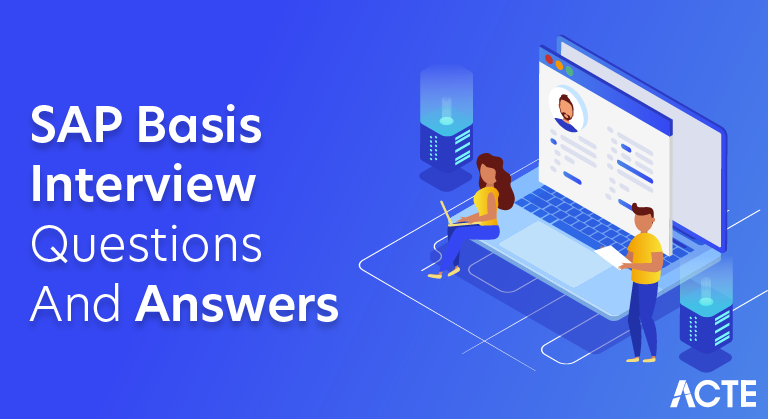
A popular general-purpose programming language for developing systems and applications is C. It is strong and versatile. C is a cornerstone programming language for many other languages and is widely used in operating systems, embedded systems, and high-performance applications. It is well-known for its effectiveness and control over system resources. Programmers must study it because of the influence its syntax and structure have had on many other contemporary languages.
1. Explain what C preprocessor directives are.
Ans:
In C, preprocessor directives are predefined functions or macros that are executed automatically. Writing and running a C program involves several steps. The primary categories of preprocessor directives include Macros, File Inclusion, Conditional Compilation, and other directives such as ‘#undef’, ‘#pragma’, etc. These directives help manage code organization, facilitate code reuse, and allow for conditional compilation based on specific conditions.
2. What makes C a programming language categorised as mid-level?
Ans:
C is regarded as a middle-level language since it can support both low-level and high-level features. It is a low-level, assembly-level language as well as a higher-level language. C programs are translated into assembly code, where they offer machine-independent high-level programming and low-level pointer arithmetic. As a result, C is frequently called an intermediate language. Operating systems and menu-driven consumer billing systems can be written in C.
3. Which fundamental data types can be used in the C programming language?
Ans:
- In C, every variable has a corresponding data type. Different memory requirements and particular operations can be carried out on different types of data.
- It describes the kinds of data integer, character, floating-point, double, etc. that the variable is capable of storing. Data types in C can be broadly divided into four categories.
- Void Types: Primitive data types include void data types. Void data types have no associated value and give their caller no outcome.
- The user User-defined data types: To improve program readability, users specify these data types.
4. What are tokens in C?
Ans:
- Keywords: In the computer language C, terms that are reserved or predefined. In a program, each keyword is designed to carry out a specific function.
- Identifying names: Identifiers are names that are defined by the user and can be any length of numbers or letters starting with a letter or the underscore (_). Names for identifiers cannot equal any reserved C programming language’s keywords.
- Constants: Once defined, constants are regular variables that cannot be changed within the program. A constant is a value that is fixed. Another name for them is literals.
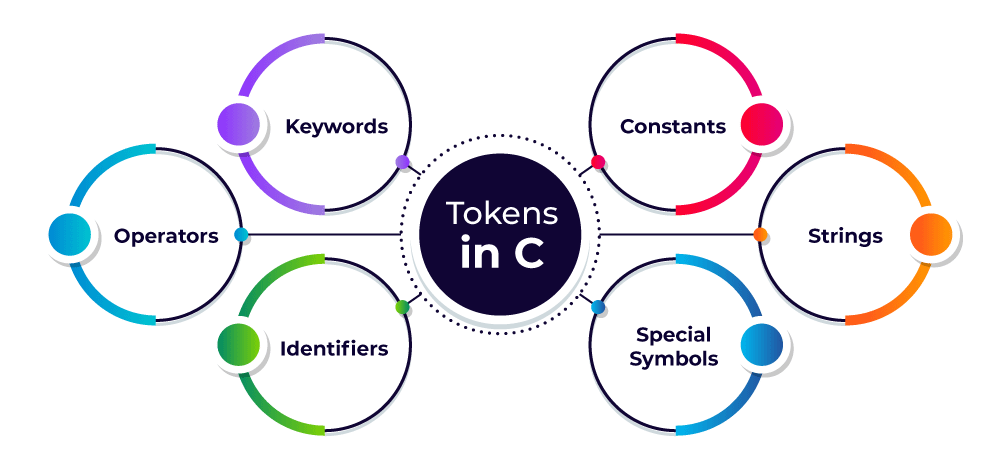
5. What is meant by a variable’s scope?
Ans:
A specified variable’s scope in a programming language is the block or region in which it will exist; if the variable extends beyond that, it will be immediately destroyed. Each variable has a specific range. A variable’s life in the program is its scope, to put it simply. Three locations can be used to declare the variable. These are the following:
- Local Variables: Within a designated block or function
- Global Variables: Among all the functions that are available globally within the program.
