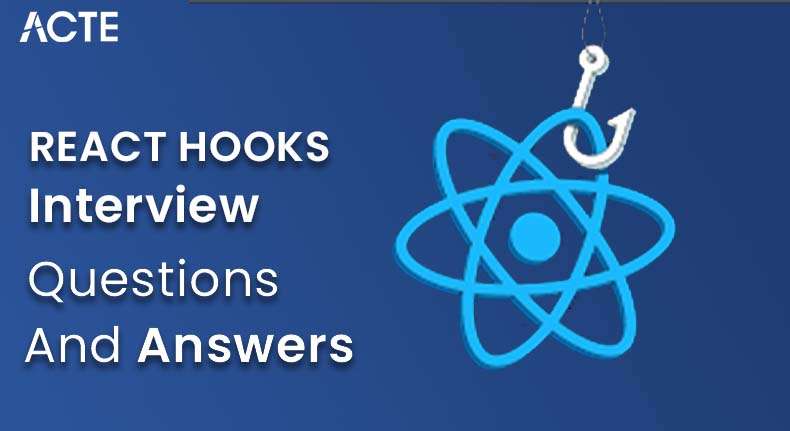
React Hooks are programs that let programmers use state and other React features within functional components. They streamline component logic by providing state management, side effects, and context access, eliminating the need for class components. Key hooks include useState for state management, useEffect for handling side effects, and useContext for context access. By using hooks, developers can write cleaner, more reusable code and enhance the flexibility of functional components.
1. What is React Hooks
Ans:
React Hooks are functions that enable state and React feature usage sans class components. They facilitate stateful logic reuse across components, maintaining hierarchy independence. Hooks empower streamlined state and side effect management within functional components. Hooks simplifies state and lifecycle event handling by offering a concise and readable syntax.
2. What advantages do React hooks offer?
Ans:
- Simplifying stateful logic management within functional components is a crucial advantage.
- They promote logic reuse across components, fostering modular and concise code.
- By eliminating class components, Hooks enhances code cleanliness and brevity.
- Developers can easily share logic between components, bypassing higher-order components or render props.
3. What are the React Hooks rules?
Ans:
- Hooks must be called exclusively at the top level of functional components or custom hooks.
- They’re confined to usage within React function components or custom hooks, not standard JavaScript functions.
- Hook calls within components must retain a consistent order to ensure predictable behavior across renders.
- Adhering to the “use” prefix for custom hooks is essential for clarity and convention.
4. Which React features are the most crucial ones?
Ans:
React’s virtual DOM is pivotal, facilitating efficient rendering by minimizing DOM updates. Its component-based architecture fosters modular and reusable code, enhancing scalability and maintainability. State management mechanisms like useState and useContext hooks are fundamental for interactive UIs.React’s declarative nature simplifies UI development by abstracting low-level DOM manipulation.
5. What benefits does React offer?
Ans:
- React offers a component-based architecture ideal for constructing user interfaces.
- Promoting code reusability, modularity, and maintainability enhances developer productivity.
- Its virtual DOM ensures efficient rendering and optimal performance by minimizing DOM manipulations.
- Declarative syntax facilitates easy comprehension and reasoning about UI components.
6. What are React’s limitations?
Ans:
- React’s learning curve may be steep, especially for developers transitioning from traditional HTML and JavaScript.
- Managing complex state interactions in large applications can pose challenges necessitating careful planning.
- React’s server-side rendering (SSR) may require complex setup and configuration to achieve optimal performance.
- Dependency on third-party libraries for features like routing and global state management can introduce complexity.
7. The Virtual DOM: What Is It?
Ans:
The Virtual DOM serves as a conceptual replica of the real DOM. Acting as a lightweight representation, it resides in memory. React utilizes it to execute updates efficiently. Initially, modifications are applied to the Virtual DOM. React then devises the most optimal strategy to update the actual DOM. This mechanism aids in mitigating performance bottlenecks commonly encountered in web applications.
8. What distinguishes functional components from class components?
Ans:
Aspect | Functional Components | Class Components |
---|---|---|
Definition | Defined as JavaScript functions | Defined using ES6 classes |
Boilerplate | Minimal boilerplate code | Requires additional boilerplate for class declaration and methods |
State | Stateless by default | Can manage their own state |
Lifecycle Methods | No access to lifecycle methods | Can utilize lifecycle methods |
9. What processes go through a react component?
Ans:
- Commencing with initialization involves configuring the initial state and props.
