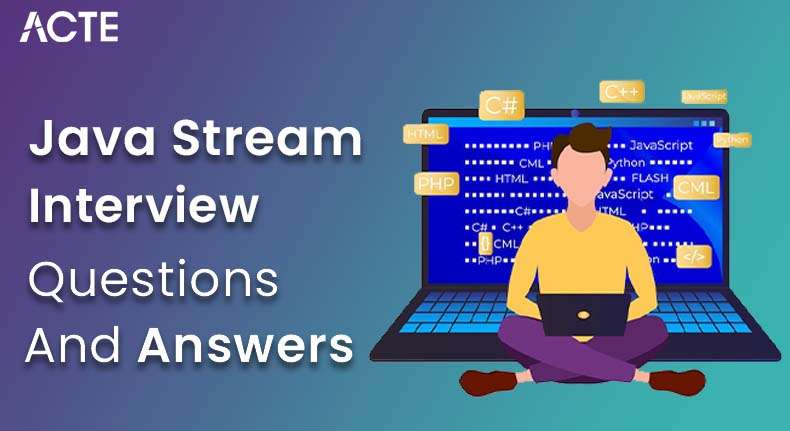
40+ [REAL-TIME] Java Stream Interview Questions and Answers
Last updated on 26th Apr 2024, Popular Course
Java Stream, which was introduced in Java 8, is a powerful API designed to allow functional-style operations on sequences of data. It provides a succinct and expressive way to run bulk operations on a variety of data sources, including collections and arrays. Java Stream enables developers to effectively handle and alter data by performing operations such as filtering, mapping, reduction, and collection. This promotes cleaner, more understandable code while also encouraging parallel execution, which improves speed. Java Stream is an essential component of modern Java development, providing a flexible and simplified approach to data processing chores.
1. What Is the Predicate?
Ans:
A Predicate in Java is a functional interface that represents a boolean-valued function of one argument. It’s often used to filter elements in collections or streams based on certain conditions. Predicates are commonly employed with methods like filter() in streams to test elements for a specified condition.
2. What Is a Stream API?
Ans:
The Java Stream API provides a mechanism for processing collections of objects in a functional and declarative manner. It enables developers to perform operations such as filtering, mapping, and reducing collections with concise and expressive syntax. Streams promote functional programming paradigms and facilitate parallel processing.
3. What makes the Stream API required?
Ans:
- The Stream API is essential in Java for efficient and concise manipulation of collections.
- It offers powerful operations to process data in a declarative manner, promoting cleaner and more readable code.
- Additionally, streams enable parallel execution, enhancing performance in multi-core environments.
4. What separates a skip from a limit?
Ans:
In Java streams, skip() is an intermediate operation that discards the first n elements from a stream, allowing subsequent elements to pass through. Conversely, limit() restricts the stream to a maximum size of n elements, effectively truncating the stream to a specified length.
5. What is the purpose of the limit() method in Java 8?
Ans:
- The limit() method in Java 8’s Stream API is used to restrict the size of a stream to a maximum number of elements.
- It is commonly employed to limit the amount of data processed or to extract a fixed-size subset from an infinite stream, improving performance and memory efficiency.
6. What sets Stream’s Terminal and Intermediate Operations apart?
Ans:
Terminal operations in Java streams produce a result or side effect, causing the stream to be consumed and potentially terminated. Intermediate operations, on the other hand, transform or filter the elements of a stream and return a new stream, allowing for further processing. This distinction ensures stream operations are lazy and efficient.
7.What makes the Stream object’s findFirst() function different from findAny()?
Ans:
Feature | findFirst() | findAny() | |
Return |
Returns the first encountered element |
Returns any element from the stream | |
Determinism | Deterministic | Non-deterministic | |
Suitable for parallel streams |
Less suitable, as it’s deterministic |
More suitable, as it allows for better load balancing | |
Performance on sequential streams | May be faster as it doesn’t search beyond the first element | Similar performance to findFirst() | |
Usage |
Typically used when order matters |
Often used in parallel processing for better performance |
8. What Is the Consumer Functional Interface?
Ans:
In Java, the Consumer functional interface represents an operation that accepts a single input argument and performs some action without returning any result. It is commonly used with methods like forEach() to process elements of collections or streams in a specified manner.
9. What Is the Supplier Functional Interface?
Ans:
The Supplier functional interface in Java represents a supplier of results capable of generating or providing values without taking any input. It is often used to lazily initialize objects or to generate streams of values dynamically.
10. What sets a map apart from the stream operation of flatMap?
Ans:
- The map() operation in Java’s Stream API applies a function to each element of the stream and returns a new stream containing the results.