6. Who created the C programming language?
Ans:
American computer scientist Dennis MacAlistair Ritchie was born September 9, 1941, and died October 12, 2011. Along with his longtime partner Ken Thompson, he developed the B programming language and the Unix operating system. They also created the C programming language. High-level, general-purpose programming languages like C are available. It offers a simple, reliable, and robust interface for system programming.
7. How do static variables in C become used?
Ans:
The const keyword indicates that once a variable is declared, its value cannot be changed. It is employed to define constants, improve the readability of code, and guard against unintentional value alteration. When a variable is declared as having a constant value, the const keyword instructs the compiler to prevent the programmer from changing it. This feature enhances code safety by reducing the risk of accidental modifications, especially in complex programs.
8. Describe the {enum} keyword’s function.
Ans:
- An enumerated type is a user-defined type made up of a collection of named integer constants.
- It is defined with the `enum` keyword. Enums make code easier to read, and related constants are more straightforward to handle.
- An enumerated (unchangeable) type is declared with the enum keyword. An enum is a unique “class” that stands for a collection of constants or immutable variables, such as final variables.
- Use the enum keyword (rather than class or interface) and comma to separate the constants to build an enum.
9. What are the functions of pointers?
Ans:
- Pointers are used to store a memory location or the address of a variable. Another usage for pointers is as pointer references.
- The pointer’s primary function is to lengthen execution times and conserve memory.
- Pointers can be used for the following purposes: Passing arguments by reference, Accessing array elements, Returning multiple values, Dynamic memory allocation, To put data structures into practice
10. Explain the differences between getche() and getch().
Ans:
Feature | getche() | getch() |
---|---|---|
Functionality | Reads a character and echoes it to console. | Reads a character without echoing to console. |
Echoing | Echoes the character entered. | Does not echo the character entered. |
Header File | Typically found in |
Typically found in |
Usage | Useful for immediate user input feedback. | Useful when user input should not be shown. |
11. Describe loops and explain how to make an infinite loop in C.
Ans:
To continually run a set of statements, utilise loops. Within the loop, the statement that needs to be repeated will be run n times until the predetermined condition is met. Two varieties of loops exist. The C programming language features loops that are entry- and exit-controlled. A Limitless A loop is a section of code that has no working exit. It, therefore, continues endlessly. When a program contains an infinite loop, there are only two possible outcomes.
12. What are the purposes of header files?
Ans:
The many libraries available in the C language, which provide predefined routines, make programming more accessible. Predefined standard library functions are contained in header files, which need to end with “.h.” Function definitions, data type definitions, and macros are also found in header files, which can be imported using the preprocessor directive “#include.” Preprocessor directives tell the compiler that processing these files beforehand is required before compilation.
13. What kinds of functions are there?
Ans:
- A function is a section of code used to carry out a particular purpose rather than repeating it in our software by writing it out several times.
- Functions improve the program’s readability by preventing code duplication.
- They also make it easy to modify programs, which lowers the likelihood of errors.
14. What is typecasting in C?
Ans:
The process of changing a variable’s data type is known as typecasting. It can be done implicitly by the compiler when necessary or directly using cast operators like `(int)` and `(float)}. In C, type casting is essentially the process of changing a variable from one data type to another. Depending on what the program to perform, the compiler will automatically switch from one data type to another during type casting.
15. What is the function of the `sizeof} operator?
Ans:
- The `sizeof` operator yields a variable’s or data type’s size in bytes.
- It is used to calculate the memory allocation needs of variables, arrays, and structures and is evaluated at build time.
- The amount of memory that an object of a type can utilise is determined by using the size of the operator to that type name.
- This includes any trailing or internal padding.
16. How do functions and macros differ from one another?
Ans:
- A preprocessor command that designates a group of C statements is called a macro.
- The preprocessor directive defines a macro. Since macros are preprocessed, our program would only have been compiled after all of the macros had been preprocessed.
- Functions are compiled rather than preprocessed, though.
