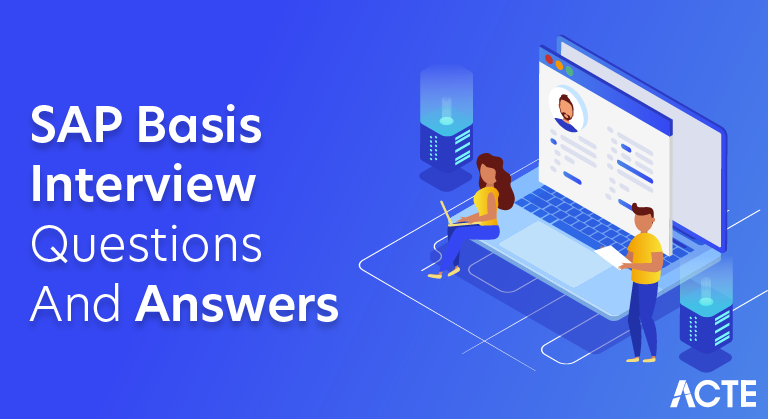
40+ [REAL-TIME] Java Concurrency Interview Questions and Answers
Last updated on 26th Apr 2024, Popular Course
Java Concurrency pertains to the programming approach in Java aimed at executing multiple tasks concurrently. It encompasses managing thread execution and ensuring synchronization among threads for effective operation in multi-threaded environments. Java offers built-in features and libraries, including the java.util.concurrent package, facilitating thread management, synchronization, and concurrent data structure usage. Proficiency in Java Concurrency is essential for developing efficient and scalable Java applications.
1. What are threads in Java?
Ans:
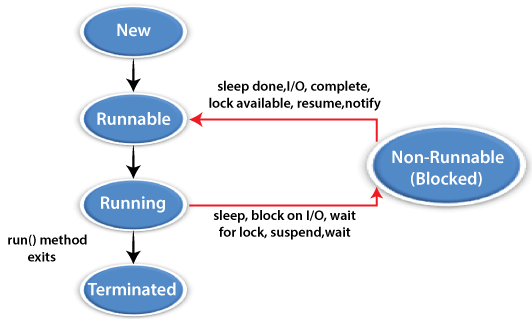
Threads in Java represent lightweight processes nested within a single process. They facilitate concurrent execution, enabling tasks to operate independently. Threads facilitate efficient communication when sharing the same memory space. Whether instances of the Thread class or implementations of Runnable, threads empower concurrent operations. Their concurrent nature enhances responsiveness, aiding in parallel processing and multitasking within Java applications.
2. Describe Semaphore in Java Concurrency.
Ans:
Semaphores serve as gatekeepers controlling access to shared resources in Java. They manage permits, limiting simultaneous access by multiple threads. Permit acquisition and release govern resource utilization by threads. Implementing resource pools or thread pools and semaphores ensures controlled resource access. Supporting fair and unfair access policies, semaphores adapt to various concurrency scenarios.
3. What distinguishes Java’s CyclicBarrier from CountDownLatch?
Ans:
- CyclicBarrier orchestrates thread synchronization, allowing them to rendezvous at a predefined point before proceeding.
- It autonomously resets post rendezvous, facilitating cyclic synchronization.
- Conversely, CountDownLatch awaits the completion of a fixed number of threads before proceeding.
- Unlike CyclicBarrier, CountDownLatch lacks automatic reset functionality.
- Primarily for one-time synchronization tasks, CountDownLatch plays a pivotal role.
4. What distinguishes a thread from a process?
Ans:
- Threads, lightweight execution units, operate within a single process.
- Multiple threads within a process share resources and memory space.
- Cost-effective creation and switching distinguish threads from processes.
- Processes, independent execution entities, possess individual memory spaces.
- While inter-thread communication is seamless, inter-process communication tends to be intricate.
5. What various stages of a thread’s lifecycle are there?
Ans:
The thread lifecycle encompasses five phases: new, Runnable, Blocked, Waiting, Timed Waiting, and Terminated. Originating in the New state, threads await activation. Transitioning to Runnable indicates readiness for execution, awaiting CPU allocation. A blocked state ensues when a thread awaits monitor lock acquisition. The waiting and Timed Waiting stages denote thread waiting for specific conditions or other threads.
6.What differentiates the Thread class’s start() and run() methods?
Ans:
Feature | start() Method | run() Method | |
Purpose |
Initiates the execution of a new thread. |
Contains the code that constitutes the thread’s task. | |
Invocation | Must be called to begin a new thread. | Called automatically when a new thread starts. | |
New Thread |
Creates a new thread of execution. |
Code executes in the context of the new thread. | |
Execution Flow | Starts a new thread and invokes its run() method. | Contains the actual code to be executed by the thread. | |
Direct Invocation |
Should never directly call the run() method. |
You can call the run() method directly, but it’s not recommended for starting a new thread. |
7. What distinguishes a class lock from an object lock?
Ans:
- Class lock, associated with the class, impacts all its instances.
