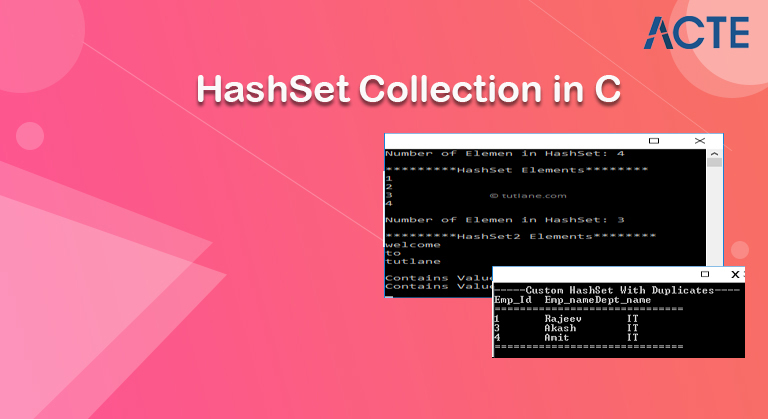
Introduction :-
In C#, HashSet is an unordered collection of unique elements. This collection is introduced in .NET 3.5. It supports the implementation of sets and uses the hash table for storage. This collection is of the generic type collection and it is defined under System.Collections.Generic namespace. It is generally used when we want to prevent duplicate elements from being placed in the collection.
About C# HashSet :-
last adjusted December 3, 2021 C# HashSet instructional exercise tells the best way to work with a HashSet assortment in C#.
HashSet:
HashSet addresses a bunch of qualities. It contains no copy components. The assortment models the numerical set deliberation. HashSet doesn’t give requesting of components. On the off chance that we want to keep control, we can utilize the Sorted Set assortment.
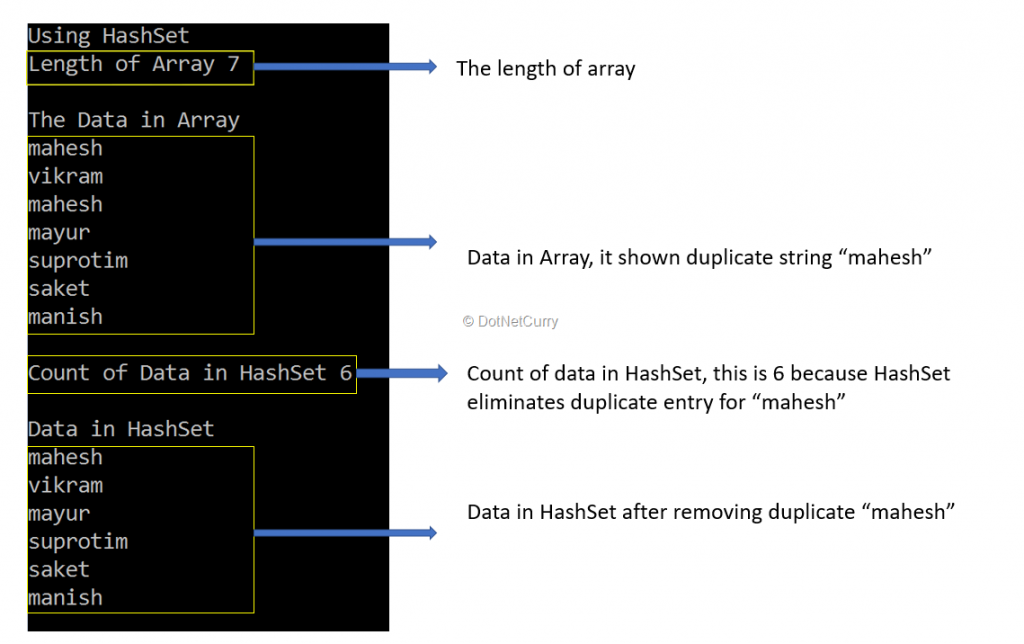
C# HashSet count components:-
The Count property returns the number of components in the HashSet.
Program. cs
var brands = new HashSet<'string'>();<'/string'>
brands.Add(“Wilson”);
brands.Add(“Nike”);
brands.Add(“Volvo”);
brands.Add(“IBM”);
brands.Add(“IBM”);
int nOfElements = brands.Count;
Console.WriteLine($”The set contains {nOfElements} components”);
Console.WriteLine(string.Join(“, “, brands));
- The HashSet Collection gives tasks superior execution.
- There are no copy components in the HashSet Collection.
- There is no most extreme limit with regards to the components in the HashSet. The limit increments as the quantity of the number of components increments.
- There is no specific request for the components in the HashSet Collection.
HashSet Collection in C#:
An unordered assortment of interesting things is known as a HashSet. It is found in System.Collections.Generic namespace. A portion of the highlights of HashSet Collection are as per the following:
What is the utilization of HashSet in C#?
