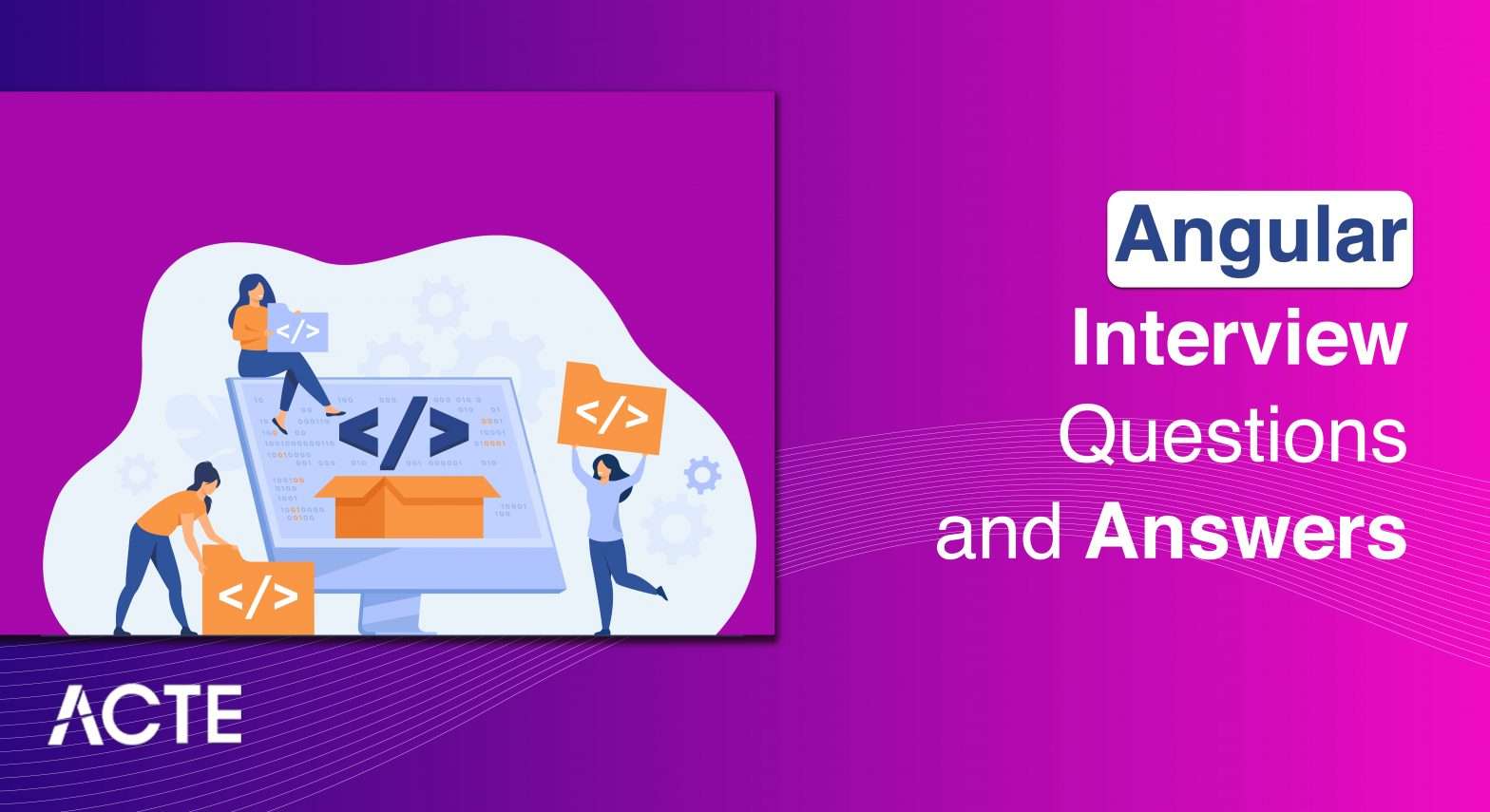
Angular is a robust, open-source web application framework developed by Google for creating dynamic single-page applications. Built on TypeScript, it offers features like two-way data binding, dependency injection, and a modular architecture, all of which enhance code maintainability and scalability. Angular’s extensive ecosystem includes tools for testing, routing, and form management, making it a favored choice among developers for building responsive and efficient user interfaces.
1. What is Angular, and how does it differ from AngularJS?
Ans:
Angular is a new generation of web application framework given out to the public domain by Google, enabling the development of dynamic SPAs. It is, therefore, a remake from scratch of what was originally called AngularJS – the original version of the framework. Unlike its predecessor, AngularJS, which works strictly on scope, Angular bases its construction on components and is mainly developed using TypeScript. This makes the application more modular and reusable with good performance.
2. Describe the architecture of an Angular application.
Ans:
The structure of an Angular application is component-based; that is, it is organized into modules. The structure of every application begins with a root module that usually goes under the name ‘AppModule’, which boots up the application. Components define what the application view will look like and encapsulate templates, styles, and even logic. Services are used in sharing data and business logic across components, which promotes a clean separation of concerns.
3. What are the components in Angular?
Ans:
- Angular components are blocks of the UI, encapsulating the view and all associated logic.
- A component comprises a TypeScript class holding the business logic and a view presentation declared using an HTML template, optionally augmenting this with CSS styles to include a presentation.
- Components can be nested to create hierarchical structures, where parent components can pass data down to the child components.
- The modular approach ensures reusability and maintainability since the components can be tested and upgraded easily. In total, components are essential for building interactive and dynamic web applications.
