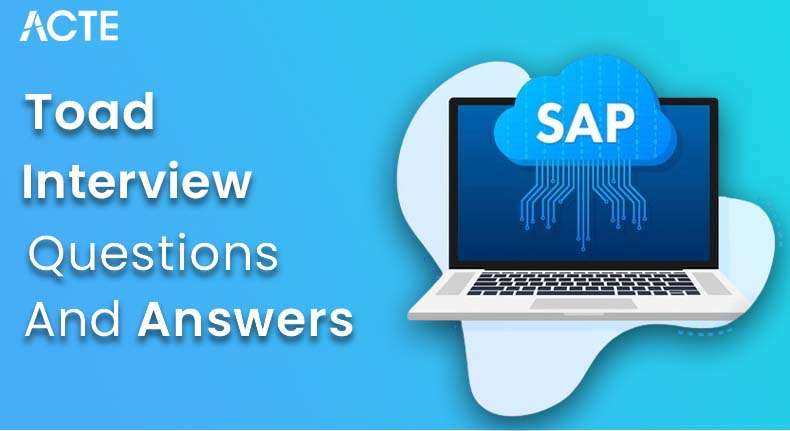
Exception handling is a programming feature that enables developers to manage errors and unforeseen events smoothly within their applications. By employing try-catch blocks, developers can anticipate potential issues, execute code that may fail, and address errors without causing the program to crash. This method enhances application stability and improves user experience by delivering meaningful feedback during errors. Effective exception handling is crucial for building robust and resilient software.
1. What is the Java exception?
Ans:
An exception in Java is an event that interrupts the normal course of a program’s execution. It is an object representing an error or unexpected situation. Java uses a mechanism called exception handling to manage these events. Various causes can lead to exceptions, including invalid user input or resource unavailability. Well-handled Exception leads to more solid, high-performance applications. Proper exceptions can be caught and handled to prevent application crashes.
2. What are runtime exceptions in Java?
Ans:
Runtime exceptions are those that are thrown during the run time of a program. They are not declared in the throws clause of the method. A few common ones are ‘NullPointerException, ArrayIndexOutOfBoundsException,’ and ‘IllegalArgumentException’. These usually denote some programming mistakes in the Code, such as logical errors or improper use of APIs. Since they are unchecked, programmers do not need to worry about them explicitly, but once again, it’s a good practice to handle the root causes so that such an exception does not arise.
3. Explain the difference between checked exceptions and unchecked exceptions in Java.
Ans:
- Checked exceptions are those which must be either caught, or declared in the method’s throws clause. They are subclasses of ‘Exception’ excluding ‘RuntimeException’.
- Examples include ‘IOException’ and ‘SQLException’. Unchecked exceptions do not necessarily need to be explicitly handled; they are subclasses of ‘RuntimeException’.
- Examples include ‘NullPointerException’ and ‘ClassCastException’. This difference encourages developers to handle exceptional cases more rigidly while handling checked exceptions.
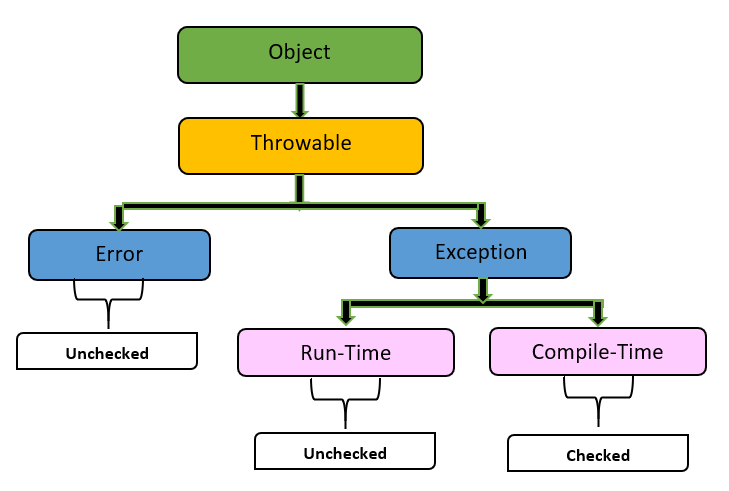
4. What is Exception Handling in Java? What are the advantages of Exception Handling?
Ans:
- Using try, catch, and finally, blocks is a mechanism for handling runtime errors in Java. This technique aids developers in writing more reliable Code by isolating the error-handling Code from regular Code.
- Its benefits include better code readability and easier debugging, as well as the ability to gracefully recover from errors.
- It also helps maintain application stability while improving the user experience. Moreover, it helps to separate concerns in code cleaner.
5. How does Java handle exceptions?
Ans:
The try, catch, and finally, blocks are used together in Java to handle exceptions. All the codes in the try block may result in an exception. In case of an exception, control transfers to a corresponding catch block, where the Exception can be processed. Finally, a final block is used to execute the Code, which must run regardless of whether an exception occurred. This design makes error handling cleaner and also guarantees resources are freed appropriately.
6. What’s the difference between the throws and throws keywords in Java?
Ans:
Aspect | throw | throws |
---|---|---|
Purpose | Used to explicitly throw an exception. | Used to declare that a method can throw an exception. |
Usage Context | Inside method bodies. | In method signatures. |
Exception Type | Can throw both checked and unchecked exceptions. | Must declare checked exceptions (unchecked exceptions can be omitted). |
Example | ‘throw new IOException(“File not found”);’ | ‘public void readFile() throws IOException’ |
Control Flow | Transfers control to the nearest catch block or terminates if uncaught. | Does not affect control flow directly; informs callers of potential exceptions. |
7. Can statements be placed between try, catch, and finally blocks?
Ans:
- Statements can be placed between try, catch, and finally, blocks. However, the context matters. Any statement that might throw an exception can be placed within a try block.
