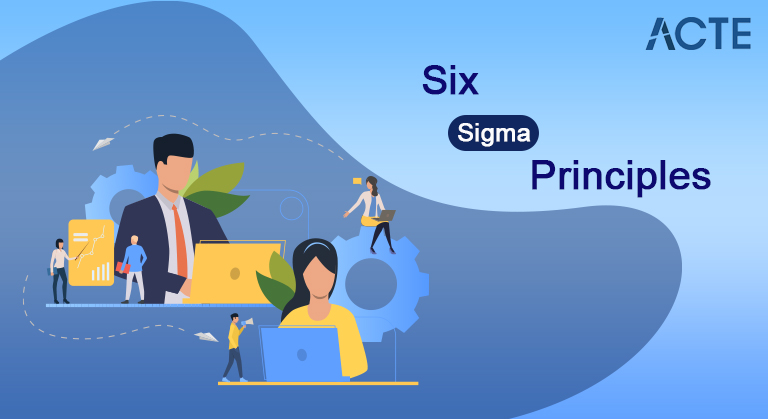
- Introduction to Angular 6
- Components in Angular 6
- Services and Dependency Injection
- Key feature of Angular 6
- Understanding Angular Architecture
- Routing and Navigation
- HTTP Client in Angular
- RxJS and Reactive Programming
- Angular 6 Development Process Without Coding
- Performance Optimization in Angular 6
- Conclusion
Angular 6 is a tutorial centred around building up your web development skills all the way you can go in the Angular framework. Whether beginner or professional developer, anyone wanting to master the full range of skills within Angular, this guide covers everything from core concepts, such as component architecture to more complex techniques, such as reactive programming, state management, and performance optimization. This fully comprehensive guide stands out through expert insights, real-life examples, and best practices, allowing you to learn how to design scalable, efficient, and maintainable web applications with Angular 6.
Introduction to Angular 6
Angular 6 is an open-source, modern framework by Google for building dynamic, responsive web applications. At Angular 6, something far better has been added to the list: an improvement in the capabilities of creating a single-page application (SPAs) with TypeScript, improved performance, an even more efficient CLI, and Angular Elements, helping developers make reusable custom elements. With modular architecture, two-way data binding, and the support of reactive programming, Angular 6 easily enables developers to build web applications that are very scalable, maintainable, and performant.
Want to Earn Your Angular 6 Professional Certification? Check Out the Angular 6 Certification Course Available at ACTE Now!
Components in Angular 6
Angular 6 developers consider components as the base units for defining a user interface’s structure and behaviour. An aspect common among every such component consists of three basic parts, including a class in TypeScript that encapsulates logic, an HTML template that defines the visual structure, and a CSS file for styling the actual structure. The Angular 6 components are highly modular, with easy building, maintaining, and scaling applications. Components can communicate with each other using inputs and outputs, enabling data to flow directly across the application. The `@Component` decorator is used for providing any component-specific details, like a template, styles, and a selector indicating how the component is invoked in the application. This architecture is more related to reusability, maintainability, and testability.
Services and Dependency Injection
- What are Services?
Services are central to organizing reusable business logic, such as retrieving data and API interactions, to reuse several components in Angular 6. This results in cleaner and more maintainable components that can focus more on UI-related tasks.
- Dependency Injection (DI) System
The dependency injection (DI) mechanism of Angular is quite important when it comes to providing these services to components. With DI, services can be injected into a component or another service effortlessly, which provides a good scope for easy modularization and reuse.
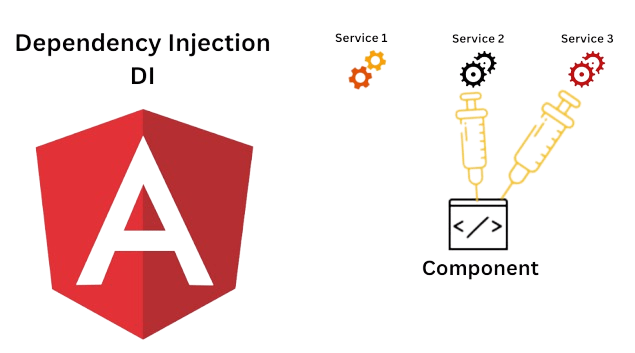
- The @Injectable Decorator
The `@Injectable` decorator marks the class as a service, available for injection at places within the application. It tells the framework that the class can be provided to other components whenever required.
- Benefits of DI
The injector in Angular efficiently manages service instances, promoting a maintainable, testable, and scalable application. This modular architecture decouples components from their dependencies, making development and testing easier. Similarly, React Redux offers a clean separation of state management in React applications, enhancing scalability and simplifying the development process.
A key feature of Angular 6
- Ivy Renderer(Preview):Ivy is a preview of an Ivy renderer in Angular 6 that improves an application’s size and performance. Ivy can make the rendering of the components more efficient. It reduces the bundle size significantly. But this is only relevant to mobile app performance.
