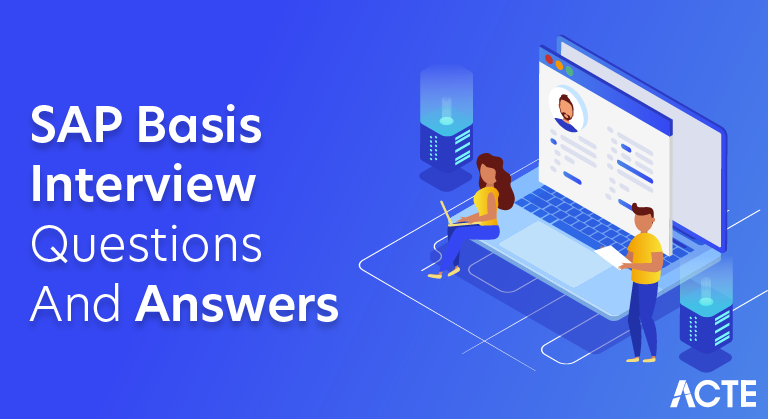
45+ [REAL-TIME] Django Interview Questions and Answers
Last updated on 30th May 2024, Popular Course
Django is a high-level Python web framework that enables developers to build web applications quickly and efficiently. It follows the “Don’t Repeat Yourself” (DRY) principle and emphasizes rapid development, clean design, and pragmatic solutions. Django provides a robust set of features and tools, including an ORM (Object-Relational Mapper) for interacting with databases, a powerful URL routing system, a template engine for generating dynamic HTML content, and a built-in admin interface for managing application data.
1. What occurs when a normal Django website receives a request?
Ans:
The Django Server receives the same request when a user types a URL into the browser. The server then checks its URL-config to see if the requested URL matches, and if it does, it returns the view function that goes with it. If any data is needed, it will then request it from the application’s model and give it to the relevant template, which is subsequently presented in the browser; if not, a 404 error is produced.
2. Why is the loosely linked framework known as Django?
Ans:
- Because Django is built on top of MTV architecture, it is said to be a loosely coupled framework.
- MVC architecture is a variation used in Django’s architecture, and MTV is beneficial since it keeps server code and client computer entirely apart.
- The client computer has Django’s Models and Views installed.
- Only templates—basically HTML and CSS code with the necessary data from the models—are sent back to the client.
- Because these components are so dissimilar, front-end and back-end developers can work on the project concurrently, and any changes made to one would hardly affect the other.
- Django is referred to as a loosely linked framework as a result.
3. Describe the contents of the settings.py file.
Ans:
Including the data and settings it contains. Upon startup, the Django server searches for settings.py. It is the primary settings file for your web application, hence its name and settings. Everything contained in this file is a list or dictionary of all the information related to your Django project, including databases, backend engines, middleware, installed applications, main URL configurations, static file addresses, templating engines, authorized hosts and servers, and security keys.
4. Could you describe how to configure a database in Django?
Ans:
The command edit mysite setting.py can be used to modify Django settings; it is a standard Python module with the module level set to Django. SQLite is the default database used by Django; as it is user-friendly, no additional installation is necessary. If your selection of database differs from what you want to match your database connection settings, the following keys must be included in the DATABASE “default” item.
5. What is the different between Django Models and Django Views?
Ans:
Aspect | Django Models | Django Views |
---|---|---|
Purpose | Define the structure of the application’s data | Handle the logic for processing requests and returning responses |
Function | Represent database tables and manage data | Map URLs to functions or classes that generate responses |
Location | Typically found in `models.py` | Typically found in `views.py` |
Components | Fields, Meta options, methods (e.g., save, delete) | Functions or classes, decorators, HTTP methods (e.g., GET, POST) |
6. Describe the contents of the Django templates. How is Django Templating implemented?
Ans:
The template is just a plain text document. It can be created using any text-based format, including HTML, CSV, and XML. A template has tags (% tag %) that govern its logic and variables that are substituted with values when the template is evaluated. Django templating The Django framework manages templating using engines. Certain template syntaxes are available that specify variables, comments, filters, and control logic.
7. Could you explain how Django uses the session framework?
Ans:
The session framework in Django lets you store and retrieve any kind of data according to the visitors to each site. It keeps information on the server side and obfuscates both cookie sending and receiving. Middleware can be utilized to implement sessions. Models of proxies: If all you want to do is alter the model’s Python-level behavior without altering the model’s fields, you can use this model.
8. Describe the Django migration process and how to use SQL.
Ans:
- In Django, making modifications to your models—such as removing a model or adding a field—into your database schema is known as migration.
- You can use several commands to work with migrations.
- Migrations of Migrants sqlmigrate You must print the SQL statement for resetting sequences for a specified app name in order to perform the migration in SQL.manage.py. sqlsequencreset
- Use this command to create SQL that corrects situations in which a sequence is not in sync with automatically incrementing field data.
