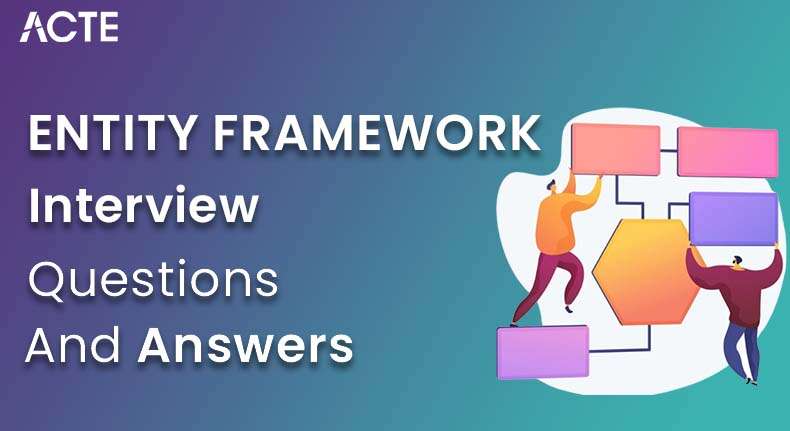
Microsoft created the Entity Framework (EF) as an Object-Relational Mapping (ORM) framework for. NET applications. It streamlines data access and manipulation in software development by enabling developers to operate with database objects as though they were regular objects, significantly simplifying the interface between a database and application code, enhancing development efficiency and ease.
1. What is Entity Framework (EF)?
Ans:
Entity Framework (EF) is a powerful and widely used Object-Relational Mapping (ORM) framework developed by Microsoft for .NET applications. It simplifies the development of data access layers by enabling developers to work with databases using strongly typed .NET objects, eliminating the need to write tedious and error-prone SQL queries manually, enhancing productivity and reducing errors.
2. What are the key components of the Entity Framework?
Ans:
- DbContext: Represents a session with the database and provides APIs for querying, saving, and managing entities.
- Entity Data Model (EDM): Defines the conceptual model of the data, including entities, relationships, and constraints.
- Entity SQL: A query language similar to SQL but designed for querying entity data models.
- LINQ to Entities: Enables querying EF data models using LINQ (Language Integrated Query).
3. What is the Code First approach in Entity Framework?
Ans:
Code First is an approach in Entity Framework that allows developers to define the domain model using Plain Old CLR Object (POCO) classes without any knowledge of the underlying database. The database schema is generated automatically based on the conventions or configurations specified in the code. This approach is ideal for scenarios where the database schema is not predefined or when developers prefer to work with domain models first.
4. What is the Database First approach in Entity Framework?
Ans:
- Database First is an approach in Entity Framework where the entity classes and DbContext are generated based on an existing database schema.
- Developers start by creating a database schema using tools like SQL Server Management Studio or Visual Studio’s Entity Framework Designer.
- Then, EF generates the corresponding entity classes and DbContext, allowing developers to interact with the database using those classes.
5. What is the Model First approach in Entity Framework?
Ans:
Model First is an approach in Entity Framework where developers create the conceptual model using Entity Framework Designer in Visual Studio. The conceptual model defines entities, relationships, and constraints graphically. From this model, EF generates both the database schema and the entity classes. This approach is suitable for scenarios where the database design is more important than the application’s domain model.
6. What is Database Context in Entity Framework?
Ans:
- Database Context is a fundamental class in Entity Framework that represents a session with the database and acts as a bridge between the domain classes (entities) and the database.
- It enables developers to query, insert, update, and delete entities and manage transactions.
- Database Context is part of the Entity Framework Core (EF Core) and replaces the ObjectContext used in previous versions of EF.
7. What is ObjectContext in Entity Framework?
Ans:
Aspect | Advantages | Disadvantages |
---|---|---|
Rapid Development | Quick generation of entity classes and DbContext from an existing database schema. | Generated code might be cluttered and difficult to maintain if the database schema changes frequently. |
Easy Integration | Seamless integration with existing databases and legacy systems. | Limited control over the generated code and mapping. |
Mature Database Design | Can leverage existing database design and stored procedures. | Dependency on the underlying database schema, which might not always align with application requirements. |
Design Consistency | Ensures consistency between the application’s data model and the database schema. | Difficulty in unit testing due to tight coupling with the database. |
8. What is an Entity Data Model (EDM)?
Ans:
Entity Data Model (EDM) is a conceptual model used by Entity Framework to describe the structure of data in terms of entities, relationships, and constraints. It provides a high-level abstraction of the underlying database schema, allowing developers to work with entities and relationships in a domain-centric way rather than focusing on database tables and columns.
