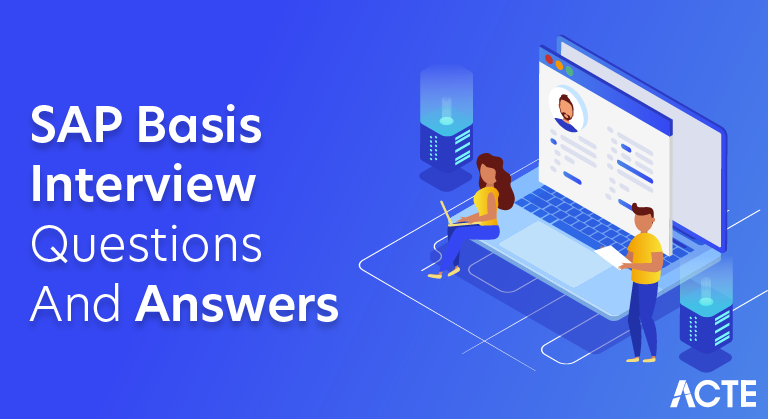
A Design Patterns course introduces students to fundamental principles and best practices in software engineering, focusing on solving common design problems encountered in software development. The course typically begins by elucidating the importance of design patterns in building scalable, maintainable, and flexible software systems. It then delves into various categories of design patterns, such as creational, structural, and behavioral patterns.
1.What are design patterns?
Ans:
Design patterns represent reusable resolutions to frequently encountered issues in software design.They establish a blueprint for addressing specific design dilemmas consistently.These patterns encapsulate superior methods within object-oriented design.They foster code reusability, maintainability, and scalability.Notable examples encompass Singleton, Factory Method, and Observer patterns.Employing design patterns enhances code comprehension and maintainability.
2.What are the advantages of Java Design Patterns?
Ans:
- Java design patterns amplify code reuse and maintainability.
- They furnish a systematic approach to tackling common design challenges.
- Patterns foster scalability and adaptability in software development.
- They facilitate communication among developers by employing a shared language.
- Java design patterns aid in error reduction and the enhancement of code quality.
- These patterns encapsulate superior practices, fostering more resilient and efficient code.
3.How can users describe a design pattern?
Ans:
A design pattern is a reusable solution to common software design problems, describing both the problem and its solution within a specific context. Documented as adaptable templates, they provide guidance for crafting software components, promoting modularity, flexibility, and maintainability. Examples include Singleton, Strategy, and Observer patterns.
4.What are the types of design patterns in Java?
Ans:
- Creational patterns deal with mechanisms of object creation, such as Singleton and Factory Method.
- Structural patterns concentrate on the composition of classes and objects, exemplified by Adapter and Composite.
- Behavioral patterns revolve around object communication, as seen in Observer and Strategy patterns.
- Each pattern type addresses specific design concerns, championing best practices in software development.
5. What is the difference between design patterns and algorithms?
Ans:
Aspect | Design Patterns | Algorithms |
---|---|---|
Purpose | Provide general reusable solutions to common design problems in software engineering | Solve specific computational problems by defining a series of steps to be followed |
Scope | Focus on high-level design and architecture of software systems | Concentrate on low-level implementation details and efficiency |
Flexibility | Allow flexibility and adaptability in implementation details and can be applied in various contexts | Typically rigid and specific, tailored to solve a particular problem efficiently |
Examples | Singleton, Factory, Observer, Strategy | Sorting algorithms (e.g., Bubble Sort, Quick Sort), Searching algorithms (e.g., Binary Search) |
6.What Is Gang of Four (GOF) in Design Patterns?
Ans:
The Gang of Four (GOF), consisting of Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides, authored “Design Patterns: Elements of Reusable Object-Oriented Software,” which catalogues 23 classic software design patterns. These patterns provide reusable solutions to common design challenges and have had a significant impact on software engineering and object-oriented design methodologies.
7.What are the SOLID Principles?
Ans:
- SRP advocates for classes having a singular reason to change.
- OCP dictates that classes should be open for extension but closed for modification.
- LSP underscores the interchangeability of objects between superclass and subclass without affecting program correctness.
- ISP suggests that clients should not rely on interfaces they don’t use.
- DIP promotes dependency inversion by separating high-level modules from low-level ones.
8.What do you understand by the Open-Closed Principle (OCP)?
Ans:
The Open-Closed Principle (OCP) advocates for software entities like classes, modules, and functions to be extendable without modification. This promotes code stability by minimizing alterations to existing code. Encouraging the use of abstractions and interfaces facilitates future extensions. Patterns like Strategy and Decorator illustrate the OCP principle, fostering modular, flexible, and maintainable software.
9.What are some of the design patterns used in Java’s JDK library?
Ans:
- Java’s JDK library integrates various design patterns to achieve modularity and extensibility.
- Prominent patterns include Singleton, Factory Method, and Observer.
- Singleton ensures a class has only one instance and offers a global access point.
- Factory Method defines an interface for object creation, delegating instantiation to subclasses.
