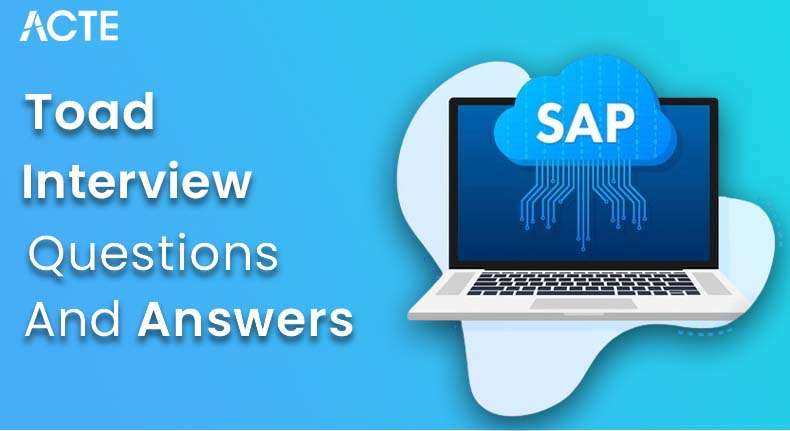
Java 8 is a major release of the Java programming language that introduced significant features aimed at improving developer productivity and code efficiency. Key enhancements include the introduction of lambda expressions for functional programming, the Stream API for processing sequences of elements, and the new Date and Time API for better date manipulation. These features promote cleaner, more concise code and enable developers to write applications that are more scalable and maintainable.
1. What are some of the key features introduced in Java 8?
Ans:
Java 8 introduces several major features, such as lambda expressions for cleaner code, the Streams API for functional-style Processing of sequences, and the Optional class for safe access to null values. It also introduces default methods in the interface, allowing them to provide method implementations without breaking existing code. A completely new Date and Time API has also been introduced, with which dates and times may be handled more holistically.
2. What Is a Lambda Expression?
Ans:
In Java 8, it is a short form of expressing an instance of a functional interface consisting of only a single abstract method. Lambda expressions can pass behaviour as parameters to functions. As a result, the code is more understandable and tidy. It is particularly useful in the Streams API and event handling context. Lambda expressions in Java enable functional programming techniques, thus making programmers more flexible and expressive in coding.
3. What would be the most significant benefits of Java 8?
Ans:
- Java 8 is especially helpful in eliminating boilerplate code, thereby enhancing readability and maintainability.
- The Streams API simplifies parallel Processing on collections, greatly improves performance, and yields more flexible and reusable code that applies functional programming.
- A new date and time API makes date manipulations easier. Furthermore, a much better representation of handling null using an Optional class has been introduced.
4. What Is the Syntax of a Lambda Expression?
Ans:
The lambda expression syntax is mostly in one of the two forms (parameters) -> expression. If there are more statements, then it is (parameters) -> { // statements }. Therefore, a simple lambda expression can be like the above example (int x, int y) -> x + y. This way, developers can create a behaviour that facilitates functional interface usage. These concise expressions enhance code readability and promote a more functional programming style in Java.
5. What are the Advantages and Disadvantages of Java 8 Features?
Ans:
Description | Advantages | Disadvantages |
---|---|---|
Definition | Complete SAP software installation | Single running copy of SAP system |
Conciseness | Reduces boilerplate code, making programs cleaner and easier to maintain. | However, it may lead to less readability for complex lambda expressions. |
Parallel Processing | Simplifies the implementation of parallel operations, improving performance on large datasets. | However, due to overhead, it is not always efficient for small datasets. |
Functional Programming Support | Encourages a functional programming style, enhancing code reusability and flexibility. | However, it requires developers to adapt to a new programming paradigm, which may have a learning curve. |
Rich APIs | Introduce classes like Optional and a new Date/Time API, improving usability and safety. | Additional complexity due to introducing new APIs and concepts. |
Default Methods | Allow interfaces to evolve without breaking existing implementations, ensuring backward compatibility. | However, when multiple interfaces provide default methods, this can lead to ambiguity. |
6. What is the intention behind the use of the @FunctionalInterface annotation?
Ans:
It is a declaration that an interface is intended to be functional, meaning it should have only one abstract method. This enhances code readableness and also helps catch bugs at compile time, as the compiler will throw an error when the interface, which is defined without intent, contains more than one abstract method. Hence, it acts as a guideline that hints to developers to declare the purpose of the interface explicitly.
7. What Does It Mean by Functional Interfaces in Java 8?
Ans:
A functional interface in Java 8 is defined as an interface with a single abstract method. This characteristic allows functional interfaces to be used as the target types for lambda expressions and method references. The most common examples are consumer, callable, and runnable interfaces. Some usage of functional interfaces supports the Functional Programming Paradigm and enables the possibility of flexibility in passing behaviour.
