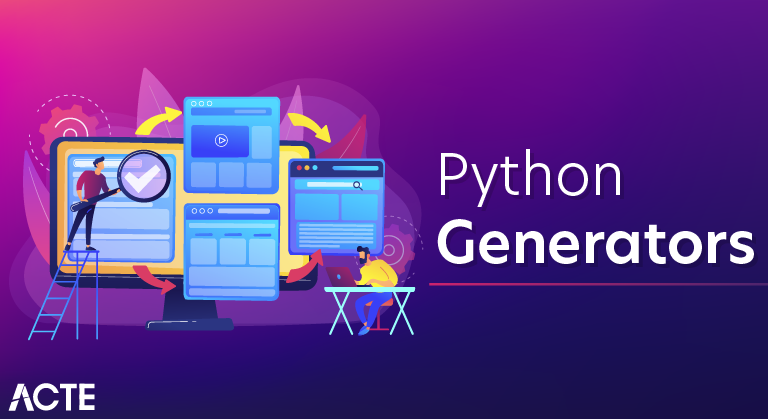
Must-Know Python Generators & How to Master It
Last updated on 28th Jun 2020, Blog, General
Python generators are a powerful, but misunderstood tool. They’re often treated as too difficult a concept for beginning programmers to learn — creating the illusion that beginners should hold off on learning generators until they are ready.
I think this assessment is unfair, and that you can use generators sooner than you think. In this tutorial, we’ll cover:
- The basic terminology needed to understand generators
- What a generator is
- How to create your own generators
- How to use a generator and generator methods
- When to use a generator
Python provides a generator to create your own iterator function. A generator is a special type of function which does not return a single value, instead it returns an iterator object with a sequence of values. In a generator function, a yield statement is used rather than a return statement. The following is a simple generator function.
Example: Generator Function Copy
def myGenerator(): print(‘First item’) yield 10 print(‘Second item’) yield 20 print(‘Last item’) yield 30
What is a Generator?
- A Python generator is a function that produces a sequence of results. It works by maintaining its local state, so that the function can resume again exactly where it left off when called subsequent times.
- Thus, you can think of a generator as something like a powerful iterator.
- Generators are very easy to implement, but a bit difficult to understand.
- Generators are used to create iterators, but with a different approach. Generators are simple functions which return an iterable set of items, one at a time, in a special way.
- When an iteration over a set of item starts using the for statement, the generator is run.
- Once the generator’s function code reaches a “yield” statement, the generator yields its execution back to the for loop, returning a new value from the set. The generator function can generate as many values (possibly infinite) as it wants, yielding each one in its turn.
- The state of the function is maintained through the use of the keyword yield, which has the following syntax
yield [expression_list]
- This Python keyword works much like using return, but it has some important differences, which we’ll explain throughout this article.
- Generators were introduced in PEP 255, together with the yield statement. They have been available since Python version 2.2.
- How do Python Generators Work?
- In order to understand how generators work, let’s use the simple example below:
# generator_example_1.py
- def numberGenerator(n):
- number = 0
- while number < n:
- yield number number += 1
myGenerator = numberGenerator(3)
print(next(myGenerator)) - print(next(myGenerator))
- print(next(myGenerator))
- The code above defines a generator named number Generator, which receives a value n as an argument, and then defines and uses it as the limit value in a while loop. In addition, it defines a variable named number and assigns the value zero to it.
- Calling the “instantiated” generator (myGenerator) with the next() method runs the generator code until the first yield statement, which returns 1 in this case.
- Even after returning a value to us, the function then keeps the value of the variable number for the next time the function is called and increases its value by one. So the next time this function is called, it will pick up right where it left off.
- Calling the function two more times, provides us with the next 2 numbers in the sequence, as seen below:
$ python generator_example_1.py
0
1
2
- If we were to have called this generator again, we would have received a StopIteration exception since it had completed and returned from its internal while loop.
- This functionality is useful because we can use generators to dynamically create iterables on the fly.
- If we were to wrap myGenerator with list(), then we’d get back an array of numbers (like [0, 1, 2]) instead of a generator object, which is a bit easier to work with in some applications.
Key takeaways: basic terms to know
Iteration is the idea of repeating some process over a sequence of items. In Python, iteration is usually related to the for loop.
An iterable is an object that supports iteration.
To be an iterable, it must describe to a for loop two things:
What item comes next in the iteration.
When should the loop stop iteration.
Generators are iterables.
Generators and you
If you’ve never encountered a generator before, the most common real-life example of a generator is a backup generator, which creates
generates — electricity for your house or office. Conceptually, Python generators generate values one at a time from a given sequence, instead of giving the entirety of the sequence at once. This one-at-a-time fashion of generators is what makes them so compatible with for loops.
If this sounds confusing, don’t worry too much. As we explain how to create generators, it will become more clear. There are two ways to create a generator.
They differ in their syntax, but the end result is still a generator. We’ll teach these concepts by covering their syntax and comparing them to a similar, but non-generator equivalent.
- A generator function versus a regular function
- A generator expression versus a list comprehension
The generator function
A generator function is just like a regular function but with a key difference: the
yield keyword replaces return.
- # Regular function
- next(a)