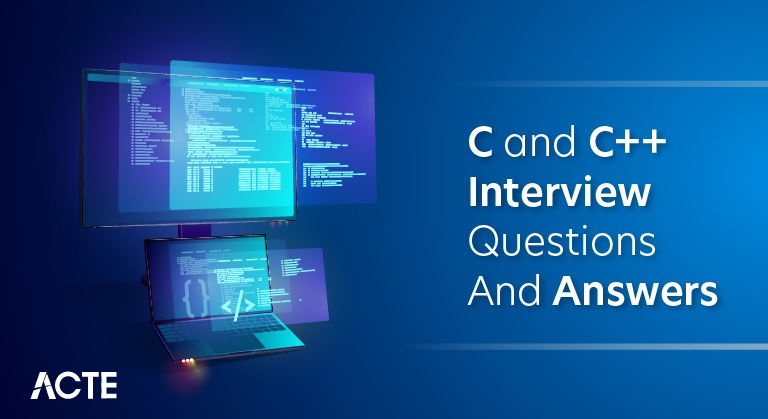
Best C & C++ Interview Questions & Answers [FREQUENTLY ASK]
Last updated on 03rd Jun 2020, Blog, Interview Questions
The C and C++ Interview Questions have been designed specially to get you acquainted with the nature of questions you may encounter during your interview for the subject of C++. As good interviewers hardly plan to ask any particular question during the interview, normally questions start with some basic concept of the subject and later they continue based on further discussion and what the candidate answers.
Here is a list of the most frequently asked C and C++ Interview Questions and Answers in technical interviews, suitable for both freshers and experienced professionals at any level.
1. What is C++?
Ans:
C++ is a versatile programming language that extends C with features like classes, objects, and inheritance, facilitating both procedural and object-oriented programming.
2. What is an object?
Ans:
A class instance that contains both data and behavior is known as an object. It depicts a thing or idea that exists in reality.
3. Explain the difference between ‘new’ and ‘malloc’.
Ans:
‘new’ is used to allocate memory for objects and invoke constructors, while ‘malloc’ allocates raw memory without invoking constructors.
4. What is a constructor?
Ans:
An object is initialized upon creation using a constructor, a specific member function. It lacks a return type and shares the same name as the class.
5. What is operator overloading?
Ans:
Operator overloading allows you to redefine the behavior of operators like +, -, etc., for user-defined data types.
6. What is the ‘this’ pointer in C++?
Ans:
‘this’ is a pointer that points to the current object. It’s used to access the object’s members and differentiate them from local variables.
7. Explain function overloading and function overriding.
Ans:
Function overloading is having multiple functions with the same name but different parameter lists in the same scope. Function overriding occurs when a derived class provides its own implementation for a virtual function declared in a base class.
8. What is a virtual function?
Ans:
A virtual function is declared in a base class with the ‘virtual’ keyword. It enables dynamic binding, allowing the correct function to be called based on the object’s type.
9. What is a destructor?
Ans:
When an object exits its scope or is expressly eliminated, a destructor, a specific member function, decomposes its resources.
10. What is a template in C++?
Ans:
A template is a blueprint for creating generic functions or classes. It allows you to work with different data types using a single definition.
11. What is the difference between a class and a struct in C++?
Ans:
In a class, members are private by default, while in a struct, members are public by default. Both can have methods and data.
12. What is RAII?
Ans:
RAII (Resource Acquisition Is Initialization) is a C++ programming concept where resource management, like memory allocation and deallocation, is tied to object lifetime.
13. Explain smart pointers in C++.
Ans:
Smart pointers are classes that manage the lifetime of dynamically allocated objects. Examples include unique_ptr, shared_ptr, and weak_ptr.
14. What are the access specifiers in C++?
Ans:
Access specifiers (public, private, protected) determine the visibility of class members. ‘Public’ members are accessible from outside the class.
15. What is the difference between a shallow copy and a deep copy?
Ans:
A shallow copy copies the object’s members, including pointers, but not the pointed-to objects. A deep copy creates new objects and copies their contents.
