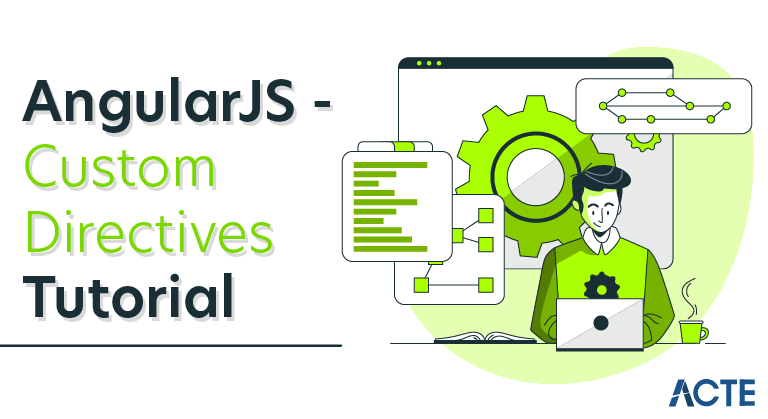
Custom directives are used in AngularJS to extend the functionality of HTML. Custom directives are defined using the “directive” function. A custom directive simply replaces the element for which it is activated. AngularJS application during bootstrap finds the matching elements and does one time activity using its compile() method of the custom directive then processes the element using link() method of the custom directive based on the scope of the directive. AngularJS provides support to create custom directives for following types of elements.
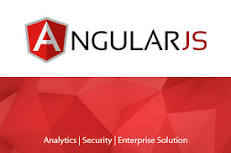
- Element directives − Directive activates when a matching element is encountered.
- Attribute − Directive activates when a matching attribute is encountered.
- CSS − Directive activates when a matching css style is encountered.
- Comment − Directive activates when a matching comment is encountered.
Understanding Custom Directive
Define custom html tags.
Define custom directive to handle above custom html tags.
- var mainApp = angular.module(“mainApp”, []);
- //Create a directive, first parameter is the html element to be attached.
- //We are attaching a student html tag.
- //This directive will be activated as soon as any student element is encountered in html
- mainApp.directive(‘student’, function() {
- //define the directive object
- var directive = {};
- //restrict = E, signifies that directive is Element directive
- directive.restrict = ‘E’;
- //template replaces the complete element with its text.
- directive.template = “Student: <b>{{student.name}}</b> ,
- Roll No: <b>{{student.rollno}}</b>”;
- //scope is used to distinguish each student element based on criteria.
- directive.scope = {
- student : “=name”
- }
- //compile is called during application initialization. AngularJS calls
- it once when the html page is loaded.
- directive.compile = function(element, attributes) {
- element.css(“border”, “1px solid #cccccc”);
- //linkFunction is linked with each element with scope to get the element specific data.
- var linkFunction = function($scope, element, attributes) {
- element.html(“Student: <b>”+$scope.student.name +”</b> ,
- Roll No: <b>”+$scope.student.roll no”</b><br/>”);
- element.css(“background-color”, “#ff00ff”);
- }
- return linkFunction;
- }
- return directive;
- });
- Define controller to update the scope for directive. Here we are using the name attribute’s value as scope’s child.
- mainApp.controller(‘StudentController’, function($scope) {
- $scope.Mahesh = {};
- $scope.Mahesh.name = “Mahesh Parashar”;
- $scope.Mahesh.rollno = 1;
- $scope.Piyush = {};
- $scope.Piyush.name = “Piyush Parashar”;
- $scope.Piyush.roll no = 2;
- });
Example
- <html>
- <head>
- <title>Angular JS Custom Directives</title>
- </head>
- <body>
- <h2>AngularJS Sample Application</h2>
- <div ng-app = “mainApp” ng-controller = “StudentController”>
- <student name = “Mahesh”></student><br/>
- <student name = “Piyush”></student>
- </div>
- <script src = “https://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js”>
- </script>
- <script>
- var mainApp = angular.module(“mainApp”, []);
- mainApp.directive(‘student’, function() {
- var directive = {};
- directive.restrict = ‘E’;
- directive.template = “Student: <b>{{student.name}}</b> ,
- Roll No: <b>{{student.rollno}}</b>”;
- directive.scope = {
- student : “=name”
- }
- directive.compile = function(element, attributes) {
- element.css(“border”, “1px solid #cccccc”);
- var linkFunction = function($scope, element, attributes) {
- element.html(“Student: <b>”+$scope.student.name +”</b> ,
- Roll No: <b>”+$scope.student.roll no”</b><br/>”);
- element.css(“background-color”, “#ff00ff”);
- }
- return linkFunction;
- }
- return directive;
- });
- mainApp.controller(‘StudentController’, function($scope) {
- $scope.Mahesh = {};
- $scope.Mahesh.name = “Mahesh Parashar”;
- $scope.Mahesh.rollno = 1;
- $scope.Piyush = {};
- $scope.Piyush.name = “Piyush Parashar”;
- $scope.Piyush.roll no = 2;
- });
- </script>
- </body>
- </html>
- Output
- Open textAngularJS.htm in a web browser. See the resul
AngularJS – Internationalization
AngularJS supports inbuilt internationalization for three types of filters : Currency, Date, and Numbers. We only need to incorporate the corresponding java script according to the locale of the country. By default, it considers the locale of the browser. For example, for Danish locale, use the following script:
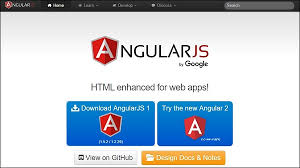
