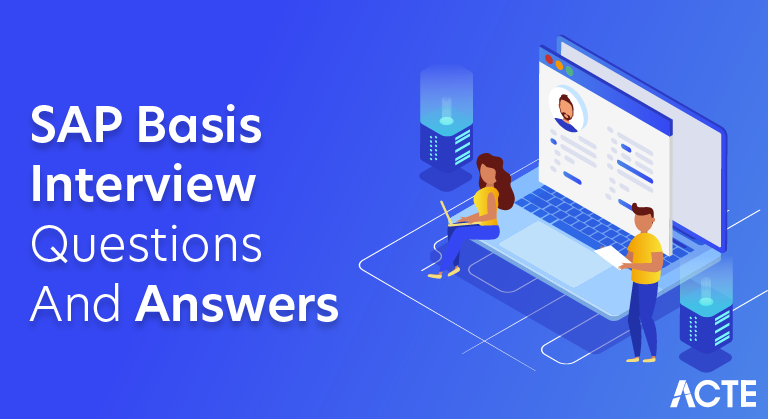
50+ [REAL-TIME] Java Collection Interview Questions and Answers
Last updated on 24th Apr 2024, Popular Course
Java Collection
Java collections provide a strong foundation for managing and arranging groupings of objects in Java programming. Java collections offer a wide range of classes and interfaces for storing, manipulating, and accessing data, from simple lists and maps to more complicated data structures like trees and queues. They are crucial components in Java programming, allowing developers to streamline data management and improve the efficiency and scalability of their programs.
1. What is the definition of Java Collection?
A Java Collection is a group of objects known as elements.It provides a single unit to store and manipulate multiple objects.Collections offer various data structures and algorithms for managing elements.Maps, sets, and lists are a few examples.Collections provide dynamic resizing and flexible storage.They are essential for efficient data management in Java.
2. What constitutes a Java framework?
A Java framework is a reusable software infrastructure.It provides generic functionality that can be customized.Frameworks typically include predefined classes, interfaces, and methods.They facilitate development by offering solutions to common problems.Examples include Spring, Hibernate, and JavaFX.Frameworks streamline application development and promote code reuse.
3. Which interfaces are utilized within the Java Collections Framework?
- The Java Collections Framework utilizes several key interfaces.
- These include List, Set, Queue, and Map interfaces.
- Interfaces provide a common set of methods for collections.
- They ensure consistency and interoperability among different collection types.
- Implementations such as ArrayList, HashSet, PriorityQueue, and HashMap adhere to these interfaces.
- Interfaces enable polymorphic behavior and ease of integration.
4. What sets an array apart from a collection?
- Arrays are fixed in size, while collections can dynamically resize.
- Arrays can hold primitive data types and objects, but collections can only hold objects.
- Collections offer more flexibility and functionality for manipulating elements.
- Arrays have a predefined length, while collections can grow or shrink as needed.
- Collections provide additional features like iteration, sorting, and searching.
- Arrays are more memory-efficient for storing primitive types.
5. How do ArrayList and Vector differ from each other?
- ArrayList is not synchronized, while Vector is synchronized.
- ArrayList is not thread-safe; Vector is.
- ArrayList is more efficient in single-threaded environments.
- Vector incurs synchronization overhead, impacting performance.
- ArrayList does not guarantee thread safety, while Vector does.
- Vector is considered legacy, while ArrayList is the preferred choice in modern Java development.
6. In Java, how is an array defined?
In Java, an array is defined as a fixed-size data structure.It stores elements of the same data type sequentially in memory.Arrays are accessed using index-based notation.They offer fast access to elements but lack dynamic resizing capabilities.Arrays can be initialized using array literals or constructed with the new keyword.Arrays provide efficient memory allocation and access for homogeneous data.
7. What categories of collections exist in Java?
Java collections are categorized into three main types: List, Set, and Map.Lists maintain an ordered collection of elements with duplicates allowed.Sets store unique elements without any particular order.Maps associate key-value pairs, allowing efficient retrieval of values based on keys.Additionally, Queues and Deques provide specialized collections for handling elements in a specific order.
8. Explain the distinctions between Java Maps and Queues.
Distinctions | Java Maps | Java Queues | |
Structure |
Stores key-value pairs |
Follows FIFO (First-In-First-Out) order | |
Key-Uniqueness | Keys must be unique | No uniqueness constraint on elements | |
Element Insertion |
Elements are inserted via key-value pairs |
Elements are inserted at the rear | |
Element Removal | Can remove elements based on keys | Removal happens from the front (oldest element) | |
Lookup Efficiency |
Fast lookup based on keys |
No key-based lookup, retrieval typically linear |
9. Provide a description of the Java collection interface.
The Java Collection interface provides a foundation for the Collections Framework.It defines essential methods for manipulating collections of objects.Collections implementing this interface support operations like add, remove, and size.The Collection interface does not guarantee specific ordering of elements.Implementations like ArrayList, HashSet, and LinkedList adhere to this interface.