- Observer establishes a one-to-many dependency between objects, facilitating state change notifications.
- These patterns are pivotal in crafting Java’s core classes and libraries.
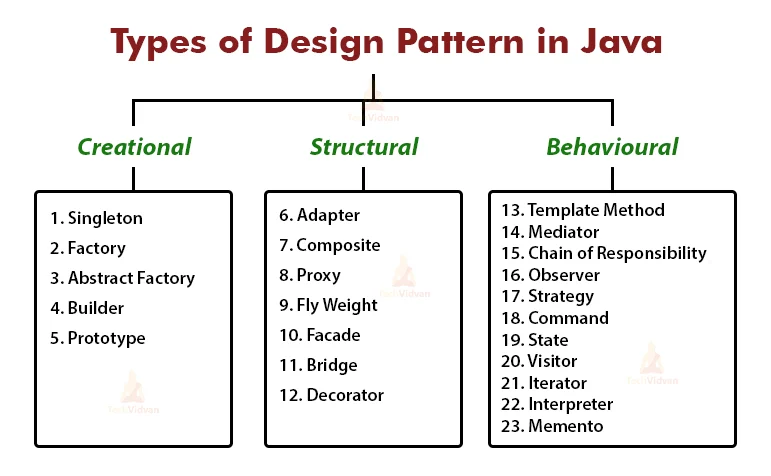
10.How are design principles different from design patterns?
Ans:
Design principles provide foundational guidelines for software system design, offering high-level concepts and philosophies guiding architecture and structure. Examples include SOLID, DRY, and KISS principles. In contrast, design patterns offer specific solutions to common design issues, embodying best practices as reusable templates. Principles guide overarching design decisions, while patterns offer concrete implementations for specific challenges.
11.What is Inversion of Control?
Ans:
Inversion of Control (IoC) is a design principle that shifts control over object creation and flow from the application to a framework or container, such as Spring or Google Guice. This framework manages object lifecycles and dependencies, promoting loose coupling and high cohesion by decoupling system components. IoC facilitates testing by enabling dependencies to be easily mocked or substituted, ultimately aiding in the creation of modular and scalable applications.
12.What are some benefits of using design patterns?
Ans:
- Design patterns promote code reusability, improving maintainability and scalability.
- They enhance communication among developers by providing a common language and solution vocabulary.
- Design patterns also encapsulate best practices, reducing development time and effort.
- Additionally, they facilitate flexibility and adaptability in software design, making systems easier to evolve and extend.
13.What is the Bridge Design Pattern?
Ans:
The Bridge Design Pattern separates abstraction from implementation, allowing them to evolve independently. It involves separate hierarchies for abstraction and implementation, connected through composition instead of inheritance. By enabling changes in either abstraction or implementation without affecting each other, this pattern promotes flexibility and extensibility in software design.
14.What is the factory pattern?
Ans:
The factory pattern is a creational design pattern that provides an interface for creating objects within a superclass. However, it allows subclasses to alter the types of objects created. It centralizes object creation logic, fostering loose coupling between creation code and objects. This pattern proves useful when the exact type of object needed is uncertain until runtime and enhances maintainability by isolating object instantiation from the rest of the codebase.
15.What is the difference between ordinary and abstract factory design patterns?
Ans:
- The ordinary factory creates individual objects, while the abstract factory generates families of related objects without specifying concrete classes.
- In the ordinary factory, each method creates one type of object, while in the abstract factory, each factory produces multiple related objects.
- The ordinary factory emphasizes specific instance creation, while the abstract factory focuses on creating related object families.
16. What are the advantages of the builder design pattern?
Ans:
- The builder design pattern provides precise control over object construction, allowing varied configurations.
- It separates construction from representation, enhancing code readability and maintainability.
- With explicit construction steps, it promotes consistency and reduces complexity.
- Additionally, it facilitates modular, step-by-step construction of complex objects.
17.How is the bridge pattern different from the adapter pattern?
Ans:
The bridge pattern focuses on disentangling abstraction from implementation, permitting them to evolve autonomously. Conversely, the adapter pattern serves as a wrapper facilitating the integration of incompatible interfaces. While the bridge pattern emphasizes design and structure, the adapter pattern focuses on aligning unrelated interfaces.
18.What is a command pattern?
Ans:
The command pattern is a behavioral design pattern that encapsulates a request as an object, enabling the parameterization of clients with queues, requests, and operations. By decoupling the request sender from the execution object, it enables flexible and extensible command handling. This pattern facilitates the implementation of undo functionality, logging, and transactional systems.
19.What are antipatterns?
Ans:
Antipatterns are solutions to recurrent problems that initially appear advantageous but ultimately yield negative outcomes. They exemplify common pitfalls or errors in software design, development, or project management, often leading to inefficiencies, poor performance, or project failure. Antipatterns typically arise from shortcuts, misconceptions, or the misapplication of design principles.
20.What is the Singleton design pattern, and how is it implemented?
Ans:
- The Singleton design pattern ensures a class has a single instance globally accessible.
