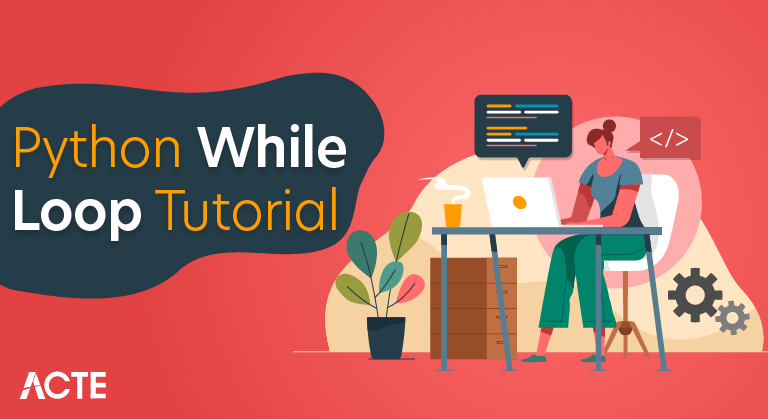
Python is a general-purpose interpreted, interactive, object-oriented, and high-level programming language. It was created by Guido van Rossum during 1985- 1990. Like Perl, Python source code is also available under the GNU General Public License (GPL). This tutorial gives enough understanding on Python programming language.
Why To Learn Python?
Python is a high-level, interpreted, interactive and object-oriented scripting language. Python is designed to be highly readable. It uses English keywords frequently where as other languages use punctuation, and it has fewer syntactical constructions than other languages. Python is a MUST for students and working professionals to become a great Software Engineer specially when they are working in Web Development Domain.
I will list down some of the key advantages of learning Python:
- Python is Interpreted : Python is processed at runtime by the interpreter. You do not need to compile your program before executing it. This is similar to PERL and PHP.
- Python is Interactive : You can actually sit at a Python prompt and interact with the interpreter directly to write your programs.
- Python is Object-Oriented : Python supports Object-Oriented style or technique of programming that encapsulates code within objects.
- Python is a Beginner’s Language : Python is a great language for the beginner-level programmers and supports the development of a wide range of applications from simple text processing to WWW browsers to games.
Characteristics Of Python
Following are important characteristics of Python Programming :
- It supports functional and structured programming methods as well as OOP.
- It can be used as a scripting language or can be compiled to byte-code for building large applications.
- It provides very high-level dynamic data types and supports dynamic type checking.
- It supports automatic garbage collection.
- It can be easily integrated with C, C++, COM, ActiveX, CORBA, and Java.
Applications Of Python
As mentioned before, Python is one of the most widely used language over the web. I’m going to list few of them here:
- Easy-to-learn : Python has few keywords, simple structure, and a clearly defined syntax. This allows the student to pick up the language quickly.
- Easy-to-read : Python code is more clearly defined and visible to the eyes.
- Easy-to-maintain : Python’s source code is fairly easy-to-maintain.
- A broad standard library : Python’s bulk of the library is very portable and cross-platform compatible on UNIX, Windows, and Macintosh.
- Interactive Mode : Python has support for an interactive mode which allows interactive testing and debugging of snippets of code.
- Portable : Python can run on a wide variety of hardware platforms and has the same interface on all platforms.
- Extendable : You can add low-level modules to the Python interpreter. These modules enable programmers to add to or customize their tools to be more efficient.
- Databases : Python provides interfaces to all major commercial databases.
- GUI Programming : Python supports GUI applications that can be created and ported to many system calls, libraries and windows systems, such as Windows MFC, Macintosh, and the X Window system of Unix.
- Scalable : Python provides a better structure and support for large programs than shell scripting.
Python While Loop
- In Python, While Loops is used to execute a block of statements repeatedly until a given condition is satisfied. And when the condition becomes false, the line immediately after the loop in the program is executed.
- While loop falls under the category of indefinite iteration. Indefinite iteration means that the number of times the loop is executed isn’t specified explicitly in advance.
- While loop is used to iterate over a block of code repeatedly until a given condition returns false. In the last tutorial, we have seen for loop in Python, which is also used for the same purpose.
- The main difference is that we use while loop when we are not certain of the number of times the loop requires execution, on the other hand when we exactly know how many times we need to run the loop, we use for loop.