10. What are the five standard techniques offered by the collection interface?
- The Collection interface offers essential methods for working with collections.
- These include add() for adding elements, remove() for removing elements, and clear() for clearing the collection.
- Other methods like contains() check if an element is present, and isEmpty() verifies if the collection is empty.
- Additionally, size() returns the number of elements in the collection.
- These standard techniques enable consistent manipulation and querying of collections in Java.
11. What advantages do generics offer in the Collections Framework?
- Generics ensure type-safety, permitting only specified object types for storage.
- They eradicate the necessity for explicit typecasting, enhancing code readability.
- Compile-time type checking with generics reduces runtime errors.
- Generics promote better code reusability and interoperability.
- Enhancing code clarity, generics specify the intended use of collections.
- They facilitate the development of more flexible and robust software components.
12. How do you characterize Java’s Collection Framework?
Java’s Collection Framework offers a unified structure for handling collections of objects.Comprising interfaces, implementations, and algorithms, it efficiently manages collections.Providing diverse data structures like lists, sets, maps, and queues.The framework promotes code reuse, readability, and maintainability through standardized interfaces.Supporting operations such as insertion, deletion, traversal, and sorting.
13. What is the primary advantage of the Properties file?
- Properties files offer a straightforward method for storing configuration data in key-value pairs.
- They provide a text-based format that is easy to read, write, and edit.
- Supporting internationalization by accommodating various language versions.
- Properties files can be dynamically loaded and modified during runtime without recompilation.
- They facilitate externalizing configuration, allowing for flexibility and customization.
14. From your perspective, what does the term “Iterator” in the Java Collection Framework signify?
In the Java Collection Framework, an Iterator permits sequential access to collection elements.It abstracts the underlying implementation, offering a uniform iteration mechanism.Iterators provide essential methods like hasNext() and next() for element navigation.They allow safe modification of elements during traversal using the remove() method.Enhancing code readability, Iterators abstract the iteration process.
15. Why is it necessary to override the equals() method in Java?
Overriding equals() in Java is vital for defining custom object equality.It allows developers to specify criteria for comparing object instances.Various collection classes like HashSet and HashMap rely on equals() to determine equality and uniqueness.Without proper equals() override, collections may not function correctly with user-defined objects.Proper implementation ensures consistency and correctness in comparing objects.
16. How are Collection objects organized in Java?
- Collection objects in Java are categorized based on their characteristics and behaviors.
- The primary categories include Lists, Sets, Maps, and Queues.
- Lists maintain ordered sequences and allow duplicates.
- Sets store unique elements without a specific order.
- Maps represent key-value pairs, while Queues follow FIFO order.
- This classification allows for diverse data handling needs in Java.
17. Elaborate on the collection hierarchy in Java.
Java’s collection hierarchy revolves around interfaces and their implementations.At the apex lies the Collection interface, serving as the root for all collections.Subinterfaces like List, Set, Queue, and Map refine collection types.Concrete implementations such as ArrayList and HashMap offer specific functionalities.The hierarchy facilitates code reuse and polymorphic behavior.
18. What are the differences between Hashset and Treeset?
- HashSet stores elements without maintaining any specific order.
- TreeSet maintains elements in sorted order based on natural ordering or a comparator.
- HashSet employs hashing for storage, ensuring constant-time performance.
- TreeSet internally uses a Red-Black Tree, offering logarithmic time complexity.
- HashSet permits null elements, while TreeSet does not.
- Selection between the two depends on ordering and performance requirements.
19. What are the distinctions between Fail-Safe and Fail-Fast Iterators?
- Fail-Safe Iterators operate on cloned copies, allowing modifications without affecting ongoing iterations.
- Fail-Fast Iterators detect concurrent modifications and immediately throw exceptions.
- Fail-Safe Iterators are slower and require more memory due to cloning.
- Fail-Fast Iterators offer immediate failure detection for maintaining collection integrity.
- Fail-Safe Iterators suit concurrent modification scenarios, while Fail-Fast ones are ideal for immediate error detection.
- The choice between them depends on specific concurrency requirements.