17. What role does the keyword “const” play?
Ans:
The const keyword indicates that once a variable is declared, its value cannot be changed. It is employed to define constants, improve the readability of code, and guard against unintentional value alteration. When a variable is declared as having a constant value, the const keyword instructs the compiler to prevent the programmer from changing it. This practice enhances code safety by ensuring that critical values remain unchanged throughout the program’s execution.
18. What terms are marked with an asterisk?
Ans:
In a program, each keyword is designed to carry out a specific function. They cannot be used for reasons other than those for which they were intended because their meaning has already been established. The programming language C has 32 keywords supported. Reserved keywords include things like ‘auto’, ‘else’, ‘if’, ‘long’, ‘int’, ‘switch’, ‘typedef’, and so on. Understanding these keywords is essential for effective programming, as they form the building blocks of the language’s syntax.
19. What does a header file do in C?
Ans:
In C, function and macro declarations are contained in header files and can be shared by several source files. This arrangement makes code reuse and modularity easier while guaranteeing consistency in function prototypes and macro definitions across the application. Code is arranged using C, which also offers shared declarations and function prototypes, encourages modularity, and makes code reuse easier.
20. What is a union?
Ans:
- Users can store many types of data in a single user-defined data type called a union Unit.
- But a union is not the totality of its members’ memories. Only the memory of the most prominent member is stored there.
- Since the union only allows one common space for each member, access is permitted for only one variable at a time.
- When wishing to use the same memory for two or more members, the union can be helpful in a variety of scenarios.
21. What do a value and r-value mean?
Ans:
An item is called a when it has a unique address or location in memory. “R-value.” A “value” will be displayed on either the left or right side of the assignment operator (=). A value of data stored in memory at a particular address is called a “r-value.” An object without a recognizable “r-value” is placed (that is, without an address) in memory. An expression that is not assignable is called a “r-value,” and it can only occur to the right of an assignment operator. (=).
22. What does the method sleep() do?
Ans:
The C ‘sleep()’ method lets users wait for a thread to finish for a predetermined amount of time. The thread will put the current executable to sleep using the ‘sleep()’ function for the specified duration, while other CPU operations continue as usual. If the required duration has passed, the ‘sleep()’ method returns 0. This function is useful for managing timing in programs, allowing for pauses that can help synchronize operations or delay actions as needed.
23. What do lists consist of?
Ans:
- Enumerations, often known as enums, are user-defined data types in C.
- Thanks to enumerations, Integral constants can be identified, which facilitates program reading and maintenance.
- For instance, the times of the Week can be used anywhere in the program and are defined as an enumeration.
24. What does the term volatile mean?
Ans:
- Because the values of volatile keywords can never be altered by code that is not part of the current code, they are used to stop the compiler from optimising.
- Even when an earlier command asks the same volatile object for its value, the system always obtains the object’s current value from memory rather than storing it in a temporary register at the time of request.
25. What is the allocation of static memory?
Ans:
Static memory allocation occurs during compilation. It reduces operating time and is faster compared to dynamic memory allocation, as it originates from the stack. This form of memory allocation is less flexible than dynamic memory allocation, as the size of the allocated memory must be determined at compile time. However, it is primarily favored within arrays and situations where memory requirements are known in advance.
26. What is the allocation of dynamic memory?
Ans:
Dynamic memory allocation occurs during execution or runtime. It is slower than static memory allocation since it allocates memory using the heap, but it is more flexible and efficient for managing varying memory requirements. The majority of the connected list members support dynamic allocation, allowing for the creation and destruction of nodes as needed. This flexibility makes it particularly useful in applications where the size of data structures can change over time, such as in linked lists and dynamic arrays.
27. What does a function’s pass-by-reference mean?
Ans:
- A function that employs pass-by-reference can change variables without requiring a copy.
- The passed variable and parameter are located in the same memory address, so any modifications made to the parameter will also be reflected in the variables.
28. What are memory leaks, and how can one prevent them?
Ans:
Memory is produced in the heap each time a variable is defined if the programmer does not delete it. A memory leak is the term for this undeleted memory on the heap. When less memory is available, the software performs less well, potentially leading to crashes or unresponsive behavior. It is crucial to monitor memory usage and implement proper cleanup routines to ensure that memory is released when it is no longer needed.
29. What do arguments in the command line mean?
Ans:
- Command-line arguments are supplied from the operating system’s command-line shell to the program’s main() method.
- When a program is executed, command-line arguments are an effective way to give it parameters.