- Subsequently, mounting incorporates the addition of the component to the DOM.
- The updating phase addresses alterations in props or state.
- Unmounting ensues, resulting in the removal of the component from the DOM.
- Lifecycle methods such as componentDidMount or componentDidUpdate may be invoked throughout these stages.
10. What makes using React recommended?
Ans:
- React presents a robust component-based architecture that is conducive to UI construction.
- Its design fosters code reusability and facilitates maintenance.
- Leveraging React’s Virtual DOM ensures streamlined DOM updates, bolstering performance.
- An extensive ecosystem and robust community support underpins React’s versatility.
- React’s declarative syntax streamlines UI development processes.
11. What sets states apart from props?
Ans:
States are internal to a component and are managed within it, while props are passed from parent to child components. States can be changed by the element itself through setState(), while props are immutable. States are used for dynamic data that can change over time within a component, whereas props are used for passing data from parent to child components.
12. What functions do React Fragments fulfill?
Ans:
React Fragments allow components to group multiple children elements without adding an extra DOM node. Allowing components to return various elements helps improve component structure and readability. Fragments are instrumental when rendering adjacent JSX elements without a parent container. They also help avoid unnecessary markup in the DOM.
13. What is JSX?
Ans:
JSX stands for JavaScript XML and is a syntax extension for JavaScript. It’s primarily used with React to describe the structure of UI components. JSX allows developers to write HTML-like syntax within JavaScript code, simplifying the creation and reading of React components. This syntax is then compiled into JavaScript objects known as “React elements.” These elements represent the structure and content of the user interface.
14. What do React props mean?
Ans:
- React props (short for properties) pass data from parent to child components in React.
- They are read-only and help to make components reusable and modular.
- Props are passed as attributes to components and can be accessed within the component using this. Props.
15. Describe the many kinds of side effects in the React component.
Ans:
In React components, side effects encompass tasks such as data fetching, managing subscriptions, or directly manipulating the DOM. These operations are essential for interacting with external systems or performing asynchronous actions. Side effects are usually handled within lifecycle methods in class components or using the useEffect() hook in functional components. Properly managing these effects ensures that components operate efficiently and update as needed based on changes in state or props.
16. What is prop drilling in React?
Ans:
Prop drilling involves passing props through multiple layers of nested components, often resulting in unnecessary props being handled by intermediate components that don’t use them. This may make the code more difficult to maintain and less readable. To address this issue, React context or custom hooks can be used to manage shared states more efficiently. These solutions help avoid passing props through every level of the component tree, making the code cleaner and more maintainable.
17. What do you mean by error boundaries?
Ans:
- Error boundaries are React components that catch JavaScript errors anywhere in their child component tree.
- They help prevent the entire application from crashing due to unhandled errors.
- Error boundaries are implemented using lifecycle or static methods and can display fallback UIs when an error occurs.
18. What are custom hooks in React?
Ans:
- Custom hooks are JavaScript functions that enable the extraction of reusable logic from components.
- They allow developers to encapsulate complex logic and share it between different components.
- Custom hooks follow a naming convention starting with “use” and can call other hooks if needed.
19. How can a custom Hook be made?
Ans:
To create a custom hook in React, define a JavaScript function with a name that begins with “use” to adhere to React’s hook naming convention. Inside this function, include the logic you want to reuse, and you can also use existing hooks or other custom hooks. This encapsulated logic can then be shared across multiple components. Once defined, custom hooks can be imported and used in functional components similarly to built-in hooks, promoting code reusability and clean component architecture.
20. What advantages come with employing personalized hooks?
Ans:
Custom hooks enhance code reusability and modularity by encapsulating complex logic away from components. This abstraction allows components to remain clean and focused solely on presentation logic, significantly improving code readability and maintainability. Additionally, custom hooks facilitate logic sharing across different components, minimizing code duplication throughout the codebase. This leads to a more organized and efficient development process.
21. Can custom Hooks accept parameters?
Ans:
- Yes, custom Hooks in React can accept parameters just like regular functions.
- Parameters enable custom Hooks to be flexible and reusable in various scenarios.
- Custom Hooks can customize their behavior by passing parameters based on specific requirements.
- This allows for greater abstraction and encapsulation of logic within the custom Hook.
- Parameters make custom Hooks more versatile and adaptable to different use cases.
22. How does React manage state without hooks?
Ans:
- React manages the state without hooks primarily through class components.