20. Why aren’t the Cloneable and Serializable interfaces extended by Collection?
Cloneable doesn’t contain methods and merely indicates cloning support.Many collection classes don’t offer meaningful cloning, making Cloneable redundant.Serializable indicates object serialization, which isn’t suitable for all collection classes.Extending Serializable may lead to inefficiencies or unexpected behavior.Collection classes provide their serialization and cloning mechanisms as needed.
21. Purpose of Comparable interface in Java Collections?
- Comparable interface allows objects to be compared for ordering.
- It enables sorting of objects in collections like TreeSet or TreeMap.
- Implementing Comparable provides a natural ordering for objects.
- Objects implementing Comparable can be easily sorted using sorting methods.
- It facilitates consistent and predictable sorting behavior in collections.
- Comparable is crucial for applications requiring sorted collections of objects.
22. Polymorphism concept in Java Collections?
Polymorphism in Java Collections allows treating objects of different types uniformly.It enables using a single interface to manipulate various collection types.Collections can hold objects of different classes that implement a common interface.This concept enhances flexibility and extensibility in code design.Polymorphism simplifies code maintenance and promotes code reuse.
23. LinkedList vs ArrayList in Java Collections?
- LinkedList uses pointers to connect elements, providing efficient insertion and deletion.
- ArrayList offers fast random access due to its underlying array structure.
- For frequent insertion and deletion operations, LinkedList is the better option.
- ArrayList is suitable for scenarios requiring frequent element access and traversal.
- LinkedList consumes more memory due to pointer overhead.
- ArrayList may require occasional resizing, impacting performance in large collections.
24. Significance of Set interface in Java Collections?
Set interface represents a collection of unique elements.It ensures no duplicate elements can exist within a Set.Sets offer methods for set operations like union, intersection, and difference.Set implementations like HashSet provide efficient lookup and insertion.Sets are used to enforce uniqueness constraints on collections.Set interface is fundamental in maintaining unique collections of elements.
25.Functionality of HashSet and LinkedHashSet in Java Collections?
- HashSet is an unordered collection of unique elements, offering constant-time performance.
- LinkedHashSet maintains insertion order in addition to uniqueness.
- HashSet uses hashing to store elements, providing fast retrieval.
- LinkedHashSet maintains a doubly-linked list to preserve insertion order.
- Both implementations prevent duplicate elements in the collection.
- HashSet is preferred for general-purpose set operations, while LinkedHashSet maintains order.
26. Role of Map interface in Java Collections?
Map interface represents a collection of key-value pairs.It allows associating keys with values for efficient retrieval.Maps facilitate fast lookup of values based on keys.Implementations like HashMap offer constant-time performance for basic operations.Maps are widely used for data organization and retrieval in Java applications.Map interface is essential for scenarios requiring key-based data storage and retrieval.
27. Differences between HashMap and HashTable in Java Collections?
- HashMap is not synced and accepts null values.
- HashTable does not permit null keys or values and is thread-safe.
- HashMap offers better performance for most operations due to lack of synchronization.
- HashTable is legacy and has been largely replaced by ConcurrentHashMap.
- Iteration over HashMap does not guarantee order, while HashTable iteration is orderly.
- HashMap is preferred in single-threaded scenarios, whereas HashTable is suitable for multi-threaded environments.
28. TreeMap vs LinkedHashMap in Java Collections?
- TreeMap is sorted based on the natural ordering of keys or a custom Comparator.
- LinkedHashMap maintains insertion order in addition to key-based ordering.
- TreeMap uses a Red-Black tree for storage, providing log(n) time complexity for basic operations.
- LinkedHashMap maintains a doubly-linked list to preserve insertion order.
- TreeMap is suitable for scenarios requiring sorted maps, while LinkedHashMap maintains insertion order.
- The choice between TreeMap and LinkedHashMap depends on whether sorted order or insertion order is required.
29. Role of Queue interface in Java Collections?
Queue interface represents a collection designed for FIFO (First-In-First-Out) ordering.It provides methods for adding, removing, and examining elements in a queue.Queues are used for modeling scenarios like task scheduling, messaging, and event handling.Implementations like LinkedList and ArrayDeque offer efficient queue operations.Queue interface supports common queue operations like enqueue, dequeue, and peek.
30. Functionality of PriorityQueue in Java Collections?
- PriorityQueue is an unbounded priority queue based on a priority heap.
- Elements are ordered according to their natural order or a custom Comparator.
- It allows constant-time retrieval of the highest-priority element.
- PriorityQueue is suitable for scenarios requiring ordered processing of elements.
- Implementations guarantee that the head of the queue is the least element.