- In contrast, flatMap() applies a function to each element and flattens the resulting streams of elements into a single stream.
- This is particularly useful when dealing with nested collections or optional values.
11. What does MetaSpace in Java 8 mean?
Ans:
Metaspace in Java 8 replaces the permanent generation (PermGen) space. It stores class metadata and internal JVM structures. Unlike PermGen, Metaspace dynamically resizes itself based on application demand. This helps prevent OutOfMemoryError due to class metadata exhaustion. Metaspace is garbage collected, reducing the risk of memory leaks.
12. What separates a stream from a collection?
Ans:
- A stream is a collection of items that a pipeline can process.
- Collections store and manage elements directly, while streams don’t store elements themselves.
- Streams are designed for functional-style operations like filtering, mapping, and reducing.
- Collections are concrete data structures, while streams are a view or abstraction of data.
13. What makes the functions map() and flatMap() different?
Ans:
- Map () transforms each element of a stream according to a provided function.
- flatMap() transforms each element of a stream into a stream of values and then flattens these streams into a single stream.
- map() preserves the stream’s structure, whereas flatMap() can flatten nested collections or maps.
- map() is one-to-one transformation, while flatMap() is one-to-many transformation.
- Map () applies a function to each element independently, whereas flatMap() can produce a different number of elements.
- flatMap() is often used for scenarios involving nested collections or optional values.
14. What is meant by Java’8 Stream pipelining?
Ans:
Stream pipelining in Java 8 involves chaining multiple stream operations together. It enables concise and expressive code for data processing tasks. Operations are performed sequentially, with each stage passing its output to the next. Pipelining encourages functional programming style and method chaining. It allows for lazy evaluation, improving efficiency by deferring computation until necessary.
15. How do the static methods in Interfaces work?
Ans:
Static methods in interfaces were introduced in Java 8 to provide utility methods. They can be called directly on the interface without implementing it, and they cannot be overridden by implementing classes. They help organize utility functions related to the interface’s purpose. Static methods promote code reuse without requiring concrete implementations.
16. Which three components make up a stream pipeline?
Ans:
- Source: The stream source provides the initial set of elements.
- Intermediate operations: These transform or filter the elements as they flow through the pipeline.
- Terminal operation: This produces a result or side-effect, triggering the execution of the entire pipeline.
- These components work together to process elements efficiently and flexibly.
17. How do streams with lazy evaluations operate?
Ans:
Streams with lazy evaluation postpone computation until necessary. Lazy evaluation enables efficiency by avoiding unnecessary computation. It supports processing large or infinite streams without running out of memory. Operations are fused, optimizing the execution of the entire stream pipeline.
18. What does Java’s arrays dot stream function do?
Ans:
- Arrays. Stream () converts an array into a stream of elements.
- It provides a convenient way to work with arrays using stream operations.
- This allows array elements to be processed using the rich set of stream API functions.
- It supports both primitive and object arrays.
- Arrays. Stream () facilitates functional-style operations on array elements.
19. In Java, what is the peak terminal operation?
Ans:
The peak terminal operation, represented by peek(), allows for side effects during stream processing. It inspects elements as they flow through the stream pipeline without modifying them. Peek () is often employed for debugging or logging purposes. Unlike other terminal operations, peek() doesn’t trigger the stream pipeline until a subsequent terminal operation is invoked.
20. What does Java’s generate from stream function aim to achieve?
Ans:
- Java’s generate() method produces an infinite stream by repeatedly invoking a supplier function.
- It’s used to create streams without fixed sizes or bounds.
- generate() is typically combined with limit() to produce finite streams.
- It’s useful for scenarios like generating random numbers or simulating infinite sequences.
- The supplier function determines the elements generated in the stream.
- Generate () enables lazy evaluation and supports a functional programming paradigm.
21. What are the parameters of stream dot iterate?
Ans:
- The parameters are the initial seed value and a function.
- The initial seed value is the starting point of the iteration.
- The function generates the next element based on the previous one.
- It continuously applies the function to the previous element.
- It can produce an infinite stream.
- The resulting stream can be limited or manipulated further.
22. What kinds of terminal activities are there in a stream?
Ans:
Terminal activities include operations that produce a result. Examples are forEach, collect, reduce, and toArray. These operations trigger the execution of the stream pipeline. Other stream operations cannot follow these operations. They consume the elements of the stream and are necessary to obtain a final result from the stream.