- Any number of statements may be allowed following a catch block to permit the program to carry out additional processing after catching an exception.
- Any statements placed in the final block will always be executed, whether or not an exception was thrown or caught.
8. What are final, final, and finalized keywords, and how are they different?
Ans:
- The keyword ‘final’ is used to declare constants or prevent inheritance and method overriding. Once declared ‘final,’ a variable cannot be re-assigned.
- A method declared ‘final’ cannot be overridden. The keyword is finally employed in exception handling. This way, some code blocks will be executed independently of whether exceptions are dealt with.
- The garbage collector calls the finalize () method of the Object class before an object is reaped.
- The two keywords have quite different purposes for Java programming. Each is related to the keyword’s aspect of behaviour about Code.
9. What are the benefits of using try-with-resources?
Ans:
Java 7 introduces a feature called try-with-resources, simplifying resource management. For example, it automatically closes resources like files or database connections when an exit from the try block occurs. Thus, it minimizes the chance of resource leaks and makes the Code cleaner and more readable. It reduces the dangers of loss due to explicit final blocks placed there for resource cleanup. Furthermore, it also ensures the closing of resources even in case of an exception.
10. What is the stack trace and what has it to do with an exception?
Ans:
A stack trace is a report that provides information about the active stack frames at any given time while a program executes. It captures method calls that led to the Exception and reports the type, message, and line numbers where the Exception came from. This information is crucial during the debugging process, where it will reveal the point of failure to the developer. A developer’s ability to interpret stack traces will enhance his error resolution skills for Java applications.
11. Which are the important methods declared within the Exception class of Java?
Ans:
- Java’s Exception class provides important methods for processing exceptions. ‘getMessage()’ returns the complete message of the Exception.
- The method ‘printStackTrace()’ prints the stack trace of the Exception to the console, which is useful in debugging. If an exception was generated with a cause, then the method ‘getCause()’ will help retrieve that cause.
- Further, ‘toString()’ will return a string representation of the Exception. These methods give developers insight into the Exception and improve error handling.
12. What does the try block do?
Ans:
- The role of a try block in Java is to contain the Code that may throw an exception during its runtime execution. It allows developers to anticipate and manage errors so that the program does not crash.
- Code within the try block is monitored for exceptions; if any such exception occurs, control goes to the corresponding catch block. This separation helps create a clean and readable code structure.
- The try block contributes to more robust applications by isolating error-prone Code and ensuring graceful program recovery in case of unexpected issues.
13. What is the purpose of the catch block in exception handling?
Ans:
The catch block serves for handling exceptions raised by the try block. When there is an exception, the control passes to the matching type of appropriate catch block at this stage. The developers may implement error-handling logic inside this catch block, such as logging or printing the error messages. Using multiple catch blocks serves a good purpose where one may have various exceptions from one try block for different types of exceptions that one wants to handle differently.
14. What occurs if an exception escapes detection?
Ans:
If an exception is not caught in Java, a propagated exception travels up the call stack to the JVM. In this case, runtime errors can occur when the JVM fails to handle it also. The application may also crash. An uncaught exception usually shows an error message plus the stack trace pointing to where it is. It is, therefore, a problem for user experience and application reliability. Proper exception handling should be done to avoid such a case.
15. What is the use of the final block in exception handling?
Ans:
- The final block specifies the Code that will always be run after the try-and-catch blocks, regardless of whether an exception has been thrown and caught.
- It is often used to clean up resources, such as closing file streams or database connections, even when a return statement is contained in the try block or if an exception occurs.
- This leads to efficient resource utilization and prevents memory leaks. Using the final block, the programmer can ensure that crucial tasks are carried out consistently.
16. How are custom exceptions in Java created?
Ans:
- Custom Exceptions in Java are created by extending the Exception class or any of its subclasses. An excellent new class can be defined with meaningful names that describe the type of error occurring in a particular situation.
- Using constructors, the exception message and/or the cause can be initialized. Custom exceptions also include other fields or methods that can improve the application context.
- When developers create specific exceptions, they make it easy to improve the extent of error handling within their Code.
- Of great importance is the contribution custom exceptions make to increasing the transparency of the error management strategy of the application.
17. What is the difference between throw and catch for the Exception?
Ans:
It notifies the application that there is an error. It can be done with the ‘throw’ keyword followed by an instance of an exception. Catch – This handles the thrown Exception, so it doesn’t propagate further. The language does this with a catch block that matches the thrown exception type. Throwing is about creating an error signal, and catching is about responding to that signal. Together, they form the basis of Java’s exception-handling mechanism.
18. What is the relation between the assert statement and exception handling?
Ans:
The assert statement is a debugging aid that tests assumptions in Code during development. It can be used to prove that some specified conditions are fulfilled; otherwise, it raises an assertion error. This behaviour corresponds to exception handling because assertions may indicate programming logic errors in the Code. However, assertions are typically commented out in production code so that they do not impact runtime performance. Exception handling deals with runtime errors, while assertions must be caught during development.
19. What is a checked exception, and how is it declared?
Ans:
- A checked exception in Java is an exception that either needs to be caught or declared in a method signature. It is a subclass of Exception, Exception excluding RuntimeException.
- Checked exceptions are provided for exceptional conditions that a program should anticipate and handle. Examples of checked exceptions are IOException and SQLException.