<script src = “https://code.angularjs.org/1.2.5/i18n/angular-locale_da-dk.js”>
</script>
Example Using Danish Locale
TestAngularJS.htm
- style=”text-align: left;”><script src = “https://code.angularjs.org/1.2.5/i18n/angular-locale_da-dk.js”>
- style=”text-align: left;”></script>
- style=”text-align: left;”>Example Using Danish Locale
- style=”text-align: left;”>testAngularJS.htm
- style=”text-align: left;”>Live Demo
- style=”text-align: left;”><html>
- yle=”text-align: left;”><head>
- style=”text-align: left;”><title>Angular JS Forms</title>
- style=”text-align: left;”></head>
- style=”text-align: left;”><body>
- style=”text-align: left;”><h2>AngularJS Sample Application</h2>
- style=”text-align: left;”><div ng-app = “mainApp” ng-controller = “StudentController”>
- style=”text-align: left;”>{{fees | currency }} <br/><br/>
- {{admission date | date }} <br/><br/>
- {{rollno | number }}
- </div>
- <script src = “https://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js”>
- </script>
- <script src = “https://code.angularjs.org/1.3.14/i18n/angular-locale_da-dk.js”>
- </script>
- <script>
- var mainApp = angular.module(“mainApp”, []);
- mainApp.controller(‘StudentController’, function($scope) {
- $scope.fees = 100;
- $scope.admission date = new Date();
- scope.rollno = 123.45;
- });
- </script>
- </body>
- </html>
- Output
- Open the file testAngularJS.htm in a web browser and see the result.
- Example Using Browser Locale
- testAngularJS.htm
- <html>
- <head>
- <title>Angular JS Forms</title>
- </head>
- <body>
- <h2>AngularJS Sample Application</h2>
- <div ng-app = “mainApp” ng-controller = “StudentController”>
- {{fees | currency }} <br/><br/>
- {{admission date | date }} <br/><br/>
- {{rollno | number }}
- </div>
- <script src = “https://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js”>
- </script>
- <!– <script src = “https://code.angularjs.org/1.3.14/i18n/angular-locale_da-dk.js”>
- </script> –>
- <script>
- var mainApp = angular.module(“mainApp”, []);
- mainApp.controller(‘StudentController’, function($scope)
- {
- $scope.fees = 100;
- $scope.admission date = new Date();
- $scope.rollno = 123.45;
- });
- </script>
- </body>
- </html>
- Output
- Open the file testAngularJS.htm in a web browser and see the result.
AngularJS – Todo Application
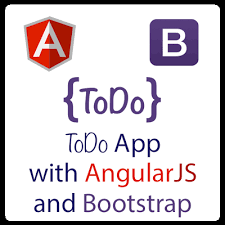
We are providing an example of a Todo app. To develop this app, we have used HTML, CSS and AngularJS. We have included javascripts such as angular-route.js, and base.js.
AngularJS – Notepad Application
We are providing an example of a Notepad app. To develop this app, we have used HTML, CSS and AngularJS. We have included javascripts such as myNoteApp.js, and myNoteCtrl.js.
AngularJS – Bootstrap Application
We are providing an example of a Bootstrap app. To develop this app, we have used HTML, CSS and AngularJS.
AngularJS – Login Application
We are providing an example of a Login app. To develop this app, we have used HTML, CSS and AngularJS.
We are providing an example of Upload File. To develop this app, we have used HTML, CSS and AngularJS. Following example shows how to upload the file using AngularJS.
- <html>
- <head>
- <script src = “https://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js”>
- </script>
- </head>
- <body ng-app = “myApp”>
- <div ng-controller = “myCtrl”>
- <input type = “file” file-model = “myFile”/>
- <button ng-click = “uploadFile()”>upload me</button>
- </div>
- <script>
- var myApp = angular.module(‘myApp’, []);
- myApp.directive(‘fileModel’, [‘$parse’, function ($parse) {
- return {
- restrict: ‘A’,
- link: function(scope, element, attrs) {
- var model = $parse(attrs.fileModel);
- var modelSetter = model.assign;
- element.bind(‘change’, function() {
- scope.$apply(function() {
- modelSetter(scope, element[0].files[0]);
- });
- });
- }
- };
- }]);
- myApp.service(‘fileUpload’, [‘$https:’, function ($https:) {
- this.uploadFileToUrl = function(file, uploadUrl) {
- var fd = new FormData();
- fd.append(‘file’, file);
- $https:.post(uploadUrl, fd, {
- transformRequest: angular.identity,
- headers: {‘Content-Type’: undefined}
- })
- .success(function() {
- })
- .error(function() {
- });
- }
- }]);
- myApp.controller(‘myCtrl’, [‘$scope’, ‘fileUpload’, function($scope, fileUpload) {
- $scope.uploadFile = function() {
- var file = $scope.myFile;
- console.log(‘file is ‘ );
- console.dir(file);
- var uploadUrl = “/fileUpload”;
- fileUpload.uploadFileToUrl(file, uploadUrl);
- };
- }]);
- </script></body>
- </html>
- Result
- Open above saved code file in a web browser. See the result.
