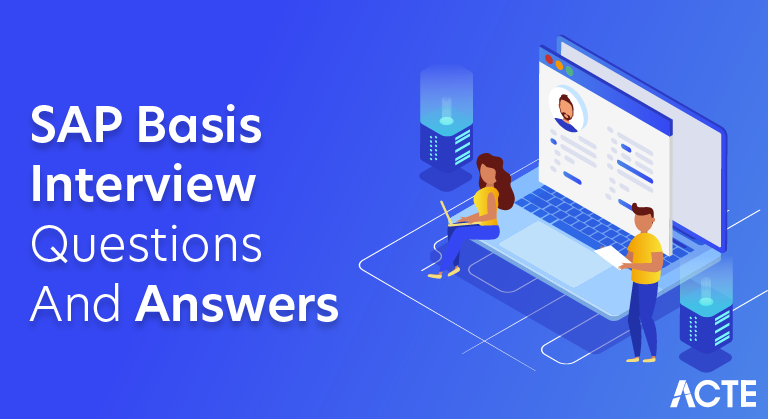
40+ [REAL-TIME] Array Interview Questions and Answers
Last updated on 20th Apr 2024, Popular Course
An Array is a data structure that stores a collection of elements in contiguous memory locations, typically of the same type. Each array component is accessed by its index, a numeric value indicating its position in the array. Arrays are commonly used in programming languages to store and manipulate data lists, such as numbers or strings.
1. What is an array?
Ans:
An array is a data structure that stores a collection of elements of the same type in contiguous memory locations. Each array component is accessed by its index, representing its position in the array. Arrays provide a convenient way to organize and manipulate data in computer programs. They offer efficient access to individual elements and support operations like insertion, deletion, and sorting. Arrays can be one-dimensional, two-dimensional, or multidimensional, depending on the number of indices needed to access their elements.
2. What are the advantages of using arrays?
Ans:
- Efficient access with constant-time indexing.
- Memory efficiency due to contiguous allocation.
- Simple syntax for element access.
- Versatility in storing elements of the same data type.
- Support for random access to elements.
- Performance optimization for various algorithms.
3. What are the limitations of arrays?
Ans:
- Fixed-size
- Homogeneous elements
- Memory allocation
- Inefficient insertions and deletions
- Searching inefficiency
- Static data structure
4. Explain the concept of multidimensional arrays.
Ans:
Multidimensional arrays are arrays that have more than one dimension. They are often used to represent tables, matrices, or grids. In languages like C and Java, multidimensional arrays are implemented as arrays of arrays. Multidimensional arrays are arrays that have more than one dimension. Instead of having one row of elements, they have multiple rows and columns. This creates a grid-like structure where each element is identified by its row and column index. For example, a 2D array is like a table with rows and columns, while a 3D array adds depth to the structure, like a cube.
5. What is the difference between an array and a linked list?
Ans:
Aspect | Array | Linked List |
---|---|---|
Data Structure | Contiguous block of memory elements | Elements are stored in nodes with pointers |
Memory Allocation | Static memory allocation | Dynamic memory allocation |
Insertion and Deletion | Insertion and deletion operations may be slower, especially in the middle, as it requires shifting elements | Insertion and deletion operations are generally faster, especially in the middle, as it only requires updating pointers |
Access Time | Random access is faster (O(1)) | Sequential access is faster (O(n)) |
Memory Usage | May lead to wasted memory if the array is resized frequently or not fully utilized | Memory-efficient as memory is allocated dynamically |
6. How do you access elements in a one-dimensional array?
Ans:
Elements in a one-dimensional array are accessed using their index. For example, array[i] accesses the element at index i. In a one-dimensional array, you access elements by using their index. Each array component is assigned a unique index starting from 0 for the first element. You access elements by specifying the index within square brackets after the array name.
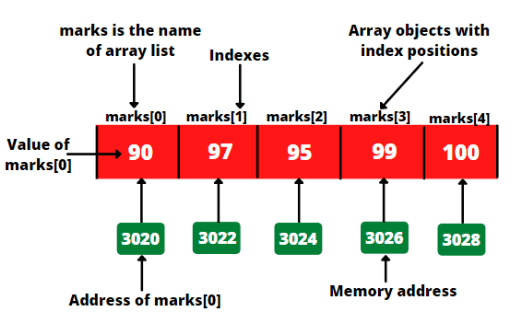
7. What is the time complexity of accessing an element in an array?
Ans:
- The time complexity of accessing an element in an array is O(1), which means it takes constant time regardless of the size of the array.
