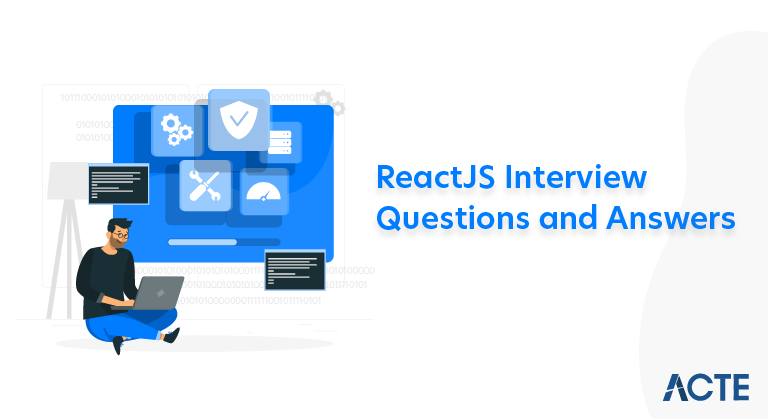
25+Top React JS Interview Questions & Answers [UPDATED]
Last updated on 15th Jun 2020, Blog, Interview Questions
Reactjs is an open-source javascript UI(User Interface) library, collaborated and maintained by Facebook and Instagram and also a huge community of developers. That’s designed to take your application state and allow you to write code that makes that status input and return virtual DOM, It’s a certainly better way to manipulate the DOM and handle events which are really just a set of the plain.
Here are a few React.js interview questions that answer about all the major elements of React Js. In this post, we have added some Basic and Advanced React Js Interview Questions. Thus, these questions are compiled with both basic and advanced level questions on React Js. Preparing for your interview with these questions, will give you an edge over the others and will help you crack the exam.
1. Why is React used?
Ans:
React is a free JavaScript package that is used to create user interfaces, especially single-page programs. It makes it possible to build reusable UI components and manage UI changes effectively.
2. What is the significance of React Fiber?
Ans:
React Fiber is a complete rewrite of the React core algorithm, designed to enable better performance, incremental rendering, and improved developer experience.
3. How has React.js evolved over the years?
Ans:
React.js has evolved by introducing new features like hooks, context API improvements, concurrent mode (in development), and performance optimizations through React Fiber.
4. What is React Native, and how does it relate to React.js?
Ans:
React Native is a framework that allows developers to build mobile applications using React principles. It shares some concepts with React.js, like component-based architecture.
5. How has React.js impacted the web development landscape?
Ans:
React.js has had a significant impact by promoting declarative UI programming, encouraging the use of reusable components, and driving the adoption of similar concepts in other libraries and frameworks.
6. What are React lifecycle methods?
Ans:
Lifecycle methods are special methods that get called at various stages of a component’s lifecycle, such as mounting, updating, and unmounting.
7. Explain the purpose of the useEffect hook.
Ans:
The useEffect hook is used to perform side effects in functional components, like data fetching, subscriptions, and DOM manipulation, after rendering.
8. How can you handle global state management in a large React application without using Redux?
Ans:
You can use context API along with hooks for global state management. Create context providers and consumers to share state across components.
9. What is React Suspense and how is it used?
Ans:
React Suspense is a feature that allows you to pause rendering components while waiting for async operations, like data fetching, to complete.
10. How can you share state between components using the useContext hook?
Ans:
The useContext hook is used to consume context values created with the React Context API, allowing components to access shared data without prop drilling.
11. Why does React use JSX?
Ans:
The structure and appearance of components in React are described using the syntactic extension known as JSX (JavaScript XML). It allows you to write HTML-like code directly within JavaScript, which is then transpired to JavaScript functions. JSX makes it easier to visualize and construct complex UIs.
12. Explain the concept of components in React.
Ans:
Components in React are reusable building blocks that encapsulate UI elements and their behavior. They can be functional components (stateless) or class components (stateful), and they encourage a modular and declarative approach to UI development. Components receive data via props and can maintain their own local state.