AngularJS – In-line Application
We are providing an example of an in-line app. To develop this app, we have used HTML, CSS and AngularJS.
AngularJS – Custom Directives
Custom directives are used in AngularJS to extend the functionality of HTML. Custom directives are defined using the “directive” function. A custom directive simply replaces the element for which it is activated. AngularJS application during bootstrap finds the matching elements and does one time activity using its compile() method of the custom directive then processes the element using link() method of the custom directive based on the scope of the directive. AngularJS provides support to create custom directives for following types of elements.
- Element directives − Directive activates when a matching element is encountered.
- Attribute − Directive activates when a matching attribute is encountered.
- CSS − Directive activates when a matching css style is encountered.
- Comment − Directive activates when a matching comment is encountered.
Understanding Custom Directive:
Define custom html tags.
- <student name = “Mahesh”></student><br/>
- <student name = “Piyush”></student>
- Define custom directive to handle above custom html tags.
- var mainApp = angular.module(“mainApp”, []);
- //Create a directive, first parameter is the html element to be attached.
- //We are attaching student html tag.
- //This directive will be activated as soon as any student element is encountered in html
- mainApp.directive(‘student’, function() {
- //define the directive object
- var directive = {};
- //restrict = E, signifies that directive is Element directive
- directive.restrict = ‘E’;
- //template replaces the complete element with its text.
- directive.template = “Student: <b>{{student.name}}</b> ,
- Roll No: <b>{{student.rollno}}</b>”;
- //scope is used to distinguish each student element based on criteria.
- directive.scope = {
- student : “=name”
- }
- //compile is called during application initialization. AngularJS calls
- it once when the html page is loaded.
- directive.compile = function(element, attributes) {
- element.css(“border”, “1px solid #cccccc”);
- //linkFunction is linked with each element with scope to get the element specific data.
- var linkFunction = function($scope, element, attributes) {
- element.html(“Student: <b>”+$scope.student.name +”</b> ,
- Roll No: <b>”+$scope.student.rollno+”</b><br/>”);
- element.css(“background-color”, “#ff00ff”);
- }
- return linkFunction;
- }
- return directive;
- });
- Define controller to update the scope for directive. Here we are using the name attribute’s value as scope’s child.
- mainApp.controller(‘StudentController’, function($scope) {
- $scope.Mahesh = {};
- $scope.Mahesh.name = “Mahesh Parashar”;
- $scope.Mahesh.rollno = 1;
- $scope.Piyush = {}; $scope.Piyush.name = “Piyush Parashar”;
- $scope.Piyush.rollno = 2;
- });
Example
- <html>
- <head>
- <title>Angular JS Custom Directives</title>
- </head>
- <body>
- <h2>AngularJS Sample Application</h2>
- <div ng-app = “mainApp” ng-controller = “StudentController”>
- <student name = “Mahesh”></student><br/>
- <student name = “Piyush”></student>
- </div>
- <script src = “https://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js”>
- </script>
- <script>
- var mainApp = angular.module(“mainApp”, []);
- mainApp.directive(‘student’, function() {
- var directive = {};
- directive.restrict = ‘E’;
- directive.template = “Student: <b>{{student.name}}</b> ,
- Roll No: <b>{{student.rollno}}</b>”;
- directive.scope = {
- student : “=name”
- }
- directive.compile = function(element, attributes) {
- element.css(“border”, “1px solid #cccccc”);
- var linkFunction = function($scope, element, attributes) {
- element.html(“Student: <b>”+$scope.student.name +”</b> ,
- Roll No: <b>”+$scope.student.rollno+”</b><br/>”);
- element.css(“background-color”, “#ff00ff”);
- }
- return linkFunction;
- }
- return directive;
- });
- mainApp.controller(‘StudentController’, function($scope) {
- $scope.Mahesh = {};
- $scope.Mahesh.name = “Mahesh Parashar”;
- $scope.Mahesh.rollno = 1;
- $scope.Piyush = {};
- $scope.Piyush.name = “Piyush Parashar”;
- $scope.Piyush.rollno = 2;
- });
- </script>
- </body>
- </html>
Conclusion:
Angular is way popular, dynamic, and most adaptable by the enterprises. Every project we did with AngularJS — and we mean every single one — was designed and developed to build an enterprise solution with the same methodology and belief.