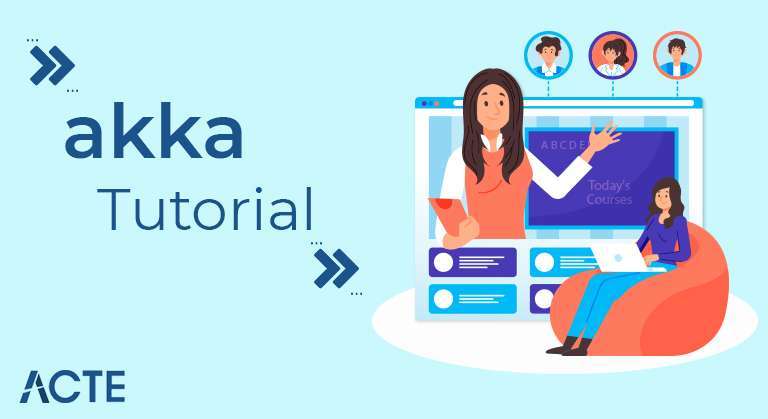
Akka is a open-source library or a toolkit. It is used to create concurrent, distributed and fault-tolerant application. You can integrate this library into any JVM(Java Virtual Machine) support language. In this tutorial, we will integrate this into Scala programming language.
Akka is written in Scala. It implements Actor Based Model. The Actor Model provides a higher level of abstraction for writing concurrent and distributed applications. It helps to developer to deals with explicit locking and thread management. Akka makes it easier to write correct concurrent and parallel application.
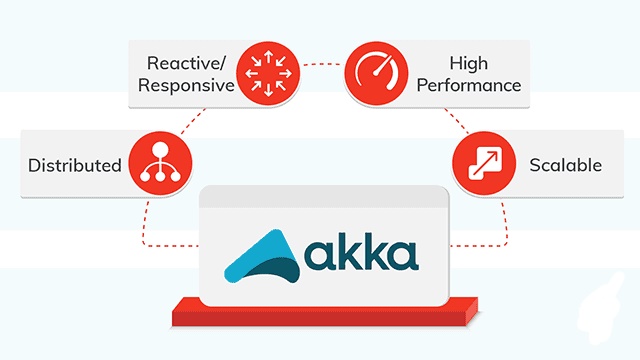
Module
- Akka is a suite of modules which allows you to build distributed and reliable systems by leaning on the actor model.
- The actor model puts emphasis on avoiding the use of locks in your system, in favour of parallelism and concurrency. As a result, actors are those ‘things’ that would ‘react’ to messages, and perhaps run some computation and/or respond to another actor via message passing. The actor model has been around for a while, and was certainly made popular by languages such as Erlang.
- The Akka eco-system has evolved fairly rapidly over the past few years. Below is a quick overview of the various Akka modules for which we will provide code snippets:
Akka Actor: This module introduces the Actor System, functions and utilities that Akka provides to support the actor model and message passing. For additional information, you can refer to the official Akka documentation on Actors.
Akka HTTP: As the name implies, this module is typically best suited for middle–tier applications which require an HTTP endpoint. As an example, you could use Akka HTTP to expose a REST endpoint that interfaces with a storage layer such as a database. For additional information, you can refer to the official Akka documentation on Akka HTTP.
Akka Streams: This module is useful when you are working on data pipelines or even stream processing. For additional information, you can refer to the official Akka documentation on Akka Streams.
Akka Networking: This module provides the foundation for having actor systems being able to connect to each other remotely over some predefined network transport such as TCP. For additional information, you can refer to the official Akka documentation on Akka Networking.
Akka Clustering: This module is an extension of the Akka Networking module. It is useful in scaling distributed applications by have actors form a quorum and work together by some predefined membership protocol. For additional information, you can refer to the official Akka documentation on Akka Clustering.
Project setup build.sbt: As a reminder, we will be using the latest version of Akka, which is 2.5.12, as per this writing. Below is a code snippet of the library dependencies in your build.sbt to import the Akka modules. Note that we’re also making use of Scala 2.12.
