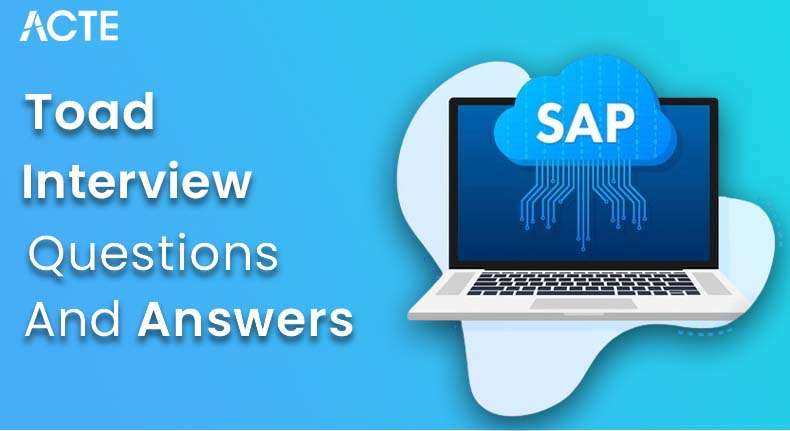
A Frontend Developer specializes in creating visually appealing and user-friendly web interfaces. They utilize technologies like HTML, CSS, and JavaScript to bring designs to life and ensure seamless interactions. With a focus on performance and responsiveness, they optimize websites for various devices and browsers. Their work bridges the gap between design and functionality, enhancing the overall user experience.
1. Define HTML. What does front-end development use it for?
Ans:
HTML is the standard markup language for creating web pages. It structures content using elements like headings, paragraphs, and links. Front-end development utilizes HTML in creating the layout and framework of web pages, thereby arranging content in an organized and accessible way. HTML provides the foundation for incorporating multimedia elements, such as images and videos, enriching the overall user experience on websites.
2. Why do we use JavaScript in front-end development?
Ans:
JavaScript introduces interactivity and dynamic behavior into web pages. It helps developers manipulate the DOM, events, and AJAX requests to create responsive user interfaces. This improves the user experience since the actual updates are carried out in real-time without reloading a page. JavaScript’s versatility allows for the integration of various libraries and frameworks, further enhancing functionality and streamlining development processes.
3. Define CSS. How is it used in the styling of web pages?
Ans:
- CSS refers to the short form of Cascading Style Sheets.
- It is a language that describes the presentation of an HTML document.
- This language targets an element and applies styles like color and fonts on an HTML element.
- Their use improves aesthetics and responsiveness, evading inconsistent styling and different device appearances.
4. What are the different kinds of CSS selectors?
Ans:
Different CSS selectors include universal, type, class, ID, attribute, descendant, child, adjacent sibling, general sibling, pseudo-classes, and pseudo-elements, each allowing for specific targeting and styling of elements on a web page. By utilizing these selectors effectively, developers can create more modular and maintainable stylesheets. For instance, using class selectors allows for the reuse of styles across multiple elements, while ID selectors provide unique styling for specific components.
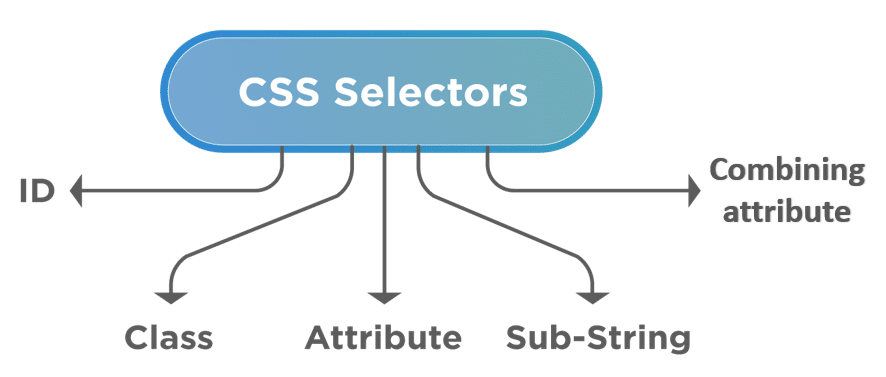
5. How to embed an outside JavaScript file into an HTML document?
Ans:
Use the <script> tag with the src attribute to include an external JavaScript file in the HTML document. It is preferable to place it within the <head> or just before the document’s closing </body> tag. For example:
- script src=”path/to/script.js”
6. What are the key components of a Responsive Web Design (RWD)?
Ans:
Component | Description | Importance |
---|---|---|
Fluid Grid Layout | Uses percentage-based widths for elements, allowing layouts to adapt to different screen sizes. | This ensures a flexible and adaptive design that maintains proportions across devices. |
Media Queries | CSS techniques that apply different styles based on device characteristics, such as width and height | Enables specific styling for other devices, enhancing user experience. |
Flexible | Images that resize within their containing elements, maintaining aspect ratio | Prevent images from overflowing their containers, ensuring they fit well on all screens. |
Viewport Meta Tag | A tag that controls layout on mobile browsers by setting the visible area of the web page | Ensures proper scaling and layout across various devices, especially mobile |
Mobile-First Approach | Designing for the smallest screens first and progressively enhancing for larger screens | Promotes performance and usability by prioritizing essential content for mobile users |
7. Which makes margin different from padding in CSS?
Ans:
Margin is the space outside an element’s border. This is the distance between it and the other elements around it. Padding is the space inside an element’s border between its border and its content. In short, the outer spacing varies by margin, and the inner spacing varies by padding. Understanding the distinction between margin and padding is crucial for effective layout design, as it allows developers to control the spacing and positioning of elements for a balanced and visually appealing interface.