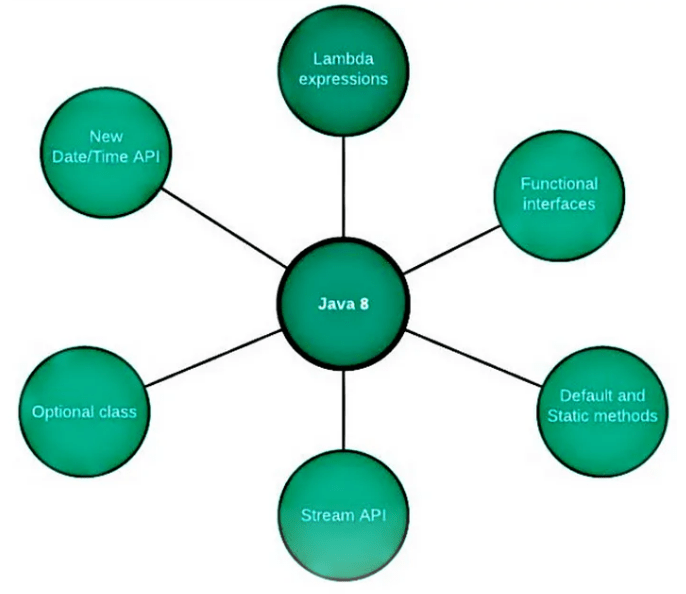
8. What is the application of String::valueOf expression in Java 8?
Ans:
- The expression String::valueOf is a method reference that refers to the class String’s valueOf method.
- It can convert almost any type to its String representation, making our code more readable and less boilerplate.
- As an example of method reference in functional programming, we take advantage of the fact that this method name can be used to form a shorthand for type conversion rather than invoke the method name itself.
- This is another way that method references make operations more than Java 8.
9. Difference between Predicates and Functions in Java 8.
Ans:
- A Predicate is a functional interface that, in Java 8, accepts an argument and yields a boolean result. It is thus well suited for testing conditions, such as whether a given string is empty.
- A Function is a functional interface that accepts one argument and returns a result of any type. It’s useful for transformations, such as converting a string to its length.
- Both are used for different purposes in functional programming, making code much more flexible.
10. What are the default methods in Java 8?
Ans:
- Default methods in Java 8 are defined as methods that can now be given an implementation within an interface.
- They make interface changes easier by allowing new methods to be added without forcing implementations on existing ones.
- They are very useful for maintaining backward compatibility-an existing class could still implement the interface without change to the class.
- The syntax for a default method is the void methodName() { // method body }, making it easy to include default behaviour.
11. What are the main classes of the Java 8 API for date and time?
Ans:
The main classes of Java. Time API package for date and time belongs to it. The main courses include LocalDate, Which represents a date without a time; LocalTime, Which is a time without a date; LocalDateTime, Both of the foregoing; ZonedDateTime, Which contains time zone information and duration. Period This class represents an amount of time. This new API addresses many shortcomings of the old Java. Util. Date and Java. Util.Calendar classes, thus making it more comprehensive and user-friendly for handling dates and times.
12. Can users Create a Custom Functional Interface in Java 8?
Ans:
Creating a custom functional interface in Java 8 is possible. A functional interface contains a single abstract method and is instantiable via lambda expressions or method references. Creating a custom functional interface may involve annotating it with @FunctionalInterface to declare its intent clearly. This Annotation may also be followed when using it through adherence to the rules of functional interfaces.
13. What does Method Reference in Java 8 mean?
Ans:
This is a shorthand to refer directly to a method of a class or instance using a lambda expression. Java 8 makes the code more readable and concise by avoiding the explicit invocation of the Method. There are three categories of method references:
- Referring to a static method.
- Referring to an instance method of a specific object.
- Refers to an instance method of any object whose type is known.
14. How to Design Specific Custom Functional Interfaces in Java 8?
Ans:
- Declare a functional interface in Java 8 by defining an interface consisting of only one abstract Method.
- Optionally, can also annotate the interface with the @FunctionalInterface keyword to emphasize its purpose and introduce a compiler check.
- Custom functional interfaces represent a proper way of encapsulating some functionality, making code more modular and reusable.
15. What Are the Differences Between Intermediate and Terminal Stream Operations?
Ans:
- A Java 8 stream intermediate operations transform a stream into another stream.
- They are lazily executed—that is, they only execute once they invoke a terminal operation. Intermediate operations include filter, map, and sort.
- Terminal operations yield a result or side effect that concludes the pipeline. Common terminal operations are collect, forEach, and reduce.
- The difference is that the intermediate operation is chainable and can potentially be reused, whereas the terminal operation completes the processing of the Stream.
16. What is the least obtrusive way to print the current date and time using the new APIs in Java 8?
Ans:
- LocalDateTime is the simplest way to print out the current date and time using Java 8’s new APIs.
- Now() returns the current date and time, so we can print that directly; as this is not meaningful in its own right, we can immediately see the new, user-friendly Date and Time API of Java 8.
- The simplest way to print out the current date and time using Java 8’s new APIs is using LocalDateTime.now(), which returns the current date and time and can be printed directly.
- This approach highlights the new Date and Time API’s user-friendly nature, making date and time manipulation more intuitive than in previous versions.
17. What Issues Were Resolved with the New Java 8 Date and Time API?
Ans:
The new Date and Time API in Java 8 resolves several issues noticed with the previous Java. Util.Date and Java. Util.Calendar classes. The major improvements are immutability for enhancing thread safety and clearer API design that distinguishes between the concepts of date and time. In addition, the class ZonedDateTime makes handling time zones easier, whereas classes Duration and Period make computations of dates and times intuitively easy. This new API brings an extremely efficient and user-friendly date and time manipulation technique.
18. What Are PermGen and Metaspace in Java 8?
Ans:
PermGen (Permanent Generation) and Metaspace are the memory areas for storing class metadata in Java 8. PermGen was a memory area in the earlier Java versions that was used to store definitions for classes and associated metadata; such a memory space might, if it filled up, increase the chances of seeing OutOfMemoryError. Metaspace is the counterpart of PermGen in Java 8, and its allocations could be made outside of the heap since they are dynamically scalable according to growing requirements.