- This is because arrays provide direct access to elements using their indices, and accessing any element requires only a single operation involving arithmetic calculation to compute the memory address of the desired element.
- Therefore, accessing an element in an array is very efficient and does not depend on the array’s size.
8. How do you initialize an array in various programming languages?
Ans:
Arrays can be initialized in various programming languages using syntax like int[] arr = {1, 2, 3}; in Java, int arr[] = {1, 2, 3}; in C/C++, and arr:= [1, 2, 3] in Python.
9. Explain the concept of dynamic arrays.
Ans:
Dynamic arrays, also known as resizable arrays or ArrayLists, automatically resize themselves when they reach capacity. They typically double in size when resizing to achieve amortized constant-time insertion.
10. How do you find the length of an array?
Ans:
The length of an array can be found using a built-in function or property provided by the programming language. For example, in Java, you can use arr. length, in C/C++, you can use sizeof(arr)/sizeof(arr[0]); and in Python, you can use len(arr)
11 . What is the difference between a static and dynamic array?
Ans:
A static array has a fixed size determined at compile time and cannot be changed during runtime. On the other hand, a dynamic array can resize itself dynamically during runtime to accommodate more elements as needed.
12. Explain how memory is allocated for arrays in programming languages like C and C++.
Ans:
In languages like C and C++, memory for arrays is typically allocated on the stack or the heap. Static arrays are usually allocated on the stack, while dynamic arrays are allocated on the heap using functions like malloc() or new.
13 . What is the difference between an array and a vector in C++?
Ans:
- In C++, an array is a fixed-size collection of elements stored in contiguous memory locations, while a vector is a dynamic array that can resize itself automatically when needed.
- Vectors also provide additional functionality, such as bounds-checking and methods for easy manipulation.
14. What is the concept of time complexity, and how does it relate to arrays?
Ans:
Time complexity measures an algorithm’s time to run as a function of the input length. Arrays are often analyzed in terms of time complexity for various operations such as access, search, insertion, and deletion to evaluate their efficiency.
15. Explain the concept of array indexing and its importance.
Ans:
Array indexing refers to accessing individual elements of an array using their position or index. It is essential because it allows for efficient retrieval and manipulation of data stored in the array, enabling algorithms to perform operations based on the specific elements they need.
16. What are sparse arrays, and how are they represented?
Ans:
Sparse arrays are arrays that contain a large number of elements with the same default value, typically zero or null. Rather than storing all elements, sparse arrays only store the non-default values and indices, reducing memory usage.
17. Discuss the concept of jagged arrays and provide an example.
Ans:
Jagged arrays, also known as arrays of arrays, are arrays whose elements are arrays themselves, and each sub-array can have a different length. An example of a jagged array in C# is int[][] jagged array = new int[3][], where each sub-array can have a different length.
18. Explain the concept of array slicing and its use cases.
Ans:
Array slicing refers to extracting a portion of an array to create a new variety. It is commonly used to work with subsets of data, perform operations on specific segments of an array, and pass array sections to functions.
19. What are the different ways to traverse an array?
Ans:
Arrays can be traversed using techniques such as iteration with a loop(e.g., for loop, while loop), recursion, and built-in functions provided by the programming language.
20. Discuss the concept of array manipulation and provide examples of joint operations.
Ans:
Array manipulation involves various operations on arrays, such as sorting, searching, merging, splitting, reversing, and resizing. These operations are fundamental for efficiently processing and analyzing data stored in arrays.
21. What is the difference between an array and a set?
Ans:
An array is an ordered collection of elements stored in contiguous memory locations, allowing duplicate elements and providing indexed access. A set is an unordered collection of unique elements, typically implemented using hash tables or trees, and does not allow duplicates.
22. Explain the concept of array sorting algorithms and provide examples.
Ans:
Array sorting algorithms are algorithms used to rearrange the elements of an array in a specific order, such as ascending or descending. Examples include bubble sort, selection sort, insertion sort, merge sort, quick sort, and heap sort, each with its trade-offs in terms of time and space complexity.
23. What is the difference between a one-dimensional and a two-dimensional array?
Ans:
A one-dimensional array represents a single list of elements arranged sequentially in memory, accessed using a single index. A two-dimensional array represents a matrix or grid of elements organized into rows and columns, accessed using two indices—one for the row and one for the column.
24. Explain the concept of array searching algorithms and provide examples.
Ans:
Array searching algorithms are algorithms used to find the presence or location of a specific element within an array. Examples include linear search, binary search (for sorted arrays), interpolation search, and exponential search, each with different time complexities and applicability depending on the properties of the variety.