9. How do Unicode and UTF-8 relate to each other?
Ans:
Unicode is a global encoding standard compatible with many scripts and languages. It is made up of characters from all over the world represented by letters, numbers, or symbols. You can transfer Unicode strings across a network or save them in files by using UTF-8, a sort of encoding that converts Unicode code points into byte form.
10. What are the Django inheritance styles listed?
Ans:
There are three different inheritance styles in Django.
Base classes abstract: This approach is used when you simply want to keep the information in the parent’s class and don’t want to spell it out for every child model.
Concrete/Multi-table Transference: As with Python classes, concrete inheritance operates by deriving from the base class. But with Django, this basis A different table will be mapped for each class. You need to do an implicit join each time you access basic fields. Horrible performance results from this.
11. Distinguish between the `static` and `media` files in Django.
Ans:
`media` files are user-uploaded files like documents or photographs, whereas `static` files are CSS, JavaScript, or image files used for style and scripting. Usually, admins or users can upload media files. MEDIA_ROOT and STATIC_ROOT should typically be two different folders. Remember that the management operation collects static and will save all static files it finds in STATIC_ROOT.
12. What is WSGI?
Ans:
- Since Django is a web framework, it requires a web server to function.
- Furthermore, we require an interface to enable such a connection because the majority of web servers do not speak Python natively. Herein lies the role of WSGI.
- The primary Python standard for facilitating communication between Web servers and applications is called WSGI, yet it is limited to synchronous coding.
- Examples of WSGI servers are Guniocrn, uWSGI, and so on.
- Note: Django is starting to become capable of asynchronous code. Withion 3.0, Django can now be uspplications as an ASGI (Asynchronous Server Gateway Interface).
13. What do you mean by fixtures?
Ans:
When you first set up an app, it can be helpful to pre-populate your database with hard-coded data. Initial data might be supplied together with fixtures. Nonetheless, the following is an illustration of how a Person model fixture may appear in JSON.
14. An explanation of middlewares and how they labor.
Ans:
Hooks to change the Django request or response object are called middlewares. It’s a simple, low-level “plugin” mechanism that allows you to change Django’s input or output worldwide. If you would like to change the request—that is, the HttpRequest object that is passed to the view—you can use middleware. Alternatively, you may choose to edit the HttpResponse object that was obtained from the view.
15. Describe signals and how to apply them. Which two parameters in signals are crucial?
Ans:
- Through signals, specific senders can inform a group of recipients about a particular action.
- When numerous pieces of code might be interested in the same events, they are extremely helpful.
- The following are some of the most popular signals from these models:
- pre_save/post_save: This signal is generated before or following the save() method.
- pre_delete/post_delete: This signal is thrown prior to and following the deletion of a model’s instance (method delete()).
- pre_init/post_init: This signal is activated either prior to or subsequent to the init() method of a model.
16. In what way will your Django project incorporate an old database?
Ans:
You must first specify the name of the database and your database connection options to Django. For the ‘default’ connection, do it by modifying the DATABASES option and setting values for the following keys. An existing database can be examined through the use of inspectdb, a tool included with Django. Using the output of the same script, create the models.py file: $ python manage.py inspected > models.py
17. How to install static files on your production server is explained in Mentions.
Ans:
- Using the same server to serve both your static files and the website: • Update the deployment server with your code.
- To copy every static file into STATIC_ROOT, execute collectstatic on the server.
- Set up your web server so that STATIC_URL is used to access the files in STATIC_ROOT.
- Using a dedicated server to provide your static files and the website
18. What is Templating by Jinja?
Ans:
Django includes one fairly strong templating engine by default and supports several popular template engines. The most recent iteration of Jinja Templating, available as of Jinja 2, is a highly well-liked Python templating engine. Several aspects of Jinja templating make it superior to Django’s built-in template system.
19. Describe Django and some of its main characteristics.
Ans:
The high-level Python web framework Django is well-known for emphasising the simplification of difficult jobs and adhering to the paradigms of “model-template-views” (MTV) and “Don’t Repeat Yourself” (DRY). It is also well known for its robustness, quick development, and extensive package ecology.
20. Describe Django’s MTV (Model-Template-View) Components pattern.
Ans:
The MVT (Model-View-Template) design, which is the foundation of Django, is almost the same as the MVC (Model-View-Controller) paradigm that is more widely used. The essential elements of Django’s MVT are broken down as follows:
MVT Parts :
- Model: in charge of business logic and data access (akin to MVC). .. In Django, a model is usually a Python class that symbolizes a table in a database.
- View (akin to MVC: in charge of data presentation): manages user input, responds to queries, and returns pertinent information. The logical flow of the program is handled by the view, which is more like a controller in Django versions more recent than that.
- Template: In charge of the user interface and presentation (akin to MVC). An HTML file that uses Django’s templating language to render data dynamically is called a template.
21. How does MTV define the Request-Response Lifecycle?
Ans:
- Client Request: When a user clicks on a link in a web browser, for instance, they are starting the action.