13. What is the difference between state and props in React?
Ans:
Both state and props are mechanisms to pass and manage data in React:
Props | State |
---|---|
Props are read-only and passed from parent to child components. They allow you to configure and customize a component when it’s created. Props are used for data that doesn’t change within the component. | State is mutable and managed within a component. It represents data that can change over time due to user interaction or other factors. The state allows a component to keep track of its internal changes. |
14. What is the virtual DOM in React, and how does it work?
Ans:
The real DOM elements in memory are represented by the virtual DOM. React uses it to optimize rendering and improve performance. When state or props change, React compares the virtual DOM with the previous virtual DOM state, identifies the differences (diffing), and then applies only the necessary changes to the actual DOM. This minimizes the number of DOM updates, resulting in faster rendering.
15. How does React handle event handling?
Ans:
React uses a synthetic event system that provides a common interface for handling events while taking browser incompatibilities. Event handlers in React are written using camelCase naming, such as onClick and onChange. When an event occurs, React’s event system wraps the native event and handles it consistently across different browsers.
16. Describe React hooks.
Ans:
Functions called “React hooks” let you use functional components as “hooks into” React state and lifecycle aspects. They were introduced in React 16.8 to provide a more convenient way to manage state and side effects in functional components without using class components. Examples of hooks include useState, useEffect, and useContext.
17. What function does React’s setState method serve?
Ans:
A React component’s state is updated using the setState function.
When the state changes, React re-renders the component and its children to reflect the new state. It’s important to note that setState is asynchronous, and React batches multiple state updates for efficiency. You can also provide a callback function as the second argument to setState, which is executed after the state update is completed.
18. How can you prevent unnecessary re-renders in React?
Ans:
- Using shouldComponentUpdate or React .memo to control when a component should update.
- Avoiding inline function definitions in render methods, as they can lead to new functions on every render.
- Using the useMemo hook to memoize computed values.
- Using the use callback hook to memoize event handlers.
19. What is React Router, and why is it used?
Ans:
React apps may route requests using the package React Router. It allows you to define routes, map them to components, and navigate between different views or pages within a single-page application. React Router helps in creating a seamless user experience by enabling navigation without full page reloads.
20. What are React keys used for?
Ans:
React keys are used to give each element in an array a stable identity. They help React optimize rendering by recognizing which items have changed, been added, or been removed in lists of components.
21. How can you conditionally render components in React?
Ans:
You can use conditional statements within your component’s render method to determine whether to render a component or not based on certain conditions.
22. How does React handle the rendering of components?
Ans:
When a component’s props or state change in React, components are automatically rendered. A component that renders returns a React element, which is a simplified version of the user interface.
23. Explain the concept of component lifecycle in React.
Ans:
Component lifecycle refers to the series of phases a component goes through, from creation to rendering and eventual removal from the DOM. It involves methods like componentDidMount, componentDidUpdate, and componentWillUnmount.
24. What is the significance of the constructor method in class components?
Ans:
The constructor method is used to initialize the state and bind event handlers in a class component. It’s called when an instance of the component is created.
25. What are the advantages of using React for building user interfaces?
Ans:
React offers better performance through the use of the virtual DOM, encourages reusability with components, and provides a declarative syntax for UI development.
26. Explain the difference between a functional component and a class component in React.
Ans:
A functional component is defined as a JavaScript function, while a class component is an ES6 class that extends React.Component. Functional components are simpler and recommended when possible.
27. What is the significance of the key prop when rendering a list of components?
Ans:
The key prop is used to uniquely identify elements in a list. It helps React optimize rendering by identifying which items have changed, been added, or been removed.
28. How can you conditionally render a component in React?
Ans:
Conditional rendering can be achieved using ternary expressions, logical operators like &&, or by writing functions that return JSX based on conditions.
29. Explain the role of the useState hook in React.
Ans:
The useState hook allows functional components to manage state without using class components. It returns the current state and a function to update it.
30. What is prop drilling, and how can you avoid it in React applications?
Ans:
Prop drilling is the process of passing props through multiple layers of components. It can be avoided by using React Context to share data across components without passing props explicitly.