31. Concept of iteration in Java Collections?
Iteration is the process of sequentially accessing elements in a collection.It allows traversal through the elements of a collection for various operations.Iterators provide a standardized way to iterate over collections in Java.Iterator pattern abstracts the underlying structure of collections for uniform access.Iteration enables operations like searching, sorting, and processing elements in collections.
32. Enhanced for loop with Collections in Java?
- The enhanced for loop (foreach loop) simplifies iteration over collections.
- It provides a concise syntax for traversing through elements of a collection.
- The loop automatically handles iteration and element retrieval from collections.
- Enhanced for loop enhances code readability and reduces potential errors.
- It’s particularly useful for iterating over arrays, lists, sets, and maps.
- Enhanced for loop is preferred for straightforward iteration tasks in Java.
33. Purpose of Comparator interface in Java Collections?
- Comparator interface allows custom sorting of objects in Java collections.
- It provides a way to define alternative sorting orders for objects.
- Comparator enables sorting based on specific attributes or criteria defined by the programmer.
- It’s particularly useful when the natural ordering of objects doesn’t meet requirements.
- Comparator facilitates flexibility in sorting objects according to different criteria.
- With Comparator, developers can implement tailored sorting logic for diverse scenarios.
34. TreeSet usage of Comparator interface in Java Collections?
TreeSet uses Comparator interface for custom ordering of elements.If provided during TreeSet instantiation, Comparator dictates the sorting order.TreeSet maintains elements in sorted order based on Comparator’s rules.Comparator allows TreeSet to sort elements differently from their natural ordering.TreeSet’s ordering is determined by Comparator’s compare method implementation.Comparator usage enhances TreeSet’s flexibility in sorting elements as desired.
35. Synchronization concept in Java Collections?
Synchronization ensures thread safety when accessing shared data structures in Java collections.It prevents concurrent access conflicts and maintains data consistency.Synchronization is achieved using synchronized blocks or methods in Java.It allows only one thread to access synchronized code blocks at a time.Synchronization prevents race conditions and ensures proper multithreading behavior.
36. Significance of synchronized keyword in Java Collections?
- The synchronized keyword ensures exclusive access to critical sections of code.
- It prevents concurrent thread interference and maintains data integrity in Java collections.
- Synchronized methods or blocks serialize access to shared resources, avoiding race conditions.
- It enables safe multithreaded access to mutable collections, preventing concurrent modification issues.
37. Behavior of iterators in multi-threaded environments in Java Collections?
Iterators in Java collections are fail-fast by default, detecting concurrent modification during iteration.In multi-threaded environments, iterators throw ConcurrentModificationException if the underlying collection is modified concurrently.Iterators don’t automatically synchronize access to collections, requiring external synchronization for thread safety.
38. ConcurrentHashMap vs HashMap in Java Collections?
- ConcurrentHashMap is thread-safe, allowing concurrent access from multiple threads without external synchronization.
- HashMap isn’t thread-safe and may lead to data corruption if accessed concurrently by multiple threads.
- ConcurrentHashMap uses internal locking mechanisms to achieve thread safety.
- HashMap doesn’t provide built-in thread safety mechanisms, requiring external synchronization for concurrent access.
- ConcurrentHashMap is suitable for high-concurrency environments where performance and thread safety are crucial.
39. Concept of immutability in Java Collections?
Immutability refers to the inability to change the state of an object after creation.Immutable collections in Java are collections whose contents cannot be modified once initialized.Immutable collections guarantee thread safety and prevent accidental modification by encapsulating internal state.They simplify concurrent programming and eliminate the need for synchronization.
40. Benefits of using immutable collections in Java?
- Immutable collections offer thread safety by preventing concurrent modification issues.
- They simplify code by eliminating the need for explicit synchronization in multi-threaded environments.
- Immutable collections provide predictable behavior and avoid unexpected changes in state.
- They facilitate easier reasoning about code and reduce the risk of bugs related to mutable state.
- Immutability encourages functional programming practices, such as method chaining and composition.
41.What is “fail-fast” in Java Collections?
Fail-fast refers to the immediate detection of any structural modification made to a collection while iterating over it.It ensures that if the collection is modified during iteration, it will throw ConcurrentModificationException to prevent potential inconsistencies.Fail-fast behavior aids in identifying issues early, ensuring data integrity and preventing unexpected behavior in concurrent scenarios.
42.Explain “fail-safe” iterators in Java Collections.
Fail-safe iterators allow safe iteration over a collection even if it undergoes structural modifications during iteration.They operate on a cloned copy of the collection, ensuring that modifications to the original collection don’t affect ongoing iteration.While fail-safe iterators provide safety against concurrent modifications, they may not reflect the latest changes in the collection.
