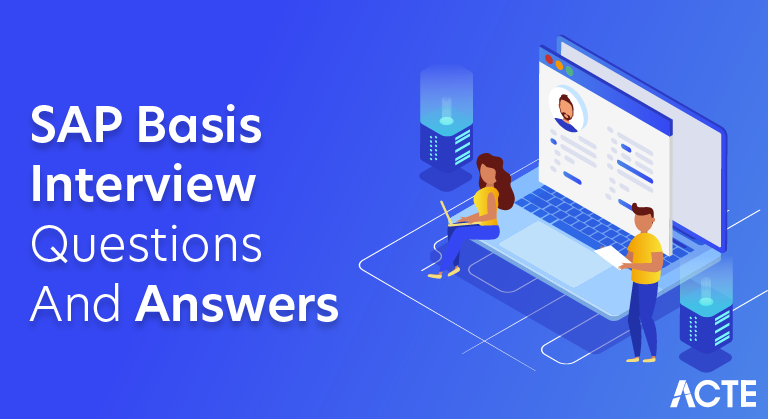
A Java architect is a professional with extensive expertise in the Java programming language and its associated technologies. They play a pivotal role in designing and implementing complex software solutions using Java-based frameworks and tools. Java architects possess a deep understanding of software architecture principles, design patterns, and best practices. They are responsible for creating scalable, high-performance, and maintainable software systems that meet the requirements and objectives of their organization or clients.
1. In Java, what is the distinction between {==} and `.equals()}?
Ans:
When comparing objects in Java, `{==}} and `.equals()} have different functions. When two references point to the same memory address, {{==}} verifies reference equality. Conversely, the `.equals()` method within the `Object` class compares the true values or contents of two objects to determine if they are equal. {.equals()} enables objects to define their sense of equality based on their internal state, whereas `{==}} compares references. Overriding the `.equals()` function is essential for classes that require specialized equality comparison.
2. Describe the foundational ideas of object-oriented programming (OOP) and how Java implements them.
Ans:
- Objects, which include both data and behaviour, are central to the idea of object-oriented programming (OOP).
- Encapsulation, inheritance, and polymorphism are important OOP concepts.
- Through classes and objects, Java implements these ideas.
- Defining an object’s structure and behaviour, a class acts as a blueprint for construction.
- By using methods to expose just the necessary features, encapsulation conceals an object’s internal state.
- Inheritance facilitates code reuse by enabling classes to inherit characteristics and attributes from other ones.
- Code design is made more flexible and extensible by polymorphism, which allows objects to be viewed as instances of their superclass.
3. Explain Java’s inheritance notion along with an example.
Ans:
A class (subclass) in Java can inherit traits and behaviours from a superclass (parent class) through inheritance. In addition to encouraging code reuse, this creates a “is-a” link between classes. Take the superclass {Animal}, for instance, which has attributes and operations that all animals share. Subclasses that inherit from `Animal}, such as `Dog} and `Cat}, can be created, and their unique properties and methods added. Subclasses can provide specific functionality while retaining common traits by overriding methods from the superclass.
4. What are Java’s access modifiers, and how do they impact the visibility of class members?
Ans:
- The four access modifiers offered by Java are default (no modifier), `public`, `private`, and `protected`.
- The visibility of class members, both inside and outside of the class hierarchy, is controlled by these modifications.
- Members marked as {public} can be accessed from any class. {private} members can only be accessed by other members of the same class.
- Subclasses and other members of the same package can access {protected} members.
- The access within the same package is restricted by default access (no modifier).
- By limiting a class member’s accessibility, access modifiers support information hiding and contribute to encapsulation.
5. Explain what polymorphism is and how Java does it.
Ans:
Java’s polymorphism makes it possible to consider objects as instances of their superclass, which promotes extensibility and flexibility in code design. It alludes to inheritance’s capacity to regard several classes as instances of the same class. The act of a subclass providing a particular implementation of a method described in its superclass is known as method overriding. A superclass reference can point to an object of its subclass through polymorphism, and the runtime determination of the proper method implementation is based on the actual object type.
6. Describe the distinction between Java’s abstract classes and interfaces.
Ans:
- Although they differ greatly, Java’s abstract classes and interfaces function as models from which additional classes might be derived.
- Concrete and abstract methods can both be found in abstract classes; subclasses must override abstract methods since they are declared without an implementation.
- Instance variables are also possible for them.
- In contrast, instance variables cannot be present in interfaces; instead, they can only express abstract constants and methods.
- Although a class can extend more than one abstract class, it can implement multiple interfaces.
- Interfaces create contracts that classes can implement; abstract classes are used to define a common base for subclasses and to reuse code.
7. How does Java’s exception handling function? Describe the finally-catch-try blocks.
Ans:
Developers may handle runtime failures gracefully thanks to Java’s exception-handling features. The code that may throw an exception is enclosed in the `try` block. Control is transferred to the matching `catch` block that matches the type of exception thrown in the event that an exception arises inside the `try` block. You can chain together many {catch` blocks to handle various kinds of exceptions. If included, the `finally` block is used for cleanup operations such as resource closure and is run whether or not an exception is thrown. Exception handling, which gracefully handles unforeseen mistakes, increases the robustness of Java programs.
8. What is the use case for the Java `final` keyword, and what is its purpose?
Ans:
- In Java, the `final` keyword is used to make a class immutable, prevent method overriding, and specify constants.
