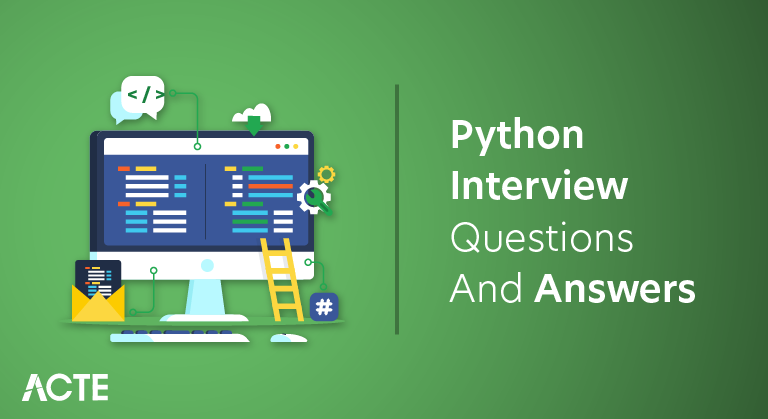
[ 35+ ] Python Interview Questions & Answers [BEST & NEW]
Last updated on 05th Jun 2020, Blog, Interview Questions
Python is a flexible, high-level programming language renowned for its readability and simplicity.Python is a great option for both beginning and expert programmers due to its clear and simple syntax. Procedural, object-oriented, and functional programming are only a few of the programming paradigms that are supported.
1. What are the benefits of Python?
Ans:
Speed and Productivity: Utilizing productivity and speed of Python will enhance process control capabilities and possesses a strong integration.
Extensive Support for Libraries: Python has a large standard library that covers topics including operating system interfaces, tools for online services, internet protocols, and string protocols.
User-friendly Data Structures: Python has in-built dictionary of data structures that are used to build the fast user-friendly data structures.
Easy Learning: Python provides an excellent readability and simple syntaxes to make it simple for beginners to learn.
2. What is the use of %s?
Ans:
- It is useful to format the value in a string.
- It is put where string is to be specified.
- Automatically provides the type conversion from value to the string.
3. What are the key features of Python?
Ans:
- Interpreted Language
- Highly Portable
- Extensible
- GUI programming Support
4. What is Interpreted language?
Ans:
The statements in an interpreter language are executed line by line. The best examples of interpreted languages include Python, Javascript, R, PHP, and Ruby. There is no intermediate compilation step required for programs written in an interpreted language; they execute straight from the source code.
5. What are the applications of Python?
Ans:
- GUI-based desktop applications
- Image processing applications
- Business and Enterprise applications
- Prototyping
- Web and web framework applications
6. Distinguish difference between a list and a tuple in Python
Ans:
List | Tuple |
---|---|
Lists are changeable, which implies they may have their contents changed after they are created. | Tuples are immutable, which means that once you construct one, you cannot modify its contents. |
A list can have elements added, removed, or changed. | A tuple’s elements cannot be added, removed, or modified. |
7. What are global and local variables in Python?
Ans:
Global Variables: A global variable is one that is declared at the beginning of a script or module, outside of any function or code block.
Local Variables: A function or a section of code that defines a local variable.It can only be used and understood within the function or block where it is declared.
8. Define PYTHON PATH
Ans:
PYTHONPATH is the environmental variable that is used when import a module. Suppose at any time import a module, PYTHONPATH is used to check the presence of modules that are imported in the different directories. Loading of module will be determined by the interpreters.
9. What are modules in Python?
Ans:
Modules are just Python files with a.py extension that can include a specified and implemented collection of functions, classes, or variables. Using an import statement, they may be imported and initialized once. If partial functionality is needed, import requisite classes or functions using from a foo import bar.
10. Explain term PEP 8
Ans:
A Python Enhancement Proposal, or PEP 8, is the most recent coding standard for the Python programming language. The key to making Python code as readable as possible is proper formatting.
11. How to count the number of instances?
Ans:
In Python memory management is done using the private heap space. The private heap is storage area for all data structures and objects. The interpreter has access to private heap and the programmer cannot access private heap.