- Acquisition via synchronized static methods or blocks synchronized on class objects characterizes class locks.
- Object lock, unique to each instance, governs instance-specific resources.
- Synchronized instance methods or blocks synchronized on instances acquire object locks.
- Class locks synchronize access to shared static resources.
8. In Java, what exactly is a volatile variable?
Ans:
Volatile variables in Java guarantee direct read/write operations from/to main memory. They prevent local caching by threads, ensuring immediate visibility of changes across threads. They are suitable for shared variables accessed by multiple threads sans synchronization.Though lacking atomicity for compound operations, volatile variables enforce visibility and ordering. They assure consistent state representation across concurrent threads.
9. What are the steps for starting and running a Thread instance?
Ans:
Define a class extending Thread or implementing the Runnable interface. Override the run() method to encapsulate the thread’s executable logic. Instantiate the thread object. Optionally, configure thread properties such as name, priority, or daemon status. Initiate thread execution by invoking the start() method on the thread object.JVM orchestrates automatic invocation of the run() method on a separate thread.
10. What distinguishes Runnable from Callable in Java?
Ans:
- Runnable, a foundational interface introduced in Java 1.0, defines concurrent executable tasks.
- Lacking return values or checked exceptions, it encapsulates simple thread logic.
- Conversely, Callable, introduced in Java 5, extends the Runnable concept, allowing return values and checked exceptions.
- Callable’s call() method facilitates error handling with checked exceptions, unlike Runnable’s run() method.
- Typically, Future objects pair with Callable, enabling asynchronous computation result retrieval.
11.FutureTask Class: What is it?
Ans:
The FutureTask class in Java facilitates asynchronous computation. It serves as a container for a task that will yield a result in the future. This class is ideal for encapsulating long-running or potentially blocking computations.FutureTask offers methods to ascertain completion status and retrieve the eventual result. It also permits the cancellation of the task if necessary.
12. What does Java’s fork-join framework do?
Ans:
Java’s fork-join framework specializes in parallelizing recursive tasks. It employs a “divide and conquer” strategy, breaking down tasks into subtasks. These subtasks are recursively divided until they reach a specific granularity, after which their results are merged. The framework employs a work-stealing algorithm to efficiently distribute tasks among threads. The ForkJoinPool class is the primary entity for executing fork-join tasks in Java.
13. What are the concurrent programming patterns used in Java?
Ans:
- Java incorporates several concurrent programming patterns, including producer-consumer, reader-writer, and thread pools.
- The producer-consumer pattern involves producers generating data while consumers consume it.
- The reader-writer pattern allows concurrent access to shared resources with exclusive access for writers.
- Thread pools manage a pool of worker threads for executing multiple tasks.
14. What is multithreading in Java?
Ans:
- Multithreading in Java enables concurrent execution of multiple threads within a single program.
- Each thread represents an independent flow of execution, allowing tasks to run concurrently.
- This concurrency enhances program efficiency, enabling simultaneous task execution.
- Java’s built-in support for multithreading via the Thread class facilitates thread creation and management.
15. What is multitasking within a program in Java?
Ans:
Multitasking in Java refers to executing multiple tasks concurrently within a program. It involves breaking down the program’s execution into multiple threads, each handling a distinct task. Multitasking optimizes resource utilization, enhancing program efficiency. Java’s multithreading capabilities facilitate multitasking within a single program, enabling concurrent task execution.
16. What is multitasking in a single-thread environment?
Ans:
- Multitasking in a single-thread environment involves simulating concurrent execution by rapidly switching between tasks.
- Although only one thread executes at a time, the illusion of multitasking is achieved through rapid task switching.
- Techniques like time slicing allocate small time slices to each task for execution.
- Common in older operating systems or resource-constrained environments, this approach provides perceived multitasking.
- However, true concurrency is absent, and tasks are executed sequentially.
17. What is a scheduler?
Ans:
A scheduler is a component responsible for managing and scheduling tasks for execution on a computing system. In operating systems, schedulers determine task/thread access to system resources and the duration of execution. Tasks are prioritized based on criteria such as priority levels, deadlines, and resource availability. Efficient scheduler algorithms ensure optimal utilization of system resources and equitable CPU allocation.
18. What properties does each Java thread have?
Ans:
- Java threads possess intrinsic properties, including a dedicated stack, program counter, and thread-local storage.
- Threads share heap memory but maintain separate method execution contexts.
- Communication and synchronization between threads occur using shared objects like locks, monitors, and semaphores.
- Thread states include NEW, RUNNABLE, BLOCKED, WAITING, TIMED_WAITING, and TERMINATED.
19.What are the wait() and sleep() methods?
Ans:
- In Java, the wait() method facilitates inter-thread communication and synchronization.
- It suspends the current thread until another thread invokes notify() or notifyAll() on the same object.
- During wait(), the lock on the object is released, allowing other threads to obtain it.
- Conversely, the sleep() method pauses the current thread’s execution for a specified duration.