- When used to variables, it signifies that once a variable is initialized, its value cannot be altered.
- {final} stops subclasses from overriding the method when it comes to methods.
- When applied to classes, it signifies that the class is final in its inheritance hierarchy and cannot be subclassed.
- This allows the compiler to inline constant values, which simplifies optimization, guarantees security, and encourages code stability.
9. What kinds of Java nested classes are there?
Ans:
- Java supports four different nested class types: local classes, anonymous classes, non-static nested classes (also known as inner classes), and static nested classes.
- It is possible to instantiate static nested classes without requiring an instance of the outer class as they are specified inside another class.
- Inner classes, which are non-static nested classes, have access to the members of the outer class and are linked to an instance of the outer class.
- Local variables are accessible to and defined by local classes, which are found inside method or scope blocks.
- Anonymous classes are used for one-time tasks like event processing and are concurrently declared and instantiated.
10. What are the differences between Java’s method overloading and overriding?
Ans:
Aspect | Method Overloading | Method Overriding |
---|---|---|
Definition | Defining multiple methods with the same name but different parameters within a single class. | Providing a specific implementation for a method that is already defined in the superclass within a subclass. |
Inheritance | Not related to inheritance. | Specifically related to inheritance. |
Class involvement | Methods are defined within the same class. | Involves methods defined in both superclass and subclass. |
Signature | Method signatures (name and parameters) must be different. | Method signatures (name and parameters) must be the same. |
11. What are the differences between Java’s method overloading and overriding?
Ans:
- Java’s term for defining many methods with the same name but distinct argument lists within a single class is called method overloading.
- Different argument types, numbers, or orders are required for overloading methods.
- The compiler uses the arguments given during the method invocation to identify which overloaded method to invoke.
- Method Overriding is the process by which a subclass offers a particular implementation of a method that is present in its superclass but has not yet been specified.
- The method signatures (name and parameters) in both the superclass and subclass have to match.
- The JVM chooses which implementation to run when a method is called on a subclass object based on the runtime object type.
12. Describe the function of Java’s `static` keyword.
Ans:
When declaring members (variables and methods) that are part of the class itself rather than any particular instance, Java uses the `static` keyword. All instances of the class have the same static members, which are accessible immediately by using the class name without requiring the creation of an object. In contrast to static variables, which can only be called with a class instance present, static methods maintain their values across class instances. Static members are frequently used in counters, constants, and utility methods.
13. Explain the importance of the Java Memory Model in multi-threaded programming.
Ans:
- When accessing shared variables, threads interact with memory according to the Java Memory Model (JMM).
- It guarantees accurate data visibility and synchronization between threads in a multi-threaded context.
- JMM defines the rules that determine which threads can see changes made to shared variables, avoiding data races and guaranteeing consistent behaviour across many platforms and architectures.
- It is essential to comprehend and follow the JMM guidelines while creating Java concurrent programs to ensure correctness and thread safety.
14. Which Java collections are there, and when would you use each one?
Ans:
The `java.util` package in Java offers a number of collection classes, such as ArrayList, LinkedList, HashSet, TreeSet, HashMap, TreeMap, and so on.
- ArrayList: This type of list is used when it is necessary to frequently access and traverse elements. It offers quick random access, but insertion and deletion operations take longer.
- LinkedList: Offers quick insertion and deletion but slower random access, making it ideal for frequent insertions and deletions, particularly at the start or middle of the list.
- HashSet: Suitable for verifying uniqueness and confirming element presence, it stores unique elements without duplication.
- TreeSet: Enables effective element retrieval in sorted order while preserving items in that order.
- HashMap: Offers quick value retrieval based on keys and key-value pairings.
15. What are the many kinds of garbage collectors in Java, and how does garbage collection operate there?
Ans:
- Serial Garbage Collector
- Parallel Garbage Collector
- CMS (Concurrent Mark-Sweep) Garbage Collector
- G1 (Garbage-First) Garbage Collector
16. Describe the distinction between Java’s LinkedList and ArrayList.
Ans:
LinkedList: This is a doubly-linked list that is implemented with a reference to the previous and next elements in each element. Ideal for regular additions and deletions, particularly at the start or middle of the list. Offers faster random access but more efficient insertion and deletion.
ArrayList: This is a dynamic array that expands on its own when new elements are added. Though insertion and deletion operations are slower than with LinkedList, this structure is appropriate for frequent access and element traversal, and it provides quick random access.
17. Describe a ConcurrentHashMap and explain your use case.
Ans:
ConcurrentHashMap is a Java thread-safe implementation of the Map interface that enables several threads to access data concurrently without requiring external synchronization.
Thanks to the segmentation of the underlying data structure, it provides higher concurrency performance than a hashtable or synchronized hash map. This allows several threads to operate on separate segments concurrently. When several threads must read and write to a shared map simultaneously, use ConcurrentHashMap since it offers effective, thread-safe operations without requiring external synchronization.18. Explain the function of Java’s `synchronized` term and its substitutes.
Ans:
To ensure that only one thread may access a synchronized block or function at a time, use Java’s `synchronized` keyword to build synchronized blocks or methods. In systems with several threads, it helps to preserve data consistency by blocking concurrent access to shared resources. The `Java.util.concurrent.locks} package’s locks, such as ReentrantLock, can be used in place of synchronized blocks to provide greater flexibility and control over locking mechanisms.