8. Let’s see how to center an element horizontally and vertically using CSS.
Ans:
Flexbox is usually relied upon to center an element with the use of CSS. Create a container: set the display to flex, then justify-content: center to center horizontally and align-items: center for centering vertically. Here is an example:
- .container {
- display: flex;
- justify-content: center;
- align-items: center;
- height: 100vh;
- }
9. What is a responsive web design, and why is it so important?
Ans:
- The responsive web design makes web pages responsive to various screen sizes and devices.
- It is supposed to provide an optimum viewing experience using fluid grids, flexible images, and media queries.
- Media queries are important in accessibility, user satisfaction, and SEO performance.
10. What exactly do CSS media queries do?
Ans:
Media queries enable to apply other styles based on the device’s characteristics, including screen width, height, or orientation. It enables responsive design due to layouts and styling that change with different devices in such a way that it is optimized for usability on all screens. This flexibility ensures that users have a seamless experience, whether they’re using a smartphone, tablet, or desktop computer.
11. Which of the most popular front-end frameworks or libraries are some of the most popular?
Ans:
Popular front-end frameworks and libraries include React, Angular, Vue.js, Bootstrap, and jQuery. These tools are riddled with pre-built components, efficient state management capabilities, and responsive design capabilities that enhance the speed of development and deliver more functionality. They often come with strong community support and extensive documentation, making it easier for developers to find solutions and share best practices.
12. Describe the CSS box model?
Ans:
- The box model explains how an element is rendered on a web page. A box comprises several subcomponents: content, padding, border, and margin.
- The content area contains the text or images, padding is the space within the border, the border is the frame that surrounds the padding, and the margin is the space out from the border.
- Knowing this would be essential to total control over the layout and spacing. This, in turn, affects how elements interact with one another and how space is used.
13. What are some techniques to optimize website performance?
Ans:
- Some techniques to optimize website performance include compressing images and files for faster download.
- Minification of the CSS and JavaScript files also reduces their sizes. A CDN also increases the delivery speed since the content is cached closer to the user.
- Enabling browser caching reduces the number of requests to the server since frequently accessed files are stored in local machines. Another technique used to enhance performance is reducing HTTP requests by combining files.
14. Do users have experience with version control systems, for example Git?
Ans:
- Knowing Git involves understanding core elements like repositories, commits, branches, and merges.
- It allows tracking code changes, collaborating with others, and archiving a project.
- And proficiency includes using commands like pushing, pulling, and reconciling conflicts.
- Using Git makes it straightforward to create branching and versioning to experiment with ideas without compromising the production code-base.
- Overall, Git improves efficiency and smoothes out co-working for development projects.
15. Describe how to debug a code.
Ans:
Typically, the debugging process begins when there is an idea about where the trouble is based on error messages or another way of conducting what does not work. The browser developer tools are helpful to inspect the elements and console logs for clues. Testing incrementally with this scenario out areas of the code can point to the exact area causing a problem. Some additional insight may be derived from documentation or an online resource that can access. Finally, adjustments are made, and extensive testing is done to confirm that the problem has been solved.
16. Explain the difference between server-side and client-side rendering.
Ans:
While server-side rendering produces all the HTML for a request on the server, sending fully rendered pages to the client decreases initial load times and improves search engine optimization but slows down interactions. On the other hand, client-side rendering sends a minimal HTML page and relies on JavaScript to render content in the browser, allowing faster interactions post-load. Each technique has its strengths depending on the application’s needs and user experience goals.
17. What makes JavaScript’s null and undefined values unique?
Ans:
Null is an intentional assignment meaning “no value” and is declared explicitly by the developer. Thus, it means there is no object or value. Undefined states that a variable has been declared but assigned no value yet. Both are false values and represent two scenarios of different purposes in code use. This difference teaches one how to address and debug it correctly and about operations in logic.
18. How does prototypal inheritance work in JavaScript?
Ans:
- Prototypal inheritance allows a derived object to inherit properties and methods from other objects.
- In JavaScript, every object has a prototype; it’s just another object that the object inherits from.
- When JavaScript cannot find a property for access, it goes up the prototype chain.
- This means the result is code that shares methods and reuses properties, making it more efficient.
- A concept often differs from classical inheritance employed in other programming languages.
19. What are higher-order functions? How are they used in JavaScript?
Ans:
- Higher-order functions arenones given functions as arguments, or returnsa fureturnas its result.
- This is used, for example, for array manipulation, where the map, filter, and reduce methods accept callback functions.
- It teaches the principles of functional programming, and the result is clean, modular code. It allows for abstraction and increases reusability when coding complex tasks.