4. What is a module in Angular, and why is it important?
Ans:
- A module in Angular is a logical container for a cohesive block of code that groups related components, directives, pipes, and services.
- Every application that uses Angular has a root module that serves as the bootstrap mechanism; it is usually seen in an application when defined in the class ‘AppModule’.
- Modules can assist in structuring an application into functional units, resulting in better maintainability and scalability. It also helps provide lazy Loading, where parts of applications are loaded on demand.
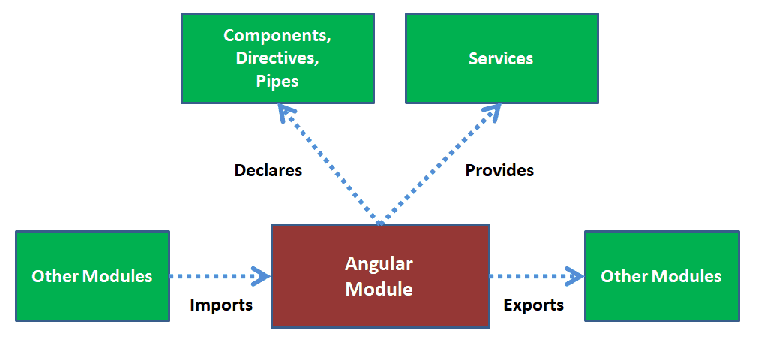
5. How is a new Angular application created with the Angular CLI?
Ans:
New Angular applications can be created using the Angular CLI in the terminal by executing the following code: ‘ng new <app-name>’. The command generates a new project’s structure with all the required files and folders and their specific configuration settings. Developers have several options like routing and stylesheet format available while generating. Once initialized, running ‘ng serve’ will start a development server which previews the application within a web browser.
6. What does the ‘@NgModule’ decorator do?
Ans:
The ‘@NgModule’ decorator defines an Angular module and configures its metadata. It involves a metadata object that specifies which components, directives, pipes, and services form part of the module. Apart from the declaration of imports, the module’s exports can also be defined with dependency injection and sharing of functionalities. The decorator is also an important feature in structuring application code such that it can compile and work with Angular.
7. What is Angular data binding?
Ans:
- This mechanism synchronizes Angular’s model (data) and the view (UI). Data binding, in general, is an essential technique that allows developers to update the user interface directly from the code without manually dealing with the DOM.
- Angular supports different types of data binding, such as two-way or one-way binding. This simplifies state management within applications and thus makes the code more readable and maintainable.
- Lastly, binding data assists in generating dynamic interactive applications that respond to data changes.
8. What are the different types of data bindings in Angular?
Ans:
- Interpolation: It allows the component’s data to be displayed within the template using double curly braces.
- Property binding allows the properties of an HTML element to be dynamically bound to the properties of the component using square brackets.
- Event binding allows applications to respond to a user event, like a click, using parentheses. Two-way binding is enabled using the ‘[(ngModel)]’ directive. It’s a method for two-way data flow that synchronizes the model and view.
9. What is the purpose of ngFor?
Ans:
The ‘ngFor’ directive is a way of iterating over an array and then rendering some template for each item. It simplifies how to render lists or tables in the UI by dynamically generating DOM elements based on the underlying data. The template example ‘*ngFor=”let item of items”‘ creates a new component instance for every ‘item’ in the array ‘items’. This directive will ensure efficiency and eliminate manually manipulating the DOM, making it very easy to update should the data change.
10. What is the difference between a component and a directive?
Ans:
Feature | Component | Directive |
---|---|---|
Definition | A self-contained unit that controls a view. | A class that modifies the behavior or appearance of elements in the DOM. |
Template | Always has an associated template. | Does not have its own template; works with existing DOM elements. |
Use Case | Used to create UI elements and encapsulate functionality. | Used to enhance or manipulate existing elements without creating new views. |
Syntax | Defined with ‘@Component’ decorator. | Defined with ‘@Directive’ decorator. |
Data Binding | Supports data binding and component interaction. | Can use property binding but does not have its own input/output properties. |
11. How does a parent component pass data to a child component?
Ans:
- In Angular, a parent component passes data to a child component using the ‘@Input’ decorator. The parent component then binds a property to the child component’s input property from the template using property binding syntax.
- For instance, ‘<child-component [inputProperty]=”parentData”></child-component>’ lets ‘parentData’ be passed to the child.
- lets ‘parentData’ be passed to the child. To capture the value, the child component decorates the corresponding property with ‘@Input()’. This allows for one-way data flow and allows components to be encapsulated and reused.
12. What is an Angular service, and how is it created?
Ans:
An Angular service is a reusable class encapsulating business logic da, ta retrieval, or other functionality that may be shared across multiple components. This promotes separation of concern and enhances maintainability by centralizing logic that might otherwise be duplicated throughout many components. A service is created using the Angular CLI with the command ‘ng generate service serviceName’, which generates a TypeScript class.
13. How does the ngIf directive work in Angular?
Ans:
- The ‘ngIf’ directive conditionally includes or excludes elements from the DOM based on a boolean expression.
- When the expression evaluates to true, the associated elements are rendered; if false, they are removed from the DOM.
- Example with ‘*ngIf=”isVisible”:’ It would only display the element if ‘isVisible’ is true. The angular directive that makes elements dynamically visible shows the relevant content, enhancing the user experience. It optimizes performance by removing the elements from the DOM when conditions are unmet.
14. What are the decorators ‘@Input’ and ‘@Output’ used for?
Ans:
- ‘@Input’ allows a parent to send data down to the child component by decorating a property in the child with ‘@Input()’. This provides for one-way data flow from parent to child.