- Class components utilize the setState method to update and manage the state.
- The state is declared within the class component’s state property.
- Updates to state trigger component re-renders, ensuring UI consistency.
- Although functional components with hooks offer an alternative, class components remain prevalent.
23. What is “lifting state up” in React?
Ans:
“Lifting state up” is a pattern in React where a common ancestor component manages a shared state. This involves moving the state management from child components to their nearest common ancestor. By lifting the state, data can be passed down to child components as props. This promotes better data flow and reduces complexity in individual components.
24. How does React optimize rendering?
Ans:
React optimizes rendering through its virtual DOM reconciliation algorithm. Changes to the virtual DOM are batched and optimized for efficient updates. React employs a diffing algorithm to identify only the necessary DOM updates. This minimizes costly re-renders and enhances application performance. Additionally, React provides mechanisms like PureComponent and memoization for further optimization.
25. What are the alternatives to Redux for state management in React?
Ans:
- Alternatives to Redux for state management in React include Context API and third-party libraries like MobX.
- Context API enables sharing state across components without prop drilling.
- MobX offers a more flexible approach to state management with observable data structures.
- Other options include Recoil, Zustand, and RxJS for managing complex application states.
- Each alternative has its strengths and is suitable for different use cases.
26. What is the purpose of the `useEffect` hook?
Ans:
- The useEffect hook in React serves to perform side effects in functional components.
- Side effects may include data fetching, subscriptions, or DOM manipulations.
- useEffect runs after every render and replaces lifecycle methods like componentDidMount, componentDidUpdate, and componentWillUnmount.
- It accepts a function (effect) and an optional dependency array to control when the effect runs.
27. What is conditional rendering in React?
Ans:
Conditional rendering in React allows components to display different content based on specified conditions. This is typically done using conditional statements such as if-else or ternary operators directly within JSX. By leveraging this feature, developers can create dynamic UI elements that respond to user interactions or data changes. This capability enhances the user experience by enabling more interactive and responsive interfaces. Overall, conditional rendering is essential for building versatile and adaptable React applications.
28. How are forms and submissions handled in React?
Ans:
React provides controlled components to handle forms, whereas the React state controls form data. The React state also controls input elements, enabling dynamic updates and validation. JavaScript event handlers typically handle form submissions like onSubmit, but React allows submissions to be dealt with using native HTML form elements and event handling.
29. What are the benefits of using React Router?
Ans:
- React Router enables declarative routing in React applications, defining routes as components.
- It allows for nested routing, facilitating complex navigation structures within the application.
- With React Router, the browser’s URL changes based on the application’s state, providing a more intuitive user experience.
- It supports dynamic route matching, enabling parameterized routing for dynamic content rendering.
30. What are the best practices for structuring React applications?
Ans:
- Structuring React applications involves organizing components, assets, and other files in a logical hierarchy.
- One common approach is to organize components based on their functionality or features.
- Separating concerns by following principles like single responsibility and modular design enhances maintainability.
- Using folders to group related files, such as components, styles, and tests, improves code organization.
31. When to use React context?
Ans:
React context is suitable for managing global states or providing data that many application components need. It’s beneficial when prop drilling becomes cumbersome, passing props through multiple layers of components. Context can streamline data sharing between deeply nested components without explicitly passing props at each level. It’s useful for theming, authentication, user preferences, and other scenarios requiring global state management.
32. How is server-side rendering (SSR) handled in React?
Ans:
React supports Server-Side Rendering (SSR), which allows elements to be delivered to the client after being rendered on the server. This approach enhances SEO and improves initial load performance by delivering fully rendered HTML pages. Libraries such as Next.js simplify implementing SSR in React applications. However, SSR requires careful management of data fetching and component hydration to ensure smooth client-side interactions. Overall, SSR leads to faster initial page loads and better indexing by search engines.
33. Why are React keys important?
Ans:
- React uses keys to identify elements uniquely during reconciliation.
- Keys help React efficiently update and reorder components.
- They optimize rendering by reducing unnecessary re-renders.
- Keys are crucial for performance and maintaining component state.
- They ensure proper tracking and identification of dynamic components.
34. What are the techniques for performance optimization in React?
Ans:
- Memoization and pure components help avoid unnecessary renders.
- Code splitting and lazy loading reduce initial bundle size and improve load times.
- Using shouldComponentUpdate or React.memo for functional components.
- They were adequately managing the state and avoiding unnecessary re-renders.