- CLI Prompts:The Angular CLI features included several improvements to prompt during project setup. This feature walks the developer through the creation of a new project, offers options for features that they may want in a project at its inception, and, in general, makes the first-time configuration a friendlier place.
- Angular Elements:Another strong feature is the opportunity for developers to use reusable components that can be applied everywhere, not just in Angular applications. These components can be used in non-angular applications or plain HTML pages. This feature is handy for teams working on legacy applications that might want to adopt Angular slowly.
- Service Worker Support:Angular 6 brings better support for service workers. These improvements make it super easy to create Progressive Web Applications, which work offline and load in a flash-super fast so that it feels native on the web. This is something crucial to web apps nowadays.
- Tree Shakable Providers:This feature optimizes the application by allowing Angular to tree-shake unused services, reducing the final bundle size. Tree shaking is a dead code elimination technique applied in module bundlers for the purpose of removing dead code from the output.
- RxJS 6:Angular 6 introduced RxJS 6, reactive programming’s powerful way of managing asynchronous data streams. The library RxJS 6 also introduces a new pipeable operators approach, improving the code’s readability and maintainability.
- HTML Template defines the view layout.
- CSS Styles are specific to the element.
- Typescript Class representation of the logic and data inside a component.
- Introduction to Routing
Routing is one of the most important features provided in Angular 6. The term refers to the nature of this feature; it lets an application’s user navigate among views or components supplied with the application. Using routing, developers can build SPAs that load content dynamically without refreshing a page in a single move.
- Configuring Routes
Routes are defined within the application by mapping URL paths to components. This is done by using the RouterModule and configuring routes in a service called a routing module.
- Routing Between Routes
Angular gives users several ways to navigate between routes. Router links can be added to templates using the routerLink directive so the user can navigate by clicking links.
- Route Parameters and Guards
Angular 6 Routing supports route parameters, in which all the dynamic data can be managed within the URLs. For instance, a route may have its parameters to fetch particular data according to the choices that users make.
- Key Concepts
Observables are data streams emitting multiple values over time, allowing subscriptions to react to new data. A lot of transforms and manipulations operators, including but not limited to `map`, `filter`, `mergeMap`, and `switchMap`.
- Integration with Angular
The integration of RxJS with Angular is smooth and more visible when applying the HTTP client, which returns observables to handle responses and errors, for instance. Angular forms also use RxJS to validate users’ input and perform subsequent operations.
- Benefits
Reactive programming with RxJS helps drive for better performing, responsive applications while using a declarative style to minimize the headache associated with why and after which side effects in cleaner code and better user experiences.
- Setting Up the Development Environment:
Install Node.js, which contains the Node Package Manager. This gives one access to the tools necessary when managing JavaScript packages in building Angular. Install the Angular CLI. The command-line interface provides a way to set up and develop applications in Angular. It contains various commands necessary for creating and managing an Angular application efficiently.
- Creating a New Angular Application:
Create a New Project: you can create a new angular application by running a command from the Angular CLI. This command will automatically set up a directory structure, configuration files, and needed dependencies, making it a basic project structure.
- Structure Basics:
Know the Directory Structure: glance around the angular application created for you. The most important directories are:Src/: all the application’s source code is located inside, including all the different components and services.app /: Hosts the core application module and page components.assets/: Location for static assets such as images and stylesheets.
- Components Registration:
Components declaration forms the base unit in an Angular application. Think about what components your app requires and the roles and responsibilities they will entail in the design. Each component is typically composed of a template (HTML), styles (CSS), and logic, usually in the form of a class typed in TypeScript. To create components effectively, one must also understand how to use various HTML Entities within the template.
- Services:
Services encapsulate the business logic and data access. Determine what services your application will require so that it may manage data operations and the rules of business. Inject Services into Components Find out how to provide these services to components so that you can promote the reusability and maintainability of the application components.
- Routing Configuration:
Routing Plan Navigation: How would you structure your application’s routes to navigate different views or components? This would encompass the identification of the main routes and the associated components. Implement Route would like to take advantage of the router module that Angular provided and let it handle navigating from one view to another. You should clearly define a structure for your routes and how the user will consume those routes.
- Managing Forms:
Form Needs Determine which forms you need in an application so that you can get input from users. For example, registration and sign-up forms, login forms, or data entry forms. Form Style Choice is to choose between template-driven forms or reactive forms. Your application will decide which one would be a better fit for you, and both are good choices for suitable applications.
- Making HTTP Requests:
API Integration Plan Begin by finding the APIs your application will communicate with. Familiarize yourself with how their APIs will resolve data extraction and modification.Configuring the HTTP Client Angular provides an HTTP client module for sending and receiving responses. Get familiar with the HTTP API call structure and data handling in the services.