25. What is the role of arrays in dynamic programming?
Ans:
Arrays play a crucial role in dynamic programming by efficiently storing and retrieving intermediate results of subproblems. Dynamic programming algorithms often use arrays to memoize solutions to overlapping subproblems, reducing redundant computations and improving runtime complexity.
26. Discuss the concept of array manipulation using pointers in C/C++.
Ans:
- In C/C++, arrays and pointers are closely related, allowing for efficient manipulation of array elements using pointer arithmetic.
- Pointers can access and modify array elements directly, iterate over array elements, and perform dynamic memory allocation for arrays using pointer-based techniques like dynamic memory allocation and deallocation.
27. What are the differences between arrays and strings?
Ans:
- Arrays are a general-purpose data structure for storing a collection of elements of the same type.
- In contrast, strings are a specific type of array used to represent sequences of characters.
- Strings often have additional functionality for string-specific operations such as concatenation, comparison, and substring extraction.
28. Explain the concept of array buffering and its importance in input/output operations.
Ans:
Array buffering temporarily stores data in an array before performing input/output operations, such as reading from or writing to files or network streams. Array buffering helps improve performance by reducing the number of system calls and minimizing data transfer overhead, especially when dealing with large datasets.
29. What are the various ways to initialize an array with predefined values?
Ans:
Arrays can be initialized with predefined values using explicit initialization with a list of values, initialization using loops or functions, copying from another variety, or built-in array initialization functions provided by the programming language or libraries.
30. Discuss the concept of array partitioning and its use cases.
Ans:
Array partitioning involves dividing an array into two or more subarrays based on specific criteria, such as a pivot value or a predicate function. Everyday use cases include sorting algorithms like quicksort and partitioning arrays based on a particular condition for further processing or analysis.
31. Explain the concept of array reversal and provide an example algorithm.
Ans:
Array reversal involves reversing the order of elements in an array. One standard algorithm for reversing an array is to iterate over the first half of the array and swap each element with its corresponding element from the second half, effectively reversing the array in place.
32. Discuss the concept of circular arrays and their applications.
Ans:
Circular arrays are arrays where the end of the array wraps around to the beginning, forming a loop. They are often used in applications where elements must be continuously cycled or rotated, such as implementing circular buffers in data structures or simulating circular motion in graphics programming.
33. What are dynamic arrays, and how are they implemented in programming languages like Python and Java?
Ans:
- Dynamic arrays, also known as resizable arrays or ArrayLists, automatically resize themselves when they reach capacity.
- In languages like Python, dynamic arrays are implemented using built-in data structures like lists, which handle resizing internally.
- In Java, dynamic arrays are implemented using the ArrayList class, which dynamically resizes its underlying array as needed.
34. Explain the concept of array copying and its importance.
Ans:
Array copying involves creating a new array and copying the elements of an existing array into it. This operation is essential for creating backups of arrays, passing arrays to functions without modifying the original, and performing operations on subsets of arrays while preserving the original data.
35. Discuss the concept of array splicing and provide an example.
Ans:
Array splicing involves removing a portion of an array and optionally replacing it with new elements, effectively modifying the array in place. For example, the splice() method in Python can remove elements from a variety at specific indices and insert new elements in their place.
36. What are jagged arrays, and how are they different from multidimensional arrays?
Ans:
- Jagged arrays, also known as arrays of arrays, are arrays whose elements are arrays themselves, and each sub-array can have a different length.
- In contrast, multidimensional arrays represent arrays with more than one dimension, where each dimension has a fixed size, and elements are accessed using multiple indices.
37. Explain the concept of array concatenation and provide examples of its use.
Ans:
Array concatenation involves combining the elements of two or more arrays to create a single array. This operation is helpful for tasks such as merging multiple arrays, joining arrays horizontally or vertically, and creating larger arrays from smaller ones.
38. What are sparse matrices, and how are they represented using arrays?
Ans:
Sparse matrices are matrices where the majority of elements are zero. They are often represented using arrays to store only the non-zero elements and their row and column indices, reducing memory usage and improving efficiency for sparse matrix operations.
39. Discuss the concept of array shuffling and provide examples of shuffling algorithms.
Ans:
Array shuffling involves randomly reordering the elements of an array. Examples of shuffling algorithms include the Fisher-Yates shuffle, also known as the Knuth shuffle, which iterates over the array and swaps each aspect with a randomly selected element from the remaining unshuffled portion of the array.
40. What are ragged arrays, and how are they different from jagged arrays?
Ans:
- Ragged arrays are arrays where the length of each sub-array can vary independently, similar to jagged arrays.
- However, unlike jagged arrays, ragged arrays do not have a fixed number of sub-arrays, and each sub-array can have a different number of elements.