- Unlike wait(), sleep() doesn’t release the object lock and doesn’t require synchronization.
20. Explain the thread pool.
Ans:
A thread pool is a collection of pre-initialized threads ready to execute tasks. It streamlines thread management, alleviating the overhead of thread creation and destruction. Thread pools maintain a task queue, allocating threads to execute queued tasks. Control over the number of active threads prevents resource depletion and enhances efficiency.
21. What do you mean by garbage collection?
Ans:
Garbage collection automates memory management in Java. It deallocates memory held by unreferenced objects. Java’s garbage collector operates in the background efficiently. By handling memory automatically, developers are relieved from manual management tasks. Garbage collection enhances application performance and stability.
22. What is the purpose of synchronized keywords in Java?
Ans:
- The synchronized keyword controls access to code blocks.
- It ensures only one thread can access a block at a time.
- This prevents data corruption and inconsistency in shared resources.
- Synchronization is vital for thread safety in Java’s concurrent environment.
- It establishes mutually exclusive locks, allowing synchronized execution.
- Java’s synchronized keyword facilitates safe concurrent programming.
23. Explain the concept of deadlock in Java concurrency.
Ans:
Deadlock occurs when threads wait indefinitely for resources held by each other. This situation halts all involved threads. Deadlocks are caused by improper resource allocation and synchronization. Detecting and resolving deadlocks require careful analysis. Proper synchronization techniques are essential to avoid deadlocks. Deadlock avoidance and detection algorithms help mitigate risks.
24. What is the significance of the wait() method in Java concurrency?
Ans:
- The wait() method aids inter-thread communication and synchronization.
- It suspends the current thread until another thread is notified.
- Wait () typically operates within synchronized blocks for thread coordination.
- Threads efficiently await specific conditions before proceeding.
- Proper use of wait() and notify() ensures effective thread management.
- This method contributes to building robust concurrent applications.
25.How does the notify() method work in Java concurrency?
Ans:
Notify () wakes up a single waiting thread on an object’s monitor. It signals the waiting thread that a condition may have been met. Notify () doesn’t release the lock immediately but signals the thread to wake up. If multiple threads wait, only one is awakened by notify(). Threads can resume execution after being notified. Effective thread coordination relies on proper notify() usage.
26. Describe the difference between synchronized blocks and methods.
Ans:
- Synchronized blocks offer finer synchronization control than methods.
- They synchronize on specific objects, while methods synchronize on their objects.
- Using synchronized blocks minimizes synchronized code scope, reducing contention.
- Synchronized methods lock entire objects, potentially affecting performance.
- Blocks provide flexibility in synchronization, enhancing concurrency.
- Selection between blocks and methods depends on synchronization granularity.
27. What is the ConcurrentHashMap class in Java, and how is it different from HashMap?
Ans:
ConcurrentHashMap is a thread-safe Map implementation. It allows concurrent access without thread blocking. In contrast, HashMap lacks thread safety.ConcurrentHashMap segments its map, each with its lock. It outperforms synchronized HashMap in multithreaded environments. Utilizing ConcurrentHashMap enhances concurrency and throughput.
28. Explain thread safety in Java concurrency.
Ans:
Thread safety enables multiple threads to access resources concurrently without data corruption. Java offers synchronization, locks, and concurrent data structures for this purpose. Synchronized blocks and methods ensure exclusive code access, preventing race conditions. Thread safety is critical for reliable concurrent application development—failure to ensure thread safety results in unpredictable behavior.
29. How does the Java memory model impact concurrent programming?
Ans:
- The Java memory model governs thread memory interaction.
- It ensures changes by one thread are visible to others.
- Understanding it is crucial for writing efficient concurrent programs.
- Java’s memory model guarantees atomicity and shared variable visibility.
- Adherence to its rules avoids concurrency issues like data races.
- Following Java memory model principles fosters reliable concurrent programming.
30. What are the benefits of using immutable objects in concurrent programming?
Ans:
- Immutable objects inherently provide thread safety.
- They eliminate synchronization needs, reducing contention and overhead.
- Immutable objects ensure consistent behavior across threads.
- Sharing them among threads is safe without synchronization.
- They enhance performance and scalability in multithreaded contexts.
- Embracing immutability fosters cleaner, maintainable code in concurrency.
31. Describe the difference between thread-safe and non-thread-safe classes.
Ans:
- Thread-safe classes ensure safe concurrent access by multiple threads without data corruption.
- Non-thread-safe classes lack such protection, leading to potential data corruption or inconsistency.
- Thread-safe classes typically use synchronization mechanisms like locks or atomic operations.
- Non-thread-safe classes may require external synchronization to ensure safe concurrent access.
- Thread-safe classes guarantee consistent behavior across multiple threads.
- Non-thread-safe classes require careful handling to avoid race conditions or data corruption.
32. How does the AtomicInteger class work in Java concurrency?
Ans:
AtomicInteger provides atomic operations on an integer value without explicit synchronization. Operations like incrementAndGet() and compareAndSet() are atomic, ensuring thread safety. It internally uses compare-and-swap (CAS) operations for atomicity.CAS compares the current value with an expected value and updates it if the comparison holds.
