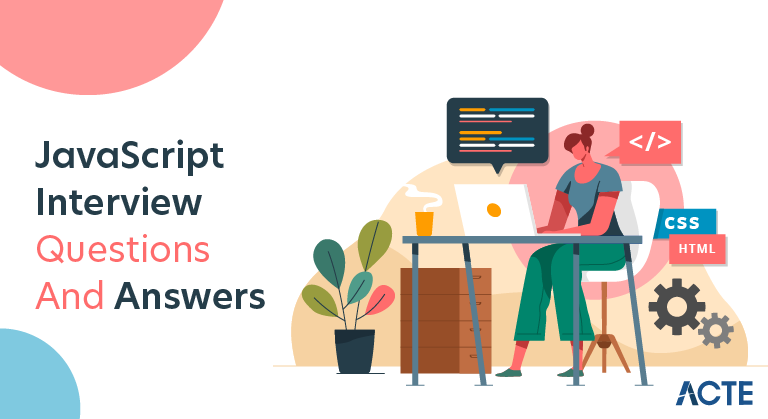
35+ [REAL-TIME] JavaScript Interview Question and Answer
Last updated on 03rd Jul 2020, Blog, Interview Questions
Programming languages such as JavaScript are very useful for making dynamic and interactive websites. By operating client-side, it makes features like dynamic content updates, interactive maps, and form validation possible without requiring page reloads. JavaScript is necessary for both front-end and back-end web development since it powers server-side applications via frameworks like Node.js. Its widespread community support, ease of integration, and ongoing development with new features and libraries are the main reasons for its popularity.
1. What is JavaScript, and how is it used in modern web development?
Ans:
The high-level, interpreted programming language JavaScript plays a crucial role in adding interactivity and dynamic behaviour to web pages. It is predominantly employed in front-end web development but also finds applications in server-side development (Node.js) and mobile app development. In essence, JavaScript is indispensable in modern web development, enabling developers to create rich and interactive user experiences.
2. What is JSON, and how is it used in JavaScript?
Ans:
JSON (JavaScript Object Notation) serves as a lightweight data-interchange format utilized to transmit data between a server and a web application. Within JavaScript, JSON data is formatted as a string and can be decoded into JavaScript objects through the `JSON.parse()` method. Conversely, JavaScript objects can be transformed into JSON format using the `JSON.stringify()` method. JSON facilitates data exchange between the server and client and is commonly used in AJAX requests and APIs.
3. How can JavaScript code be included within an HTML page?
Ans:
JavaScript code is integrated into an HTML page using the <script> tag. This tag allows developers to embed JavaScript directly within the HTML document. There are multiple ways to include JavaScript:
- Inline: By using the <script> tag directly within the HTML file
- Internal: By placing JavaScript code within a <script> tag inside the <head> or <body> section.
- External: By linking an external JavaScript file using the <script src=”path/to/file.js”></script> tag.
4. What are the different ways to declare variables in JavaScript?
Ans:
- Variables in JavaScript can be declared using `var`, `let`, or `const` keywords.
- `Var~ can be redefined and reassigned, and it has a function scope within its scope.
- `let` is block-scoped and can be reassigned but not redeclared within the same block scope.
- `const` is also block-scoped but cannot be reassigned after initialization, making it suitable for declaring constants.
5. What are the different data types in JavaScript?
Ans:
JavaScript has several data types:
- Primitive types: `number`, `string`, `boolean`, `null`, `undefined`, `symbol`.
- Non-primitive types: `object` (including arrays, functions, and objects created with constructors).
- Understanding data types is essential for effective data manipulation and type coercion in JavaScript.
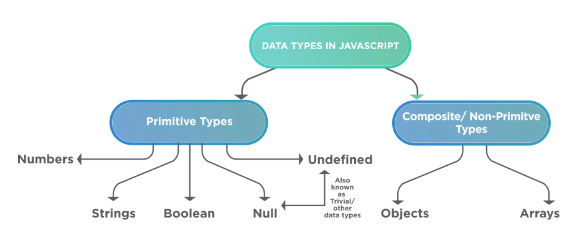
6. Explain the difference between == and === operators.
Ans:
- `==` is the equality operator that performs type coercion if the operands are of different types before comparing. It converts the operands to the same type before comparison.
- `===` is the strict equality operator that does not perform type coercion and checks for both value and type equality. It returns true only if the operands are of the same type and have the same value.
7. What is hoisting in JavaScript?
Ans:
Hoisting in JavaScript refers to the process during compilation where variables and function declarations are moved to the top of their respective scope. This occurs before the code is executed, enabling variables to be accessed before they are formally declared. It’s important to note that only the declarations themselves are hoisted, not their initial assignments. Hoisting helps understand the behaviour of JavaScript code, but it’s important to note that hoisting applies only to declarations, not initializations, and can lead to unexpected behaviour if not understood correctly.
8. What is the difference between let, const, and var in JavaScript?
Ans:
- `var` is function-scoped and can be redeclared and reassigned within its function scope. It is not block-scoped.
- `let` is block-scoped and can be reassigned but not redeclared within the same block scope.
- `const` is also block-scoped but cannot be reassigned after initialization, making it suitable for declaring constants. However, the properties of objects declared with `const` can still be modified.