16. How does exception handling work in C++?
Ans:
Exceptions are thrown using ‘throw’ and caught using ‘try’ and ‘catch’. ‘catch’ handles exceptions and can be used to gracefully handle errors.
17. What is a lambda expression?
Ans:
An anonymous function that can be defined in-line is a lambda expression. It is employed in functional programming and compact code.
18. Explain the ‘const’ keyword in C++
Ans:
‘const’ is used to indicate that a variable, parameter, or member function does not modify the value it refers to.
19. What is the preprocessor in C++?
Ans:
The preprocessor is a phase of compilation that processes directives starting with ‘#’, like ‘#include’ and ‘#define’, before the actual code is compiled.
20. What is the difference between stack and heap memory allocation?
Ans:
Stack memory allocation is fast and managed by the compiler, used for local variables. Heap memory allocation is slower, requires manual management, and is used for dynamically allocated objects.
21. What is a namespace in C++?
Ans:
A namespace is a mechanism to organize code by grouping related classes, functions, and variables under a common name to prevent naming conflicts.
22. Explain the concept of multiple inheritance.
Ans:
Multiple inheritance is when a class inherits properties and behaviors from more than one base class. C++ supports multiple inheritance, which can lead to the “diamond problem” of ambiguity.
23. What are inline functions?
Ans:
Inline functions are small functions whose code is directly inserted at the call site to reduce the function call overhead. They are declared with the ‘inline’ keyword.
24. What is a static member of a class?
Ans:
Static members are the property of the class rather than specific objects. It is accessible without generating an instance and is shared by all class members.
25. What is the role of a friend function?
Ans:
A friend function is not a member of the class but can access its private and protected members. It’s often used to achieve certain functionalities while maintaining encapsulation.
26. What is a pointer to a function?
Ans:
A pointer to a function stores the memory address of a function. It can be used to dynamically call different functions during runtime.
- Class Bird
- {
- char name [50];
- public:
- Bird ()
- {
- printf(“\nThis is Default Constructor of Class Bird\n”)
- }
- };
27. Explain the scope resolution operator (::)
Ans:
The scope of a class or namespace member is specified using the scope resolution operator, to be precise. It can be used to access static members, global variables, or overridden base class members.
For example:
- Class Bird
- { char name [50];
- public:
- ~Bird ()
- {
- printf(“\nThis is Destructor of Class Bird\n”)
- }
- };
28. What is the difference between ‘delete’ and ‘delete[]’?
Ans:
‘delete’ is used to deallocate memory allocated with ‘new’, while ‘delete[]’ is used to deallocate memory allocated with ‘new[]’ for arrays.
29. How does the virtual destructor work?
Ans:
If a base class has a virtual destructor, then when an object of a derived class is deleted through a base class pointer, the appropriate derived class destructor is called, ensuring proper cleanup.
30. What are C++ templates used for?
Ans:
C++ templates are used to write generic code that can work with different data types. They enable you to define classes or functions with placeholders for types or values.
31. Explain the difference between deep copy and shallow copy.
Ans:
Shallow copy duplicates the memory addresses of objects, so changes in one object affect the other. Deep copy duplicates the entire object’s content, creating separate instances.
32. What is the purpose of ‘const’ member functions?
Ans:
‘const’ member functions promise not to modify the object’s state. They can be called on both const and non-const objects.
33. What is a static_cast in C++?
Ans:
‘static_cast’ is a type of C++ casting used for safer type conversion between related data types. It’s checked during compile-time.
34. What are the differences between a class template and a function template?
Ans:
A class template defines a blueprint for creating classes with members, while a function template defines a blueprint for creating functions that can work with various data types.
35. How can you prevent an object from being copied in C++?
Ans:
You can make the copy constructor private or delete it to prevent objects from being copied, thus controlling their copying behavior.
36. What is the purpose of ‘volatile’ keyword in C++?
Ans:
‘volatile’ tells the compiler that a variable’s value can change at any time, preventing certain optimizations that might lead to incorrect behavior.