- URL Dispatcher: The URL dispatcher transfers an incoming URL to a matching function (in more recent versions, the ‘path’ or ‘re_path’ function).
- View Processing: The view carries out any required logic, such as using models to retrieve data from the database.
22. Can you explain how MVC and MTV are related?
Ans:
Model: Represents data and business rules while tightly adhering to the MVT and MVC principles.
View vs Controller: In MVT, the view is more akin to the conventional controller idea. This is because it manages the application’s flow, handles incoming requests, and communicates with models as needed.
View vs Template: MVT view Template vs. View: The MVT view, which shows data to the user, is comparable to the MVC view. If the MVC view is more passive and only shows controller-provided data, the MVT view, on the other hand, additionally handles user requests. Similar to the MVC view, the MVT template concentrates on the display layer.
23. What distinguishes a Django project from a Django app?
Ans:
The high-level framework used to create a web application is called Django Project. It includes numerous parts, including settings, URLs, and the ability to host several apps. On the other hand, a Django App adheres to the notion of “application” as a separate module intended to support a particular functionality or business sector. It is a collection of connected characteristics.
24. Explain the function of a Django project’s settings.py file.
Ans:
A Django project’s settings.py file is essential for setting up the project’s apps and external resources. It also enables control and customization of the Django project in several areas.
Important Elements :
- Global Settings: Controls configurations for the entire project, including middleware, URLs, installed apps, and more.
- Dynamic Configuration: Offers many configurations for development, testing, and production environments and secures critical data by using environment variables.
25. What does settings.py mean in a Django project?
Ans:
- Centralised Configuration: Makes sure all settings are easily accessible and does away with the necessity for dispersed configuration files.
- Adaptability: Its adaptability allows it to be adjusted to different deployment circumstances and project requirements.
- Consistency: Encourages consistent configuration settings during the stages of development, testing, and production.
- Version Control: Monitoring changes to this file in multi-developer setups guarantees that all developers are using the same configuration.
26. Could you explain any Example Use-Cases in Django’s settings.py file?
Ans:
- Installed Applications: o Locating programs particular to a project for functionalities like REST APIs and authentication, among others.
- Middleware: Global management of requests and responses, such as authentication or CORS.
- Database Configuration: Selecting the destination database and establishing the main connections to the database.
- Unchanging & Media Files: Setting up file storage for material that users have uploaded.
- Internationalisation: Setting up and enabling support for many languages.
27. What function does a Django project’s urls.py file serve?
Ans:
- The urls.py file is essential to Django’s routing and view mapping. Each application usually has its own urls.py file for handling modular URLs.
- App URLs versus Global URLs Global (project. urls): Paths to different apps are typically included in the project’s primary urls.py file.
- Local (app.urls): Each app’s urls.py file manages its unique URL mappings.
- URL patterns, specified with path() or re_path(), are used in all urls.py files.
- For basic text-based URLs, use path().
- re_path(): For regular expression-based complicated URLs.
- Providing Views with URLs
- The view argument of the path() method usually refers to the associated view function. Additional data, such as query parameters or URL fragments, can also be sent.
28. What Are the Main Ideas of Django’s ORM?
Ans:
- Model classes are Python classes with Django as their ancestor.model_db.Model. Each class attribute represents a database field.
- Field Types: Django offers several field types (including CharField, IntegerField, and ForeignKey) to accommodate a variety of data needs.
- Relationships between Models: Models may be related to one another in a many-to-many or one-to-many configuration, for instance.
- Manager Methods: Model Objects. The manager class provides database access utility operations. At least one manager, usually called an object, exists for each model.
- QuerySet Methods: A model’s object attribute provides a QuerySet. Before evaluation, this can be sorted, filtered, and worked with in various ways.
29. What Are the Advantages of Using ORM?
Ans:
- Mobility & Flexibility: Programs built on the ORM’s foundation can easily switch between many database backends.
- Security: Common security risks like SQL injection are guarded against by the ORM.
- Productivity: Shorter development cycles are achieved by reducing the requirement for complex database-specific code at higher abstraction levels.
30. What is the definition of a Django model?
Ans:
Django models provide the architectural basis of database-driven applications. A model has all the necessary attributes and behaviors and functions as a data structure. Model Defined – A Python subclass of Django is called a model class.model_db.Model. Each attribute in the class represents a database field. The database table name, any data linkages, metadata, and data manipulation techniques are all specified by the model class.
31. What Are the Essential Django Model Components?
Ans:
Domains :
- Meaning: Act as the columns of the table.
- Types: DateField, CharField, etc.
- Primary Key: id can be modified or is the default value.
- Descriptors: List field characteristics such as blank and null.
- Alternatives Meta
- table_name: Name of a database table.
- unique_together: A list of tuples representing fields that, when taken as a whole, ought to be unique.
- The order of results is set to default.
- Techniques
- Items: Personal information managers.