19. What are the advantages and disadvantages of utilizing the serialization method that comes with Java?
Ans:
Automatically serializes object graphs, making the process of transferring and storing Java objects easier. Objects can be serialized on one platform and deserialized on another thanks to platform-independent serialization formats. The Java platform has built-in support, which makes it simple to use. Reflection-based serialization and deserialization processes result in performance overhead.
20. How is multi-threading supported by Java, and what synchronization techniques are available?
Ans:
The `java.lang.Thread` class and the `java.util.concurrent` package allow Java to handle multithreading.
Java synchronization methods consist of:
- Granting exclusive access to shared resources by employing synchronized blocks or procedures.
- Locks, like ReentrantLock, are used from the `Java.util.concurrent.locks` package for more precise synchronization control.
- Concurrent HashMap and CopyOnWriteArrayList are two examples of thread-safe data structures offered by the `java.util.concurrent` package. They allow concurrent access without the requirement for external synchronization.
21. How is Java bytecode executed by the Java Virtual Machine (JVM), and what is it?
Ans:
- Java bytecode serves as a conduit between the Java source code and the underlying platform’s machine code.
- During compilation, the Java compiler transforms Java source code into bytecode.
- This bytecode can run on any computer with a suitable Java Virtual Machine (JVM) because it is platform-independent.
- The JVM is in charge of executing Java bytecode.
- It either interprets or compiles the class bytecode into native machine code after loading it and verifying its integrity.
- In addition to managing memory, collecting trash, and resolving exceptions during program execution, the Java Virtual Machine (JVM) also creates a consistent environment across many platforms for Java programs.
- Additionally, Just-In-Time (JIT) compilation is used to convert frequently executed bytecode into native machine code for faster runtime.
22. Give an explanation and an example of the Java reflection concept.
Ans:
Runtime analysis and modification of class fields, constructors, methods, and metadata are made possible via Java’s reflection functionality. It provides a way to call methods, access fields programmatically, and dynamically and introspectively retrieve class data. For example, you can use reflection to call methods, instantiate objects, load classes dynamically, and access fields without knowing their compile-time identities. Frameworks, libraries, and tools that need to evaluate or change classes during runtime will find this adaptability to be quite useful. For instance, object-relational mapping, aspect-oriented programming, and dependency injection make extensive use of reflection in frameworks such as Spring and Hibernate.
23. How are annotations used in Java, and what is their purpose?
Ans:
- Java’s reflection feature enables runtime examination and modification of class fields, constructors, methods, and metadata.
- It offers a mechanism to programmatically access fields, invoke methods, and introspect and dynamically retrieve class information.
- For instance, you can dynamically load classes, instantiate objects, call methods, and access fields using reflection without having to know their compile-time identifiers.
- This adaptability is especially helpful in frameworks, libraries, and tools that need to analyze or modify classes at runtime.
- For example, reflection is heavily used in frameworks like Spring and Hibernate for object-relational mapping, aspect-oriented programming, and dependency injection.
24. Explain the distinctions between Java’s checked and unchecked exceptions.
Ans:
In Java, there are two sorts of exceptions: checked exceptions and unchecked exceptions, each with its handling procedures. When the compiler examines an exception during compilation, it means that it needs to be handled by a try-catch block or specified using the ‘throws’ clause in the method signature. Usually, they indicate mistakes that can be recovered from during program execution, like file input/output problems or problems with network connections. Conversely, unchecked exceptions can happen during runtime and are exceptions that are not verified during compilation. They typically represent programming faults or unusual conditions outside the programmer’s control, such as null pointer exceptions or arithmetic exceptions, and they don’t need to be explicitly handled or declared.
25. What are the various memory locations in the JVM, and how does Java manage memory?
Ans:
- The Java Virtual Machine (JVM) divides memory into many sections to make memory management easier.
- It also handles garbage collection and memory allocation for Java programs.
- The heap, stack, method area (PermGen in previous versions), native method stack, and PC register are some examples of these memory regions.
- Method invocations and local variables are stored on the stack, and objects and instance variables are stored on the heap.
- Static variables, bytecode, and class metadata are kept in the method area.
- The address of the presently running instruction is stored in the PC register, and native method calls are made using the native method stack.
- Garbage collection, a feature of Java that regularly finds and recovers memory used by objects that are no longer referenced, is used for automatic memory management.
26. Describe the fundamentals of design patterns and give instances of popular Java design patterns.
Ans:
Reusable answers to typical software design issues that arise during the software application development process are known as design patterns. They offer an organized method for developing software by compiling best practices and tested fixes for common issues. In Java, the Singleton, Factory, Observer, Strategy, Builder, and Adapter patterns are popular design patterns. The Singleton pattern, for instance, guarantees that a class has a single instance and offers a global point of access to it. Although subclasses might modify the kind of objects that are created, the Factory pattern offers an interface for object creation. When an object’s state changes, the Observer pattern creates a one-to-many dependency between it and all of its dependents, notifying and updating them all.
27. How does Java’s `Comparable} interface relate to sorting objects, and what is its purpose?
Ans:
- In Java, a natural ordering of objects is defined using the Comparable interface.
- In order to specify how instances of the class should be compared to one another, classes that implement the Comparable interface include a method called compareTo().
- The return values of this method indicate whether the current object is less than, equal to, or greater than the provided object, respectively: a negative integer, zero, or a positive integer.
- When sorting items in collections like TreeSet or using sorting methods like Collections.
- Sort (), Comparable is frequently employed.
