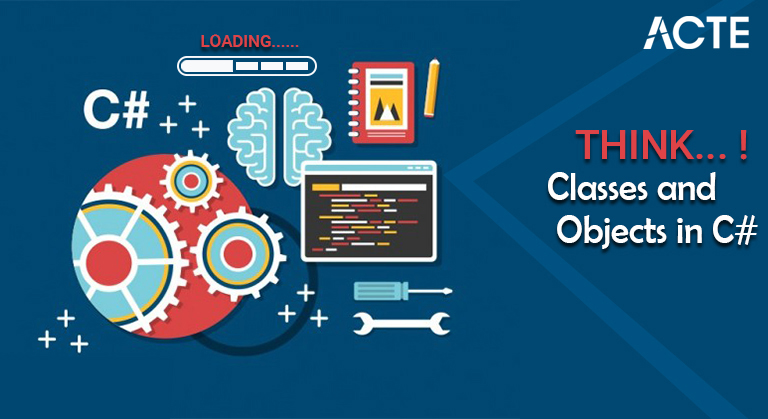
- Introduction Classes and objects
- About of C# Classes and objects
- C# objects and classes
- Create an object
- Classes And Objects In C#
- Multiple objects
- Object And Class Instance
- Class Members
- Using multiple classes
- Methods of C# objects and classes
- What Are Parameters?
- Class inheritance
- Create stores and withdrawals
- Conclusion
- Class and Object are the basic concepts of ObjectOriented Programming which revolve around the reallife entities. A class is a userdefined blueprint or prototype from which objects are created.
- Everything in C# is associated with classes and objects, along with its attributes and methods. Example: In real life, a car is an object. Cars have attributes such as weight and color, as well as methods such as propulsion and braking.
- Classes are like object constructors, or “blueprints” for creating objects. All data members and member functions of the class can be accessed using objects. No memory is allocated when the class is defined, but memory is allocated when it is instantiated (that is, when the object is created).
- In C#, the object is the actual entity. For example, chairs, cars, pens, cell phones, laptops, etc.
- In other words, an object is an entity with state and behavior. Here, state means data and behavior means function.
- Objects are run-time entities and are created at run time.
- The object is an instance of the class. All members of the class can be accessed through the object.
- Let’s look at an example of creating an object with the new keyword.
- Student s1 = new Student (); // Create an object for Student
- In this example, Student is a type and s1 is a reference variable that points to an instance of the Student class. The new keyword allocates memory at run time.
- In C#, a class is a group of similar objects. This is the template in which the object will be created. It can contain fields, methods, constructors, and so on. Let’s look at an example of a C# class that has only two fields.
- When a variable is declared directly in a class, it is often called a field (or attribute).
- Although not required, it is recommended that you start with a capital letter when naming your class. Also, to keep your code organized, it’s common for C# files and class names to match. However, this is not required (as in Java).
- cases
- Create an object named “myObj” and use it to return a color value.
- class Car
- {
- string color = “red”;
- static void Main (string [] args)
- {
- Car myObj = new Car ();
- Console.WriteLine (myObj.color);
- }
- }
- An article in a programming language is like a genuine item. Object-Oriented Programming is an idea where the projects are planned utilizing sets of classes and has a problem with to work on program advancement and support.
- A class is an intelligent assortment of comparable sorts of articles. It is one of the most major sorts in C#. It is an information structure that is a mix of Methods, Functions, and Fields. It characterizes the powerful occurrences for example objects that should be made for the class.
- Class car
- {
- string color = “red”;
- static void Main (string [] args)
- {
- Car myObj1 = new Car ();
- car myObj2 = new car ();
- Console.WriteLine (myObj1.color)
- Console.WriteLine (myObj2.color);
- }
- }
- Understudy stu = new Student();
- prog2.cs
- prog.cs
- prog2.cs
- Klasse Auto
- {
- public string color = “red”;
- }
- prog.cs
- Class program
- {
- static void Main (string [] args)
- {
- Auto myObj = new Car ();
- Console.WriteLine (myObj.color);
- }
- }
- Student s1 = new student (); // Create an object for Student
- Reference Parameters
- Esteem Parameters
- Yield Parameters
- Exhibit Parameters
- Public class student
- {
- int id; // Field or data member
- string name; // Field or data member
- }
- When a category broadcasts a base elegance, it inherits all of the individuals of the bottom elegance besides the constructors. For greater information, see Inheritance.
- A elegance in C# can most effective without delay inherit from one base elegance. However, due to the fact a base elegance can also additionally itself inherit from some other elegance, a category can also additionally not directly inherit more than one base lessons. Furthermore, a category can without delay put into effect one or greater interfaces. For greater information, see Interfaces.
- A elegance may be declared summary. An summary elegance carries summary techniques which have a signature definition however no implementation. Abstract lessons can’t be instantiated. They can most effective be used via derived lessons that put into effect the summary techniques. By contrast, a sealed elegance does now no longer permit different lessons to derive from it. For greater information, see Abstract and Sealed Classes and Class Members.