37. How is memory leak avoided in C++?
Ans:
Memory leaks are avoided by ensuring proper deallocation of dynamically allocated memory using ‘delete’ or smart pointers and managing object lifetimes.
38. Explain the role of the ‘new’ and ‘delete’ operators.
Ans:
‘new’ is used to dynamically allocate memory for objects on the heap, invoking the constructor. ‘delete’ is used to deallocate memory allocated with ‘new’, invoking the destructor.
39. What is a reference variable in C++?
Ans:
A reference variable is an alias for an existing variable. Once assigned, it always refers to the same object and can’t be re-assigned.
40. What are the differences between ‘public’, ‘private’, and ‘protected’ inheritance?
Ans:
‘public’ inheritance makes public members of the base class public in the derived class, ‘private’ inheritance makes them private, and ‘protected’ inheritance makes them protected.
41. What is the difference between a shallow copy and a deep copy?
Ans:
A shallow copy copies the memory addresses of objects, while a deep copy duplicates the entire object, creating independent instances.
42. Explain the use of ‘auto’ keyword in C++.
Ans:
The ‘auto’ keyword eliminates the need for explicit type declarations by allowing the compiler to infer a variable’s data type from its initializer.
43. What are the advantages of using references over pointers in C++?
Ans:
References are safer as they cannot be null and offer a more natural syntax. They also eliminate the need for dereferencing.
44. What is CRTP (Curiously Recurring Template Pattern)?
Ans:
CRTP is a C++ template pattern where a derived class template inherits from a base class template with the derived class itself as
45. Explain the use of ‘decltype’ in C++.
Ans:
‘decltype’ is used to deduce the type of an expression at compile-time. It’s often used in template metaprogramming and for declaring variables with the same type as an existing expression.
46. What is the ‘nullptr’ keyword in C++?
Ans:
‘nullptr’ is a keyword introduced in C++11 that represents a null pointer. It’s recommended to use ‘nullptr’ instead of ‘NULL’ for better type safety.
47. What is the Standard Template Library (STL)?
The STL is a collection of C++ template classes and functions that provide common data structures (like vectors, lists, maps) and algorithms (like sorting and searching).
48. What distinguishes’std::vector’ from’std::array’?
Ans:
‘std::vector’ is a dynamically resizable array with automatic memory management, while ‘std::array’ is a fixed-size array with a compile-time size.49. How can you sort elements in a container using the STL?
Ans:
You can use the ‘std::sort’ algorithm from the STL to sort elements in a container, providing iterators that define the range to be sorted.
50. What are move semantics in C++?
Ans:
Move semantics allow resources, like dynamically allocated memory, to be transferred from one object to another without unnecessary copying, enhancing performance.
51. Explain the role of ‘decltype(auto)’ in C++.
Ans:
‘decltype(auto)’ is used to deduce the type of an expression just like ‘decltype’, but it also retains the value category of the expression.
52. What is a functor in C++?
Ans:
A functor is an object that can be called as if it were a function. It’s achieved by overloading the function call operator ‘operator()’ in a class.
53. How does the ‘override’ keyword work in C++?
Ans:
When a function in a derived class is intended to override a virtual function from a base class, the term “override” is used to indicate this. It improves the readability of the code and eliminates errors.
54. What are smart pointers in C++?
Ans:
Smart pointers are classes that manage the lifetime of dynamically allocated objects. ‘std::unique_ptr’, ‘std::shared_ptr’, and ‘std::weak_ptr’ are examples.
55. Explain the role of ‘const’ in member functions.
Ans:
‘const’ in a member function’s declaration promises not to modify the object’s state, allowing the function to be called on const objects.
56. How does the ‘const_cast’ operator work?
Ans:
‘const_cast’ is used to add or remove the ‘const’ qualifier from a variable. It’s often used to modify non-const objects within a ‘const’ context.