- Java sorting procedures are made easier by implementing Comparable, which allows objects to be compared according to their inherent ordering.
28. What are the features of a Singleton pattern, and how would you implement it in Java?
Ans:
A creational design pattern called the singleton pattern makes sure a class has just one instance and offers a global point of access to it. When only one object is required to coordinate systemic actions, it is helpful. A static variable to store the one instance, a static method to grant access to the single instance, and a private constructor to prevent instantiation are the characteristics of a singleton pattern. This guarantees that during the lifecycle of the application, there will only be one instance of the class. To offer centralized logging or configuration management capabilities, for instance, a Logger class or a Configuration class can be constructed as a Singleton.
29. Explain the distinction between Java’s publisher-subscriber and observer design patterns.
Ans:
- Behavioural design patterns like Publisher-Subscriber (Pub-Sub) and Observer both let components of a system communicate with each other.
- The Publisher-Subscriber paradigm enables several subscribers to receive notifications from a single publisher, thereby decoupling components.
- Through the use of an event bus or message broker, publishers and subscribers are loosely connected, allowing for dynamic subscription and event handling.
- By creating a one-to-many dependency between objects, the Observer pattern, on the other hand, notifies and updates all of its dependents (observers) when one object’s state changes.
- When a subject’s status changes, observers who have registered with the subject are notified.
- Although Publisher-Subscriber and Observer emphasize loose coupling dynamic event processing and object consistency between states, both styles help components communicate with each other.
30. How do you use enumerations, and what is the use case for the Java `enum` keyword?
Ans:
Special types in Java that are made up of a predetermined collection of constants are called enumerations. An enumeration type is defined with the enum keyword, and every constant value is defined as an instance of the enumeration type. Compared to utilizing integer constants or texts, enumerations offer type safety, readability, and clarity. Frequently, they are employed to depict fixed collections of constants such as weeks, months, or object states. Because Java has built-in support for enums, developers may construct and use enumerated types with ease thanks to the enum keyword. Enum can be used to define constants for switch statements, represent options or choices in a program, and enhance the readability of code by giving constants meaningful names.
31. Describe how object-oriented design’s SOLID principles relate to Java development.
Ans:
- Java’s Single Responsibility Principle (SRP) states that a class should only have one change request.
- Stated differently, a class ought to concentrate on mastering a single task.
- Java encourages the usage of interfaces and abstract classes, which permit classes to be open for extension but closed for modification.
- This promotes the Open/Closed Principle (OCP).
- Liskov Substitution Principle (LSP): This principle guarantees that objects of a superclass can be substituted with objects of its subclasses without compromising the program’s correctness.
- Java interfaces and inheritance mechanisms facilitate adherence to this principle.
- The Interface Segregation Principle (ISP) states that methods can be divided into more focused and smaller interfaces by using Java interfaces, which keeps customers from becoming dependent on methods they are not using.
32. In a multi-threaded Java program, how would you manage concurrent access to shared resources?
Ans:
Java has the `synchronized` keyword for synchronized blocks or methods, which guarantees that a single thread can only use the shared resource concurrently. Compared to built-in synchronization, Java’s `Lock` interface and its implementations, such as `ReentrantLock`, offer greater flexibility and fine-grained control over locking methods. Atomic variables are thread-safe operations on shared variables that don’t require explicit locking. They are provided by Java’s `java.util.concurrent.atomic` package and include `AtomicInteger`, `AtomicLong`, and other similar variables.
33. Explain the distinction between Java string comparison methods `==} and `.equals()}?
Ans:
- {==}: Compares two objects’ memory addresses. It determines if two references make use of the same memory address.
- It compares the values of primitive kinds in this scenario.
- {.equals()}: This function compares two objects’ actual contents.
- Many classes override this function to compare their internal state, but the `Object` class’s default implementation compares memory addresses.
34. How do the Java `StringBuilder` and `StringBuffer` classes differ from `String`, and what is their purpose?
Ans:
A fixed string consisting of a series of characters. Its content cannot be altered after it is created. Character sequence that can be changed. Without generating new objects, it enables dynamic string alteration, such as character addition, replacement, or insertion. Thread-safe and synchronized, `StringBuffer` is comparable to `StringBuilder`. In single-threaded contexts, it performs less efficiently than {StringBuilder}; but, in multi-threaded environments, it guarantees data integrity.
35. What are lambda expressions, and how does Java support functional programming?
Ans:
- The extensive usage of lambda expressions, functional interfaces (interfaces with a single abstract method), and utility methods in `Java.
- Util. function’ package, such as map, filter, reduce, etc., supports functional programming in Java.
- Because of this, code may be written more clearly and expressively, emphasizing immutability and behaviour composition.
36. Explain the function of Java multithreading java. Util. Concurrent package?
Ans:
Java’s `java.util.concurrent` package offers sophisticated concurrency tools to facilitate multi-threaded programming. It provides high-level building blocks such as concurrent collections, atomic variables, thread pools, barriers, and semaphores as synchronizers. These tools handle thread execution, synchronization, and coordination, making it easier and safer for developers to design concurrent programs.
37. What are the benefits and drawbacks of Java’s use of synchronized blocks?
Ans:
- Benefits: Java’s synchronized blocks minimize race situations and data corruption by limiting the number of threads that can access a crucial portion of code at once.
- They offer a straightforward method for controlling concurrent access to shared resources and are simple to implement and comprehend.
- Cons: On the other hand, excessive synchronization may result in deadlock situations and performance problems owing to contention and thread conflict.