19. Explain Intermediate and Terminal Operations in Java 8.
Ans:
- Intermediate operations are transformations which return another stream. Thus, they enable the chaining of operations.
- They run lazily, which means that execution does not take place immediately; instead, it happens only when a terminal operation is invoked.
- Terminal operations produce some result or side effect, thus marking the termination of the stream pipeline. These operations are among the likes of collect, which trigger the stream processing.
- The key difference is that intermediate operations themselves can be chained, but stream processing is considered complete once a terminal operation is invoked.
20. When Is the Stream API Used in Java 8?
Ans:
- The Stream API in Java 8 is used to process a sequence of data-like collections of data in a functional and declarative way.
- It is very useful for performing complex operations like filtering, mapping, and reduction in data with a concise syntax.
- The Stream API provides benefits in leveraging parallel processing when trying to make performance improvements on larger datasets or searching for cleaner and more readable code.
- This API also permits various operations over collections without forcing modification to the original data structure, which supports immutability.
21. What does the Stream API in Java 8 represent?
Ans:
The significance of the Stream API in Java 8 is that it makes it easier to do operations on collections in a functional style. That is, through this approach, developers achieve these very complex data manipulation tasks like filtering, mapping, and reduction in a concise and readable form. The declarative style of programming improves the code’s quality because it is clearer. Further, it supports parallel processing, thus making performance for large datasets better.
22. How does the Optional class help in handling null values?
Ans:
Java 8 introduces an optional class that provides for the object container, which may or may not hold a value that can help avoid NullPointerExceptions. Using Optional allows developers to express the explicit absence of a value instead of null. Then, it provides methods like isPresent(), ifPresent(), or orElse() to handle values safely. It encourages better programming practices and the readability of code. Moreover, it relies less on defensive programming techniques; thus, it is cleaner and more robust.
23. What’s the Difference Between map() and flatMap() in Streams?
Ans:
- The method map() in streams takes an object from a stream and transforms it into another object. This Method generates a new stream of the same size as the previous Stream but with elements transformed using the given function.
- If the transformation results in multiple elements for every one of the input elements, then instead of the map() method, flatMap() combines the resulting streams into one Stream.
- For example, may use map() to convert a list of names to uppercase and can apply the conversion of a list of lists into a single list of elements using the flatMap().
- The only difference between these methods is the Method in which these methods treat the resulting Stream’s structure.
24. What is the forEach() Method in Java 8 Used for?
Ans:
This Method is used for executing an action for each element of a stream. It’s a terminal operation because it causes the stream pipeline to be realized. Developers use each () so much for side effects, such as printing elements or modifying external states. This Method has a lambda expression or method reference describing what needs to be done. While good for iteration over elements, it should only sometimes be used for further stream processing.
25. How Does the Method collect() Work in Streams?
Ans:
This is a terminal operation; the Method collects () in streams and transforms the elements of a stream into another kind of data, such as a list, a set, or a map. It accepts a Collector, which determines an accumulation strategy. Some of the commonly used collectors are Collectors.tolist(), Collectors.toSet(), and Collectors.toMap(). This function allows the accumulation of elements from a stream in a required form, thus facilitating the process of data transformation. The collect() Method is predominantly used for converting the data in stream form to collections that can be used.
26. What Do We Use the filter() Method for in Streams?
Ans:
The filter() Method in streams keeps only those elements that pass some criteria. It takes a predicate as an argument and defines which condition those elements will need to meet for the output. The Method returns another stream with the elements that satisfy the given condition. An application, for example, can filter a number list, keeping only even numbers. The filter() Method handles data processing better in the case of selective inclusion of elements based on predefined rules.
27. How Would Parallel Streams Impact the Performance in Java 8?
Ans:
- Parallel streams in Java 8 offer a great performance advantage by allowing data to be processed in parallel.
- When a stream is executed in parallel, it splits the job into several threads and uses multi-core processors, which have a greater chance of completing the data processing quicker.
- However, the nature of the task and the overhead of managing parallelism affect performance improvement. Parallel streams make it relatively easy to improve performance without using low-level thread management.
28. What are Method References, and How Do They Work?
Ans:
Java 8 represents method references as shorthand form expressions for invoking methods without requiring an explicit invocation. They are a cleaner, more concise way of referring to methods rather than lambda expressions. Method references come in four flavours: static methods, instance methods of a definite object, instance methods of an arbitrary object, and constructor references. They are also very common contexts in which streams work, where one can easily pass around behaviour.
29. How Does the reduce() Method Work in a Stream?
Ans:
The reduce() Method in a stream is a terminal operation that aggregates the elements of the Stream into a single value. It takes a binary operator as an argument, defining how two elements are to be combined. The Method can also take an initial value to begin the accumulation process. For example, it can be used to compute the sum of a list of numbers. The reduce() Method is powerful for aggregation over stream elements, functionally supporting complex event processing.
30. What distinguishes noneMatch(), allMatch(), and anyMatch()?
Ans:
anyMatch(), allMatch(), and noneMatch() methods are used for checking conditions on stream elements. anyMatch() returns true if any elements in the given collection match the specified Predicate and partially satisfy conditions. allMatch() returns whether all the elements in the collection satisfy the Predicate and thus return true only if all the elements in the collection satisfy the condition. The inverse of allMatch() is nonmatch (), returning true if no elements are matched by the given Predicate, meaning the given condition is unsatisfied.