20. How to optimize a website’s performance?
Ans:
Image and file compression is the first step in reducing the load time, and another is browser caching. Browser caching is the storage of often-used resources locally. Then, CSS and JavaScript files are minified, bringing their sizes down. Another implementation is using a CDN. This accelerator delivers content faster by distributing static content for different levels of web infrastructure. Another method is reducing the number of HTTP requests, and that’s possible by combining files as well as lazy loading offscreen images and finally speeding up performance.
21. What are the purposes of a viewport meta tag in HTML, and how do people use it?
Ans:
The viewport meta tag plays a crucial role in responsive web design by allowing developers to control how a web page is rendered on various devices. DefiningDefining parameters such as width and initial scale ensure that content fits appropriately within the screen, enhancing usability and readability. This is particularly important for mobile devices, where screen sizes vary significantly. A common implementation is
- meta name=”viewport” content=”width=device-width, initial-scale=1.0″
, which sets the viewport width to match the device’s width and establishes a default zoom level. Proper use of the viewport meta tag helps create a seamless user experience, effectively adapting the layout to different environments.
22. What is semantic HTML, and why is it important?
Ans:
Semantic HTML elements add meaning to their enclosed content, thus enhancing the structure of a document. Some examples include the use of <header>, <footer>, <article>, and <section> elements. They improve accessibility by enabling screen readers to read elements to users meaningfully. Semantic tags also assist search engines in better indexing and thus improve SEO. They also contribute to cleaner, better-maintained code overall. This would contribute to a better user experience and help with web standards.
23. How does the Document Object Model relate to HTML?
Ans:
Document Object Model is an application programming interface that models HTML documents as a tree. In other words, the structure and contents of the HTML will be treated as objects in this tree. The scripts can be manipulated to create dynamic and interactive interactivity. The changes reflected will happen on the browser by activating the dynamic interactivity of such interactivity. This relationship forms the basis for developers to create interactivity in web-based applications. The DOM is very important to using HTML elements via JavaScript. Understanding the DOM is critically important in front-end development.
24. What does the <head> tag do within an HTML document?
Ans:
- The <head> contains meta-information about the HTML document not rendered on the page.
- Instead, it includes elements such as <title>, <meta>, <link>, and <script>, which further use the tag to define the character set, title, styles, and scripts of the document.
- If used correctly, these will improve the SEO and enhance the loading performance. They give the document proper context.
- The browser uses this to determine the full-page rendering. It plays a very important role in organizing the document.
25. What are the differences between block-level elements and inline elements in HTML?
Ans:
- Block-level elements include the full width of the parent element and take up an entire line in the document.
- Inline elements occupy only as much space as their contents demand and do not start a new line, such as using <span> and <a>.
- This creates and distinguishes how one’s elements will flow throughout the document layout.
- Block-level elements may contain any other block and inline element, whereas an inline element may only have text or another element as a child.
26. How does an ARIA attribute make a web page more accessible?
Ans:
- Now, ARIA attributes make access easier for users with disabilities who use assistive technologies. They add more semantic information to any element, hence aiding assistive technologies in better interpretation.
- This includes roles such as role=”navigation” and states such as aria-expanded, which help describe dynamic changes in the web application.
- This makes using web applications easier by using ARIA attributes. These attributes, added to semantic HTML, ensure that interactive elements are described well. In general, ARIA is part and parcel of inclusive web design.
27. How to create a responsive navigation menu using CSS?
Ans:
Implement media queries to adapt your styles to different screen sizes. For smaller screens, the horizontal menu adjusts its layout to a vertical orientation. Also, usability with JavaScript involves toggling visibility. Can use the CSS properties display: none and display: block to toggle off, hide, or show the menu items.
28. What are CSS preprocessors, and how do they help with development?
Ans:
Using SASS and LESS preprocessors makes it possible to add variable, nesting, and mixin functionality to CSS. These tools enable more reorganized, maintainable stylesheets with reduced redundancy. Variables control colors and fonts in the same manner for any project. Nesting is used to improve page readability by providing a familiar HTML structure.
29. Explain what differences between position: relative and position: absolute have.
Ans:
Position: relative; places an element about its normal flow position in the document flow but keeps the possibility of offsetting without moving the aspect out. On the other hand, absolute position lets an element move out of the document flow and position with respect to the nearest positioned ancestor or the viewport. That is, absolutely positioned elements do not affect elements nearby. Knowing these two is important for proper and effective layout design and control because each plays a different role in the positioning of elements.
30. That is the way the display property works in CSS.
Ans:
The display property determines what is the rendering of an element on the page and how it interacts with other elements on the page. For example, it would use full width, draw height, and start a new line for the block, but inline would take up as much width as necessary without creating a new line. Flex can create flexible layouts. The property affects the general layout flow and the spacing between elements. Sometimes, a change in the type of display can greatly change where the elements are placed and rendered; hence, knowing how to understand this will help approach styling effectively and correctly.
31. What is Flexbox, and how is it used for layout design?
Ans:
- Flexbox is a model of CSS layout that arranges elements in one-dimensional space along a row or column. It offers flexible alignment and, consequently, space distribution among items within a container.