23. How do you create a stream in Java?
Ans:
Streams can be created from collections using the stream() method. Arrays can be converted to streams using Arrays. stream(). The stream. The () method can be used to create streams of individual elements. The stream. Iterate () and Stream. Generate () methods can create infinite streams. The files. The lines () method can create streams from files.IntStream, LongStream, and DoubleStream can be created using respective range() methods.
24. What is the difference between a sequential and parallel stream in Java?
Ans:
- Sequential streams process elements in a single-threaded manner.
- Parallel streams distribute elements across multiple threads for processing.
- Parallel streams may offer performance benefits for computationally intensive tasks.
- Sequential streams are typically easier to debug and reason about.
- Parallel streams may not guarantee a deterministic order of processing.
- Proper synchronization is required for parallel streams to avoid race conditions.
25. How do you convert a Collection to a Stream in Java?
Ans:
- Use the stream() method provided by the Collection interface.
- It returns a sequential stream containing the collection elements.
- For Sets and Lists, this method traverses the elements in the order they were inserted.
- For Map, stream() returns a stream of key-value pairs.
- Streams can be processed sequentially or in parallel based on the context.
- The resulting stream can then be manipulated using stream operations.
26. Explain the concept of “short-circuiting” in Java streams.
Ans:
Short-circuiting allows streams to terminate early, improving performance by avoiding unnecessary processing. Operations like findFirst(), find (), and limit() are short-circuiting. When the desired condition is met, further elements are not processed. This is similar to short-circuit evaluation in logical expressions. Short-circuiting operations can improve the efficiency of stream processing.
27. What is the purpose of the filter() method in Java streams?
Ans:
- The filter() function in Java streams is utilized to selectively extract elements from a stream based on a specified predicate.
- Each element within the stream is assessed against the provided condition, and only those that meet the criteria are preserved.
- Its primary function is to eliminate undesirable elements from the stream, thereby retaining only those that fulfill the specified condition.
- By doing so, it aids in the creation of more refined and focused streams by excluding elements that do not meet the specified criteria.
28. How do you find the maximum element in a Java stream?
Ans:
- In Java streams, the max () method is employed to ascertain the maximum element within a stream.
- Upon invocation, this method yields an Optional<T> object containing the highest element present in the stream based on the provided comparator.
- It’s essential to ensure that the elements within the stream are comparable for accurate comparison.
- If the stream happens to be empty, the method returns Optional. empty().
29. Explain the usage of the distinct() method in Java streams.
Ans:
The distinct() method in Java streams guarantees that the resultant stream contains solely distinct elements. Its function entails removing duplicate elements from the stream, thereby ensuring uniqueness in the resulting output. Upon application, it preserves solely the initial occurrence of each unique element while discarding subsequent repetitions. The determination of distinctness is carried out using the equals() method of the elements.
30. What is the difference between forEach() and forEachOrdered() in Java streams?
Ans:
- Both forEach() and forEachOrdered() represent terminal operations employed for iterating over the elements of a stream.
- Nonetheless, each () executes in an unordered manner, processing elements as they are encountered.
- Conversely, forEachOrdered() guarantees that elements are processed in the same order as they appear within the stream.
- While forEach() may offer superior performance for unordered streams, forEachOrdered() becomes indispensable when maintaining order is imperative.
31. How do you sort elements in a stream in Java?
Ans:
- You use the sorted() method in Java streams.
- This method sorts the elements based on their natural order or a custom comparator.
- It returns a new stream with the elements sorted accordingly.
- Their Comparable implementation defines the natural order for objects.
- For custom sorting, you can pass a Comparator using the sorted() method.
- Sorting is a terminal operation in streams.
32. What is the purpose of the reduce() method in Java streams?
Ans:
The reduce() method in Java streams reduces the elements of the stream and combines them into a single result. The method takes a BinaryOperator as a parameter and can perform tasks like summing, finding max or min, or even string concatenation. It returns an empty Optional if the stream is empty. It’s a terminal operation in streams.
33. Explain the usage of the collect() method in Java streams.
Ans:
The collect() method is used to accumulate elements into a collection. It takes a Collector as a parameter, defining how the elements should be collected. Collectors can collect elements into different types of collections, like lists, sets, or maps. Collectors also support the grouping and partitioning of elements. It provides a mutable reduction operation. This operation can be parallelized and is a terminal operation in streams.
34. How do you create an infinite stream in Java?
Ans:
- You can create an infinite stream using methods like Stream. Iterate () or Stream. generate().
- Stream. Iterate () takes an initial value and a function to generate the next value.
- Stream. Generate () takes a Supplier to generate values.
- Both methods produce streams of unlimited size unless bounded.
