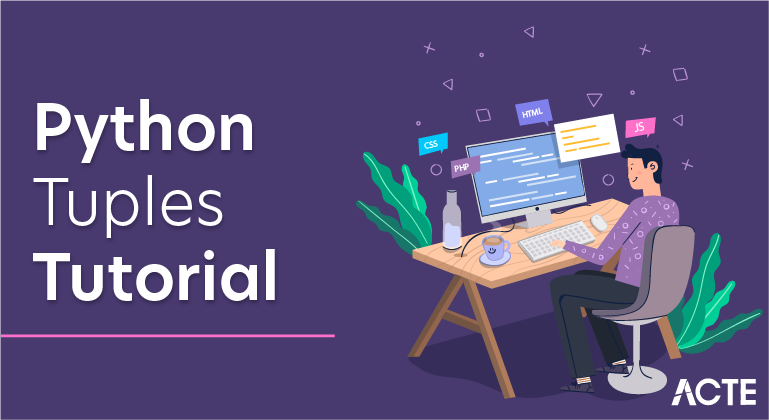
A tuple is a collection of objects which are ordered and immutable. Tuples are sequences, just like lists. The differences between tuples and lists are, the tuples cannot be changed unlike lists and tuples use parentheses, whereas lists use square brackets.Creating a tuple is as simple as putting different comma-separated values. Optionally you can put these comma-separated values between parentheses also. For example:
- tup1 = (‘physics’, ‘chemistry’, 1997, 2000);
- tup2 = (1, 2, 3, 4, 5 );
- tup3 = “a”, “b”, “c”, “d”;
The empty tuple is written as two parentheses containing nothing:
tup1 = ();
To write a tuple containing a single value you have to include a comma, even though there is only one value:
tup1 = (50,);
Like string indices, tuple indices start at 0, and they can be sliced, concatenated, and so on.
Accessing Values in Tuples:
To access values in tuple, use the square brackets for slicing along with the index or indices to obtain values available at that index. For example :
- #!/usr/bin/python
- tup1 = (‘physics’, ‘chemistry’, 1997, 2000);
- tup2 = (1, 2, 3, 4, 5, 6, 7 );
- print “tup1[0]: “, tup1[0];
- print “tup2[1:5]: “, tup2[1:5];
When the above code is executed, it produces the following result :
- tup1[0]: physics
- tup2[1:5]: [2, 3, 4, 5]
Updating Tuples:
Tuples are immutable which means you cannot update or change the values of tuple elements. You are able to take portions of existing tuples to create new tuples as the following example demonstrates:
- #!/usr/bin/python
- tup1 = (12, 34.56);
- tup2 = (‘abc’, ‘xyz’);
- # Following action is not valid for tuples
- # tup1[0] = 100;
- # So let’s create a new tuple as follows
- tup3 = tup1 + tup2;
- print tup3;
- #!/usr/bin/python
- tup1 = (12, 34.56);
- tup2 = (‘abc’, ‘xyz’);
- # Following action is not valid for tuples
- # tup1[0] = 100;
- # So let’s create a new tuple as follows
- tup3 = tup1 + tup2;
- print tup3;
When the above code is executed, it produces the following result:
(12, 34.56, ‘abc’, ‘xyz’)
When the above code is executed, it produces the following result:
(12, 34.56, ‘abc’, ‘xyz’)
Delete Tuple Elements:
Removing individual tuple elements is not possible. There is, of course, nothing wrong with putting together another tuple with the undesired elements discarded.
To explicitly remove an entire tuple, just use the del statement. For example
- #!/usr/bin/python
- tup = (‘physics’, ‘chemistry’, 1997, 2000);
- print tup;
- del tup;
- print “After deleting tup : “;
- print tup;
This produces the following result. Note an exception raised, this is because after del tup tuple does not exist any more −
- (‘physics’, ‘chemistry’, 1997, 2000)
- After deleting tup :
- Traceback (most recent call last):
- File “test.py”, line 9, in <module>
- print tup;
- NameError: name ‘tup’ is not defined
Basic Tuples Operations:
- Tuples respond to the + and * operators much like strings; they mean concatenation and repetition here too, except that the result is a new tuple, not a string.