35. How are React portals used?
Ans:
React portals allow children to be rendered into a different DOM subtree. They are helpful for modals, tooltips, or UI elements needing to escape the parent component’s DOM hierarchy. Portals maintain React’s event system and ensure proper event bubbling. They provide a clean solution for rendering components outside the usual DOM flow. Portals facilitate building more modular and flexible React applications.
36. How is internationalization (i18n) managed in React?
Ans:
- React can integrate with libraries like react-i18next or react-intl for i18n support.
- These libraries offer features like message translation, locale management, and formatting.
- i18n involves managing text content, date, time, and number formats for different locales.
- React components can dynamically adjust content based on the user’s preferred language.
- Proper i18n implementation enhances the user experience for global audiences.
37. What are the differences between React Memo and useMemo?
Ans:
React.memois a higher-order component that prevents a component from re-rendering unless its props change, optimizing performance. In contrast, useMemo is a hook inside an element to memoize a value or computation result between renders, avoiding costly recalculations. React.memo is used to optimize entire components, while useMemo focuses on specific values or computations within an element. Both tools help enhance performance but serve different purposes.
38. How is the `memo` function used to memoize components?
Ans:
- Use React.memo to create a memoized version of a functional component.
- The component will re-render only if its props change, using a shallow comparison by default.
- You can give `React.memo} a custom comparison function to refine when re-renders should happen.
- This helps enhance performance by preventing unnecessary re-renders of components with unchanged props.
39. What are higher-order components (HOCs) in React?
Ans:
HOCs are functions that take a component and return a new enhanced component. They enable code reuse, logic abstraction, and cross-cutting concerns.HOCs can add state, props, or behavior to components. They facilitate cleaner and more modular component composition. Famous examples include withRouter, connect from Redux, and withStyles from Material-UI.
40. How is state synchronization managed in React concurrent mode?
Ans:
Concurrent mode ensures that React keeps the UI responsive during rendering. It uses a scheduler to prioritize updates and manage component rendering. React employs techniques like time-slicing and Suspense to optimize rendering. State synchronization ensures consistency across asynchronous updates. React’s concurrent mode enhances user experience by preventing UI freezes.
41. What are the limitations of React’s server-side rendering?
Ans:
- SSR can increase server load and response times, impacting scalability.
- The handling of client-side interactions and state management may need to be clarified.
- SSR requires server-side infrastructure and configuration, adding complexity to deployments.
- It may face challenges with third-party libraries and browser-specific code.
- SSR might only be suitable for some applications, especially those heavily relying on client-side interactivity.
42. What is the concept of lazy loading in React?
Ans:
Lazy loading in React is a technique where components are loaded only when needed. It improves initial load time and reduces the amount of resources used upfront. Components are dynamically imported using features like React. Lazy and Suspense. This helps in optimizing performance by loading components asynchronously. Lazy loading is beneficial for large applications with many components.
43. What are the benefits of using TypeScript with React?
Ans:
- TypeScript adds static typing to JavaScript, providing better code quality and readability.
- It helps catch errors during development, reduce bugs, and improve maintainability.
- TypeScript offers enhanced IDE support with features like IntelliSense and type checking.
- It facilitates easier refactoring and collaboration within a team.
- TypeScript’s type definitions provide clear documentation for code interfaces.
44. How are security vulnerabilities handled in React?
Ans:
React recommends following security best practices like input validation and proper authentication. Developers should sanitize user inputs to prevent XSS (Cross-Site Scripting) attacks. Using HTTPS and secure cookies ensures data privacy during transmission. Regularly updating React and its dependencies helps patch security vulnerabilities. Implementing a Content Security Policy (CSP) can mitigate risks associated with third-party scripts.
45. What are the differences between controlled and uncontrolled components?
Ans:
- Controlled components have their state managed by React via props, making them controlled by React.
- Uncontrolled components maintain their state internally, with React having no control over their state.
- Controlled components provide more control and synchronization between UI and data.
- Uncontrolled components are helpful for simpler forms or when integrating with non-react libraries.
- Controlled components are typically preferred for better predictability and testability.
46. What are the component lifecycle methods in functional components?
Ans:
- Functional components can use lifecycle methods with the useEffect hook in React.
- useEffect allows side effects like data fetching or subscription handling to be performed.
- It replaces componentDidMount and componentDidUpdate, and the component will unmount in class components.
- The useEffect hook accepts a callback function and an optional array of dependencies.