- Ragged arrays are often used in dynamic programming and computational geometry.
41. Explain the concept of array rotation and provide examples of rotation algorithms.
Ans:
Array rotation involves shifting the elements of an array cyclically by a certain number of positions. One standard algorithm for array rotation is the reversal algorithm, where the array is reversed in place in two steps: first, the elements up to the rotation point are reversed, and then the remaining elements are reversed.
42 . Discuss the concept of sparse arrays and their representation in memory.
Ans:
Sparse arrays are arrays where most elements are empty or contain a default value. They are typically represented using data structures that store only the non-empty or non-default elements and their indices, reducing memory usage compared to dense arrays.
43. What are associative arrays, and how are they different from regular arrays?
Ans:
Associative arrays, also known as maps, dictionaries, or hash tables, are arrays where each element is associated with a unique key instead of an index. They allow for efficient lookup, insertion, and deletion of elements based on their keys, unlike regular arrays where elements are accessed using numeric indices.
44. Discuss the concept of array mapping and provide examples of mapping operations.
Ans:
Array mapping involves applying a function to each element of an array to transform it into another value. Examples of mapping operations include:
- Applying mathematical functions to numerical arrays.
- Converting string arrays to uppercase or lowercase.
- Transforming arrays of objects into arrays of specific attributes or properties.
45. What is the role of arrays in data structures such as stacks and queues?
Ans:
Arrays are the underlying data structure for implementing stacks and queues. In a stack, arrays store elements with Last-In-First-Out (LIFO) semantics, while in a queue, arrays store elements with First-In-First-Out (FIFO) semantics.
46. Explain the concept of array filtering and provide examples of filtering operations.
Ans:
Array filtering involves selecting a subset of elements from an array based on a specific condition or criteria. Examples of filtering operations include:
- Selecting even or odd numbers from a numerical array.
- Filtering strings based on a particular substring or pattern.
- Selecting elements that satisfy specific criteria from a variety of objects.
47. What are the advantages and disadvantages of using arrays compared to other data structures?
Ans:
The advantages of using arrays include constant-time access to elements, efficient memory usage for homogeneous data, and support for random access and indexing. However, arrays have limitations such as fixed size, inefficiency for dynamic resizing, and lack of support for efficient insertion and deletion operations in the middle.
48. Discuss the concept of array transposition and provide examples of transposition operations.
Ans:
Array transposition involves converting rows into columns and vice versa, effectively rotating a two-dimensional array by 90 degrees. Examples of transposition operations include converting a matrix from row-major order to column-major order, or vice versa, and performing matrix operations such as matrix multiplication and inversion.
49. What are circular queues, and how are they implemented using arrays?
Ans:
Circular queues are queues where the front and rear pointers wrap around to the beginning of the array when they reach the end, forming a circular buffer. They are typically implemented using arrays with additional logic to handle wrap-around and prevent overflow or underflow conditions.
50. Explain the concept of array slicing and provide examples of slicing operations.
Ans:
Array slicing involves extracting a contiguous subset of elements from an array to create a new array. Examples of slicing operations include selecting a range of elements from an array using start and end indices, extracting specific columns or rows from a two-dimensional array, and creating views or subarrays of larger arrays without copying the data.
51. Discuss the concept of dynamic arrays and their advantages over static arrays.
Ans:
- Dynamic arrays, also known as resizable arrays, can dynamically resize during runtime to accommodate various elements.
- Unlike static arrays, which have a fixed size determined at compile time, dynamic arrays offer flexibility in memory allocation.
- They can grow or shrink as needed, making them suitable for scenarios where the data size is unknown or may change over time.
52. What is the difference between an array and a linked list? Compare their advantages and disadvantages.
Ans:
- Arrays store elements in contiguous memory locations and provide constant-time access to elements using their indices.
- On the other hand, Linked lists store elements in nodes scattered throughout memory and require sequential traversal to access elements.
- Arrays offer efficient random access and better cache locality but have fixed sizes and expensive insertion/deletion operations.
- Linked lists support dynamic resizing and efficient insertion/deletion but have slower access times and higher memory overhead due to pointers.
53. Explain the concept of array cloning and its use cases.
Ans:
Array cloning involves creating a deep copy of an array and duplicating the array structure and elements. This operation is helpful for tasks such as creating independent copies of arrays for modification without affecting the original, implementing undo/redo functionality, and passing arrays to functions without modifying the original data.