- You need to use methods like limit() to make them finite.
- Infinite streams are useful for representing sequences or generating data.
35. What is the difference between map() and flatMap() when working with streams in Java?
Ans:
- Map () transforms each element of a stream into another object using the provided function.
- flatMap() transforms each element into a stream of objects and then combines all the streams into a single stream.
- map() is suitable for one-to-one transformation.
- flatMap() is used for one-to-many transformations.
- map() doesn’t flatten the result, while flatMap() does.
- flatMap() is useful when dealing with nested collections or optional values.
36. How do you find the minimum element in a Java stream?
Ans:
You can use the min() method in Java streams to find the minimum element. This method takes a Comparator to determine the ordering. It returns an Optional containing the minimum element or an empty Optional if the stream is empty. The Comparator can be a natural order or a custom comparator. It’s a terminal operation in streams. You can combine it with orElse() to handle the case when the stream is empty.
37. Explain the concept of “stream chaining” in Java.
Ans:
Stream chaining involves combining multiple stream operations in a single statement. Each intermediate operation returns a new stream, allowing for a fluent API. Operations like filter, map, and sort can be chained together to perform complex transformations. Chaining allows for lazy evaluation, improving performance. Terminal operations trigger the execution of the entire stream pipeline. Stream chaining promotes concise and expressive code.
38. What is the purpose of the allMatch() method in Java streams?
Ans:
- The `match ()` function determines if every element in the stream satisfies a specified criterion.
- It returns true if all elements satisfy the predicate; otherwise, it is false.
- It short-circuits, meaning it stops processing once a non-matching element is found.
- It’s useful for checking conditions on all elements of a stream.
- It’s a terminal operation in streams.
- It’s often used in conjunction with lambda expressions or method references.
39. How do you convert a stream of objects to a stream of primitive types in Java?
Ans:
- You can use the mapToX() method, where X represents the primitive type.
- For example, mapToInt(), mapToLong(), or mapToDouble(), depending on the primitive type.
- These methods perform the necessary boxing and unboxing operations internally.
- They allow for efficient operations on primitive values.
- You provide a function to convert object types to primitive types.
40. Explain the usage of the skip() method in Java streams.
Ans:
The skip() method in Java streams skips the first n elements of the stream. It returns a new stream with the remaining elements after skipping. If the stream has fewer than n elements, an empty stream is returned. It’s useful for pagination or skipping initial elements based on some condition. It’s a stateful intermediate operation in streams. It’s often used in conjunction with limit() to paginate through large datasets.
41. How do you convert a stream to an array in Java?
Ans:
To transform a stream into an array in Java, you utilize the toArray() method. Invoke toArray() on the stream, returning an array encompassing the stream’s elements. Should you require a specific array type, you can supply a generator function using the toArray() method. For instance, for converting a stream of integers to an array, you can employ toArray(IntFunction generator). This functionality facilitates the conversion of stream elements into a fixed-size array.
42. What is the purpose of the findAny() method in Java streams?
Ans:
- The findAny() method within Java streams seeks to identify any element from the stream.
- It furnishes an Optional containing any element encountered within the stream.
- This is particularly beneficial in parallel streams, where the order is non-deterministic.
- In the event the stream is devoid of elements, it returns an empty Optional.
- findAny() yields a non-deterministic outcome in parallel streams.
43. Explain the usage of the anyMatch() method in Java streams.
Ans:
anyMatch() in Java streams verifies if any stream element satisfies a given predicate. It delivers a boolean denoting whether any element meets the specified condition. Employing short-circuiting, it halts processing upon encountering a matching element. It is valid for confirming whether at least one element meets a condition. Its utility extends to diverse conditions like existence checks, validation, or filtering.
44. How do you concatenate two streams in Java?
Ans:
Java provides the Stream—Concat () method, which is used to concatenate two streams. Simply invoke Stream.concat(stream1, stream2), with stream1 and stream2 representing the streams to concatenate. This operation engenders a new stream comprising elements from the first stream followed by those from the second stream. Preservation of element order is maintained within the concatenated stream. It facilitates amalgamating multiple streams into a singular one.
45. What is the purpose of the peek() method in Java streams?
Ans:
- Peek () within Java streams is employed for debugging or executing side-effect operations.
- It permits the execution of actions on each stream element without affecting the stream itself.
- Frequently employed for logging, debugging, or monitoring endeavors.
- Yields a new stream, preserving the original stream unaltered.
- Operating as an intermediate step, it doesn’t prompt terminal operations.