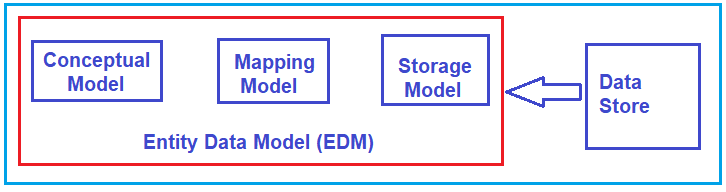
9. What is lazy loading in Entity Framework?
Ans:
- Lazy loading is a feature in Entity Framework that defers the loading of related entities until they are explicitly accessed.
- When an entity with navigation properties is retrieved from the database, EF does not load the related entities immediately.
- Instead, it generates additional SQL queries to load related entities when they are accessed for the first time.
- Lazy loading helps improve performance by only fetching related data when needed.
10. What steps are needed to disable lazy loading in Entity Framework?
Ans:
To stop lazy loading in Entity Framework, set the LazyLoadingEnabled attribute of the Database Context or ObjectContext to false. Entity Framework will no longer load linked entities automatically when accessed if lazy loading is turned off. To ensure that data is loaded as needed, developers must instead use eager loading or explicit loading strategies to retrieve relevant data.
11. What is eager loading in Entity Framework?
Ans:
Eager loading is a technique in Entity Framework in which related entities are explicitly loaded from the database along with the main entity in a single query. Unlike lazy loading, where related entities are loaded on demand, eager loading fetches all related entities upfront, reducing the number of database round-trips and improving performance and efficiency.
12.What methods can be used to implement eager loading in Entity Framework?
Ans:
- Eager loading in Entity Framework can be achieved using the Include method or the Include extension method in LINQ queries.
- By specifying the navigation properties to be included in the query, EF retrieves the related entities along with the main entity in a single database query.
- Developers can retrieve complex object graphs in one go by chaining many Include methods together, which will eagerly load multiple tiers of linked entities.
13. What is explicit loading in Entity Framework?
Ans:
Explicit loading is a technique in Entity Framework in which related entities are loaded from the database on demand, explicitly by the developer. Unlike lazy loading or eager loading, where associated entities are loaded automatically or eagerly, explicit loading gives developers more control over when and how associated entities are fetched from the database.
14. What is the difference between Add and Attach methods in Entity Framework?
Ans:
- In Entity Framework, the Add method adds a new entity to the context and marks it as Added, indicating that it should be inserted into the database upon calling SaveChanges.
- On the other hand, the Attach method attaches an existing entity to the context.
- It marks it as Unchanged, indicating that the context already tracks it and should not be considered for insertion or update during SaveChanges.
15. What is the purpose of the EntityState enumeration in Entity Framework?
Ans:
The Entity Framework’s EntityState enumeration keeps track of the entities that the context is managing. It depicts different states, such as Added, Modified, Deleted, or Unchanged, that signify whether an entity is brand-new, has undergone changes, has been scheduled for removal, or stays the same in the information base. This aids in precisely managing and maintaining changes.
16. What is a Database Set in Entity Framework?
Ans:
In the Entity Framework, a table or group of database entities is represented by a generic collection called a Database Set. It provides methods for querying, adding, updating, and deleting specific kinds of things. A Database Set, a database table or view that facilitates data operations, is commonly defined as a property in the Database Context class.
17. What are the methods for executing raw SQL queries in Entity Framework?
Ans:
- Entity Framework allows developers to execute raw SQL queries against the database using the SqlQuery method or the ExecuteSqlCommand method provided by the DbContext.
- These methods enable developers to execute parameterized SQL queries or execute database commands directly, bypassing EF’s entity tracking and object materialization features.
- When working with raw SQL, developers can map query results to entity types using the SQL function, which allows them to support more complex or optimized searches.
18. What is migration in Entity Framework?
Ans:
Migration in Entity Framework is a technique for managing database schema changes in the Code First approach. It enables developers to evolve the database schema over time while preserving the existing data. Migrations capture the changes made to the domain model and generate corresponding SQL scripts to update the database schema accordingly, ensuring smooth transitions and data integrity.
19. What steps are required to enable migrations in Entity Framework?
Ans:
- Migrations enable developers to manage database schema changes over time while preserving data integrity.