In C#, HashSet is an unordered assortment of interesting components. This assortment is presented in. NET 3.5. It upholds the execution of sets and uses the hash table for capacity.
What is a HashSet?
A HashSet – addressed by the HashSet class relating to the System.Collections.Generic namespace – is a superior presentation, unordered assortment of novel components. Subsequently, a HashSet isn’t arranged and doesn’t contain any copy components. A HashSet likewise doesn’t uphold files – you can utilize enumerators as it were. A HashSet is normally utilized for elite execution activities including a bunch of exceptional information.
What is the HashSet assortment?
Java HashSet class is utilized to make an assortment that utilizes a hash table for capacity. It acquires the AbstractSet class and carries out Set connection point. HashSet stores the components by utilizing an instrument called hashing. HashSet contains exceptional components as it were. HashSet permits invalid worth.
Constructors in HashSet Collection:
The various constructors and their portrayal is given as follows:
Table: Constructors in HashSet Collection in C#
Source: MSDN
|
|
---|---|
HashSet <'T'>() | This constructor initializes a new instance of HashSet <'T'> class. This instance is empty and it uses the default equality comparer. |
HashSet<'T'>(IEnumerable<'T'>) | This constructor initializes a new instance of HashSet <'T'> class. It contains elements copied from the specified collection with a capacity to accomodate all elements copied. |
IEqualityComparer<'T'>) | HashSet <'T'> class. It uses the specified equality comparer that contains elements from the specified collection. |
HashSet<'T'>(IEqualityComparer<'T'>) | This constructor initializes a new instance of HashSet <'T'> class. This instance is empty and it uses the specified equality comparer. |
HashSet<'T'>(IEnumerable<'T'>, HashSet<'T'>(Int32) | This constructor initializes a new instance of HashSet <'T'> class. This instance is empty but it has a reserved space for capacity items. It uses the default equality comparer. |
HashSet<'T'>(Int32, IEqualityComparer<'T'>) | This constructor initializes a new instance of HashSet <'T'> class. It can accomodate the capacity elements and uses the specified quality compare. |
HashSet<'T'>(SerializationInfo, StreamingContext) | This constructor initializes a new instance of HashSet <'T'> class using serialized data. |
Methods in HashSet Collection:
The different methods and their description is given as follows:
Table: Methods in HashSet Collection in C#
| |
---|---|
Add(T) | This property gets the IEqualityComparer<'T'> object. This object is used to determine equality for the values in the set. |
Clear() | This property gets the number of elements that are contained in a set. |
Clear() | This method removes all the elements from a HashSet<'T'> object. |
Contains(T) | This method determines whether a HashSet<'T'> object contains the specified element. |
CopyTo(T[]) | This method copies the elements of a HashSet<'T'> object to an array. |
CreateSetComparer() | This method returns an IEqualityComparer object. this object can be used for the equality testing of a HashSet<'T'> object. |
Equals(Object) | This method determines if the specified object is equal to the current object or not. |
GetEnumerator() | This method returns an enumerator that iterates through a HashSet<'T'> object. |
GetHashCode() | This method is the default hash function. |
GetType() | This method gets the Type of the current instance. |
IntersectWith(IEnumerable<'T'>) | This method modifies the current HashSet<'T'> object to contain only those elements that are present in that object and in the specified collection. |
MemberwiseClone() | This method creates a shallow copy of the current Object. |
Remove(T) | This method removes the specified element from the HashSet<'T'> object. |
RemoveWhere(Predicate<'T'>) | This method removes all the elements that match the conditions that are defined by a specified predicate from a HashSet<'T'> collection. |
SetEquals(IEnumerable<'T'>) | This method determines if a HashSet<'T'> object and the specified collection contain the same elements. |
SymmetricExceptWith(IEnumerable<'T'>) | This method modifies the current HashSet<'T'> object to contain only those elements that are present either in that object or in the specified collection, but not in both. |
ToString() | This method returns a string that represents the current object. |
TrimExcess() | This method sets the capacity of a HashSet<'T'> object to the actual number of elements that it contains, rounded up to a nearby, implementation-specific value. |
TryGetValue(T, T) | This method searches the set for a given value and returns the equal value if it finds it. |
UnionWith(IEnumerable<'T'>) | This method modifies the current HashSet<'T'> object to contain all the elements that are present in itself, the specified collection, or both. |
Search a thing in a HashSet in C#:
To look through a thing in a HashSet you can involve the Contains technique as displayed in the code piece given beneath:
static void Main(string[] args)
{
HashSet<'string'> hashSet = new HashSet<'string'>();
hashSet.Add(“A”);
hashSet.Add(“B”);
hashSet.Add(“C”);
hashSet.Add(“D”);
if (hashSet.Contains(“D”))
Console.WriteLine(“The required component is available.”);
else
Console.WriteLine(“The required component isn’t available.”);
Console.ReadKey();
}
HashSet components are exceptional all of the time:
Assuming that you endeavor to embed a copy component in a HashSet it would essentially be disregarded yet no runtime exemption will be tossed. The accompanying code scrap shows this.