- However, using ‘@Output’, a child component can emit events back to the parent, allowing for two-way communication.
- This is often done using ‘EventEmitter’, allowing the parent to respond to actions taken in the child component.
15. How do events work in Angular?
Ans:
Angular uses event binding so developers can listen to the UI for events. Using parentheses in the template allows an event to be bound up to a method in the class. Thus, for example: ‘(click)=\\\”handleClick()\\\”‘ would call ‘handleClick’ when an element was clicked. Angular provides several events that it uses to manage typical user interactions: such as clicks, key presses, and mouse movements. This sort of event handling ensures things remain clean, maintains separation of concerns, and makes the code more maintainable.
16. What is the lifecycle of an Angular component?
Ans:
These lifecycle hooks consist of the Creation stage when the component is instantiated; Change Detection, which allows Angular to go through all its input properties in search of any changes; and View Initialization, once the view for the component is rendered. Also included among the important hooks are ‘ngOnInit’: this hook is called after the initialization of an element; ‘ngOnDestroy’: this hook is called before the destruction of a component.
17. What are Angular template reference variables?
Ans:
- In Angular templates, a template reference variable refers to a DOM element or an instance of a component.
- A variable is declared by prefixing its name with a hash sign: ‘#myInput’. It can then be accessed within the same template to refer to the properties and methods of the referenced element or component, as shown in ‘#myInput.value’. ‘ to get the value of an input field.
- Template reference variables are useful for managing DOM elements and interacting with components directly within templates.
18. How are pipes used in Angular templates?
Ans:
- Pipes in Angular Transform displayed data in templates. They take input data and return a transformed output, allowing developers to format and manipulate data without changing the underlying component code.
- There are built-in and custom-defined pipes like ‘date’, ‘currency’, and ‘json’. They are used in templates via the pipe operator (‘|’), e.g., ‘{{ value | currency }}’.
- Pipes make templates easier to read and maintain by separating presentation logic for data from the component’s logic.
19. What is the purpose of the ‘ng-content’ directive?
Ans:
The ‘ng-content’ directive in Angular is used to create projection points for content, which can then be inserted into a component’s template. It helps dev and elopers create reusable components that accept and show any arbitrary content defined in the parent template. Any content wrapped within the component tag in the parent will appear at the location of ‘<ng-content></ng-content>’ by putting this in the component template. This feature allows flexibility and reusability in creating versatile components that adapt to different use cases.
20. How to dynamically create components in Angular?
Ans:
- Dynamically create a component in Angular using a service called ‘ComponentFactoryResolver’.
- This service allows developers to programmatically create instances of components and insert them into the DOM.
- A placeholder in the template can be defined using ‘ng-template’.
- The dynamic component is resolved in the class using ‘ComponentFactoryResolver’ to create an instance.
- Finally, the dynamic component can be attached to the view using the ‘ViewContainerRef’ to finish this, allowing rendering components to be conditionally based on user interactions or application state.
21. What is dependency injection in Angular?
Ans:
Dependency Injection is one of the design patterns used by Angular to create more modular, readable, and reusable code. It allows components and services to get their dependencies through an external source instead of getting them created inside the component or service. Separation of concern builds on the testability and maintainability capabilities of components. Angular’s injector is in charge of creating and managing the lifecycle of dependencies and injects them into components and services where needed.
22. How does one create a service in Angular?
Ans:
A service in Angular can be created with the ‘@Injectable’ decorator. This is the mark of a class so that it will be available to the dependency injection. For example, use the following command to generate the service: ‘ng generate service serviceName’. A new service file is added to contain the code related to the business logic. After that, the service needs to be registered with a module in an Angular application; normally, it is done by adding it as an item into the provider’s array of a module or by using the provided property inside the @Injectable decorator to make the service available application-wide. Once registered, the service can be injected into any component or another service.
23. What’s the difference between singleton and shared services?
Ans:
- A singleton service in Angular is a service that has been instantiated only once during an application’s lifecycle; it implies that just one instance of the service is shared across all those components, depending on it.
- This is the default way to use a service when provided at the root level; however, a shared service can exist, implying that multiple instances of the same service may exist, especially if supplied at different module or component levels.
- It helps create several unique instances for parts of an application and allows them to share the same logic.
24. Describe how angular implements hierarchical dependency injection.
Ans:
- Hierarchical dependency injection in Angular supports creating numerous injectors across a component tree. Each component may have its injector, which would provide the services independently, inheriting services from the parent’s injector.
- If a requested service is not found in the local injector, Angular goes up to its parent and continues this search through its parents until it reaches a root injector for the requested service.
- This architecture allows for more flexibility because services can be scoped to specific components, encouraging encapsulation and minimizing side effects from using the wrong service.
25. How can a service be implemented at the component level?
Ans:
To offer a service for one specific component level, apply the ‘providers’ array within the component’s decorator. This way, an application creates a new instance of the service specifically for that component and its children, separate from all the others in the application. For example:
- @Component({
- selector: ‘app-my-component’,
- templateUrl: ‘./my-component.component.html’,
- providers: [MyService]
- })
- export class MyComponent {
- constructor(private myService: MyService) {}
- }
26. What is Angular Router, and how does it work?
Ans:
Angular Router is an exceedingly powerful module enabling navigation and routing in Angular applications. It makes it easy to define routes and map URL paths to components, which can be used to create single-page applications. Routing breaks up the URL into its components and loads the appropriate element, automatically updating the view without refreshing the whole page when a user navigates to a URL. Angular Router supports nested routes, route parameters, lazy Loading, and guards to handle complex routing scenarios.