38. Describe Java’s inner class idea and its applications.
Ans:
A class can be defined inside another class in Java thanks to the inner class notion. Because the inner class may access all members of the outer class, including private members, this method makes encapsulation easier. Inner classes are frequently used to bundle similar functionality into a single class, implement callbacks, handle events, and produce more legible and maintainable code.
39. Explain the concepts of Test-Driven Development (TDD) and the Java code that embodies them.
Ans:
- Software development using Test-Driven Development (TDD) involves writing tests before writing any code.
- In Java, TDD entails creating unit tests that specify the intended behavior of the code by utilizing frameworks such as JUnit or TestNG.
- After that, developers write the bare minimum of code needed to pass the tests.
- As requirements drive the design, this iterative process helps guarantee code correctness, maintainability, and test coverage.
40. What is dependency injection, and how is it applied in Spring and other Java frameworks?
Ans:
A design approach known as dependency injection (DI) allows a class’s dependencies to be obtained outside rather than being generated internally. Inversion of control containers is used to implement DI in Spring and other Java frameworks. Loose coupling, simpler unit testing, and enhanced modularization are made possible by these containers, which control object generation and dependency injection depending on the configuration. Better concern separation is made possible by DI, which also encourages code reuse and maintainability.
41. What are the various methods for supporting asynchronous programming in Java?
Ans:
- Various methods for supporting asynchronous programming in Java include Callbacks, Futures, Promises, completeFutures, and Reactive Streams.
- These mechanisms enable non-blocking execution, enhancing application performance and responsiveness.
42. Describe the fundamentals of Java’s aspect-oriented programming (AOP) system.
Ans:
Aspects of a program that impact numerous components, like logging, security, or transaction management, can be modularized thanks to Java’s aspect-oriented programming (AOP) architecture. The fundamental ideas of AOP are as follows: join points are places in the program’s execution when a particular aspect’s code might be applied; aspects are modules that include cross-cutting concerns; and advise is the action that an aspect takes at a join point. Advice comes in several forms, including before, after, and around advice, which indicate the order in which the aspect’s code should run in relation to the join point.
43. What distinguishes Java frameworks like Spring’s XML-based setup from annotations?
Ans:
- Java frameworks like Spring offer both XML-based configuration and annotations for setup.
- XML configuration provides a more lengthy and explicit approach, while annotations offer a more concise and declarative method, enhancing code readability and reducing configuration overhead.
44. Explain the purpose and appropriate usage cases for Java’s `@Override} annotation.
Ans:
When a method in a subclass overrides a method in its superclass, Java uses the @Override annotation to indicate this. Its primary purpose is to guarantee that the subclass method has the right signature, assisting in the prevention of mistakes like incorrect method names or argument types. Additionally, this annotation allows for compile-time verification, which lowers the possibility of runtime problems by having the compiler report an error if a method fails to correctly override a superclass method.
45. What Java offers concurrent tools, and how does it facilitate parallel programming?
Ans:
- Java provides concurrent tools such as Executors, Threads, Concurrent Collections, and the Fork/Join framework, which facilitate parallel programming and concurrent task execution.
- These tools simplify the development of multi-threaded applications, improving performance and scalability.
46. Describe the fundamentals of microservices architecture and how Java frameworks make it easier to put into practice.
Ans:
Microservices architecture decomposes applications into smaller, independent services that can be developed, deployed, and scaled independently. Java frameworks like Spring Boot and Dropwizard streamline micro services development by providing features such as dependency injection, embedded servers, and configuration management.
47. What is the difference between an architectural and a design pattern, and what is a design pattern?
Ans:
- An architectural pattern defines the overall structure and organization of a software system, while a design pattern addresses specific design problems within that structure.
- A design pattern is a reusable solution to a commonly occurring problem in software design, promoting code reusability and maintainability.
48. Explain Java’s `this` keyword’s function and how it affects method and constructor calls.
Ans:
This Java keyword is frequently used to distinguish between parameters and instance variables when they have the same name. It alludes to the active instance of the class. Use this, for example, in a procedure or constructor.If variableName is used without this, it refers to the class instance variable rather than the method or constructor parameter.
49. What are the distinctions between a Java `HashMap` and a `HashTable}?
Ans:
- Java’s HashMap and HashTable are both implementations of the Map interface, but they have key distinctions.
- HashMap is not synchronized, making it more efficient for non-thread-safe operations, while HashTable is synchronized, ensuring thread safety but potentially impacting performance.
50. Describe domain-driven design (DDD) and how it is used in Java projects.
Ans:
A technique for creating complex software systems that concentrates on the logic and core domain is called domain-driven design, or DDD. In order to build a shared understanding of the domain and utilize that understanding to design software that effectively reflects the business needs, it places a strong emphasis on cooperation between domain experts and developers.
51. What are event listeners, and how is Java support for event-driven programming implemented?
Ans:
- Event listeners in Java are objects that wait for specific events to occur and then execute predefined actions.
- Java supports event-driven programming through interfaces and classes such as ActionListener and EventHandler.
- These interfaces define methods that are invoked when events like button clicks or mouse movements occur, enabling developers to create responsive and interactive applications.
52. Explain the function of transactions and how they are managed in Java EE applications.
Ans:
Transactions in Java EE applications ensure that multiple database operations are executed as a single atomic unit. They maintain data integrity and consistency by either committing all changes or rolling back if an error occurs. Java EE manages transactions through APIs like Java Transaction API (JTA) and Java Persistence API (JPA), which provide annotations and configuration options to control transaction behaviour.