47. How is error handling managed in React?
Ans:
Error boundaries in React are used to catch JavaScript errors that occur during rendering, in lifecycle methods, or in constructors of components. By catching these errors, error boundaries prevent the entire UI from crashing, allowing developers to display a fallback UI instead. In class components, error boundaries are implemented using the componentDidCatch lifecycle method. For functional components, error boundaries can be managed using the ErrorBoundary component.
48. How do React hooks dependencies impact performance?
Ans:
- React hooks dependencies influence when a component re-renders and can impact performance.
- Adding unnecessary dependencies may lead to excessive re-renders, affecting performance negatively.
- Carefully managing dependencies ensures that components re-render only when necessary.
- Memoizing expensive computations or using callback can optimize performance by preventing unnecessary re-evaluations.
49. What is event delegation in React?
Ans:
This allows handling events for multiple children with a single event listener. Event delegation is efficient, especially in cases with dynamic or large numbers of elements. Minimizing the number of event listeners reduces memory consumption and improves performance. React’s synthetic event system facilitates event delegation by providing normalized events.
50. What are the differences between shallow rendering and full rendering in testing?
Ans:
- Shallow rendering in testing renders only the component under test without rendering its children.
- On the other hand, complete rendering renders the entire component tree, including all children’s components.
- Shallow rendering is faster and focuses on testing the component’s behavior.
- Full rendering provides more comprehensive testing by including interactions with child components.
51. What is code splitting in React?
Ans:
Code splitting in React involves breaking down the application’s codebase into smaller chunks. These chunks are loaded dynamically, improving initial loading times and reducing bandwidth usage. React. Lazy and Suspense are used to implement code splitting in React applications. It enhances performance by loading only the necessary code for a particular route or feature.
52. What are the differences between React Native and React for web development?
Ans:
- React Native: Framework for building mobile applications.
- React: Library for building user interfaces primarily for the web.
- React Native uses native components, and React uses web components.
- The codebase can be shared between the web and mobile in React.
- React Native requires compiling native code for each platform.
- React is more suited for web-specific functionalities.
53. How is data fetching from APIs handled in React?
Ans:
- Use fetch or Axios to fetch data from APIs.
- It is typically done using the Effect hook.
- Data fetching can be asynchronous.
- Data is often stored in a state.
- Error handling is crucial for robustness.
- Use the useEffect cleanup function to prevent memory leaks.
54. What are the benefits of using Redux with React?
Ans:
Centralized state management is crucial for complex applications, ensuring predictable state changes through actions and reducers. Redux enhances debugging and allows time-traveling with Redux DevTools. It facilitates a clear separation of concerns between UI and state logic. Sharing state across components becomes more straightforward, and the approach significantly improves the scalability and maintainability of large applications.
55. How does React Suspense work with lazy loading components?
Ans:
- Suspense allows components to wait for data fetching.
- Lazy loading enables loading components on demand.
- Reduces initial bundle size and speeds up loading.
- Enhances user experience by showing content progressively.
- Simplifies code splitting and improves performance.
- It helps in optimizing application load time.
56. How is memory management addressed in React?
Ans:
React manages memory automatically through garbage collection. Proper resource cleanup in useEffect hooks prevents memory leaks. Avoiding unnecessary state or prop updates reduces memory usage. Efficient component unmounting and rendering optimize memory usage. Using useMemo or useCallback for memoization can improve memory efficiency. Monitoring memory usage with browser developer tools is essential for optimization.
57. How does React context compare with Redux for state management?
Ans:
- React context: Built-in feature for passing data through the component tree.
- Redux: External library for managing global state in React applications.
- Context is more straightforward and suitable for smaller applications.
- Redux provides more advanced features like middleware and time-travel debugging.
- Context can lead to performance issues with deeply nested consumers.
58. What are forward refs in React?
Ans:
Forward refs allow passing refs through components to child elements. This is useful for accessing DOM elements or React components from parent components. It also improves the flexibility and reusability of components. Forward refs are used in cases where a parent component needs to interact with a child’s DOM. This enables imperative operations like focusing on an input element.
59. How are accessibility concerns addressed in React?
Ans:
- Ensure proper semantic HTML elements are used.
- Use aria-* attributes to enhance accessibility.
- Implement keyboard navigation and focus management.
- Provide descriptive alt text for images.
- Test accessibility using tools like aXe or Lighthouse.
- Consider users with disabilities throughout the design and development process.