33. Explain the concept of race condition.
Ans:
A race condition occurs in concurrent programs when the outcome depends on the timing of uncontrollable events. It arises when multiple threads access shared resources without proper synchronization. When threads execute non-atomic operations, the interleaving of their execution can lead to unexpected outcomes. Race conditions often result in incorrect behavior or data corruption.
34. Describe thread-local variables in Java.
Ans:
- Each thread has its independent copy of a thread-local variable.
- Thread-local variables are typically declared using the ThreadLocal class in Java.
- They are often used to maintain a per-thread state or to avoid synchronization overhead.
- Thread-local variables are initialized separately for each thread and are not shared among threads.
35. What is the purpose of the volatile keyword?
Ans:
- The volatile keyword in Java ensures visibility and atomicity of variable updates across threads.
- It prevents threads from caching the variable’s value in their local memory.
- Volatile variables are suitable for flags or status indicators accessed by multiple threads.
- They guarantee that changes made by one thread are visible to other threads immediately.
36. Explain reentrant locks in Java concurrency.
Ans:
Reentrant locks allow a thread to acquire the same lock multiple times. They support nested locking, where a thread can reacquire the lock it already holds. Reentrant locks use a lock count to track the number of times a lock has been acquired. They ensure that a thread releasing the lock doesn’t unlock it prematurely for other threads. Reentrant locks offer more flexibility and control over synchronization compared to intrinsic locks.
37. Describe the Executor framework.
Ans:
The Executor framework in Java provides a higher-level abstraction for asynchronous task execution. It separates task submission from task execution, allowing better control over thread management. Executors manage a pool of worker threads, which execute submitted tasks. They abstract away low-level thread creation and management details, improving scalability and resource management in concurrent applications.
38. What is the purpose of the ExecutorService interface?
Ans:
- ExecutorService extends the Executor interface and provides additional methods for task management.
- It represents an asynchronous execution service capable of managing thread pools and executing tasks.
- ExecutorService allows tasks to be submitted for execution and provides mechanisms for task completion and shutdown.
- It supports task cancellation, result retrieval, and asynchronous execution control.
39. How does the ForkJoinPool class work?
Ans:
ForkJoinPool is a specialized ExecutorService implementation designed for divide-and-conquer algorithms. It operates on the principle of work-stealing, where idle threads steal tasks from other threads’ queues.ForkJoinPool is particularly efficient for tasks that can be recursively divided into smaller subtasks. It dynamically adjusts the number of threads based on workload and available processor cores.
40.Describe the difference between Executors.newFixedThreadPool() and Executors.newCachedThreadPool().
Ans:
- Executors.newFixedThreadPool() creates a thread pool with a fixed number of threads that remain active.
- The number of threads in the pool remains constant, even if some are idle.
- It is suitable for scenarios where a fixed number of threads is desirable, regardless of the workload.
- Executors.newCachedThreadPool() creates a thread pool that dynamically adjusts its size based on workload.
- It creates new threads as needed and reuses idle threads if available.
- Cached thread pools are suitable for handling tasks with varying execution times and unpredictable workloads.
- Fixed thread pools provide better resource control and predictability, while cached thread pools offer better scalability and resource utilization.
41. Explain thread interruption in Java concurrency.
Ans:
Thread interruption in Java is a mechanism to politely request a thread to terminate its execution. It’s done via the interrupt() method. When a thread is interrupted, it sets its interrupt status. The interrupted thread can check its interrupt status periodically using isInterrupted() or handle the interruption using InterruptedException.
42. What is the purpose of the join() method?
Ans:
- The join() method in Java waits for a thread to complete its execution before proceeding to the next steps in the program.
- It allows for synchronization between multiple threads, ensuring that certain operations occur only after the completion of specific threads.
- By calling join(), a thread can effectively “wait” for another thread to finish. This is useful in scenarios where the main thread needs results from other threads.
43. Describe thread groups in Java.
Ans:
Thread groups in Java are a way to manage and categorize threads. They provide a convenient means to perform operations on multiple threads simultaneously. Threads can be organized into a hierarchical structure of thread groups, simplifying the management of related threads. Thread groups offer functionalities like setting priorities, interrupting, and suspending threads within the group.
44. How does the ThreadLocalRandom class work?
Ans:
- The ThreadLocalRandom class provides a way to generate random numbers without contention between threads.
- Each thread has its instance of ThreadLocalRandom, ensuring that random number generation is thread-safe and efficient.
- It uses a different seed for each thread, derived from a shared source of randomness but with thread-local properties, preventing contention and synchronization overhead.
45. Explain atomic operations in Java concurrency.
Ans:
Atomic operations in Java are executed as a single, indivisible unit of work, ensuring thread safety. They are performed without interference from other threads, preventing race conditions and ensuring consistent state transitions. Java provides atomic classes like AtomicInteger, AtomicLong, etc., which offer methods for performing atomic operations like compare-and-swap, increment, decrement, etc.