54. What are jagged arrays, and how are they implemented in languages like C# and JavaScript?
Ans:
Jagged arrays, also known as arrays of arrays, are arrays whose elements are arrays themselves, and each sub-array can have a different length. In languages like C# and JavaScript, jagged arrays can be implemented using array literals with nested arrays of varying lengths, providing flexibility in representing irregular data structures or matrices.
55. Discuss the concept of array flattening and provide examples of flattening operations.
Ans:
Array flattening involves converting a multidimensional array into a one-dimensional array by concatenating its elements. Examples of flattening operations include:
- Recursively flattening nested arrays.
- Using built-in functions like flat() in JavaScript or numpy. Flatten () in Python.
- Manually traversing multidimensional arrays to extract individual elements into a flat array.
56. Explain the concept of array partitioning and provide examples of partitioning algorithms.
Ans:
Array partitioning involves dividing an array into two or more subarrays based on a specific criterion, such as a pivot value or a predicate function. Examples of partitioning algorithms include quicksort’s partitioning step, which partitions an array into two halves based on a pivot value, and Dutch national flag partitioning, which partitions a variety into three segments based on a pivot value’s relative order.
57. What are circular arrays, and how are they implemented using modulo arithmetic?
Ans:
Circular arrays, also known as ring buffers or cyclic buffers, are arrays where the end wraps around to the beginning, forming a circular structure. They are typically implemented using modulo arithmetic to handle index wrap-around when incrementing or decrementing indices. This ensures that array accesses remain within bounds and allows for efficient cycling or rotation of elements.
58. Discuss the concept of array interleaving and provide examples of interleaving operations.
Ans:
Array interleaving involves combining multiple arrays into a single array by alternating elements from each input array. Examples of interleaving operations include:
- Merging two sorted arrays into a single sorted array by interleaving their elements.
- Combining multiple audio streams into a single interleaved audio stream.
- Zipping or interlacing arrays of data for parallel processing or communication.
59. What are the differences between static and dynamic multidimensional arrays?
Ans:
- Static multidimensional arrays have fixed dimensions and sizes determined at compile time, while dynamic multidimensional arrays can resize dynamically during runtime.
- Static multidimensional arrays are typically allocated on the stack and have fixed sizes, making them less flexible but more efficient regarding memory and access time.
- On the other hand, dynamic multidimensional arrays are typically allocated on the heap and can resize themselves as needed, offering flexibility but requiring additional memory management overhead.
60. Explain the concept of array buffering and its role in input/output operations.
Ans:
Array buffering temporarily stores data in an array before performing input/output operations, such as reading from or writing to files, network streams, or other external sources. Array buffering helps improve performance by reducing the number of system calls and minimizing data transfer overhead, especially when dealing with large datasets or streaming data sources.
61. Discuss the concept of array intersection and provide examples of intersection algorithms.
Ans:
Array intersection involves finding the common elements shared by two or more arrays. Examples of intersection algorithms include iterating over one array and checking for the presence of each component of the other arrays using techniques like linear search or hash sets for efficient lookups.
62. What are sparse arrays, and how are they implemented efficiently in languages like Python and JavaScript?
Ans:
Sparse arrays are arrays where most elements are empty or contain a default value. They can be efficiently implemented using sparse data structures such as dictionaries or maps in Python and JavaScript, where only non-empty or non-default elements are stored along with their indices, reducing memory usage compared to dense arrays.
63 . Explain the concept of array deduplication and provide examples of deduplication algorithms.
Ans:
- Array deduplication involves removing duplicate elements from an array to create a new array with unique elements.
- Examples of deduplication algorithms include sorting the array and then iterating over it to remove adjacent duplicates, using a hash set to track unique elements while iterating over the array, and using built-in functions or libraries that support deduplication operations.
64. What are associative arrays, and how are they implemented in languages like PHP and Perl?
Ans:
Associative arrays, also known as maps, dictionaries, or hash tables, are arrays where each element is associated with a unique key instead of an index. In languages like PHP and Perl, associative arrays can be implemented using built-in data structures such as dictionaries or hash maps. These provide efficient lookup, insertion, and deletion operations based on keys.
65. Discuss the concept of array merging and provide examples of merging algorithms.
Ans:
Array merging involves combining the elements of two or more arrays to create a single array. Examples of merging algorithms include iterating over the input arrays and copying aspects into a new array, using built-in functions like concat() in JavaScript or numpy. Concatenate () in Python, and merge sorted arrays into a single sorted array using techniques like merge sort or two-pointer merge.
66. What are dynamic arrays, and how are they implemented efficiently in languages like Python and Java?
Ans:
Dynamic arrays, also known as resizable arrays or ArrayLists, are arrays that can dynamically resize themselves during runtime to accommodate a varying number of elements. In Python, dynamic arrays are implemented using built-in data structures like lists, which automatically resize themselves as needed. In Java, dynamic arrays are implemented using the ArrayList class, which dynamically resizes its underlying array when it reaches capacity.