12. In Python, what does pass mean?
Ans:
Pass is a placeholder statement that has no effect in Python. When a statement is needed by the language’s syntax but no code should be executed, it is frequently used as a syntactical placeholder. It functions primarily as a “no-op” or a means of momentarily leaving a piece of code empty.
13. Define modules in Python
Ans:
Modules allow you to organize your Python code into separate files. This helps keep your codebase modular and maintainable by breaking it into smaller, manageable pieces.
14. What built-in types are available in Python?
Ans:
- Integer
- Complex numbers
- Floating-point numbers
- Strings
- Built-in functions
15. Define Python Decorators
Ans:
Decorator is most useful tool in Python as it allows the programmers to alter changes in the behavior of class or function.
16. How do you find bugs and statistical problems in a Python?
Ans:
Bugs and statistical problems in a python source code can be detected using the static analysis tool named PyChecker. Moreover, there is another tool called the PyLint that checks whether Python modules meet tcoding standards or not.
17. What are unit tests in Python?
Ans:
Unit tests in Python are a fundamental aspect of software development, used to verify that individual components or units of code, such as functions, methods, or classes, perform as expected. These tests are typically automated and isolated, focusing on checking the correctness of small, specific parts of a program.
18. What is the use of help() and dir() functions?
Ans:
help() function in the Python is used to display documentation of modules, classes, functions, keywords, etc. If no parameter is passed to be help() function, then interactive help utility is launched on a console.
dir() function tries to return valid list of attributes and methods of object it is called upon. It behaves a more differently with various objects, as it aims to produce most relevant data, rather than a complete information.
19. Define String in Python
Ans:
String in a Python is formed using the sequence of characters. Value once assigned cannot be modified because they are immutable objects. String literals in a Python can be declared using the double quotes or single quotes.
Examples:
- print(“Hi”)
- print(‘Hi’)
20. Explain the term namespace in Python
Ans:
A namespace in the Python can be defined as a system that is designed to provide the unique name for each object in python. Types of namespaces that are present in a Python are:
- Local namespace
- Global namespace
- Built-in namespace
21. How do you create a Python function?
Ans:
Functions are explained using the def statement.
- def square(number):
- result = number ** 2
- return result
22. Define iterators in Python
Ans:
In Python, iterator can be explained as an object that can be iterated or traversed upon. In another way, it is majorly used to iterate the group of containers, elements, the same as a list.
23. What does iterators in Python?
Ans:
Iterators are objects with which can iterate the over iterable objects like lists, strings, etc.It remembers its state i.e., where it is a during iteration.It is also a self-iterable.
- __iter__() method initializes iterator.
24. Define slicing in a Python
Ans:
Slicing is the procedure used to select the particular range of items from the sequence types like Strings, lists, and so on.As the name suggests, ‘slicing’ is taking parts of.
- Syntax for a slicing is [start : stop : step]
- start is t starting index from where to slice a list or tuple
- stop is ending index or where to sop.
- step is a number of steps to jump.
- Default value for a start is 0, stop is number of items, step is 1.
25. How can Python be interpreted language?
Ans:
As in Python code which write is not machine-level code before a runtime so, this is reason why Python is called the interpreted language.
26. What happens when a function doesn’t have a return statement?
Ans:
Yes, this is valid. The function will return a None object. The end of the function is defined by a block of code that is executed (i.e., the indenting) not by any explicit keyword.c
27. What does *args and **kwargs mean?
Ans:
*args | Tuple |
---|---|
*args is the special syntax used in function definition to pass variable-length arguments. | **kwargs is the special syntax used in function definition to pass variable-length keyworded arguments. |
“*” means variable length and “args” is name used by the convention. | Here, also, “kwargs” is used just by the convention. |
28. How can make a Python script executable on Unix?
Ans:
In order to make Python script executable on Unix, need to perform two things. They are:
A Script file mode must be executable and
A first line must always begin with #.
29. What is the use of PYTHON PATH?
Ans:
PYTHONPATH is used by Python to search for modules and packages (collections of modules) that are not part of the standard library and are not in the same directory as your script. When you import
a module or package in your Python script, the interpreter searches for it in the directories specified in PYTHONPATH.