27. Explain the application of route parameters in Angular.
Ans:
- Route parameters in Angular are dynamically part of the route; that is, the ability to pass data into the URL.
- They are useful for capturing values such as user IDs, product IDs, or any other identifier required to show relevant information about a component.
- Route parameters are defined using a colon inside our route configuration, like this: ‘[{ path: ‘user/:id’, component: UserComponent }]’. Now, in ‘UserComponent’, get the parameters using the ‘ActivatedRoute’ service.
- This service can provide the value of ‘id’ from the route, enabling the component to fetch and display the appropriate data.
28. How is lazy Loading enabled in Angular?
Ans:
Lazy Loading Improves application performance since modules are loaded only when called and not at the time of application loading. Use Angular CLI to create a feature module, then configure routes for that module using loadChildren on the main routing configuration. For example, here’s how lazy Loading can be added using load children in the main routing configuration:
- { path: ‘feature’, godchildren: () => import(‘./feature/feature.module’).then(m => m.FeatureModule) }
This configuration optimizes the initial load time and overall bundle size since the application is broken down into more manageable pieces.
29. What are guards in Angular routing, and how are they used?
Ans:
Angular routing guards implement ‘CanActivate’, ‘CanDeactivate’, ‘Resolve’, ‘CanLoad’, or ‘CanActivateChild’ interfaces to control route access. They can protect routes from unauthorized access, manage route activation based on conditions, or even preload data before navigation. For example, a ‘CanActivate’ guard can check whether a user is authenticated before allowing them to navigate to some specific route. Guards are added to the routing configuration using the property ‘can activate’, ensuring the route navigation adheres to the specified condition.
30. How is data passed to a route in Angular?
Ans:
- Data can be passed to a route in Angular using route parameters or query parameters. Route parameters are defined within the route configuration and retrieved within the component using the service ‘ActivatedRoute’.
- For example, suppose that set up a route as ‘path: ‘user/:id”. In this case, able to retrieve the ‘id’ in a component. Whereas query parameters are optional parameters added to the URL in the format ‘? key=value’.
- They can be accessed using the observable ‘queryParams’ from the ‘ActivatedRoute’ service, and it is a very flexible way to pass some extra data for routes without interfering with the route structure.
31. What’s the difference between reactive forms and template-driven forms?
Ans:
- There are two methods of handling forms with Angular: template-driven and reactive.
- Reactive forms are more explicit and predictable. Instead of modifying the form state and validation as needed, they rely on the class for the component managing these form states and validating the forms.
- Forms are created dynamically and validated with robust mechanisms using ‘FormGroup’ and ‘FormControl’ objects. On the other hand, template-driven forms depend on Angular directives found in the HTML template.
32. How is a reactive form created in Angular?
Ans:
A reactive form in Angular is created by importing the ReactiveFormsModule and utilizing FormGroup and FormControl classes to define the form structure. First, define a FormGroup in the component class, instantiating it with form controls:
- import { FormGroup, FormControl } from ‘@angular/forms’;
- export class MyComponent {
- myForm = new FormGroup({
- name: new FormControl(”),
- email: new FormControl(”)
- });
- }
33. Explain form validation in Angular.
Ans:
Form validation in Angular checks user-submitted data against certain criteria before form submission. Angular provides two types of validation: synchronous and asynchronous. Synchronous validation can be performed inline on the form control definitions with the availability of built-in validators like ‘Validators.required’ or ‘Validators.email’ or custom validators. For example,
- name: new FormControl(”, Validators.required)
An example of asynchronous validation is checking for the availability of a username via an API call. Developers could easily surface validation errors in the template and present them to users in real-time, ultimately improving the application’s user experience.
34. What are FormGroup and FormControl?
Ans:
- FormGroup and FormControl are the most basic classes within the Angular module of reactive forms. A ‘FormGroup’ represents the group of controls within a form and encapsulates its structure and state.
- It allows for managing validation and tracking the value in groups of controls. ‘FormControl is an individual field within the form, thereby managing its value and validation status.
- Together, they make possible even more complex forms with dynamic updates and manage validation in an organized and structured manner.
35. How is form submission handled in Angular?
Ans:
Form submission in Angular is handled using the directive ‘(ngSubmit)’ in the template, which calls out to a method in the component once the form is submitted. All of the values from the form controls can be accessed by calling the ‘value’ property of the ‘FormGroup’. Here’s an example:
- onSubmit() {
- console.log(this.myForm.value);
- }
To avoid the form’s default submission behaviour, you can use ‘type=” button”‘ on the submit button or explicitly use the method signature to control the submission behaviour. Validation could be checked before processing the form data so only valid inputs are passed.
36. What are Observables in Angular, and how is it different from Promises?
Ans:
Observables in Angular are a part of the RxJS library, a stream of data that an observer can follow along with time. Unlike a Promise, which resolves only one asynchronous event, Observables allow for multiple emitted values over time, with many powerful operators manipulating those values. Therefore, Observables can be cancelled or unsubscribed, making them highly flexible and similar to dynamic data streams like user inputs or HTTP requests.
37. How does the ‘HttpClient’ service make API calls?
Ans:
- The HTTPClient is a way to make HTTP requests to external APIs outside of an Angular application. To enable its usage, import the HTTPClientModule into the application module and inject it into a component or service when the need to make API calls arises.
- HttpClient offers get(), post(), put(), and delete() methods to send different types of HTTP requests. For example, import { HttpClient } from ‘@angular/common/http’;
- The ‘HttpClient’ transforms data on JSON, making it easy to work with responses. It also offers functionality like interceptors and error management for requesting and responding.