- Properties such as display flex, justify-content, and align-items help developers responsively control layout. Flexbox simplifies complex layouts requiring floats or positioning.
- It is much sought after for achieving adaptable designs across different screen sizes. In general, it maximizes layout efficiency.
32. What is Grid layout in CSS, and when is it useful?
Ans:
- CSS Grid layout defines a two-dimensional layout system involving the design’s rows and columns.
- It aids developers in specifying and defining complex layouts easily through grid areas and item placements.
- It is perfect for larger layouts, such as whole web pages or components, that require alignment in both dimensions.
- Compared to Flexbox, Grid is more flexible when dealing with larger layouts. With Grid, design processes are simplified, and responsiveness is enhanced.
33. What is the easiest solution to apply a CSS reset?
Ans:
- A CSS reset removes the default browser styles to ensure consistent rendering in different environments.
- It can be achieved through a set of CSS rules that normalize margins, padding, font size, and other properties.
- The most common reset is a universal selector to apply as * { margin: 0; padding: 0; box-sizing: border-box; }.
- Thus, styling begins cleanly, making it much more maintainable and giving a solid base for custom styles.
34. What is the use of the property z-index in CSS?
Ans:
CSS The z-index property defines the stack order of overlapped elements. It only applies to positioned elements, which means their position is set to relative, absolute, or fixed. The bigger the z-index value, the more front of other components it is with smaller values. This layered layout is good for designing such layouts where elements need to be layered up, like a dropdown menu or a modal dialog. Therefore, understanding the z-index is an important design concept for visual hierarchy establishment.
35. How can images be optimized for the web?
Ans:
This includes web image optimization, wherein file size is minimized without compromising quality. Techniques include format selection, specifically JPEG, PNG, and WebP, employing tools or online services to compress images, and resizing so they match the dimensions of the display. Images can also be implemented with <picture> element or srcset attribute so that images can load easily on different devices. Lazy loading further ensures the deferred loading of offscreen pictures until they are needed to improve initial load times. All of these best practices improve overall website performance.
36. The Differences Between Local Storage, Session Storage, and Cookies?
Ans:
Local Storage stores data with no expiration date and persists beyond the browser’s closing. When a tab is closed, session storage clears all the stored data for that page’s session duration. Cookies are small files stored on the client side; however, the most common usage of cookies is for state management and tracking purposes with their expiration dates. Cookies have less storage capacity than local storage, whereas session storage will only be available until the session ends. Each has specific usage depending on the lifespan and scope of the data.
37. Describe Single Page Applications.
Ans:
- Single-page applications will load just one HTML page into a browser while ensuring that its contents are updated dynamically as the user interacts with the application.
- This means reducing the reload of pages to provide faster interactions and experiences to the user. SPAs use state and routing management by utilizing JavaScript frameworks, such as React or Angular, and often rely on AJAX to get data so that updates will not have the whole page refreshed.
38. The use of AJAX improves a web application in terms of user experience.
Ans:
- AJAX stands for Asynchronous JavaScript and XML. It enables web applications to asynchronously send and receive data, allowing parts of a page to update dynamically without the need for a full page reload.
- This is more beneficial because it allows real-time interactions, such as form submissions, content loading, and other similar events.
- With AJAX, users can continue interacting with the page while other requests are made in the background.
- It makes the interface relatively smoother and faster, making an application feel faster and more interactive. In this way, AJAX plays a crucial role in the functionality of a modern web application.
39. What is JSON in web development?
Ans:
- JSON is a lightweight, readable, and writeable data interchange format. It is mainly used for communicating data between a server and a web application, mostly in RESTful APIs.
- JSON also allows data structures to be formed in a hierarchy that is very amenable to expressing complex data.
- JavaScript’s simplicity and familiarity have earned it wide usage on web pages. Generally, it facilitates communication from client to server.
40. What are the async and defer attributes in the script tag?
Ans:
This allows for downloading a script in an asynchronous order. While continuing to download, an HTML document can start executing the script as soon as it is available. The defer attribute downloads the script in the background but will ensure that it only runs once the full document is parsed, ensuring the execution orders are correct. Both attributes enhance the load performance of your pages, preventing render blocking. Placing them in strategic positions will improve user experience and how manage scripts.
41. How to implement form validation in JavaScript?
Ans:
Another way of performing form validation in JavaScript is by using event listeners on form submissions. With this approach, the developer can give instant feedback to the users and ensure that the user control inputs are checked against certain criteria, such as required fields or formats. For example, using addEventListener(‘submit,’ function(event) {.}) allows for error handling before submission. Validations may involve checking empty fields or matching patterns with regular expressions. This ensures that only valid data is processed, enhancing data integrity.
42. What does the meta HTML tag do?
Ans:
The <meta> tag encompasses metadata about an HTML document, including its character set, author, and viewport settings. It affects how browsers present and communicate with search engines. They normally set up an anchor for a responsive design for the document’s description, keywords, and viewport. In short, <meta> tags enhance user experience and improve Search Engine Optimization based on correct content rendering. They constitute a very important part of document configuration and optimization.