53. In Java programs, what are the recommended strategies for handling exceptions?
Ans:
In Java programs, best practices for handling exceptions include:
- Using try-catch blocks, too.
- Throwinggracefully handles specific exception exceptions to indicate errors that cannot be handled locally.
- Logging exceptions for debugging purposes.
Additionally, employing exception hierarchies, such as catching broader exceptions before specific ones, can improve code readability and maintainability.
54. Describe the fundamentals of RESTful web services and how Java implements them.
Ans:
RESTful web services follow the principles of Representational State Transfer (REST) architecture, utilizing HTTP methods for resource manipulation and employing stateless communication. Java implements RESTful web services through frameworks like JAX-RS (Java API for RESTful Web Services), which simplify the creation and deployment of RESTful endpoints using annotations and POJOs (Plain Old Java Objects).
55. Explain the advantages of Spring’s annotation-based setup over its XML-based counterpart.
Ans:
- Spring’s annotation-based setup offers advantages over XML-based configuration by reducing verbosity, improving code readability, and promoting convention over configuration.
- Annotations enable developers to define dependencies, configurations, and behaviours directly within Java classes, resulting in more concise and maintainable code.
56. What are the benefits of utilizing Spring and other dependency injection frameworks in Java applications?
Ans:
Utilizing Spring and other dependency injection frameworks in Java applications provides benefits such as increased modularity, easier unit testing, and reduced coupling between components. Dependency injection enables components to be loosely coupled, promoting reusability and scalability while facilitating the management of object dependencies and lifecycle.
57. Considering memory and CPU use, how would you maximize the performance of a Java application?
Ans:
- To maximize a Java application’s performance considering memory and CPU usage, developers can employ techniques such as optimizing data structures and algorithms, minimizing object creation and garbage collection overhead, and leveraging concurrency and parallelism.
- Additionally, profiling tools like JProfiler and VisualVM can help identify performance bottlenecks and areas for optimization.
58. What distinguishes RESTful web services from SOAP web services, and under what circumstances would you prefer one over the other?
Ans:
RESTful web services differ from SOAP web services in their architectural style, communication protocol, and message format. RESTful services use lightweight JSON or XML payloads over HTTP, emphasizing simplicity, scalability, and flexibility, whereas SOAP services rely on XML-based messaging with a predefined contract (WSDL). Developers may prefer RESTful services for their simplicity and compatibility with web standards, while SOAP services are favoured for their robustness and support for complex messaging requirements.
59. How does Java support application localization and internationalization?
Ans:
- Java supports application localization and internationalization through features like ResourceBundle for managing locale-specific resources, MessageFormat for formatting localized messages, and Locale for representing user preferences.
- These features enable developers to create applications that adapt to different languages, regions, and cultural conventions without code modifications.
60. Describe the fundamentals of reactive programming and how Java frameworks use it.
Ans:
- Reactive programming is an asynchronous programming paradigm that emphasizes event-driven and non-blocking operations.
- It enables scalable and responsive applications.
- Java frameworks like Reactor and RxJava implement reactive programming principles by providing APIs for composing and processing asynchronous data streams using functional constructs such as Observables and Monads.
61. Explain the fundamentals of developing cloud-native applications and how they affect Java architects.
Ans:
Cloud-native applications are built to run in cloud environments, leveraging scalability and elasticity. They utilize containerization, microservices architecture, and automated deployment. For Java architects, understanding cloud-native principles means adapting Java applications to be more modular, scalable, and resilient. It involves designing for distributed systems, utilizing cloud services, and adopting DevOps practices.
62. In what ways could a Java web application be made secure?
Ans:
- Employ HTTPS to encrypt data transmitted between clients and servers.
- Utilize secure authentication mechanisms such as OAuth, JWT, or OAuth 2.0.
- Implement input validation to prevent SQL injection, XSS, and other common attacks.
- Apply proper session management techniques and avoid storing sensitive data in cookies.
- Regularly update dependencies and frameworks to patch known vulnerabilities.
63. Describe the fundamentals of OAuth 2.0 and how Java apps use it.
Ans:
- OAuth 2.0 is an authorization framework allowing third-party applications limited access to a user’s resources.
- Java apps use OAuth 2.0 to authenticate and authorize users through access tokens.
- It facilitates secure, delegated access without sharing credentials.
- Java apps typically integrate OAuth 2.0 via libraries like Spring Security OAuth or Apache Oltu.
- OAuth 2.0 involves various components, such as the authorization server, resource server, and client.
64. Explain the concepts of Command-Query Responsibility Segregation (CQRS) and event sourcing as they apply to Java applications.
Ans:
Command Query Responsibility Segregation (CQRS) separates read and write operations in applications. Event Sourcing captures all changes to an application’s state as a sequence of events. In Java applications, CQRS and event sourcing are often implemented together to achieve scalability and maintainability. CQRS allows for optimized read and write operations tailored to specific use cases.
65. When would you employ each of the many data caching techniques available for Java applications?
Ans:
- Employ in-memory caching for frequently accessed data to improve performance.
- Use distributed caching systems like Redis or Hazelcast for scalability and fault tolerance.
- Employ HTTP caching mechanisms such as ETags and Cache-Control headers for web applications.
- Implement database caching to reduce database load and latency.