- __str__(): Admin’s human-readable instance name, among other things.
32. Explain the function of the admin interface in Django.
Ans:
CRUD (create, read, update, and delete) actions for your application’s models are just a few of the common data administration chores that can be easily completed with the robust Django admin interface.
Important Elements :
- Fast Implementation: Offers pre-built data management solutions with little setup required.
- Model-Centric Interface: The data models in your application determine how operations are arranged.
- DRY (Avoid Duplicating Yourself) Philosophy: The admin interface automatically updates when you make changes to your models.
- Typical Administrative Tasks
- Records in the database: Access, add, amend, and remove records.
33. When to Utilise the Admin Interface and When Not to
Ans:
When to Apply
- Rapid prototyping: To obtain early feedback or demonstrate a concept.
- Administrative Tasks: For internal instruments or in the first phases of a project.
- Ad hoc data adjustments or debugging: Quick fixes for data.
34. Describe a Django view and explain how it’s made.
Ans:
A Django view serves as a web request endpoint. It takes information from a database, modifies it as necessary, and then sends back a response that could be an HTML page, a reroute, or a JSON object. Function-Based Views (FBV) are one type of view that is made utilizing functions. Class-Based Views (CBV): These offer a more structured approach and are generated using classes. They frequently come with built-in functionality like view mixins and HTTP method processing.
35. Describe how Django’s URL patterns work.
Ans:
URL patterns in Django send online requests to the relevant view so they can be processed. They might be regular expressions or plain strings, and they are defined in each application’s urls.py file. Typical URL Patterns Fundamental Syntax: An inbound request path matched by a string is called a URL pattern. In order for a match to happen, the urls.py URL pattern has to be customized for several databases, including MySQL and PostgreSQL.
36. Explain the differences between the `classmethod` and `staticmethod` in Django.
Ans:
While `static method` is used to define methods that are independent of the class and its instances, without access to class or instance variables, `class method` is used to define methods that operate on the class itself, getting the class as the first parameter (`cls`). These are utility-style methods that operate on certain parameters after receiving them. On the other hand, class is a required argument for class methods. In Python, we may define class methods using the class method decorator and static methods using the static method decorator.
37. Describe the distinction between a QuerySet’s `filter()` and `exclude()` methods in Django.
Ans:
Objects that meet the provided lookup parameters are returned by `filter()}, whereas objects that do not match the parameters are returned by `exclude()}. A new QuerySet with objects that match the specified lookup parameters is returned by filtering with kwargs. A new QuerySet with objects that don’t match the specified lookup parameters is returned by the exclude(kwargs) function.
38. Describe the distinction between a ManyToManyField and a ForeignKey in Django models.
Ans:
- Relationships in Django models can be created with a ForeignKey or a ManyToManyField, each with its function.
- When there is a ForeignKey, relationships are many-to-one. This technique is employed when every record in the current model needs to be connected to precisely one record in a different model.
- For instance, a ForeignKey pointing to the Publisher model is used in the Book model to indicate that there is only one Publisher for a given book.
39. In Django, what is a QuerySet, and how is it used?
Ans:
A group of database queries that can be chained together and run slowly is referred to in Django as a QuerySet. It offers a simple method for Python to obtain and modify data from the database.
QuerySet Fundamentals
- Initialization: When you use a Django model’s Manager to communicate with it, QuerySets are automatically produced.
- Chaining: Before the QuerySet is evaluated, a number of procedures can be chained together.
- Lazy Evaluation: Only when data is needed, such as iterating over the results or specifically invoking evaluation functions like list() or count(), are QuerySets processed.
- Unchangeability Following Evaluation: The outcomes of a QuerySet cannot be changed once it has been reviewed. For example, once a list is created, it cannot be expanded.
40. In Django, what is a QuerySet Method, and how is it used?
Ans:
QuerySet Techniques Information Recovery
- all(): Gives back the query set’s whole object count.
- get(): Returns a single item that satisfies the given parameters.
- filter() Provides a subset of objects that meet predetermined standards.
- exclude(): Provides a list of items that don’t fit the given parameters.
- Data Fabrication
- create(): Produces a new object instantaneously and stores it in the database.
41. How to Set Up a Django View
Ans:
- Specify the View Class or Function: This means defining the view’s distinct logic. For a function-based view, this is an HTTP method and a Python function with the @ decorator. You define a class for a class-based view that has distinct method names for various HTTP methods.
- Set the View’s URL mapping: For a view to be accessible, it needs to be linked to a specific URL pattern. The urls.py file orchestrates this. For basic or sophisticated URL matching, you can use path or re_path, accordingly.
- Handle the Request and Produce a Response: The HTTP request is received by the view and parsed as required. The response must comply with the view’s criteria; this may include returning a certain data format (e.g., JSON), redirecting the user, or rendering a template.