- To enable migrations in Entity Framework, use the Enable-Migrations command in the Package Manager Console (PMC) within Visual Studio.
- This command initializes the migrations configuration for the project, allowing to add and apply migrations.
20. What is the purpose of the Add-Migration command in Entity Framework?
Ans:
The Add-Migration command is used to scaffold a new migration based on the changes made to the model since the last migration. Making changes to the domain model, such as adding new entities, modifying existing entities, or altering relationships, use this command to generate a new migration file. This file contains the necessary code to update the database schema to reflect the changes in the model.
21. What is the purpose of the Update-Database command in Entity Framework?
Ans:
- The Update-Database command applies pending migrations to the database.
- Once added a new migration using the Add-Migration command, use Update-Database to execute the migration and update the database schema accordingly.
- This command ensures that the database schema stays synchronized with the application’s domain model.
22. What is a navigation property in Entity Framework?
Ans:
A navigation property in Entity Framework is a property on an entity class that represents a relationship to another entity. It allows to navigate from one entity to another through defined relationships. For example, in a one-to-many relationship between Author and Book entities, the Author entity may have a navigation property called Books, which represents the collection of books authored by that author.
23. What are the different types of relationships supported by Entity Framework?
Ans:
- One-to-One: There is a precise relationship between each instance of one entity and every other instance.
- One-to-Many: Any given instance of an entity is associated with a collection of several examples from a separate related entity.
- Many-to-Many: Each instance of one entity is connected to a collection of cases related to another, and vice versa.
24. How can relationships be configured in Entity Framework Code First?
Ans:
Relationships in Entity Framework, Code First approach, can be configured using either Fluent API or data annotations. With Fluent API, can define relationships and specify additional configuration options programmatically. Data annotations, on the other hand, allow to configure relationships by adding attributes to the properties of entity classes.
25. What is the purpose of the ForeignKey attribute in Entity Framework?
Ans:
- The ForeignKey attribute is used in Entity Framework to specify the foreign key property in a relationship between two entities.
- By decorating a property with this attribute, explicitly specify which property serves as the foreign key for a particular navigation property, providing EF with the necessary information to establish the relationship.
- Imposing appropriate foreign key constraints at the database level aids in ensuring data integrity.
26. What is the purpose of the InverseProperty attribute in Entity Framework?
Ans:
The InverseProperty attribute is used in Entity Framework to specifices the navigation property at the other end of a relationship. When bidirectional associations exist between entities, the InverseProperty attribute helps EF identify the corresponding matching navigation property on the other entity, establishing the correct relationship between them by mapping.
27. How is inheritance configured in the Entity Framework Code First approach?
Ans:
- Table Per Hierarchy (TPH): In this strategy, all classes in the inheritance hierarchy are mapped to a single database table, and a discriminator column differentiates between different types.
- Table Per Type (TPT): In the TPT strategy, each class in the inheritance hierarchy is mapped to its database table, with shared columns placed in a base table.
- Table Per Concrete Type (TPC): The TPC strategy maps each class in the inheritance hierarchy to its database table without any shared columns, resulting in a more denormalized database schema.
28. What is the purpose of the Discriminator column in Entity Framework?
Ans:
The Discriminator column is used in Entity Framework to differentiate between different types in a hierarchy when using inheritance mapping. It stores a value that indicates the type of entity in the database table, allowing Entity Framework to determine the appropriate type when retrieving data from the database and identifying and materializing entities.
29. What is Change Tracking in Entity Framework?
Ans:
- Change Tracking in Entity Framework refers to the process of keeping track of changes made to entities in the context so that these changes can be persisted in the database.
- EF automatically detects changes made to entity properties and tracks their state, allowing to save those changes to the database using the SaveChanges method.
- It makes concurrency management easier by detecting incompatible changes made to the same data by several users or processes.
30. What does Entity Framework support the different Change Tracking modes?
Ans:
- Automatic: EF automatically detects changes made to entities when SaveChanges is called.
- Snapshot: EF takes a snapshot of the entity state when it is first loaded and compares it with the current state to detect changes.
- Change Tracking Proxy: EF generates proxy classes that override property setters to track changes automatically.
31. How can Change Tracking be disabled in Entity Framework?
Ans:
Set the AutoDetectChangesEnabled attribute of the Database Context to false to stop Change Tracking in Entity Framework. Disabling change tracking can help with performance when doing bulk operations or read-only queries. For example, To improve performance when performing bulk operations or read-only queries in Entity Framework, can disable automatic change tracking.