46. Explain the usage of the count() method in Java streams.
Ans:
- The count() method within Java streams serves to ascertain the count of elements in the stream.
- It furnishes a long value denoting the element count.
- This method constitutes a terminal operation, initiating the execution of the stream pipeline.
- Tailored for both sequential and parallel streams, ensuring efficiency.
- It is particularly advantageous for finite streams, facilitating the determination of stream size.
47. How do you group elements in a Java stream?
Ans:
In Java streams, element grouping is accomplished using the Collectors. Grouping () method. This method necessitates a classifier function delineating the grouping criteria. It yields a Collector grouping stream elements based on the classifier function. The outcome is a Map featuring grouped elements, with keys representing groups and values containing respective element lists.
48. What is the purpose of the flatMapToInt() method in Java streams?
Ans:
- flatMapToInt() within Java streams functions to flatten a stream of elements into an IntStream.
- It applies the provided function to each element, culminating in an IntStream.
- This method proves instrumental in converting object streams to streams of primitive integers.
- It is particularly advantageous when operations necessitate primitive streams for efficiency.
- Operating as an intermediate step, it alters elements while retaining the stream type.
49. Explain the usage of the mapToInt() method in Java streams.
Ans:
- The mapToInt() method in Java streams transforms stream elements into an IntStream.
- It applies the provided function to each element, yielding an IntStream containing the outcomes.
- Valuable for converting objects into primitive integers for numerical or arithmetic operations.
- As an intermediate operation, it doesn’t trigger stream pipeline execution.
- Typically deployed in conjunction with other stream operations like filtering or mapping.
50. How do you create a parallel stream in Java?
Ans:
To create a parallel stream in Java, utilize the parallelStream() method on a collection or existing stream. Invoke parallel stream () on a collection or extant stream to acquire a parallel stream. Parallel streams leverage multiple threads for processing, accelerating computations for sizable datasets. They effectively facilitate concurrent operations, capitalizing on multi-core processors. However, parallel streams introduce overhead due to thread management, making them ideal for CPU-bound tasks.
51. What is the purpose of the toArray() method in Java streams?
Ans:
- toArray() converts a stream into an array in Java, aiding in data manipulation.
- It’s a convenient way to collect stream elements into an array for further processing.
- This method facilitates scenarios requiring array manipulation or compatibility with array-based methods.
- It simplifies the conversion of stream elements to an array, enhancing code readability.
- toArray() ensures seamless integration with methods expecting arrays as input parameters.
52. Explain the usage of the range() method in Java streams.
Ans:
- Range () generates a sequential stream of integers within a specified range, fostering numerical operations.
- It accepts a start (inclusive) and an end (exclusive) value as parameters, defining the range.
- This method aids in creating streams representing sequences of numbers for various computations.
- It’s commonly employed alongside map() or filter() operations for further data processing.
- Range () simplifies the generation of streams containing sequential integers, enhancing code clarity.
53. How do you convert a stream of strings to uppercase in Java?
Ans:
- Transform each string to uppercase using map() with String::toUpperCase.
- String::toUpperCase refers to the method of converting strings to uppercase.
- This functional operation is non-destructive, creating a new stream with uppercase strings.
- It’s a fundamental transformation task in string processing, ensuring data consistency.
- The operation maintains the original stream intact while generating a modified stream.
- Utilizing map() with String::toUpperCase enhances readability and simplifies uppercase conversion.
54. What is the purpose of the limit() method in Java streams?
Ans:
Limit () restricts the size of the stream, aiding in efficient data processing. It accepts an integer parameter indicating the maximum number of elements to process. This method is beneficial for managing large or infinite streams, focusing on a subset of elements.limit() ensures streamlined processing by avoiding unnecessary computations on excessive data. It’s commonly employed to extract a specific portion of the stream for further analysis.
55. Explain the usage of the max() method in Java streams.
Ans:
- Max () retrieves the maximum element from the stream based on a comparator.
- It supports both natural ordering and custom comparators for element comparison.
- This terminal operation triggers computation to determine the maximum element.
- Max () facilitates finding the largest element within the stream.
- It returns an Optional containing the maximum element or an empty Optional if the stream is empty.
56. How do you remove duplicates from a stream in Java?
Ans:
Eliminate duplicate elements using distinct() to ensure uniqueness in the stream. This operation relies on the equals() method to determine element uniqueness. Distinct () preserves the original order while creating a new stream without duplicates.Removing duplicates simplifies downstream processing and enhances efficiency. It’s a common data manipulation task for maintaining data integrity.
57. What is the purpose of the flatMapToDouble() method in Java streams?
Ans:
- flatMapToDouble() converts a stream of elements to a DoubleStream, facilitating numerical computations.