- Utilize caching libraries like Ehcache or Caffeine for transparent caching.
66. Describe the fundamentals of resilience and fault tolerance in distributed Java systems.
Ans:
- Resilience ensures systems can withstand and recover from failures gracefully.
- Fault tolerance involves designing systems to continue operating in the presence of faults.
- In distributed Java systems, resilience is achieved through techniques like circuit breakers, retries, and fallbacks.
- Fault tolerance involves redundancy, isolation, and graceful degradation.
- Java frameworks like Hystrix and resilience4j provide tools for building resilient systems.
67. In what way would you create a scalable architecture for an ecosystem of microservices running on Java?
Ans:
- Implement a modular architecture based on microservices principles.
- Utilize container orchestration platforms like Kubernetes for scalability and management.
- Design services to be stateless and independent to facilitate horizontal scaling.
- Use asynchronous communication patterns and event-driven architectures.
- Implement distributed tracing and monitoring for performance optimization and scalability assessment.
68. Explain how Java microservices architectures use service registration and discovery.
Ans:
- Use service registry and discovery patterns to facilitate communication between microservices.
- Services register themselves with a central registry upon startup.
- Client services dynamically discover and connect to available instances of required services.
- Java microservices architectures commonly utilize service registries like Netflix Eureka or HashiCorp Consul.
- Service discovery enables flexible and resilient communication between microservices.
69. In Java applications, what are the recommended techniques for versioning RESTful APIs?
Ans:
- Use URI versioning by including the version number in the API endpoint.
- Utilize custom request headers for versioning to keep URIs clean and expressive.
- Employ content negotiation to serve different API versions based on client preferences.
- Consider backward compatibility to ensure existing clients can still interact with newer API versions.
- Implement versioning strategies consistent with RESTful principles and industry best practices.
70. What libraries and frameworks are available for Java that allow Reactive Stream Processing?
Ans:
Reactive stream processing is made possible for Java by a number of tools and frameworks, which let programs handle asynchronous data streams with non-blocking backpressure. One of the main components of Spring WebFlux is Project Reactor, a widely-used library that supports reactive programming with an emphasis on reactive streams. It was created by the Spring team. Another well-liked package that provides comprehensive assistance for creating event-based and asynchronous programming with observable sequences is RxJava. The Akka toolkit’s Akka Streams feature offers a robust and expressive approach for creating backpressure-aware reactive streaming applications.
71. Describe the ideas behind Java project continuous deployment (Cd) and continuous integration (CI).
Ans:
In order to expedite the software delivery process, current Java project development relies heavily on Continuous Deployment (CD) and Continuous Integration (CI). Code changes from several contributors are regularly merged into a shared repository as part of continuous integration, which also includes automated builds and tests to find integration problems early. This guarantees code quality and seamless integration of new code with the current codebase. By automatically delivering each code update to production or staging environments that passes automated tests, continuous deployment expands on this approach. This procedure shortens the time between development and production by guaranteeing that consumers receive new features, fixes, and upgrades promptly and consistently.
72. Explain the fundamentals of orchestration and containerization in Java microservices deployments.
Ans:
- Orchestration manages the deployment, scaling, and lifecycle of microservices.
- Containerization packages microservices and their depe
- ndencies for easy deployment.
- Tools like Kubernetes and Docker Swarm facilitate orchestration and containerization in Java microservices.
73. How many database connections are managed in Java programs using various strategies?
Ans:
- Connection Pooling
- Direct Connection
- Connection Pooling Libraries
- Connection Pooling Configuration
- Database Connection Limits
- Load Balancers
74. In a distributed Java program, how would you guarantee data integrity and consistency?
Ans:
Ensuring data consistency and integrity in a distributed Java program requires multiple approaches. To prevent partial updates, start by putting atomic procedures into place to guarantee that data changes are either successfully completed or not at all. To ensure data integrity across various services, use distributed transactions with technologies like SAGA patterns or Two-Phase Commit (2PC). It is possible to trace changes as a sequence of events with event sourcing, which enables reliable state reconstruction. By determining if data has changed prior to committing transactions, optimistic concurrency control assists in managing concurrent updates.
75. Explain the fundamentals of GraphQL and how Java applications use it.
Ans:
- Single Endpoint: Unlike REST APIs, which employ numerous endpoints, this type of API offers a single endpoint for queries and changes.
- Flexible Queries: Clients define precisely what information they require, including related or nested data in a single request.
- Strongly-Typed Schema: Provides client-server consistency by specifying the kinds and structures of data that are accessible through the API.
- Mutations: Gives clients the ability to change server data.
- Subscriptions: Allows clients to receive notifications when data changes in real-time. Supports real-time updates.
76. In Java applications, what are the benefits of a microservices architecture over a monolithic architecture?
Ans:
- GraphQL is a query language for APIs, enabling clients to request specific data.
- Java applications use GraphQL to efficiently fetch data from multiple sources with a single query.
- It offers flexibility and reduces data over-fetching or under-fetching.
77. Describe the ideas behind bounded contexts and domain-driven design (DDD) in Java programs.
Ans:
- Bounded contexts define clear boundaries within a domain, encapsulating specific functionality.
- DDD emphasizes modelling a domain’s concepts and relationships in code.
- Bounded contexts prevent domain complexity and enable better collaboration between teams.