- A checked exception is declared in the method signature with the ‘throws’ keyword, specifying which the method may throw.
20. How can multiple exceptions be caught in a single catch block?
Ans:
- Multiple exceptions can be caught in a single catch block using Java’s pipe ‘|’ operator. Javas is a more convenient and brief way of handling many exceptions if common sense is used.
- For example, one can declare a catch block as ‘catch (IOException | SQLException e)’. One can refer to the caught Exception within the block using the variable ‘e’.
- This, in turn, reduces unnecessary duplication and makes Code easier to read and comprehend. It’s very useful in cases where several exceptions relate to a common strategy for handling them.
21. What is the use of the throw keyword?
Ans:
The ‘throw’ keyword in Java explicitly throws an exception from a method or a block of Code. With its help, developers can define and indicate exceptional conditions in the program. When an exception is thrown, then normal execution flow is terminated, and control is passed on to a catch block that is nearest to the place where it was thrown. The exception type must be a class that is a subclass of the Throwable class. The use of ‘throw’ supports fine-grained control over error signalling, and it’s an important part of realizing custom error-handling logic.
22. What is a Null Pointer Exception?
Ans:
A ‘NullPointerException’ occurs when a program attempts to use an object reference that has not been initialized or set to null. Common examples are the attempt to call a method on a null object, access a field of a null object, and so on, or try to change a null array. It indicates a programming error, which usually results from an inappropriate absence of checks for a null value. It is an unchecked exception, meaning no declaration is necessary.
23. What is the ClassCastException, and when does it occur?
Ans:
- A ‘ClassCastException’ is thrown when an attempt is made to cast an object to a class of which it is not an instance. This often occurs during downcasting, when one incorrectly casts a superclass reference to a subclass type.
- For example, this Exception would occur if an attempt is made to cast an object of type ‘Animal’ to a type ‘Dog’ when the actual object is ‘Cat’.
- The class is unchecked and should not be mentioned in method headers. This Exception results from a logic error. Even proper type-checking may avoid such errors.
24. How can the IllegalArgumentException be used efficiently?
Ans:
- This Exception, ‘IllegalArgumentException,’ informs that a method has been passed with an illegal or inappropriate argument. It is a helpful mechanism for validating input parameters before any processing may be done on them.
- With such an exception, developers can indicate what went wrong. Using this Exception also helps promote good programming practice by ensuring that methods receive valid input.
- Techniques of effective use include providing informative messages through which one would understand what could have gone wrong.
25. What are the subclasses of ‘Throwable’?
Ans:
Errors are the most important classes that extend the ‘Throwable’ class in Java. Javais very useful to consider the class of ‘Error’, which represents serious conditions the application cannot normally recover from. The type of conditions like ‘OutOfMemoryError’ or ‘StackOverflowError’ should not be caught by applications to avoid their dismissal by an application-level exception handler. The class of ‘Exception’ is used for the conditions that a reasonable application should catch and handle.
26. What is the Exception class used for?
Ans:
Java’s ‘Exception’ class is the Javaper class for all checked exceptions. It is a base class for creating exceptions that a program may anticipate and handle. Abstraction of the ‘Exception’ class enables developers to develop application-specific exception types. Including it in the exception class hierarchy is essential to allow for structured error handling. It clearly distinguishes recoverable conditions from non-recoverable conditions. The ‘Exception’ class makes error management clearer and easier to maintain.
27. How can the cause of an exception be determined?
Ans:
- The ‘getCause()’ method of the Throwable class can be used to determine the cause of an exception. It returns the underlying reason for the Exception enclosed within another throwable object.
- This allows for the incorporation of cause specifications in constructors of custom-built exceptions, which will help track the errors in detail.
- The cause can also be set with the ‘initCause()’ method after the occurrence of an exception. Debugging and handling errors much better are characterized by analyzing the cause.
28. What is a RuntimeException, and how is that different from other exceptions?
Ans:
- A ‘RuntimeException’ is a subclass of ‘Exception’ that serves for exceptions that can arise while running a program. Unlike checked exceptions, ‘RuntimeExceptions’ do not have to be declared in a method’s throws clause.
- Typical ones are ‘NullPointerException’, ‘ArrayIndexOutOfBoundsException’, and ‘ClassCastException’. These are generally signs of a programming error, which can and should have been avoided through good programming practice.
- The primary difference is that checked exceptions require explicit handling to avoid compile-time errors, yet programmers have greater latitude in their error-handling techniques.
29. Can a final block exist without a try block?
Ans:
No, a fiJavablock cannot exist in Java without a corresponding try block. The final block is designed to follow a try block and will be executed whether an exception was thrown or caught. This block guarantees that critical cleanup actions are done, such as closing of resources. If a try block does not exist, a final block cannot do anything within the latter’s context. This association is indispensable for maintaining the integrity of the resource and handling the Exception properly.
30. How are exceptions in a Java application logged?
Ans:
Exceptions in a Java application are usually logged using logging frameworks, which can be Log4j, SLF4J, or the native API in Java. These frameworks provide means to log exceptions at varied levels, such as info, warn, or error. Allow stack traces to be logged so the designer can quickly assume the particular issue when logging an exception. Proper logging practices always ensure the gathering of relevant information regarding the Exception so that further analysis is possible for debugging and monitoring application behaviour.
31. What is a ConcurrentModificationException, and when does it occur?
Ans:
- A ‘ConcurrentModificationException’ is thrown if a collection is modified in any way other than as specified by one of its iteration methods, even in multiple threads.