57. What are inline namespaces in C++?
Ans:
Inline namespaces allow you to separate a namespace’s interface from its implementation without adding an additional layer of nesting.
58. What is the ‘final’ keyword in C++?
Ans:
‘final’ is used to prevent a class from being inherited further or a virtual function from being overridden in derived classes.
59. Explain the role of ‘decltype’ with expressions.
Ans:
‘decltype’ deduces the type of an expression at compile-time. It’s often used to declare variables with the same type as a specific expression.
60. What is the ‘auto’ return type in C++?
Ans:
‘auto’ return type allows you to return values from functions without explicitly specifying the return type. The type is deduced from the return statement.
61. What is type erasure in C++?
Ans:
Type erasure is a technique to store objects of different types in a container while preserving a common interface for interaction.
62. Explain the purpose of ‘std::move’ in C++.
Ans:
‘std::move’ is used to convert an object into an rvalue, enabling efficient resource transfer and move semantics.
63. How can you iterate through a container using a range-based for loop?
Ans:
A range-based for loop iterates through each element of a container without needing explicit iterators, simplifying iteration code.
64.What are lambda expressions in C++?
Ans:
Lambda expressions are anonymous functions that can be defined in-line. They provide a concise way to define functions for one-time use.
65. Explain the difference between ‘std::list’ and ‘std::vector’.
Ans:
‘std::list’ is a doubly linked list with dynamic memory allocation for elements, while ‘std::vector’ is a dynamic array with contiguous memory allocation.
66. What is the difference between ‘std::make_shared’ and ‘std::shared_ptr’?
Ans:
‘std::make_shared’ creates a shared pointer by allocating memory for both the control block and the object in a single allocation, optimizing memory usage.
67. How do you implement a copy constructor for a class?
Ans:
A copy constructor is implemented by defining a constructor that takes a reference to another object of the same class and copies its data members.
68. What are type traits in C++?
Ans:
Type traits are a set of templates that provide information about the properties of types. They can be used for compile-time type analysis.
69. Explain the use of ‘std::swap’ function.
Ans:
‘std::swap’ is used to swap the values of two objects of the same type. It’s more efficient than using assignment for user-defined types.
70. How does the ‘std::unique’ algorithm work?
Ans:
‘std::unique’ is used to remove consecutive duplicate elements from a range. It returns an iterator to the new end of the range after removing duplicates.
71. What is SFINAE (Substitution Failure Is Not An Error)?
Ans:
SFINAE is a C++ template mechanism where a compiler doesn’t treat a failed template substitution as an error but tries to find alternative overloads.
72. Explain the purpose of ‘std::cin’ and ‘std::cout’.
Ans:
‘std::cin’ is the standard input stream used to read data from the user, and ‘std::cout’ is the standard output stream used to display data to the console.
73. How do you reverse a string in C++?
Ans:
You can reverse a string using ‘std::reverse’ algorithm from the STL or by swapping characters from the beginning and end of the string.
74. What are C++ attributes (attributes syntax)?
Ans:
C++ attributes provide additional information to the compiler about declarations. They are introduced using the syntax [[attribute]].
75. Explain the difference between ‘std::map’ and ‘std::unordered_map’.
Ans:
‘std::map’ is an ordered associative container that stores key-value pairs, while ‘std::unordered_map’ is a hash table-based container for fast key-value lookups.
76. How do you create a copy of an object in C++?
Ans:
Depending on the situation, you can either use the copy constructor or the assignment operator to make a copy of an object.
77. What is the difference between a function declaration and a function definition?
Ans:
A function declaration provides information about a function’s name, return type, and parameters. A function definition includes the implementation of the function.
78. Explain the purpose of ‘std::initializer_list’.
Ans:
‘std::initializer_list’ allows you to initialize objects with a list of values. It’s often used in constructor overloads and range-based for loops.
79. How does the ‘std::move’ function work with rvalue references?
Ans:
‘std::move’ casts an object to an rvalue reference, enabling move semantics and resource transfer in functions.