31. Which of the Following are Types of Method References in Java 8?
Ans:
- In Java 8, four types of method references are available: static method reference, instance method reference on a particular object, instance method reference on an arbitrary object of a given type, and constructor reference.
- A static method reference is a method defined within a class that gets invoked on that class. An instance method reference of a specific object is a method of a particular instance.
- An instance method reference of any object enables a method to be invoked on any instance of a particular type. Constructor references instantiate objects of a class based on the class’s constructor.
32. How Do Streams Get Created from Collections?
Ans:
- In Java 8, streams can be accessed from collections using the Stream () declared on collection classes such as List, Set, and Map.
- Returns a sequential stream consisting of all elements in this collection. The parallel Stream () Method can delegate collections to parallel streams.
- This ability helps function-style operations on data structures. Therefore, the developer can exploit streams’ ability in data manipulation.
33. What Is Comparator Interface and Its Use in Java 8?
Ans:
- Comparators can be used for sorting or ordering when objects of a particular class are to be compared.
- The comparator interface provides methods to compare objects. This can be done with lambda expressions, making it concise and readable.
- Apart from classical comparison methods, Java 8 has offered static methods—comparing(), comparing (), and then Comparing ()—for creating more complex comparators.
- The Comparator interface also helps sort collections and streams and thus enriches the flexibility in data arrangement.
34. How does the Sorting mechanism work with streams?
Ans:
The sorted() Method can give a sorting mechanism on streams, which calls on a stream to order its elements in a specified order. The sorted() Method considers the sorting element by default through natural ordering. Still, in addition to that, a custom Comparator may also be supplied to define specific logic for ordering. A sorted stream returns a new stream with elements in the desired order. The original Stream remains unchanged. This makes for very elegant and efficient sorts in functional programming style.
35. What are the advantages of Using Optional Over Traditional Null Checks
Ans:
The advantages of using the Optional class in Java 8 over traditional null checks are It presents the possibility of absence with clarity, which improves coding and shows intent more clearly. This avoids the chances of getting a null-pointer exception-null reference is very commonly associated with. Optional has several methods; one is present, then Present and or Else to handle the values safely and concisely.
36. How Is the New Date and Time API Different from the Old Java? Util.Date Class?
Ans:
The new Date and Time API in Java 8 significantly differs from the old Java. Util.Date class in the following ways: One, it’s immutable; that means date and time objects cannot be changed after creation, making it another thread-safe offering. Java’s built-in type java. Util. The date is mutable and can behave erratically in concurrent environments. The new API clearly distinguishes between the date and time concepts, with classes like LocalDate, LocalTime, and LocalDateTime.
37. What is LocalDate in Java 8 Class?
Ans:
- In Java 8, LocalDate represented a date without its time element or time zone. It is part of a newly designed Date and Time API.
- LocalDate supports all operations, such as subtracting or adding days, months, or years, making date-related calculations very simple.
- This class is extremely useful in applications that work heavily with dates, without regard for time or time zones, in scheduling or managing tasks or events, for instance.
- Overall, the LocalDate class greatly contributes to more clarity and functionality of work over dates.
38. How Time Zones Can Be Managed Using the New Date and Time API?
Ans:
- The new Date and Time API allows for the control of time zones through the use of ZonedDateTime and ZoneId classes.
- ZoneId is the class that provides a time zone. With ZoneId, for the first time in Java, time zone information can be specified explicitly.
- In a ZonedDateTime, can associate a date-time with a particular time zone; this makes time calculations across different regions quite accurate.
- Methods to switch time zones are also available, which makes things pretty simple when daylight saving changes are involved.
39. What is the ZonedDateTime Class in Java 8?
Ans:
- A class in Java 8 called ZonedDateTime represents a date and time along with the attached time zone. ZonedDateTime makes date-time management more precise and readable in applications.
- The class combines locality and timezone information to describe a local date time, which allows such date-time calculations based on different regions.
- ZonedDateTime is immutable and supports several operations, like adding or subtracting time and converting easily to another time zone.
- This class is very useful for applications that need knowledge of time zones, such as scheduling and event planning.
40. How is the Duration Class Used in Java 8?
Ans:
The Duration class in Java 8 represents a quantity of time in terms of hours, minutes, or seconds. This allows developers to express the interval between two-time objects, simplifying straightforward computation and comparison. Durations can be created by using factory methods such as ofDays(), ofHours(), and between(), which computes the interval between two instances of Temporal. The class will find broader applications in any form of time arithmetic, such as adding or subtracting some time from a date or time object.
41. What Are the Local Dates vs LocalDateTime?
Ans:
Local Date and LocalDateTime are two classes of Java 8 API representing date and time. The LocalDate class is a date class that does not consist of any time or time zone. LocalDate is mainly used for applications that only demand a date instead of a date with time information. LocalDateTime can represent both dates and times; therefore, it can show more detail in temporal expressions. Whereas the class LocalDate operates on year, month, and day only, LocalDateTime contains time-including hours, minutes, and even seconds and nanoseconds.
42. How to use the Period Class to Computer Date Differences.
Ans:
The Java 8 Period class represents periods, such as years, months, or days. It offers a straightforward way to compute the difference between two dates through the between() Method. In this class, if one works with two LocalDate instances, they receive the duration as a Period object and can query its individual components, such as the number of years or months, etc. This class simplifies date arithmetic, making clear and concise calculations of date differences possible.