30. Define pickling and unpickling
Ans:
Pickling involves converting a Python object into a binary representation that can be saved in a file or sent over a network, while unpickling involves restoring the original Python object from the binary representation.
31. Is indentation required in python?
Ans:
Indentation is necessary for a Python. It specifies the block of code. All code within the loops, classes, functions, etc is specified within indented block. It is usually done using the four space characters. If code is not indented necessarily, it will not execute the accurately and will throw an errors as well.
32. Define boolean in Python
Ans:
Boolean is one of built-in data types in Python, it mainly contains a two values, which are true and false. Python bool() is a method used to convert the value to boolean value.
- Syntax for bool() method: bool([a])
33. What is Python String format?
Ans:
Python provides the str.format() method and the Template strings module for string formatting, offering flexibility and versatility in creating formatted text for a wide range of use cases.
Syntax for String format() method:
- template.format(p0, p1, …, k0=v0, k1=v1, …)
Syntax for a String replace() method:
- str.replace(old, new [, count])
34. List some of the built-in modules in Python
Ans:
- OS module
- random module
- collection module
- JSON
- Math module
35. Define functions in Python
Ans:
A function is a reusable section of code that carries out a single job or group of related actions.It is then followed by the function name and a block of code that describes what the function performs.
36. Differentiate between deep and shallow copies.
Ans:
Shallow Copy: A shallow copy creates a new object, but it only copies references to the objects contained within the original object, rather than recursively copying the objects themselves.
Deep Copy: In contrast, a deep copy makes an entirely separate duplicate of the original object and every item it contains. This implies that alterations to the duplicated structure do not impact the original.
37. Which Python libraries have used for visualization?
Ans:
Data visualization is most important part of data analysis. get to see a data in action, and it helps to find hidden patterns.
The most famous Python data visualization libraries are:
- Matplotlib
- Seaborn
- Plotly
- Bokeh
38. Distinguish difference between Arrays and Matrices
Ans:
Arrays: A list of items with related data types. A matrix is created by joining two arrays.
Matrices: Two-dimensional representation of a data. It consists of robust mathematical operation set that lets manipulate a data in many ways
39. Define self in Python
Ans:
In Python self is explained as an object or an instance of class. This self is explicitly considered as a first parameter in Python. Moreover, can also access all the methods and attributes of a class in the Python programming using self keyword.
40. What is _init_?
Ans:
The _init_ is the special type of method in Python that is called automatically when memory is allocated for a new object.
41. Define generators in Python
Ans:
Generator is a specific kind of iterable that resembles a list or a tuple but differs significantly in that it creates values as needed rather than holding them in memory. Functions and the yield keyword are used to define generators.
42. What is Pandas Series?
Ans:
Series is a one-dimensional data structure that can store data of nearly any kind. It resembles an excel column. It is used for single-dimensional data manipulations and supports multiple operations.
43. What is PIP software in Python?
Ans:
PIP stands for a Python Installer Package, which provides the seamless interface to install various Python modules. It is the command-line tool that searches for packages over Internet and installs them without any user interaction.
44. What are pandas groupby?
Ans:
A pandas groupby is the feature supported by pandas that are used to be split and group an object. Like sql/mysql/oracle groupby it is used to group data by the classes, and entities which can be further used for the aggregation. A dataframe can be grouped by a one or more columns.
45. How does for Loop and while Loop differs in Python?
Ans:
“for” Loop is generally used to iterate through elements of various collection types like List, Tuple, Set, and Dictionary. Developers use “for” loop where they have both conditions start and the end.“while” loop is actual looping feature that is used in any other programming language. A Developers use while loop where they just have end conditions.
46. How to implement JSON in Python?
Ans:
Python has built-in support to handle the JSON objects. The user just has to import JSON module and use functions such as loads and dumps to convert the JSON string to JSON object and vice versa.
47. How do you use C to access the Python module?
Ans:
Can access the module written in Python from C by using following method.