- It’s implemented by making the class’s constructor private to prevent external instantiation.
- To ensure thread safety in a multithreaded environment, synchronization mechanisms may be used.
- This pattern is commonly utilized for managing resources like database connections or logging functionality.
21.What is the Observer design pattern, and can you provide an example?
Ans:
The Observer pattern establishes a one-to-many relationship among objects. When one object undergoes a change, all its dependents are notified and updated automatically. Example: Imagine a weather station sending weather updates to various displays. Each display, acting as an observer, receives notifications of weather changes and updates its display accordingly.
22.What is the Strategy design pattern, and when would you use it?
Ans:
The Strategy pattern defines a set of algorithms, encapsulates each, and allows them to be interchanged. It enables algorithms to vary independently from the clients using them. Use Case: It proves valuable when selecting an algorithm at runtime or accommodating multiple variations of an algorithm. This pattern promotes flexibility and reuse by isolating algorithm-specific code, making it easier to add new algorithms without modifying existing code. Additionally, it helps maintain a clean separation of concerns, as the algorithm implementation details are abstracted from the client.
23.How does the Decorator design pattern work, and what problem does it solve?
Ans:
- Defines the common interface for objects that can have additional responsibilities added. Implements the Component interface, providing the core functionality.
- Abstract class that implements the Component interface and contains a reference to a Component object. It delegates core functionality to the wrapped Component.
- Extend the Decorator class to add specific responsibilities or behaviors.
- Provides a flexible and reusable way to extend an object’s functionality dynamically.
- Allows for feature enhancement without altering existing code, adhering to the Open/Closed Principle by keeping classes open for extension but closed for modification.
24.What is the Template Method design pattern?
Ans:
- The Template Method pattern outlines the basic structure of an algorithm in the superclass but permits subclasses to override specific steps without altering the core structure.
- Example: Consider a template for beverage preparation where steps like boiling water, brewing, and pouring are outlined in the superclass but customizable for specific beverages.
25.What is the Proxy design pattern, and what are its use cases?
Ans:
- The Proxy design provides a stand-in or proxy so that another object can control access to it.
- It finds utility in scenarios such as lazy initialization, access control, and logging.
- Use Cases: Caching expensive operations, controlling access rights, and implementing virtual proxies are typical applications.
26.What is the Chain of Responsibility design pattern, and how does it work?
Ans:
The Chain of Responsibility pattern permits multiple handlers to process an event, with each capable of either handling the request or passing it along the chain. Operation: Requests traverse the chain until handled or reaching the chain’s end. This allows for flexible and decoupled request handling, as new handlers can be added without modifying existing code.
27.What is the State design pattern, and can you provide an example?
Ans:
- The State pattern enables an object to modify its behavior as its internal state changes.
- It proves beneficial when an object’s behavior needs adjustment based on its state.
- This pattern promotes cleaner and more maintainable code by localizing state-specific behavior in separate classes.
- Additionally, it allows for easy extension of new states and transitions without modifying the existing code structure. Example: A vending machine adapting its behavior according to its state—idle, product selection, dispensing, etc.
28.What is the Flyweight design pattern, and when is it useful?
Ans:
- Flyweight: Defines an interface for operations that can be performed on shared objects and maintains the intrinsic state.
- ConcreteFlyweight: Implements the Flyweight interface and contains the intrinsic state.
- FlyweightFactory: Manages the creation and management of Flyweight objects, ensuring that shared instances are reused.
- Client: Maintains references to Flyweight objects and supplies extrinsic state.
- Memory Optimization: Useful when dealing with a large number of objects that have shared state, as it reduces memory usage by reusing common parts.
- Performance Improvement: Helps improve performance in applications with many similar objects by reducing the overhead of object creation.
- Complexity Management: Simplifies the management of numerous objects by consolidating their shared characteristics, making the system easier to maintain and extend.
29. What is the Composite design pattern, and what is its structure?
Ans:
- Component: An abstract class or interface that defines the common interface for both leaf and composite objects. It declares methods for managing child components and any operations that can be performed on them.
- Leaf: A concrete class that implements the Component interface. It represents individual objects in the composition that do not have children. Leaf nodes implement the behavior specific to the individual objects.
- Composite: A concrete class that implements the Component interface. It maintains a collection of child components and implements methods for adding, removing, and accessing child components.
30. Explain the Iterator design pattern and its benefits.
Ans:
The Iterator design pattern provides a way to access elements of a collection sequentially without exposing its internal structure. It uses an iterator interface to traverse elements and a concrete implementation to manage the iteration process. This pattern encapsulates iteration logic, allows for uniform access to various collections, supports multiple iterators for concurrent traversal, and simplifies adding new collection types.
31. What is the Interpreter design pattern, and where is it commonly used?
Ans:
The Interpreter design pattern defines a grammar for a language and provides an interpreter to evaluate sentences in that language. It involves creating a set of classes that represent the grammar rules and an interpreter that interprets these rules to execute operations. This pattern is commonly used in scenarios where a language needs to be interpreted, such as scripting languages, query languages, or domain-specific languages, allowing for flexible and extensible language processing.