- This is very common in multithreaded applications, such as when one thread modifies a collection while another reads it or when the collection is modified directly within a loop.
- The following example also triggers a ‘ConcurrentModificationException’. This Exception helps prevent unpredictable behaviour in concurrent applications. Developers can avoid it with proper synchronization or using concurrent collection classes.
32. What are some best practices for Java exception handling?
Ans:
- Best Practices for Exception Handling Java Each type of Exception in Java can be handled with a specific catch block. Furthermore, meaningful error messages help clarify things.
- Structured logging frameworks can be used for debugging exceptions. Moreover, proper resources must be handled; therefore, try-with-resources should be used wherever possible.
- A catch block should not be left blank because it will mask potential problems. Finally, documentation of strategies for exception handling makes Code easier to maintain and understand.
33. How does a method declare that it throws an exception?
Ans:
A method declares that it may throw an exception using a ‘throws’ clause in the signature. It is positioned after the parameter list and names the exceptions the method could throw. For instance, ‘public void myMethod() throws IOException {}’ states that ‘myMethod()’ could throw an ‘IOException’. It notifies the caller of the method they must handle or declare the Exception. Checked exceptions ensure that error conditions are explicitly identified, which helps improve code reliability and readability.
34. How does Exception Handling affect the performance?
Ans:
Exception Handling may impact performance because the overhead is involved in throwing and catching the exons. Generating a stack trace that accompanies every Exception is an expensive operation, so there is an exception to be an ex whenever possible. Using exceptions for normal control flow degrades performance because of the repeated throwing and catching. But if used correctly, exception handling does not degrade performance essentially in most cases.
35. How does a program recover from an exception?
Ans:
- A program can recover from an exception by using catch blocks that handle known exceptions and implement recovery logic.
- This can include retrying the operation, providing an alternative execution path, or prompting the user to correct it. Finally, resources should be cleaned up in final blocks so that there will be no leaks.
- Custom exceptions can provide more context for recovery actions. Correct exception logging helps in debugging for prevention next time.
36. What is the function of the try-catch-finally construct?
Ans:
- The try-catch-finally construct in Java handlJavaxceptions. The try block holds Code capable of throwing an exception, while the catch block provides a means of handling the Exception.
- If an exception is thrown, control transfers to the appropriate catch block, which enables specific error handling.
- The final block will always run; this is usually where resource cleanup is performed in case an exception occurs.
- It lets the programmer separate regular Code from Code for error handling, encouraging cleaner, maintainable Code overall.
37. How does Java’s excJava’s hierarchy work?
Ans:
The Java exception hierarchy is organized around the class ‘Throwable,’ with two big branches, ‘Error and Exception’. ‘Error’ is for serious problems that a program should not catch. Everything else is an ‘Exception,’ which can be seen and handled. A critical split within ‘Exception’ is between checked and unchecked exceptions. Checked exceptions must be declared in method signatures; unchecked exceptions do not need such declarations. This structure allows structured error handling and supports robust exception-handling practices.
38. What is the difference between Throwable and Exception?
Ans:
The ‘Throwable’ class forms the parent for all errors and exceptions in Java. The Javaeption class is a sub-class of the ‘Throwable’ class. The ‘Throwable’ class thus encompasses checked exceptions (sub-classes of ‘Exception’) and unchecked exceptions such as errors that happen to be its other sub-class. Errors are serious conditions that applications cannot recover from, such as ‘OutOfMemoryError’. Exceptions, however, are conditions a program can catch and recover from.
39. How to override the ‘toString()’ method in a custom exception?
Ans:
The ‘toString()’ method can be overridden in a custom exception class to provide meaningful string representations of the exceptions. This would be in the definition of the custom exception class, commonly about information regarding an exception’s message and state:
- @OverJava
- public String toString() {
- return “CustomException: ” + getMessage();
- }
40. Why are checked exceptions more useful than unchecked exceptions?
Ans:
- Checked exceptions are essential as they enforce compile-time error handling, wherein the developer is aware of such errors or not.
- This implies that the programmer needs to either handle the Exception or declare it in the method signature. This helps promote better method documentation and robust Code.
- Checked exceptions result in less flexibility but produce more predictable runtime behaviour if not discovered and addressed beforehand.
- Implementing checked exceptions may also boost API maintainability and readability. This difference inspires the best methods of anticipation through error management in design.
41. How to implement Exception chaining in Java?
Ans:
- ExceJavan chaining in Java is imJavaented using a cause exception passed to the constructor of a new exception. The new exception wrapper retains all information from the old Exception, including its stack trace and context.
- In this case, let us consider a custom exception class that accepts a ‘Throwable’ in its constructor and then passes it on to the superclass constructor.
- This provides more details on why the new Exception. Then, one can get the original Exception using the method ‘getCause()’. Such a technique enhances the approach to error handling and debugging.
42. What is a try-with-resources statement?
Ans:
The try-with-resources statement is intended for automatic resource management in Java. It has been supported since Java 7, making dealing with resources such as files or database connections easier. The resources declared within the parentheses of the try statement are automatically closed at the end of the block, even if an exception happens. This helps avoid resource leaks and reduces boilerplate cboilerplateode caused by closing resources programmatically. It enhances code readability and reliability.
43. What happens if a checked exception is thrown but not declared?
Ans:
The Java compiler generates a compile-time error if a checked exception is thrown but not declared within a method’s signature. This is so that the programmer is conscious of the possible Exception and deals with the problem: if the process does not handle the checked Exception, it must declare a ‘throws’ clause as part of the method declaration. If this is not done, then the Code will not even compile. Hence, disciplined exception-handling practices are enforced by this mechanism. It catches runtime errors due to unchecked exceptions that were not seen.