9. Explain the concept of closures with an example.
Ans:
Closures are functions that maintain access to external scope variables even after the outer function has completed execution. Here’s an example:
- function outer() {
- var outerwear = ‘I am from the outer function’;
- function inner() {
- console.log(outerwear); // Inner function has access to outerwear
- }
- return inner;
- }
- var innerFunc = outer();
- innerFunc(); // Output: My home is in the outside function.
In this example, the `inner()` function forms a closure over the `outerVar` variable, retaining access to it even after the `outer()` function has completed execution.
10. Explain the difference between null and undefined.
Ans:
Feature | null | undefined |
---|---|---|
Definition | Represents intentional absence of any object value | Indicates a variable has not been assigned a value |
Assigned By | Explicitly assigned by developers | Default value when a variable is declared but not initialized |
Type | Object | Undefined (type itself) |
Behavior | Actively set to signify absence of value | Passive indication of absence or uninitialized state |
Use Cases | Explicitly indicate no value | Default value in uninitialized variables or properties |
11. How do you deal with JavaScript errors?
Ans:
In JavaScript, `try…catch` can be used to manage errors. ` blocks. The `try` block encloses code that could potentially generate an error, whereas the `catch` block is utilized to manage and respond to any errors that arise. The `finally` block can also be used to run code, regardless of whether an error occurs. Error handling is crucial in JavaScript to gracefully manage exceptions and prevent unexpected crashes in applications.
12. What are truthy and falsy values in JavaScript?
Ans:
- In JavaScript, values are considered truthy if they coerce to true when evaluated in a Boolean context.
- False values, on the other hand, coerce to false. Examples of falsy values include `false`, `0`, `”` (empty string), `null`, `undefined`, and `NaN`, while truthy values include non-empty strings, non-zero numbers, objects, arrays, and the Boolean value `true`.
- Understanding truthy and falsy values is essential for writing conditional statements and evaluating expressions accurately in JavaScript.
13. What is the purpose of the ‘this’ keyword in JavaScript?
Ans:
- In JavaScript, the `this` keyword pertains to the context in which a function is executed, with its value determined by the method of function invocation.
- In the global scope, `this` refers to the global object (e.g., `window` in browsers).
- Inside a function, `this` refers to the object on which the function is called (the receiver object) or the global object if the function is not called on any object.
- Understanding how the `this` keyword behaves is essential for effectively utilizing object-oriented programming patterns, managing event handling, and mastering asynchronous JavaScript programming.
14. Explain prototype-based inheritance in JavaScript.
Ans:
Prototype-based inheritance is a core principle object in JavaScript that inherits attributes and functions from other objects. Every object in JavaScript possesses an internal property known as `[[Prototype]]` (accessible via `__proto__` or `Object.getPrototypeOf()`), which points to another object referred to as its prototype. When gaining access to an object’s method or property, JavaScript checks the object itself first. If the property isn’t found, it then traverses up the prototype chain until it locates the property or reaches the end of the chain (typically `Object. prototype`).
15. What are JavaScript promises?
Ans:
In JavaScript, promises are objects that symbolize the eventual outcome—whether success or failure—of an asynchronous operation and the value it resolves to. They offer a more organized and easier-to-follow approach compared to callback-based asynchronous code. Promises operate in three states: pending while waiting for resolution, fulfilled when the operation succeeds, and rejected upon encountering an error. They can be chained using `.Use the `.catch()` and `then()` methods to handle error and success cases, respectively.
16. Describe event capturing and event bubbling. In the context of DOM event handling?
Ans:
- Event bubbling and event capturing are two mechanisms employed by the DOM for event handling.
- Event bubbling is the process where an event triggered on the most profound element within the DOM hierarchy will propagate upwards through its ancestors.
- Event capturing, on the other hand, is the opposite process, where the event is captured by the most profound element first and then propagated downwards through its ancestors.
- Event listeners can be attached during either the capturing or bubbling phase using the `addEventListener()` method.
17. What is JSONP, and how is it used in JavaScript?
Ans:
- JSONP (JSON with Padding) is a technique used to overcome the same-origin policy limitation in web browsers when making cross-origin requests using the `<script>` tag.
- It works by dynamically creating a `<script>` tag with a source URL that returns JSON data wrapped in a JavaScript function call.
- The server responds with JSON data padded with the specified callback function, allowing the data to be retrieved and executed in the client-side JavaScript environment.
18. What is a callback function in JavaScript?
Ans:
A callback function in JavaScript is a function passed as an argument to another function, designed to execute after completing a specific task or when a particular event happens. Callbacks are commonly used in asynchronous programming to handle responses from asynchronous operations such as AJAX requests, timers, and event handlers. They provide a way to ensure that particular code is executed only after specific tasks are completed, enabling non-blocking behaviour and better handling of asynchronous operations.
19. How do you handle exceptions in JavaScript?
Ans:
Exceptions in JavaScript are managed through `try…catch` constructs. Within the `try` block, code that may generate an error is enclosed, and any resulting errors are managed within the subsequent `catch` block. Furthermore, the `finally` block allows the execution of code independent of whether an error occurs. To handle specific error situations, developers can employ the `throw` statement to throw custom errors. Proper error handling is essential for writing robust and reliable JavaScript code, as it allows you to handle unexpected situations and provide meaningful feedback to users gracefully.
20. What are the advantages of using JavaScript frameworks like Angular, React, or Vue.js?
Ans:
- They provide a structured and organized way to build large-scale web applications.
- They offer powerful features for building user interfaces, including components, routing, and state management.