43. Advantages of fail-safe iterators in Java Collections?
- Fail-safe iterators prevent ConcurrentModificationException by iterating over a cloned copy of the collection.
- They provide safety during concurrent modification scenarios, ensuring uninterrupted iteration.
- Fail-safe iterators offer a robust approach for handling concurrent access to collections, promoting stability and reliability in multithreaded environments.
44. How does CopyOnWriteArrayList differ from ArrayList?
- CopyOnWriteArrayList creates a new copy of the underlying array whenever modifications are made, ensuring thread safety during iteration.
- Unlike ArrayList, CopyOnWriteArrayList supports concurrent reads and writes without the need for external synchronization.
- CopyOnWriteArrayList is preferred for scenarios requiring frequent iteration and rare modifications, offering better performance in read-heavy environments.
45. Define concurrent modification in Java Collections.
Concurrent modification occurs when a collection is structurally modified while being iterated over concurrently.It can lead to inconsistencies or unexpected behavior in the iteration process if not properly handled.Java collections provide fail-fast mechanisms to detect and prevent concurrent modifications during iteration.
46. Significance of Iterator interface in Java Collections?
- Iterator interface enables traversal of elements in a collection, providing a uniform way to access elements irrespective of the underlying collection type.
- It allows sequential access to elements and supports safe removal of elements during iteration.
- Iterator facilitates efficient and flexible iteration over various collection types, enhancing code readability and maintainability.
47. Removing elements while iterating in Java Collections?
Elements can be safely removed during iteration using the Iterator’s remove() method.Iterator.remove() removes the last element returned by the iterator from the underlying collection.Removing elements directly using collection methods during iteration may lead to ConcurrentModificationException.
48. Functionality of EnumSet class in Java Collections?
- EnumSet is a specialized Set implementation optimized for enum types in Java.
- It provides high-performance set operations tailored for enum constants, such as union, intersection, and complement.
- EnumSet internally uses bit vectors for efficient storage and manipulation of enum values, offering compact representation and fast operations.
49. Benefits of EnumSet over other Set implementations?
- EnumSet is specifically designed for enum constants, providing type safety, performance, and memory efficiency.
- It offers constant-time performance for common set operations, making it ideal for enum-based scenarios.
- EnumSet supports a rich set of operations tailored for enum types, ensuring convenient and efficient manipulation of enum constants.
50. Concept of Deque interface in Java Collections?
Deque (Double Ended Queue) interface represents a linear collection that supports element insertion and removal at both ends.It extends the Queue interface, providing additional methods for insertion, retrieval, and removal operations.Deque implementations offer versatile data structures suitable for implementing stack, queue, or double-ended queue functionalities.
51. Differences between ArrayDeque and LinkedList?
- ArrayDeque: Implements deque using resizable array.
- LinkedList: Implements deque using doubly linked list.
- ArrayDeque: Faster for adding/removing elements at both ends.
- LinkedList: Better for adding/removing elements at middle.
- ArrayDeque: Random access using indexes.
- LinkedList: Sequential access from head or tail.
52. Role of BlockingQueue interface in Java Collections?
- Facilitates data exchange between producer and consumer threads.
- Provides blocking operations for insertion and removal.
- Ensures thread safety in concurrent environments.
- Allows waiting for space or elements in the queue.
- Essential for implementing producer-consumer design pattern.
- Key component for managing shared resources safely.
53. Functionality of ArrayBlockingQueue?
Implements BlockingQueue backed by array.Fixed capacity, elements added at end removed from front.Supports blocking operations for put and take.Ensures thread-safe access to elements.Ideal for fixed-size bounded buffers.Efficient in scenarios with a fixed number of producers and consumers.
54. Differences between PriorityQueue and TreeSet?
- PriorityQueue: Implements unbounded priority queue using heap.
- TreeSet: Implements sorted set using balanced tree (Red-Black tree).
- PriorityQueue: Allows duplicates and does not maintain sorted order.
- TreeSet: Does not allow duplicates and maintains sorted order.
- PriorityQueue: Faster for adding and removing elements.
- TreeSet: Faster for retrieval and traversal operations.
55. Purpose of CollectionUtils class in Java Collections?
Provides utility methods for common collection operations.Offers functionalities like sorting, filtering, transforming collections.Simplifies common tasks such as finding max/min element.Contains methods for creating immutable collections.Enhances code readability and reduces boilerplate.helps write code that is easier to maintain and cleaner.
56. Functionality of ConcurrentSkipListSet class?
- ConcurrentSkipListSet: Concurrent, navigable set implementation.
- Utilizes skip list data structure for fast access.
- Allows concurrent access without external synchronization.
- Supports efficient range-based operations.