42. A web API: what is it?
Ans:
An underlying database’s specific sections are made available through a group of endpoints known as a web API. As developers, we control the URLs for each endpoint, the underlying data that is accessible, and the actions that can be performed with HTTP verbs
43. Describe a RESTful API.
Ans:
An API that complies with the requirements of the REST architectural style and facilitates communication with RESTful web services is called a REST (Representational State Transfer) API, sometimes referred to as a RESTful API. When a client makes a request, a RESTful API forwards a representation of the resource’s state to the requester or endpoint.
44. Describe an endpoint.
Ans:
In a web API, the URLs that identify the various actions (HTTP verbs) that expose data (usually in JSON, the most popular data format these days and the default for the Django REST Framework) are called endpoints. A collection is a kind of endpoint that provides several data resources.
45. How do HTTP and HTTPS vary from one another?
Ans:
Hypertext Transfer Protocol Secure, or HTTP over TLS or HTTP over SSL, is what HTTPS stands for. HTTPS transmits and receives data packets using TCP (Transmission Control Protocol), but it does so over port 443 and over a connection that is secured by Transport Layer Security (TLS). Sites that use HTTPS typically have a redirect in place, so even if you use http://, it will still deliver content over a secure connection.
46. Describe it Main Distinctions Between HTTP and HTTPS?
Ans:
- HTTPS is secure, while HTTP is not.
- HTTPS uses port 443, whereas HTTP transfers data on port 80.
- HTTPS functions at the transport layer, whereas HTTP operates at the application layer.
- SSL certificates are not necessary for HTTP; HTTPS needs an SSL certificate that is signed by a CA in order to be used.
- Why does HTTPS require at least domain validation and, for some certificates, validation of legal documents? HTTP does not require any of these.
- HTTP does not encrypt data; HTTPS encrypts data before sending it.
47. How do status codes work?
Ans:
The status codes for HTTP responses provide information about whether a particular HTTP request was fulfilled successfully. Five classes are created from the responses:
- 1xx: Informational — Transfers data at the protocol level.
- 2xx: Success – Signifies a successful acceptance of the client’s request.
- 3xx: Redirection: This indicates that the client needs to proceed with their request differently.
- 4xx: Client Error: This group of error status numbers identifies clients as the source of the problem.
- 5xx: The server Error: These error status codes are the server’s responsibility.
48. What distinguishes permission from authentication?
Ans:
Through authentication, users can be verified as who they say they are. A resource can only be accessed by those users who have been authorized. Authorization must always come after authentication in safe contexts. Before being granted access to the resources they have sought, managers of an organization must first verify the integrity of their identities. Let’s illustrate the distinctions using an analogy.
49. How does a browsable API work?
Ans:
When the HTML format is requested, the Django REST Framework facilitates the creation of human-readable HTML output for every resource. These pages have forms for POST, PUT, and DELETE data submissions to the resources and convenient resource browsing. It makes using any web browser to connect with RESTful web services easier. We must include text/HTML as the Content-Type key in the request header to activate this feature.
- Include cors headers in the installed applications
- In the middleware section, put CorsMiddleware above CommonMiddleWare.
- Make a list called CORS_ORIGIN_WHITELIST.
50. How do Django’s `ForeignKey` and `OneToOneField` vary from each other?
Ans:
In contrast to `OneToOneField}, which creates a one-to-one relationship where each instance of one model corresponds to precisely one instance of the other, `ForeignKey` creates a many-to-one relationship between two models. A foreign key connects items. Whereas a OneToOneField, as the name suggests, establishes a one-to-one link in a relational database with a many-to-one relationship.
51. What distinguishes a state from a non-state?
Ans:
- One can comprehend a stateless program or process on its own. No information about or reference to previous transactions is kept on file. Every transaction is completed as though it were the first.
- On the other hand, stateful apps and procedures are repeatable, such as email or online banking.
- They are carried out within the framework of earlier transactions, and the outcomes of those earlier transactions may impact the current transaction.
- Because of these reasons, stateful applications employ the same servers every time they handle a user request.
52. Define Django Rest Framework.
Ans:
A web framework called Django REST Framework was developed on top of Django to facilitate the creation of web APIs, which are groups of URL endpoints with accessible HTTP verbs that return JSON. Building authenticated model-backed APIs is a pretty simple process of policies that can be browsed.
53. What advantages does the Django Rest Framework offer?
Ans:
- A major plus for your developers’ usability is its web-browsable API.
- OAuth1 and OAuth2 packages are included in authentication policies.
- Both ORM and non-ORM data sources are supported via serialization.
- You may customize every aspect of it. If you don’t require the more robust features, just use standard function-based views.
- It offers excellent community support and a wealth of documentation.
- Reputable businesses around the world, like Mozilla, Red Hat, Heroku, and Eventbrite, utilize and trust it.
54. Describe serializers.
Ans:
With the use of serializers, complicated data—such as query sets and model instances—can be simply transformed into native Python data types for rendering into other content types, such as JSON or XML. Additionally, serializers offer deserialization, enabling validation of the incoming input before converting the parsed data back into complicated kinds.