31. What is the role of the map() function in React, and how is it used?
Ans:
The map() function is used to iterate over an array and transform each item into a React element. It’s commonly used for rendering lists of components.
32. What is the purpose of a React fragment, and how is it different from a regular DOM element?
Ans:
A React fragment allows you to group elements without introducing an additional parent DOM element. Fragments don’t create a new DOM node in the resulting HTML.
33. How can you optimize the performance of a React application?
Ans:
Performance optimization techniques include using memoization, lazy loading, code splitting, and optimizing component rendering with shouldComponentUpdate or React.memo.
34 . What are the popular animation packages in the React ecosystem?
Ans:
Popular animation package in React ecosystem are:
- React Motion
- React Transition Group
35. What is the benefit of strict mode?
Ans:
This will be helpful in the below cases
- Identifying components with unsafe lifecycle methods.
- Warning about legacy string ref API usage.
- Detecting unexpected side effects.
- Detecting legacy context API.
- Warning about deprecated findDOMNode usage
36. Explain the concept of one-way data flow in React.
Ans:
Data travels from parent components to child components in a single direction when there is a one-way data flow. Data in parent components cannot be directly modified by child components.
37. What are higher-order components (HOCs) in React?
Ans:
Higher-order components are functions that take a component and return a new component with enhanced functionality. They are used for code reuse and logic abstraction.
38. How can you use React Router to handle routing in a single-page application?
Ans:
React Router provides components like BrowserRouter, Route, and Link that allow you to define and navigate between different views within a single-page application.
39. Define the Representational segment
Ans:
A presentational part is a segment which allows you to render HTML. The segment’s capacity is presentational in markup.
40. Explain the purpose of the componentDidUpdate lifecycle method in React.
Ans:
The componentDidUpdate method is called after a component’s state or props update. It’s often used for performing side effects based on changes.
41. How does the virtual DOM work in React, and why is it important for performance?
Ans:
You can use various techniques, such as the fetch API, third-party libraries like Axios, or the useEffect hook with async/await to fetch data from an API.
42. What is memoization, and how can it be applied to React components?
Ans:
Memoization is the process of caching the results of expensive function calls. In React, components can be memoized using React.memo to avoid unnecessary re-renders.
43. Name two types of React component
Ans:
Two types of react Components are:
- Function component
- Class component
44. How can you access route parameters in React Router?
Ans:
Route parameters are accessed using the useParams hook or the match.params object within a component rendered by the Route component.
45. State the main difference between Pros and State
Ans:
The main difference between the two is that the State is mutable and Pros are immutable.
46. What is the purpose of the shouldComponentUpdate method in class components?
Ans:
The shouldComponentUpdate method allows you to control whether a component should re-render after state or props change. By default, it returns true, but you can implement custom logic to optimize rendering.
47. Explain the concept of lazy loading in React.
Ans:
Lazy loading is a technique where you only load components or assets when they are needed, improving initial page load times and reducing the amount of data transferred.
48. How can you use the React.lazy function to perform code splitting?
Ans:
React.lazy allows you to load components lazily, creating a separate bundle for each component. It’s commonly used with the Suspense component to handle loading states.
49. What is the purpose of the Suspense component in React?
Ans:
The Suspense component is used to handle asynchronous operations, such as code splitting or data fetching, while providing a fallback UI during loading.
50. How do you handle errors that occur during rendering with the ErrorBoundary component?
Ans:
The ErrorBoundary component, introduced in React 16, wraps a section of your application and handles errors that occur during rendering. It enables the presentation of fallback UI and the smooth handling of faults.
51. What is the significance of the key prop in the reconciliation process?
Ans:
The key prop helps React efficiently update and reconcile elements in the virtual DOM. When elements are added, removed, or reordered, the key prop helps React identify them uniquely.
52. Explain the concept of forward refs in React.
Ans:
Forwarding refs is a technique that allows a parent component to pass a ref directly to a child component, enabling the parent to access the child’s DOM node or React component.