32. What is the purpose of the UseRelationalNulls method in Entity Framework Core?
Ans:
- The UseRelationalNulls method in Entity Framework Core configures how the underlying relational database provider handles null values.
- It specifies whether database null values should be treated as database nulls or CLR nulls, affecting how null comparisons and operations are performed in queries.
- Applications that must retain particular null handling semantics across several database systems may find this to be especially helpful.
33. What is the purpose of the UseApplicationServiceProvider method in Entity Framework Core?
Ans:
Entity Framework Core’s UseApplicationServiceProvider function sets up the context to use the application’s service provider for dependency injection. By specifying the service provider that EF Core should use to resolve dependencies needed for data access activities, developers may make sure that the services are compatible with the ones set up in the application’s overall service container.
34. What are Shadow Properties in Entity Framework Core?
Ans:
- Shadow Properties in Entity Framework Core are properties that are not defined in the entity class but are used internally by EF Core to store additional information, such as foreign key values or other metadata.
- Shadow Properties are typically used in scenarios where need to map a database column that does not have a corresponding property in the entity class.
- Shadow Properties to add features, such as audit fields or tracking properties, that are required for database operations or business logic but are not displayed explicitly in domain model.
35. How can Shadow Properties be configured in Entity Framework Core?
Ans:
The HasShadowProperty function in the OnModelCreating method of DbContext class or defining them as navigation properties are two ways to configure Shadow Properties using the Fluent API. More flexible and dynamic mappings are possible by mapping database columns to properties that aren’t expressly stated in entity classes by setting shadow properties.
36. What is the purpose of Value Conversions in Entity Framework Core?
Ans:
- Value Conversions in Entity Framework Core serve as a bridge between the representation of data in the application and its storage in the database.
- They allow developers to customize how values are mapped between entity properties and database columns.
- For example, can use value conversions to store enums as integers in the database or to serialize complex types to JSON strings for storage.
37. How can Value Conversions be set up in Entity Framework Core?
Ans:
Value Conversions can be configured using the HasConversion method provided by the Fluent API in Entity Framework Core. This method allows to specify conversion functions to transform property values when reading from or writing to the database. Can configure value conversions in the OnModelCreating method of DbContext class, providing flexibility in how data is stored and retrieved.
38. What is Query Tagging in Entity Framework Core?
Ans:
- Query Tagging in Entity Framework Core enables developers to attach custom data or metadata to queries for logging, tracking, or identification purposes.
- It allows to tag queries with additional information that can be useful for debugging, performance monitoring, or auditing.
- For example, can tag queries with user IDs, request IDs, or timestamps to trace their origin and impact.
39. How can Query Tagging be configured in Entity Framework Core?
Ans:
Query Tagging can be configured using the WithTag method when executing queries in Entity Framework Core. This method allows to attach a tag or metadata object to a query, which is then propagated throughout the query execution pipeline. Can use this feature to add context-specific information to questions and retrieve it later for analysis or logging purposes.
40. What are Global Query Filters in Entity Framework Core?
Ans:
- Global Query Filters in Entity Framework Core allow developers to define filters that are automatically applied to all queries against a particular entity type.
- These filters act as predicates that restrict the results returned by queries, ensuring that only entities matching the specified criteria are retrieved from the database.
- Global query filters are useful for enforcing data access policies, implementing soft delete functionality, or applying row-level security.
41. How can Global Query Filters be set up in Entity Framework Core?
Ans:
Global Query Filters can be configured using the HasQueryFilter method in the OnModelCreating method of DbContext class. This method allows to specify a filter expression that defines the conditions for filtering entities of a particular type. Once configured, the global query filter is applied automatically to all queries targeting the specified entity type, ensuring consistent data access behavior across application.
42. What is the Owned Entity Type in Entity Framework Core?
Ans:
- The Owned Entity Type in Entity Framework Core allows developers to define complex types that are owned by another entity and do not have their own identity.
- These owned types are part of the owning entity and are stored inline within its table in the database rather than having separate tables.
- Owned types are typically used to represent value objects or components that are logically part of an entity but do not have a standalone identity.
43. How can Owned Entity Types be configured in Entity Framework Core?
Ans:
Owned Entity Types can be configured using the OwnsOne or OwnsMany methods provided by the Fluent API in Entity Framework Core. These methods allow to define owned types as navigation properties of the owning entity and specify their mappings to database columns. By configuring owned entity types, can encapsulate complex data structures within entities and improve the organization and clarity of domain model.