32. Describe the Visitor design pattern and its role in object structure traversal.
Ans:
- Visitor separates algorithms from the object structure they operate on.
- It allows adding new operations to objects without modifying their structure.
- Encapsulates related behaviors into separate visitor classes.
- Objects accept visitor objects and delegate operations to them.
- Facilitates traversing complex object structures and performing operations.
- Promotes clean and extensible designs by adhering to the open-closed principle.
33. What are the benefits of using the Memento design pattern?
Ans:
- Memento captures and externalizes an object’s internal state.
- It allows restoring an object’s state to a previous snapshot.
- Encapsulates the object’s state, ensuring data privacy and consistency.
- Supports undo mechanisms in applications.
- Promotes separation of concerns by keeping state management separate from the object.
- Facilitates easy rollback to previous states without coupling to the object’s implementation.
34. How does the Mediator design pattern facilitate communication between objects?
Ans:
Mediator centralizes communication logic between objects.Objects interact through a mediator instead of directly communicating.Reduces dependencies between communicating objects.Simplifies the maintenance and modification of interactions.Supports loose coupling between components.Promotes scalability and flexibility in complex systems.
35.What is the Prototype design pattern, and how is it implemented?
Ans:
Prototype creates new objects by copying an existing prototype object.It avoids the need for subclasses by cloning existing objects.Typically used when the creation of new objects is expensive.Provides a way to produce new objects with minimal cost.Supports dynamic runtime object creation.Promotes flexibility and scalability in object creation scenarios.
36.What is the Command design pattern, and what are its components?
Ans:
- A command allows parameterization by encapsulating a request as an object.
- Invoker triggers commands without knowing their implementation details.
- Commands decouple sender and receiver of a request.
- Facilitates undo operations by storing command history.
- Promotes extensibility and flexibility in request handling.
37.What is the Builder design pattern, and when would you use it?
Ans:
- Builder distinguishes between the representation of a complex item and its production.
- Used when the construction process must allow different representations.
- Encapsulates the construction logic within separate builder objects.
- Allows constructing objects step by step or using a fluent interface.
- Supports building complex objects without exposing their internal representation.
- Promotes code readability, maintainability, and flexibility in object construction.
38.Explain the Abstract Factory design pattern and its relationship with factory patterns.
Ans:
An interface for building families of linked or dependent objects is offered by Abstract Factory.Differs from Factory Method by creating families of related objects, not just one.Encapsulates the object creation logic within factory classes.Promotes loose coupling between client code and concrete implementations.Supports changing the product families without modifying client code.Facilitates adherence to the Open-Closed Principle by allowing extension through new factories.
39.What is the Object Pool design pattern, and what problems does it address?
Ans:
- Object Pool manages a pool of reusable objects to improve performance and resource utilization, focuses on the overhead associated with creating and destroying objects.
- Reuses objects instead of instantiating new ones.
- Maintains a pool of initialized objects ready for use.
- Controls the number of objects created to prevent resource exhaustion.
- Promotes efficiency and scalability in applications with heavy object creation overhead.
40.What is the Proxy design pattern, and what are its variants?
Ans:
A proxy acts as a stand-in or proxy for another item so that access can be restricted.Acts as an intermediary, adding functionality or restricting access.Variants include virtual proxy, remote proxy, protection proxy, and smart reference.Virtualproxy creates objects on demand, optimizing resource usage.Remote proxy provides a local representation of a remote object.Promotes security, performance optimization, and easier resource management.
41.What is the difference between the Adapter design pattern and the Bridge pattern?
Ans:
- The Adapter pattern reshapes the interface of a class to match client expectations.
- Adapters adjust existing interfaces, while Bridges separate abstraction from implementation.
- Typically, a single adapter class is involved in Adapter pattern, while Bridge employs distinct hierarchies.
- Adapter promotes interoperability, while Bridge enhances flexibility in design.
42.What is the difference between the Strategy and State design patterns?
Ans:
- The Strategy pattern facilitates runtime algorithm selection.
- Conversely, the State pattern enables object behavior alteration based on internal state changes.
- In contrast, State focuses on behavior changes linked to internal states.
- Strategy permits interchangeable algorithms, while State transitions objects between states, influencing behavior.
43.What is the Null Object design pattern, and what is its purpose?
Ans:
The Null Object pattern furnishes default behaviors for classes instead of returning null references.It ensures absence of null references from objects.Instead of null, it yields a neutral “null object” imbued with default behavior.The aim is to streamline code by obviating null checks.It mitigates NullPointerException occurrences.Ultimately, it instills predictability into applications.
44.Describe the Observer pattern and its variations.
Ans:
The Observer pattern establishes a one-to-many relationship among objects, ensuring state changes in one notify all dependents.Variants include employing varied communication channels such as push or pull.Pull model entails observers retrieving updates from the subject when necessary.In contrast, the push model prompts the subject to actively dispatch updates to observers.Bidirectional communication is possible, allowing observers to update the subject.