- In fact, tuples respond to all of the general sequence operations we used on strings in the prior chapter
Python Expression | Results | Description |
---|---|---|
len((1, 2, 3)) | 3 | Length |
(1, 2, 3) + (4, 5, 6) | (1, 2, 3, 4, 5, 6) | Concatenation |
(‘Hi!’,) * 4 | (‘Hi!’, ‘Hi!’, ‘Hi!’, ‘Hi!’) | Repetition |
3 in (1, 2, 3) | True | Membership |
for x in (1, 2, 3): print x, | 1 2 3 | Iteration |
Indexing, Slicing, and Matrixes:
Because tuples are sequences, indexing and slicing work the same way for tuples as they do for strings. Assuming following input:L = (‘spam’, ‘Spam’, ‘SPAM!’)
Python Expression | Results | Description |
---|---|---|
L[2] | ‘SPAM!’ | Offsets start at zero |
No Enclosing Delimiters:
Any set of multiple objects, comma-separated, written without identifying symbols, i.e., brackets for lists, parentheses for tuples, etc., default to tuples, as indicated in these short examples:
- #!/usr/bin/python
- print ‘abc’, -4.24e93, 18+6.6j, ‘xyz’;
- x, y = 1, 2;
- print “Value of x , y : “, x,y;
When the above code is executed, it produces the following result −
- abc -4.24e+93 (18+6.6j) xyz
- Value of x , y : 1 2
Built-in Tuple Functions:
Python includes the following tuple functions
Function | Description |
---|---|
cmp(tuple1, tuple2) | Compares elements of both tuples. |
len(tuple) | Gives the total length of the tuple. |
max(tuple) | Returns item from the tuple with max value. |
min(tuple) | Returns item from the tuple with min value. |
Python – Dictionary :
- Each key is separated from its value by a colon (:), the items are separated by commas, and the whole thing is enclosed in curly braces. An empty dictionary without any items is written with just two curly braces, like this: {}.
- Keys are unique within a dictionary while values may not be. The values of a dictionary can be of any type, but the keys must be of an immutable data type such as strings, numbers, or tuples.
Accessing Values in Dictionary:
To access dictionary elements, you can use the familiar square brackets along with the key to obtain its value. Following is a simple example:
- #!/usr/bin/python
- dict = {‘Name’: ‘Zara’, ‘Age’: 7, ‘Class’: ‘First’}
- print “dict[‘Name’]: “, dict[‘Name’]
- print “dict[‘Age’]: “, dict[‘Age’]
When the above code is executed, it produces the following result:
- dict[‘Name’]: Zara
- dict[‘Age’]: 7
If we attempt to access a data item with a key, which is not part of the dictionary, we get an error as follows:
- #!/usr/bin/python
- dict = {‘Name’: ‘Zara’, ‘Age’: 7, ‘Class’: ‘First’}
- print “dict[‘Alice’]: “, dict[‘Alice’]
When the above code is executed, it produces the following result:
- dict[‘Age’]: 7
If we attempt to access a data item with a key, which is not part of the dictionary, we get an error as follows:
- #!/usr/bin/python
- dict = {‘Name’: ‘Zara’, ‘Age’: 7, ‘Class’: ‘First’}
- print “dict[‘Alice’]: “, dict[‘Alice’]
When the above code is executed, it produces the following result:
- dict[‘Alice’]:
- Traceback (most recent call last):
- File “test.py”, line 4, in <module>
- print “dict[‘Alice’]: “, dict[‘Alice’];
- KeyError: ‘Alice’
Updating Dictionary:
You can update a dictionary by adding a new entry or a key-value pair, modifying an existing entry, or deleting an existing entry as shown below in the simple example:
- #!/usr/bin/python
- dict = {‘Name’: ‘Zara’, ‘Age’: 7, ‘Class’: ‘First’}
- dict[‘Age’] = 8; # update existing entry
- dict[‘School’] = “DPS School”; # Add new entry
- print “dict[‘Age’]: “, dict[‘Age’]
- print “dict[‘School’]: “, dict[‘School’]
When the above code is executed, it produces the following result
- dict[‘Age’]: 8
- dict[‘School’]: DPS School
Delete Dictionary Elements:
You can either remove individual dictionary elements or clear the entire contents of a dictionary. You can also delete the entire dictionary in a single operation.