- Maintains elements in sorted order.
- Ideal for concurrent applications requiring sorted sets.
57. Description of ConcurrentHashMap class?
ConcurrentHashMap: Concurrent hash map implementation.Provides thread-safe operations without locking the whole map.Divides map into segments, allowing concurrent access.Ensures scalability in concurrent environments.Offers similar functionalities to HashMap with thread safety.
58. Benefits of ConcurrentHashMap over synchronized collections?
- Concurrent read and write operations are supported by ConcurrentHashMap.
- Synchronized collections: Lock entire collection for each operation.
- ConcurrentHashMap: Provides better scalability under high concurrency.
- Synchronized collections: May lead to contention and reduced throughput.
- ConcurrentHashMap: Optimized for concurrent access without blocking.
- Synchronized collections: Prone to performance bottlenecks in concurrent scenarios.
59. Role of NavigableSet interface in Java Collections?
- NavigableSet: Extension of SortedSet with navigation methods.
- Provides methods for navigating the set in both directions.
- Supports range-based operations like headSet, tailSet, subSet.
- Enables finding closest matches to a given element.
- Useful for applications requiring sorted sets with advanced navigation.
- Enhances flexibility and efficiency in set manipulation.
60. Differences between NavigableSet and other Set implementations?
- NavigableSet: Extends SortedSet, providing navigation methods.
- HashSet: No defined ordering of elements.
- TreeSet: Maintains elements in sorted order.
- NavigableSet: Supports range-based operations efficiently.
- HashSet: Generally faster for basic operations but unordered.
- TreeSet: Slower for insertion and removal but maintains sorted order.
61. Purpose of BitSet class in Java Collections?
BitSet enables manipulation of sets of bits or flags efficiently.It’s valuable in scenarios prioritizing memory efficiency.BitSet offers bitwise operations such as AND, OR, and XOR effectively.Particularly useful for compact storage of boolean values.Suitable for representing flags or executing set operations efficiently.Overall, BitSet streamlines bit-level manipulation tasks.
62. Functionality of WeakHashMap in Java Collections?
WeakHashMap serves as a specialized Map implementation.It retains weak references to keys, allowing garbage collection if no strong references exist.Valuable for managing caching or temporary mappings effectively.Automatically removes entries when their keys become unreachable.Commonly used where automatic cleanup of unused mappings is desired.Assists in preventing memory leaks in specific caching scenarios.
63. Benefits of WeakHashMap over other Maps?
- WeakHashMap automatically eliminates entries with unreachable keys.
- This functionality aids in preventing memory leaks, especially in caching.
- Simplifies management of temporary mappings seamlessly.
- Useful in scenarios with dynamically created and discarded keys.
- Offers a memory-efficient approach to handling short-lived mappings.
- Overall, WeakHashMap enhances memory management with automatic cleanup.
64. Functionality of Identity HashMap?
- One specific Map implementation is called IdentityHashMap.
- It utilizes reference equality (==) instead of object equality (equals method) for key comparison.
- Keys are treated as identical only if referencing the same object.
- Particularly suitable for scenarios prioritizing object identity over value.
- Unlike other Maps, IdentityHashMap disregards the equals method.
- Commonly applied in situations requiring precise object identity control.
65. Differences of IdentityHashMap from other Maps?
- IdentityHashMap employs reference equality, not object equality, for key comparison.
- Unlike typical Maps, it doesn’t rely on the equals method for key matching.
- Keys are considered identical based solely on referencing the same object.
- Ideal for situations emphasizing object identity significance.
- Offers precise control over key distinctions based on object identity.
- Overall, IdentityHashMap prioritizes reference equality in key comparison.
66. Purpose of LinkedHashMap in Java Collections?
LinkedHashMap merges HashMap features with a linked list.Ensures preservation of insertion order, unlike HashMap.Valuable for scenarios where insertion order is critical.Provides predictable iteration order based on insertion sequence.Particularly beneficial for maintaining a consistent order.Overall, LinkedHashMap retains the order of inserted elements.
67. Functionality of LinkedHashMap for maintaining insertion order?
LinkedHashMap upholds the sequence of inserted elements.Achieves this by utilizing a doubly linked list alongside the hash table.Ensures a consistent iteration order based on insertion.Offers methods for accessing elements in insertion sequence.Especially practical for scenarios requiring preserved insertion order.Overall, LinkedHashMap guarantees preservation of insertion order for its elements.
68. Role of SortedMap interface?
- SortedMap is a vital interface in the Java Collections Framework.
- Extends the Map interface to organize key-value pairs in sorted order.
- Ensures keys are maintained in ascending order.
