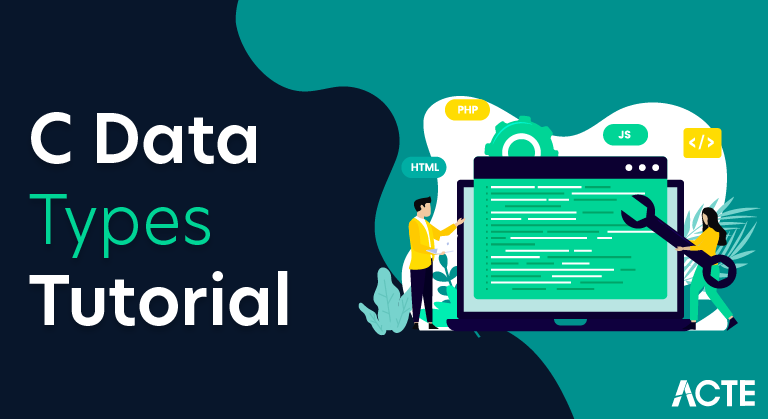
Data types in c refer to an extensive system used for declaring variables or functions of different types. The type of a variable determines how much space it occupies in storage and how the bit pattern stored is interpreted.
- Data types specify how we enter data into our programs and what type of data we enter. C language has some predefined set of data types to handle various kinds of data that we can use in our program. These datatypes have different storage capacities.
C language supports 2 different type of data types:
Primary data types:
- These are fundamental data types in C namely integer(int), floating point(float), character(char) and void.
Derived data types:
- Derived data types are nothing but primary data types but a little twisted or grouped together like array, structure, union and pointer. These are discussed in detail later.
- Data type determines the type of data a variable will hold. If a variable x is declared as int. it means x can hold only integer values. Every variable which is used in the program must be declared as what data-type it is
Data Types in C
A data type specifies the type of data that a variable can store such as integer, floating, character, etc.
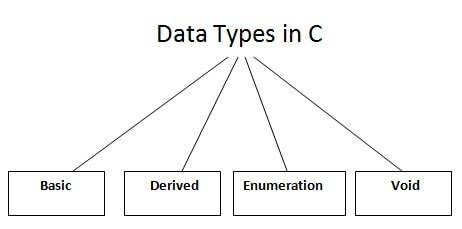
These are the following data types in C language.
Types | Data Types |
---|---|
Basic Data Type | int, char, float, double |
Derived Data Type | array, pointer, structure, union |
Enumeration Data Type | enum |
Void Data Type | void |
The types in C can be classified as follows:
Types | Description |
Basic types | They are arithmetic types and are further classified into: (a) integer types and (b) floating-point types. |
Enumerated types | They are again arithmetic types and they are used to define variables that can only assign certain discrete integer values throughout the program. |
The type void | The type specifier void indicates that no value is available. |
Derived types | They include (a) Pointer types, (b) Array types, (c) Structure types, (d) Union types and (e) Function types. |
The array types and structure types are referred collectively as the aggregate types. The type of a function specifies the type of the function’s return value. We will see the basic types in the following section, whereas other types will be covered in the upcoming chapters.
Integer Types:
The following table provides the details of standard integer types with their storage sizes and value ranges:
Type | Storage size | Value range |
char | 1 byte | -128 to 127 or 0 to 255 |
unsigned char | 1 byte | 0 to 255 |
signed char | 1 byte | -128 to 127 |
int | 2 or 4 bytes | -32,768 to 32,767 or -2,147,483,648 to 2,147,483,647 |
unsigned int | 2 or 4 bytes | 0 to 65,535 or 0 to 4,294,967,295 |
short | 2 bytes | -32,768 to 32,767 |
unsigned short | 2 bytes | 0 to 65,535 |
long | 8 bytes | -9223372036854775808 to 9223372036854775807 |
unsigned long | 8 bytes | 0 to 18446744073709551615 |
To get the exact size of a type or a variable on a particular platform, you can use the sizeof operator. The expression sizeof(type) yields the storage size of the object or type in bytes. Given below is an example to get the size of various type on a machine using different constant defined in limits.h header file.