- They let users alter the program’s behaviour without changing the source code.
30. What is an automatic keyphrase?
Ans:
When using the C programming language, every function’s local variable is called an automatic variable—programming language. The default storage class for all variables specified inside of a block or function is Auto. Only the block or function in which they have been defined can access auto variables. Pointers allow us to use them for purposes other than what they were designed for. By default, auto keywords have a null value.
31. Describe the use of modifiers.
Ans:
Modifiers are keywords in the C language that change the meaning of basic data types. They specify how much RAM needs to be allocated to the variable. The data type modifiers available in the C programming language are five:
- long long
- long signed
- unsigned
- signed
- signed short long
32. How does an external storage specifier get used?
Ans:
The extern keyword in the C programming language makes C variables and functions more visible. Extern is a shorthand for external. It is utilized when this file must access a specific A variable from any other file. Redundancy is increased since variables with the extern keyword are just declared rather than defined. External functions do not need to be defined or declared because they are visible by default throughout the program.
33. What are the purposes of the C programming language’s printf() and scanf() functions?
Ans:
- Moreover, describe format specifiers.
- The value that is supplied to the printf() function as an argument is printed on the console screen.
- Print(“%X”, variable_of_X_type); is the syntax.The scanf() method reads values from the console according to the provided data type.
34. Can a format specifier be briefly explained?
Ans:
A format specifier is a sequence in programming languages like C and Java used within strings to format data. It begins with a percent sign (%) followed by a character that indicates the type of data being formatted, such as %d for integers, %f for floating-point numbers, and %s for strings. Format specifiers allow for precise control over the output appearance, including field width and decimal precision. They are commonly used in functions like printf and sprintf.
35. What are extensive, far, and near pointers in C?
Ans:
- Near Pointers: Only 16-bit addresses are stored in near pointers. Unable to save an address that is more significant than the one used by the close pointer. Sixteen bits.
- Far Pointers: A 32-bit pointer is referred to as a far pointer. However, data from the current segment that is not in the computer’s memory can also be accessed.
- Huge Pointers: A 32-bit pointer is generally regarded as a huge pointer. However, bits kept or situated outside of the segments can also be accessed.
36. How are getc(), getchar(), getch(), and getche() different from one another?
Ans:
- get (): If the function is successful, it reads one character from an input stream and returns an integer value, which is usually the character’s ASCII value. If it fails, the EOF is returned.
- getchar(): This function is the same as getc(stdin), but it can read from standard input instead of getc().
- getch(): It is a nonstandard function found in the header file “conio. h,” which is mainly utilised by Turbo C and other MS-DOS compilers.
37. What are the distinguishing characteristics of the C programming language?
Ans:
This programming language offers numerous benefits, including machine independence, allowing it to run on various hardware platforms without modification. It boasts function-rich libraries that provide a wide array of pre-built functions for efficient development. Its memory management capabilities ensure optimal use of system resources, preventing memory leaks and enhancing performance. The language supports structured programming, promoting clear and maintainable code through the use of functions and control structures.
38. What are some distinct C storage class specifiers?
Ans:
Any variable can be stored using classes. There are four different kinds of storage in C:
- Auto
- Register
- External
- Static
39. Which data types does the C programming language support?
Ans:
One of the frequently asked interview questions for C programming is this one. The data type specifies the type of data. Utilised when writing computer programs. Several predefined data types with various storage sizes are available in the C language:
- Inbuilt data types include char, double, float, void, and int.
- Derived data types: These comprise references, arrays, and pointers.
- User-defined data types: Enumeration, Union, and Structure
40. What is meant by a variable’s lifespan and scope in C?
Ans:
A variable’s scope and lifetime are defined in the part of the code that makes it executable or visible. Which software elements can access the variable is determined by its scope. Within a given block or context, access is limited to the variables specified within that block. Depending on where and how the variable is defined (e.g., local variables within functions, global variables, etc.), the lifetime of a variable determines how long it will remain in memory before being deleted.
41. What is meant by a pointer in C?
Ans:
In C, pointers are variables that hold the address of another variable, allowing for indirect access to that variable’s value. They are mutable, meaning their stored address can be changed during program execution. At runtime, memory is allocated for the variable to which the pointer points. Pointers can be of various data types, such as char, float, double, int, etc., corresponding to the type of data they are intended to reference.
42. What is a null pointer?
Ans:
- The empty region in the computer’s memory is represented by a null pointer, which serves several functions in C.
- The pointer variable will be assigned to the variable without any allocated memory.