- They facilitate efficient DOM manipulation and virtual DOM rendering, leading to better performance and responsiveness.
- They have vibrant communities and ecosystems with extensive documentation, libraries, and plugins, making development faster and more efficient.
21. What distinguishes synchronous from asynchronous processes? Programming in JavaScript?
Ans:
Synchronous programming executes tasks sequentially, one after the other, blocking further execution until the current task is completed. However, asynchronous programming enables the execution of tasks. Concurrently, without waiting for each other to complete it. Asynchronous operations typically involve callbacks, promises, or async/await syntax, enabling non-blocking behaviour and improving responsiveness in applications.
22. Explain the concept of higher-order functions in JavaScript.
Ans:
Functions that take in other functions as arguments are known as higher-order functions. And return functions as results. They enable functional programming paradigms such as map, filter, and reduce, allowing for more expressive and concise code. Higher-order functions promote code reuse and abstraction, facilitating the development of modular and maintainable software. By leveraging higher-order functions, developers can encapsulate common patterns and behaviours into reusable functions, leading to cleaner and more declarative code.
23. What are immediately invoked function expressions (IIFE), and why are they used?
Ans:
Immediately Invoked Function Expressions (IIFE) are JavaScript functions that are executed immediately after they are defined. They are typically enclosed within parentheses to create a function expression, followed by an additional pair of parentheses to invoke the function immediately. IIFE is often used to create a new scope, encapsulate variables, and avoid polluting the global namespace.
24. What is the difference between a function declaration and a function expression?
Ans:
- – Function Declaration: Declares a named function employing the function name, the `function} keyword, and parameters.
- Function declarations are hoisted to the top of their containing scope and can be called before they are declared.
25. What are arrow functions, and how do they differ from regular functions?
Ans:
- Arrow functions are a concise syntax introduced in ES6 for writing anonymous functions.
- Arrow functions cannot be used as constructors and do not have their own `this`, `arguments`, `super`, or `new—target` bindings.
- Arrow functions are highly beneficial for concise and straightforward function writing, particularly suited for callback functions and function expressions.
- Nevertheless, it’s essential to recognize that arrow functions come with certain restrictions when compared to regular functions.
26. How do you create a class in JavaScript?
Ans:
In JavaScript, classes are constructed using the `class` keyword, providing a more concise syntax compared to the traditional prototype-based inheritance mechanism. A class can have a constructor method for initializing instance properties, as well as other methods for defining behaviour. Here’s an example:
- class MyClass {
- constructor(name) {
- this.name = name;
- }
- greet() {
- console.log(`Hello, ${this.name}!`);
- }
- }
- const obj = new MyClass(‘World’);
- obj.greet(); // Output: Hello, World!
27. What are the different types of scopes in JavaScript?
Ans:
JavaScript has two main types of scopes:
- Global Scope: Specified variables not included in any function are accessible globally throughout the program.
- Local Scope: Function-declared variables are confined to that function’s scope and cannot be accessed from outside. JavaScript also supports block scope with `let` and `const` declarations.
28. What are generators in JavaScript?
Ans:
Generators in JavaScript are unique functions capable of pausing and resuming their execution, enabling the lazy generation of a sequence of values. Generators offer a powerful tool for asynchronous programming, iteration, and infinite sequences. Unlike regular functions, generators maintain their internal state, allowing them to suspend and resume execution at any point. This makes generators particularly useful for implementing custom iteration behaviour, asynchronous control flow, and lazy evaluation of data.
29. Explain the concept of memoization and how it is implemented in JavaScript.
Ans:
Memoization is a technique used to improve the performance of functions by caching costly function calls’ outcomes and giving back the cached result when identical inputs are entered again. It can be implemented manually using objects or maps to store computed values for specific inputs or by using higher-order functions and closures to create memoized versions of existing functions. Memoization exploits the principle of referential transparency, where a function produces the same result for the same inputs, allowing for the reuse of previously computed results.
30. What are closures, and how do they work?
Ans:
- Closures are functions that retain access to variables from their containing lexical scope even after the scope has closed.
- As a result, the function can access and modify the variables even when it is executed outside of its original scope.
- Closures capture the variables themselves, not just their current values, enabling powerful patterns such as data encapsulation, private variables, and callbacks in JavaScript.
31. What is the purpose of the ‘new’ keyword in JavaScript?
Ans:
- The `new` keyword in JavaScript creates instances of user-defined object types, also known as constructor functions.
- When `new` is used with a constructor function, it initiates the creation of a new object.
- This process involves setting the object’s prototype to the constructor function’s prototype property and invoking the constructor function with `this` referring to the newly created object.
- This allows the constructor function to initialize the newly created object with properties and methods.
32. How do you implement inheritance in JavaScript?
Ans:
Inheritance in JavaScript can be implemented using prototype-based inheritance. Objects in JavaScript have an internal property called `[[Prototype]]` (accessible via `__proto__` or `Object.getPrototypeOf()`), which refers to another object called its prototype. To implement inheritance, you can set the prototype of a child object to be an instance of the parent object, allowing the child object to receive its parent’s methods and properties. ES6 introduced the `class` syntax, which provides syntactic sugar for defining constructor functions and setting up inheritance using the `extends` keyword.
33. What are mixins in JavaScript?
Ans:
Mixins are a design pattern in JavaScript that permits objects to inherit attributes and methods from multiple sources. Unlike traditional inheritance, mixins do not create a parent-child relationship between objects but instead, copy the methods and properties from one object to another. Composing functionality from multiple sources into a single object allows for greater flexibility and code reuse.