60. What are the differences between React’s development and production builds?
Ans:
- Development build: Includes debugging information and hot reloading for rapid development.
- Production build: Optimized for performance and reduced file size.
- The development build is slower but aids in debugging with readable code.
- Production build undergoes minification, bundling, and code splitting.
- Production build eliminates development-specific warnings and logging.
61. How are browser compatibility issues managed in React?
Ans:
React employs polyfills and transpilation to ensure compatibility. It also provides warnings and errors for unsupported features. Testing across various browsers is crucial for identifying issues. Community libraries and resources offer solutions for compatibility. Regular updates and patches address emerging compatibility concerns. Employing feature detection techniques can aid in graceful degradation.
62. What is React’s approach to single-page application routing?
Ans:
React utilizes libraries like React Router for routing. It enables dynamic rendering of components based on URL changes. Routes are defined with specific components to render. Nested routes and route parameters are supported for complex navigation. Browser history manipulation ensures seamless navigation transitions. Server-side rendering with routing ensures SEO optimization.
63. What are the benefits and functionality of React hooks?
Ans:
- They simplify component logic and enable the reuse of stateful logic.
- It looks like useState and useEffect replace lifecycle methods.
- Custom hooks encapsulate reusable logic for sharing among components.
- Hooks promote a cleaner and more modular code structure.
- They facilitate better separation of concerns in React components.
64. What is the importance of the “key” prop in React lists?
Ans:
- The “key” prop helps React identify list items uniquely.
- It aids React inefficient list item updates and reordering.
- Without keys, React may re-render entire lists for minor changes.
- Keys enable React to track changes and optimize rendering performance.
- They prevent unnecessary DOM manipulations and improve user experience.
- Keys are essential for React’s reconciliation algorithm to work effectively.
65. How is CSS styling managed in React components?
Ans:
React supports various styling approaches, such as inline styles, CSS modules, and CSS-in-JS libraries. Inline styles can be applied directly to JSX elements using JavaScript objects. CSS modules provide scoped styles for individual components to avoid global namespace conflicts.CSS-in-JS libraries, like styled components, offer a more dynamic and expressive way to style components.
66. What is the significance of React component keys?
Ans:
- React component keys help in efficient virtual DOM reconciliation.
- They provide a unique identifier for each component in a list.
- Keys ensure proper component recycling and reordering without re-rendering.
- Incorrectly used or missing keys can lead to unexpected behavior and performance issues.
- Properly assigned keys optimize React’s rendering process and improve UI responsiveness.
67. What are the advantages of React’s PureComponent?
Ans:
- React’s PureComponent implements shouldComponentUpdate() with a shallow prop and state comparison.
- This ensures that components only re-render when their data has changed.
- PureComponent helps optimize performance by preventing unnecessary re-renders.
- It’s beneficial when dealing with complex UI components.
68. What is the concept of “props drilling” in React?
Ans:
Props drilling refers to passing props down multiple levels of nested components. It occurs when intermediate components do not need the props but must pass them down to child components. This can lead to prop pollution and make the code harder to maintain. While context API or state management libraries can alleviate props drilling, they add complexity.
69. What is the role of the virtual DOM in React’s performance?
Ans:
- React’s virtual DOM acts as an intermediary representation of the actual DOM.
- Before any updates, React compares the virtual DOM with the real DOM to identify changes.
- This process, known as reconciliation, allows React to minimize actual DOM manipulations.
- By batching updates and efficiently updating only changed parts, React improves performance.
- The virtual DOM ensures that the entire DOM isn’t re-rendered on every state or prop change.
70. How are state updates and re-renders handled in React?
Ans:
React components automatically re-render when their state or props change. State updates can be managed using the setState() method or the useState() hook. React employs reconciliation to determine what parts of the DOM need to be updated. It then efficiently updates only the changed elements, minimizing unnecessary re-renders. Techniques like memoization and PureComponent help optimize performance by preventing unnecessary re-renders.
71. What is the difference between React’s synthetic events and native events?
Ans:
- React’s synthetic events are cross-browser wrappers around native DOM events.
- They provide a consistent interface across different browsers.
- Synthetic events are normalized to ensure consistent behavior regardless of the browser.
- This abstraction simplifies event handling and reduces compatibility issues.
- However, native events offer direct access to the browser’s event system, which can sometimes be necessary.