- If there is no intention to send any valid memory location to the variable, a null pointer can be passed to handle errors in pointer-related code by checking for a null pointer before assigning any pointer variables.
43. How is a function used in C?
Ans:
In C, a function is used to encapsulate a block of code that performs a specific task. Functions are defined with a return type, a name, and parameters (if any). To use a function, it must first be declared and defined, and then called from within the code using its name and passing any required arguments. This promotes code reuse and modularity. Functions can return values or perform actions without returning anything (void).
44. What are header files in C?
Ans:
Header files contain the C function declarations and macro definitions that must be shared across sourced files. The. h suffix generates header files.
Two categories of header files exist:
- A header file created by the programmer
- Files included with compiler
45. Explain stack.
Ans:
It’s a type of data structure that stores information according to a specific order of operations. Two categories exist. LIFO (last in, first out) and FIFO (first in, last out) are two methods of order storage.
Fundamental actions carried out in the stack:
- Push
- Pop
- Peek or Top
46. What are huge pointers?
Ans:
Huge pointers are a type of pointer used in C, mainly within older systems that employ segmented memory architectures, like DOS. They enable access to a larger address space than standard pointers by combining a segment address with an offset. This allows programs to reference more memory than regular pointers can manage. However, huge pointers are largely outdated today, as modern operating systems and architectures utilize flat memory models.
47. How are arbitrary numbers produced?
Ans:
The rand () command can be used to create random numbers.
For instance:
- #include “time.h”
- #include “stdlib.h”
- Time (NULL) = Srand; Int r = rand();
48. Describe what a union is.
Ans:
Different kinds of data can be stored in a single unit or database using a user-defined data type called a union in the same region of memory. A union can have more than one member, but only one member can have a value at any one moment. Furthermore, it only stores the memory of the most prominent member and does not store the whole memory of all members.
49. What occurs when a header file is referenced more than once?
Ans:
Including a header file twice in C can cause an error because the compiler will process its contents twice, leading to multiple definitions and conflicts. To prevent this, header guards can be used. A header guard is a preprocessor directive that ensures the header file is included only once during compilation. It typically involves #ifndef, #define, and #endif directives. When the header file is included the first time, the guard allows the contents to be processed.
50. What is meant by a memory leak in C?
Ans:
- A leak of memory is a specific type of resource leak that results from programmers creating heap memory and then forgetting to remove it.
- As a result, the memory that is no longer required is left intact.
- It can also happen when a memory-stored object is rendered unusable by executing code.
- A memory leak may cause the software to use more memory than necessary and may have an impact on its performance.
51. What do the terms `while(0)` and `while(1)` signify?
Ans:
Meanwhile, ‘1’ and ‘0’ denote true (or ON) and false (or OFF), respectively. ‘while(0)’ indicates that the code inside the while loop will not be executed, as the looping condition is always false. Conversely, ‘while(1)’ creates an endless loop that continues until it encounters a clearly stated ‘break’ statement. This type of loop is often used in scenarios where continuous execution is required until a specific condition is met. Care should be taken when using infinite loops, as improper handling can lead to unresponsive programs or excessive resource consumption.
52. Describe the distinction between increments that come before and after a prefix.
Ans:
- Prefix increment and postfix increment both increase the variable by one, as indicated by their syntax.
- The prefix increment increases a variable’s value prior to program execution and returns the variable’s value following incrementation.
- Conversely, the postfix increment returns the value of a variable before it has been increased and increases the value of the variable after the program has been executed.
- ++a <- Increase in prefix
- a++ <- Increase in postfix
53. What distinguishes a void pointer from a null pointer?
Ans:
When a pointer has no acceptable destination pointed to, and its value is unknown at the time of declaration, it is often initialized to NULL. Conversely, void pointers are generic pointers that are not associated with any specific data type. Their syntax is as follows: *ptr = NULL. Any variable’s address may be stored in them, allowing for flexible memory management. Void pointers can point to all data types, enabling the creation of functions that can operate on different data types without needing to know their specific types at compile time.
54. What distinguishes a call by reference from a call by value?
Ans:
A function that receives a call by value gets the variable’s value as a parameter, meaning any changes made to the parameter within the function do not affect the original variable. In contrast, a call by reference transmits the variable’s address rather than its value. In this case, the function’s internal processes may have an impact on the original variable’s value, allowing for modifications to persist after the function execution.