Syntax Of While Loop
while condition:
#body_of_while
The body_of_while is set of Python statements which requires repeated execution. These set of statements execute repeatedly until the given condition returns false.
Flow Of While Loop
- First the given condition is checked, if the condition returns false, the loop is terminated and the control jumps to the next statement in the program after the loop.
- If the condition returns true, the set of statements inside loop are executed and then the control jumps to the beginning of the loop for next iteration.
These two steps happen repeatedly as long as the condition specified in while loop remains true.
Python – While loop Example
Here is an example of while loop. In this example, we have a variable num and we are displaying the value of num in a loop, the loop has a increment operation where we are increasing the value of num.
This is very important step, the while loop must have a increment or decrement operation, else the loop will run indefinitely, we will cover this later in infinite while loop.
- num = 1
- # loop will repeat itself as long as
- # num < 10 remains true
- while num < 10:
- print(num)
- #incrementing the value of num
- num = num + 3
Output:
1
4
7
Working Of While Loop :
Single Statement While Block :
Just like the if block, if the while block consists of a single statement the we can declare the entire loop in a single line. If there are multiple statements in the block that makes up the loop body, they can be separated by semicolons (;).
filter_none
edit
play_arrow
brightness_4
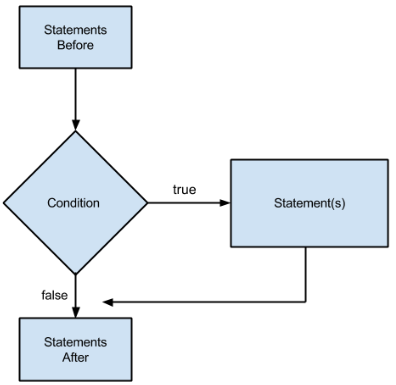
Output:
Hello Geek
Hello Geek
Hello Geek
Hello Geek
Hello Geek
Loop Control Statements
Loop control statements change execution from its normal sequence. When execution leaves a scope, all automatic objects that were created in that scope are destroyed. Python supports the following control statements.
Continue Statement:
It returns the control to the beginning of the loop.
filter_none
edit
play_arrow
brightness_4
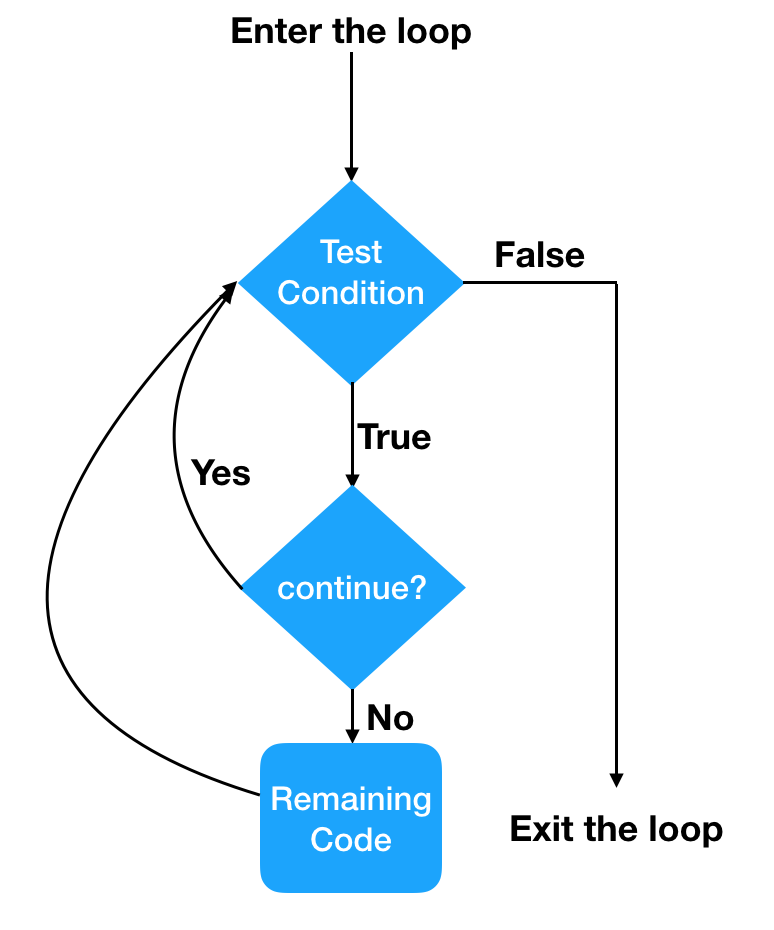
Output:
Current Letter : g
Current Letter : k
Current Letter : f
Current Letter : o
Current Letter : r
Current Letter : g
Current Letter : k
Break Statement:
It brings control out of the loop.