34. What are Symbols in JavaScript, and how are they used?
Ans:
- Symbols are a new primitive data type introduced in ES6 that represent unique identifiers.
- They are immutable and can be used as property keys in objects.
- Symbols are helpful in creating private or non-enumerable object properties, preventing name collisions in object properties, and implementing custom behaviour in built-in JavaScript objects like iterators and generators.
35. What is the difference between call, apply, and bind in JavaScript?
Ans:
- `call()` and `apply()` are methods available on all functions in JavaScript that allow you to invoke a function with a specified `this` value and arguments. The difference between them is in how arguments are passed: `call()` accepts individual arguments, while `apply()` accepts an array-like object of arguments.
- `bind()` is a method available on all functions in JavaScript that returns a new function with the `this` value bound to a specified object. Unlike `call()` and `apply()`, `bind()` does not immediately invoke the function but creates a new function with the specified context bound to it.
36. What is the role of the event loop in JavaScript?
Ans:
The event loop in JavaScript manages asynchronous operations like callbacks, timers, and I/O tasks, ensuring they are handled efficiently without blocking the main execution thread. It continuously checks the call stack for synchronous code execution and the task queue for asynchronous tasks to be executed. JavaScript utilizes synchronous code execution and manages asynchronous tasks through a task queue. When the call stack is cleared, the event loop selects the next task from the queue and places it on the call stack for processing.
37. How do you handle asynchronous operations in JavaScript?
Ans:
- Asynchronous operations in JavaScript can be handled using callbacks, promises, or async/await syntax.
- Functions that are supplied as inputs to other functions are called callbacks. It is called when an asynchronous operation completes.
- Promises are objects that represent the outcome, whether successful or failed, of an asynchronous operation.
- They offer a streamlined approach to managing asynchronous code through the use of `.then()` and `.catch()` methods.
38. What are WeakMap and WeakSet in JavaScript?
Ans:
- WeakMap and WeakSet are particular types of collections introduced in ES6 that hold weak references to the keys and values stored in them.
- Unlike regular Map and Set objects, WeakMap and WeakSet do not prevent the garbage collector from collecting keys and values that are no longer referenced elsewhere in the program.
- This makes them useful for scenarios where you want to associate metadata with objects without preventing the objects from being garbage-collected when they are no longer needed.
39. Explain the concept of event delegation in JavaScript.
Ans:
Event delegation is a JavaScript technique for handling the events of several components with a single event listener attached to a common ancestor element. Instead of attaching event listeners to individual components, event delegation takes advantage of event bubbling by listening for events at a higher level in the DOM hierarchy and then determining the target element that triggered the event.
40. What are Web Workers in JavaScript?
Ans:
Web Workers are a feature in JavaScript that allows scripts to run in background threads separate from the main execution thread of the web page. They enable concurrent execution of JavaScript code, allowing tasks such as heavy computations, network requests, and other CPU-intensive operations to be performed without blocking the main thread and affecting the responsiveness of the web page.
41. What are the differences between ES5, ES6 (ES2015), and ES2016 in JavaScript?
Ans:
- ES5: Introduced in 2009, ES5 is the fifth edition of the ECMAScript standard and includes features such as strict mode, JSON support, and methods like `forEach()`, `map()`, `filter()`, and `reduce()` for arrays.
- ES6 (ES2015): launched in 2015, represents a significant enhancement to the language, bringing forth notable additions, including arrow functions, classes, template literals, destructuring assignments, the `let` and `const` keywords, and promises.
- ES2016: Introduced in 2016, ES2016 is a smaller update that includes features such as the “ operator for exponentiation, `Array.prototype.includes()`, and the `Array.prototype.entries()` and `Array.prototype.keys()` methods.
42. What are decorators in JavaScript?
Ans:
- Decorators are a proposal for a new feature in JavaScript that allows you to attach metadata or behaviour to classes, methods, or properties using a declarative syntax.
- Decorators are currently a stage 2 proposal in the ECMAScript specification and are commonly used in frameworks like Angular and TypeScript to add functionality such as logging, validation, or caching to classes and their members.
43. What is currying, and how is it implemented in JavaScript?
Ans:
Currying is a functional programming technique that turns a function with several arguments into a list of functions. Each takes a single argument. Currying enables the partial application of a function, resulting in the creation of a new function where some arguments have already been specified. In JavaScript, currying can be implemented manually using closures or using built-in methods like `bind()`. For example:
- function multiply(a) {
- return function(b) {
- return a b;
- };
- }
- const multiplyByTwo = multiply(2);
- console.log(multiplyByTwo(3)); // Output: 6
44. What is a Promise chain in JavaScript?
Ans:
A Promise chain in JavaScript is a sequence of asynchronous operations chained together using promise methods like `.then()` and `.catch()`.Promises symbolize the eventual fulfilment or failure of an asynchronous task, enabling you to link multiple asynchronous operations clearly and sequentially. Each `.then()` callback in the chain receives the result of the previous operation as its argument and returns a new promise, enabling you to perform additional asynchronous operations or handle errors at each step in the chain.
45. What is the difference between ECMAScript and JavaScript?
Ans:
- ECMAScript is the official name of the scripting language standardized by ECMA International, while JavaScript is the most well-known implementation of ECMAScript.