55. How can one resolve the scope of a global symbol in C?
By extending the visibility or scope of variables and functions, external storage can be used to manage the scope of a global symbol effectively. This allows global variables and functions to be accessed across different files within the same program. Additionally, you can utilize C functions without explicitly declaring or defining them in every file, as they are available by default throughout the entire program.
56. Describe what modular programming is.
Ans:
- Using the modular programming approach, a program can be divided into separate, interchangeable modules or sub-programs, such as functions and modules, to attain specific functionality.
- The functionality is divided so that every sub-program has all the components needed to carry out a single part of the intended functionality.
57. What is a sequential access file?
Ans:
- Sequential access files, as their name implies, are used to store files sequentially that is, data is inserted from one file after another.
- This means that accessing files requires verifying them one after the other (reading each piece of data one at a time) until the desired file is found.
- There are additional restrictions because of the lengthy access times and expensive storage.
58. Describe the C #pragma directive.
Ans:
A particular purpose directive called #pragma is used to turn specific functionality on and off. Among the commands for #pragma are:
- #pragma startup: This file specifies the functions that must be executed prior to the program starting.
- Exiting the #pragma: It defines the functions that must be executed immediately before the program terminates.
- #pragma warning: This is a tool for hiding the alerts that show up during compilation.
- #pragma GCC poison: This command eliminates an identifier from the software.
59. In C programming, what does variable initialization mean?
Ans:
In C programming, variable initialization refers to the process of assigning an initial value to a variable at the time of its declaration. This ensures that the variable starts with a defined value rather than an unpredictable one. For example, `int x = 10;` initializes `x` with 10. Initialization helps prevent undefined behavior caused by using uninitialized variables. It can be done for various data types, including integers, floats, arrays, and structures.
60. Define Preprocessor?
Ans:
For the purpose of acting as a directive to the compiler, a preprocessor directive is a built-in predefined function or macro that is performed before the primary C program. The C compiler automatically transforms software before it is compiled using the C preprocessor, which functions as a macro processor. It is known as a macro processor because it enables the definition of macros—shorthand for lengthier constructs.
61. What makes C the Mother of All Languages?
Ans:
C introduced several fundamental concepts and data structures, including strings, functions, lists, and arrays. These core elements provided a robust framework that has influenced the design and development of many subsequent programming languages. Due to its pivotal role in the evolution of programming, C is often regarded as the “mother of all languages.”
62. What is typedef in C?
Ans:
The typedef keyword in C programming defines an alias for an already-existing type. typedef can abbreviate the name of any variable, be it an integer, function parameter, or structure declaration. The syntax for using typedef is straightforward. In this case, the type that already exists is assigned a new name, referred to as the alias name. This feature enhances code readability and maintainability, making it easier to work with complex data types, such as structures or pointers.
63. What are the storage classes in C?
Ans:
- auto: Local variables’ default storage class.
- register: To enable quicker access, it is suggested that the variable be kept in a CPU register.
- static: If used at the global level, limits the variable’s scope to the file and maintains its value across function calls.
- extern: In another file, a global variable or function is declared.
- typedef: Gives an existing type a new name.
64. In what context does a function employ the keyword “void”?
Ans:
When declaring a function in programming, it is necessary to determine whether or not it will return a value. When “void” is positioned in the function header’s leftmost section, the function won’t return a value. Only a portion of the function’s outputs will be shown on the screen. Furthermore, in the second scenario, only the data type of the return is returned once the function has executed and the return value has been excluded. “Void” is replaced with value.
65. Define a dynamic data structure.
Ans:
There are two kinds of data structures: static and dynamic. The size of the dynamic data structure is flexible during operations and can change based on the needs of the static data structure. The purpose of this data structure is to enable run-time data structure changes. The data stored in DDS’s memory might expand or contract in size. If this data structure goes past its limit, it may “overflow.”
66. What is a segmentation fault in C?
Ans:
- When a program tries to access a memory address that it is not authorized to access or employs a method that is prohibited in the current system, it causes a segmentation fault in C.
- Writing to read-only memory, dereferencing null or uninitialized pointers, and accessing memory outside of an array’s boundaries are common causes of this.
- Segmentation faults are a typical error observed during development that often indicate defects that need to be fixed for stable software functioning. They result in anomalous program termination.
67. What is a macro?
Ans:
One command that automatically grows into a collection of instructions to carry out a certain operation is called a macro. With preprocessor directives in languages like C and C++, macros—which are frequently used in programming to automate repetitive tasks—can be defined. They do this by enclosing complicated code sequences, which helps to enhance code readability and maintainability. Prior to the code actually being compiled, macros are usually processed.
