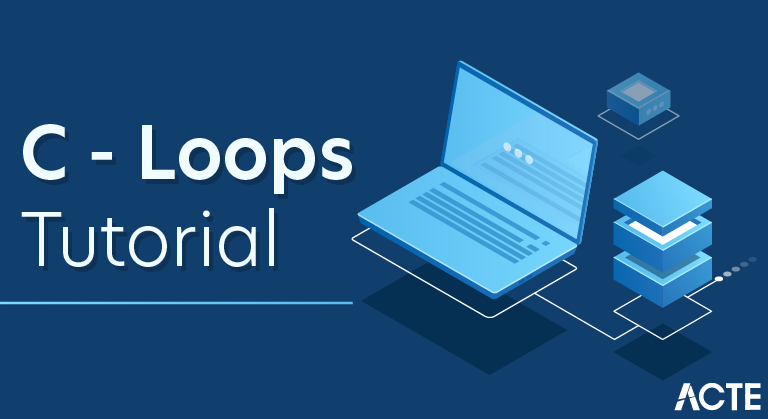
C programming is a general-purpose, procedural, imperative computer programming language developed in 1972 by Dennis M. Ritchie at the Bell Telephone Laboratories to develop the UNIX operating system. C is the most widely used computer language. It keeps fluctuating at number one scale of popularity along with Java programming language, which is also equally popular and most widely used among modern software programmers.
The UNIX operating system, the C compiler, and essentially all UNIX application programs have been written in C. C has now become a widely used professional language for various reasons −
- Easy to learn
- Structured language
- It produces efficient programs
- It can handle low-level activities
- It can be compiled on a variety of computer platforms
What are Loops?
A Loop executes the sequence of statements many times until the stated condition becomes false. A loop consists of two parts, a body of a loop and a control statement. The control statement is a combination of some conditions that direct the body of the loop to execute until the specified condition becomes false. The purpose of the loop is to repeat the same code a number of times.
You may encounter situations, when a block of code needs to be executed several number of times. In general, statements are executed sequentially: The first statement in a function is executed first, followed by the second, and so on.
Programming languages provide various control structures that allow for more complicated execution paths.
A loop statement allows us to execute a statement or group of statements multiple times. Given below is the general form of a loop statement in most of the programming languages −
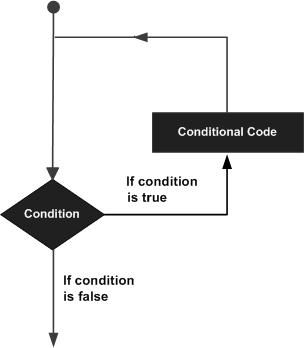
C programming language provides the following types of loops to handle looping requirements.
Types of Loops
Depending upon the position of a control statement in a program, a loop is classified into two types:
- Entry controlled loop
- Exit controlled loop
In an entry controlled loop, a condition is checked before executing the body of a loop. It is also called as a pre-checking loop.
In an exit controlled loop, a condition is checked after executing the body of a loop. It is also called as a post-checking loop.
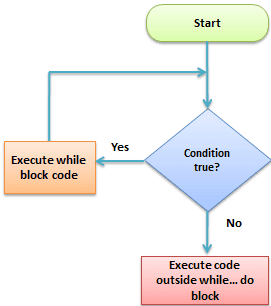
Sample Loop
The control conditions must be well defined and specified otherwise the loop will execute an infinite number of times. The loop that does not stop executing and processes the statements number of times is called as an infinite loop. An infinite loop is also called as an “Endless loop.” Following are some characteristics of an infinite loop:
- No termination condition is specified.
- The specified conditions never meet.
The specified condition determines whether to execute the loop body or not.
‘C’ programming language provides us with three types of loop constructs:
- The while loop
- The do-while loop
- The for loop
While Loop
A while loop is the most straightforward looping structure. The basic format of while loop is as follows:
- while (condition)
- {
- statements;}
It is an entry-controlled loop. In while loop, a condition is evaluated before processing a body of the loop. If a condition is true then and only then the body of a loop is executed. After the body of a loop is executed then control again goes back at the beginning, and the condition is checked if it is true, the same process is executed until the condition becomes false. Once the condition becomes false, the control goes out of the loop.
After exiting the loop, the control goes to the statements which are immediately after the loop. The body of a loop can contain more than one statement. If it contains only one statement, then the curly braces are not compulsory. It is a good practice though to use the curly braces even we have a single statement in the body.
In while loop, if the condition is not true, then the body of a loop will not be executed, not even once. It is different in do while loop which we will see shortly.