72. How does React compare to other frameworks like Angular or Vue.js?
Ans:
React is more lightweight and focused on the view layer, offering greater flexibility in integrating with other libraries and frameworks. Unlike Angular, it doesn’t impose a strict architecture, allowing developers to structure their applications as they see fit. Compared to Vue.js, React’s ecosystem and community support are more extensive, providing more resources and tools for developers.
73. What are controlled and uncontrolled components in React forms?
Ans:
Controlled components are tied to the React state, where form inputs reflect the state and trigger state updates through event handlers. Uncontrolled components rely on the DOM to manage state, with form inputs directly accessing and modifying DOM elements. Controlled components offer more control and easier state management, while uncontrolled components can be simpler to implement for less complex forms.
74. How does React handle server-side rendering for SEO?
Ans:
- React SSR involves rendering React components on the server and sending the fully-rendered HTML to the client, improving search engine indexing and initial page load times.
- SSR ensures that search engine crawlers can access and index content accurately, contributing to better rankings and visibility.
- It enables web applications to provide meaningful content to users before client-side JavaScript execution, enhancing SEO performance.
75. What is the purpose and introduction of React hooks?
Ans:
- React hooks were introduced to simplify state management and side effects in functional components, replacing class components’ lifecycle methods.
- They allow functional components to use state and other React features without converting them into class components, improving code readability and reusability.
- Hooks provides a more elegant solution for sharing stateful logic across components, promoting cleaner and more modular code.
76. How do dynamic imports and code splitting work in React?
Ans:
React supports dynamic imports, allowing components or modules to be loaded asynchronously at runtime, enhancing performance by reducing initial bundle size. Code splitting breaks down the application into smaller chunks, loading only the necessary code for each route or component, improving loading times and resource utilization. It enables React applications to be more scalable and responsive, especially for larger projects with complex dependencies.
77. What are the benefits of using React Memo for optimization?
Ans:
Memo is a higher-order component that memoizes functional components, preventing unnecessary re-renders when props remain unchanged. It improves application performance by reducing the number of renders and unnecessary updates, especially for components with expensive rendering logic. Memoization enhances the efficiency of React components, particularly in scenarios where performance optimization is critical, such as rendering large lists or complex UIs.
78. What is the data flow in React’s unidirectional manner?
Ans:
- React ensures data flows one way, from parent to child components.
- Parent components transmit data down to their children via props.
- Child components communicate with parents via callback functions.
- This unidirectional flow guarantees predictable data handling and simplifies debugging.
79. Why are “keys” important in React’s reconciliation?
Ans:
- “Keys” serve as unique identifiers for React’s virtual DOM elements.
- They facilitate React in identifying changes, additions, or removals within lists.
- Proper utilization of keys enables React to optimize rendering efficiency.
- It ensures accurate management of component lifecycles.
- Practical key usage is pivotal for enhancing rendering performance in React apps.
80. How are compatibility issues handled in React?
Ans:
React provides polyfills and compatibility libraries to support older browsers. It promotes the adoption of modern JavaScript features with tools like Babel. The React community actively maintains compatibility across significant browser versions. Regular updates and patches address compatibility issues promptly. React’s virtual DOM abstraction aids in mitigating browser-specific inconsistencies.
81. What are the limitations of React for large-scale applications?
Ans:
What challenges does React present in large-scale project development? React’s flexible nature may result in diverse project structures. Managing state in extensive applications can become intricate without proper architectural planning. The learning curve of React may need help for novice developers. Performance optimization in large React applications demands careful attention.
82. How is context switching managed between React components?
Ans:
- Context switching refers to changing the context data passed down through the component tree.
- React provides a Context API to share data between components without explicitly passing props.
- Using context, components can access shared data at any level of the component tree.
- Context switching ensures efficient data flow across components without prop drilling.
83. What are the differences between useEffect and useLayoutEffect hooks?
Ans:
- useEffect runs after the browser has painted, which is asynchronous and doesn’t block painting.
- useLayoutEffect runs synchronously after all DOM mutations but before the browser paints.
- Use useLayoutEffect when you need to read the layout from the DOM and then immediately re-render.
- useEffect is suitable for data fetching, subscriptions, or side effects that do not impact layout.
84. What is the overview of React’s Fiber Architecture?
Ans:
React Fiber is a reimplementation of the React reconciliation algorithm. It enables incremental rendering, allowing React to prioritize and interrupt rendering as needed. Fiber architecture breaks rendering work into smaller chunks, improving responsiveness and perceived performance. It powers async rendering, error boundaries, and Suspense for data fetching.