68. What is union in C?
Ans:
In C, a union is a data type that enables to list and create a set of variables that are only accessible by the object of this data type. With one exception, a union and a structure are similar. In a union, every element declared has a shared memory location, whereas in a structure, each element has its own memory location.
- 1 2 3 4 5 6 union amusement
- using int a; char b[10];
- funobj;
69. How can prime numbers be found in C?
Ans:
- Any natural number greater than one that isn’t the product of itself and two smaller natural numbers other than one is known as a prime number.
- For instance, five is a prime number (whose factors are one and five). The following C code determines whether the entered number is prime or not.
70. What is an identifier in C?
Ans:
Identifiers are any names that assigns to any data type, variable, label, or function that are user-specified. For example, in a union, every element is declared to have a shared memory location, whereas in a structure, each component has its memory location.
- 1 2 3 4 5 6 union amusement
- using int a; char b[10];
- funobj;
71. Describe Operator for stringing (#).
Ans:
The “stringizing” or number-sign operator (#) takes macro parameters and turns them into string literals without extending the parameter description. It works only with macros that accept parameters. The actual argument given by the macro invocation is surrounded in quote marks and handled as a string literal if it comes before a formal parameter in the macro definition. Then, in the macro definition, the string literal takes the place of every instance where the formal parameter and stringizing operator are combined.
72. Define Preconditioning the Compilation.
Ans:
- Preconditioning the compilation involves preparing the source code before the actual compilation process begins.
- This includes steps like preprocessing directives, macro expansions, and conditional compilation.
- It ensures that the code is in a suitable state for the compiler to process efficiently.
- By handling these preliminary tasks, preconditioning can help optimize the compilation process and reduce potential errors.
73. What are the differences between a C `for` loop and a `while` loop?
Ans:
In most cases, a ‘for’ loop is utilized when the number of iterations is predetermined. In a single line, it incorporates increment/decrement, condition checking, and initialization. When the number of iterations is unknown ahead of time, a ‘while’ loop is used, which checks the condition at the beginning of every iteration. The ‘for’ loop is particularly effective for iterating over arrays or known ranges, providing a clear and concise structure.
74. Is C or Python a better language?
Ans:
Python and C are both helpful languages for creating various apps. Python finds applications in machine learning, web development, natural language processing, and other applications, while C is mainly used for hardware development, such as network drivers and operating systems. One cannot become proficient in just one thing, but knowing both programming languages can help one stand firm in today’s competitive market.
75. What is a static variable in C?
Ans:
A static variable in C is a variable that retains its value between function calls and is initialized only once during the program’s lifetime. It has a local scope within the function it is defined in, but its lifetime extends for the duration of the program. This feature makes static variables useful for maintaining state information without exposing the variable outside its defining function. However, care should be taken to manage their use, as they can lead to unexpected behavior if not properly handled.
76. What are identifiers in C?
Ans:
- Names given to program elements like variables, functions, arrays, and structures.
- Must start with a letter or an underscore, followed by letters, digits, or underscores.
- Case-sensitive, distinguishing between identifiers like `Variable` and `variable`.
- Should be meaningful and descriptive to improve code readability.
- Examples include `int count;`, `float average_score;`, and `void calculateSum();`.
77. What is volatile in C?
Ans:
When a variable in C is subject to change due to external forces beyond the program’s control, such hardware or another thread, it is marked using the `volatile` keyword. As a result, the compiler is unable to optimize the code in a way that depends on the variable’s value holding steady between accesses. Consequently, each read and write operation to a `volatile} variable is executed straight from memory. This is important because variables in embedded systems, device drivers, and multi-threaded applications might change suddenly.
78. Why is function overloading not supported by C?
Ans:
Function overloading, which allows the definition of multiple functions with the same name but different parameters, is not supported in C. This limitation exists because C employs a simple naming system that does not keep track of argument types. When a function is called, the compiler resolves it solely by its name, leading to potential ambiguity if multiple functions share that name. Moreover, C prioritizes simplicity and performance, and introducing function overloading would complicate these aspects.
79. What does C stand for when it comes to argument?
Ans:
The definition of an argument in the C99 Standard is as follows: “A series of preprocessing tokens enclosed within the parentheses of a function-like macro call, or an expression within the parentheses of a function call expression.” Examples of arguments include values passed to functions or macros. To illustrate this with code, consider the following function declaration: ‘int add(int a, int b)’, where ‘a’ and ‘b’ are the arguments. When calling the function with ‘add(5, 10)’, the values ‘5’ and ’10’ serve as the actual arguments passed to the function during execution.