- Provides navigation methods based on key order.
- Particularly useful for scenarios necessitating sorted key-value pairs.
- Overall, SortedMap establishes a contract for maintaining sorted keys.
69. How does TreeMap implement SortedMap interface?
TreeMap is a class implementing the SortedMap interface.Internally employs a Red-Black tree for efficient storage.Guarantees keys are stored in sorted order, either naturally or via a custom comparator.Offers methods for accessing elements by their sorted order.Provides logarithmic time complexity for key-based operations.Overall, TreeMap fulfills the SortedMap interface by maintaining sorted keys.
70. Significance of NavigableMap interface?
- NavigableMap is a subinterface of SortedMap in Java Collections.
- Equips with navigation methods for accessing entries based on their relationships.
- Offers functionalities for finding nearest key matches and retrieving map subsets.
- Provides enhanced navigation capabilities beyond SortedMap.
- Valuable for efficient navigation and retrieval based on entry order.
71. Functionality of TreeMap as NavigableMap?
TreeMap, as a NavigableMap, allows navigation methods like lowerKey(), higherKey(), etc.It provides efficient retrieval of keys and values in sorted order.NavigableMap interface extends SortedMap for additional navigation methods.Supports operations based on the closest match to given search targets.Facilitates the creation of subsets based on ranges of keys.Enables efficient operations for floor, ceiling, lower, and higher entries.
72. TreeMap differences from other Maps?
- TreeMap maintains keys in a sorted order, unlike other Maps.
- It uses red-black tree for storage, ensuring log(n) time complexity for most operations.
- TreeMap doesn’t allow null keys but permits null values.
- Provides efficient sorted key retrieval methods.
- Iteration order is based on the natural ordering of keys or a Comparator.
- Offers functionalities like ceilingEntry, floorEntry, etc., absent in other Maps.
73. Benefits of immutable collections?
- Immutable collections ensure thread safety without synchronization.
- They simplify concurrent programming by eliminating shared mutable state.
- Immutable objects are inherently thread-safe and can be shared across threads.
- Facilitate safer and easier parallel processing.
- Immutable collections promote cleaner, more predictable code.
- Immutable objects are inherently easier to reason about and debug.
74. Functionality of Collections class?
Collections class offers various utility methods for collections manipulation.It includes methods like sort(), reverse(), shuffle(), etc.Provides methods for synchronized collections creation via synchronizedCollection().Offers methods for finding min and max elements like min() and max().Facilitates the creation of unmodifiable collections via unmodifiableCollection().
75. How does Collections class provide utility methods?
Collections class provides static methods for common collection operations.These methods enable sorting, shuffling, reversing, and searching within collections.Allows creation of synchronized and unmodifiable collections.Ensures ease of use and consistency in collection manipulation across different data structures.Methods like binarySearch() provide efficient searching within sorted collections.
76. Purpose of Arrays class?
- The Arrays class provides utility methods for array manipulation.
- It includes methods for sorting, searching, and filling arrays.
- Offers convenient methods for comparing, converting, and copying arrays.
- Facilitates parallel sorting and searching of arrays.
- Ensures efficient and consistent operations on arrays across different data types.
- Arrays class serves as a centralized utility for array-related operations.
77. How does Arrays class work with arrays?
Arrays class provides static methods for various array operations.These methods can be applied directly to arrays of primitive types or objects.Offers sorting, searching, and manipulation functionalities.Ensures consistent and efficient handling of arrays across different contexts.Array methods like binarySearch() and sort() optimize performance for large data sets.
78. Role of Comparator interface?
- Comparator interface defines custom comparison logic for objects.
- It allows sorting objects based on criteria other than their natural ordering.
- Provides flexibility in defining sorting rules for complex data types.
- Comparator interface enables sorting collections of objects that do not implement Comparable.
- Facilitates sorting in ascending or descending order based on custom rules.
- Comparator enhances the versatility of sorting operations in Java.
79. How does Comparator enable custom sorting?
Comparator interface defines custom comparison rules for objects.It allows sorting based on properties or criteria not defined in the object’s natural ordering.Comparator’s compare() method enables flexible sorting logic implementation.Facilitates sorting objects based on multiple attributes or complex conditions.Custom comparators can be provided to collections’ sorting methods for tailored sorting behavior.
80. Significance of Comparable interface?
Comparable interface provides a natural ordering for objects.It enables objects to define their default sorting behavior.Objects implementing Comparable can be sorted using the Collections.sort() method.Comparable interface ensures consistency in sorting across collections.Simplifies sorting of objects without needing an external comparator.