44. What is the purpose of the Include method in Entity Framework?
Ans:
The Include function of the Entity Framework loads the primary entity and any related entities from the database in a single query with great eagerness. It lets designate which navigation attributes to include, so relevant entities are obtained right away instead of loading slowly later. This strategy can lessen the number of database queries and improve performance.
45. What is the difference between eager loading and lazy loading in Entity Framework?
Ans:
- Eager loading retrieves related entities along with the main entity in a single query, reducing the number of database round-trips.
- In contrast, lazy loading defers the loading of related entities until they are accessed for the first time, which can lead to additional database queries being executed on-demand.
- Lazy loading might be helpful in situations where related data is not always necessary, but eager loading is usually more effective when know will need relevant data upfront.
46. How does Entity Framework handle transactions?
Ans:
- Entity Framework supports transactions through the DbContext class, allowing developers to perform atomic operations that either succeed or fail as a single unit.
- Transactions can be managed explicitly using the BeginTransaction, Commit, and Rollback methods or implicitly through the SaveChanges method, which wraps multiple operations in a transaction by default.
- Find can potentially reduce database roundtrips for frequently visited entities by utilizing the context’s cache.
47. What is the purpose of the Find method in Entity Framework?
Ans:
The Entity Framework’s Entry function gives access to the metadata and state data that the context is tracking for an entity. Developers can examine and modify the entity’s state, designating it as added, changed, or removed. Furthermore, it allows for access to both the original and current values of the properties, allowing for thorough tracking and personalized management of changes to the entities before they are saved to the database.
48. What is the purpose of the Entry method in Entity Framework?
Ans:
The entry method in the Entity Framework provides access to an entity’s metadata and state information that is being tracked by the context. It allows developers to inspect and manipulate entities’ states, including marking them as added, modified, or deleted and accessing their original and current property values. For instance, To access and modify an entity’s state, use the entry method in Entity Framework, to mark an entity as modified.
49. What is database seeding in Entity Framework?
Ans:
- To make sure the program has the information it needs to run properly right away, this generally entails adding default or required records into tables.
- Entity Framework, the OnModelCreating function of the DbContext class is usually used to configure seeding. Using the HasData method, which enables data to be specified directly in the code.
- To guarantee that the designated data is added to the database, Entity Framework automatically carries out the seeding procedure.
50. What is the purpose of the AsNoTracking method in Entity Framework?
Ans:
The Entity Framework’s AsNoTracking method queries entities without keeping track of their context-based modifications. By avoiding the change tracking techniques, this technique enhances performance and is especially useful in cases where entities are not anticipated to be modified or remain. NoTracking minimizes processing overhead and memory use, particularly in situations in which extensive datasets are retrieved.
51. What is the purpose of the ToListAsync method in Entity Framework?
Ans:
- The ToListAsync method in Entity Framework is used to asynchronously execute a query and retrieve the results as a list of entities.
- It allows developers to perform non-blocking database operations, improving scalability and responsiveness in applications that require asynchronous data retrieval.
- The approach speeds up application performance by releasing the caller thread while awaiting the completion of the database operation.
52. What is the purpose of the FromSqlRaw method in Entity Framework?
Ans:
With the From SqlRaw function, Entity Framework enables the direct execution of raw SQL queries against the database. By using this method or technique, can still benefit from Entity Framework’s entity tracking and materialization features and utilize all the SQL’s power. It offers a versatile method for handling entities and working with raw SQL.
53. What is the purpose of the ExecuteSqlRaw method in Entity Framework?
Ans:
- The ExecuteSqlRaw method in Entity Framework executes parameterized SQL commands against the database.
- Unlike query methods like FromSqlRaw, ExecuteSqlRaw is intended for executing non-query SQL commands, such as INSERT, UPDATE, DELETE, or stored procedure calls, which do not return entity results.
- ExecuteSqlRaw can be used for database-affecting operations that don’t call for data retrieval.
54. What is the purpose of the RemoveRange method in Entity Framework?
Ans:
Removing numerous entities from the context at once is made easier by using the RemoveRange function in Entity Framework. With this method, can eliminate multiple entities in a batch at once, cutting down on database round trips and improving speed and efficiency. Large datasets benefit greatly from it as well because it streamlines the elimination procedure.
55. What is the purpose of the ValueGeneratedOnAddOrUpdate method in Entity Framework?
Ans:
The ValueGeneratedOnAddOrUpdate method in Entity Framework configures properties to be automatically populated with values generated by the database upon insertion or update. It allows developers to define properties such as timestamps or version numbers that are managed by the database, ensuring consistency and accuracy in concurrent environments.