44. How can debug information be extracted from stack traces?
Ans:
- Debug information from stack traces can be extracted using the ‘printStackTrace()’ method invoked on the caught Exception.
- The method calls print out the stack trace to the console, revealing the methods that caused the Exception.
- Each stack trace entry also has a class name, a method name, and a line number so that one can have an excellent view of the state when an exception occurs.
- Stack trace analysis can identify causes of problems or erroneous outcomes, which is invaluable for debugging and application reliability. Debuggers may also log stack traces for later reference.
45. How do exceptions caught at different levels within the application influence the final results?
Ans:
- Handling at different levels impacts the architecture and maintainability of Code. Centralized error management occurs when the Exception is dealt with at a high level, maintaining consistency in response to errors.
- Overhandling at high levels may obscure the exceptions’ original context, but close-to-source exception handling may provide more specific responses while duplicating codes.
- Balance is key to getting error management right. Code for exception handling works well when structured for clarity and reliability.
46. How can throws be included in method signatures?
Ans:
Add the ‘throws’ keyword in a method signature to specify that a method may throw some checked exceptions. This informs callers that they might have to handle or declare the exceptions. For example, the declaration ‘public void readFile() throws IOException’ specifies that ‘readFile’ can raise an ‘IOException’. This restriction encourages developers to anticipate potential exceptions and respond appropriately. It raises the abstraction for the method’s behaviour and enables people to understand how to apply it without leaving it vulnerable to failure.
47. What are some pitfalls in exception handling?
Ans:
Common pitfalls associated with exception handling are rather broad catch blocks that catch any exception without providing any real handling logic. This tends to hide the actual root cause of the error and makes debugging a hassle. The other pitfall is that one needs to log exceptions or provide meaningful error messages, which hampers troubleshooting. Finally, a final block can fail to clean up resources, leading to resource leaks. The use of exceptions instead of real error handling also degrades performance.
48. How can resource leaks be avoided while handling exceptions?
Ans:
Resource leaks can be avoided while Exception handling by closing the resources even if an exception occurs. A final solution using the try-with-resources statement is robust because resources would be automatically closed at the end of the try block; resources that were opened in a traditional try-finally construction would have to be closed in the final block to ensure their eventual release. Let’s remember careful coding practices, like checking for null references before accessing resources to prevent leaks and code reviews regularly to catch potential leaks.
49. What is the relationship of Java exception handling to flow control?
Ans:
- In Java, exceJavans and flow control are separate notions that interconnect. Exceptions present a manner through which error conditions are handled that interfere with the program’s normal flow.
- In most cases, exceptions are not encouraged to control flows because they may create unintuitive and sometimes inefficient codes.
- However, exceptions can be elegantly used as exceptional conditions, allowing programs to recover from errors and making an application more robust in general.
- Exceptions should be understood relative to traditional clean and maintainable code control structures.
50. How can a specific exception type be targeted for handling?
Ans:
- Declaring catch blocks for a particular exception type can target its handling. For example, in ‘catch (IOException e)’, the program can explicitly handle the exceptions of the ‘IOException’ type.
- It will then provide much more customized responses or ways for recovery. A program can use any number of catch blocks to handle the types of exceptions separately so that it does not have redundant error flow, which would enhance understanding of the Code.
- In this manner, developers can explicitly deal with different conditions of errors. Catching specific exceptions enhances code maintainability and benefits users.
51. What are the best practices for creating custom exceptions?
Ans:
Custom exceptions should be derived from an appropriate superclass, including ‘Exception’ or ‘RuntimeException’. Each custom exception class should have a meaningful, appropriate name explaining its purpose. Multiple constructors are often implemented where one will accept a cause. Meaningful messages should be provided in custom exceptions to help with debugging and error tracking. Documentation of the context in which the Exception is thrown is a must.
52. What is a Throwable class in Java?
Ans:
The theJavarowable class is the parent of all the errors and exceptions in Java. It is the root of the exception-handling mechanism. It has two subclasses, ‘Error’ and ‘Exception’, defining two throwable condition categories. The ‘Throwable’ class has methods like ‘getMessage()’, ‘getCause()’, and ‘printStackTrace()’, which can be used to extract information from an exception. This class supports reporting errors and debugging by allowing access to error information.
53. What are checked exceptions, and how do they enhance Code readability?
Ans:
- Checked exceptions can make the Code more readable if it explicitly denotes which methods throw checked exceptions that must be caught.
- This forces developers to consider different error conditions and properly handle them. Too many checked exceptions can overclassify method signatures, thus making them complex.
- When using checked exceptions, one needs to strike a balance so as not to lose readability. Properly documented exceptions increase the readability of a method’s behaviour and pitfalls.
54. Where should unchecked exceptions be applied?
Ans:
- Unchecked exceptions should be applied where there is a strong possibility of programming bugs, such as logic bugs or misuse of APIs. These include ‘NullPointerException’, ‘ArrayIndexOutOfBoundsException’, and ‘ClassCastException’, among others.
- Unchecked exceptions point toward bugs that the developer should rectify rather than catch at runtime runtime. They are best used when the caller is never expected to trap the Exception.
- Unchecked exceptions encourage cleaner Code with fewer superfluous try-catch blocks. They offer flexibility and allow the application to decide how to handle errors at runtime rather than during compile-time checks.