67. Explain the concept of array masking and provide examples of masking operations.
Ans:
Array masking involves selecting a subset of elements from an array based on a boolean mask or condition. Examples of masking operations include:
- A boolean mask filters out elements that meet a specific condition.
- Applying logical operations to create complex masks.
- Using masked arrays in numerical computing libraries to handle missing or invalid data.
68. Discuss the concept of array broadcasting and provide examples of broadcasting operations.
Ans:
Array broadcasting involves performing element-wise operations on arrays with different shapes by implicitly extending smaller arrays to match the shape of larger arrays. Examples of broadcasting operations include adding a scalar value to an array, multiplying two arrays with compatible shapes, and performing operations between arrays of different dimensions by automatically aligning their shapes.
69. What are the differences between arrays and sets regarding data structure and operations?
Ans:
Arrays are ordered collections of elements stored in contiguous memory locations, allowing duplicate elements and providing indexed access. Sets, conversely, are unordered collections of unique elements typically implemented using hash tables or trees and do not allow duplicates. Arrays support operations like random access, iteration, and slicing, while sets support operations like union, intersection, difference, and membership testing.
70. Explain the concept of array padding and provide examples of padding operations.
Ans:
Array padding involves adding additional elements to the edges or boundaries of an array to increase its size or reshape it to a desired shape. Examples of padding operations include:
- Adding zeroes or a default value to the edges of an array to make it square or rectangular.
- Adding boundary conditions to handle edge cases in image processing or convolutional operations.
- Padding sequences for input to recurrent neural networks or convolutional neural networks.
71. Discuss the concept of array transposition and provide examples of transposition operations.
Ans:
Array transposition involves converting rows into columns and vice versa, effectively rotating a two-dimensional array by 90 degrees. Examples of transposition operations include converting a matrix from row-major order to column-major order, or vice versa, and performing matrix operations such as matrix multiplication and inversion.
72. What is the role of arrays in dynamic programming, and how are they utilized in solving problems efficiently?
Ans:
Arrays play a crucial role in dynamic programming by efficiently storing and retrieving intermediate results of subproblems. Dynamic programming algorithms often use arrays as memoization tables to cache solutions to overlapping subproblems, reducing redundant computations and improving runtime complexity.
73. Discuss the concept of array manipulation using pointers in C/C++ and provide examples of pointer-based array operations.
Ans:
In C/C++, arrays and pointers are closely related, allowing for efficient manipulation of array elements using pointer arithmetic. Examples of pointer-based array operations include accessing array elements using pointer notation (*(arr + i)), iterating over array elements using pointer arithmetic (ptr++), and dynamically allocating arrays using pointer-based techniques like malloc() or new.
74. What are the differences between arrays and vectors in C++ STL, and when would you prefer one?
Ans:
- Arrays in C++ are fixed-size collections of elements stored in contiguous memory locations, while vectors are dynamic arrays that can resize themselves automatically.
- Vectors offer additional functionality such as bounds-checking, dynamic resizing, and methods for easy manipulation, making them preferred over arrays when flexibility and convenience are required.
75. Discuss the concept of array manipulation using numpy in Python, and provide examples of everyday numpy array operations.
Ans:
Numpy is a powerful library for numerical computing in Python, offering efficient array manipulation and operations. Examples of everyday numpy array operations include array creation (np. array()), element-wise arithmetic operations (+, -, *, /), slicing and indexing (arr[start: end]), reshaping (arr. reshape()), and aggregation functions (np. sum(), np. mean(), np. max()).
76. What are sparse matrices, and how are they represented using arrays? Discuss the advantages and disadvantages of sparse matrix representations.
Ans:
- Sparse matrices are matrices where the majority of elements are zero. They are typically represented using arrays to store only the non-zero elements and their row and column indices, reducing memory usage and improving efficiency for sparse matrix operations.
- The advantages of sparse matrix representations include reduced memory overhead and faster computations for sparse matrices.
- In contrast, the disadvantages include increased complexity for implementing operations and limited support for dense matrix operations.
77. Explain the concept of array buffering and its role in input/output operations. Provide examples of buffering mechanisms in programming languages.
Ans:
Array buffering temporarily stores data in an array before performing input/output operations, such as reading from or writing to files, network streams, or other external sources. Buffering helps improve performance by reducing the number of system calls and minimizing data transfer overhead. Examples of buffering mechanisms in programming languages include stream buffering in C/C++, buffered I/O classes in Java, and buffering options in file I/O functions in Python.