- scalaVersion := “2.12.4”
- libraryDependencies ++= Seq(
- “com.typesafe.akka” %% “akka-actor” % “2.5.12”,
- “com.typesafe.akka” %% “akka-testkit” % “2.5.12” % Test
- )
Uses
- Akka handles all of the underlying threading complexities by allowing you to just focus on dividing your tasks into actors, defining messages to pass among actors, and wiring together their communication logic.
- The real power of Akka is realized when you distribute actors across multiple machines. You could spin off hundreds of Akka servers to run your actors, and the Akka toolkit seamlessly handles the distribution and communication of the messages among the actors.
Setup:
Step 1: Create a simple Java based Maven project. “-B” is to run the maven archetype plugin in non-interactive mode. In order to learn how to set up maven, look at Setting up Java, Maven, and Eclipse.
c:\projects> mvn archetype:generate -DgroupId=com.mytutorial -DartifactId=simple-akka -B
Step 2: Import the “simple-akka” into eclipse.
File –> Import –> “Existing Maven Projects” –> “select the pom.xml” file that gets created from the step 1 in the folder “c:\projects\simple-akka”.
Step 3: Open the “pom.xml” file within the imported project and add the “akka-actor_2.10” library as dependency.
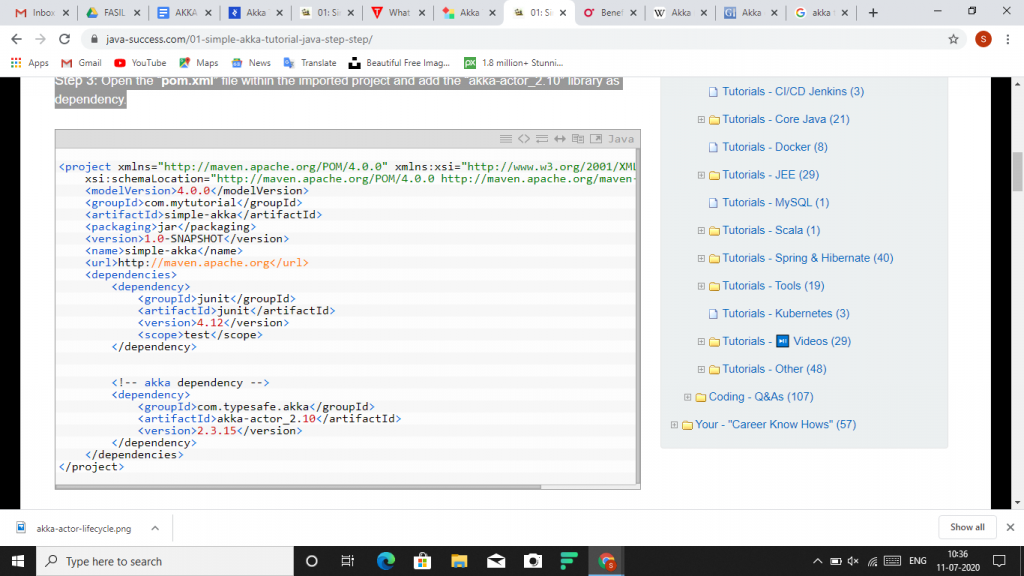
Step 4: Let’s write a very simple Akka example where a “Producer” creates numbers from 1 to 10, and a “Consumer”, receives these numbers and just print them. The “Producer” and “Consumer” are asynchronous.