filter_none
edit
play_arrow
brightness_4
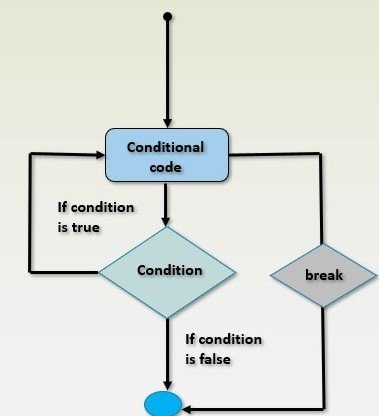
Output:
Current Letter : g
Pass Statement:
We use pass statement to write empty loops. Pass is also used for empty control statements, functions and classes.
filter_none
edit
play_arrow
brightness_4
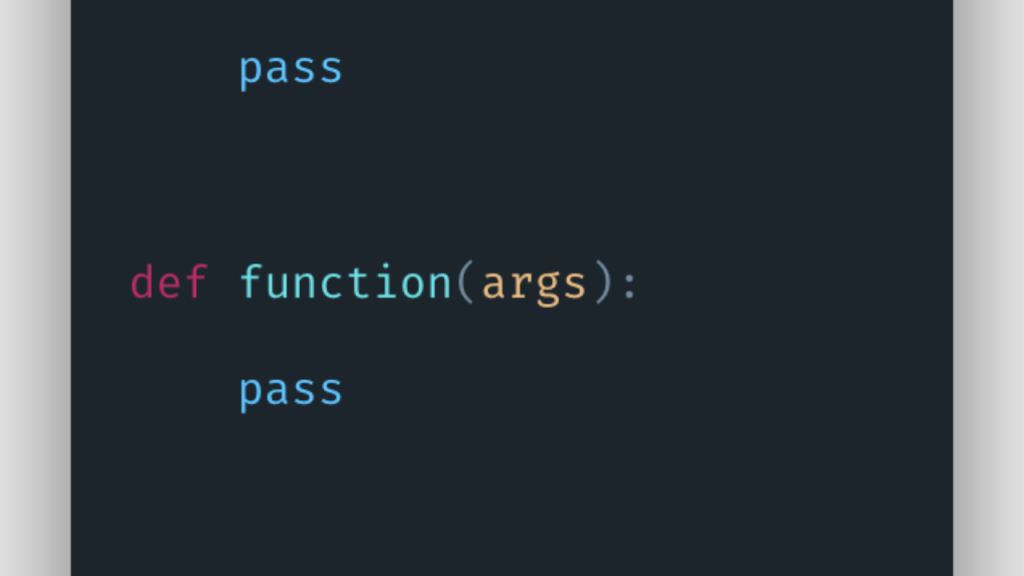
Output:
Value of i : 13
While-Else Loop
As discussed above, while loop executes the block until a condition is satisfied. When the condition becomes false, the statement immediately after the loop is executed.
The else clause is only executed when your while condition becomes false. If you break out of the loop, or if an exception is raised, it won’t be executed.
filter_none
edit
play_arrow
brightness_4
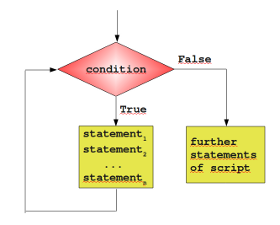
Output:
1
2
3
4
No Break
1
Nested While Loop In Python
When a while loop is present inside another while loop then it is called nested while loop. Lets take an example to understand this concept.
- i = 1
- j = 5
- while i < 4:
- while j < 8:
- print(i, “,”, j)
- j = j + 1
- i = i + 1
Output:
1 , 5
2 , 6
3 , 7
Python – While Loop With Else Block
We can have a ‘else’ block associated with while loop. The ‘else’ block is optional. It executes only after the loop finished execution.