- It’s particularly useful for handling nested streams or collections.
- The method transforms each element into a DoubleStream and flattens them into a single stream.
- flatMapToDouble() streamlines the processing of numerical data, enhancing efficiency.
- It supports concise transformation and manipulation of nested structures containing numerical values.
58. How do you perform the mapping of elements in a stream in Java?
Ans:
Use map() to apply a function to each element, transforming the stream. This operation creates a new stream with elements modified according to the provided function.map() facilitates conversion between different types of custom transformations. It preserves the order of elements in the original stream during mapping. Mapping is essential for data transformation tasks in stream processing.
59. What is the purpose of the findFirst() method in Java streams?
Ans:
- findFirst() retrieves the first element of the stream, wrapped in an Optional.
- It’s a short-circuiting operation, halting after finding the first element.
- findFirst() is useful for scenarios prioritizing the first element’s retrieval.
- It ensures efficient processing by stopping once a match is found.
- When the stream is empty, findFirst() returns an empty Optional.
- Utilizing findFirst() provides a straightforward approach to accessing the first element.
60. Explain the usage of the noneMatch() method in Java streams.
Ans:
noneMatch() verifies whether no elements in the stream satisfy a given predicate. It returns true if none of the elements match the predicate; otherwise, it returns false. NoneMatch () triggers computation as a terminal operation on the stream.It’s instrumental in validating that none of the elements meet specific criteria. The method enables negative assertions on stream elements, enhancing data validation.
61. How do you convert a stream to a list in Java?
Ans:
- In Java, you can transform a stream into a list using the collect() method provided by the Stream API.
- By passing Collectors.toList() as an argument to collect(), elements are accumulated into a list.
- Example usage: List<Type> list = stream.collect(Collectors.toList());
- This operation gathers stream elements into a new list instance.
- Collect () is a terminal operation that initiates stream execution.
62. How do you convert a stream of integers to a list in Java?
Ans:
- To convert a stream of integers into a list in Java, you apply the collect() method with Collectors.toList().
- Similar to other conversions, this process involves collecting elements into a list.
- Example syntax: List<Integer> list = instream.boxed().collect(Collectors.toList());
- The integers are gathered into a list of Integer objects.
- Precede with boxed() to wrap integers into Integer objects.
63. What is the purpose of the min() method in Java streams?
Ans:
The min() method in Java streams facilitates the identification of the smallest element based on a comparator. It yields an Optional wrapping of the minimum element or an empty Option if the stream is empty. Example application: Optional<Integer> min = stream.min(Comparator.naturalOrder()); Custom comparators can be specified for defining element order.
64. Explain the usage of the flatMapToLong() method in Java streams.
Ans:
- flatMapToLong() in Java streams is employed to flatten a stream of elements into a LongStream.
- Each element undergoes mapping to a LongStream, which is then flattened into a single LongStream.
- This operation proves beneficial for efficiently transforming streams into long values.
- Usage example: LongStream longStream = stream.flatMapToLong(element -> LongStream.of(element.getId()));
65. How do you concatenate multiple streams in Java?
Ans:
To merge several streams in Java, utilize the Stream. concat() method. This function merges two streams into a new stream encompassing elements from both. Example: Stream<Type> concatenatedStream = Stream.concat(stream1, stream2); Original streams remain unchanged; a new stream containing all elements is produced. Concatenating streams is useful for combining disparate elements into a single stream.
66. What is the purpose of the toArray(IntFunction generator) method in Java streams?
Ans:
- The toArray(IntFunction generator) method in Java streams converts a stream into an array.
- The resultant array comprises elements from the stream.
- Specifying generator determines the array type.
- Example usage: Type[] array = stream.toArray(Type[]::new);
- It offers flexibility in array creation based on the desired type.
- Terminal operation: execution of stream is triggered upon invocation.
67. Explain the usage of the sorted() method in Java streams.
Ans:
- In Java streams, the sorted() method arranges the elements of the stream either based on their natural order or a custom comparator.
- It generates a new stream containing the elements sorted accordingly.
- For instance, I am invoking stream.sorted() organizes the elements in ascending order.
- When a custom comparator is specified, the method sorts the elements according to that comparator’s logic.
68. How do you filter elements in a Java stream?
Ans:
Element filtering in Java streams is accomplished through the application of the filter() method. This method accepts a predicate as input and determines whether each element should be present in the resultant stream.Elements satisfying the predicate condition are retained, while those failing it are omitted—for example, stream. Filter (x -> x > 5) eliminates elements less than or equal to 5.