43. Describe the Fetch API and all of its use cases.
Ans:
- The Fetch API creates a more modern way of making network requests in JavaScript instead of the older XMLHttpRequest. It is easier to make resource fetches and work with responses using Promises.
- Getting data from APIs, loading resource images or scripts, or sending form data are some of the functions where would use it. Further support for GET, POST, and PUT, among others, creates a flexible setting for data interactions.
- This would make error handling and response parsing much easier than traditional development methods.
44. How to handle state within a front-end application.
Ans:
- There are many ways state management can be managed in front-end applications. Local component states usually work for smaller applications, but for more significant ones, solutions like Redux or Context API seem to be a good fit for centralized solutions.
- State management libraries that are globally useful help when the applications are huge as they make accessing the state a unified process within components.
- State management is up to updating and retrieving data so that UI adjusts when what’s going on within the current state needs to update the view accordingly.
45. What does this keyword in JavaScript mean?
Ans:
This keyword returns the context in which a function was called- access to the calling object. Its value depends on how a function is invoked. It. It refers to the object the method belongs to if invoked as a methods method, and it defaults to the global object or undefined in strict mode if used as a function. In arrow functions, this captures the value from the enclosing lexical context. This is important to understand in working with objects and event handlers. Making proper use ensures correct data access within functions.
46. How to handle errors in JavaScript?
Ans:
Error handling using try. catch blocks in JavaScript allows code execution to continue despite an error. In this approach, the code likely to generate an error is placed in the try block while the catch block takes care of the error. Finally can also be used to execute code regardless of whether an error has been raised. With Promises, the asynchronous errors can be captured.catch() Strong error handling enhances the application’s stability and improves user experience since meaningful feedback can be passed to the user.
47. What are Promises, and how do they work in JavaScript?
Ans:
- Promises are objects that can represent the eventual completion (or failure) of an asynchronous operation and its resulting value.
- Methods like `.then()` and `.catch()` can be used to handle either the resolved value in case of a successful operation or the error if an operation fails.
- Promises give cleaner code than the classical callback approach and help avoid the dreaded “callback hell.”
- Promises improve the readability and maintainability of asynchronous code, making it easier to chain the actions.
48. What is event delegation, and how does it improve event handling in the DOM?
Ans:
- Event delegation is a technique in which event bubbling is exploited to handle events at a higher level in the DOM instead of attaching them directly to individual elements.
- Attaching a single event listener to a parent allows managing multiple child elements. Memory usage drops, and efficiency improves, especially when dealing with dynamically added elements.
- Event delegation reduces the complexity of maintaining code because fewer event listeners are needed. This is extremely useful when dealing with constantly updating lists or menus.
49. What are Template Literals in JavaScript?
Ans:
Template literals are string literals with backticks ( “ ), which support multi-line strings and explicit interpolation using ${} syntax. This makes them more readable because, at the same time, it decreases the number of string interpolations in the code, thus enabling dynamic content inclusion directly within the strings. The cluttering created by the necessity of concatenation with + disappears, and the overall visual readability increases. This also helps boost multiline formatting. Overall, they provide a more elegant approach to string manipulation in JavaScript.
50. What does the Array.prototype.map method do?
Ans:
The Array.prototype.map method returns a new array with the elements filled based on a given function’s return values for every component of the calling array. It goes through each item and calls the callback function applied to it, returning a new array without doing anything to the original. It is very useful for transforming data, such as only using specific properties or changing the value of certain items. Since the method does not modify the original array, that’s one reason immutability is maintained. In general, map fosters functional programming in JavaScript.
51. What are the benefits of a module bundler such as Webpack?
Ans:
A module bundler like Webpack organizes and compiles the JavaScript modules into one bundle to enhance performance. It helps optimize codes by removing unused parts and offers code splitting, making the loading process much faster. It allows support within the building process through various asset types, such as CSS and images. This results in streamlining the use of modern JavaScript features through transpilation. The usage of Webpack makes development quite streamlined, enhances performance, and ultimately makes code more maintainable.
52. Explain the role of service workers in web applications.
Ans:
- Service Workers are essentially background scripts, not part of the web page, which enable concepts like offline access and background syncing.
- They help intercept network requests and, therefore, offer the possibility of caching resources, thus improving performance and reliability.
- They enable Progressive Web Apps to work offline as well as serve users with a native app-like experience.
- They manage their push notifications and update resources very effectively. In general, they are important for improving the user experience and improving the application.
53. What is CORS?
Ans:
- CORS is another security feature of web browsers. It enables or disables web pages’ requests for resources from domains other than the domain requesting the webpage.
- It inhibits potential malicious websites from accessing sensitive data available on other sites. If a request is being sent to another origin, the server must include specific CORS headers to permit that type of request.
- This mechanism increases security but does not block legitimate cross-origin requests. APIs and web applications involving interaction with multiple domains need to be suitably configured.
54. How to make a web application more accessible?
Ans:
Improving accessibility involves such best practices as semantic HTML’s proper usage to clarify structuring content, providing accessible alternative text for images combined with using ARIA attributes for further understanding by assistive technologies, sufficient color contrast, keyboard navigability, proper form labeling, and proper error handling, all in support of people who are impaired in their vision. Finally, regularly testing with accessibility tools will help confirm compliance with standards like WCAG to ensure disabled people can access all your pages. In general, all of these measures will create a much more inclusive web experience.