- #include <stdio.h>
- #include <stdlib.h>
- #include <limits.h>
- #include <float.h>
- int main(int argc, char** argv) {
- printf(“CHAR_BIT : %d\n”, CHAR_BIT);
- printf(“CHAR_MAX : %d\n”, CHAR_MAX);
- printf(“CHAR_MIN : %d\n”, CHAR_MIN);
- printf(“INT_MAX : %d\n”, INT_MAX);
- printf(“INT_MIN : %d\n”, INT_MIN);
- printf(“LONG_MAX : %ld\n”, (long) LONG_MAX);
- printf(“LONG_MIN : %ld\n”, (long) LONG_MIN);
- printf(“SCHAR_MAX : %d\n”, SCHAR_MAX);
- printf(“SCHAR_MIN : %d\n”, SCHAR_MIN);
- printf(“SHRT_MAX : %d\n”, SHRT_MAX);
- printf(“SHRT_MIN : %d\n”, SHRT_MIN);
- printf(“UCHAR_MAX : %d\n”, UCHAR_MAX);
- printf(“UINT_MAX : %u\n”, (unsigned int) UINT_MAX);
- printf(“ULONG_MAX : %lu\n”, (unsigned long) ULONG_MAX);
- printf(“USHRT_MAX : %d\n”, (unsigned short) USHRT_MAX);
- return 0;
- }
When you compile and execute the above program, it produces the following result on Linux:
CHAR_BIT : 8
CHAR_MAX : 127
CHAR_MIN : -128
INT_MAX : 2147483647
INT_MIN : -2147483648
LONG_MAX : 9223372036854775807
LONG_MIN : -9223372036854775808
SCHAR_MAX : 127
SCHAR_MIN : -128
SHRT_MAX : 32767
SHRT_MIN : -32768
UCHAR_MAX : 255
UINT_MAX : 4294967295
ULONG_MAX : 18446744073709551615
USHRT_MAX : 65535
Floating-Point Types
The following table provide the details of standard floating-point types with storage sizes and value ranges and their precision:
Type | Storage size | Value range | Precision |
float | 4 byte | 1.2E-38 to 3.4E+38 | 6 decimal places |
double | 8 byte | 2.3E-308 to 1.7E+308 | 15 decimal places |
long double | 10 byte | 3.4E-4932 to 1.1E+4932 | 19 decimal places |
The header file float.h defines macros that allow you to use these values and other details about the binary representation of real numbers in your programs.
The following example prints the storage space taken by a float type and its range values:
- #include <stdio.h>
- #include <stdlib.h>
- #include <limits.h>
- #include <float.h>
- int main(int argc, char** argv) {
- printf(“Storage size for float : %d \n”, sizeof(float));
- printf(“FLT_MAX : %g\n”, (float) FLT_MAX);
- printf(“FLT_MIN : %g\n”, (float) FLT_MIN);
- printf(“-FLT_MAX : %g\n”, (float) -FLT_MAX);
- printf(“-FLT_MIN : %g\n”, (float) -FLT_MIN);
- printf(“DBL_MAX : %g\n”, (double) DBL_MAX);
- printf(“DBL_MIN : %g\n”, (double) DBL_MIN);
- printf(“-DBL_MAX : %g\n”, (double) -DBL_MAX);
- printf(“Precision value: %d\n”, FLT_DIG );
- return 0;
- }
When you compile and execute the above program, it produces the following result on Linux:
Storage size for float : 4
FLT_MAX : 3.40282e+38
FLT_MIN : 1.17549e-38
-FLT_MAX : -3.40282e+38
-FLT_MIN : -1.17549e-38
DBL_MAX : 1.79769e+308
DBL_MIN : 2.22507e-308
-DBL_MAX : -1.79769e+308
Precision value: 6
The void Type
Void type means no value. This is usually used to specify the type of functions which returns nothing. We will get acquainted with this datatype as we start learning more advanced topics in C language, like functions, pointers etc.
The void type specifies that no value is available. It is used in three kinds of situations:
Types | Description |
Function returns as void | There are various functions in C which do not return any value or you can say they return void. A function with no return value has the return type as void. For example, void exit (int status); |
Function arguments as void | There are various functions in C which do not accept any parameter. A function with no parameter can accept a void. For example, int rand(void); |
Pointers to void | A pointer of type void * represents the address of an object, but not its type. For example, a memory allocation function void*malloc( size_t size ); returns a pointer to void which can be casted to any data type. |
C is a general-purpose, high-level language that was originally developed by Dennis M. Ritchie to develop the UNIX operating system at Bell Labs. C was originally first implemented on the DEC PDP-11 computer in 1972.
- In 1978, Brian Kernighan and Dennis Ritchie produced the first publicly available description of C, now known as the K&R standard.
The UNIX operating system, the C compiler, and essentially all UNIX application programs have been written in C. C has now become a widely used professional language for various reasons −
- Easy to learn
- Structured language
- It produces efficient programs
- It can handle low-level activities
- It can be compiled on a variety of computer platforms
Facts about C
- C was invented to write an operating system called UNIX.
- C is a successor of B language which was introduced around the early 1970s.
- The language was formalized in 1988 by the American National Standard Institute (ANSI).
- The UNIX OS was totally written in C.
- Today C is the most widely used and popular System Programming Language.
- Most of the state-of-the-art software has been implemented using C.
- Today’s most popular Linux OS and RDBMS MySQL have been written in C.
Why use C?
C was initially used for system development work, particularly the programs that make-up the operating system. C was adopted as a system development language because it produces code that runs nearly as fast as the code written in assembly language. Some examples of the use of C might be:
- Operating Systems
- Language Compilers
- Assemblers
- Text Editors
- Print Spoolers
- Network Drivers
- Modern Programs
- Databases
- Language Interpreters
- Utilities
C Programs
A C program can vary from 3 lines to millions of lines and it should be written into one or more text files with extension “.c”; for example, hello.c. You can use “vi”, “vim” or any other text editor to write your C program into a file.