- Module == PyImport_ImportModule(“”);
48. What is multi-threading?
Ans:
Multithreading is the programming technique that allows the multiple threads of execution to run concurrently within a single process. A thread is a lightweight unit of an execution within a program that can perform a tasks independently.
49. Distinguish difference between tuple and a dictionary
Ans:
One major difference between the tuple and a dictionary is that the dictionary is mutable while tuple is not. Meaning the content of the dictionary can be changed without changing its identity, but in tuple, that’s not possible.
50. Distinguish between SciPy and NumPy
Ans:
NumPy | SciPy |
---|---|
Numerical Python is called NumPy. | Scientific Python is called SciPy. |
It is used for performing the general and efficient computations on numerical data which is saved in arrays. | This is the entire collection of tools in Python mainly used to perform the operations like differentiation, integration and many more. |
51. How do Python arrays and lists differ from each other?
Ans:
Arrays : The array is explained as a linear structure that is used to save only homogeneous data. Since array stores the only a similar type of data so it occupies less amount of a memory when compared to list. The length of array is fixed at a time of designing and no more elements can be added in middle.
Lists: The list is used to save arbitrary and heterogeneous data. List stores the different types of data so it requires the huge amount of memory . The length of the list is no fixed, and adding an items in middle is possible in lists.
52. Can you make multi-line comments in Python?
Ans:
In python, there is no specific syntax to display the multi-line comments like in the other languages. In order to display the multi-line comments in Python, programmers use the triple-quoted (docstrings) strings. If docstring is not used as a first statement in present method, it will not be considered by a Python parser.
53. Distinguish between range and xrange
Ans:
Range() method : The range() method is not supported in the Python3 so that range() method is used for an iteration in for loops. The list is returned by this range() method.
Xrange() method: The xrange() method is used only in the Python version 2 for iteration in loops. It only returns generator object because it does not produce a static list during the run time.
54. What is Django?
Ans:
Django is the advanced python web framework that supports the agile growth and clean a pragmatic design, built through an experienced developers, this cares much about trouble of web development, so can concentrate on writing the app without wanting to reinvent the wheel.
55. List the features of Django
Ans:
- Excellent documentation
- Python web framework
- SEO optimized
- High scalability
- Versatile in nature
56. What is a classifier?
Ans:
A classifier is used to predict class of any data point. Classifiers are special hypotheses that are used to assign the class labels to any particular data point. A classifier often uses training a data to understand the relation between the input variables and the class.
57. What are the advantages of Django?
Ans:
- Django is the multifaceted framework
- When it comes to a security Django is the best framework
- A Scalability is added advantage of Django
58. Why should I use the Django framework?
Ans:
The main goal to designing the Django is to make it easy to its users, to do this Django uses:
- The principles of concerning rapid development, which implies the developers can complete more than one iteration at a time without beginning a full schedule from scratch;
- DRY philosophy —that means developers can reuse the surviving code and also focus on individual one.
59. What common security issues are avoided by using Django?
Ans:
- Clickjacking
- Cross-site scripting and
- SQL injection
60. What are global attribute in Python?
Ans:
Global attributes generally refer to variables, functions, or objects that are defined in the global scope of a program or module. The global scope is the outermost scope in a Python script or module and is not contained within any function or class.
61. What is Django framework?
Ans:
Django is a high-level, open-source Python web framework that simplifies and accelerates web development by providing a robust set of tools and features. It follows the “batteries-included” philosophy, meaning it comes with many built-in components and libraries to handle common web development tasks, allowing developers to focus on writing application-specific code rather than dealing with low-level details.
62. List steps for setting up a static files in Django
Ans:
- Firstly set the STATIC_ROOT in settings.py
- Run manage.py collect static
- Setting up the static file entry pythonAnywhere tab
63. What is recursion?
Ans:
Recursion is the function calling itself one or more times in it body. One very important condition of recursive function should have to be used in a program is, it should be terminate, else there would be problem of an infinite loop.
64. Differentiate Django reusability code with the other frameworks
Ans:
Django web framework is can operated and also maintained by the autonomous and non-profit organization designated as a Django Software Foundation (DSF). The initial foundation goal is to be promote, support, and advance this Django Web framework.