45.What are the advantages of using the Chain of Responsibility pattern?
Ans:
- The Chain of Responsibility pattern empowers multiple objects to handle requests, shielding senders from knowledge of which object will process the request.
- It disentangles senders from request receivers.
- The architecture accommodates dynamic chain structure adjustments.
- Handlers can be added or removed without client code interference.
- Configuration flexibility enables varied request handling within the chain.
46.How does the Strategy pattern differ from the Template Method pattern?
Ans:
- Strategy pattern delineates algorithm families, encapsulates each, and offers interchangeability.
- Strategy encapsulates algorithms, fostering independent variation.
- Template Method structures algorithms, enabling subclasses to tailor steps.
- Strategy affords runtime algorithm selection, while Template Method allows for step modification.
- Different algorithms interchange seamlessly under Strategy, while Template Method orchestrates algorithmic steps.
47.What is the Command pattern, and how is it used to implement undo functionality?
Ans:
Requests are encapsulated by the Command pattern, which enables client parameterization with queues, actions, and requests.It disassociates command senders from receivers.Undo capability is feasible via stored command execution history.Commands can be reversible, easing undo implementation.Command invocation is orchestrated by an invoker, preserving extensibility.It streamlines the addition of new commands sans existing code alterations.
48.What is the Proxy pattern, and how is it used to implement virtual proxies?
Ans:
The Proxy pattern offers surrogates to control access to objects, furnishing additional functionality upon access.Virtual proxies defer instantiation of resource-intensive objects until required.They mimic real objects, providing an identical interface.Virtual proxies optimize resource usage by postponing object creation.They excel in scenarios necessitating costly or resource-intensive object creation.Enhanced performance and resource utilization ensue from deferred object instantiation.
49.What is the purpose of the Interpreter design pattern?
Ans:
- The Interpreter pattern crafts a language grammar and furnishes an interpreter for sentence interpretation.
- It defines and interprets expressions within a specific language.
- Expression parsing and execution are facilitated.
- Dominant in domain-specific languages (DSLs) or parsing tasks.
- Complex grammars are simplified through object representation of rules.
- Well-suited for applications requiring language processing or rule evaluation.
50.What is the difference between the Visitor pattern and the Observer pattern?
Ans:
- The Visitor pattern introduces new operations to classes sans structural changes.
- Visitor augments existing class functionalities.
- Observer notifies dependent objects about state alterations.
- In Visitor, visitors traverse object structures and apply operations.
- Observer promotes loose coupling, facilitating subject-observer interaction.
51.What is the Template Method pattern, and what are its implementation details?
Ans:
Defines algorithm structure in a method, allowing subclasses to redefine steps.Encapsulates common behavior in superclass, with subclasses providing specifics.Involves abstract methods in superclass and concrete methods for algorithm.Implementation: Abstract base class with template method; subclasses provide details.Promotes code reuse, ensures consistency across subclasses.
52.What problems does the Composite pattern solve in software design?
Ans:
- Addresses treating individual objects and compositions uniformly.
- Enables working with hierarchical structures seamlessly.
- Simplifies client code by treating objects identically.
- Facilitates recursive composition for complex structures.
- Uniform handling of structural changes without client impact.
53.What is the Mediator pattern, and what are its benefits in software architecture?
Ans:
- Promotes loose coupling by centralizing communication logic.
- Encapsulates object interactions, reducing direct dependencies.
- Centralization simplifies system maintenance and modifications.
- Enhances reusability and interchangeability of components.
- Facilitates adding new components without altering existing ones.
- Commonly used in GUI toolkits for implicit component interaction.
54.What is the Facade pattern, and how does it simplify interfaces?
Ans:
Provides unified interface to subsystem, easing usage.Hides subsystem complexities, offering high-level interface.Promotes loose coupling by shielding clients from details.Reduces dependencies, minimizing subsystem changes impact.Enhances readability and maintainability with clear entry point.Applied in complex systems with subsystems like APIs or libraries.
55.What is the difference between the Prototype pattern and the Singleton pattern?
Ans:
The Prototype pattern involves creating new objects by copying an existing object (prototype), allowing for the cloning of objects without needing to know their concrete classes. The Singleton pattern ensures that a class has only one instance throughout the application and provides a global point of access to that instance. In essence, the Prototype pattern focuses on object creation through cloning, while the Singleton pattern focuses on restricting object instantiation to a single instance.
56.What is the difference between the Flyweight and Proxy patterns?
Ans:
- Flyweight optimizes memory by sharing common state among objects.
- Reduces memory footprint by externalizing shared state.
- Proxy provides surrogate for controlled access to objects.
- Adds indirection for features like lazy initialization or access control.
- Flyweight focuses on memory efficiency; Proxy on access control and behavior.
- Flyweight shares state; Proxy manages object access.