53. What is the purpose of the dangerouslySetInnerHTML prop in React?
Ans:
The dangerouslySetInnerHTML prop is used to set HTML content inside a component. It must be used carefully since if misused, it exposes your application to cross-site scripting (XSS) assaults.
54. How does React handle the removal of event listeners when a component unmounts?
Ans:
React automatically cleans up event listeners when a component unmounts to prevent memory leaks. You don’t need to manually remove event listeners in most cases.
55. What is the purpose of the useMemo hook, and when would you use it?
Ans:
The useMemo hook is used to memoize the result of a computation, preventing unnecessary recalculations. It’s useful when you have expensive calculations that depend on props or state.
56. How can you prevent a component from rendering unnecessarily when its parent re-renders?
Ans:
You can use the React.memo higher-order component or the useMemo hook to prevent unnecessary renders when props haven’t changed.
57. What is the significance of the strictMode in React?
Ans:
The strictMode is a development mode feature that helps identify potential problems in your application by highlighting issues such as deprecated lifecycle methods, unsafe side effects, and more.
58. Explain the concept of code splitting and why it’s important for modern web applications.
Ans:
Code splitting involves breaking down your JavaScript bundle into smaller chunks that are loaded on-demand. This improves performance by reducing initial loading times and improving resource utilization.
59. How does React handle handling user input, like forms and controlled components?
Ans:
In controlled components, React maintains the state of the input field, and the value is set via props. This allows React to have full control over the input’s behavior and value.
60. What is the purpose of the unstable_batchedUpdates function in React?
Ans:
unstable_batchedUpdates is used internally by React to batch multiple setState calls together to optimize performance by reducing the number of renders.
61. Explain the concept of the key prop and why it’s important when rendering lists.
Ans:
The key prop is used to uniquely identify elements within a list. It helps React efficiently update the DOM by matching new elements with their corresponding old elements based on keys.
62. How can you prevent unnecessary renders caused by frequent prop changes?
Ans:
You can use the React.memo higher-order component or the useMemo hook to memoize the component’s output and prevent unnecessary re-renders.
63. How To Embed Two Components In One Component?
Ans:
- import React from ‘react’;
- class App extends React.Component {
- render() {
- return (
- <div>
- <Header/>
- <Content/>
- </div>
- );
- }
- }
- class Header extends React.Component {
- render() {
- return (
- <div>
- <h1>Header</h1>
- </div>
- );
- }
- }
64. What is the purpose of the forwardRef function in React?
Ans:
By forwarding refs from a parent component to a child component using the forwardRef function, the parent component can access the child’s DOM node or React component.
65. How do you manage the global state in a React application without using external libraries?
Ans:
You can use React Context to manage a global state by creating a context and providing its value to the component tree. This allows components to consume the context data without prop drilling.
66. Explain the significance of the dangerouslySetInnerHTML prop and its potential security risks.
Ans:
The dangerouslySetInnerHTML prop is used to render HTML content as-is. However, using it incorrectly can expose your application to cross-site scripting (XSS) attacks if the content is not sanitized properly.
67. What are the main components of React Intl?
Ans:
React Intl provides components such as FormattedMessage for rendering translated messages and FormattedDate, FormattedTime, and FormattedNumber for formatting dates, times, and numbers.
68. What is a locale in React Intl?
Ans:
A locale is a specific combination of a language and region, like “en-US” for English in the United States.
69. Explain the concept of plural categories in React Intl.
Ans:
Plural categories are different forms of a message based on the count value. In languages with complex plural rules, React Intl handles selecting the appropriate form for you.
70. What are fallback messages in React Intl?
Ans:
Fallback messages are default messages that are displayed if a translation is not available for the current locale. They help ensure that users always see some meaningful content.
71. How can you dynamically change the locale in React Intl?
Ans:
You can change the locale by wrapping your application with a parent component that updates the locale prop of the IntlProvider.
72. How can you implement route guards in React Router to control access to routes?
Ans:
You can implement route guards by conditionally rendering a component based on certain conditions, such as authentication status.
73. How can you implement redirects in React Router?
Ans:
You can use the Redirect component to redirect users from one route to another based on certain conditions, such as when a user is not authenticated.