80. What is a virtual destructor?
Ans:
When a derived class object is deleted using a base class pointer, the proper destructor is invoked thanks to the use of a virtual destructor.
81. How do you prevent object slicing in C++?
Ans:
When an object of a derived class is assigned to an object of a base class, object slicing takes place. leading to the loss of derived class-specific data. To prevent it, use pointers or references.
82. What is the role of the ‘volatile’ keyword in C++?
Ans:
‘volatile’ indicates that a variable’s value can change at any time, preventing certain compiler optimizations that might lead to incorrect behavior.
83. Explain RAII (Resource Acquisition Is Initialization).
Ans:
RAII is a C++ programming concept where resource management is tied to object lifetime. Resources like memory or files are acquired during object creation and released during destruction.
84. What is the purpose of ‘std::async’ in C++?
Ans:
‘std::async’ is used to asynchronously launch a function and retrieve a future object that can be used to get the result or status of the function’s execution.
85. What is a unique lock in C++ threading?
Ans:
‘std::unique_lock’ is a C++ synchronization primitive used for mutual exclusion. It provides more flexibility compared to ‘std::lock_guard’.
86. How does the ‘constexpr’ keyword work?
Ans:\
When a function or variable can be evaluated at compile time, the notation “constexpr” is used. It is frequently employed to maintain constants and optimise performance.
87. Explain the use of ‘std::forward’ in C++.
Ans:
‘std::forward’ is used to preserve the value category (lvalue or rvalue) of a function argument while forwarding it to another function.
88. What is CRTP (Curiously Recurring Template Pattern)?
Ans:
CRTP is a C++ template pattern where a derived class template inherits from a base class template with the derived class itself as the template parameter.
89. How do you handle memory management in C++?
Ans:
Memory management involves allocating memory using ‘new’ or smart pointers and deallocating it using ‘delete’ or ‘delete[]’ to avoid memory leaks.
90. Explain the role of ‘mutable’ in C++.
Ans:
‘mutable’ is used to allow modification of a member variable in a ‘const’ member function, even though the object is considered ‘const’.
91. What is a conversion constructor?
Ans:
A conversion constructor is a constructor that takes a single argument of a different type and is used to implicitly convert one type to another.
92. How do you implement a copy assignment operator for a class?
Ans:
The copy assignment operator is implemented by defining an operator= function that takes a reference to another object of the same class and copies its data members.
93. Explain the use of ‘std::tuple’ in C++.
Ans:
‘std::tuple’ is an ordered collection of elements of different types. It’s often used to return multiple values from a function.
94. What are the differences between C++03 and C++11?
Ans:
C++11 introduced features like move semantics, lambda expressions, ‘nullptr’, smart pointers, and more, enhancing the language and standard library.
95. What is the purpose of ‘std::async’ in multithreading?
Ans:
‘std::async’ is used to asynchronously launch a function and retrieve a future object that can be used to get the result or status of the function’s execution.
96. How do you handle exceptions in C++?
Ans:
Exceptions are handled using ‘try’, ‘catch’, and ‘throw’ keywords. ‘try’ contains code that might throw an exception, and ‘catch’ handles the exception.
97. What is a ‘noexcept’ specifier?
Ans:
‘noexcept’ is used to indicate that a function doesn’t throw exceptions. It’s useful for optimizing code and improving exception safety.
98. Explain the use of ‘std::tie’ in C++.
Ans:
‘std::tie’ is used to unpack the elements of a tuple into individual variables. It’s often used with ‘std::tuple’ for multiple return values.
99. What are the differences between ‘std::shared_ptr’ and ‘std::weak_ptr’?
Ans:
While’std::weak_ptr’ offers a non-owning reference to an object maintained by’std::shared_ptr,”std::shared_ptr’ controls the ownership of dynamically allocated objects.
100. How do you optimize performance in C++?
Ans:
Performance optimization involves using efficient algorithms, avoiding unnecessary copying, using move semantics, optimizing memory usage, and profiling to identify bottlenecks.