56. What is the purpose of the InMemory provider in Entity Framework Core?
Ans:
- The InMemory provider in Entity Framework Core allows developers to create an in-memory database for testing or prototyping purposes.
- It simulates a relational database entirely in memory, providing fast and lightweight data access without the need for an external database server.
- To make sure that test scenarios don’t alter actual data, it helps isolate tests from the production database.
57. What is the purpose of the AsSplitQuery method in Entity Framework Core?
Ans:
Dividing a query into numerous SQL queries using Entity Framework Core’s AsSplitQuery function to retrieve pertinent data more quickly. By simplifying the execution of complex queries involving several related entities and reducing the quantity of data fetched in each query, and this method may enhance overall performance and query efficiency.
58. What is the purpose of the UseLazyLoadingProxies method in Entity Framework Core?
Ans:
- The UseLazyLoadingProxies method in Entity Framework Core enables lazy loading for navigation properties on entity classes.
- EF Core dynamically generates lazy loading proxies to transparently load related entities from the database when accessed for the first time, allowing for more efficient resource use and improved performance.
- By automatically handling the loading of entities only when necessary, it streamlines the management of linked data.
59. What is the purpose of the EnsureCreated method in Entity Framework Core?
Ans:
The EnsureCreated method in Entity Framework Core ensures that the database schema corresponding to the context’s model is created in the database. It is primarily intended for development and testing scenarios where automatic migrations are not desired, providing a quick way to create the database schema based on the current model.
60. What is the purpose of the EnsureDeleted method in Entity Framework Core?
Ans:
- The EnsureDeleted method in Entity Framework Core ensures that the database corresponding to the context’s model is deleted from the database server.
- It is often used in conjunction with the EnsureCreated method to recreate the database from scratch, allowing for a clean slate when testing or debugging.
- Resetting the database state in between tests helps to guarantee that every test begins in a uniform and new environment.
61. What is the purpose of the ModelSnapshot file in Entity Framework Core?
Ans:
Entity Framework Core automatically generates a code file named ModelSnapshot that takes a snapshot of the model in its present state. Comprehensive metadata about the entities, their traits, and the connections between them are included in this file. It is essential to the development of the database schema and acts as a point of reference for migrations.
62. What is the purpose of the AsNoTrackingWithIdentityResolution method in Entity Framework Core?
Ans:
The AsNoTrackingWithIdentityResolution method in Entity Framework Core executes a query without tracking changes to entities while still ensuring that entities with the same key are returned as the same instance. It combines the benefits of no-tracking queries and identity resolution, providing predictable behavior when querying entities with relationships.
63. What is the purpose of the UseQuerySplittingBehavior method in Entity Framework Core?
Ans:
- The UseQuerySplittingBehavior method in Entity Framework Core is used to configure how queries are split when querying entity types with inheritance hierarchies.
- It allows developers to specify whether queries should be divided into separate SQL statements for each derived type or combined into a single SQL statement for better performance and efficiency.
- Developers may select the best strategy depending on their unique use cases and performance requirements thanks to this technology, which helps control the trade-offs between query performance and database load.
64. What is the purpose of the HasData method in Entity Framework Core migrations?
Ans:
Entity Framework Core migrations use the HasData function to seed initial data into the database during migration processes. With the help of this function, developers can specify precisely which data should be added to tables when the database schema is established or modified. This helps with the initial configuration of the program and guarantees that the database contains the necessary predefined entries from the outset.
65. What is the purpose of the HasAnnotation method in Entity Framework Core?
Ans:
- The HasAnnotation method in Entity Framework Core attaches custom annotations or metadata to various model elements, such as entities, properties, relationships, or indexes.
- Annotations provide additional information about the model that EF Core or third-party libraries can use for various purposes, such as code generation, serialization, or documentation.
- They enable more advanced model element configuration and customization beyond the pre-installed EF Core norms.
66. What is the purpose of the Detach method in Entity Framework Core?
Ans:
Use Entity Framework Core’s Detach function to remove an entity from the context and cease tracking changes to it. Wish to stop tracking changes made to an entity or stop it from inadvertently being saved in the database during save operations, this approach is quite helpful. Using the Detach approach helps retain control over which entities are being tracked and guarantees more effective management of entity states.
67. What is the purpose of the Local property in Entity Framework Core?
Ans:
The local property in Entity Framework Core provides access to the local attributes gives users access to the locally stored entities that the context is monitoring. After an entity has been loaded into memory, It allows to enumerate and manipulate entities that have been loaded into memory without executing additional database queries.