81. How does Comparable enable natural ordering?
- The Comparable interface defines compareTo() for natural ordering.
- Objects implementing Comparable can be sorted with Collections.sort() or Arrays.sort().
- Natural ordering is dictated by the compareTo() implementation.
- It organizes object collections logically for the specific type.
- Ensures uniform sorting across various collections and algorithms.
- Offers a standardized approach to defining object default ordering.
82. Functionality of BitSet class?
- BitSet manages sets of bits, each representing true or false.
- Supports bitwise operations like AND, OR, XOR, and NOT.
- Efficiently handles large boolean sets with minimal memory usage.
- Ideal for tasks requiring storage efficiency and bitwise manipulations.
- Useful for flag representation, filtering, and bit-level operations.
- Provides methods for individual bit manipulation and querying.
83. How does BitSet represent a set of bits?
Internally, BitSet employs a long array for bit representation.Each long value holds 64 bits, indexed in the array.True/false values are determined by bit settings.Enables efficient management of large bit sets with minimal memory overhead.Offers methods for both individual bit manipulation and bulk operations.BitSet’s design ensures effective handling of extensive bit data.
84. Purpose of WeakHashMap?
- WeakHashMap stores key-value pairs with weakly referenced keys.
- It allows keys to be garbage collected when not strongly reachable.
- Valuable for temporary mappings or memory-efficient applications.
- Prevents memory leaks by automatically removing unused entries.
- Widely used in caching and resource-heavy object management.
- Enhances memory management by clearing unused mappings automatically.
85. How does WeakHashMap handle weak references?
WeakHashMap employs weak references for its keys.Weakly referenced keys are eligible for garbage collection when no longer strongly reachable.Garbage collection eligibility occurs when keys lose strong references.Periodically cleans up entries with garbage-collected keys.Automatically removes values associated with collected keys.Ensures WeakHashMap doesn’t impede garbage collection.
86. How does IdentityHashMap use reference equality?
IdentityHashMap utilizes reference equality (==) for key comparison.Keys are compared based on memory addresses rather than content.Different memory addresses render even similar content as distinct keys.Ideal for scenarios prioritizing object identity over content.Especially useful when equals() method is overridden.Facilitates mappings based on reference identity.
87. Purpose of LinkedHashMap?
- LinkedHashMap merges HashMap and LinkedList functionalities.
- Preserves insertion order, unlike unordered HashMap.
- Facilitates iteration in insertion sequence.
- Valuable where insertion order is significant.
- Guarantees predictable iteration order, unaffected by key hash codes.
- Retains similar performance traits to HashMap.
88. How does LinkedHashMap maintain order?
LinkedHashMap manages a doubly-linked list and a hash table.Each entry contains references to preceding and succeeding entries.The linked list maintains insertion order.Iteration returns entries in the order of insertion.Structural changes like insertion or deletion update the linked list.Predictable iteration order is based on insertion sequence.
89. TreeMap implementation of SortedMap?
- TreeMap implements the SortedMap interface.
- Utilizes red-black tree for key-value storage.
- SortedMap maintains entries in ascending key order.
- TreeMap ensures sorting based on natural order or custom comparator.
- Provides efficient logarithmic-time operations.
- Valuable for tasks requiring ordered key-value management.
90. TreeMap navigation methods?
- TreeMap offers methods for entry navigation.
- firstKey() retrieves the lowest key.
- lastKey() retrieves the highest key.
- lowerKey(K key) returns the greatest key less than the given key.
- higherKey(K key) returns the least key greater than the given key.
- These methods enable efficient key retrieval and navigation.
- Particularly useful for range queries and ordered data structure navigation.
91. Purpose of Collections class?
The Collections class provides utility methods for operations on collections, like sorting, searching, etc.It offers static methods for manipulating collections like lists, sets, and maps.Collections class provides a convenient way to work with collections that are not modifiable.It acts as a container for static methods that operate on or return collections.This class is a part of the Java Collections Framework.
92. Role of Arrays class?
- The Arrays class provides various static methods to work with arrays in Java.
- It offers methods for sorting, searching, and filling arrays.
- This class provides convenience for manipulating arrays without writing boilerplate code.
- Arrays class contains methods for comparing arrays, converting arrays to lists, etc.
- It simplifies common array operations and enhances code readability.
- In essence, it serves as a utility class for array manipulation in Java.
93. Functionality of Comparator interface?
- It allows sorting objects based on specific criteria not inherent to the objects themselves.
- By implementing this interface, custom comparison logic can be defined for sorting.
- Comparator facilitates sorting objects in collections like lists and arrays.
- It enables sorting based on attributes or properties of objects rather than their natural order.