- Lazy Loading: The method of lazy loading allows modules to be loaded only when necessary, decreasing the application’s initial load time. Routes can also be set to initialize modules asynchronously. This approach significantly enhances performance, making it particularly beneficial in large applications and essential for effective Full Stack Web Development .
- Change Detection Strategy: Angular’s change detection mechanism tracks the state of your application for changes. This operation can be optimized using the OnPush change detection strategy of components, which yields fewer checks by Angular for better performance, especially when dealing with extensive component trees.
- TrackBy in NgFor: When you use *ngFor to render lists, you can use the track function to improve rendering. This will help Angular tell which items have changed, been added or removed so that it only re-renders the necessary parts of the DOM instead of re-rendering the whole list.
- How to prevent Memory Leaks: This will deal with subscriptions to prevent memory leaks when using observables. To clean up, use subscriptions within components. This should be done in the ngOnDestroy lifecycle hook.
- Ahead-of-Time (AOT) Compilation: Can speed up application loads. Compiling the application during the build rather than at runtime can result in smaller bundle sizes and enhanced rendering in the browser.
- Tree Shaking: Tree shaking removes unused code from the final bundle. Using Angular CLI and following best practices ensures that only the necessary portions of your application will make it into production.
Angular 6 Sample Resumes! Download & Edit, Get Noticed by Top Employers! Download
Conclusion
Mastering Angular 6 demonstrates the significance of Angular 6 in every step to green-handed people and veterans who want to learn and enhance their knowledge about Angular 6. This book covered basic features, best practices, as well as advanced techniques that will enable developers to create strong and scalable applications. By mastering concepts such as components, services, routing, and performance optimization, you will grow your development capabilities and build high-quality applications that give outstanding user experiences. With the state of the Angular ecosystem in constant evolution, mastering the latest trends and practices will be essential to your success. We encourage you to put this guide into practice and continue your journey toward mastering Angular.
To Obtain Your Angular 6 Certification, Learn from Leading Angular 6 Experts and Advance Your Career with ACTE’s Angular 6 Certification Course .
Understanding Angular Architecture
Modules:Angular applications consist of several modules encapsulating related functionalities. Every Angular application has at least one root module, usually called AppModule, which bootstraps the application.
Components:Components are building blocks of an angular application. Every component controls a part of the user interface and consists of three main parts:
TemplatesAngular templates are HTML views with Angular directives that include expressions and bindings. Dynamically, data can be displayed using those templates. The capability to react to user events, such as mouse clicks, also makes them eligible.
ServicesServices are reusable chunks of code that embody business logic and data access. Based on the requirements, services will be injected into components or other services through Angular’s dependency injection system. It supports code reusability and separation of concerns.
Dependency InjectionAngular comes with a dependency injection system that helps you manage service instances. This system allows you to easily inject any dependency into services and components you create while improving the code’s cleanliness and maintainability.
Routing and Navigation
HTTP Client in Angular
The Angular HTTP Client module provides a strong and flexible solution for communication over HTTP with backend services. Angular 4.3 simplifies the process of making HTTP requests, handling responses, and managing data interactions in an Angular application. To use the HTTP Client, it’s necessary to import `HttpClientModule` into your application module. Once you are set up, you will inject the `HttpClient` service into your components or services and thus can enjoy using several request methods, such as GET, POST, PUT, and DELETE.
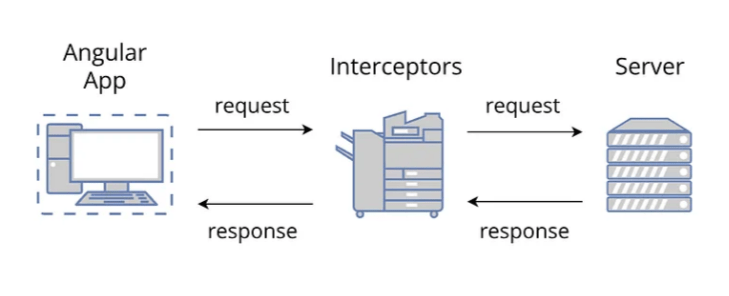
Each will return an `Observable` for you to handle asynchronous data efficiently. The HTTP Client simplifies the management of responses and data transformation through RxJS operators, such as `map`, `catchError`, and `tap`. Moreover, it supports angular’s HttpClient with interceptors, by globally handling the request and response flow. It can be necessary while handling authentication tokens or error responses.
Ready to Master Full Stack Developer? Explore the Full Stack Developer Masters Program Available at ACTE Today!
RxJS and Reactive Programming
RxJS is a library of extremely strong reactive extensions for JavaScript that enables observables to work on asynchronous data streams. Regarding Angular, RxJS is central in managing events, handling HTTP responses, and building an interactive user interface.
Angular 6 Development Process Without Coding
Geared Up for an Angular 6 Job Interview? Browse Our Comprehensive Set of Angular 6 Interview Questions to Help You Prepare!