46. Describe the difference between fair and unfair locks.
Ans:
- Fair locks guarantee that the longest waiting thread gets access to the lock when it becomes available, ensuring fairness.
- Unfair locks do not provide such guarantees and may allow recently blocked or waiting threads to acquire the lock before older ones.
- Fair locks can introduce additional overhead due to the need to maintain a waiting queue, while unfair locks might lead to thread starvation in certain scenarios.
47. What is the purpose of the ReentrantReadWriteLock class?
Ans:
- The ReentrantReadWriteLock class in Java provides a locking mechanism that allows multiple threads to read a shared resource concurrently while ensuring exclusive access for writing.
- It improves concurrency by allowing multiple readers but only one writer at a time.
- This can be beneficial in scenarios where reads are frequent and writes are less frequent, reducing contention and improving overall throughput.
48. Explain condition variables in Java concurrency.
Ans:
Condition variables in Java concurrency provide a way for threads to wait for a certain condition to become true before proceeding. They are associated with locks and are typically used in conjunction with ReentrantLock or ReentrantReadWriteLock.Threads can wait on a condition variable using await() and signal/wait for condition changes using signal() and signal () methods.
49. How does the LockSupport class work?
Ans:
- The LockSupport class in Java provides low-level thread synchronization primitives, such as blocking and unblocking threads.
- It allows threads to park and unpark, essentially suspending and resuming their execution.
- Unlike traditional locking mechanisms, LockSupport operates at the thread level, enabling more flexible synchronization patterns.
50. Describe thread confinement.
Ans:
Thread confinement is a technique in Java concurrency in which an object is accessed only by a single thread throughout its lifetime. It ensures that the object’s state remains consistent and avoids concurrency issues such as data races and inconsistent states. Thread confinement can be achieved by ensuring that the object is created and manipulated within the context of a single thread or by using thread-local variables.
51. What is the purpose of the Phaser class?
Ans:
- The Phaser class aids in synchronizing threads in distinct phases.
- Threads can pause until all reach a designated phase before continuing.
- This class dynamically adjusts to the number of participating threads.
- It’s beneficial for tasks requiring synchronized progress at different stages.
- Compared to barriers and countdown latches, Phasers offer enhanced flexibility.
- They are commonly applied in parallel algorithms and concurrent tasks.
52. Explain parallel streams in Java concurrency.
Ans:
Parallel streams empower concurrent execution of stream operations. They automatically segment data and process it concurrently. Utilizing the ForkJoinPool framework, they ensure efficient multithreading. Their application notably enhances performance for CPU-bound tasks on multi-core systems. However, meticulous handling is crucial to guarantee thread safety and prevent race conditions. Parallel streams excel in tasks involving large datasets amenable to parallelism.
53. How does the CompletableFuture class work?
Ans:
- CompletableFuture enables asynchronous, non-blocking tasks in Java.
- It embodies the future result of asynchronous computations.
- Chaining multiple CompletableFuture instances enables sequential or parallel execution.
- Support for callbacks permits the specification of actions upon completion.
- Offering various methods for combining, composing, and handling exceptions in asynchronous computations.
- CompletableFuture simplifies intricate asynchronous programming in Java.
54. Describe the purpose of the StampedLock class.
Ans:
- StampedLock furnishes a locking mechanism incorporating optimistic read and write locks.
- It allows multiple readers simultaneous access to shared resources, barring a writer’s presence.
- This class facilitates upgrading from a read lock to a write lock.
- Notably, it is more efficient than ReentrantReadWriteLock for read-heavy workloads.
- StampedLock finds utility in scenarios marked by high contention for read access.
55. What is the significance of the Java.util.concurrent.atomic package?
Ans:
The Java.util.concurrent.atomic package supplies atomic variables for thread-safe operations. Ensuring indivisible and thread-safe operations, atomic variables utilize low-level CPU instructions like compare-and-swap (CAS). They serve as a cornerstone for lock-free concurrent programming. Mitigating the necessity for explicit synchronization primitives like locks in many instances.
56. Explain thread starvation in Java concurrency.
Ans:
Thread starvation arises when a thread fails to access shared resources. It typically results from unfair scheduling or indefinite postponement of a thread’s execution. Left unaddressed, thread starvation may precipitate deadlock or livelock situations. It’s a prevalent challenge in concurrent programming marked by resource contention. Strategies like fair scheduling and meticulous resource management can mitigate thread starvation.
57. How does the CopyOnWriteArrayList class work?
Ans:
- The CopyOnWriteArrayList serves as a thread-safe alternative to ArrayList.
- It achieves thread safety by generating a new copy of the underlying array upon mutation.
- Iterators operate on a snapshot of the array during their creation.
- Although mutation operations incur a relatively high cost due to array copying, it’s ideal for scenarios with high read frequency.
- CopyOnWriteArrayList is commonly adopted in environments with low write contention and high read throughput.