43. What is a Stream Pipeline?
Ans:
A Stream Pipeline comprises a source, a few intermediary operations, and a terminal operation in Java 8. The source may be a collection, an array, or an I/O channel from which a data stream is produced. Intermediate operations like filter(), map(), and sorted() transform the Stream into another stream and can be chained together. A terminal operation could be collect(), forEach(), or reduce(), which subsequently triggers the computation of the Stream and returns a result.
44. What does lazy Evaluation mean for streams?
Ans:
- Lazy Evaluation for streams means that every intermediate operation applied is evaluated once some terminal operation is invoked.
- Lazy Evaluation means that map() or filter() transformations are executed only when the final result is needed.
- Thirdly, lazy Evaluation enables the processing of potentially infinite streams since data can be produced and then consumed on the fly.
- This reduces the number of stream operations since unrequired computations are avoided, hence enabling better performance.
45. How Can Collect () Be Applied to Transforming Data from Streams?
Ans:
- The collect() method is a terminal operation in streams that transforms the elements in a stream into another data structure, such as a list, set, or map.
- A Collector, which defines the accumulation strategy, may be used for flexible data transformation.
- Collectors.toList() collects elements from a stream into a List, while Collectors.toMap() collects elements from a stream into a map.
- This Method aggregates and customizes the result, which can be of exceptional utility when processing and aggregating data from streams.
46. What are primitive streams, and how do they differ from object streams?
Ans:
- Primitive streams in Java 8 are called specialized stream types because they work with primitive data types. The streams are created based on IntStream, LongStream, and DoubleStream.
- Such streams tend to optimize performance because they avoid overhead encountered during the boxing and unboxing of primitive types into their respective wrapper classes.
- Primitive streams provide methods natively from their type, operations, statistical computation can be applied directly to primitive value optimizes performance and efficiency with large amounts of primitive data.
47. What is the Significance of the @FunctionalInterface Annotation in Defining Interfaces?
Ans:
The @FunctionalInterface Annotation of Java 8 is an intent that an interface is a functional interface that contains exactly one abstract Method. It is a form of documentation that indicates the purpose of the interface and enhances code readability. The compiler checks to ensure that the interface meets the rules the functional interface imposesthe functional interface imposes. If an interface that carries this Annotation has more than one abstract Method, it throws a compilation error.
48. How does the Java 8 Release improve the performance of lambda expressions?
Ans:
The Java 8 release improves lambda expression performance in several ways, such as invocation and memory management. The most significant optimization is the invocation of the invokedynamic instruction. This Method invokes a much more efficient lambda because it generates optimized bytecode at runtime rather than depending on the heavyweight anonymous inner classes, which are time-consuming. Further, the JVM optimizes lambda expressions at runtime and depending on usage context, resulting in long-term efficiency.
49. What Are the Common Functional Interfaces Available in Java 8?
Ans:
The Java. Util. Function package in Java 8 provides a set of common functional interfaces that assist with functional programming. Among the most interesting are Predicate, a boolean-valued function of one argument; Function, a function that takes one argument and delivers a result; Consumer, an operation that takes exactly one input argument and returns no result; and Supplier, a supplier of results. These are the interfaces on which lambda expressions and method references are built.
50. What Is BiFunction in Java 8?
Ans:
- The BiFunction interface in Java 8 represents a function that takes two arguments and returns a single result. It holds a method named apply() that accepts two parameters and returns an output.
- This interface is most useful when we must perform a computation over two inputs, such as adding two numbers or joining two strings.
- BiFunction can also produce more interesting behaviours with other functional interfaces. By combining BiFunction with methods like map or reduce, developers can create powerful data transformations.
51. How Does the Stream API Process Huge Amounts of Data Efficiently?
Ans:
- Large datasets can be processed using the Stream API, accommodate huge amounts of data with lazy Evaluation and optimized processing.
- Lazy Evaluation means that operations on a stream are executed at runtime only when a terminal operation is applied to the Stream. This minimizes overhead and allows for efficient processing even of infinite streams.
- Parallel processing is also available in the Stream API multiple threads running on multi-core processors create workloads which can be distributed. This parallelization enhances the performance of the code by handling large datasets.
52. What is the Role of the Spliterator Interface in Stream Processing?
Ans:
- The Spliterator interface plays an important role in stream processing by providing efficient traversal and partitioning for elements in a data source.
- The trySplit() Method allows a Spliterator to obtain another Spliterator for some portion of the data to enable parallelism.
- This interface also provides methods to estimate the size of the remaining elements and determines whether the Spliterator can be traversed.
- It further enhances the performance of overall operations besides providing direct access to data and parallel execution of stream operations.
53. How to Implement a Custom Collector in Java 8?
Ans:
A custom collector in Java 8 can be implemented by creating a class that implements the Collector interface. The Collector interface consists of four primary collection stages: supplier, accumulator, combiner, and finisher. The supplier creates a new mutable result container, the accumulator adds elements to the container, the combiner merges two containers, and the finisher transforms the result to the desired type. Besides, using the Collector.of() Method, one may define one’s Collector in a very compact form.
54. What Is the CompletableFuture Class in Java 8?
Ans:
CompletableFuture class for Java 8 can represent a future result of an asynchronous computation. Such class allows developers to write non-blocking code, which then executes in parallel and improves the responsiveness of any application, supporting chaining several asynchronous operations together: thenApply(), then accept (), then combine (). This class also provides features for handling exceptions and the manual completion of functions. This allows more control over the execution workflow of an asynchronous process. In totality,
55. How CompletableFuture Supports Asynchronous Programming?
Ans:
CompletableFuture supports asynchronous programming through the option for non-blocking execution regarding the task itself and multiple operations chaining. Methods like supplyAsync() allow developers to start a computation in another thread but do not block the main thread. CompletableFuture also supports combining multiple futures, using allOf() and anyOf() methods to wait on several tasks. In addition, it allows proper exception handling with methods like exceptionally() and handle().