- JavaScript is a programming language that operates at a high level and is interpreted according to the ECMAScript standard.
- Other implementations of ECMAScript include ActionScript (used in Adobe Flash) and JScript (used in Microsoft’s Internet Explorer).
46. Explain the concept of object cloning in JavaScript.
Ans:
- Object cloning in JavaScript refers to creating a copy of an existing object.
- There are two main approaches to object cloning: shallow cloning and deep cloning. Shallow cloning creates a new object with the original object’s characteristics, but the attributes themselves are not cloned.
- Deep cloning, on the other hand, recursively clones all nested objects and their properties.
- Object cloning can be implemented using methods like `Object.assign()` for shallow cloning and libraries like Lodash or custom functions for deep cloning.
47. How do you handle memory leaks in JavaScript?
Ans:
Memory leaks in JavaScript occur when objects are no longer needed but are still referenced, preventing them from being garbage-collected and leading to memory consumption over time. To handle memory leaks, it’s essential to identify and remove unnecessary references to objects, especially long-lived objects like event listeners, closures, and global variables. Techniques for preventing memory leaks include:
- Removing event listeners.
- Nullifying references to objects when they are no longer needed.
- Tools like Chrome DevTools can be used to profile memory usage.
48. What are ES modules, and how do they differ from CommonJS modules?
Ans:
ES modules (ESM) are a standardized module system introduced in ES6 (ES2015) for organizing and encapsulating code in JavaScript applications. ES modules use `import` and `export` statements to define dependencies between modules and expose functionality to other modules. Unlike CommonJS modules, which use `require()` and `module. Exports` syntax is synchronous; ES modules are asynchronous and support features such as static imports, named exports, default exports, and circular dependencies.
49. What is the purpose of the fetch API in JavaScript?
Ans:
The fetch API in JavaScript is a modern replacement for the XMLHttpRequest (XHR) object, giving users a more capable and adaptable method of sending HTTP requests from web pages. Fetch allows you to make network requests to fetch resources like JSON data, HTML, or images from a server using the `fetch()` function. It returns a promise that resolves with the response to the request, allowing you to handle the response asynchronously using `.then()` and `.catch()` methods.
50. What are the differences between map, filter, and reduce functions in JavaScript?
Ans:
- `map()`: Utilizing the `map()` method involves putting a callback function on each array element to generate a new array containing the results. Significantly, this operation does not alter the original array.
- `filter()`: The `filter()` method creates a fresh array by combining the components of an already-existing array that satisfy a specified condition, determined by a callback function. It does not modify the original array.
- `reduce()`: The `reduce()` method applies a callback function to each element of an array, reducing the variety to a single value. It takes an optional initial value and an accumulator that accumulates the results of each iteration.
51. What are the different ways to handle asynchronous code in JavaScript?
Ans:
- Callbacks: Functions that are passed as arguments to other functions and are executed upon the completion of asynchronous operations.
- Promises: Objects that represent the future completion or failure of asynchronous operations, offering a more organized and readable approach to handling asynchronous code through methods like `.then()` and `.catch()`.
- Async/await: A syntactic sugar for writing asynchronous code in a synchronous style using `async` functions and `await` keyword, making asynchronous code appear more sequential and readable.
52. What is lazy loading in JavaScript, and why is it important?
Ans:
Lazy loading is a method employed in web development to delay the loading of resources that are only immediately necessary once they are required. By loading resources asynchronously and on-demand, lazy loading can decrease bandwidth usage and speed up page loads. It is significant for optimizing the performance of web pages with large or complex content, such as images, videos, or scripts, allowing critical content to be loaded first while deferring the loading of secondary content until later.
53. What are design patterns, and how are they applied in JavaScript?
Ans:
Design patterns are reusable solutions to common problems encountered in software design and development. In JavaScript, design patterns are applied to solve specific design and architectural challenges, improve code organization, and promote best practices. Examples of design patterns commonly used in JavaScript include the Module pattern, Singleton pattern, Factory pattern, Observer pattern, and MVC (Model-View-Controller) pattern.
54. How do you implement single-page applications (SPAs) in JavaScript?
Ans:
- Single-page applications (SPAs) are web applications that initially load one HTML page and then update its content dynamically based on user interactions, all without requiring the entire page to reload.
- These applications often leverage JavaScript frameworks/libraries like Angular, React, or Vue.js.
- These tools facilitate routing, state management, and component-based architecture, enabling developers to create interactive and responsive user interfaces effectively.
55. What is WebAssembly, and how does it relate to JavaScript?
Ans:
WebAssembly (Wasm) is a binary instruction format enabling high-performance, low-level programming languages such as C, C++, and Rust to run within web browsers nearly as fast as native code. WebAssembly runs alongside JavaScript in the browser and can be integrated with JavaScript code using WebAssembly APIs, allowing developers to take advantage of existing libraries and tools written in other languages and achieve better performance for compute-intensive tasks such as gaming, multimedia processing, and virtual reality.
56. How do you optimize JavaScript code for better performance?
Ans:
- Minifying and compressing code to reduce file size and improve load times.
- Eliminating unnecessary code, such as unused variables, functions, and imports.
- Avoid unnecessary DOM manipulation and frequent reflows and repaints.
- Optimizing network requests and reducing latency by caching resources and using CDNs.
- Implementing lazy loading and code splitting to defer the loading of non-essential resources.