58. Describe the purpose of the BlockingQueue interface.
Ans:
- The BlockingQueue interface represents a thread-safe queue offering blocking operations in Java.
- It furnishes methods for adding, removing, and inspecting elements within the queue.
- It supports operations that await the queue’s transition to a non-empty or non-full state.
- It’s widely employed in producer-consumer scenarios within concurrent programming.
- BlockingQueue implementations feature diverse blocking strategies such as FIFO, priority-based, etc.
59. What is the purpose of the Exchanger class?
Ans:
The Exchanger class facilitates coordination between two threads by exchanging objects. It establishes a synchronization point at which threads exchange data. Typically, it is utilized in scenarios necessitating object swapping between two threads in a producer-consumer pattern.Threads await at the exchange point until both counterparts arrive, affecting the exchange.
60. Explain memory consistency errors in Java concurrency.
Ans:
Memory consistency errors manifest when different threads observe inconsistent states of shared variables. They arise due to modern processors’ reordering and caching optimizations. Such errors can lead to unforeseen behavior, undermining program correctness. Java’s memory model delineates rules ensuring predictable behavior in concurrent programs. Employing synchronization, volatile variables, and memory barriers aids in addressing memory consistency issues.
61. How does the ConcurrentLinkedQueue class work?
Ans:
- ConcurrentLinkedQueue serves as a thread-safe Queue implementation in Java.
- Employing a non-blocking approach, it utilizes linked nodes for addition, removal, and traversal.
- Multiple threads can add and remove elements concurrently without explicit synchronization.
- Leveraging atomic operations like CAS (compare-and-swap), it achieves high concurrency.
- Iterators in ConcurrentLinkedQueue ensure weakly consistent behavior.
62. What is the purpose of Phaser in Java concurrency?
Ans:
- Phaser emerges as a flexible alternative to CountDownLatch and CyclicBarrier.
- It orchestrates synchronization across multiple threads spanning various phases.
- Threads dynamically register, arrive, advance, or deregister at distinct phases.
- Valuable for tasks with dynamically varying participant counts, it adapts seamlessly.
- Phaser accommodates synchronization points for a variable number of participants.
63. How does CompletableFuture work in Java concurrency?
Ans:
CompletableFuture embodies the outcome of asynchronous computations. It facilitates manual completion, cancellation, and composition with other CompletableFutures.With a fluent API, it enables chaining operations for streamlined concurrency management. Callbacks can be specified upon completion, exception, or cancellation, enhancing control.
64. Describe StampedLock in Java concurrency.
Ans:
StampedLock, introduced in Java 8, introduces optimistic locking capabilities. It permits multiple readers alongside a single writer, facilitating efficient read operations.StampedLock offers optimistic read-write locks, notably through the tryOptimisticRead method. Ideal for scenarios with a surplus of reads and minimal contention, it optimizes performance. Flexibility extends to upgrading from read locks to write locks and vice versa.
65. What is the significance of Java.util.concurrent.atomic package?
Ans:
- The Java.util.concurrent.atomic package furnishes lock-free, thread-safe operations on variables.
- Essential atomic classes like AtomicInteger, AtomicLong, and AtomicBoolean cater to primitive types.
- These classes execute atomic read-modify-write operations sans explicit synchronization.
- Critical for constructing lock-free algorithms and highly concurrent data structures, they ensure consistency.
66. How does CopyOnWriteArrayList work in Java concurrency?
Ans:
- CopyOnWriteArrayList emerges as a thread-safe variant of ArrayList.
- It safeguards thread integrity by crafting fresh copies of the underlying array upon modifications.
- Reads operate sans synchronization, fostering concurrent execution.
- However, the expense of write operations arises from copying the complete array.
- Suited for scenarios marked by frequent reads and infrequent writes, it ensures safety.
67. Describe the BlockingQueue interface in Java concurrency.
Ans:
BlockingQueue signifies a Queue variant supporting operations awaiting element availability. Blocking methods like put() and take() halt until completion, enhancing synchronization. Prevalent in producer-consumer setups, it fosters seamless thread coordination. Ensuring thread safety, it orchestrates harmonious interaction between producer and consumer threads.
68. What is the purpose of Exchanger in Java concurrency?
Ans:
Exchanger streamlines thread coordination, enabling the exchange of objects at synchronization points. Threads await mutual arrival at the exchange juncture, facilitating seamless object swapping. Vital for scenarios necessitating inter-thread data exchange, it fosters synchronization. Acting as a rendezvous point for thread-to-thread data transfer, it streamlines communication.
69. How does ConcurrentLinkedQueue work in Java concurrency?
Ans:
- ConcurrentLinkedQueue stands out as a non-blocking, thread-safe Queue implementation.
- Leveraging a lock-free algorithm based on linked nodes, it supports insertion, removal, and traversal.
- Multiple threads engage with ConcurrentLinkedQueue concurrently, sans contention.
- Ensuring weakly consistent iterators, it upholds the visibility of pre-iteration element additions.