56. What’s the main difference between Future and CompletableFuture?
Ans:
- The main differences between Future and CompletableFuture are functionalities and flexibility. Future has a much simpler interface and represents the result of an asynchronous computation.
- However, it does not conveniently support the composition of multiple tasks or handle exceptions. CompletableFuture supports chaining or composing multiple asynchronous tasks, so more complex workflows can be easily handled.
- CompletableFuture also provides exception-handling methods and supports completion stages, providing greater control over task execution.
57. How can streams be used to perform batch processing?
Ans:
- Streams may be used for batch processing by processing large collections of data functionally, allowing transformations and aggregations to be composed concisely.
- Using streams and their methods like filter(), map(), and collect(), developers can draw upon data sets for easy manipulation and summarization. Batch operations with streams support the application of some collection operations without explicit loops, improving code readability.
- Parallel streams can also be used to concurrently process batches, which would greatly improve performance if an application contains large datasets.
58. What Is the Use of Static Methods in Interfaces in Java 8?
Ans:
- In Java 8, static methods in interfaces were introduced, so utility methods can now be called on the interface itself without necessarily requiring a concrete implementing class.
- This improves code organization since functions related to each other are grouped in the interface. Static methods can provide a default implementation of operations likely to prove common for many instantiations of that interface.
- They encourage better encapsulation of functionality specific to the interface, leading to cleaner code structures. Generally, static methods enhance the expressiveness and usefulness of interfaces in Java.
59. How do Default Methods Support the Maintenance of Backward Compatibility of Java Interfaces?
Ans:
In Java, an interface offers support for backward compatibility through its built-in Method and introduces new techniques to interfaces without any error in existing implementations. This means new default methods can be added to an interface without forcing classes that implement that interface to provide an implementation of the new Method. The gradual evolution of interfaces makes it easier to add enhancements while maintaining backward compatibility with older code.
60. What are the advantages of New Stream API and traditional iteration?
Ans:
The most important advantage of using the new Stream API is improving readability, expressiveness, and performance against the traditional iteration method. Stream API helps enable the functional programming style, making it easier for developers to write data processing tasks concisely and declaratively. It reduces boilerplate code, which usually boils down to loops and conditional statements.
61. How Can Lambda Expression Implement Better Readability and Maintainability in Code?
Ans:
Lambda expression enables improving the readability and maintainability of code because it represents functional interfaces in a compact form. It avoids using wordy, anonymous inner classes, which minimizes the code’s complexity and makes it easier to understand. The developers can clearly express behaviour as parameters by using a lambda expression, which promotes flexibility in code. Because of that, the boilerplate is reduced, and the intent of the code is much clearer so that readers can comprehend the functionality more rapidly.
62. What Is the Syntax to Define a Method Reference?
Ans:
- The syntax of reference to a method declaration is the class or object name followed by the double colon (::) operator and then the method name.
- The syntax to reference a static method is ClassName::staticMethodName. For the instance method of a specific object, the syntax is instance::instanceMethodName.
- For instance, the Method of an arbitrary object of a specific type is ClassName::instanceMethodName.
- This concise syntax enhances code readability while reducing verbosity and allowing one to see how a method reference is related to its context.
63. How do default methods get introduced into a class?
Ans:
- Default methods are defined in an interface by simply declaring the Method. Interface implemented classes can directly inherit the default behavior without the need for an override using methods declared with the default keyword in their declaration.
- By allowing interfaces to have default methods, classes implementing the interfaces can opt to use the default implementation provided by the interface or instead use a local override.
- This gives flexibility in the evolution of interfaces over time that does not affect existing implementations. Default methods result in better code reuse and less boilerplate in the implementing classes.
64. What is the Optional.empty() Method For?
Ans:
- The Optional. The empty () Method is significant because it returns an empty instance of the Optional class, which represents the absence of any value.
- This helps developers deal with cases where the value is not found and minimizes the chances of a NullPointerException.
- If the code described no value using Optional. Empty () would make the code clearer. This is particularly helpful when returning an Optional from a method with no appropriate result available.
65. How Does Java 8 Handle Exceptions in Lambda Expressions?
Ans:
- Java 8 does not have built-in exception handling for lambda expressions but rather has ways around it, and those developers can implement it themselves.
- One way is that the lambda expression encloses a try-catch block, where checked exceptions within the expression are handled.
- Another way is to declare a specific functional interface that allows for the throwing of exceptions, which could then take checked exceptions if exceptions are thrown from lambda expressions.
- In such a manner, lambda expressions can be robust by properly handling exceptions.
66. What are Suppliers and Consumers in Java 8?
Ans:
A supplier in Java 8 is a functional interface that encapsulates results. A supplier provides information without requiring any arguments in input form. It has one Method, get(), which returns an object. The Consumer is a functional interface specifying an operation that takes an input argument and returns nothing. The accept() Method of the Consumer processes the given input. This implies that the concerns get separated. It supplies the data but doesn’t process the data-providing methods; it concentrates on processing by the Consumer.