68. What is the purpose of the Entry? State property in Entity Framework Core?
Ans:
- The Entry. State property in Entity Framework Core represents the state of an entity being tracked by the context.
- It indicates whether the entity is new, modified, deleted, or unchanged, providing information about how the entity will be handled when changes are saved to the database.
- It can be helpful in situations when need to programmatically control how EF Core records and manages the persistence and lifetime of the entity. It lets developers manually set the state of the entity.
69. What is the purpose of the ChangeTracker property in Entity Framework Core?
Ans:
Work with the state of the entities being tracked by using the ChangeTracker property of Entity Framework Core, which gives access to the context’s change-tracking capabilities. Can attach or remove entities, query pending modifications, and control how changes are handled and tracked within the context. Observe and alter an entity’s state to provide with more precise control over how changes are handled and maintained.
70. What is the purpose of the ToQueryString method in Entity Framework Core?
Ans:
- The ToQueryString method in Entity Framework Core is used to generate the SQL query corresponding to a LINQ query or IQueryable expression.
- It allows to inspect the generated SQL code for debugging or optimization purposes, providing visibility into how EF Core translates LINQ queries into SQL statements.
- It can help diagnose performance problems or confirm query correctness in addition to assisting in ensuring the resulting SQL requirements.
71. What is the purpose of the conversion method in Entity Framework Core migrations?
Ans:
When creating or executing migrations, value conversions for properties are configured using the conversion method in Entity Framework Core migrations. Using this technique, can define custom conversion routines to change property values between the database representation and the application. This guarantees data integrity across several platforms and interoperability amongst diverse data types.
72. What is the purpose of the UseSqlServer method in Entity Framework Core?
Ans:
- The UseSqlServer method in Entity Framework Core configures the context to use SQL Server as the underlying database provider.
- It specifies the connection string and other options required to establish a connection to the SQL Server database instance, enabling data access operations using EF Core.
- It enables the setting of features unique to SQL Server, like logging and connection resilience.
73. What is the purpose of the UseInMemoryDatabase method in Entity Framework Core?
Ans:
Entity Framework Core’s UseInMemoryDatabase function sets up the context to use an in-memory database provider, usually for prototyping or testing. An in-memory database instance is created, and it stays that way for the runtime of the application. An external database server is not necessary with this configuration, which enables quick and lightweight data access.
74. What is the purpose of the AsQueryable method in Entity Framework Core?
Ans:
- The AsQueryable method in Entity Framework Core is used to convert an IEnumerable collection or a DbSet into an IQueryable queryable collection.
- It allows to compose LINQ queries dynamically and apply additional query operators before executing the query against the database.
- It offers the option to use postponed execution, which guarantees that the query won’t run until the results are truly listed.
75. What is the purpose of the UseQueryHint method in Entity Framework Core?
Ans:
- The UseQueryHint method in Entity Framework Core provides query hints to the underlying database provider.
- It allows developers to specify hints or directives that influence the execution plan of SQL queries, such as index hints or query optimizer directives, to improve query performance.
- More precise control over query performance tuning is possible with this strategy, which is particularly helpful in circumstances with high demand or complexity when default query optimization might not be adequate.
76. What is the purpose of the FindAsync method in Entity Framework Core?
Ans:
- The FindAsync method in Entity Framework Core is an asynchronous version of the Find method, which retrieves an entity from the context by its primary key.
- It allows for non-blocking database operations when fetching entities, improving performance and scalability in asynchronous applications.
- To facilitate cooperative cancellation and improve the timeliness of persistent searches, FindAsync can make use of cancellation tokens.
77. What is the purpose of the UseQueryCache method in Entity Framework Core?
Ans:
- The UseQueryCache method in Entity Framework Core is used to enable query caching for queries executed by the context.
- It allows EF Core to cache query results in memory, improving performance by avoiding redundant database round-trips for identical queries.
- The method lessens the overall strain on the database server, which can be especially helpful in situations with heavy traffic and repeated execution of the same queries.
78. What is the purpose of the ExecuteSqlCommand method in Entity Framework Core?
Ans:
Use Entity Framework Core’s ExecuteSqlCommand function to run raw SQL commands against a database directly. Developers can execute stored procedures or carry out operations like INSERT, UPDATE, and DELETE without depending on the default query translation techniques provided by EF Core. Enabling direct SQL command execution offers more flexibility and helpful for tasks that are difficult to accomplish using the standard EF Core querying.