38. What does the ‘switchMap’ operator in RxJS do?
Ans:
- The ‘switchMap’ operator in RxJS turns items emitted by an Observable into Observables. It subscribes to each new Observable while unsubscribing from the previous one.
- This operator is very useful in modelling scenarios such as search capabilities, where a new search input cancels the previous request to avoid wasting processing time.
- Consider an API call invoked by entering some user data: ‘switchMap’ ensures that only the last response from the API is taken into account in calculating new results, thus preventing race conditions and improving performance and responsiveness.
39. How is error handling described in an Observable stream?
Ans:
Errors in an Observable stream could be caught using the ‘catchError’ operator. It helps catch errors and thus allows graceful recovery or alternative handling. It can provide a fallback Observable, or an empty result to keep it alive. About subscribing to an Observable, supply an error callback function to handle an error explicitly. An example of a subscription with an error callback: This way, with the help of error handling, developers will create strong and user friendly applications even under those situations that are not expected.
40. What are Subjects in RxJS, and how do they work?
Ans:
In RxJS, Subjects are special kinds of Observables that enable multicasting many values to Observers. They represent Observable and Observer, meaning they can emit values besides being subscribed to. Subjects are valuable scenarios where multiple components want to share and respond to the same data source. For example, a Subject can be used to create an application state-shared service.
41. What is state management, and why is it needed in an Angular application?
Ans:
State management refers to defining the pattern through which the state of an application can be managed in a predictable, consistent, and reliable manner. It becomes important in Angular applications for reasons such as
- Data consistency ensures that the same UI components have the same data throughout the application.
- Predictability: easier to understand how data changes over time: fewer bugs
- Debugging: tracking state changes makes debugging easier and allows tracking.
- Scalability: allows larger apps by being clear on the structure for complex state interactions
42. How does state management work in Angular?
Ans:
- Service-based approach: Angular services are used for managing shared state.
- Local component state: The state is managed at the component level for the small application.
- NgRx is a famous state management library based on the principles of Redux, such as centralized stores, actions, reducers, and selectors.
43. What are the advantages NgRx offers in state management?
Ans:
- Centralized state management: Control and debug the state of the application in a single store
- Immutable state: This state encourages immutability, which decreases side effects and bugs. State updates are predictable with actions and reducers.
- Assemblage of powerful tooling: NgRx offers a robust debugging and development tool, NgRx Store Devtools.
- Integration with Angular: Designed for Angular, NgRx integrates well with the Angular ecosystem.
44. Describe the concept of actions, reducers, and selectors in NgRx.
Ans:
In NgRx, actions, reducers, and selectors are essential concepts for state management in Angular applications. Actions serve as payloads representing changes or events within the application, facilitating communication between components and the store. Reducers are pure functions that take the current state and an action as inputs, producing a new state based on the action’s type and payload, which helps maintain immutability and predictability.
45. How does NgRx handle side effects?
Ans:
Side effects in NgRx are handled by NgRx Effects, a different class that listens for actions dispatched from the store. Effects can execute any asynchronous operation, such as HTTP requests, and then dispatch new actions based on the result. This ensures that the state management logic remains clean and focused on pure functions. Additionally, NgRx Effects promotes better separation of concerns, making the application more modular and easier to maintain.
46. What is Angular Universal, and how does it make server-side rendering feasible?
Ans:
The Angular Universal technology allows rendering the Angular application on the server side instead of the client, thereby enabling server-side rendering. This ensures better performance and SEO because it saves loading time, as the server sends a fully rendered page to the client. Fully rendered HTML pages are crawlable by search engines, thus improving discovery.
47. What is the difference between AOT and JIT compilation in Angular?
Ans:
- AOT (Ahead-of-Time): The Angular application is compiled at build time and generates efficient JavaScript code. As a result, rendering becomes faster, and the bundle sizes are smaller because templates are precompiled.
- JIT (Just-in-Time): Compiles the application in the browser at runtime. It makes development easier because of live reloads but can make initial load times slower and the bundle sizes larger.
48. How an Angular application can be optimized for performance?
Ans:
Optimizing an Angular application for performance involves several key strategies. First, Ahead-of-Time (AOT) compilation reduces the application size and enhances load times by compiling templates during the build process. Second, implementing lazy Loading allows feature modules to load only when necessary, which decreases initial loading time. Third, adopting ChangeDetectionStrategy.OnPush helps minimize unnecessary change detection by updating the view only when input properties change.
49. What are Angular decorators, and what are some common examples?
Ans:
Angular decorators are special functions that add metadata to classes. Common examples include:
- @Component: Defines a component and its metadata.
- @Injectable: Marks a class as a service that can be injected.
- @NgModule: Defines a module and its components, services, and other modules.
- @Directive: Defines a directive with its metadata.
50. How can custom pipes be created in Angular?
Ans:
Creating custom pipes in Angular involves defining a new class that implements the `PipeTransform` interface. First, the class should be annotated with the `@Pipe` decorator, which includes a name for the pipe. Next, the class must implement the `transform` method, allowing it to take input data and any additional arguments to modify it as required. After defining the pipe, it should be added to the `declarations` array of the relevant module.
51. How are Angular components tested?
Ans:
- Setting up the testing module: Utilize TestBed to set up the environment under which testing needs to be performed.
- Component instances: Instantiates the component to test.
- Simulate User Interactions: Trigger events and validate outcomes with ‘fixture’.
- Assertions: Use Jasmine’s ‘expect’ to assert expected behaviour and outcome.
52. What are Jasmine and Karma, and how are they used in Angular testing?
Ans:
- Jasmine: A behaviour-driven development framework for writing unit tests. It offers functions for defining specs and expectations.