85. How is server-side rendering with Node.js implemented in React?
Ans:
- Server-side rendering (SSR) with Node.js involves rendering React components on the server before sending HTML to the client.
- SSR improves initial load time, SEO, and performance by delivering pre-rendered content to users.
- React provides tools like ReactDOMServer for server-side rendering in Node.js environments.
- SSR requires setting up a server to handle HTTP requests and render React components dynamically.
86. What are the best practices for state management in React?
Ans:
Keep the state as localized as possible to minimize complexity and improve maintainability. Use stateful components sparingly and prefer state management libraries like Redux or Context API for complex applications. Normalize state shape for easier manipulation and debugging. Avoid mutating state directly and prefer immutable updates to ensure predictable behavior.
87. How is animation and transition handling managed in React?
Ans:
React provides libraries like React Spring or React Transition Group for animation and transition effects. Use CSS transitions or animations for simple effects and libraries for more complex animations. Ensure smooth performance by optimizing animations for GPU acceleration and reducing layout thrashing. Leverage lifecycle methods or useEffect hook for timing animations with component mounts and updates.
88. What is the significance of “shouldComponentUpdate” in React?
Ans:
- shouldComponentUpdate is a lifecycle method used to optimize component rendering performance.
- It allows components to decide whether a re-render is necessary based on changes in props or state.
- Implementing shouldComponentUpdate prevents unnecessary re-renders from being avoided, improving performance.
- It’s beneficial for optimizing components with expensive rendering operations or frequent updates.
89. What are the benefits of React’s error boundaries?
Ans:
- Error boundaries in React allow gracefully handling runtime errors in components.
- They prevent the entire application from crashing by catching errors and displaying a fallback UI.
- Error boundaries isolate errors to specific components, preventing them from affecting the entire UI tree.
- They improve user experience by showing informative error messages and guiding users to recover from errors.
90. How are memory leaks and performance managed in React?
Ans:
Monitor component lifecycles and memory usage with React DevTools or browser developer tools. To prevent memory leaks, avoid creating unnecessary references or subscriptions in components. The components ‘ cleanup resources, subscriptions, and timers will unmount or useEffect cleanup functions. To reduce rendering overhead, implement performance optimizations like memoization, lazy loading, and code splitting.
91. What are the differences between createElement and JSX syntax in React?
Ans:
- createElement is a function that creates React elements programmatically without JSX.
- JSX is more readable and expressive than createElement, making code easier to understand.
- createElement is typically used when generating React elements dynamically or in non-JSX environments.
- JSX is transpiled to createElement calls during the build process, so both achieve the same result.
92. What are the benefits of using React’s “StrictMode”?
Ans:
- React’s StrictMode helps identify potential issues in your code during development.
- It highlights unsafe lifecycle methods, deprecated APIs, and more.
- It encourages best practices and prepares your codebase for future updates.
- Helps catch and warn about unsafe practices, improving code quality.
- It aids in identifying and fixing common React performance issues.
93. How does data immutability affect performance in React?
Ans:
Immutability ensures data remains unchanged after creation, which enhances predictability and stability. In React, immutable data allows the framework to detect changes efficiently and update the DOM with minimal performance overhead. React can quickly compare previous and current states using immutable data structures, optimizing rendering processes. This approach avoids direct data mutation, making debugging easier and preventing unintended side effects.
94. What is the difference between React’s PureComponent and `shouldComponentUpdate`?
Ans:
PureComponent automatically implements a shallow comparison of props and state for shouldComponentUpdate.It prevents unnecessary re-renders when the props or state haven’t changed.shouldComponentUpdate allows developers to define custom logic to determine if a component should be updated.PureComponent is suitable for simple cases where props and state updates can be shallowly compared.
95. How is code splitting used for bundle optimization in React?
Ans:
- Code splitting divides the application’s codebase into smaller bundles, improving load times.
- It allows loading only the necessary code for the current view or user interaction.
- Laziness and Suspense enable easy implementation of code splitting in React applications.
- Code splitting reduces the initial bundle size, enhancing performance and user experience.
96. What are the limitations of React’s context API for state management?
Ans:
- React’s context API could be more suitable for managing complex or deeply nested states.
- It lacks built-in features for handling updates in a granular or optimized manner.
- Changes to context values trigger re-renders for all components consuming that context.
- Managing multiple related state values with context can lead to performance issues.
- It may need to offer more granularity for controlling state updates in large applications.