78. Discuss the concept of array compression and provide examples of compression techniques used in numerical arrays.
Ans:
Array compression involves reducing the size of an array by encoding it in a more compact form. Examples of compression techniques used in numerical arrays include run-length encoding (RLE), where consecutive elements with the same value are replaced with a count and a single value, and differential encoding, where the differences between consecutive elements are stored instead of the absolute values.
79. What are the differences between arrays and lists in Python, and when would you prefer one over the other?
Ans:
- Arrays and lists in Python are both mutable sequences. Still, arrays are more efficient for storing homogeneous numerical data and support element-wise arithmetic operations.
- In contrast, lists are more flexible and support heterogeneous data types and a more comprehensive range of operations, such as appending, inserting, and removing elements.
- Arrays are preferred over lists when working with large numerical datasets for performance reasons.
80. Discuss the concept of array slicing and provide examples of slicing operations in various programming languages.
Ans:
Array slicing involves extracting a contiguous subset of elements from an array to create a new variety. Examples of slicing operations in various programming languages include Python’s slice notation (arr[start:end: step]), MATLAB’s colon operator (arr(start: end)), and R’s subsetting syntax (arr[start: end]). Slicing allows for efficient manipulation and extraction of array subsets without copying the entire array.
81. Explain the concept of array rotation and provide examples of rotation algorithms in place.
Ans:
Array rotation involves shifting the elements of an array cyclically by a certain number of positions. An example of an in-place rotation algorithm is the reversal algorithm: first, reverse the entire array, then reverse the first k elements and the remaining elements separately.
82. Discuss the concept of array blocking and its applications in parallel computing.
Ans:
Array blocking involves partitioning an array into smaller blocks or chunks to improve cache locality and parallelism in algorithms. In parallel computing, array blocking can facilitate parallel processing of array segments across multiple processors or threads, reducing synchronization overhead and improving performance.
83. What are ragged arrays, and how are they represented in programming languages like Python and JavaScript?
Ans:
Ragged arrays are arrays where each row can have a different length. In Python, ragged arrays can be represented using nested lists, where each inner list represents a row with a variable number of elements. In JavaScript, ragged arrays can be represented similarly using arrays of arrays.
84. Discuss the concept of array manipulation using slice operations in Python, and provide examples of everyday slice operations.
Ans:
Slice operations in Python allow for extracting contiguous subsets of elements from an array. Examples of everyday slice operations include selecting a range of elements (arr[start: end]), specifying a step size (arr[start:end: step]), and reversing an array (arr[::-1]). Slicing provides a convenient and efficient way to manipulate arrays without modifying the original data.
85. What are parallel arrays, and how are they used in programming? Provide examples of parallel array applications.
Ans:
Parallel arrays store related data in separate arrays with corresponding indices. They are commonly used in programming to represent tabular data, where each array represents a column of data, and the indices correspond to rows. Examples of parallel array applications include databases, spreadsheet software, and numerical simulations.
86. Explain the concept of array masking and provide examples of masking operations in MATLAB.
Ans:
Array masking in MATLAB involves applying a logical mask to select or filter elements based on a condition. For example, you can use the expression A(A > threshold) to select elements greater than a threshold in an array A. Masking operations allow for efficient selection and manipulation of array elements based on specific criteria.
87. Discuss the concept of array broadcasting and provide examples of broadcasting operations in NumPy.
Ans:
- Array broadcasting in NumPy allows for performing element-wise operations on arrays with different shapes by automatically aligning their dimensions.
- For example, you can add a scalar to a 1D array, a 1D array to a 2D array, or multiply two arrays with different shapes. NumPy will automatically broadcast the smaller variety to match the shape of the enormous array.
88. What are disjoint-set data structures, and how are they implemented using arrays? Discuss the union-find algorithm.
Ans:
Disjoint-set data structures, or union-find data structures, are used to maintain a collection of disjoint sets with efficient operations for merging sets and determining set membership. They are commonly implemented using arrays to represent sets as trees, where each element points to its parent in the tree. The union-find algorithm efficiently merges sets and determines set membership by performing path compression and union by rank.
89. Discuss the concept of array serialization and provide examples of serialization formats commonly used in data interchange.
Ans:
Array serialization involves converting an array into a format that can be stored or transmitted as a sequence of bytes. Examples of serialization formats commonly used in data interchange include JSON (JavaScript Object Notation), CSV (Comma-Separated Values), XML (eXtensible Markup Language), and Protocol Buffers. Serialization allows for efficient storage, transmission, and interoperability of array data between different systems and platforms.