- Karma: A test runner that runs the Jasmine tests in multiple browsers. It watches for file changes and automatically re-runs the tests in real time with feedback.
53. What is the purpose of TestBed in Angular?
Ans:
‘TestBed’ is an Angular testing utility that allows developers to create a testing module, set up dependencies, and instantiate all the components, services, and classes they want to test. It provides a method for configuring the Angular testing environment and generates a context for executing tests. Additionally, TestBed facilitates dependency injection, making it easier to manage and mock services during testing.
54. How does one go about doing end-to-end testing in Angular?
Ans:
These extend to defining tests that simulate user interactions with the application, running these tests via the command line interface in real browsers, and validating the application’s behaviour to ensure it functions as intended according to user scenarios. Furthermore, this comprehensive testing approach helps identify issues early in development, improving overall application quality and user satisfaction.
55. What is Protractor doing in Angular testing?
Ans:
Protractor is the end-to-end testing framework specifically designed for Angular applications. It extends WebDriverJS to automate browser interactions and is particularly optimized for testing Angular apps. The protractor understands Angular-specific features, allowing for more reliable and concise tests. Additionally, it provides built-in synchronization with Angular’s asynchronous operations, ensuring that tests run smoothly without the need for manual waits or timeouts.
56. How is an Angular application deployed?
Ans:
- To run ‘ng build –prod’ creates the application in production mode. Additionally, to deploy it to any web server (such as Apache or Nginx) or hosting platforms like Firebase Hosting or AWS S3, the contents of the ‘dist’ folder should be transferred.
- Servers must be configured to serve the application, including routing. It is also essential to ensure the server is set up to handle any necessary fallback routes for single-page applications.
57. What are some best practices for the structure of an Angular application?
Ans:
- Feature-based structure: Organize files by feature or module rather than type.
- Consistent naming conventions: Use consistent naming conventions for components, services, and modules.
- Separation of concerns: Separate the components, services, and models to ease maintainability.
- Using Angular CLI: Use the Angular CLI to create a consistent component, service, and module in the application.
58. How to ensure security on an Angular application?
Ans:
- Sanitization: The angular offers sanitization as part of the security feature to avoid XSS attacks
- Authentication and Authorization: Implement strong user authentication and role-based access control.
- CORS: Proper configuration of Server-side Cross-Origin Resource Sharing.
- Secure storage: Avoid storing secret information locally in storage; use secure cookies instead.
59. What are environment files in Angular?
Ans:
In Angular, environment files, such as ‘environment.ts’ and ‘environment.prod.ts’, are defined with a certain configuration depending on the environment. A developer can change settings dependent on whether they use a development or production environment without editing core application code. This really comes in handy for features such as API endpoints or toggles for feature flags.
60. Describe how Angular CLI empowers the developer in their dev process.
Ans:
Angular CLI is the acronym for Command Line Interface to initiate, develop, scaffold, and maintain applications in Angular. These are the development aspects where it supports development:
- Project setup: Fast scaffolding of new Angular projects with best practices.
- Code generation: Automatic creation of components, services, and modules.
- Development server: Running a local development server with live reload.
- Build optimizations: Optimizing the build process to be ready for production.
61. What are some common performance issues in Angular applications?
Ans:
- Too many change detection cycles.
- Huge bundle sizes.
- Inefficiently using third-party libraries.
Poorly implemented change detection often results in poor performance, as does using the default change detection strategy. Too many components or modules-load all at once, causing slow loading times. Other issues include redundancy re-renders, too much DOM manipulation, and failure to use lazy Loading for routes and modules.
62. How to optimize Angular application bundle size?
Ans:
Some strategies can ensure the optimization of the size of the Angular application bundle. Among them are tools of the Angular CLI built to give an analysis and graphical representation of bundle sizes, tree shaking to expunge unused code, and lazy Loading, which loads modules only as needed. Minifying and compressing assets, AOT compilation, and image optimization will help reduce the total bundle size. Being mindful of third-party library selection and avoiding unnecessary dependencies will further help with optimization.
63. What is lazy Loading, and how does it enhance the application performance?
Ans:
- Lazy Loading is one of the design patterns that Angular utilizes to load modules only when they are needed rather than loading all modules when the application is started.
- It reduces an application’s initial load time and bandwidth usage by only loading the necessary code immediately. This strategy helps create a faster and more responsive feel of an application as it is in use.
- The required modules are only loaded when the user requests specific features or routes while navigating the application.
64. What does trackBy in ngFor do?
Ans:
- Inside an Angular directive, ‘ngFor’ uses the ‘trackBy’ function to optimize rendering lists. The ‘trackBy’ function tracks changes in items based on a unique identifier instead of a default object reference.
- In this manner, it would prevent the unnecessary re-renders that some items in the list would cause should some items within the list change because Angular can easily identify what has changed, been added, or removed.
- Adding a ‘track’ function can enhance performance in applications with big lists or complex data structures. This allows better updates and smoother responses when updating large lists or complex data structures.
65. What does Angular apply to the ChangeDetectionStrategy?
Ans:
The application ChangeDetectionStrategy in Angular defines how and when Angular checks for changes in the component’s data. Basically, two of them are ‘Default’ and ‘OnPush’. The ‘Default’ strategy checks for changes on every event or async operation of the component and all of its children, whereas ‘OnPush’ checks only for input property changes or an event that occurs inside the element. The use of ‘OnPush’ can speed up performance dramatically by reducing the number of checks Angular has to perform, particularly in components containing immutable data.