This tutorial assumes that you know how to edit a text file and how to write source code inside a program file.
Features of C Language
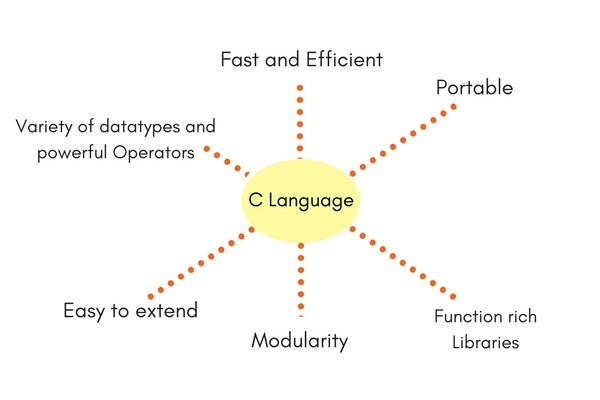
C is the widely used language. It provides many features that are given below.
- Simple
- Machine Independent or Portable
- Mid-level programming language
- structured programming language
- Rich Library
- Memory Management
- Fast Speed
- Pointers
- Recursion
- Extensible
1) Simple
C is a simple language in the sense that it provides a structured approach (to break the problem into parts), the rich set of library functions, data types, etc.
2) Machine Independent or Portable
Unlike assembly language, c programs can be executed on different machines with some machine specific changes. Therefore, C is a machine independent language.
3) Mid-level programming language
Although, C is intended to do low-level programming. It is used to develop system applications such as kernel, driver, etc. It also supports the features of a high-level language. That is why it is known as a mid-level language.
4) Structured programming language
C is a structured programming language in the sense that we can break the program into parts using functions. So, it is easy to understand and modify. Functions also provide code reusability.
5) Rich Library
C provides a lot of inbuilt functions that make the development fast.
6) Memory Management
It supports the feature of dynamic memory allocation. In C language, we can free the allocated memory at any time by calling the free() function.
7) Speed
The compilation and execution time of C language is fast since there are lesser inbuilt functions and hence the lesser overhead.
8) Pointer
C provides the feature of pointers. We can directly interact with the memory by using the pointers. We can use pointers for memory, structures, functions, array, etc.
9) Recursion
In C, we can call the function within the function. It provides code reusability for every function. Recursion enables us to use the approach of backtracking.
10) Extensible
C language is extensible because it can easily adapt new features.
Variables in C:
A variable is a name of the memory location. It is used to store data. Its value can be changed, and it can be reused many times.
It is a way to represent memory location through symbols so that it can be easily identified.
Let’s see the syntax to declare a variable:
- type variable_list;
The example of declaring the variable is given below:
- int a;
- float b;
- char c;
Here, a, b, c are variables. The int, float, char are the data types.
We can also provide values while declaring the variables as given below:
- int a=10,b=20;//declaring 2 variable of integer type
- float f=20.8;
- char c=’A’;
Rules for defining variables
- A variable can have alphabets, digits, and underscore.
- A variable name can start with the alphabet, and underscore only. It can’t start with a digit.
- No whitespace is allowed within the variable name.
- A variable name must not be any reserved word or keyword, e.g. int, float, etc.
Valid variable names:
- int a;
- int _ab;
- int a30;
Invalid variable names:
- int 2;
- int a b;
- int long;
Types of Variables in C
There are many types of variables in c:
- local variable
- global variable
- static variable
- automatic variable
- external variable
Local Variable
A variable that is declared inside the function or block is called a local variable.
It must be declared at the start of the block.
- void function1(){
- int x=10;//local variable
- }
You must have to initialize the local variable before it is used.
Global Variable
A variable that is declared outside the function or block is called a global variable. Any function can change the value of the global variable. It is available to all the functions.
It must be declared at the start of the block.
- int value=20;//global variable
- void function1(){
- int x=10;//local variable
- }
Static Variable
A variable that is declared with the static keyword is called static variable.
It retains its value between multiple function calls.
- void function1(){
- int x=10;//local variable
- static int y=10;//static variable
- x=x+1;
- y=y+1;
- printf(“%d,%d”,x,y);
- }
If you call this function many times, the local variable will print the same value for each function call, e.g, 11,11,11 and so on. But the static variable will print the incremented value in each function call, e.g. 11, 12, 13 and so on.
Automatic Variable
All variables in C that are declared inside the block, are automatic variables by default. We can explicitly declare an automatic variable using auto keyword.
- void main(){
- int x=10;//local variable (also automatic)
- auto int y=20;//automatic variable
- }
External Variable
We can share a variable in multiple C source files by using an external variable. To declare an external variable, you need to use extern keyword.
- myfile.h
- extern int x=10;//external variable (also global)
program1.c
- #include “myfile.h”
- #include <stdio.h>
- void printValue(){
- printf(“Global variable: %d”, global_variable);
- }