90. What are the differences between arrays and matrices in MATLAB, and when would you use one over the other?
Ans:
- In MATLAB, arrays are general-purpose data structures that can store multidimensional data of any size and type, while matrices are used explicitly to represent mathematical matrices with two dimensions.
- Arrays are more flexible and can have any number of dimensions, while matrices are optimized for linear algebra operations and support matrix-specific functions and operations.
- You would use arrays for general-purpose data storage and manipulation and matrices for mathematical computations and linear algebra operations.
91. Explain the concept of array flattening and provide examples of flattening operations in programming languages like Python and JavaScript.
Ans:
Array flattening involves converting a multidimensional array into a one-dimensional array. In Python, you can flatten an array using methods like list comprehension or NumPy’s flatten() function. In JavaScript, you can flatten an array using techniques like recursion or the flat() method.
92. Discuss the concept of array interpolation and provide examples of interpolation techniques used in image processing.
Ans:
Array interpolation involves estimating the value of elements in between existing data points. In image processing, techniques like nearest-neighbour interpolation, bilinear interpolation, and bicubic interpolation are used for upsampling or downsampling images, resizing images, and generating smooth transitions between pixels.
93. What are bit arrays, and how are they implemented using arrays of boolean values? Discuss the advantages and disadvantages of bit arrays.
Ans:
- Bit arrays, also known as bitmaps or bitsets, are arrays where each element represents a single bit of information, typically stored as boolean values.
- They are used to represent sets or collections of elements efficiently, with each bit indicating the presence or absence of a component.
- The advantages of bit arrays include compact representation and efficient bitwise operations, while the disadvantages include limited precision and increased complexity for operations involving non-boolean data types.
94. Explain the concept of array mapping and provide examples of mapping operations in numerical computing.
Ans:
Array mapping involves applying a function to each element of an array to transform it into another value. In numerical computing, mapping operations are commonly used to apply mathematical functions like logarithms, exponentials, trigonometric functions, and custom transformations to arrays of numerical data, such as vectors or matrices.
95. Discuss the concept of array shaping and reshaping and provide examples of reshaping operations in programming languages like Python and MATLAB.
Ans:
Array shaping and reshaping involve changing an array’s dimensions or size while preserving its elements’ order. In Python, you can reshape arrays using NumPy’s reshape() function. In MATLAB, you can reshape arrays using the reshape() function or by directly specifying the desired dimensions in square brackets.
96. What are circular queues, and how are they implemented using arrays? Discuss the advantages and disadvantages of circular queues.
Ans:
- Circular queues, also known as ring buffers or cyclic buffers, are queues where the front and rear pointers wrap around to the beginning of the array when they reach the end, forming a circular structure.
- They use arrays with additional logic to handle wrap-around and prevent overflow or underflow conditions. The advantages of circular queues include efficient use of memory and constant-time enqueue and dequeue operations.
- In contrast, the disadvantages include limited capacity and potential waste of memory due to unused slots in the array.
97. Explain the concept of array permutation and provide examples of permutation algorithms used in combinatorial problems.
Ans:
Array permutation involves rearranging the elements of an array into different orders. Permutation algorithms are commonly used in combinatorial problems like generating permutations of a set of elements, solving puzzles like the Rubik’s Cube, and enumerating all possible outcomes in permutation-based algorithms like backtracking or branch and bound.
98. What are the differences between arrays and tensors in deep learning frameworks like TensorFlow and PyTorch?
Ans:
Arrays in deep learning frameworks like TensorFlow and PyTorch are multidimensional data structures used to represent tensors, the fundamental building blocks of neural networks. Tensors generalize arrays to higher dimensions and provide additional operations optimized for deep learning, such as automatic differentiation, GPU acceleration, and support for neural network layers and operations.
99. Discuss the concept of array concatenation and provide examples of concatenation operations in programming languages like Python and MATLAB.
Ans:
Array concatenation involves combining the elements of two or more arrays to create a single array. In Python, you can concatenate arrays using NumPy’s concatenate() function or array concatenation operators like np. hstack() and np. vstack(). In MATLAB, you can concatenate arrays using functions like cat() or by directly concatenating arrays using square brackets.
100. Explain the concept of array masking and provide examples of masking operations in programming languages like R and MATLAB. –
Ans:
Array masking involves selecting a subset of elements from an array based on a boolean mask or condition. You can apply masking operations in R using logical indexing or functions like subset() or filter(). You can apply masking operations in MATLAB using logical indexing or functions like find() or logical(). Masking operations allow for efficient selection and manipulation of array elements based on specific criteria.