66. What are Angular modules, and how do they help organize code?
Ans:
Angular modules are considered very important components of any application made in Angular to organize code into fairly coherent blocks. One module can contain components, directives, pipes, and services related to a certain functionality. The modular architecture will foster further reusability and maintainability of the code. Modules also make lazy Loading possible; they facilitate loading only parts of an application on demand, thus increasing performance and keeping its structure clean and manageable.
67. How to deal with the third party libraries in Angular?
Ans:
- In Angular, third-party libraries can be managed with the help of package managers such as npm or yarn. Developers install libraries through a command-line client, and the Angular CLI integrates them into the application itself.
- Installed libraries can then be imported and used within application modules. This means that the libraries used should be compatible with Angular’s version and support best practices for performance and security.
- Most importantly, updating libraries is essential to ensure the application operates steadily.
68. What is the Angular Workspace?
Ans:
- In Angular, a workspace can be a single repository with multiple applications and libraries under the same management.
- It is a centralized environment for projects, configurations, and all dependencies. Workspaces support common code sharing between applications and their libraries.
- This factor supports the reuse of code to achieve enough consistency. Developers can easily generate new projects, libraries, and components using the Angular CLI in the workspace, which streamlines the development process and makes it easier to collaborate within the team.
69. How is a custom directive created in Angular?
Ans:
The definition of an Angular custom directive is made using the ‘@Directive’ decorator, which defines the class of the directive. The developers define the selector, used by the directive in the template. The developers could define lifecycle hooks inside the class, for example, ‘ngOnInit’ or ‘ngOnChanges’. Custom directives allow manipulation of the DOM or enrich existing functionalities with additional functionality. Last but not least, to declare an Angular module with a name where this directive will be available.
70. Explain why the NgModule Angular RouterModule exists.
Ans:
The Angular RouterModule is the central module offering routing and navigation possibilities in Angular applications. It allows developers to define routes that map the URL path to the view component, thus giving them dynamic views depending on how the user navigates. The RouterModule supports functions like route parameters, lazy Loading, nested routes, authentication, and authorization guards.
71. What are some common security vulnerabilities in Angular applications?
Ans:
- Common security vulnerabilities in Angular applications include Cross-Site Scripting (XSS), Cross-Site Request Forgery (CSRF), and insecure handling of sensitive data.
- XSS vulnerabilities arise when untrusted data is rendered in the application without proper sanitization. CSRF attacks can occur when malicious requests are sent from authenticated users without their knowledge.
- In addition, improper management of authentication tokens and inadequate implementation of secure data storage may also result in a data breach. That, too, needs to be addressed to ensure secure applications.
72. How is authentication and authorization implemented in Angular?
Ans:
- Authentications and authorizations in Angular can be handled using services and guards. In normal practice, a separate service, such as JWT, will be provided for user login, registration, and token management.
- Route guards shall ensure authentication for the routes. Users can also be redirected wherever necessary. The implementation may require role-based access control.
- This might be handled by verifying the user’s respective roles with the routes’ requirements. Third-party authentication providers and custom strategies can be implemented to integrate authentication.
73. What is Cross-Site Scripting, and how does Angular prevent it?
Ans:
Cross-site scripting (XSS) is a condition in which attackers inject malicious scripts into web applications, which are then executed in the context of a user’s browser. Angular automatically sanitizes untrusted data while rendering templates. A built-in security mechanism is used to escape harmful content and limit the execution of harmful scripts. If necessary, developers can specifically manage safe values by using Angular’s ‘DomSanitizer service to ensure that all dynamic content is dealt with properly.
74. How to deal with sensitive data in Angular applications?
Ans:
Handling sensitive data in Angular applications involves implementing the best practices in terms of security. This includes using secure transfer protocols, such as HTTPS, and never hard-code sensitive information, such as API keys or passwords, within the source code. Instead, environment variables or mechanisms for secure storage should be utilized. Besides this, sensitive data must also be safely stored on the server and can only be sent in with such detail as is needed.
75. What are Content Security Policies (CSP), and why are they important in Angular?
Ans:
- Content Security Policies (CSP) are security measures aimed at preventing all attacks, such as XSS and others, that rely on executing scripts in the victim’s context.
- They define which content sources our web application can load and execute for scripts, styles, and other resources. Implementing CSP in Angular applications reduces the risk of injecting malicious scripts and helps protect user data.
- Developers can configure CSP headers to allow sources for scripts and other resources, enhancing the application’s overall security posture.
76. What are some of the popular libraries or frameworks that complement Angular?
Ans:
- Many libraries and frameworks support Angular to extend its feature sets. The most popular UI component libraries include Angular Material and PrimeNG, which can provide pre-built components to expedite application development.
- RxJS is integrated into Angular for reactive programming, which efficiently handles asynchronous data streams. NgRx provides state management solutions based on Redux principles that help effectively and efficiently manage state in large applications.
- Some other libraries include ngx-bootstrap and ngx-formly, which offer more functionalities and support different development workflows.
77. How can one stay updated on Angular’s new trends and updates?
Ans:
Therefore, such updates could be followed through official Angular blogs and documents, community forums, and social media, such as Twitter and LinkedIn. The developer can also subscribe to newsletters or podcasts related to Angular developments. The open-source projects that one may be working on or attending conferences, workshops, and meetups will not stop them from staying in touch with the community and keeping up with emerging practices and technologies.
78. What is the role of the Angular community in creating the framework?
Ans:
The Angular community has very important roles in developing the framework, from feedback on the product to contributions in discussions and then sharing knowledge. Community members often contribute to open-source projects, write tutorials, and develop libraries that extend the functionality of Angular. These user groups and forums help work together among developers and share knowledge to better practices and common problems.