57. What are the best practices for reducing page load time in JavaScript applications?
Ans:
- Minimizing HTTP requests by combining and compressing assets.
- Using browser caching and leveraging CDNs to deliver content faster.
- Optimizing images and multimedia content for web delivery.
- Implementing lazy loading and code splitting to load resources asynchronously and on-demand.
- Minimize render-blocking resources and defer JavaScript execution when possible.
58. What are some common performance bottlenecks in JavaScript applications?
Ans:
- Excessive DOM manipulation and frequent reflows and repaints.
- Large and unoptimized JavaScript bundles that increase page load times.
- Memory leaks and inefficient memory usage, leading to increased memory consumption.
- CPU-intensive operations and long-running JavaScript code blocking the main thread.
59. How do you profile JavaScript code for performance optimization?
Ans:
JavaScript code can be profiled for performance optimization using browser developer tools, such as Chrome DevTools and Firefox Developer Tools, which provide built-in profiling and debugging capabilities. These tools allow you to analyze JavaScript execution, memory usage, rendering performance, network activity, and other performance metrics, identify performance bottlenecks, and optimize code for better performance. Profiling techniques include CPU profiling, memory profiling, network profiling, and timeline recording.
60. What tools can you use to measure the performance of JavaScript code?
Ans:
- Browser developer tools: Built-in tools provided by web browsers, such as Chrome DevTools and Firefox Developer Tools, which offer profiling, debugging, and performance monitoring features.
- Lighthouse: An open-source tool from Google for auditing and analyzing the performance, accessibility, and best practices of web pages, providing recommendations for optimization.
- WebPageTest: A free online tool for testing and measuring.
61. What is Angular, and how does it differ from other JavaScript frameworks?
Ans:
Angular, a front-end JavaScript framework created and supported by Google, is utilized for constructing single-page applications (SPAs). It offers a complete solution for client-side development, encompassing functionalities such as templating, data binding, routing, form management, HTTP client capabilities, and dependency injection. Angular differs from other frameworks like React and Vue.js in its use of a two-way data binding approach, opinionated structure, and a full-featured ecosystem that includes tools like Angular CLI for project scaffolding and management.
62. What are React hooks, and how are they used?
Ans:
React hooks are functions that enable you to utilize state and other React functionalities without the necessity of writing a class. They were introduced in React 16.8 as a way to reuse stateful logic across components. Hooks such as `useState`, `useEffect`, and `useContext` enable functional components to manage local states, perform side effects, and access context without needing to use class components or higher-order components.
63. What is Vuex in Vue.js, and what problem does it solve?
Ans:
Vuex is a library and state management pattern designed for Vue.js applications. It acts as a centralized repository for all components within an application, ensuring consistent state management and enabling seamless communication between components. Vuex solves the problem of managing shared state and communication between components in large Vue.js applications.
64. What are the lifecycle hooks in React, and how are they used?
Ans:
- React lifecycle hooks are methods invoked at various stages in a React component’s lifecycle.
- They allow developers to perform actions such as initializing the state, fetching data, updating the DOM, and cleaning up resources in response to component lifecycle events.
- Some of the most commonly used lifecycle hooks in React include `componentDidMount`, `componentDidUpdate`, `componentWillUnmount`, and `shouldComponentUpdate`.
65. What are Vue components, and how are they used?
Ans:
- Vue components are reusable and self-contained units of UI functionality in Vue.js applications.
- They encapsulate HTML, CSS, and JavaScript code into a single file, making it easier to organize and maintain complex user interfaces.
- Vue components can have their state, methods, and lifecycle hooks, allowing for modular development and code reuse.
- Components can be arranged hierarchically, nesting within one another to form a structure where data and events propagate downwards and upwards through the component tree.
66. How do you handle routing in Angular applications?
Ans:
Routing in Angular applications is handled using the Angular Router module. Angular Router provides a client-side router that allows for navigating between different views or pages within a single-page application (SPA). It enables developers to define routes using the `RouterModule.forRoot()` method, configure routes with path and component mappings, and navigate between routes using router links, route parameters, and programmatic navigation methods such as `router.navigate()`.
67. What is Redux, and how does it relate to React?
Ans:
Redux serves as a reliable state container designed for JavaScript applications, often paired with React, to manage application states effectively. It establishes a centralized store that comprehensively holds the application’s state, allowing modifications solely through action dispatching. React components integrate with the Redux store via the `connect` function or `useSelector` hook to retrieve state and dispatch actions.
68. What is JSX, and how is it used in React?
Ans:
- JSX (JavaScript XML) extends JavaScript syntax by enabling the incorporation of HTML-like code directly into JavaScript files.
- This feature offers a concise and recognizable format for defining React elements and organizing component structures using XML-like syntax.
- JSX is transpiled into plain JavaScript by tools like Babel, allowing React components to be described using a combination of JavaScript logic and HTML-like markup, which is more expressive and easier to understand than creating elements using `React.createElement()`.
69. How do you handle state management in Vue.js?
Ans:
- State management in Vue.js applications can be handled using Vuex, Vue’s official state management library, or by managing the state locally within components using Vue’s reactive data system.
- Vuex offers a centralized store to manage application states, incorporating elements like states, getters, mutations, and actions.
- These facilitate structured access and modification of the state, ensuring predictability in state management.
- Alternatively, Vue’s reactivity system allows components to define and reactively update their local state using the `data` option or `reactive` API.