65. List the mandatory files of Django project?
Ans:
- manage.py
- settings.py
- __init__.py
- urls.py
- wsgi.py
66. Explain about Django session
Ans:
A session comprises the mechanism to store information on specific server-side at interaction by web application. By default, session reserves in database and allows file-based and cache-based sessions.
67. Why do use a cookie in Django?
Ans:
A cookie is the piece of information that is stored in the client’s browser for specific time. When specific time is completed cookie gets an automatically removed from a client browser.
68. Mentions the methods used for a setting and getting cookie values
Ans:
- Set_cookie this method is used to set values of a cookie
- Get_cookie this method is used to get values of a cookie
69. What is the ‘with statement’?
Ans:
Python’s “with” statement is utilized in exception handling. Without utilizing the close() method, a file can be opened and closed while a block of code containing the “with” expression is being run. In essence, it makes a code easier to read.
70. What is the use of manage.py?
Ans:
It is automatically built file inside every Django project. It is the flat wrapper encompassing Django-admin.py. It possesses following usage:
- It establishes the project’s package on sys.path.
- It fixes DJANGO_SETTING_MODULE environment variable to point to the project’s setting.py file.
71. Why is Django loosely packed?
Ans:
Django has described as loosely coupled framework because of MTV architecture it’s based upon. Django’s architecture means the variant of MVC architecture and also MTV is more helpful because this completely separates server code of client’s machine.
72. How to launch sub-processes within the main process of Python application?
Ans:
Python has built-in module called sub-process. And can import this module and either use the run() or Popen() function calls to launch subprocess and get control of its return code.
73. What are the different environment variables identified by Python?
Ans:
- PYTHONPATH: This environment variable helps interpreter where to locate module files imported into the program.
- PYTHONSTARTUP: This environment variable contains a path of Initialization file containing source code.
- PYTHONCASEOK: This variable is used to find first case-insensitive match in import statement.
74. What is the use of Assertions in Python?
Ans:
Assertions in Python are used to check whether a given condition is true or false and are primarily used for debugging and testing purposes. When you use assertions in your code, you are essentially stating that a particular condition should always hold true at a specific point in your program’s execution.
75. What is break statement in Python?
Ans:
The break statement is a control flow statement used to exit or terminate a loop prematurely. It is commonly used in for and while loops to stop the loop’s execution when a certain condition is met, even if the loop’s specified iteration conditions are not fully satisfied.
76. Is flask an MVC model?
Ans:
Flask is not a strict MVC (Model-View-Controller) framework like some other web frameworks, such as Django. Instead, Flask is often described as a microframework for web development.
77. How Database connections made in Python ?
Ans:
Database-powered the applications are supported by a flask. The relational database systems need to create the schema that requires a piping the schema.sql file into SQLite3 command. So, in this case, need to install SQLite3 command on the system to initiate and create a database in the flask.
78. Explain the procedure to minimize or lower the outages of the Memcached server in the Python development
Ans:
High Availability Setup
Monitoring and Alerts
Regular Backups
Automatic Failover
Load Balancing
Graceful Degradation
79. What is Dogpile effect?
Ans:
This is defined as occurrence of an event when cache expires and also when websites are hit with more requests by a client at a time. This dogpile effect can be averted by use of a semaphore lock. If in particular system value expires then, first of all, particular process receives a lock and begins generating a new value.
80. What OOP’s concepts available in Python?
Ans:
- Object
- Class
- Method
- Encapsulation
- Abstraction
- Inheritance
- Polymorphism
81. Define object in Python
Ans:
An object in a Python is defined as an instance that has both the state and behavior. Everything in Python is made of the objects.Below are important module for a Data Science :
82. What is class in Python?
Ans:
Class is defined as the logical entity that is huge collection of objects and it also contains both the methods and attributes. The class creates the user-defined data structure, which holds its a own data members and member functions, which can be accessed and used by creating the instance of that class.
83. How to create a class in Python?
Ans:
In Python programming, the class is created using the class keyword. syntax for creating the class is:
- class ClassName:
- #code (statement-suite)
84. What is the syntax for creating an instance of class in Python?
Ans:
The syntax for creating the instance of a class is as follows:
= ( )
85. Define what is “Method” in Python programming
Ans:
The Method is defined as a function associated with the particular object. The method which define should not be unique as class instance. Any type of object can have the methods.