70. Describe ConcurrentHashMap in Java concurrency.
Ans:
- ConcurrentHashMap emerges as a thread-safe rendition of the Map interface.
- It enables concurrent access to key-value pairs without necessitating explicit synchronization.
- Partitioning its underlying data structure mitigates contention, fostering high concurrency.
- It bolsters reliability by supporting atomic operations like putIfAbsent(), replace(), and remove().
- A preferred choice for concurrent hash map needs, it upholds thread safety.
71. Explain concurrent collections in Java.
Ans:
Concurrent collections in Java furnish thread-safe operations for structures such as lists, maps, and queues. They enable multiple threads to access and modify collections simultaneously sans explicit synchronization. Instances include ConcurrentHashMap, CopyOnWriteArrayList, and ConcurrentLinkedQueue.These collections heighten performance in concurrent settings by curbing contention and ensuring consistency.
72. Compare LinkedBlockingQueue with ArrayBlockingQueue in Java concurrency.
Ans:
- LinkedBlockingQueue, an unbounded queue, employs a linked list, while ArrayBlockingQueue, a bounded queue, utilizes an array.
- LinkedBlockingQueue dynamically adjusts its capacity, whereas ArrayBlockingQueue maintains a fixed capacity.
- In scenarios with variable loads like producer-consumer setups, LinkedBlockingQueue typically offers superior throughput.
- ArrayBlockingQueue is preferable when a fixed capacity is requisite, and there’s a known upper limit on elements.
73. What is the purpose of Semaphore in Java concurrency?
Ans:
Semaphores in Java concurrency furnish a mechanism to regulate access to a shared resource by constraining the number of threads concurrently accessing it. They uphold a set of permits that govern the number of concurrent threads permitted to access the resource. Semaphore’s acquire() method is employed to procure a permit, potentially blocking until one becomes available.
74. Describe CountDownLatch in Java concurrency.
Ans:
CountDownLatch in Java concurrency enables one or more threads to wait until a set of operations performed in other threads concludes. It initializes with a count representing the number of events to await. Threads invoking await() on the latch will stall until the count reaches zero. Each event diminishes the count via the countDown() method. Once the count attains zero, all awaiting threads are liberated.
75. How does CyclicBarrier work in Java concurrency?
Ans:
- CyclicBarrier in Java concurrency permits a group of threads to halt at a barrier until all threads reach the barrier.
- It commences with a fixed number of parties (threads) that must reach the barrier.
- Threads invoke the await() method on the barrier and block until all parties have arrived.
- Upon all parties’ arrival, the barrier releases all waiting threads, resetting for the next cycle.
76. Explain thread pooling in Java concurrency.
Ans:
Thread pooling in Java concurrency encompasses establishing a cohort of reusable threads supervised by a pool manager. Instead of spawning a new thread for each task, threads from the pool are utilized, ameliorating performance and resource management.ThreadPoolExecutor, a native Java class, administers thread pools, permitting customization of pool size, queueing behavior, and thread instantiation.
77. Describe the ScheduledExecutorService interface in Java concurrency.
Ans:
ScheduledExecutorService in Java concurrency extends ExecutorService to accommodate delayed and recurring task execution. It furnishes methods like schedule(), scheduleAtFixedRate(), and scheduleWithFixedDelay() for task scheduling.ScheduledExecutorService abstracts complexities entailed in managing scheduled tasks within a concurrent environment.
78. How does ScheduledThreadPoolExecutor work in Java concurrency?
Ans:
- ScheduledThreadPoolExecutor is an implementation of ScheduledExecutorService in Java concurrency.
- It orchestrates a pool of threads for executing scheduled tasks with configurable concurrency.
- Tasks can be scheduled with a delay, at fixed intervals, or with a fixed delay between executions.
- ScheduledThreadPoolExecutor ensures optimal utilization of threads by reusing them for multiple tasks.
79. What is the purpose of ThreadFactory in Java concurrency?
Ans:
- ThreadFactory in Java concurrency furnishes a means to tailor thread creation within an Executor or Thread Pool.
- It encapsulates the logic for spawning new threads, enabling developers to regulate thread properties such as priority, name, and daemon status.
- ThreadFactory disentangles thread creation from application logic, fostering modularity and reusability.
- By furnishing a custom ThreadFactory, developers can enforce consistent thread creation behavior across their applications.
80. Explain thread-local variables in Java concurrency.
Ans:
Thread local variables in Java concurrency provide a means to attach data with a thread. Each thread accessing a thread-local variable retains its distinct copy of the variable’s value. Thread local variables typically harbor per-thread contextual data without explicit synchronization. They assure thread safety by precluding data sharing among threads.
81. How does ThreadLocal work in Java concurrency?
Ans:
ThreadLocal furnishes thread-specific variables in Java. Each thread accessing ThreadLocal obtains its unique instance. It is valuable for preserving individual thread context or state. It guarantees thread safety devoid of synchronization requirements. It prevents mutable state sharing across threads. It enhances performance by alleviating contention.