55. What is the purpose of the alt attribute of images?
Ans:
- The alt attribute simply provides alternative text for images explaining their content or function. This text will appear if the image fails to load. If are a blind user, a screen reader will read the text.
- This means visually impaired users will be able to see the content. Using it will enhance SEO as search engines learn the image’s context.
- The text should be brief but precise, stating the exact purpose of the image. Properly applying the alt attribute entails a great contribution to the user experience and conformance to accessibility.
56. Describe how can create an animated transition with CSS.
Ans:
Animated transitions in CSS are defined using the transition property on the target element. It defines which CSS properties to animate, for how long, and the timing function. For instance,
- .box {
- transition: background-color 0.5s ease;
- }
- .box: hover {
- background-color: blue;
- }
57. What are the benefits of using a CSS framework like Bootstrap?
Ans:
It makes it easier for developers by already having pre-designed components and its grid system. They ensure responsive design, make creating layouts that adapt to screen size easier, help with consistent styling, and save time from writing custom CSS. Such frameworks provide extensive documentation and community support, making these easier to use and troubleshoot. Using a CSS framework makes development faster and of quality design.
58. How to add a lazy load for images?
Ans:
Lazy loading delays the loading of the images until they are needed. Thus, the page loads faster, initially lessening the loading time. This can be achieved by using the loading attribute in <img> with a set value of lazy. Here is how do it.
- img src=”image.jpg” loading=”lazy” alt=”Description”
- ;
59. What is CDN?
Ans:
- A Content Delivery Network is geographically distributed inside servers to make web content delivery much faster.
- This actually stores its content-including images, stylesheets, and JavaScript-both on closer-and farther-way servers so that it can reach users as fast as possible, reducing latency and subsequent load times.
- CDNs help reduce latency significantly and allow high traffic that improves web-site performance and reliability.
- The content is also provided with redundancy, so it stays available even when some of the CDNs fail.
60. What keyboard navigation will be the key to accessibility?
Ans:
- Keyboard navigation is a great need for those who cannot use a mouse as they do with their disabilities.
- All interactive elements must be accessible through keyboard shortcuts to improve the usability of this type of user.
- Proper tab order and focus management benefit users in navigating. Implementing keyboard shortcuts for common actions allows more efficient interactions.
- Supporting keyboard navigation helps meet accessibility standards and creates a more inclusive web environment.
61. How to use CSS custom properties (variables)?
Ans:
CSS variables, or variables, are declared using the — syntax inside a CSS rule. Example:
- : root {
- –main-color: #3498db;
- }
Custom properties can be accessed using the var() function. They allow for dynamic styling of several elements and support maintainability through centralized updates, thereby making maintenance easier. This approach also supports theming and responsive designs, where variable values depend on some conditions. In a nutshell, CSS variables improve the organization and flexibility of code.
62. What is the difference between display: none and visibility: hidden?
Ans:
Display: none; This removes the element from the document flow. As if it is not occupying the space of the page. On the other hand, visibility is hidden, keeps the element in the document flow but doesn’t make it appear. Its space is preserved. This gives an implication at the layout level since the elements with display, none, are rendered while those with visibility, hidden, still occupy space. Both properties may be used for hiding elements, but their difference at the layout level is dramatic.
63. How would design toward a mobile-first approach?
Ans:
While beginning a design with designing for smaller screens and then using responsive techniques to add features for larger screens does employ the use of CSS media queries to control style adjustments in correspondence with increases of the viewport, a mobile-first approach involves prioritizing important content in the design to present a streamlined user experience on a mobile device. Focusing on mobile-first enables developers to work toward more efficient designs that improve performance; this can also help alleviate the prospect of progressive enhancement and easier access considerations.
64. What is the purpose of the rel attribute in a <link> tag?
Ans:
- The rel attribute is used to specify the relationship between the current document and the linked resource.
- For example, a <link> tag often specifies the relationship to stylesheets, such as stylesheets for CSS files.
- Other examples include icons for favicons and preload for preloading resources.
- Correct use of the rel attribute helps browsers use resources effectively, improving web application performance and resource management.
65. How to deal with asynchronous operations in JavaScript?
Ans:
- Promises, callbacks, or async control asynchronous operations in JavaScript/await.
- A promise represents that something will be done eventually and allows for chaining with .then() and .catch().
- Async/await syntax makes handling asynchronous code look like writing a synchronous version of the code by easing its control, making it even more readable.
- Async function fetchData() { const response = await fetch(url); } makes handling asynchronous requests neatly. These control better handling for flows involving asynchronous operations and error handling.
66. Arrow functions, what are they, and how are they different from a usual function?
Ans:
Arrow functions are shorthand notations for writing function expressions in JavaScript. They use the => syntax and do not bind their own but inherit it from the surrounding lexical context; the main use case of arrow functions is in callbacks, where this needs to refer to the enclosed scope. Besides, they cannot be used as constructors and do not have arguments or super bindings. Overall, arrow functions improve readability and simplify the management of scope.