67. What Are the Disadvantages When Using Lambda Expressions?
Ans:
The disadvantages of lambda expressions are that they cannot access non-final local variables and cannot throw checked exceptions directly. Moreover, lambdas are applied only in the context of functional interfaces, so they cannot replace regular methods or constructors. Furthermore, debugging the lambda expressions is sometimes even more problematic than for regular methods because stack traces do not point to the source of an error by necessity. Furthermore, overuse of lambdas will lead to very complex code that would be nearly impossible to read or maintain.
68. How Do the New Time API Classes Relieve Thread Safety Issues?
Ans:
The Java 8 new Time API classes, such as LocalDate, LocalTime, and LocalDateTime, are based on immutable constructs, thus assuaging thread-safety issues. Immutable objects can never be changed once produced; therefore, problems of concurrent access and modification are deleted. In this design, the representation of date and time is consistent in numerous threads, making mistakes less likely to occur. Additionally, the API distinguishes concerns with dates from concerns with times and thus simplifies calculations and manipulations.
69. What is an Instant class in Java 8?
Ans:
- The Instant class in Java 8 represents a point in time measured in seconds and nanoseconds since the epoch (January 1, 1970).
- The class offers a simple way of dealing with timestamps in machine-readable format. It supports adding and subtracting times or comparing two instances to establish a relationship for the purpose of order.
- It is especially useful for applications that require a long time tick for such operations as logging and event handling.
70. How Are Lambda Expressions Used in Event Handling?
Ans:
- An event listener can be defined more concisely with the help of lambda expressions.
- Rather than attempting to implement an entire listener interface of often verbosely named methods, developers can use a lambda expression to specify event handler behaviour.
- An example might be specifying a button’s action listener in terms of a lambda directly stating what should happen when the button is clicked-more readable and maintainable than boilerplate code.
- It is more elastic because one can easily change the behavior of event handlers without having to write new classes. Overall, lambda expressions streamline and improve event-driven programming.
71. What Does the @Override Annotation Do with Functional Interfaces?
Ans:
Java’s @Override Annotation uses the following notation to declare that a method is intended to override a method declared within a superclass or an interface. In the context of functional interfaces, this will clarify that a lambda expression or a method reference is implementing the abstract Method of the functional interface. @, Override is used within the code, and it will reflect that a method has been overridden. It also supports compile-time verification; therefore, the method signature might avoid potential errors if not matched.
72. How does the Stream API Enhance Filtering and Mapping Operations?
Ans:
The Java Stream API makes it possible to process filtering and mapping operations on collections more fluently and expressively than ever before. The filter() Method is used for filtering. Using this Method, a developer can specify the criteria of elements that are to be included in the resulting Stream. With the map() method, mapping or transforming every aspect of the Stream into a new form is possible. That results in a declarative style that makes code more readable and understandable about its intent.
73. What are the advantages of Immutable Data Structures in Java 8
Ans:
There are several advantages to using immutable data structures in Java 8, including enhanced thread safety and decreasing complexity when managing their states. Immutable objects cannot be changed once created, so there is no chance of discovering the class of problems called concurrent modification in a multi-threaded environment. This predictability makes reasoning about code behaviours easier and less buggy. In addition, immutability improves performance in caching and reusing instances.
74. Why Does Stream.of() Method Matter?
Ans:
- The Stream.of() Method is essential because, among the methods used to initiate a stream, it makes initiating a stream quite easy.
- In this regard, the Method enables passing numerous input values, such as an array and a single value. It returns a sequential stream.
- The flexibility of Stream. Of () allows the developers to build streams directly without working against collections. It simplifies cases where one needs to process fewer items or small datasets using the Stream API.
75. How Can the flatMap() Method Simplify Nested Collections?
Ans:
- The flatMap() method reduces nested collections to a single stream of elements, eliminating the need for multiple iterations.
- When processing a stream of collections, flatMap() merges the elements of each collection into the resulting Stream, allowing developers to work intuitively with these structures.
- FlatMap() eliminates the need to work with deep collections while keeping boilerplate code to a minimum and improving readability.
- It will prove helpful when operations are supposed to be applied to each element of multiple collections.
76. What is the role of GroupingBy Collector in Java 8.
Ans:
The grouping collector in Java 8 groups elements in a stream according to the criterion provided, and the result is like a Map, where the keys are criteria, and the values are collections of items. An approach like this delivers a powerful way of organizing data according to properties or attributes, making it easier to analyze then and process groups of related data. The grouping collector can also be composed with other collectors, providing even more operations such as counting or summing within groups.
77. What Does the toMap() Collector Function Do?
Ans:
In Java 8, to collect elements of a stream into a Map, would be using a toMap() collector. Two key parameters are essential a key mapper function that maps the keys and a value mapper function that returns appropriate values. A third parameter is optional but can be useful in case of conflicts on keys because it’s generally used together with a merge function. This Collector supports flexible and customizable mapping of stream elements to the map structure, allowing the developer to create maps on different criteria.
78. What are some of the key applications of the Collectors Class?
Ans:
The Java. Util. Collectors class in Java 8 provides static methods for general data aggregation while using streams. Probably the most important usage of that class is element collecting into lists, sets, or maps by using techniques like toList(), toSet() and toMap(), counting statistics such as counting(), summarizing () or averagingDouble() -collecting elements to concatenate the strings of the Stream with joining().
79. How Lambda Expressions Can Replace Anonymous Inner Classes?
Ans:
- Since 2014, this version of Java has started using lambda expressions to replace anonymous inner classes using a more concise and readable approach to implementing functional interfaces.
- With anonymous inner classes, boilerplate code is always needed to define the full class structure. This reduces noise and improves readability because anonymous inner classes focus on the behavior being implemented.