57. What distinguishes Abstract Factory patterns from the Factory Method?
Ans:
The Abstract Factory pattern provides an interface for creating families of related or dependent objects without specifying their concrete classes, allowing for the creation of multiple related objects through a unified interface. In contrast, the Factory Method pattern defines an interface for creating a single object but lets subclasses alter the type of objects that will be created, focusing on creating one product at a time.
58.What is the differences between the Command and Strategy patterns.
Ans:
- Command encapsulates requests as objects, separating requester from performer.
- Strategy defines algorithms and makes them interchangeable.
- Command focuses on actions, decoupling invoker and receiver.
- Strategy enables algorithm variability independent of clients.
- Command deals with actions and queuing; Strategy with algorithms interchangeability.
- Command facilitates invoker-receiver decoupling; Strategy enables algorithm interchange.
59.How does the Observer pattern facilitate loose coupling in software systems?
Ans:
Establishes one-to-many dependency, triggering updates in dependents.Subjects notify observers without specifying concrete classes.Decouples subject from observers, promoting flexibility.Enables adding/removing observers without modifying subject.Maintains independence, reducing ripple effects from changes.Used in event handling, GUI components, and distributed systems.
60.What is the difference between the Proxy and Decorator design patterns?
Ans:
- Proxy provides surrogate for controlled object access.
- Adds indirection for features like lazy initialization or logging.
- Decorator dynamically attaches additional responsibilities to an object.
- Allows behavior addition/removal without affecting other objects.
- Proxy manages object access; Decorator enhances object behavior.
- Proxy offers similar interface; Decorator enhances or modifies it.
61.What are the differences between the Iterator and Visitor patterns?
Ans:
Iterator sequentially accesses collection elements, while Visitor allows adding operations without altering the objects themselves. The Iterator pattern traverses elements, maintaining their structure, whereas the Visitor pattern performs operations on elements, potentially altering their behavior. The Iterator doesn’t modify element structures, but the Visitor may extend or modify element behavior. Additionally, the Iterator pattern is useful for iterating through a collection, while the Visitor pattern is ideal for applying diverse operations across different object types.
62.What is the differences between the Command and Chain of Responsibility patterns.
Ans:
- Command encapsulates requests into objects.
- Chain of Responsibility delegates requests through a handler chain.
- Command decouples sender and receiver of a request.
- Chain of Responsibility enables multiple handlers for a request.
- Command typically involves a single receiver.
- Chain of Responsibility may involve multiple potential receivers.
63. How is the Interpreter pattern different from the Strategy pattern?
Ans:
Interpreter defines language grammar and focuses on parsing and interpreting syntax structures to handle language semantics. Strategy defines interchangeable algorithms and focuses on behavior by providing diverse algorithm options for varied algorithmic requirements. The Interpreter pattern is used for parsing and interpreting language constructs, whereas the Strategy pattern enables dynamic selection of algorithms at runtime. Additionally, Interpreter deals with complex language rules, while Strategy offers flexibility in algorithm choice and application.
64.What are the differences between the State and Strategy patterns.
Ans:
- State captures an object’s varying behavior.
- Strategy encapsulates interchangeable algorithms.
- State alters behavior with internal state changes.
- Strategy lets clients select appropriate algorithms.
- State shifts due to internal transitions.
- Strategy adapts to diverse client demands.
65.What are the benefits of using the Facade pattern in software development?
Ans:
- Simplifies complex subsystem interfaces.
- Offers a unified interface to multiple interfaces.
- Conceals complexities from client modules.
- Encourages loose coupling between subsystems and clients.
- Enhances code readability and maintenance.
- Reduces dependencies and code duplication.
66.How does the Bridge pattern differ from the Adapter pattern?
Ans:
- Bridge separates abstraction from implementation.
- Adapter facilitates compatibility between interfaces.
- Bridge decouples abstraction and implementation.
- Adapter converts one interface to another.
- Bridge’s design typically precedes implementation.
- Adapter is employed for adapting existing interfaces.
67.What is the differences between the Flyweight and Prototype patterns.
Ans:
- Flyweight shares objects for memory efficiency.
- Prototype creates new objects by copying existing ones.
- Flyweight minimizes memory usage by sharing objects.
- Prototype generates new instances based on existing ones.
- Flyweight employs a shared object pool.
- Prototype clones objects for new instances.
68.What are the differences between the Mediator and Observer patterns.
Ans:
- Mediator serves as a centralized communication controller.
- Observer establishes a publish-subscribe relationship.
- Mediator orchestrates communication among objects.
- Observer enables objects to subscribe and receive notifications.
- Mediator encapsulates communication protocols.
- Observer emphasizes notification broadcasting.
69.How does the Composite pattern differ from the Decorator pattern?
Ans:
- Composite represents objects in a tree structure.
- Decorator adds responsibilities to individual objects.
- Composite embodies part-whole hierarchies.
- Decorator dynamically augments object functionalities.
- Composite structures objects hierarchically.
- Decorator wraps objects to enhance behavior.
70.What is the purpose of using the Null Object pattern in software design?