74. How can you define nested routes using React Router?
Ans:
To define nested routes, you render a Route component within the component that’s already associated with a parent route.
75. How do you handle the scenario where you want to render multiple components based on a single condition?
Ans:
You can wrap the conditional rendering in a parent container and render multiple components inside it. The parent container’s condition determines which child component gets rendered.
76. How does Jest simplify testing in React applications?
Ans:
Jest simplifies testing by providing a testing framework, assertion library, and mocking utilities all in one package. It offers features like automatic test discovery, parallel test execution, and built-in code coverage reporting.
77. What are the benefits of using Jest alongside React Testing Library?
Ans:
React Testing Library complements Jest by providing utilities to interact with and assert on rendered components in a way that mirrors how users interact with the UI, promoting more meaningful tests.
78. Why would you use Redux for state management?
Ans:
Redux provides a single source of truth for application state, makes state changes predictable, and simplifies state management, especially in larger and more complex applications.
79. How can you combine reducers in Redux?
Ans:
Redux provides the combineReducers function, which takes an object that maps reducer functions to their corresponding state slices.
80. What is the concept of “lifting state up” in React?
Ans:
“Lifting state up” refers to the practice of moving shared state from multiple child components to their common parent, making it a single source of truth.
81. How can you handle forms with complex validation and logic in React?
Ans:
You can manage complex form logic using libraries like Formik or by creating custom form handling components.
82. What are React Portals?
Ans:
Portals provide a way to render children outside their parent component’s DOM hierarchy, allowing you to render components into a different part of the DOM.
83. Explain the concept of High-Order Components (HOCs).
Ans:
HOCs are functions that take a component and return a new component with added behavior or props. They’re used to enhance component functionality.
84. What is the role of memoization in optimizing React applications?
Ans:
Memoization involves caching the results of function calls to avoid redundant computations, which can significantly improve performance in certain scenarios.
85. What is the purpose of unit testing in React?
Ans:
Unit testing ensures that individual components and functions behave as expected, helping to catch bugs early in the development process.
86. What is server-side rendering (SSR) in React?
Ans:
SSR is a technique that renders React components on the server and sends the HTML markup to the client, improving initial load time and SEO.
87. How does Next.js help with server-side rendering?
Ans:
Next.js is a framework for React that simplifies server-side rendering, routing, and other advanced features, making it easier to build SSR applications.
88. Why is key management important in React lists?
Ans:
Keys help React efficiently update components within lists. Properly managing keys prevents unnecessary re-rendering and ensures accurate updates.
89. How can you optimize the rendering performance of a React application?
Ans:
You can optimize performance by using techniques like memoization, code splitting, lazy loading, and reducing unnecessary renders.
90. How can you handle errors in React components?
Ans:
You can handle errors using the componentDidCatch lifecycle method, which catches errors that occur in child components.
91. What are React error boundaries?
Ans:
Error boundaries are components that catch and handle errors in their child component tree, preventing the entire application from crashing.
92. How can you handle asynchronous actions in Redux?
Ans:
To handle asynchronous actions in Redux, you can use middleware like Redux Thunk or Redux Saga. These middleware allow you to dispatch asynchronous actions, like API requests, and update the store with the fetched data.
93. What are the main features of React Intl?
Ans:
- Display numbers with separators.
- Display dates and times correctly.
- Display dates relative to “now”.
- Pluralize labels in strings.
- Support for 150+ languages.
- Runs in the browser and Node.
- Built on standards.
94. What is the purpose of Redux Thunk middleware?
Ans:
Redux Thunk is a middleware that allows you to write action creators that return functions instead of plain action objects. This enables you to perform asynchronous operations and dispatch actions based on the result.
95. How does the concept of “render props” work in React?
Ans:
“Render props” is a technique where a component’s prop is a function that returns a React element. This allows for code reuse and sharing behavior between components. It’s often used for sharing stateful logic.
96. How are events different in React?
Ans:
In React, event handlers use camelCase naming, and you pass functions as handlers in JSX, rather than strings.