70. What are Angular directives, and how are they used?
Ans:
Angular directives are a powerful feature that allows you to extend HTML with custom behaviour and functionality. Directives in Angular serve various purposes, such as creating reusable components, manipulating the DOM, attaching event listeners, and interacting with data within Angular templates. Angular provides built-in directives such as `ngIf`, `ngFor`, and `ngModel` for everyday tasks like conditional rendering, list rendering, and two-way data binding.
71. What tools can you use to debug JavaScript code?
Ans:
- Visual Studio Code Debugger: Integrated debugger in Visual Studio Code that allows you to debug JavaScript code directly within the code editor, with support for breakpoints, variable inspection, and step debugging.
- Node.js Debugger: Built-in debugger in Node.js that allows you to debug JavaScript code running in Node.js environments using commands like `node inspect` or `node –inspect-brk`.
- Remote Debugging: Tools like Weinre or Chrome Remote Debugging Protocol allow you to debug JavaScript code running on remote devices or browsers from a local development environment.
72. How do you write unit tests for JavaScript code?
Ans:
Unit tests for JavaScript code can be written using testing frameworks like Jest, Mocha, Jasmine, or QUnit. Unit tests usually concentrate on testing discrete code segments, like functions or procedures, separately from the broader application context. Tests are written using assertions to verify the behaviour and output of the code under test. Standard testing techniques include test-driven development (TDD), where tests are written before the code, and behaviour-driven development (BDD), where tests are written based on desired behaviours.
73. What is Test-Driven Development (TDD), and how does it apply to JavaScript?
Ans:
- Writing a test that fails.
- Composing the code required to pass the evaluation.
- Refactoring the code while ensuring all tests continue to pass.
TDD encourages developers to think about the requirements and behaviour of their code upfront, leading to better-designed, more modular, and more maintainable code. TDD can be applied to JavaScript development using testing frameworks like Jest, Jasmine, or Mocha, combined with tools like Karma or Jest for running tests in different environments.
74. What is linting, and what tools are available for linting JavaScript code?
Ans:
Linting involves examining code to detect potential errors, stylistic inconsistencies, and violations of best practices. Linters are tools designed to enforce coding standards and pinpoint issues in code before they lead to problems. In the realm of JavaScript, notable lines include:
- ESLint: This highly adaptable tool is tailored for JavaScript code, offering support for plugins and customizable rule sets. It enables developers to uphold coding standards rigorously and identify prevalent errors effectively.
- JSHint: A lightweight linter for JavaScript code that focuses on detecting potential errors and enforcing coding conventions using predefined or custom rules.
- JSLint: A strict linter for JavaScript code developed by Douglas Crockford, with a focus on enforcing a specific coding style and catching common programming mistakes.
75. How do you profile JavaScript code for performance optimization?
Ans:
JavaScript code can be profiled for performance optimization using browser developer tools like Chrome DevTools or Firefox Developer Tools, which provide built-in profiling and performance monitoring features. These tools allow you to analyze JavaScript execution, memory usage, rendering performance, network activity, and other performance metrics. Profiling techniques include CPU profiling, memory profiling, network profiling, and timeline recording.
76. What are the common security vulnerabilities in JavaScript?
Ans:
- Cross-Site Scripting (XSS): Attackers inject malicious scripts into web pages viewed by other users.
- Cross-Site Request Forgery (CSRF): Attackers trick users into executing unintended actions on a web application in which they are authenticated.
- Injection Attacks: Attackers inject malicious code into an application to execute unauthorized commands or access sensitive data.
- Insecure Direct Object References (IDOR): Attackers manipulate references to objects in an application to access unauthorized resources.
77. How do you prevent XSS attacks in JavaScript?
Ans:
- Sanitize input data: Filter and validate user input to remove or escape potentially dangerous characters.
- Use Content Security Policy (CSP): Define a strict CSP to restrict the sources from which scripts can be loaded and executed.
- Encode output data: Encode user-generated content using functions like `encodeHTML()` or `encodeURIComponent()` before rendering it in HTML.
- Use secure cookies: Set the `HttpOnly` and `Secure` flags on cookies to prevent them from being accessed by client-side JavaScript.
78. What is a Content Security Policy (CSP), and how does it improve security in JavaScript applications?
Ans:
Content Security Policy (CSP) is a security measure designed to mitigate XSS (Cross-Site Scripting) attacks by regulating the origins from which content is permitted to load and run on a web page. CSP allows website owners to define an allowlist of trusted sources for scripts, stylesheets, images, fonts, and other resources. This helps mitigate the risks of XSS attacks by preventing malicious scripts from being injected into a web page and executed.
79. How do you prevent CSRF attacks in JavaScript applications?
Ans:
- Use CSRF tokens: Create distinct tokens for every user session and incorporate them into AJAX queries or forms. Check the token’s validity on the server side and verify the token to ensure that requests are coming from legitimate users.
- Use anti-CSRF headers: Set custom HTTP headers like `X-Requested-With` or `Origin` to identify legitimate requests and block cross-origin requests that do not include these headers.
- Implement secure authentication: Use secure authentication mechanisms like session cookies with secure and HttpOnly flags and enforce strong password policies to protect user accounts from unauthorized access.