82. Describe the purpose of ForkJoinTask in Java concurrency.
Ans:
- ForkJoinTask facilitates the implementation of divide-and-conquer parallelism.
- Employed within ForkJoinPool for effective task management.
- Tasks are recursively divided into smaller subtasks.
- Utilizes multiple processors to exploit parallelism.
- It is particularly beneficial for tasks with substantial computational demands.
- Ensures equitable distribution of workloads.
83. Purpose of Phaser in Java concurrency?
Ans:
- Phaser aids in synchronizing threads across various phases.
- It permits the dynamic addition of threads to synchronization processes.
- Introduces advanced synchronization mechanisms.
- Supports coordination among multiple parties within a system.
- Facilitates synchronization patterns where threads wait for others.
- Particularly suitable for tasks with dynamically evolving dependencies.
84. Concept of parallel streams in Java concurrency.
Ans:
Parallel streams enable the simultaneous processing of collections. Tasks are divided and distributed across multiple threads. They harness the processing power of multi-core processors for speed. They simplify parallelism without explicit threading management. However, they require caution when dealing with shared mutable states. Parallel streams offer performance enhancements for handling large datasets.
85. Working of CompletableFuture in Java concurrency.
Ans:
- CompletableFuture represents the eventual result of asynchronous computations.
- It supports the chaining of dependent tasks for seamless execution.
- Enables composition of asynchronous operations for complex workflows.
- Provides robust mechanisms for handling errors during execution.
- Allows aggregation of multiple asynchronous results for streamlined processing.
- Enhances code readability and maintainability in asynchronous contexts.
86. Purpose of StampedLock in Java concurrency.
Ans:
- StampedLock furnishes optimistic read-write locks.
- Allows concurrent access for multiple readers and exclusive access for writers.
- Offers superior concurrency compared to traditional locking mechanisms.
- Reduces contention for read operations through optimistic locking.
- It is particularly effective in scenarios with a higher volume of reads than writes.
- Contributes to preventing situations of writer starvation.
87. Significance of Java.util.concurrent.atomic package?
Ans:
The Java.util.concurrent.atomic package offers thread-safe, lock-free operations. It provides atomic variables for precise concurrency control, facilitates the development of non-blocking algorithms suitable for high concurrency environments, ensures consistent and predictable behavior in concurrent executions, and mitigates risks associated with race conditions and synchronization overhead. It is essential for constructing high-performance concurrent applications.
88. Concept of thread starvation in Java concurrency.
Ans:
Thread starvation occurs when threads are unable to progress due to various reasons. It typically arises from unfair scheduling or resource contention within the system.Threads may remain indefinitely delayed from executing their tasks. This can result in performance degradation or even deadlock scenarios. Thread starvation can be addressed by employing appropriate synchronization and scheduling strategies. It impacts application responsiveness and overall throughput.
89. Working of CopyOnWriteArrayList in Java concurrency.
Ans:
- CopyOnWriteArrayList ensures thread safety during concurrent access operations.
- Copies of the underlying array are made before modifications occur.
- Reading operations are expedited and do not necessitate synchronization.
- Writing operations are slower due to the complete array copying process.
- Particularly suited for scenarios characterized by frequent reads and infrequent writes.
- Guarantees consistency and prevents ConcurrentModificationException.
90. Purpose of BlockingQueue interface in Java concurrency.
Ans:
- The BlockingQueue interface facilitates communication between producer and consumer threads.
- Offers blocking operations for efficient waiting and retrieval of data.
- Supports both bounded and unbounded queue implementations.
- Ensures thread safety during concurrent access to the queue.
- It alleviates the need for busy waiting, thus conserving CPU resources.
- Integral for implementing producer-consumer design patterns effectively.
91. Purpose of Exchanger class in Java concurrency.
Ans:
The Exchanger class facilitates thread communication by allowing two threads to exchange objects. It provides a synchronization point at which threads can pair and swap elements within a concurrent environment. This class is useful in scenarios where one thread produces data, and another consumes it, ensuring coordination. Both threads call the exchange() method, blocking until both have reached the exchange point.
92. Concept of memory consistency errors in Java concurrency.
Ans:
Memory consistency errors occur when threads have inconsistent views of shared data in a concurrent program. This inconsistency arises due to the reordering and caching optimizations performed by modern processors. Without proper synchronization mechanisms, changes made by one thread may not be visible to others immediately, leading to unexpected behavior and bugs in multithreaded applications.
93. Working of ConcurrentLinkedQueue in Java concurrency.
Ans:
- ConcurrentLinkedQueue is a non-blocking, thread-safe queue implementation in Java.
- It allows multiple threads to insert and remove elements concurrently without explicit synchronization.
- This is achieved through lock-free algorithms and CAS (Compare-And-Swap) operations.
- ConcurrentLinkedQueue provides high throughput by minimizing contention among threads.
- It offers better scalability compared to traditional synchronized queues in multithreaded environments.