67. How can the fetch method be used to make API requests?
Ans:
The fetch method is a native JavaScript function that begins network requests and returns a Promise. Fetch accepts a URL and a configuration object with optional methods, headers, and body content. Then, after a call to response.json(), one has his response converted to JSON to extract data from it easily. Fetch is pretty simple in the making of asynchronous JavaScript API calls.
68. What are common performance bottlenecks in front-end development?
Ans:
Some of the common performance bottlenecks encountered in front-end development include enormous file sizes for images and scripts that cause slow load times, unnecessary DOM manipulation leading to reflows and repaints that affect the speed of rendering, blocking JavaScript and CSS that prevent page rendering and delays user interaction due to the quality of rendering speed, and too much dependency on synchronous requests that make them terrible for end-users.
69. How would conditional rendering be in React?
Ans:
In React, Conditional rendering is done using JavaScript expressions, so JSX accepts it. We can make the ternary operator, or we can use logical && to decide what we want to render, like so:
- export default function LoginForm {
- const isLoggedIn = true;
- return (
- logged in flag
- is logged in? UserProfile / : LoginForm /
70. What is the role of the render method in React components?
Ans:
- This is also used to describe the UI’s appearance. The render method returns JSX, which React transforms into actual HTML elements.
- The method is called each time the component’s state or props change, making it dynamic in updates.
- This method plays a very significant role in outputting inside class components, while in function components, JSX is generated directly.
- Overall, the render method forms the core of React’s component-based architecture.
71. Explain the application of hooks in React.
Ans:
- Hooks are similar to functions that enable developers to use state and lifecycle features in functional components.
- Of the many hooks, the most commonly used include useState to handle local state and useEffect, which deals with the side effects of operations such as fetching data.
- Hooks help create cleaner, reusable code by enabling stateful logic without requiring class components. They also improve the composition of elements and help manage side effects more properly.
- Hooks make managing the state very easy, improving the maintainability of the code in any React application.
72. What is a Virtual DOM, and how does it optimize performance?
Ans:
- The Virtual DOM is a lighter version of the real DOM used by React for optimizing rendering.
- Whenever an object’s state changes, React first changes the Virtual DOM and then compares it to the real DOM in a procedure called “reconciliation.” This minimizes the direct manipulations of the DOM, which can be really slow.
- It also enhances performance by batching updates and reducing the number of direct interactions with the DOM. Overall, the Virtual DOM enriches the efficiency and speed of UI updates.
73. How to handle routing in a front-end application?
Ans:
Can manage routing in front-end applications with the help of libraries such as React Router, Vue Router, or Angular Router. With these libraries, can declare routes and navigate from one view or component to another. These libraries offer dynamic routes, nested routes, authentication route guards, and more. An application that uses routing defines routes and renders the appropriate components depending on which URL the user is viewing.
74. The key prop in React serves what purpose?
Ans:
The key prop in React uniquely identifies elements in a list, hence updating and reordering components efficiently. When it renders lists, React uses the key to know what items have changed, been added, or removed, optimizing rendering. Keys should be stable, predictable, and unique so that it is less probable to do unnecessary re-renders. This makes for better performance within dynamic lists where many items are changed. Therefore, using the key prop will enhance the rendering efficiency in React.
75. What is the best method for optimizing the loading of a web application?
Ans:
Optimize the load time by reducing the HTTP requests, compressing files, and implementing lazy loading for images and scripts. Implementing caching strategies reduces server requests on subsequent visits and lowers load times. Using Content Delivery Networks (CDNs) accelerates asset delivery by serving content through geographically nearer servers. Image optimization and minification of CSS and JavaScript files help optimize performance. These all help to improve the rate of user experience.
76. What are CSS animations, and where do they differ from?
Ans:
- CSS animations make visual effects far more complex than transitions, allowing for the possibility of running animations based on time.
- Animations can have more than one stage, and styles change at different points. Transitions, such as hover effects, are relatively less complex effects that occur when an element’s state changes.
- Transitions require a trigger event to happen, whereas animations will automatically run and can thus cause loops and repeats. Both enrich user experience but for completely different reasons in visual design.
77. How to create a CSS grid layout with columns?
Ans:
Here is how create a CSS grid layout. Apply the display: grid; property on a container element. The number of columns is set with the grid-template-columns property like in the example above:
- .grid-container {
- display: grid;
- grid-template-columns: repeat(3, 1fr);
This creates a three-column layout. Items added to the grid container will be automatically filled into the defined columns. Gaps and other such properties manage space between grid items beyond declared columns. CSS Grid can become very flexible for creating responsive layouts.
78. What are some best practices for structuring your CSS?
Ans:
The best practice for organizing your CSS code is using a naming convention, such as BEM, for more clarity in coding. Related styles should always be grouped; comments can be applied for breaking up sections to help in reading. Scalability is better with code that has modular styles and components written in separate files. A CSS preprocessor can also help with nesting and variable usage, thus aiding maintainability. Regular refactoring and removal of unused styles help keep the code base clean.
79. Compare and contrast “mobile-first” vs. “desktop-first” design ideas.
Ans:
- “Mobile-first” design createscreates a responsive experience first for smaller screen sizes and then adapts for bigger screen sizes.