80. What is CORS, and how does it affect JavaScript applications?
Ans:
Cross-Origin Resource Sharing (CORS) is a security feature that controls which resources a web page can request from another domain. CORS is implemented by web browsers to restrict unauthorized websites from initiating requests to different domains on behalf of users, thus preventing malicious activities. JavaScript applications running in a browser are subject to CORS restrictions when making cross-origin requests using techniques like AJAX or Fetch API.
81. What are vendor prefixes, and why are they used in CSS and JavaScript?
Ans:
Vendor prefixes are prefixes added to CSS properties and JavaScript APIs by browser vendors to implement experimental or non-standard features before W3C or ECMAScript standardizes them. Vendor prefixes are used to allow developers to use new features in their code while they are still in development or under consideration. Typical vendor prefixes include `-webkit-` (for WebKit-based browsers like Chrome and Safari), `-moz-` (for Mozilla-based browsers like Firefox), and `-ms-` (for Internet Explorer).
82. What is the compatibility of JavaScript across different browsers?
Ans:
JavaScript is a widely supported programming language that is executed by web browsers to provide dynamic and interactive functionality on web pages. JavaScript compatibility across different browsers is generally high, with most modern browsers supporting the ECMAScript standard and providing consistent implementations of JavaScript APIs. However, variations in browser versions, rendering engines, and JavaScript engines can lead to differences in behaviour and performance.
83. How do you handle browser compatibility issues in JavaScript?
Ans:
- Feature detection: Detect the availability of specific features or APIs before using them in code using techniques like `typeof`, `in` operator, or `Object.hasOwnProperty()`.
- Polyfills: Use polyfills or shims to provide fallback implementations for missing or unsupported features, allowing code to run consistently across browsers.
- Transpiration: Transpilers like Babel can be used to convert modern JavaScript code using ES6+ syntax into backwards-compatible code targeting older browsers.
- Vendor prefixes: Use vendor prefixes for CSS properties and JavaScript APIs to ensure compatibility with specific browser versions or rendering engines.
84. What are Web APIs, and how are they used in JavaScript?
Ans:
Web APIs (Application Programming Interfaces) are interfaces provided by web browsers to interact with browser features and functionality using JavaScript. Web APIs empower developers to interact with and control diverse elements of web pages and browser environments. This includes tasks such as manipulating the DOM, making AJAX requests, enabling multimedia playback, accessing geolocation data, managing storage, and handling additional functionalities.
85. What is the difference between localStorage and sessionStorage in JavaScript?
Ans:
- localStorage: Data stored using localStorage persists across browser sessions and tabs and has no expiration time. Data stored in localStorage persists across all windows and tabs originating from the same source, ensuring accessibility even after closing and reopening the browser.
- sessionStorage: Data stored using sessionStorage is accessible only within the same browser tab or window that created it and is cleared when the tab or window is closed. Data stored in sessionStorage is specific to each tab or window and is not shared across different tabs or windows from the exact origin.
86. How do you detect the user’s browser using JavaScript?
Ans:
You can detect the user’s browser using JavaScript by accessing the `navigator` object and examining its properties. Here’s an example of how to detect the user’s browser:
- const userAgent = navigator.userAgent;
- if (userAgent.includes(‘Chrome’)) {
- // The user is using Google Chrome
- } else if (userAgent.includes(‘Firefox’)) {
- // The user is using Mozilla Firefox
- } else if (userAgent.includes(‘Safari’)) {
- // The user is using Apple Safari
- } else if (userAgent.includes(‘Edge’)) {
- // The user is using Microsoft Edge
- } else if (userAgent.includes(‘Opera’) || userAgent.includes(‘OPR’)) {
- // The user is using Opera
- } else {
- // The user’s browser is not recognized
- }
87. How do you handle cross-origin requests in JavaScript?
Ans:
Cross-origin requests in JavaScript can be handled using techniques like CORS (Cross-Origin Resource Sharing) or JSONP (JSON with Padding). CORS enables web servers to define which origins can access their resources, whereas JSONP circumvents the same-origin policy by fetching scripts from alternative domains. Additionally, server-side proxies can be used to forward cross-origin requests from the client to the server, effectively circumventing the same-origin policy.
88. What are service workers, and how do they work in JavaScript?
Ans:
Service workers are JavaScript files that run in the background of web browsers and act as proxy servers between web applications, the browser, and the network. They enable features like offline caching, push notifications, and background synchronization, allowing web applications to work reliably even when the network is unavailable. Service workers are event-driven and run independently of web pages, making them suitable for handling tasks that require persistent background processing and network communication.
89. What is the Geolocation API in JavaScript, and how is it used?
Ans:
- The Geolocation API, offered by web browsers, enables web applications to retrieve geographical location information (latitude and longitude) from the device using JavaScript.
- This API includes methods such as `getCurrentPosition()` and `watchPosition()` to obtain the device’s current location or monitor changes in its location over time.
- Geolocation data serves diverse purposes in web applications, including location-based services, mapping, navigation, weather forecasts, and local search functionalities.
90. What is the purpose of the fetch API in JavaScript?
Ans:
The fetch API is a modern JavaScript API for making asynchronous HTTP requests in web applications. It provides a more flexible and powerful alternative to traditional XMLHttpRequest (XHR) for fetching resources from servers or APIs. The fetch API uses Promises to handle asynchronous operations and provides a simple and consistent interface for making requests and handling responses. Fetch requests support various options and configurations, including custom headers, request methods (GET, POST, PUT, DELETE, etc.), request bodies, and response handling using the `then()` and `catch()` methods.