To explicitly remove an entire dictionary, just use the del statement. Following is a simple example:
- #!/usr/bin/python
- dict = {‘Name’: ‘Zara’, ‘Age’: 7, ‘Class’: ‘First’}
- del dict[‘Name’]; # remove entry with key ‘Name’
- dict.clear(); # remove all entries in dict
- del dict ; # delete entire dictionary
- print “dict[‘Age’]: “, dict[‘Age’]
- print “dict[‘School’]: “, dict[‘School’]
This produces the following result. Note that an exception is raised because after del dict dictionary does not exist any more:
- dict[‘Age’]:
- Traceback (most recent call last):
- File “test.py”, line 8, in <module>
- print “dict[‘Age’]: “, dict[‘Age’];
- TypeError: ‘type’ object is unsubscriptable
- Note − del() method is discussed in subsequent sections.
Properties of Dictionary Keys:
Dictionary values have no restrictions. They can be any arbitrary Python object, either standard objects or user-defined objects. However, the same is not true for the keys.
There are two important points to remember about dictionary keys:
(a) More than one entry per key not allowed. Which means no duplicate key is allowed. When duplicate keys are encountered during assignment, the last assignment wins. For example:
- #!/usr/bin/python
- dict = {‘Name’: ‘Zara’, ‘Age’: 7, ‘Name’: ‘Manni’}
- print “dict[‘Name’]: “, dict[‘Name’]
When the above code is executed, it produces the following result
- dict[‘Name’]: Manni
(b) Keys must be immutable. Which means you can use strings, numbers or tuples as dictionary keys but something like [‘key’] is not allowed. Following is a simple example:
- #!/usr/bin/python
- dict = {[‘Name’]: ‘Zara’, ‘Age’: 7}
- print “dict[‘Name’]: “, dict[‘Name’]
When the above code is executed, it produces the following result:
- #
- Traceback (most recent call last):
- File “test.py”, line 3, in <module>
- dict = {[‘Name’]: ‘Zara’, ‘Age’: 7};
- TypeError: unhashable type: ‘list’
Built-in Dictionary Functions & Methods:
Python includes the following dictionary functions :
Function | Description |
---|---|
cmp(dict1, dict2) | Compares elements of both dict. |
len(dict) | Gives the total length of the dictionary. This would be equal to the number of items in the dictionary. |
Python includes following dictionary methods :
Methods | Description |
dict.clear() | Removes all elements of dictionary dict |
dict.copy() | Returns a shallow copy of dictionary dict |
dict.fromkeys() | Create a new dictionary with keys from seq and values set to value. |
dict.get(key, default=None) | For key key, returns value or default if key not in dictionary |
dict.has_key(key) | Returns true if key in dictionary dict, false otherwise |
dict.items() | Returns a list of dicts (key, value) tuple pairs |
Python – Date & Time:
A Python program can handle date and time in several ways. Converting between date formats is a common chore for computers. Python’s time and calendar modules help track dates and times.
What is Tick?
Time intervals are floating-point numbers in units of seconds. Particular instants in time are expressed in seconds since 12:00am, January 1, 1970(epoch).There is a popular time module available in Python which provides functions for working with times, and for converting between representations. The function time.time() returns the current system time in ticks since 12:00am, January 1, 1970(epoch).
Example
- #!/usr/bin/python
- import time; # This is required to include a time module.
- ticks = time.time()
- print “Number of ticks since 12:00am, January 1, 1970:”, ticks
This would produce a result something as follows:
Number of ticks since 12:00am, January 1, 1970: 7186862.73399
Date arithmetic is easy to do with ticks. However, dates before the epoch cannot be represented in this form. Dates in the far future also cannot be represented this way the cutoff point is sometime in 2038 for UNIX and Windows.
What is TimeTuple?