Producer.java in package com.mytutorial
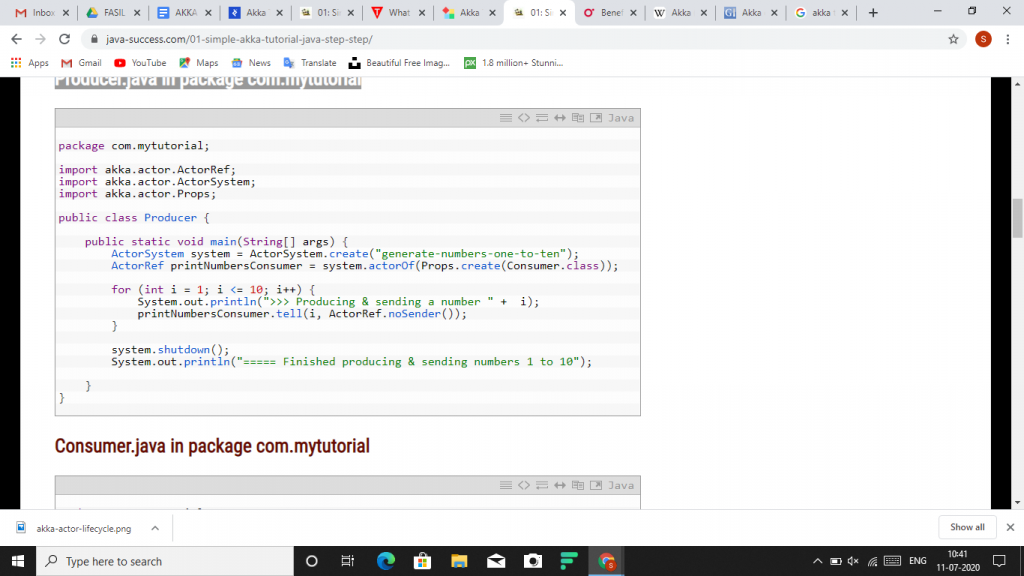
Consumer.java in package com.mytutorial
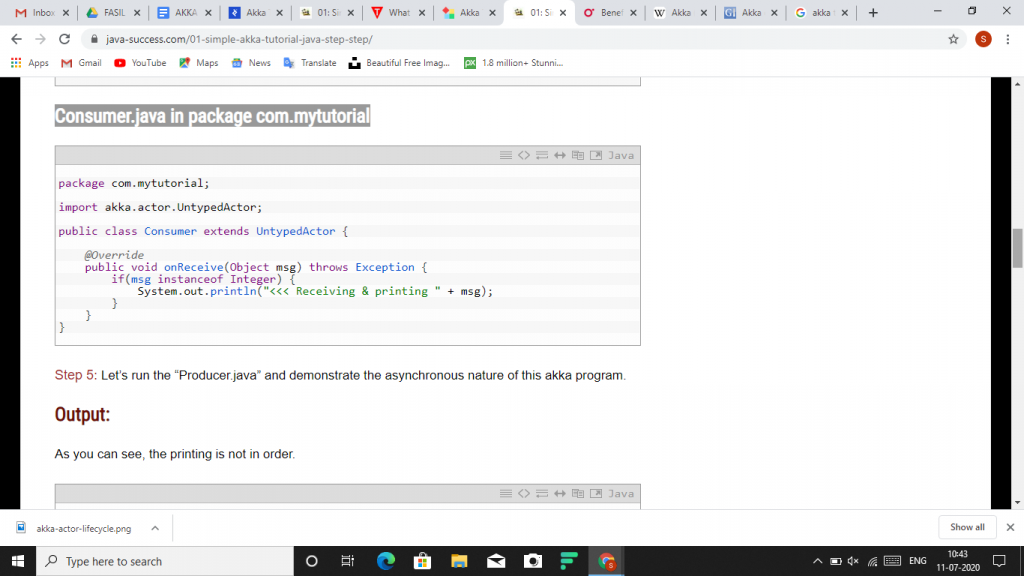
Step 5: Let’s run the “Producer.java” and demonstrate the asynchronous nature of this akka program.
Output:
As you can see, the printing is not in order.