55. In DRF, what are Permissions?
Ans:
Permission checks are always performed at the very beginning of the view before allowing any additional code to run. To decide if an incoming request should be allowed, permission checks usually employ the authentication data in the request, user, and request auth attributes. Different user classes can be denied or given access to different parts of the API based on their permissions.
56. What Permissions Are at the Project Level?
Ans:
We may take advantage of some pre-installed project-level rights settings that come with the Django REST Framework, such as: AllowAny: Full access is granted to any user, authenticated or not. Unauthorised users can browse any page; only authenticated users can write, update, or delete content. o IsAuthenticated—only authenticated, registered users have access. o IsAdminUser—only admins/superusers have access.
57. How can I create personalized permission groups?
Ans:
Construct a file called permissions.py, import permissions at the beginning, and then construct your custom class (IsAuthorOrReadOnly, for example, which extends BasePermission), overriding has_object_permission in the process.
58. What’s Baseline Verification?
Ans:
- “Basic” authentication is the most often used type of HTTP authentication.
- Before access is allowed, the client must send an authorised authentication credential in an HTTP request.
- This is how the entire request/response flow appears
- v. An HTTP request is made by the client. The server replies with an HTTP response that includes information on how to authorize vii along with a 401 (Unauthorised) status code and WWW-Authenticate HTTP header. Credentials are returned by the client using the Authorization HTTP header viii.
- After verifying the credentials, After approval, the client includes the Authorization HTTP header credentials in all upcoming requests.
59. What drawbacks does Basic Authentication have?
Ans:
Drawbacks of Simple Authentication:
- In response to each request, the server has to search for and confirm the password and username, which is ineffective.
- User credentials are transmitted in plain text, which is readily intercepted and reused because it is not encrypted in the slightest.
60. What is authentication for a session?
Ans:
In essence, the client sends its credentials (password and username) for authentication, and the server sends back a session ID (stored as a cookie). After then, the session ID is sent in the header of each subsequent HTTP request. The server looks for a session object with all the information, including credentials, for a specific user when the session ID is submitted. Because a record needs to be preserved and maintained on the server (the session object) and the client (the session), this method is stateful.
61. What do the various authentication classes mean?
Ans:
The user inputs their login information, which is usually their username and password xi. After confirming that the credentials are accurate, the server creates a session object, which is subsequently saved in the database table. The session ID, which is saved as a cookie on the browser, is sent by the server to the client rather than the actual session object xiii. The session ID is added as an HTTP header to all upcoming requests, and the request moves forward if the database verifies it xiv. Both the client and the server delete the session ID when a user logs out of an application xv.
62. What benefits and drawbacks do session authentication systems have?
Ans:
Advantages: Unlike Basic Authentication, where user credentials are supplied on each request/response cycle, they are sent just once. It is also more efficient because the server only needs to quickly look up the session ID and session object rather than constantly verifying the user’s credentials.
Cons: A session ID cannot be used on several domains; it is only valid within the browser used to log in. When an API has to support more than one front-end, like a website and a mobile app, this is a clear issue.It is inefficient to send the cookie with every request, including ones that don’t need authentication.
63. Definition of Token Authentication.
Ans:
- Tokens are bits of information that contain just enough details to make it easier to identify a user or give them permission to do something.
- To sum up, tokens are objects that facilitate the permission and authentication processes carried out by application systems.
- Stateless token-based authentication works by having a client submit the server the initial user credentials.
- This generates a unique token that the client stores locally or in a cookie.
- The server then uses this token, which is given in the header of every incoming HTTP request, to confirm that a user is verified.
64. What benefits and drawbacks come with token authentication?
Ans:
Advantages: Since tokens are kept on the client, scaling the servers to keep session objects current is no longer a problem. Tokens can be shared between several front-ends; for example, a single token can represent a user on a mobile app and a website.
Cons: Unlike when a session ID and session object are set up, a token comprises all user information, not just an ID. Keeping the token small can become a performance problem because it is sent with every request.
65. What distinguishes cookies from localStorage?
Ans:
Cookies are used to read data from the server. They are automatically delivered with every HTTP request and have a lower (4KB) size. Client-side data is intended to be stored in LocalStorage. It is somewhat larger (5120 KB), and each HTTP request does not automatically provide its contents.
66. Should tokens be saved in local storage or cookies?
Ans:
When using token-based auth, you can choose where to store the JWT. For the majority of use scenarios, it is common practice to store the JWT in the browser’s local storage. However, it’s important to be aware of several problems while keeping JWTs locally stored. Local storage, in contrast to cookies, is sandboxed to a single domain, and its contents are inaccessible to any other domain, not even sub-domains.
67. What are the drawbacks of integrated token authentication in the Django REST Framework?
Ans:
- It only creates one token per user; it does not permit setting tokens to expire.