Many of Python’s time functions handle time as a tuple of 9 numbers, as shown below:
Index | Field | Values |
0 | 4-digit year | 2008 |
1 | Month | 1 to 12 |
2 | Day | 1 to 31 |
3 | Hour | 0 to 23 |
The above tuple is equivalent to struct_time structure. This structure has following attributes:
Index | Attributes | Values |
0 | tm_year | 2008 |
1 | tm_mon | 1 to 12 |
2 | tm_mday | 1 to 31 |
3 | tm_hour | 0 to 23 |
4 | tm_min | 0 to 59 |
5 | tm_sec | 0 to 61 (60 or 61 are leap-seconds) |
6 | tm_wday | 0 to 6 (0 is Monday) |
7 | tm_yday | 1 to 366 (Julian day) |
8 | tm_isdst | -1, 0, 1, -1 means library determines DST |
Getting current time:
To translate a time instant from a seconds since the epoch floating-point value into a time-tuple, pass the floating-point value to a function (e.g., localtime) that returns a time-tuple with all nine items valid.
- #!/usr/bin/python
- import time;
- localtime = time.localtime(time.time())
- print “Local current time :”, localtime
This would produce the following result, which could be formatted in any other presentable form −
Local current time : time.struct_time(tm_year=2013, tm_mon=7,
tm_mday=17, tm_hour=21, tm_min=26, tm_sec=3, tm_wday=2, tm_yday=198, tm_isdst=0)
Getting formatted time:
You can format any time as per your requirement, but simple method to get time in readable format is asctime()
- #!/usr/bin/python
- import time;
- localtime = time.asctime( time.localtime(time.time()) )
- print “Local current time :”, localtime
- This would produce the following result:
- Local current time : Tue Jan 13 10:17:09 2009
Getting calendar for a month:
The calendar module gives a wide range of methods to play with yearly and monthly calendars. Here, we print a calendar for a given month ( Jan 2008 ) −
- #!/usr/bin/python
- import calendar
- cal = calendar.month(2008, 1)
- print “Here is the calendar:”
- print cal
This would produce the following result:
Here is the calendar:
- January 2008
- Mo Tu We Th Fr Sa Su
- 1 2 3 4 5 6
- 9 10 11 12 13
- 14 15 16 17 18 19 20
- 21 22 23 24 25 26 27
- 28 29 30 31
The time Module
There is a popular time module available in Python which provides functions for working with times and for converting between representations. Here is the list of all available methods:
Function | Description |
---|---|
time.altzone | The offset of the local DST timezone, in seconds west of UTC, if one is defined. This is negative if the local DST timezone is east of UTC (as in Western Europe, including the UK). Only use this if daylight is nonzero. |
time.asctime([tupletime]) | Accepts a time-tuple and returns a readable 24-character string such as ‘Tue Dec 11 18:07:14 2008’. |
time.clock( ) | Returns the current CPU time as a floating-point number of seconds. To measure computational costs of different approaches, the value of time.clock is more useful than that of time.time(). |
time.ctime([secs]) | Like asctime(localtime(secs)) and without arguments is like asctime( ) |
time.gmtime([secs]) | converts a time expressed in seconds since the epoch to a struct_time in UTC in which the dst flag is always zero. If secs is not provided or None, the current time as returned by time() is used. |
Let us go through the functions briefly
There are following two important attributes available with time module:
Attribute | Description |
---|---|
time.timezone | Attribute time.timezone is the offset in seconds of the local time zone (without DST) from UTC (>0 in the Americas; <=0 in most of Europe, Asia, Africa). |
time.tzname | Attribute time.tzname is a pair of locale-dependent strings, which are the names of the local time zone without and with DST, respectively. |
The calendar Module:
The calendar module supplies calendar-related functions, including functions to print a text calendar for a given month or year. By default, the calendar takes Monday as the first day of the week and Sunday as the last one. To change this, call calendar.setfirstweekday() function.Here is a list of functions available with the calendar module.
Conclusion:
We can conclude that although both lists and tuples are data structures in Python, there are remarkable differences between the two, with the main difference being that lists are mutable while tuples are immutable. A list has a variable size while a tuple has a fixed size.