- Also, lambda expressions can be easily passed as arguments to methods or stored in variables, enabling a more functional programming style.
80. What is the Significance of Classes IntStream, LongStream, and DoubleStream?
Ans:
Java 8 has introduced IntStream, LongStream, and DoubleStream classes as special stream classes. They are meant to be worked on primitive data types. This approach would result in far better performance and reduce memory overhead. These streams allow for operations native to their respective primitive types: summing, averaging, and other statistical computations on the data themselves. Since they do not have the overhead of boxing and unboxing primitive types into their wrapper type, these streams are more efficient when dealing with large datasets.
81. How does the Optional Class Prevent NullPointerExceptions?
Ans:
The Optional class prevents NullPointerExceptions by providing a container object that can either contain or not contain a value, explicitly representing the absence of a value. Instead of returning null, methods can return an Optional to help handle the possibility of absence with much grace. There are methods like isPresent() or ifPresent(); using those methods, code can check if a value exists before access to not trigger runtime errors. More than this, Optional provides methods such as orElse() orElseGet() to provide default values if the optional is empty.
82. What Are Common Mistakes When Using Lambda Expressions?
Ans:
- Common mistakes when using lambda expressions include overlooking the scope of variables since, by nature, a lambda can only effectively access final variables in their enclosing context.
- Developers will likely overlook handling exceptions since checked exceptions cannot be thrown from a lambda unless wrapped in a custom functional interface.
- Besides, code that is too full of lambda expressions is easier to read if its logic is complex. Also, the developer might face a type mismatch error when its functional interface type is not well known.
- Another performance issue occurs when lambda expressions are used without care. Here, the choice of the outer variables can become unnecessary when using a lambda expression.
83. Simplifying Tasks on Date Manipulation with Java 8’s New Date and Time API.
Ans:
- Java 8’s new date and time API simplifies data manipulation tasks through an intuitive and more complete set of classes for a date, time, calendar system, zones, and their interrelationships.
- Classes that include LocalDate, LocalTime, and LocalDateTime are immutable, ensuring thread safety and minimizing errors. The API also separates dates and time into understandable components, making it easier to manipulate the date without confusing the two.
- Methods for common operations, such as adding or subtracting days or months, are very simple and user-friendly. The new API also supports time zones through the class ZonedDateTime, which can enhance flexibility when dealing with a broad range of temporal scenarios.
84. What’s the benefit of Java 8’s Embedded Functional Interfaces?
Ans:
- Java 8’s predefined functional interfaces offer a standard set of interfaces that make the functional programming model easy to use.
- For instance, Function, Consumer, Supplier, and Predicate are interfaces that have enhanced reusability and consistency across applications.
- Using the above-predefined interfaces will decrease boilerplate code for developers automatically while developing lambda expressions or method references.
- They also make integrating more seamlessly with the Stream API and other functional programming constructs easier.
85. How does Lambda Expression support functional Programming in Java?
Ans:
Lambda expressions help improve the functional programming concepts in Java to an extent wherein one can make the creation of functional interfaces even more concise and expressive. They enable one to treat behaviour as parameters, enabling a higher-order programming mode and allowing functions to be passed around like objects. This enables a more declarative coding mode in which what is sought after, rather than how to achieve it, is stressed.
86. Why Do Methods Overload with Lambda?
Ans:
Methods overloading with lambda is an advantage where one would easily have several functions named and parameterized differently yet the same name for this functionality. The flexibility and usability make it prominent. Another technique in lambda expressions is Method overriding where multiple methods of similar functional interface are handled using the technique of method overloading, thus making the code clean utilizing the help of method overloading.
87. How Could CompletableFuture Handle Concurrency?
Ans:
CompletableFuture manages concurrency with a simple way to do asynchronous computations while also maintaining a straightforward programming model. It is non-blocking; hence, multiple operations can be executed together without waiting for each before completing. Developers can chain together many asynchronous tasks by using methods like the apply (), then accept (), and then combine (), which state what should be done with a result of a previous computation.
88. What is the difference in Java 8 between a Synchronous and Asynchronous Execution?
Ans:
- In Java 8, synchronous operations run serially one after another, and completion of the programmer’s task is waited for before proceeding to the next operation.
- This can, therefore, sometimes cause blocking, where one thread waits for the completion of its task, which might, in turn, cause bottlenecks in performance.
- Asynchronous execution allows running tasks independently. Thus, while different operations may run at nearly the same time, they don’t have to be completed before running something else.
- This non-blocking model improves responsiveness-especially in applications that involve I/O operations or long-running tasks.
89. How do we use the predicate interface to filter collections?
Ans:
- The Predicate interface can be used to filter collections. It describes conditions that elements must meet to be included in the results.
- Using the filter() method with streams, developers can apply a Predicate to specify criteria for retaining elements.
- This allows clean, expressive code; filtering logic can easily be applied without explicit loops. The Predicate interface can also be combined with methods like and(), or(), and negate() to handle more complex filtering scenarios.
- Generally speaking, applying the Predicate interface greatly improves flexibility and readability in Java collection filtering.
90. What Is the UnaryOperator Functional Interface Used For?
Ans:
The UnaryOperator functional interface represents an operation that takes one operand and delivers a result of the same type. This is a specialization of the Function interface and, therefore, very useful when the input and output types are identical. It may transform values or apply mathematical operations to numbers, manipulate objects, etc. The UnaryOperator interface encourages functional programming practices because developers can define concise reusable operations that can be easily integrated into streams or other functional contexts.