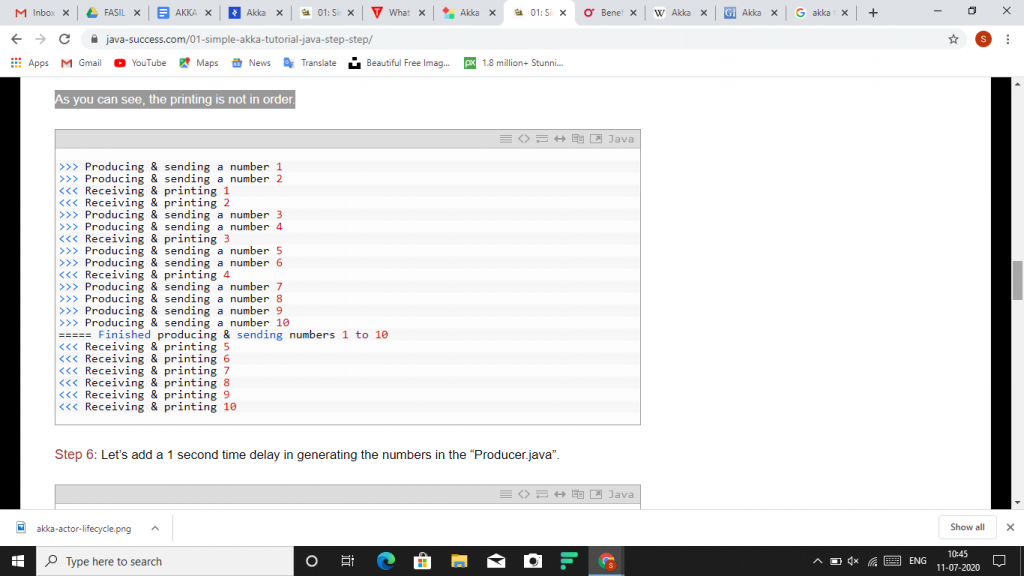
Step 6: Let’s add a 1 second time delay in generating the numbers in the “Producer.java”.
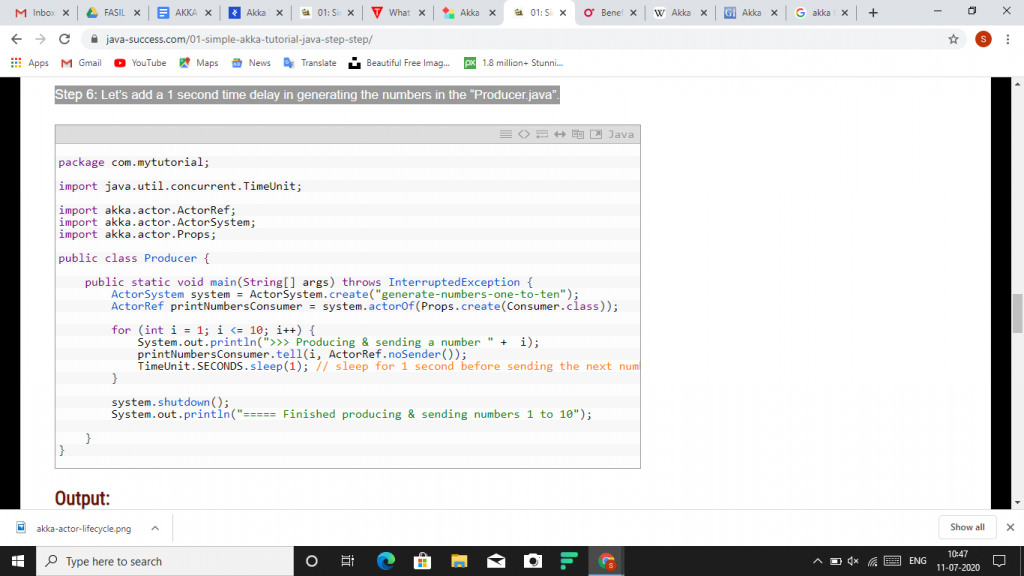
Output:
Now, you get the output
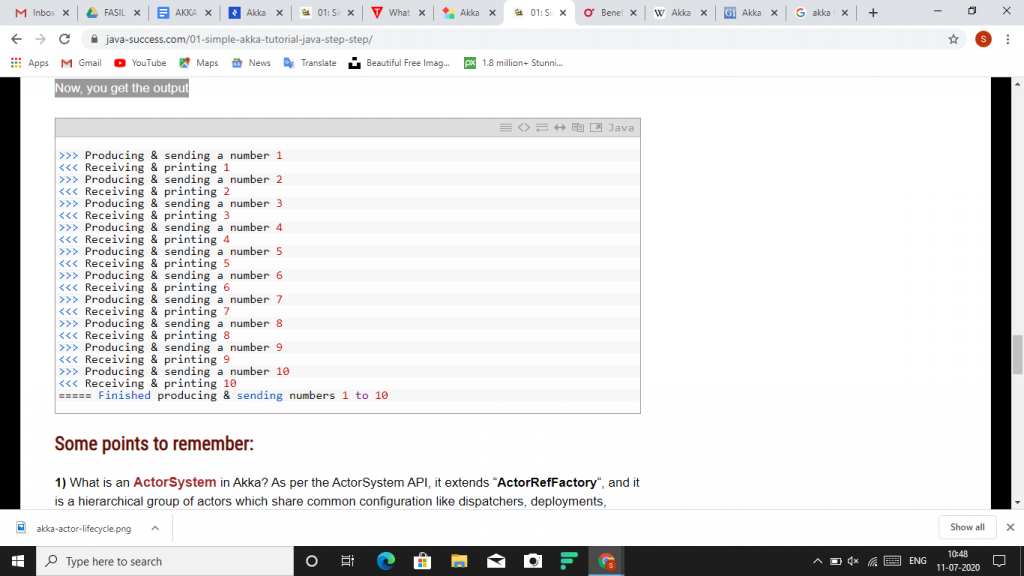
- Akka strictly adheres to the The Reactive Manifesto. Reactive applications aim at replacing traditional multi threaded applications with an architecture that satisfies one or more of the following requirements:
- Event-driven: Using Actors, one can write code that handles requests asynchronously and employs non-blocking operations exclusively.
- Scalable: In Akka, adding nodes without having to modify the code is possible, thanks both to message passing and location transparency.
- Resilient: Any application will encounter errors and fail at some point in time. Akka provides “supervision” (fault tolerance) strategies to facilitate a self-healing system.
- Responsive: Many of today’s high performance and rapid response applications need to give quick feedback to the user and therefore need to react to events in an extremely timely manner. Akka’s non-blocking, message-based strategy helps achieve this.
What is the Akka Framework?
This post introduces Akka and explores the ways in which it facilitates and simplifies the implementation of concurrent, distributed applications.
An Actor in Akka
- An actor is essentially nothing more than an object that receives messages and takes actions to handle them. It is decoupled from the source of the message and its only responsibility is to properly recognize the type of message it has received and take action accordingly.
- Upon receiving a message, an actor may take one or more of the following actions:
- Execute some operations itself (such as performing calculations, persisting data, calling an external web service, and so on)
- Forward the message, or a derived message, to another actor
- Instantiate a new actor and forward the message to it
- Alternatively, the actor may choose to ignore the message entirely (i.e., it may choose inaction) if it deems it appropriate to do so.
- To implement an actor, it is necessary to extend the akka.actor.Actor trait and implement the receive method. An actor’s receive method is invoked (by Akka) when a message is sent to that actor. Its typical implementation consists of pattern matching, as shown in the following Akka example, to identify the message type and react accordingly:
- import akka.actor.Actor
- import akka.actor.Props
- import akka.event.Logging
- class MyActor extends Actor {
- def receive = {
- case value: String => doSomething(value)
- case _ => println(“received unknown message”)
- }
- }
- Pattern matching is a relatively elegant technique for handling messages, which tends to produce “cleaner” and easier-to-navigate code than a comparable implementation based on callbacks. Consider, for example, a simplistic HTTP request/response implementation.