- public elegance Manager : Employee
- {
- // Employee fields, properties, techniques and activities are inherited
- // New Manager fields, properties, techniques and activities pass here…
- }
- A method is a member of a class that implements a logical or computational action performed by an object or class. Static methods, if defined, are accessed through the class, and all instance methods are accessed using instances of the class. The method can also contain parameters that indicate that a variable reference is passed to the method.
- The method can also include a return type that specifies the calculated final product / value of the returned method. Here are some things to keep in mind when working with the method: If the method does not return a value, the return type must be invalid. The method signature or name must be unique within the class. The signature of a method means the name of the method, parameters, qualifiers, and the data type of the parameters.
- Reference parameter
- Value parameter
- Output parameters
- Array parameters
- Let’s take a closer look. If you want to pass arguments by reference, the reference parameter is used. That is, the argument passed as a parameter to the method is a single-valued variable and must represent the location of the value of the running variable.
- The value parameter is used to pass the input value as an argument. The value parameter refers to a local variable that is passed as an argument as an initial value and then as a parameter.
- customer object3 = new customer ();
- Customer object4 = object3;
- Your ledger class needs to acknowledge stores and withdrawals to work accurately. How about we carry out stores and withdrawals by making a diary of each exchange for the record. That enjoys a couple of upper hands over basically refreshing the equilibrium on every exchange. The set of experiences can be utilized to review all exchanges and oversee day by day adjusts. By figuring the equilibrium from the historical backdrop of all exchanges when required, any mistakes in a solitary exchange that are fixed will be accurately thought about yet to be determined the following calculation.
- How about we start by making another sort to address an exchange. This is a basic sort that doesn’t have any liabilities. It needs a couple of properties. Make another record named Transaction. cs. Add the accompanying code to it:
- Presently, how about we add a List<'T> of Transaction objects to the BankAccount class. Add the accompanying statement after the constructor in your BankAccount.cs document:
- private List<'Transaction> allTransactions = new List<'Transaction>();
Introduction Classes and objects
From the previous chapter, we learned that C# is an object-oriented programming language. All C# maps to classes and objects, along with their attributes and methods. Example: In real life, a car is an object. Cars have attributes such as weight and color, as well as methods such as propulsion and braking. The class is like an object constructor or “blueprint” for creating objects.
About
C# objects and classes
Because C# is an object-oriented language, programs are designed using C# objects and classes.
C# object
C# class
Create an object
Objects are created from the class. We have already created a class called Car, which we can use to create objects. To create a car object, specify the object name after the class name and use the keyword new.
Note that we are using the dot (.) Syntax to access the variables / fields in the class (myObj.color). You will learn more about the field in the next chapter.
Classes And Objects In C#
For Example in the event that you are gathering information for an understudy in an application. There can be a few properties of an understudy like a roll number, class, segment, subject, and so forth Every one of these properties can be named a property of understudy class and the understudy can be considered as an assortment of this multitude of properties.
In this way, here a class addresses an understudy for example the assortment of articles and understudy credits/properties can be named as its items. We will concentrate on these exhaustively in impending subjects.
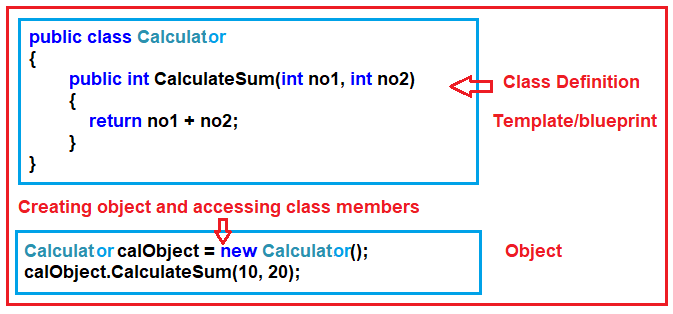
Multiple objects
You can create multiple objects in a class:
Example: Create two car objects.
Object And Class Instance
In some cases the terms class and item are utilized conversely yet both are various substances. A class is the meaning of the article however it’s not simply the item. The article is otherwise called an occasion of the class. Class examples are made by utilizing the “new” administrator. Another administrator dispenses memory for an occasion and summons a constructor to introduce it and returns a reference object.
For Example, to make a case for the class understudy then
Here we have made a case of class “Understudy” and we have characterized “stu” as a source of perspective item.
Class Members
Individuals from a class can be of static or case type. Case individuals are a piece of the item though static individuals are a piece of the class.
Let’s view a portion of the class members:
Fields: Variables present inside a class are called fields.
Constants: Constant qualities present inside the class.
Methods: Logical activities performed by the class.
Constructors: Required to introduce the class or example of the class.
Using multiple classes
You can also create an object of one class and access it in another class. This is often used to better organize a class (one class has all the fields and methods, the other has the Main () (code to execute) method).
C# Objects and Classes
Because C# is an objectoriented language, programs are designed using C# objects and classes.