The above program illustrates the use of while loop. In the above program, we have printed series of numbers from 1 to 10 using a while loop.
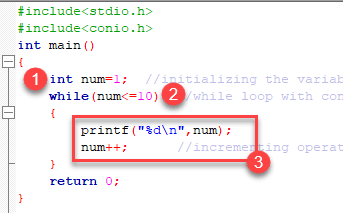
- We have initialized a variable called num with value 1. We are going to print from 1 to 10 hence the variable is initialized with value 1. If you want to print from 0, then assign the value 0 during initialization.
- In a while loop, we have provided a condition (num<=10), which means the loop will execute the body until the value of num becomes 10. After that, the loop will be terminated, and control will fall outside the loop.
- In the body of a loop, we have a print function to print our number and an increment operation to increment the value per execution of a loop. An initial value of num is 1, after the execution, it will become 2, and during the next execution, it will become 3. This process will continue until the value becomes 10 and then it will print the series on console and terminate the loop.
\n is used for formatting purposes which means the value will be printed on a new line.
Do-While loop
A do-while loop is similar to the while loop except that the condition is always executed after the body of a loop. It is also called an exit-controlled loop.
The basic format of while loop is as follows:
- do {
- statements }
- while (expression);
As we saw in a while loop, the body is executed if and only if the condition is true. In some cases, we have to execute a body of the loop at least once even if the condition is false. This type of operation can be achieved by using a do-while loop.
In the do-while loop, the body of a loop is always executed at least once. After the body is executed, then it checks the condition. If the condition is true, then it will again execute the body of a loop otherwise control is transferred out of the loop.
Similar to the while loop, once the control goes out of the loop the statements which are immediately after the loop is executed.
The critical difference between the while and do-while loop is that in while loop the while is written at the beginning. In do-while loop, the while condition is written at the end and terminates with a semi-colon (;)
In the above example, we have printed multiplication table of 2 using a do-while loop. Let’s see how the program was able to print the series.
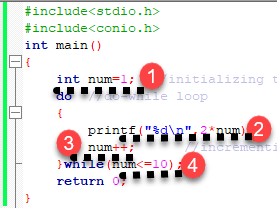
- First, we have initialized a variable ‘num’ with value 1. Then we have written a do-while loop.
- In a loop, we have a print function that will print the series by multiplying the value of num with 2.
- After each increment, the value of num will increase by 1, and it will be printed on the screen.
- Initially, the value of num is 1. In a body of a loop, the print function will be executed in this way: 2*num where num=1, then 2*1=2 hence the value two will be printed. This will go on until the value of num becomes 10. After that loop will be terminated and a statement which is immediately after the loop will be executed. In this case return 0.
For loop
A for loop is a more efficient loop structure in ‘C’ programming. The general structure of for loop is as follows:
- for (initial value; condition; incrementation or decrementation )
- {
- statements;
- }
- The initial value of the for loop is performed only once.
- The condition is a Boolean expression that tests and compares the counter to a fixed value after each iteration, stopping the for loop when false is returned.
- The incrementation/decrementation increases (or decreases) the counter by a set value.
The above program prints the number series from 1-10 using for loop.
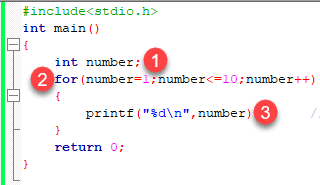
- We have declared a variable of an int data type to store values.
- In for loop, in the initialization part, we have assigned value 1 to the variable number. In the condition part, we have specified our condition and then the increment part.
- In the body of a loop, we have a print function to print the numbers on a new line in the console. We have the value one stored in number, after the first iteration the value will be incremented, and it will become 2. Now the variable number has the value 2. The condition will be rechecked and since the condition is true loop will be executed, and it will print two on the screen. This loop will keep on executing until the value of the variable becomes 10. After that, the loop will be terminated, and a series of 1-10 will be printed on the screen.
In C, the for loop can have multiple expressions separated by commas in each part.
Notice that loops can also be nested where there is an outer loop and an inner loop. For each iteration of the outer loop, the inner loop repeats its entire cycle.
The nesting of for loops can be done up-to any level. The nested loops should be adequately indented to make code readable. In some versions of ‘C,’ the nesting is limited up to 15 loops, but some provide more.
The nested loops are mostly used in array applications which we will see in further tutorials.
Break Statement
The break statement is used mainly in in the switch statement. It is also useful for immediately stopping a loop.
Continue Statement
When you want to skip to the next iteration but remain in the loop, you should use the continue statement.
Which loop to Select?