Ans:
The Null Object pattern provides neutral behavior for an object, eliminates reliance on null references, and streamlines null checks in client code. It also facilitates the handling of absent or optional objects, enhances code readability and maintainability, and ensures consistent and predictable behavior. By implementing this pattern, developers can reduce the risk of null reference errors, leading to more robust applications.
71. What distinguishes the Singleton pattern from the Factory Method?
Ans:
- Factory Method creates instances of subclasses, allowing flexible object creation. Singleton restricts instantiation to a single instance globally.
- Factory Method emphasizes subclassing for object creation, promoting extensibility. Singleton ensures only one instance exists throughout the application’s lifecycle.
- Factory Method provides a way to defer object creation to subclasses, enhancing flexibility. Singleton ensures global access to a single instance, simplifying resource management.
72.What are the differences between the Abstract Factory and Builder patterns?
Ans:
- Abstract Factory creates families of related objects without specifying their concrete classes.
- Builder constructs complex objects step by step, allowing different representations.
- Abstract Factory focuses on creating families of related objects, promoting consistency among them.
- Builder separates the construction of a complex object from its representation, enabling varied construction processes.
73.How does the Prototype pattern differ from the Abstract Factory pattern?
Ans:
Prototype is perfect for cases involving sophisticated object generation since it creates new objects by cloning an existing instance. An interface called Abstract Factory makes it possible to create families of related objects without having to describe their particular classes. Prototype pattern emphasizes object cloning for efficient object creation, especially for complex objects. Abstract Factory focuses on creating families of related objects, promoting consistency among them.
74.What are the benefits of using the State pattern in software architecture?
Ans:
- State pattern allows objects to alter behavior when internal state changes, enhancing flexibility and maintainability.
- It encapsulates state-specific behavior into separate classes, promoting cleaner code organization.
- State pattern simplifies complex conditional logic related to object state, improving code readability and maintainability.
- It enables better separation of concerns by isolating state-specific behavior into distinct classes.
75.What are the differences between the Observer and Publish-Subscribe patterns?
Ans:
- Observer pattern establishes a one-to-many dependency between objects, where changes in one object trigger updates in its dependents.
- Publish-Subscribe pattern facilitates message distribution to multiple subscribers without direct dependencies between publisher and subscribers.
- Observer pattern typically involves a single observable object and multiple observers, maintaining direct communication.
- Publish-Subscribe pattern decouples publishers from subscribers through intermediary components like message brokers, enabling scalable communication.
76.What are the differences between the Command and Strategy patterns?
Ans:
- Observer pattern establishes a one-to-many dependency between objects, where changes in one object trigger updates in its dependents. Publish-Subscribe pattern facilitates message distribution to multiple subscribers without direct dependencies between publisher and subscribers.
- Observer pattern typically involves a single observable object and multiple observers, maintaining direct communication. Publish-Subscribe pattern decouples publishers from subscribers through intermediary components like message brokers, enabling scalable communication.
77.How does the Template Method pattern differ from the Strategy pattern?
Ans:
Template Method defines the skeleton of an algorithm in a method, allowing subclasses to redefine certain steps. Strategy pattern encapsulates interchangeable algorithms and allows clients to choose among them. Template Method pattern focuses on defining the overall structure of an algorithm with certain steps customizable by subclasses. Strategy pattern emphasizes encapsulating algorithms separately and making them interchangeable at runtime.
78.What are the advantages of using the Adapter pattern in software development?
Ans:
- Adapter pattern allows incompatible interfaces to work together by converting the interface of a class into another interface expected by clients.
- It promotes code reusability by enabling integration of existing classes with different interfaces.
- Adapter pattern facilitates seamless integration of legacy systems with modern applications, enhancing interoperability and extending the lifespan of existing codebases.
- It simplifies client code by providing a consistent interface to interact with diverse objects.
79.What are the differences between the Proxy and Adapter patterns?
Ans:
- A proxy pattern acts as a stand-in or proxy for another object so that access to it can be controlled, usually for access control or lazy initialization.
- An adapter pattern changes a class’s interface to one that clients are expecting.
- Proxy pattern acts as a surrogate or placeholder for another object, controlling access to it, while Adapter pattern enables communication between incompatible interfaces by converting the interface of a class into another interface.
80.What are the differences between the Composite and Bridge patterns?
Ans:
- Composite pattern composes objects into tree structures to represent part-whole hierarchies, allowing clients to treat individual objects and compositions uniformly. Bridge patterns allow an abstraction to be changed independently of its implementation.
- Composite pattern focuses on representing part-whole hierarchies as tree structures, allowing clients to manipulate individual objects and compositions uniformly. Bridge pattern separates an abstraction from its implementation, facilitating independent variations of both.
81.What is the difference between the Factory Method pattern and the Abstract Factory pattern?
Ans:
- Factory Method centers on creating a singular object, often through inheritance.
- Abstract Factory generates families of related or dependent objects without specifying their concrete classes.
- Factory Method revolves around singular object creation while Abstract Factory handles multiple related objects.