- You can store the token in a cookie instead. However, as cookies can only hold 4 KB of data, this could be an issue if your token is linked to a lot of claims.
- Apart from local storage, you can also keep the token in session storage, which gets deleted as soon as the user quits the browser.
- XSS attacks can be used against tokens that are kept in localStorage and cookies.
- Tokens should currently be stored in cookies that have the httpOnly and Secure cookie flags set.
68. What are JWTs or JSON Web Tokens?
Ans:
A free and open standard is JSON web token (JWT). It outlines a condensed, independent method of securely sending data as a JSON object between parties. A JWT is delivered rapidly and can be sent using an HTTP header, a POST parameter, or a URL because of its relatively tiny size. In order to minimize the need for repeated database queries, a JWT includes all the necessary information about an entity. In order to validate a JWT, the recipient does not also need to make a server call.
69. What advantages does JWT offer?
Ans:
More compact: A JWT is smaller when encoded from JSON than a plain token because it is less verbose than XML. Because of this, JWT is an excellent option to pass in HTTP and HTML contexts.
More secure: JWTs have the option to sign using a public/private key pair. The HMAC approach can also be used to symmetrically sign a JWT using a shared secret.
70. What distinguishes a cookie from a session?
Ans:
Every time a browser sends a request to the server, it stores a small piece of information called a cookie. Cookies identify sessions. A collection of information saved on the server and linked to a specific user is called a session (typically via a cookie carrying an ID code). Sessions save data on the server, while cookies store data on the visitor’s browser. This is the primary distinction between the two types of data storage. Because sessions are saved on the server, they are more secure than cookies.
71. What distinguishes tokens from cookies?
Ans:
- Stateful authentication is based on cookies. This implies that both the server and the client must maintain an authentication record or session.
- The term “cookie-based authentication” refers to the requirement that the server keep track of active sessions in a database while a cookie is created on the front end and contains a session identifier.
- Stateless authentication is used with tokens. The server does not record which users are logged in or which JWTs have been issued.
- Rather, each request sent to the server is accompanied by a token that the server uses to confirm the request’s legitimacy.
72. Describe an access token.
Ans:
An access token, an artifact that client applications can use to perform secure calls to an API server, is issued by the authorization server to logged-in users. The access token enables a client application to inform a server that it has been granted permission by the user to carry out specific actions or access specific resources when the client needs to access protected resources on the server on the user’s behalf.
73. What does the term “bearer token” mean?
Ans:
A “bearer token” is a type of access token used in authentication protocols, particularly in OAuth 2.0 and similar frameworks. It is a security credential that is issued by an authorization server and granted to a client application. The bearer token is included in the HTTP Authorization header of requests made by the client to access protected resources on a server.
74. What is the access token’s security risk?
Ans:
- In theory, malicious users may break into a system and take access tokens.
- They could then use those tokens to present the access tokens to the server directly, granting them access to things that are protected.
- Therefore, it’s imperative to implement security measures that reduce the possibility of access token compromise.
- One mitigation technique is to create access tokens with a limited lifespan—that is, ones that are only usable for a predetermined number of hours or days.
75. What is a token for a refresh?
Ans:
Tokens for access control may have a limited validity period for security reasons. Client apps can “refresh” the access token after it expires by using a refresh token. In other words, a credential is a refresh token. A refresh token is a mechanism that enables a client application to obtain fresh access tokens without requiring the user to re-log in. As long as the refresh token is active and has not expired, the client application is eligible to receive a new access token.
76. When it comes to token authentication, what are the recommended practices?
Ans:
When utilizing tokens, bear the following fundamental points in mind:
- Keep it a secret. Preserve it.
- Don’t include sensitive information in the payload.
- Set an expiry date for tokens.
- Adopt HTTPS.
- Take into account every use case for authorization.
77. What is authentication based on cookies?
Ans:
An authorization cookie is always used to sign in to a request made to the server. Advantages: o “http-only” cookies can be set, which prevents clients from reading them. In terms of XSS-attack defense, this is preferable.
- Works right out of the box; no client-side code implementation is required.
- Capable of XSRF. In order to defend your website from cross-site request forgery, you must take additional precautions.
- Are sent out for each and every request, even those that don’t call for authentication.
78. In DRF, what are view sets?
Ans:
A view set is A code for several connected views that can be consolidated into a single class using a view set. Put otherwise, numerous views can be replaced by a single view. It’s a class that’s just another kind of class-based view; instead of offering handlers for methods like get() or post(), it offers actions like list() and create().
79. In DRF, what are routers?
Ans:
- Routers create URL patterns for us automatically by interacting directly with view sets. SimpleRouter and DefaultRouter are the two default routers included in the Django REST Framework.
- The SimpleRouter router includes routes for the common list, create, retrieve, update, partial update, and delete actions.
- Default Router: This router has an API root view that returns by default, and it is comparable to SimpleRouter.
- Reply with links to each of the list views. Additionally, it creates routes for optional format suffixes in the.json style.