86. What is data abstraction in Python?
Ans:
Abstraction can be defined as hiding the unnecessary data and showing or executing necessary data. In the technical terms, abstraction can be explained as hiding internal processes and showing only functionality. In Python abstraction can be achieved by using the encapsulation.
87. Define encapsulation in Python
Ans:
Encapsulation is one of the most important aspects of the object-oriented programming. The binding or wrapping of a code and data together into the single cell is called encapsulation. Encapsulation in the Python is mainly used to be restrict access to methods and variables.
88. What is polymorphism in Python?
Ans:
By using the polymorphism in Python will understand how to perform the single task in different ways. For example, designing the shape is the task and various possible ways in the shapes are a triangle, rectangle, circle, and so on.
89. Does multiple inheritances are supported in the Python?
Ans:
The Multiple inheritances are supported in a python. It is the process that provides flexibility to inherit the multiple base classes in a child class.
90. Does Python make use of the access specifiers?
Ans:
Python does not make use of the access specifiers and also it does not provide the way to access an instance variable. Python introduced the concept of prefixing name of the method, function, or variable by using the double or single underscore to act like a behavior of private and protected access specifiers.
91. How to create an empty class in Python?
Ans:
Empty class in a Python is defined as the class that does not contain any code defined within block. It can be created using the pass keywords and object to this class can be created outside class itself.
92. Define Constructor in Python
Ans:
Constructor is the special type of method with the block of code to initialize a state of instance members of a class. A constructor is called only when instance of a object is created. It is also used to verify they are sufficient resources for the objects to perform specific task.
93. What is a dynamically typed language?
Ans:
Type checking is important part of any programming language which is about an ensuring minimum type errors. The type defined for the variables are checked either at a compile-time or run-time. When type-check is done at compile time then it is called a static typed language and when type check is done at run time, it’s called a dynamically typed language.
94. Define Inheritance in Python
Ans:
When an object of a child class has ability to acquire the properties of the parent class then it is called inheritance. It is majorly used to acquire a runtime polymorphism and also it provides a code reusability.
95. What are ODBC modules in Python?
Ans:
The Microsoft Open Database Connectivity is the interface for the C programming language. It is standard for all the APIs using database C. If use a Python ODBC interface with standard ODBC drivers that ship with the most databases, can likely connect the Python application with most databases in a market. The various Python ODBC modules are pyodbc, PythonWin ODBC, and MxODBC.
96. What does docstring mean in Python?
Ans:
Docstring is a special type of string literal that is used to document a module, function, class, or method. It provides a way to describe the purpose, behavior, and usage of the code to make it more understandable and user-friendly. Docstrings are not just comments; they serve as a form of documentation that can be accessed and displayed programmatically.
97. In Python, what does Polymorphism?
Ans:
In Python, Polymorphism makes us understand how to perform tasks in various ways in Python. It is useful in providing flexibility in task processes. Through polymorphism, a class’s objects can invoke the other class’s methods, allowing for code reuse. Polymorphism also allows the subclasses to override the methods of superclass, allowing for further code reuse.
98. Is indentation required in python?
Ans:
Yes,Python does require indentation. To fix ridiculous indentation issues, substitute tabs with single spaces. Indentation helps developers comprehend code in all programming languages, but in Python, it’s very crucial to indent the code in a particular sequence.
99. What does Python “slicing” mean?
Ans:
In Python, “slicing” refers to the process of extracting a portion (a subsequence) of a sequence, such as a string, list, or tuple. Slicing allows you to create a new sequence that contains a subset of the elements from the original sequence.
100. What is swapcase() function in Python?
Ans:
The string’s purpose is to convert all uppercase letters to lowercase letters and vice versa. It is used to alter the string’s current instance. In the string produced by this procedure, every character in the swap case is copied. When a string is lowercase, it creates a small case string, and vice versa. All non-alphabetic letters are automatically ignored by it.