82.What are the benefits of using the Observer pattern in software systems?
Ans:
- Enables loosely coupled systems where objects communicate without awareness of each other’s identities.
- Supports one-to-many dependency relationships between objects.
- Facilitates efficient communication and updates between objects.
- Enhances modularity and flexibility by segregating concerns.
- Aids in the development of systems with distributed components.
83.What are the differences between the Decorator and Proxy patterns?
Ans:
- Decorator dynamically adds behavior to objects without altering their interface.
- Proxy offers a placeholder for another object to control access to it.
- Decorator enhances functionality by wrapping objects, while Proxy governs access to objects.
84.What are the differences between the Chain of Responsibility and Command patterns?
Ans:
- Chain of Responsibility establishes a chain of handlers to sequentially process requests until one handles it.
- Command encapsulates a request as an object, permitting client parameterization with requests.
- Chain of Responsibility delegates requests dynamically, whereas Command encapsulates requests for deferred execution.
85.How does the Interpreter pattern differ from the Visitor pattern?
Ans:
- Chain of Responsibility establishes a chain of handlers to sequentially process requests until one handles it.
- Command encapsulates a request as an object, permitting client parameterization with requests.
- Chain of Responsibility delegates requests dynamically, whereas Command encapsulates requests for deferred execution.
86.What are the advantages of using the Builder pattern in software design?
Ans:
The Builder pattern provides a flexible way to construct complex objects step by step, allowing for the creation of different representations using the same construction process. It enhances code readability and maintainability by separating the construction and representation of an object, making it easier to manage variations in object creation. Additionally, it promotes immutability by allowing the final product to be constructed only after all required parameters are set, reducing the likelihood of errors.
87.Explain the differences between the Template Method and Strategy patterns.
Ans:
- Template Method defines the skeleton of an algorithm in a superclass, enabling subclasses to redefine specific steps.
- Strategy pattern encapsulates interchangeable behaviors, permitting runtime selection.
- Template Method concentrates on defining the overall algorithm structure, whereas Strategy encapsulates algorithms.
88. How do the Bridge and Composite patterns differ from one another?
Ans:
Bridge design allows for independent variation by separating an abstraction from its implementation, enabling them to evolve independently. To depict part-whole hierarchies, Composite patterns combine elements into tree structures, facilitating uniform treatment of individual objects and compositions. While Composite focuses on managing hierarchical structures and their components, Bridge emphasizes the decoupling of abstraction from implementation, promoting flexibility and extensibility in the design.
89.How does the Facade pattern differ from the Adapter pattern?
Ans:
The Facade pattern simplifies a complex system’s interface.It encapsulates subsystems, offering a higher-level interface.Conversely, the Adapter pattern transforms one class interface into another expected by clients.It enables compatibility between incompatible interfaces.Facade streamlines interactions, while Adapter deals with interface adjustments.Essentially, Facade simplifies, while Adapter adjusts.
90.What are the benefits of using the Mediator pattern in software architecture?
Ans:
- The Mediator pattern fosters loose coupling among components.
- It centralizes communication logic, diminishing dependencies.
- By utilizing a mediator object, it enhances maintainability through organized interactions.
- Modifications and extensions to communication behavior become easier.
- Encourages component reusability by detaching them from direct communication.
- Overall, the Mediator pattern bolsters flexibility and scalability.
91.What are the differences between the Observer and Publish-Subscribe patterns?
Ans:
- The Observer pattern orchestrates a one-to-many relationship between objects.
- It notifies dependent objects of state changes in a subject.
- In contrast, the Publish-Subscribe pattern is more versatile, accommodating multiple publishers and subscribers.
- Publishers and subscribers operate independently via a message broker.
- Observer manifests tighter coupling, whereas Publish-Subscribe fosters looser coupling.
- Publish-Subscribe accommodates broader communication scenarios.
92.What are the differences between the State and Strategy patterns?
Ans:
- The State pattern enables an object to modify its behavior based on internal state changes.
- It encapsulates state-specific behavior within distinct state objects.
- Conversely, the Strategy pattern encapsulates interchangeable algorithms within dedicated classes.
- It facilitates dynamic algorithm selection at runtime.
- While the State pattern manages object states and transitions, the Strategy pattern focuses on algorithm encapsulation and dynamic selection.
93.What is the difference between the Prototype pattern and the Abstract Factory pattern?
Ans:
- The Prototype pattern concentrates on generating objects from a prototype instance.
- In contrast, the Abstract Factory pattern furnishes an interface for producing families of related objects.
- It encapsulates object creation logic sans specifying concrete classes.
- While Prototype emphasizes cloning, Abstract Factory emphasizes crafting related objects.
94.What are the advantages of using the Flyweight pattern in software development?
Ans:
- The Flyweight pattern economizes memory by sharing common object parts.
- It particularly reduces memory overhead for numerous similar objects.
- Performance gains arise from fewer instances being created.
- It facilitates efficient management of numerous fine-grained objects.
- By segregating intrinsic and extrinsic states, shared objects remain immutable.