80. What is an algorithm in C?
Ans:
A clear-cut and sequential algorithm is a system of instructions used to solve problems. For example, this might be an algorithm for subtracting two numbers.
- Step 1: Get going
- Step 2: Declare variables a, b, and sub in step two.
- Step 3: Read values a and b in step three.
- Step 4: Add a and b, then give sub the result. sub↞a – b
- Step 5: Show the subtitle. Step 6: Give up
81. How is the C language used?
Ans:
- A vast built-in function library.
- C allows for the dynamic allocation of memory.
- C makes it easier for programs to compute quickly.
- C is incredibly portable. The language of C is case-sensitive.
- Scripting applications utilise C.
- C permits unrestricted data flow between the functions.
82. What do functions and static variables mean?
Ans:
The value of static variables is maintained between function calls. Static functions are restricted to the area included in the file that they are declared in. Additionally, static functions can only be called within the scope of the current file; they cannot be called from other files. Programming languages must have static variables and functions to manage data and regulate program behaviour.
83. What does the term “middle level” C mean?
Ans:
C shares characteristics with both low- and high-level languages; it is referred to as a middle-level language. For instance, specific C instructions are like standard algebraic expressions combined with high-level language-like English keywords like if, else, and for. On the other hand, C is similar to low-level language in that it allows for the manipulation of individual bits.
84. What distinguishes a union from a structure?
Ans:
- The main distinction between a union and a structure is that a union’s members all share the exact memory location.
- Thus, a value can only ever be held by one member at a time. When variables have a mutual exclusion relationship, unions are utilised.
- Only one of a union variable’s members’ values can be represented at a moment.
- Unions and structures in C++ are similar to classes in that they have public inheritance and members by default.
85. What is an array?
Ans:
An array is a collection of data elements of the same type, which is accessible using the same name. Arrays can have multiple dimensions, such as one-dimensional and two-dimensional arrays, among others. A 2D array is similar to a table with rows and columns, while a 1D array resembles a list. The size of an array must be defined at compile time, allowing for efficient memory allocation. Additionally, arrays enable easy manipulation and access to elements through indexing, making them a fundamental data structure in programming.
86. How can two C strings be compared?
Ans:
The built-in function ‘strcmp()’ allows for the comparison of two strings. It yields 0 when the two strings are identical, a negative integer if the first unmatched character’s ASCII value is less than the second, and a positive integer if the first unmatched character’s ASCII value is greater than the second. This function is case-sensitive, meaning that it distinguishes between uppercase and lowercase letters in the comparison. Additionally, ‘strcmp()’ stops comparing as soon as it finds a difference, making it an efficient way to determine string equality or order.
87. How can CMD be used to start a program?
Ans:
- Step 1: Set up the C compiler (for for instance, gcc
- Step 2: Write the C code in step two and save it as a
.c
file. For example, the file can be named ‘family.c’ and saved on the Desktop. - Step 3: Click the Start button, select All Apps, Windows System folder, and then click the Command Prompt icon, which will open it.
- Step 4: Open Command Prompt and navigate to the directory containing the saved code file. Consider CD Desktop.
- Step 5: Use the installed compiler to compile the code file.
88. Describe the meaning of a preprocessor directive.
Ans:
Preprocessor directives are commands that the preprocessor processes ahead of the start of the code compilation process. “#define}, “#if}, and “#include}” are a few examples. They are employed in conditional compilation, constant definition, and file inclusion. Preprocessor directives are lines in the source file that are distinguished from other lines of text by having # as their first non-whitespace character. Every preprocessor directive modifies the text; the end product is a transformed version of the text that is devoid of both directives and comments.
89. What does the keyword “volatile” mean?
Ans:
The compiler is informed by “The `volatile` keyword” that a variable’s value is subject to change at any point without any intervention from code it finds nearby. As a result, the value is always read from memory, and the compiler is prevented from optimising the variable. The erratic keyword guarantees that every assignment and read of a volatile variable has corresponding memory access by preventing the compiler from optimising code handling volatile objects.
90. Why is C harmful?
Ans:
C is risky because performing a mistaken operation could cause the entire program to lose significance. Since incorrect procedures are said to exhibit undefinable behaviour, the outcome would become unpredictable in this scenario. Furthermore, C programming may not be memory-safe since it permits unrestricted pointer arithmetic using pointers implemented as direct memory locations without any support for bound checking.