static void Main(string[] args)
{
HashSet<'string'> hashSet = new HashSet<'string'>();
hashSet.Add(“A”);
hashSet.Add(“B”);
hashSet.Add(“C”);
hashSet.Add(“D”);
hashSet.Add(“D”);
Console.WriteLine(“The number of components is: {0}”, hashSet.Count);
Console.ReadKey();
}
At the point when you execute the program, the result will be as displayed. Presently consider the accompanying code scrap that outlines how to copy components are disposed of:
string[] urban communities = new string[] {
“Delhi”,
“Kolkata”,
“New York”,
“London”,
“Tokyo”,
“Washington”,
“Tokyo”
};
HashSet<'string'> hashSet = new HashSet<'string'>(cities);
foreach (var city in hashSet)
{
Console.WriteLine(city);
}
Whenever you execute the above program, the copy city names would be taken out.
Eliminate components from a HashSet in C#:
To eliminate a thing from a HashSet you should call the Remove strategy. The language structure of the Remove technique is given below.On the off chance that the thing is found in the assortment, the Remove technique eliminates a component from the HashSet and returns valid on progress, bogus in any case.
Promotion:
The code bit given below shows how you can utilize the Remove technique to eliminate a thing from a HashSet.
string thing = “D”;
if(HashSet.Contains(item))
{
hashSet.Remove(item);
}
Promotion:
HashSet<'string'> setA = new HashSet<'string'>() { “A”, “B”, “C”, “D” };
HashSet<'string'> setB = new HashSet<'string'>() { “A”, “B”, “C”, “X” };
HashSet<'string'> setC = new HashSet<'string'>() { “A”, “B”, “C”, “D”, “E” };
in the event that (setA.IsProperSubsetOf(setC))
Console.WriteLine(“setC contains all components of setA.”);
if (!setA.IsProperSubsetOf(setB))
Console.WriteLine(“setB doesn’t contains all components of setA.”);
- The HashSet class carries out the ICollection, IEnumerable, IReadOnlyCollection, ISet, IEnumerable, IDeserializationCallback, and ISerializable connection points.
- In HashSet, the request for the component isn’t characterized. You can’t sort the components of HashSet.
- In HashSet, the components should be one of a kind.
- In HashSet, copy components are not permitted.
- Is gives numerous numerical set activities, like crossing point, association, and distinction.
- The limit of a HashSet is the quantity of components it can hold.
- A HashSet is a unique assortment implies the size of the HashSet is naturally expanded when the new components are added.
- In HashSet, you can store similar sort of components.
Importance of Hashset Collection in C#:-
- HashSet doesn’t keep everything under control.
- HashSet is quicker than tree set [time intricacy O(1)].
- HashSet utilizes approaches().
- HashSet permits invalid.
- TreeSet sorts in light of comparator.
- TreeSet more slow than hashset [time intricacy of O(log (n))].
- TreeSet utilizes compareTo().
- TreeSet doesn’t permit invalid.
What are the distinctions between a HashSet and TreeSet assortment in Java?
HASH SET
TREE SET
- HashSet versus List – Add() technique
- HashSet versus List – Contains() technique
- HashSet versus List – Remove() strategy
Features for HashSet:
The segment of HashSet C#:
- Taking out the Duplicate information section in HashSet
- Alter HashSet utilizing UnionWith() Method
- Alter Hashset utilizing ExceptWith() Method
- Alter Hashset utilizing SymmetricExceptWith() strategy
- Checking execution of activities like Add, Remove, Contains on HashSet and List.
- This class address a bunch of qualities.
- This class gives an elite execution set of activities.
- This is a bunch of assortment that contains no copy components and there is no particular request for the components put away in it.
- In the .NET Framework 4.6 delivery, the HashSet executes the IReadOnlyCollection interface alongside the ISet interface.
- The HashSet class doesn’t have any most extreme limit with regards to the number of components put away in it. This limit continues to increment as the number of components is included.
Scopes of HashSet C#:
Here are a few striking highlights of HashSet.
- HashSet stores the components by utilizing a system called hashing.
- HashSet contains novel components as it were.
- HashSet permits invalid worth.
- Simple to began
- Less Hardware Requirement
- Cross-Platform
- HashSet class is nonsynchronized.
- HashSet doesn’t keep the inclusion control. Here, components are embedded based on their hashcode.
- not adaptable
- Absence of scalability
- Incapable to Copy and Paste
Advantage and Disadvantage:
Advantage:
Disadvantage :
Conclusion:
HashSet in C# .NET is a superior exhibition assortment store. The benefit of utilizing the HashSet object is to perform standard tasks like Union, Intersection, and so forth which gives a simple and viable coding experience. Then again, List object has the element of thing requesting, duplication, and so forth