79. How can contributions be made to the Angular ecosystem?
Ans:
- Contributions to the Angular ecosystem can be made in several different ways. Developers can contribute to open-source projects by reporting bugs or creating feature requests.
- They can contribute code to the Angular repository on GitHub or write articles, guides, and documentation to share the knowledge they acquire with community members.
- Another method is to create libraries or modules that enhance Angular with more feature-rich functionalities and publish them on NPM, which can also be an add-on to the same.
80. What are some good resources or tools for learning Angular?
Ans:
- Several resources and tools help one learn how to use Angular. The official Angular documentation is the source with the most detailed guides and tutorials for developers of all levels.
- Some structured courses or even practical projects are included in the content published on online platforms such as Udemy, Coursera, or Pluralsight. Community forums like Stack Overflow or Angular’s GitHub discussions also offer collaboration and troubleshooting chances.
- Angular CLI, Angular DevTools, or IDEs like Visual Studio Code can make this learning process and the subsequent development much smoother.
81. How would large forms with many input fields be handled in Angular?
Ans:
Angular forms have two forms: reactive forms and template-driven forms. For complex structures of form, larger forms can be managed effectively. Reactive forms provide a higher-scale approach and can efficiently use form groups and form arrays for their complex structures. They can then have better validation for controls and dynamically allow easy integration with observables. Many built-in validators can also be combined with custom validators for data integrity.
82. What difficult problem was faced while working with Angular, and how was it solved?
Ans:
Handling the state of more than one component in Angular sometimes becomes complex, particularly for large applications. This easily results in inconsistent data or complex communications among the components. For this reason, as illustrated by the scenario above, a centralized state management solution like NgRx was implemented to avoid unwanted intricacies about inconsistent data or complex communication issues among different components in the application.
83. How would one approach the integration of a REST API into an Angular application?
Ans:
- Adding the REST API to the Angular application involves many important steps. First, ‘HttpClientModule’ needs to be imported into the application module in Angular, enabling HTTP communications.
- Then, a service will encapsulate all API calls with Angular’s ‘HttpClient’. Methods can, therefore, include GET, POST, PUT, and DELETE.
- Observables make asynchronous requests with HTTP so that the application responds to changing data. Finally, inject the service into a component to make seamless requests and get data back.
84. Describe how the state would be managed within a large-scale Angular application.
Ans:
- In a large-scale Angular application, state management can be achieved easily with libraries like NgRx or Akita.
- These libraries implement the Redux pattern in a way that retains one-way data flow, or unidirectional, with a single source of truth in the application state.
- Actions are dispatched through reducers to change the state, and the selectors draw the state needed for components. This structure will make debugging more efficient, support time travel, and provide better performance.
- Besides this, using services for an accruing shared state between components can complement the state management strategy.
85. What is the end-to-end process of using an Angular application?
Ans:
Improving the user experience in an Angular application might use various techniques. Make the Angular application route lazy load: This requires fewer components to be loaded at the onset, thus lightening the initial load time. Use of Angular Material or Custom UI Components Enhances the look and feel of the application. Loading indicators, error messages, and tooltips inform users of how things are going during interaction.
86. What does Angular’s change detection mechanism do?
Ans:
Angular’s change detection mechanism updates the user interface whenever the application’s state changes. It monitors changes in data-bound properties and updates to inform the viewer of model changes. Angular is designed with a tree structure for managing its components. It uses a change-detection strategy for better performance; two common strategies are the default change-detection strategy and the OnPush strategy.
87. What is lazy Loading, and how is it configured in Angular routing?
Ans:
- Lazy Loading in Angular can be configured in the routing module by specifying feature modules to be loaded on demand. This would be done by using the’ load children’ property in route configuration to point toward a module that should be lazily loaded.
- For example, this route definition could look like ‘{ path: ‘feature’, loadChildren: () => import(‘./feature/feature.module’).then(m => m.FeatureModule) }’.
- This setup would ensure that the actual feature module and its components are only loaded when the user navigates to that specific route. This would enhance the application’s overall performance and initial page loads.
88. What are the different ways to style Angular components?
Ans:
- There are several ways to style Angular components: inline styles, external CSS files, and Angular’s ViewEncapsulation. Inline styles are defined directly within the component’s decorator, whereas external styles are linked via CSS files.
- The Angular framework supports preprocessors such as SCSS, SASS, and LESS for styling requirements that are more complex.
- Three strategies, Emulated, None, and ShadowDom, can control how styles are applied and scoped using ViewEncapsulation.
- This flexibility lets developers choose the best styling approach depending on the application’s requirements.
89. What is RxJS observable chaining?
Ans:
RxJS enables developers to compose many operators together to influence and alter streams of data declaratively. Through the use of ‘map’, ‘filter’, ‘mergeMap’, and ‘concatMap’ operators, it creates complex data flows that respond to asynchronous events. The chaining of observables allows for clean, readable code structures, as each operation on the data stream is made by every operator before passing it on down the line. This simplifies the reuse of components and clearly separates concerns regarding asynchronous operations.
90. How is a global error handler implemented in Angular?
Ans:
A global error handler in Angular can be set up by defining a service that implements the ‘ErrorHandler’ interface. This allows us to determine the ‘handleError’ method, putting all error-handling logic in one location. To apply the global error handler, the service must be provided in an application’s root module, typically ‘app.module.ts’. It catches all the application’s unhandled errors, thus allowing the application to process these errors. Since it centralizes handling capability, an application is more robust and easier to maintain.