- num = 10
- while num > 6:
- print(num)
- num = num-1
- else:
- print(“loop is finished”)
Output:
10
9
8
7
loop is finished
Premature Termination Of A While Loop
So far, a while loop only ends, if the condition in the loop head is fulfilled. With the help of a break statement a while loop can be left prematurely, i.e. as soon as the control flow of the program comes to a break inside of a while loop (or other loops) the loop will be immediately left. “break” shouldn’t be confused with the continue statement. “continue” stops the current iteration of the loop and starts the next iteration by checking the condition. Here comes the crucial point: If a loop is left by break, the else part is not executed.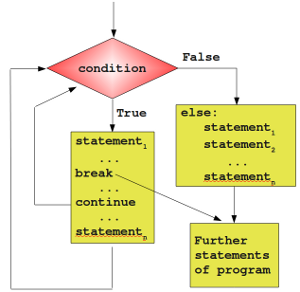
Advantages Of Python :
With the emerging python community and open source libraries python has grown into a complete software development package.
Driven By Vast And Active Community :
Python has one of the most known and active community which helps them in continuous improvement of the python. No wonder it was the top ranked platform on stack overflow. Python language is distributed under open source license which makes its development easy via open source contributions.Learning Curve :
With most of the programming languages their learning curves tends to grow parabolic with time that means it is hard to grasp early but as soon you become familiar with this language the learning becomes easy. But in case of python the learning is easy because of easy syntax and short hand writing.
Third Party Libraries :
Standard python package installer (PIP) can help you install numerous modules that make python interactive. These libraries and modules can interact from internet protocols, operating system calls and many more. You can do socket programming for networking and use os module for system calls that runs user level threads.
Integration With Other Languages :
Integration libraries like Cython and Jython makes python integrate with c/c++ and java for cross platform development. This makes python even more powerful since we all know no language is complete and advisable to use single language for everything development. Every language has its own forte, so using these libraries you can enjoy powerful, features of each language.
Productivity :
With python batteries included philosophy the developers get a head start without downloading separate modules and packages. Alongside python easy syntax and rapid prototyping the productivity increases nearly 40% as compared to traditional programming languages.
Disadvantages Of python :
We have seen the major advantages of the popular programming language Python. But we all know there are two sides of a coin!Python has indeed several drawbacks too, that makes developers stay away from it. So let’s see one by one:-Slow Speed :
Python uses interpreter that loads it line by line instead of compiler that executes the whole file at once. This makes compilation slower and tends to perform slowly. This is the major reason competitive programmers don’t use python. C++ provides more computation per seconds instead of python. Moreover this is why python is not extensively used in application development.
Error Detection In Codes :
Since python uses interpreter the error in codes does not come up during code compiling. Python uses dynamically typed variables which makes testing more hectic. All these errors came out to be a run-time error which no developers want in their program. This makes python less usable for GUI applets developments.
Weak In Mobile Devices:
We have seen python in web servers and desktop applications along with scripts that its used for. But it is not considered favorable for mobile devices because it uses more memory and slow processing compared to other languages.
Large Memory Consumption :
Python design structure is such that it uses large memory while processing as compared to other languages as C/C++. This makes Python a complete no no for development under tight memory restrictions.
Conclusion:
In comparison to different programming languages Python is the most broadly used via the developers currently. The critical Python language benefits are that it is easy to read and smooth to check and learn. It is less complicated to install packages and writing a software in Python than in C or C++. Some other benefits of Python programming is that no computer virus can originate a segmentation fault since there are no concepts of pointers or references in python. An important advantage of Python language over traditional programming languages is that it has wide applicability and acceptance, and is appreciably utilized by scientists, engineers, and mathematicians.
It is due to this that Python is so beneficial for prototyping and all kinds of experiments. It is also used at the same time as generating animation for films and in machine learning and deep learning. The language is seen as a less suitable platform for cellular development and game development. It’s far frequently used on desktop and server, but there are the handiest several mobile packages that were used majorly with Python. Every other drawback Python has is the runtime errors. The language has a whole lot of design limitations .Python executes with an interpreter instead of the compiler, which speeds down the performance.