78. How would you use Java messaging technologies to construct a message-driven architecture?
Ans:
Using Java messaging technologies, you may build a message-driven architecture by first utilizing Java Message Service (JMS), which offers a stable API for messaging exchanges between distributed components. Start by building your system using message brokers to manage message delivery and routing, like Apache ActiveMQ or RabbitMQ. Message producers and consumers are defined as follows: producers create messages and transmit them to queues or topics, and consumers receive and process them asynchronously.
79. Explain how event-driven architecture works and how Java applications use it.
Ans:
- Event-driven architecture allows components to communicate through events.
- Events represent significant state changes or occurrences in the system.
- Java applications use event-driven architecture to build loosely coupled, scalable systems.
80. In Java microservices, what are the distinctions between synchronous and asynchronous communication?
Ans:
- Synchronous communication blocks until a response is received, which is suitable for immediate responses.
- Asynchronous communication decouples components, allowing them to process requests independently.
- Java microservices use both communication styles based on use cases and performance requirements.
81. Describe the fundamentals of reactive microservices and how Java frameworks are used to implement them.
Ans:
- Utilize asynchronous communication for efficient handling of concurrent requests.
- Java frameworks such as Spring Boot and Vert. x are commonly employed.
- They offer features like non-blocking I/O and event-driven architecture.
- Enable responsiveness, resilience, and elasticity in distributed systems.
- Leverage reactive streams or message brokers to manage data streams.
82. Explain the fundamentals of the API gateway pattern and how Java microservices architectures use it.
Ans:
With the API Gateway paradigm, all client requests in a microservices architecture are handled by a single entry point. In addition to aggregating responses and resolving cross-cutting issues like load balancing, authentication, and logging, this gateway also sends requests to the relevant microservices. By offering a consistent API and eliminating the need for clients to directly engage with numerous services, the API Gateway streamlines client interactions in Java microservices systems. It may apply security features like rate limitation and token validation, centralizes the administration of API requests, and routes queries.
83. How would a distributed Java microservices ecosystem handle data consistency?
Ans:
- Utilize distributed transaction management or eventual consistency.
- Techniques such as the Saga pattern or two-phase commit ensure consistency.
- Employ event sourcing, CQRS, distributed databases, or data replication mechanisms.
- Implement idempotent operations and compensating transactions.
- Effective monitoring and logging are crucial for issue detection and resolution.
84. Describe the ultimate consistency principles and how distributed Java systems are affected by them.
Ans:
The ultimate consistency principles in distributed systems are centered on making sure that, in spite of network partitions and failures, all nodes in the system eventually come to the same conclusion. These guidelines are essential for preserving data coherence and integrity in distributed Java systems that span several nodes. A fundamental tenet of the system is eventual consistency, which states that all updates will spread to all nodes and eventually converge on the same state if given enough time.
85. What are the various approaches to Java microservices service orchestration and choreography?
Ans:
- Orchestration centralizes control logic, often implemented using Spring Cloud.
- Choreography decentralizes control logic, enabling independent service reactions.
- Event-driven architectures with messaging systems like Kafka facilitate choreography.
- Service mesh technologies offer advanced features for service communication management.
- Orchestration and choreography are often combined based on system requirements.
86. Explain the fundamentals of Java microservices’ circuit breakers and fallback systems.
Ans:
Circuit breakers and fallback mechanisms are essential parts of Java microservices architecture that improve fault tolerance and resilience. Circuit breakers are made to stop the breakdown of one service from affecting other services in a domino effect. They function by keeping an eye on service requests and identifying errors. The circuit breaker “trips,” preventing more requests to the failed service and giving it time to recover, when a threshold of failures is reached. The circuit breaker may be in a “half-open” condition, which permits a limited number of requests to see if the service has recovered, or it may be in a “open” state, which prohibits calls to the failing service.
87. How do you design fault-tolerant architectures for Java applications in cloud environments?
Ans:
In cloud contexts, designing fault-tolerant architectures for Java applications entails putting many solutions into practice to guarantee resilience and reduce downtime. To isolate faults and enable individual components to fail without affecting the system as a whole, start by implementing microservices architecture. To disperse traffic and dynamically scale resources in response to demand, employ load balancing and auto-scaling. This will help to ensure that the system can withstand errors and continue to function. Use circuit breakers to identify and isolate failed services so that recovery is possible and cascading failures are avoided.
88. Explain the principles of reactive programming and their application in building scalable Java applications.
Ans:
- Emphasize asynchronous and non-blocking operations for scalability.
- Reactor and Akka provide support for reactive programming in Java.
- Reactive streams enable efficient data stream handling with backpressure.
- Foster responsiveness, resilience, and elasticity in Java applications.
- Handle high concurrency and I/O-bound operations effectively.
89. What are the key security considerations for microservice communication in Java-based distributed architectures?
Ans:
Securing microservice communication in distributed architectures based on Java necessitates taking numerous important factors into account. First, authentication which frequently makes use of protocols like OAuth 2.0 or JWT is essential to guarantee that every microservice confirms the identity of users or services making requests. Equally crucial to authorization are fine-grained access controls, which are usually implemented via role-based or attribute-based access control to guarantee that services can only access resources that they are authorized.
90. How can performance in large-scale Java applications be optimized?
Ans:
- Identify and mitigate bottlenecks for performance optimization.
- Employ techniques like caching, database optimization, and asynchronous processing.
- Java profiling tools such as VisualVM and JProfiler aid in performance analysis.
- Distributed caching solutions like Redis or Memcached improve data access.
- Conduct load testing and monitor performance for scalability assessment.