55. What is the significance of exception messages?
Ans:
Exception messages usually form a “golden clue” that lets a developer know about the nature of an error occurring in an application. A good message is user-friendly and greatly assists the developers in easily identifying problems during debugging or when scanning through logs. Therefore, The messages should be brief, informative, and self-explaining of what went wrong and why it didn’t go wellwell. Such messages can be the first-tier communication between the application, developer, or user.
56. How can Exception handling make applications more robust?
Ans:
Exception handling makes applications more robust through graceful failure response to unexpected conditions and erroneous situations. While considering possible failures and using suitable catch blocks, applications do not crash, and functionality is maintained. Meaningful feedback during errors will lead to a better user experience. Log exceptions also help in recurring mistakes, which can then be pre-fixed. More specific error management can be achieved using customized exceptions.
57. What if multiple catch blocks are defined for the same try block?
Ans:
- The JVM evaluates each in sequence if multiple catch blocks are defined for one try block. It seeks a matching exception type and throws it with the nearest catch block that handles it.
- Once matched, the catch block will be executed, while subsequent ones are ignored. This allows developers to handle different types of exceptions differently.
- All catch blocks must be ordered from the most specific to the general, lest unreachable code errors occur. This design ensures that the error is handled as clearly and effectively as possible.
58. Can more than one exception type be caught in one catch block?
Ans:
- Yes. From Java 7 onwards, a catch block may capture multiple types of exceptions with a multi-catch syntax. This is done by listing multiple exception types within a single catch block, separated by vertical bars (|).
- For example, ‘catch (IOException | SQLException e)’ would mean that the block can capture both ‘IOException’ and ‘SQLException’.
- This feature eliminates code duplication and promotes readability by combining similar error-handling logic into one place.
- The catch block, conversely, can only catch unconnected exceptions in the sense that these are unrelated via inheritance. This makes Exception handling much easier.
59. What does the catch keyword do?
Ans:
The ‘catch’ keyword in Java designates a code block that catches exceptions thrown by the matched try block. If an exception occurs within the body of the try block, then control transfers to the catch block, where developers can define how that Exception might be dealt with. Each catch block may be designed to handle a particular type of Exception, which may lead to such customized error responses. The use of the catch keyword encourages structured error management and eliminates program crashes.
60. How does the final block interact with the return statement in a method?
Ans:
In Java, the Javal block is always executed after the try-and-catch blocks, whether an exception was thrown or a return statement was executed. Suppose there is a return statement in the try block. In that case, it prepares the return value but runs finally before ever returning the method: it means anything in the final block gets executed and if it also includes another return statement, that potentially alters the return value. This interaction management of resource cleanup vs. return logic relies upon understanding.
61. What are the best practices for logging exceptions?
Ans:
- Best practices for logging exceptions include using a structured logging framework that supports various log levels. Logs should contain critical information such as exception type, message, stack trace, and contextual details (e.g., method names and parameters).
- Avoid logging sensitive information to protect user data and comply with security standards. Ensure that logs are written to a persistent store for future analysis.
- Review and refine logging strategies regularly to maintain clarity and relevance. Using meaningful log messages enhances the debugging process and aids in identifying recurring issues.
62. How can exceptions be rethrown in Java?
Ans:
ExceJavans can be rethrown in Java using a ‘throw’ statement within a catch block. This allows developers to catch an exception, perform some logging or cleanup, and then throw the Exception again, optionally wrapping it in a new exception. For example:
- catchJavaException e) {
- // Log the Exception
- throw new CustomException(“An error occurred”, e);
- }
Rethrowing can provide additional context while preserving the original Exception’s details. This practice is useful for maintaining the exception chain and improving error transparency. It also enhances the handling of exceptions at different application layers.
63. What does the ExceptionInInitializerError do?
Ans:
ExceptionInInitializerError. This is thrown when there is an exception during the static initialization of a class. The invocation of static initializers or the initialization of static variables may cause it. The error wraps up the original Exception that caused the failure, making debugging problems easier at class loading time. This type of error is critical because it prevents the class from proper initialization. Therefore, this type of error needs to be known to facilitate the debugging process of initialization bugs.
64. What is the role of exceptions in adding more robustness to resource management?
Ans:
- Resource management can be more robust by using exceptions to ensure the resources are released on failure. A try-with-resources statement simplifies managing resources automatically at the end of the block.
- Resource leaks are minimized because every declared resource is guaranteed to be closed without a program. In addition, explicit resource cleanup is possible if a try-with-resources is not available through final blocks.
- These enable efficient resource handling so that the system may successfully deliver the expected performance with reliability. Proper resource handling is crucial for maintaining a system’s performance.
65. How does exception handling affect code maintenance?
Ans:
- Improved structured error handling and increased readability of Code improve code maintenance. Explicit exception-handling mechanisms make it clear to developers how error handling is dealt with.
- Uniform Exception handling results in better maintainable Code. However, overuse or poor organization of Exception handling may muddy the Code, making it less intelligible and more difficult to maintain.
- Code review and refactoring can keep Code clean. In general, exception-handling exception-handling best practices contribute to the Code’s sustainability contribute to code sustainability.
66. What is the difference between Exception and Error?
Ans:
‘Exception’ and ‘Error’ are subclasses of ‘Throwable’ but relate to different class problems. Exceptions are those exceptions applications can catch and handle, mainly because it is expected or predictable (such as ‘IOException’ and ‘SQLException’). Errors, on the other hand, are serious problems that the application program should not attempt to catch; some examples include ‘OutOfMemoryError’ and ‘StackOverflowError’. Errors point to core defects in the JVM or system resources and lie entirely out of an application’s control.