69. Explain the usage of the flatMapToObj() method in Java streams.
Ans:
- The flatMapToObj() method within Java streams flattens a sequence of elements into a stream of objects.
- It expects a mapper function as its argument, mapping each stream element to a stream of objects, which are then amalgamated into a single stream.
- This method is particularly useful when dealing with streams of collections or arrays, where each initial element corresponds to multiple objects in the resultant stream.
70. How do you convert a stream of doubles to an array in Java?
Ans:
- Transforming a stream of doubles into an array in Java involves utilizing the toArray() method.
- This method converts stream elements into an array of the specified type.
- For example, stream.toArray(Double[]:: new) converts doubles into an array of Double objects.
- Alternatively, stream.toArray() can be employed to generate an array of primitive doubles.
- As a terminal operation, toArray() initiates the stream processing.
71. What is the purpose of the collect() method in Java streams?
Ans:
The collect() method assembles elements from a stream into a collection or another data structure. It allows customization through various collectors, such as toList(), toSet(), and more. This method serves as a terminal operation to transform streams into a more manageable form. It facilitates mutable reduction operations on stream elements. It is typically used in conjunction with other stream operations like map and filter.
72.Explain the usage of the mapToDouble() method in Java streams.
Ans:
mapToDouble() converts elements of a stream into double primitives. It is particularly useful with streams of numeric data. It applies a mapper function to each element, transforming it to a double value. The resulting stream is of type DoubleStream, containing the converted elements. This is a specialized mapping operation for primitive double values.mapToDouble() can be followed by other stream operations or terminal operations.
73. How do you perform mapping to a different type in a Java stream?
Ans:
- Use the map() method followed by a mapper function to map to a different type.
- This function defines how each element of the stream is transformed.
- Useful for converting objects from one type to another, like from String to Integer.
- The resulting stream contains elements of the new type based on the mapping.
- Common for data transformation in stream processing.
- Mapping to a different type enables flexibility in stream operations.
74. What is the purpose of the forEach() method in Java streams?
Ans:
- forEach() executes an action for each element of the stream.
- Similar to traditional for-each loop but for streams.
- Executes a specified action for each stream element.
- Often used for side-effect operations like printing or logging.
- Enables easy traversal and processing of stream elements.
- Helps in performing bulk operations on stream elements.
75. How do you perform filtering based on multiple conditions in a Java stream?
Ans:
Use the filter() method multiple times, each with different conditions.Each filter() call operates independently, refining the stream based on a specific condition.Chaining filter() calls apply multiple conditions to the stream. This allows for complex filtering logic based on various criteria. It is a flexible way to narrow down elements in a stream according to multiple criteria. The resulting stream contains only elements satisfying all specified conditions.
76.Explain the usage of the flatMapToInt() method in Java streams.
Ans:
flatMapToInt() flattens the stream of elements to an IntStream after applying a mapping function. It is useful with streams of collections or arrays. The mapper function is applied to each element, resulting in a stream of int values. The resulting IntStream is a flattened representation of all mapped int values. We have specialized flat-mapping operations for primitive int values.
77. How do you convert a stream of objects to a set in Java?
Ans:
- Utilize the collect() method with Collectors.toSet() to convert a stream to a set.
- Collects stream elements into a Set data structure.
- toSet() ensures that the resulting set contains unique elements.
- An efficient way to remove duplicates and obtain distinct elements.
- The resulting set maintains order from the original stream.
- Useful for operations requiring uniqueness and unordered collection.
78. What is the purpose of the map() method in Java streams?
Ans:
- Map () is an intermediate operation for transforming each element using a mapper function.
- Applies the mapper function to each element, producing a new stream with transformed elements.
- The mapper function defines the transformation logic for each element.
- Facilitates one-to-one mapping between elements of original and new streams.
- Common for data transformation and extraction in stream processing.
79. How do you sort elements in a Java stream?
Ans:
Use the sorted() method to sort stream elements. Returns new stream with elements sorted according to natural order. Custom sorting can be done by providing a Comparator to the sorted() method. Sorting can be applied before or after other stream operations. Arrange elements in ascending order by default or per specified Comparator. Enables orderly processing of stream elements based on certain criteria.
80.Explain the usage of the allMatch() method in Java streams.
Ans:
allMatch() determines if a specified predicate is satisfied by every stream element. Returns true if all elements satisfy condition; false otherwise. Predicate defines the condition against which each element is evaluated and is commonly used for validation or checking if the condition holds for all elements. Terminates stream once the non-matching element is found, ensuring efficiency. It provides a way to perform bulk checks on stream elements with a single operation.