- This implies basic content and functionality are optimized for mobile users.
- A “Desktop-first” design starts with a layout for larger screens and scales down for mobile.
- Generally, people favor this method for better performance and accessibility.
- Each one influences how styles and layouts are structured and the overall user experience.
80. What are the advantages of using TypeScript in front-end development?
Ans:
- TypeScript offers static typing that helps capture errors at the development stage and not at runtime; hence, the code becomes better and more reliable.
- Its integration with IDEs provides better autocompletion, navigation, and refactoring support. TypeScript improves code maintainability by allowing clear interfaces and type definitions.
- It also facilitates large-scale application development by encouraging modularity and code organization. Overall, TypeScript improves collaboration and efficiency in front-end projects.
81. How to use error boundaries in React?
Ans:
A boundary is a React component that catches any JavaScript errors in its child component tree and returns to rendering a fallback UI. Will define a class component with static getDerivedStateFromError() and componentDidCatch(). These methods allow to update the state and log the error.
- // Log error
- }
- render() {
- if (this.state.hasError) {
- return ;
- }
- Return this. Props. children;
- }
- }
82. What is the purpose of the data-* attribute in HTML?
Ans:
The attribute data-* stores custom data directly inside HTML elements. It is used for attaching additional information without influencing the markup or styling. For example,
- carries a user ID
The JavaScript dataset available allows access to such data and even more of its possibilities and interactivity. Generally speaking, data—* attributes provide flexible embedding of any additional information inside HTML.
83. What are the best practices for using images in a responsive design?
Ans:
Best practices on responsive images include using srcset based on the <img> tag, so different image sizes are served according to the viewport. CSS properties like max-width: 100% ensure images are scaling within their containers. Formats such as WebP or optimizing images could save loading time. An important principle of lazy loading images is improving performance by delaying load until they enter the viewport. All these altogether improve user experience across devices.
84. How to handle user authentication in a front-end application?
Ans:
- User authentication may be accomplished using tokens, usually JSON Web Tokens (JWT), emitted with login and kept in local storage or cookies.
- With every subsequent request, the token is forwarded to the server for validation. Most front-end libraries or frameworks have built-in authentication systems to minimize complexity.
- The states inside the UI must always update based on the authentication status during every login cycle. To protect the user’s data, the tokens should be kept and transferred securely.
85. What does a DOMContentLoaded event in JavaScript do?
Ans:
The DOMContentLoaded event is fired when the initial HTML document has been completely loaded and parsed without waiting for stylesheets, images, or subframes to finish loading. Scripts that are set to execute after the DOM is ready allow elements to be accessed for manipulation. It is used to initialize scripts or attach event listeners. Using DOMContentLoaded would improve performance, as scripts do not block rendering. It positively impacts user experience because it ensures timely script execution.
86. How can an SEO for a web application be improved?
Ans:
- SEO optimization involves page speed optimization, mobile friendliness, and semantic HTML for better content structuring.
- Accurate utilization of meta tags, such as titles and descriptions, helps the search engine understand what is happening on the pages.
- Applying schema markup with structured data enhances the search prominence and gets rich snippets.
- Optimal keyword usage further improves quality content relevant to the niche and topic. Updating content regularly and utilizing backlinks help further appreciate the effectiveness of SEO.
87. What is Progressive Web App Technology?
Ans:
Progressive Web Apps, or PWAs, are web applications that tap into modern web capabilities to give an app-like experience. These technologies support offline access, push notifications, and faster load times through service workers and caching. A PWA is responsive, making sure that it is usable across devices. It also supports home screen installation, just like native apps. Thus, they enhance user engagement and retention by combining the best of web and mobile app experiences. Overall, they improve performance and usability.
88. How can one implement pagination in a web application?
Ans:
Pagination can be applied where data is broken into chunks, and only a portion is shown at any given time. That typically requires fetching the data for this page based on the page number being looked at, combined with the items to display per page. For example, if the user goes to page 2, the application requests the appropriate data segment. The front-end frameworks will have components to render the pagination controls, so navigation is relatively trivial. Effortless fetching of data and refreshing the UI add even more to the usability.
89. What is the difference between CSS modules and global CSS?
Ans:
- CSS Modules provide a scoped way of styling. This means that class names are unique to a component, thus preventing any naming conflicts.
- They generate unique identifiers for classes; therefore, styles are modular and reusable without unintentional overrides.
- Whereas globally applied CSS applies universally throughout the entire application and introduces conflicts and maintainability problems in large projects.
- Although it becomes easier to configure global CSS, it gets very cumbersome with large codebases. CSS modules help better encapsulate and maintainability.
90. What are the benefits of using a Content Delivery Network (CDN) for web applications?
Ans:
- CDNs reduce latency by serving content from servers geographically closer to users, resulting in faster loading speeds.
- CDNs enhance redundancy and minimize downtime by distributing content across multiple servers, ensuring higher availability.
- CDNs can handle spikes in traffic more effectively, allowing web applications to scale seamlessly during high-demand periods.
- Many CDNs offer security features, such as DDoS protection and secure token authentication, helping to safeguard web applications.