67. What is the role of exceptions in unit testing?
Ans:
Exceptions can be used in unit testing to ensure proper error handling has been implemented in the Code. Test cases might be constructed to assert that certain exceptions are thrown under specific conditions. This way, the application will behave as it should when errors arise. With the help of such frameworks as JUnit, assertions can check if expected exceptions indeed occur. For example, ‘assertThrows’ allows developers to check if an exceptional condition is thrown at runtime.
68. What is the use of user-defined unchecked exceptions?
Ans:
- User-defined unchecked exceptions allow developers to define certain error conditions for the developed application. They are subclasses of ‘RuntimeException’, so they provide flexibility in error handling without being declared strictly.
- Custom unchecked exceptions let developers communicate better about application-specific problems to make the Code more comprehensible.
- They can grab context and create more informative error messages. This helps improve debugging and opens up the possibility of individualized error responses.
69. How to use nested try-catch blocks?
Ans:
- Exceptions can be caught at different levels of granularity across a method by using nested try-catch blocks. This approach allows for distinguishing between exceptions under various circumstances, while all error control remains accessible.
- For example, the outer try-catch block may catch a higher-level exception, whereas an inner try-catch block may address a more granular issue.
- This makes the Code explicit and decouples concerns, allowing specific responses to different error conditions. However, nested try-catch blocks can get complex, making the Code hard to read.
70. How do exceptions relate to asynchronous programming?
Ans:
Error handling can become confusing when using asynchronous programming, where exceptions may occur in callbacks or promises. Unlike synchronous Code, where exceptions can be caught directly, asynchronous contexts need more mechanism creation to catch errors that would occur during execution efficiently. For instance, JavaScript promises contain methods, such as ‘.catch()’, for error handling that may occur in asynchronous operations.
71. How can exception handling be implemented in lambda expressions?
Ans:
Exception handling in lambda expressions can be achieved by wrapping the lambda in a try-catch block. Since lambda expressions do not allow checked exceptions to be thrown directly, any checked exceptions must be caught within the lambda or rethrown as unchecked exceptions. For instance:
- RunnaJavatask = () -> {
- try {
- //Code that may throw an exception
- } catch (IOException e) {
- // Handle Exception
- }
- };
Alternatively, creating a utility method encapsulating exception handling can simplify lambda usage. This method encourages more readable and manageable Code, and proper handling of exceptions in lambdas enhances robustness.
72. What is NoSuchElementException, and when is it thrown?
Ans:
- ‘NoSuchElementException’ is a runtime exception thrown when an attempt to access an absent element in a collection or some iterator has been made.
- Generally, it occurs when trying to access any element from an empty collection or when there are no more elements to return ‘next()’ for an iterator without checking for aspects in advance.
- The error message means that the desired data isn’t available. It also ensures that the code checks correctly for the existence of elements before trying to access the element.
73. How does it, in turn, make debugging easier?
Ans:
- Exception handling makes debugging easier because it provides structure error reporting that helps locate bugs in Code.
- Exceptions caught and logged included stack traces that could pinpoint exactly where the error was, thus tracing the underlying problem. Developers can use meaningful exception types to recognize particular error conditions.
- Clear exception messages improve insight into the context in which a problem occurs. Issues are solved faster, and effective exception handling would, therefore, become the best means of stability for an application.
74. What is meant by stack overflow error and out-of-memory error?
Ans:
An out-of-memory error results when the call stack overflows its limit, mainly due to excessive recursion or deep method calls. It expresses the actuality of exhausted stack space reserved for method calls. In comparison, an out-of-memory error will be thrown when the JVM can no longer allocate more memory for objects due to the memory being exhausted.
75. What could the try block do to make the Code safer?
Ans:
The try block increases the safety of our Code by incorporating a mechanism for catching an exception, which could occur at runtime. In good programming, where potentially error-prone Code is enclosed in a try block, runtime crashes through unhandled exceptions can be avoided. Also guaranteed through proper use of try is graceful degradation; for example, fallback behaviours or informative error messages can be provided. Lastly, checks ensure critical cleanup operations are done regardless of success or failure.
76. What are some considerations that need to be made during the designing of exception hierarchies?
Ans:
- Certain considerations are made when designing exception hierarchies. The hierarchy should reflect the organic structure of the application, with all closely related exceptions together under a common superclass.
- This would make the application more understandable and error handling more intuitive. Meaningful messages and context also characterize custom exceptions.
- Controlling the depth of the hierarchy for usability purposes avoids unnecessary complexity. Consistent naming conventions and included documentation promote ease of understanding.
77. How does Java handlJavaceptions in multithreaded environments?
Ans:
Java handles exceptions in multithreaded environments by letting each thread independently manage its exceptions. An exception in a thread can be caught and handled inside the context of the thread, to which it can propagate to the uncaught exception handler of the thread in case no one catches it and then logs an error or takes remedial action. This ensures that failure in a thread does not disturb the execution of other threads.
78. What is the difference between RuntimeException and Error?
Ans:
- ‘RuntimeException’ is a subclass of ‘Exception’ that describes exceptions that can arise while executing the Java Virtual Machine normally.
- These are generally programming problems like ‘NullPointerException’ or ‘ArrayIndexOutOfBoundsException’, which a developer can avoid.
- In comparison, ‘Error’ denotes critical problems that are typically beyond the reach of an application, such as ‘OutOfMemoryError’ or ‘StackOverflowError’.