- First, let’s implement this using a callback-based paradigm in JavaScript:
- route(url, function(request){
- var query = buildQuery(request);
- dbCall(query, function(dbResponse){
- var wsRequest = buildWebServiceRequest(dbResponse);
- wsCall(wsRequest, function(wsResponse) {
- sendReply(wsResponse);
- });
- });
- });
Now let’s compare this to a pattern-matching-based implementation:
- msg match {
- case HttpRequest(request) => {
- val query = buildQuery(request)
- dbCall(query)
- }
- case DbResponse(dbResponse) => {
- var wsRequest = buildWebServiceRequest(dbResponse);
- wsCall(dbResponse)
- }
- case WsResponse(wsResponse) => sendReply(wsResponse)
- }
The Actor System
- Taking a complex problem and recursively splitting it into smaller sub-problems is a sound problem solving technique in general. This approach can be particularly beneficial in computer science (consistent with the Single Responsibility Principle), as it tends to yield clean, modularized code, with little or no redundancy, that is relatively easy to maintain.
- In an actor-based design, use of this technique facilitates the logical organization of actors into a hierarchical structure known as an Actor System. The actor system provides the infrastructure through which actors interact with one another.
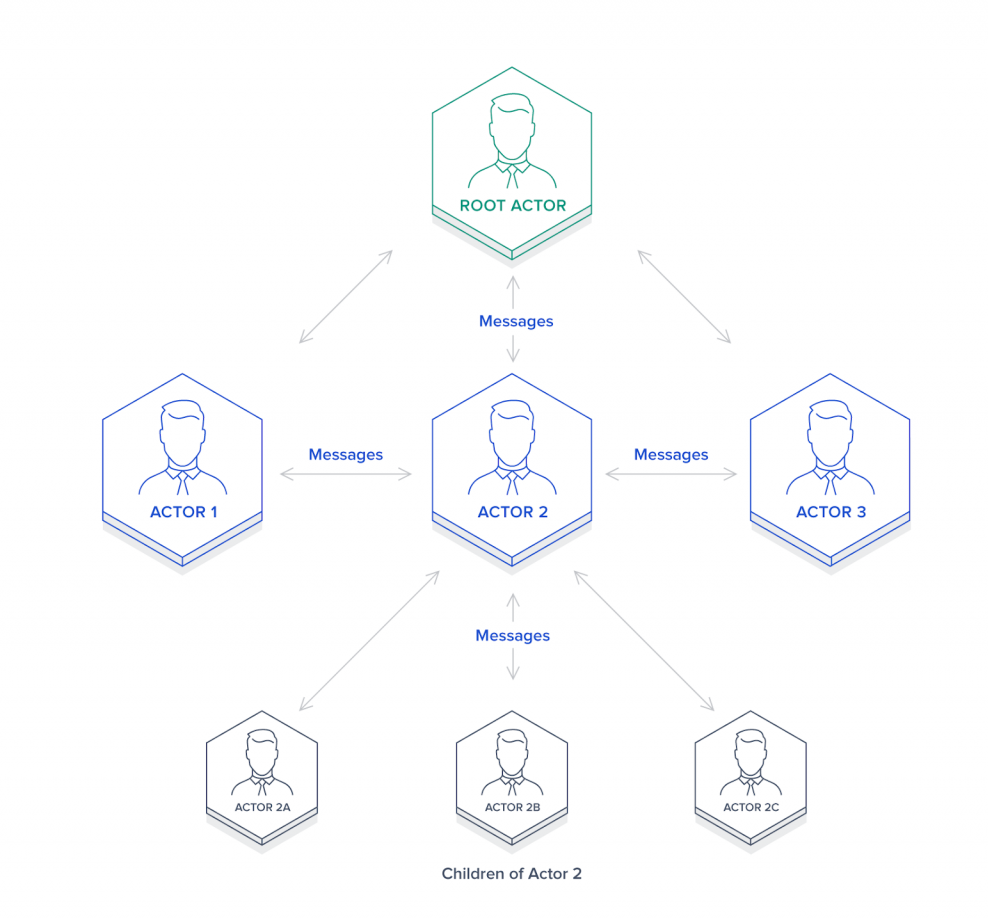
- In Akka, the only way to communicate with an actor is through an ActorRef. An ActorRef represents a reference to an actor that precludes other objects from directly accessing or manipulating that actor’s internals and state. Messages may be sent to an actor via an ActorRef using one of the following syntax protocols:
- ! (“tell”) – sends the message and returns immediately
- ? (“ask”) – sends the message and returns a Future representing a possible reply
- Each actor has a mailbox to which its incoming messages are delivered. There are multiple mailbox implementations from which to choose, with the default implementation being FIFO.