79. What is the purpose of the ApplyConfiguration method in Entity Framework Core?
Ans:
Entity Framework Core’s ApplyConfiguration function applies entity-type configurations from different configuration classes to the model. Divided into distinct classes, it aids developers in organizing and modularizing their model configuration. This method makes the configuration easier to manage and update by improving the readability and maintainability of the code.
80. What is the purpose of the UseQueryTrackingBehavior method in Entity Framework Core?
Ans:
- The UseQueryTrackingBehavior method in Entity Framework Core configures the context’s query-tracking behavior.
- It allows developers to specify whether queries should track changes to entities or not, providing flexibility in managing the performance and behavior of data access operations.
- When updating entities is not necessary, query speed can be enhanced by setting the tracking behavior to NoTracking.
81. What is the purpose of the GetDatabaseValuesAsync method in Entity Framework Core?
Ans:
Entity Framework Core’s GetDatabaseValuesAsync method asynchronously fetches the current values of an entity from the database. Developers can use it to compare these values to the initial values of the entity, which can help with conflict resolution and optimistic concurrency control. This approach is helpful in situations when there may be simultaneous updates.
82. What is the purpose of the IsConcurrencyToken attribute in Entity Framework Core?
Ans:
- The IsConcurrencyToken attribute in Entity Framework Core is used to specify that a property should be included in concurrency checks when updating entities.
- It marks the property as a concurrency token, allowing EF Core to detect and handle concurrent updates to the same entity by multiple users or processes.
- By guaranteeing that updates are applied only if the data has not changed since it was last read, this feature helps prevent data loss.
83. What is the purpose of the UseSnakeCaseNamingConvention method in Entity Framework Core?
Ans:
The UseSnakeCaseNamingConvention method in Entity Framework Core configures the naming convention for database objects to use the snake_case naming style. It converts entity and property names to lowercase and separates words with underscores when generating database tables, columns, and constraints, ensuring consistency with database naming conventions.
84. What is the purpose of the AsTracking method in Entity Framework Core?
Ans:
- The AsTracking method in Entity Framework Core enables change tracking for entities returned by a query.
- It ensures that any changes made to the entities are tracked by the context, allowing for automatic modification detection and synchronization with the database when changes are saved.
- It guarantees that EF Core maintains track of these entities for actions in the future and can be helpful in situations where need to update entities after querying them.
85. What is the purpose of the UseSqlite method in Entity Framework Core?
Ans:
Entity Framework Core’s UseSqlite function sets up the context to utilize SQLite as the underlying database provider. It provides the options and connection string required to establish a connection to the SQLite database file. This configuration supports scenarios involving lightweight and embedded databases and allows data access operations with EF Core using SQLite databases.
86. What is the purpose of the ConfigureWarnings method in Entity Framework Core?
Ans:
The ConfigureWarnings method in Entity Framework Core is used to control how the context manages warnings. It enables developers to suppress specific warnings or customize how warnings are reported by EF Core during various operations such as query execution, migration generation, and other processes. This helps in managing and optimizing warning handling effectively.
87. What are the attributes of Entity Framework?
Ans:
- Database tables are mapped to.NET objects via ORM.
- Code First uses C# classes to define the schema.
- Database First uses an already-existing database to generate code.
- Model First use a visual designer to create the schema.
- Change tracking keeps track of entity changes automatically.
88. What does the term “optimistic locking” mean?
Ans:
A concurrency management technique called optimistic locking allows transactions to continue without locking data. To determine whether data has changed since it was last read, it uses version numbers or timestamps; if a conflict is discovered, the transaction is rolled back or retried. More concurrency is possible and performance is enhanced with this method.
89. What are the Entity Framework’s main purposes?
Ans:
- ORM: Database tables are mapped to .NET classes via object-relational mapping.
- CRUD Operations: Consolidates the processes of Create, Read, Update, and Delete.
- Change tracking: The process of automatically recording and storing database changes.
- Migration: Oversees version control and modifications to the database structure.
- Validation: Verifies that data complies with specified guidelines before storing.
90. What is meant when a SQL injection attack is discussed?
Ans:
SQL injection attack arises when a hacker manages to “inject” or enter malicious SQL code into a query that a database is running. Usually occurs when user input is not adequately sanitized or validated, which gives the attacker the ability to alter the query inadvertently. This may result in data tampering, illegal access to data, or even total control over the database. It essentially poses a serious security risk by taking advantage of flaws in the way apps process database requests.