C# object
In C#, the object is the actual entity. For example, chairs, cars, pens, cell phones, laptops, etc. In other words, an object is an entity with state and behavior. Here, state means data and behavior means function. Objects are run-time entities and are created at run time. The object is an instance of the class. All members of the class can be accessed through the object. Let’s look at an example of creating an object using the new keyword.
Methods
Strategies are the individuals from the class that execute a coherent or computational activity that is intended to be performed by the article or the class. Static techniques in the event that characterized are gotten to through the class and all the case strategies are gotten to utilizing an occurrence of the class. Techniques can likewise contain boundaries that imply variable references being passed to the strategy. A technique may likewise contain a return type that means the computational final result/worth of the strategy that can be returned.
Some focuses to recollect while working with the strategy are:
On the off chance that a technique doesn’t return any worth then the return types should be void. The mark or name of the technique ought to be interesting inside a class. The mark of a technique implies the name of the strategy alongside the Parameters, Modifiers, and Data sort of the Parameters.
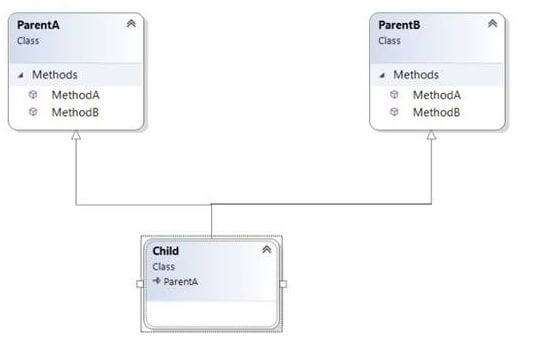
What Are Parameters?
Boundaries are the qualities or reference factors that are passed to the technique. Boundaries accept their qualities from contentions that are determined while summoning a specific technique.
Different Types Of Parameters Are:
In a large portion of our models, we will utilize either reference type or worth sort.
How about we check out these exhaustively?
A reference parameter is utilized when you need to pass a contention by means of reference. This implies that the contention pass as a boundary to the technique should be a variable with a worth and during execution, it ought to address the capacity area of the worth of the variable.
The esteem parameter is utilized to pass the info esteems to a contention. The worth boundary alludes to a nearby factor that is passed as an underlying worth to the contention that is then passed as the boundary.
C# Class In
In this example, Student is a type and s1 is a reference variable that points to an instance of the Student class. The new keyword allocates memory at run time. C#, a class is a group of similar objects. This is the template in which the object will be created. It can contain fields, methods, constructors, and so on. Let’s look at an example of a C# class that has only two fields.
C# Object and class example
Example of class with two fields id and name Let’s look at. Create an instance of the class, initialize the object, and output the object value.
Class inheritance
Classes absolutely guide inheritance, a essential function of object-orientated programming. When you create a category, you may inherit from every other elegance that isn’t always described as sealed, and different lessons can inherit out of your elegance and override elegance digital techniques. Furthermore, you may put into effect one or greater interfaces.
Inheritance is achieved via way of means of the use of a derivation, this means that a category is asserted via way of means of the use of a base elegance from which it inherits information and behavior. A base elegance is targeted via way of means of appending a colon and the call of the bottom elegance following the derived elegance call, like this:
Method
What are parameters?
A parameter is a value or reference variable passed to a method. The parameter gets its value from the arguments provided when calling a particular method.
The various types of parameters are:
Most examples use either reference or value types
Create an object
Sometimes used interchangeably, but classes and objects are different. The class defines the object type, but not the object itself. An object is a concrete entity based on a class, sometimes called an instance of the class. A object can be created using the new keyword followed by the name of the class on which the object is based, as follows:
This code creates two object references, both pointing to the same object. Therefore, any changes made to the object by object3 will be reflected in subsequent use of object4. Classes are called reference types because objects that are based on a class are referenced by reference.
Create stores and withdrawals
Prerequisites
The instructional exercise expects that you have a machine set up for neighborhood improvement. On Windows, Linux, or macOS, you can utilize the .NET CLI to make, fabricate, and run applications. On Windows, you can utilize Visual Studio 2019.
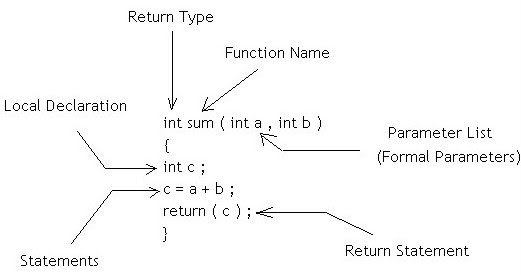
Conclusion
C# shares a significant number of the elements of other item arranged dialects, like C++. C# incorporates support for virtual techniques, theoretical classes, and strategy over-burdening. C# offers extra highlights that advance more reusable and more strong classes, for example, a predominant model for abrogating techniques in determined classes and a better special case taking care of model.
Part 3 presents and talks about esteem types and references. We’ll inspect the significant contrasts between esteem types and references, including object lifetime, stockpiling, and trash assortment. Part 3 likewise examines properties and how they can make your classes more easy to understand.