- While ‘RuntimeException’ is catchable and recoverable, ‘Error’ denotes fatal conditions normally, which should not be encapsulated programmatically. This aids in effective error management and recovery strategies.
79. In what way do customized error messages help improve the process of error handling?
Ans:
Customized error messages prove useful in error handling as they present specific context information on the exact error situation that has arisen. Meaningful information can easily allow developers to identify the type of problem. Hence, the processes of debugging and remedying can take place much more quickly. Customized messages can present relevant data in the form of names and values of parameters, method names, or even error codes; this allows for an increased understanding of the problem.
80. What is FileNotFoundException, and how does it occur?
Ans:
‘FileNotFoundException’ is a checked exception that arises when any attempt to access a file that doesn’t exist fails. This Exception is often when one attempts to open a file through classes such as ‘FileInputStream’, ‘FileReader,’ or ‘Scanner’. These can be due to wrong paths that lead to the file not being found, or maybe because of needing permission to access the file in question. Handling ‘FileNotFoundException’ will require that the developer ensures that the application has proper authorization and that it is indeed a file the application is trying to open that exists.
81. How does Exception handling affect the overall user experience of an application?
Ans:
Exception handling should enhance the user experience of applications since this is one of the primary ways through which errors in the application are prevented, and feedback is produced; with proper exception handling, the user gets an error message from the system describing exactly what has been wrong and how he should remedy the situation. Properly handled exceptions reduce frustration. Users would be given obscure error messages, or the application would crash.
82. What is the SuppressWarnings annotation used for?
Ans:
- ‘SuppressWarnings’ annotation specifies that certain compiler warnings should be ignored. This is useful when some warnings would otherwise appear unnecessary or a developer has a valid reason for bypassing them.
- It eliminates warning messages from cluttering the Code, indicating that the developer has inspected them. Common usages include ignoring unchecked warnings when using generics, deprecated method warnings, etc.
- However, unnecessary caution should be hidden from real problems. Suppressed warnings must be documented appropriately for better code maintainability.
83. How would one classify the exceptions in the severity model?
Ans:
- Exceptions may be classified into three tiers of severity: critical, major, and minor. Severe exceptions, wherein the application crashes or loses some vital data, are fatal and require immediate attention.
- Major exceptions impact the functionality but do not crash the application; instead, they have problems related to data integrity.
- Minor exceptions are relatively less serious and may be logged without impacting the application’s performance; these exceptions can then be classified for the strategies and resources to use in remedying such problems.
84. What is the difference between framework-specific exceptions and standard exceptions?
Ans:
Framework-specific exceptions are predefined based on a given framework or library’s functionality and usage pattern. They often include more specific information on the operations executed within that framework, possibly configuration problems or failure to access a resource. Standard exceptions are usually purpose-built and used within many different contexts, like the exceptions that are part of the Java core library.
85. What is an ‘SQLIntegrityConstraintViolationException’, and in what situation is it thrown?
Ans:
‘SQLIntegrityConstraintViolationException’ is one of the checked exceptions. This Exception is thrown if an operation violates the database integrity constraint of an SQL operation. This can happen during attempts to insert a duplicate primary key, when violating a foreign key constraint, or when trying to nullify a non-nullable column. The Exception, hence, gives insights into problems related to data integrity within the database. Handling this Exception properly means maintaining consistent and valid states of data.
86. How are throws applied within overridden methods?
Ans:
- In overridden methods, the ‘throws’ keyword can be applied to identify exceptions potentially thrown by the process so the subclass suitably warns of potential issues.
- When overriding a method, it is possible either to retain the declared exception types of the parent method or to declare a subset of those. Other subclasses can also throw unchecked exceptions without any limitation.
- Checked exceptions in a parent method must be stated in an overriding method unless handled within it. In this way, exception handling does not conflict within class hierarchies.
87. How does Exception handling affect performance tuning?
Ans:
- Heavy use of try-catch blocks will introduce overhead, especially if exceptions occur so frequently that they’re constantly thrown and caught.
- This overhead arises from the overhead required to capture stack traces and maintain exception states. Of course, this often has little effect on performance unless exceptions happen within tight loops in our Code.
- Proper exception handling and minimizing the frequency of exceptions will help ensure optimal performance. In summary, although critical for robustness, exception handling should be implemented with performance in mind.
88. How can a global exception handler be implemented?
Ans:
A global exception handler can be implemented by defining a centralized mechanism that catches unhandled exceptions across the application. That can be achieved in Java usingJavaread.setDefaultUncaughtExceptionHandler()’ for uncaught exceptions in the thread and the framework-specific approach for web applications, perhaps using ‘@ControllerAdvice’ of Spring in this case. The specific logic would be to log exceptions and provide user-readable error messages.
89. What are the consequences of not catching exceptions?
Ans:
Not catching exceptions can have severe effects, including application crashes, data corruption, or unintended behaviour. Failure to catch an exception may allow it to propagate up the call stack, thus causing uncontrolled shortcomings of the application, leading to loss of users’ trust and satisfaction. Users may experience frustrating application crashes or erratic states instead of the expected behaviour.
90. How can conditional logging be used with exceptions?
Ans:
Applying conditional logging, developers would log exceptions according to certain criteria or conditions and avoid noisy logs at unnecessary times. For instance, a developer may want to log an exception only if it happens several times or reaches certain levels of severity. It can be achieved through the use of flags or counters for counting the occurrences of exceptions. Conditional logging reduces the overhead related to overlogging, hence improving performance. It also provides better logs that are focused on the main problems.