80. What distinguishes Viewsets in DRF from APIViews?
Ans:
DRF handles views using two primary systems:
- APIView: This offers handler methods for the following HTTP verbs: put, patch, delete, get, and post.
- ViewSet: An abstraction of APIView that offers actions as methods is this one.
- List: return several resources (HTTP verb: get), read-only. Yields a list of dicts back.
- Read-only resource retrieval (HTTP verb: get; will expect an id in the URI). Gives back a single dictator.
81. What distinguishes GenericViewset from GenericAPIView?
Ans:
Generic API View: this provides you with APIView shortcuts that closely correspond to your database models. Adds behavior that is frequently needed for list and detail views. It provides you with several features, such as the serializer class; additionally, it provides a pagination class, filter backend, etc.
GenericViewSet: The most popular GenericViewSet is ModelViewSet. They have a complete implementation of every action, including list, retrieve, destroy, update, and so on, and they derive from GenericAPIView.
82. How is a Django application secured?
Ans:
- One of the best practices and strategies for securing a Django application is using HTTPS to encrypt data in transit.
- Putting in place robust authorization and authentication systems.
- To stop injection attacks, user input must be validated and sanitized.
- Safely adjusting the Django settings, like turning off the DEBUG mode in production.
- Updating Django and its dependencies on a regular basis to address security flaws.
83. Describe Django signals and their applications.
Ans:
Django signals allow decoupled apps to interact with one another through events. The Django.db.models.signals module contains these. The most often used signals are pre_save, post_save, pre_delete, and post_delete. When particular events occur, like saving or deleting a model instance, they can carry out particular actions.
84. Describe the distinction between Django’s class-based and function-based views.
Ans:
In Django, there are two ways to define views: class-based views (CBVs) and function-based views (FBVs). These are their primary distinctions from one another:
Views based on functions: These straightforward Python methods accept a request object as a parameter and give back an HTTP answer. They are simple to comprehend and use, but as the program expands, they may become complex and challenging to maintain.
Class-based views: These are classes that implement particular methods to handle various HTTP methods (GET, POST, etc.) and are derived from Django’s base view classes. CBVs facilitate code modularity and reusability, which facilitates application maintenance and expansion.
85. In Django, how are file uploads handled?
Ans:
To manage file uploads in Django, take the following actions:
- To allow file uploads, create a Django form containing a `FileField` or `ImageField}.
- Create an instance of the form in the view, and then manage the file upload by ensuring that the form is valid and the request type is POST.
- You can manually handle the file object or use the `save()` method on the form to save the uploaded file to the appropriate location.
- Set up the `settings.py` file’s `MEDIA_URL` and `MEDIA_ROOT` parameters to serve uploaded files while the project is under construction.
86. What is the template system in Django, and how does it operate?
Ans:
The text-based language used by Django’s template system specifies the organization and HTML page layout of a web application. It simplifies the maintenance and upgrade of the user interface by enabling developers to isolate the presentation from the application logic. The placeholders and template tags found in template files are processed by the template system, which then replaces them with real data taken from the context.
87. Describe the directory structure of the Django project.
Ans:
- When a Django project is initially launched, it includes some basic 4. views.py—The View presents the data from the model to the user. The view is aware of what the data means or what the user might do with it.
- urls.py – We save all project links and call functions in this universal resource locator, which has all of the endpoints.
- models.py—This file represents web application models as classes but lacks logic that specifies how data should be presented to users.
- wsgi.py — Web Server Gateway Interface, or WSGI, is the file that is used to deploy the project in WSGI. It facilitates communication between the web server and your Django application.
88. Give a brief overview of the Django admin panel.
Ans:
Django features an easy-to-use default admin interface that lets the user perform administration activities such as creating, reading, updating, and removing model objects. This module is integrated and creates a fast interface where the user can change the application’s contents by reading a set of data that describes and explains data from the model.
89. What are URLs in Django?
Ans:
The URLs of a website control its routing. We create a urls.py file or a Python module in the program. This file determines how your website navigates. The URLs in the urls.py file are compared when a user opens a specific URL route in the browser. Once a corresponding view method has been retrieved, the user is presented with the response for the requested URL.
90. Describe Django’s MVC architecture.
Ans:
- Django uses the Model-View-Template (MVT) design, in which Views manage user interaction and business logic, Models describe the data structure, and Templates produce the HTML display.
- A product development architecture is the MVC pattern.
- It addresses the problem with the traditional technique of having all of the code in one file, namely the fact that the MVC design uses separate files for each part of our website or web application.
- Three elements make up the MVC pattern: Model, View, and Controller.
91. What is Django ORM?
Ans:
Django ORM (Object-Relational Mapping) is an integrated feature that eliminates the need for developers to manually write SQL queries by enabling interaction with the database using Python classes and methods. One of Django’s most potent tools is the Object-Relational Mapper (ORM), which lets you communicate with your database using the same methods as SQL.