81. How do you perform distinct operations in a Java stream?
Ans:
- To perform distinct operations, you use the distinct() method.
- It eliminates duplicate elements from the stream.
- The method returns a stream consisting of distinct elements.
- It relies on the equals() method for equality comparison.
- This operation maintains the order of elements.
- It’s commonly used to ensure uniqueness in the stream.
82. What is the purpose of the noneMatch() method in Java streams?
Ans:
- The noneMatch() method checks if no elements match a given predicate.
- It returns true if no elements satisfy the condition.
- Otherwise, it returns false.
- It’s a short-circuiting terminal operation.
- Useful for validating if no elements meet certain criteria.
- Often used for negative assertions.
83.Explain the usage of the forEachOrdered() method in Java streams.
Ans:
forEachOrdered() ensures the order of execution in a parallel stream. It guarantees that elements are processed sequentially according to their encounter order. This method is useful when order preservation is necessary. It’s similar to forEach() but maintains order. It is particularly important for side-effect operations.It enhances predictability in parallel streams.
84. How do you map elements to their lengths in a Java stream?
Ans:
Use the map() method with a function to transform elements. Pass a function that returns the length of each element. For example, stream. map(String::length) maps strings to their lengths. The resulting stream contains the lengths of elements. This technique is useful for extracting properties of elements and facilitates data transformation concisely.
85. How do you filter elements based on a predicate in a Java stream?
Ans:
- Use the filter() method along with a predicate.
- Provide a condition to determine which elements to keep.
- The predicate returns true for elements to be included.
- Retained are those elements that fulfill the predicate.
- Others are filtered out of the stream.
- This method facilitates the selective inclusion of elements.
86. What is the purpose of the range() method in Java streams?
Ans:
- Range () generates a sequential stream of integers within a specified range.
- It takes a starting value (inclusive) and an ending value (exclusive).
- The method produces integers from start to end-1.
- This operation is useful for creating streams of numeric ranges.
- Frequently employed in conjunction with additional stream operations.
- It provides a convenient way to iterate over a range of numbers.
87.Explain the usage of the limit() method in Java streams.
Ans:
limit() restricts the size of a stream to a specified number. It truncates the stream to the first N elements. Useful for limiting resource usage or controlling output size. The resulting stream contains, at most, the specified number of elements. It’s a short-circuiting intermediate operation. Facilitates efficient processing of large streams.
88. How do you perform mapping to a different type with a custom function in a Java stream?
Ans:
- Utilize the map() method with a function that converts elements to the desired type.
- Define a custom mapping function to transform elements accordingly.
- For instance, stream.map(element -> customFunction(element)) maps elements to a different type.
- The resulting stream contains elements of the new type.
- This approach enables flexible data transformation.
- It’s particularly useful for adapting stream elements to different domains.
89. Collect, what is a mutable reduction?
Ans:
In a mutable reduction, the result container can be modified during the accumulation phase. Intermediate results are mutable, allowing modifications. Mutable reduction typically uses methods like addAll to accumulate elements. This approach is useful for performance optimization and reducing memory overhead. However, proper synchronization in parallel contexts is required to avoid data corruption.
90. Why does Java require predefined API collectors to be passed?
Ans:
Predefined API collectors encapsulate common reduction operations and provide optimized implementations for various reduction tasks. Passing collectors ensure consistent and efficient reduction behavior across different streams.These collectors abstract complex reduction logic, promoting code readability and maintainability. They also offer a standardized way to perform common aggregation tasks on streams.
91. How does Java’s two-map function?
Ans:
- Java’s map() function is used to transform each element of a stream using a provided function.
- It operates on each element of the stream individually, applying the function to each element.
- The result is a new stream containing the transformed elements.
- This allows for easy conversion or manipulation of stream elements.
- It’s often used in functional programming paradigms to process collections efficiently.
92. What primitive stream classes does Java offer?
Ans:
- Java offers primitive stream classes for int, long, and double types.
- These are IntStream, LongStream, and DoubleStream, respectively.
- They provide specialized operations for handling primitive data types efficiently.
- Primitive streams avoid the overhead of boxing and unboxing associated with their object counterparts.
- They offer methods tailored for numerical operations on primitive values.
93. What does Java programming’s dot stream function entail?
Ans:
Java’s stream() function obtains a stream from a collection or array. It allows for processing elements in a collection using functional-style operations, enabling easier and more concise data manipulation. The resulting stream can be operated on using various stream operations, promoting a more functional programming approach to data processing.