Selection of a loop is always a tough task for a programmer, to select a loop do the following steps:
- Analyze the problem and check whether it requires a pre-test or a post-test loop.
- If pre-test is required, use a while or for a loop.
- If post-test is required, use a do-while loop.
Loop Control Statements
Loop control statements change execution from its normal sequence. When execution leaves a scope, all automatic objects that were created in that scope are destroyed.
C supports the following control statements.
Methods | Description |
---|---|
Break statement | Terminates the loop or switch statement and transfers execution to the statement immediately following the loop or switch. |
Continue statement | Causes the loop to skip the remainder of its body and immediately retest its condition prior to reiterating. |
Goto statement | Transfers control to the labeled statement. |
The Infinite Loop
A loop becomes an infinite loop if a condition never becomes false. The for loop is traditionally used for this purpose. Since none of the three expressions that form the ‘for’ loop are required, you can make an endless loop by leaving the conditional expression empty.
When the conditional expression is absent, it is assumed to be true. You may have an initialization and increment expression, but C programmers more commonly use the for(;;) construct to signify an infinite loop.
If you want to set up your environment for C programming language, you need the following two software tools available on your computer, (a) Text Editor and (b) The C Compiler.
Text Editor
This will be used to type your program. Examples of few a editors include Windows Notepad, OS Edit command, Brief, Epsilon, EMACS, and vim or vi.
The name and version of text editors can vary on different operating systems. For example, Notepad will be used on Windows, and vim or vi can be used on windows as well as on Linux or UNIX.
The files you create with your editor are called the source files and they contain the program source codes. The source files for C programs are typically named with the extension “.c”.
Before starting your programming, make sure you have one text editor in place and you have enough experience to write a computer program, save it in a file, compile it and finally execute it.
The C Compiler
The source code written in source file is the human readable source for your program. It needs to be “compiled”, into machine language so that your CPU can actually execute the program as per the instructions given.
The compiler compiles the source codes into final executable programs. The most frequently used and free available compiler is the GNU C/C++ compiler, otherwise you can have compilers either from HP or Solaris if you have the respective operating systems.
The following section explains how to install GNU C/C++ compiler on various OS. We keep mentioning C/C++ together because GNU gcc compiler works for both C and C++ programming languages.
Advantages
- Redundancy of code is less.
- Used to reduce the code.
- Effectively solves all the difficult codes using loops very easily, especially pattern programs.
- Generally we use loops in the case of repetitive occurance of some processes.
- Suppose you do not know ahead of time how many times you wanted to repeat a certain sequence of instructions, or the number of times might be different each time you run the program. Writing a loop which uses variables for its limits is often the best way to do this, and the easiest to understand.
- Even if you know the instructions will be repeated a constant number of times, using a loop means not having to copy-and-paste the code. This has several advantages: i)You do not have to worry as much about changing the code for each iteration (for example, changing a variable name). You can re-use a variable, or maybe use an array.ii)Probably the biggest: If you need to change or fix the code, you only have to do it one place.
- In general, anything you can do with looping you could do instead with recursion (and some things, such as tree traversal, are much easier with recursion). But if you can do it either way, looping usually has lower overhead than recursion, because the program does not need to repeatedly gather parameters, call a function, return a value, and return from the function, just to perform each step. Using a loop, the program mostly just needs to do a conditional jump. Yes, it might also have to do an increment or something like that, but it would most likely have to do that in recursion also.
Disadvantages
- In both C and C++, the traditional for-loop is very flexible. Depending on your point of view, that could be either an advantage or disadvantage.
- C++ also offers a more concise (and more constrained) range-based for-loop. It has the advantage of being both more concise and more constrained. It has the disadvantage of being more constrained.
- Pascal for-loops are very constrained compared to their C counterparts. Again, whether you consider this an advantage or disadvantage is a matter of perspective.
- For-loops are a tool, like hammers and screwdrivers. And just like hammers and screwdrivers, you can wield them inappropriately.
- Now the issues were that is it has a hard coded loop limit; it made us make a loop variable and we are going to have to use it in some possibly not convenient way inside the loop. Or even worse, perhaps we don’t need it at all and it is just there to count loop iterations. Another issue, if something in the loop changes the value of j this loop won’t do what the for loop expresses. It might loop forever!
Conclusion
- Looping is one of the key concepts on any programming language.
- It executes a block of statements number of times until the condition becomes false.
- Loops are of 2 types: entry-controlled and exit-controlled.
- ‘C’ programming provides us 1) while 2) do-while and 3) for loop.
- For and while loop is entry-controlled loops.
- Do-while is an exit-controlled loop.