- An actor contains many instance variables to maintain state while processing multiple messages. Akka ensures that each instance of an actor runs in its own lightweight thread and that messages are processed one at a time. In this way, each actor’s state can be reliably maintained without the developer needing to explicitly worry about synchronization or race conditions.
- Each actor is provided with the following useful information for performing its tasks via the Akka Actor API:
- sender: an Actor Ref to the sender of the message currently being processed
- context: information and methods relating to the context within which the actor is running (includes, for example, an actor of method for instantiating a new actor)
- supervision Strategy: defines the strategy to be used for recovering from errors
- self: the Actor Ref for the actor itself
Benefits
- As the Akka Toolkit has the following benefits, it’s good to use Akka to develop your Reaction Applications/Systems:
- It is open source
- By design, it is distributed, and it’s very easy to use distributed applications
- It supports clustering
- It supports Reactive Streams using the Akka Streams module
- It is easy to develop highly performant, highly scalable, highly maintainable, and highly available applications using Akka
- It supports concurrency using the Actor Model. Unlike Java’s Shared-State Model (which is low-level API), the Actor-based Concurrency Model is a high-level API to write Concurrency and Parallelism programming without threads, locking, and other issues.
- It supports scalability in both.
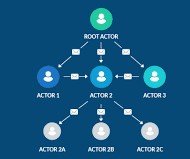
Features
- The key points distinguishing applications based on Akka actors are:
- Concurrency is message-based and asynchronous: typically no mutable data are shared and no synchronization primitives are used; Akka implements the actor model.
- The way actors interact is the same whether they are on the same host or separate hosts, communicating directly or through routing facilities, running on a few threads or many threads, etc. Such details may be altered at deployment time through a configuration mechanism, allowing a program to be scaled up (to make use of more powerful servers) and out (to make use of more servers) without modification.
- Actors are arranged hierarchically with regard to program failures, which are treated as events to be handled by an actor’s supervisor (regardless of which actor sent the message triggering the failure). In contrast to Erlang, Akka enforces parental supervision, which means that each actor is created and supervised by its parent actor.
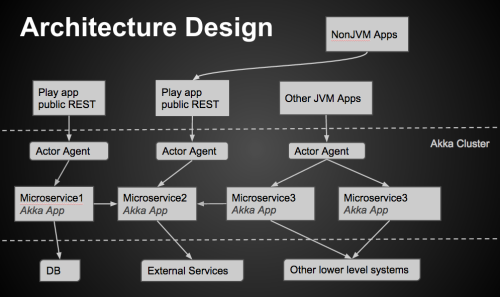
Conclusion
Akka, written in Scala, simplifies and facilitates the development of highly-concurrent, distributed, and fault tolerant applications, hiding much of the complexity from the developer. Doing Akka full justice would require much more than this single tutorial, but hopefully this introduction and its examples were sufficiently captivating
Akka is a very powerful toolkit that allows you to create complex reactive systems without worrying about thread-safeness, or message passing, or failures, etc. Akka provides a clean and simple API to write modern software. Remember, Akka is not a golden hammer, and should not be used to solve every problem. But, if you’re fortunate enough to be working on a problem that can utilize Akka, I highly recommend that you do.