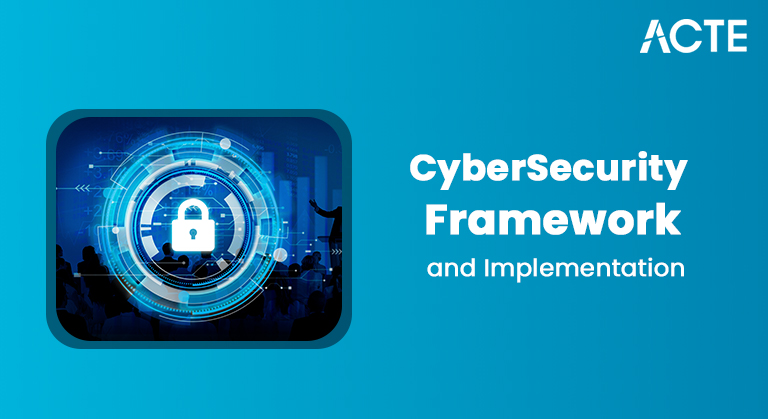
- Introduction to use memo Hook
- How useMemo Works
- When to Use useMemo
- useMemo vs use callback
- Common Pitfalls with useMemo
- Performance Considerations and Best Practices
- Practical Example: Optimizing Complex Components
- Conclusion
Introduction to use memo Hook
The useMemo hook in React allows developers to optimize expensive calculations by memoizing the result of a function call. Memoization is the process of storing the result of a computation so that it doesn’t need to be recalculated when the inputs remain the same. This is particularly useful in performance-critical applications or in cases where a component re-renders frequently. If you’re looking to strengthen your React skills along with broader front-end and back-end concepts, explore our Web Designing & Development Courses to gain hands-on experience. Whenever a component re-renders in React, it executes the functions and recalculates values. Sometimes, re-rendering a component triggers recalculations that aren’t necessary if the inputs haven’t changed. The useMemo hook prevents these unnecessary recalculations, thus improving the application’s performance.
Syntax:const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]); Here:
- computeExpensiveValue(a, b) is the function you want to memorize.
- [a, b] is the dependency array that determines when the value should be recomputed (i.e., when any value in the array changes).
How useMemo Works
useMemo is primarily used to optimize performance in React by memorizing a function’s return value and recomputing it only when specific dependencies change. It works by “remembering” the previously computed value and only re-running the function when the dependencies change. When a component re-renders, React checks whether the dependencies in the array have changed. If they have, React recalculates the function. If not, React uses the previously stored value, avoiding a recalculation. This can be particularly useful when dealing with complex or expensive computations, such as filtering large lists or recalculating values for a chart. If you’re exploring a career in front-end or full-stack development, understanding tools like useMemo is key check out our guide on How to Become a Web Developer to learn more.
<>bExample:- const expensiveCalculation = (num) => {
- console.log(‘Recalculating…’);
- return num * 100;
- };
- const MyComponent = ({ number }) => {
- const memoizedValue = useMemo(() => expensiveCalculation(number), [number]);
- return {memoizedValue};
- };
In this example, the expensive calculation will only be called again if the number prop changes. If the number remains the same, React will use the memoized result from the previous render, avoiding unnecessary recalculations.
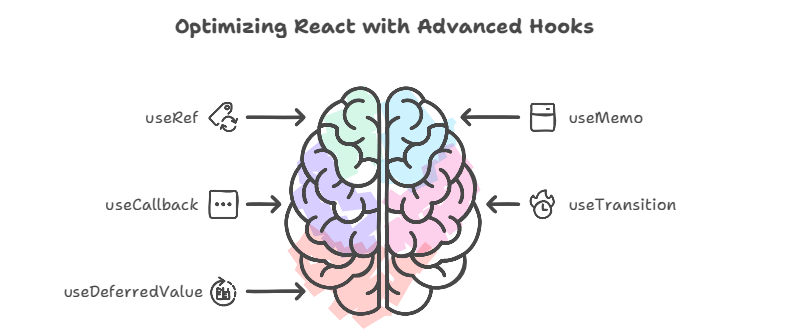
When to Use useMemo
Although useMemo can help improve performance, it is not always necessary and should be used selectively. It’s important to consider whether the computational cost of recalculating the value is high enough to warrant optimization. For developers aiming to make informed decisions about performance techniques like this, pursuing a Web Developer Certification can provide deeper insights into when and how to apply such tools effectively. For simple computations, the overhead of using useMemo may outweigh its benefits.
You should consider using useMemo in the following situations:
- Expensive Calculations: If a calculation takes a long time, use a memo to memorize the result.
- Rendering Large Lists: If you render an extensive list or complex components that depend on computations, memoization can prevent unnecessary recalculations during re-renders.
- Referential Equality: If you pass an object or array as a prop to a child component, and it is recreated on every render, useMemo can help maintain referential equality and prevent unnecessary re-renders. Example Scenario:
- const ListComponent = ({ items }) => {
- const filteredItems = useMemo(() => {
- return items.filter(item => item.isActive);
- },
- [items]); // Recompute only if ‘items’ change
- return (
- {filteredItems.map(item =>
- (
- ))}
- );
- };
- const DataList = ({ data }) => {
- const sortedData = data.sort((a, b) => a.name.localeCompare(b.name));
- const filteredData = sortedData.filter(item => item.isActive);
- return (
- {filteredData.map(item => (
- {item.name}
- ))
- }
- );
- };
- const DataList = ({ data }) => {
- const sortedData = useMemo(() => data.sort((a, b) => a.name.localeCompare(b.name)), [data]);
- const filteredData = useMemo(() => sortedData.filter(item => item.isActive), [sortedData]);
- return (
- {filteredData.map(item => (
- {item.name}
- ))}
- );
- };
In this example, filtered items will be recalculated only if items change, which improves performance when the list is extensive.
useMemo vs use callback
The useMemo hook and useCallback hook in React are both used to optimize performance by memoizing values and functions, but they serve slightly different purposes and are applied in different scenarios. useMemo is primarily used to memoize the result of an expensive function call or computation, meaning that it stores the return value of a function so that it does not have to be recalculated on every render. This is particularly useful when dealing with expensive calculations or transformations that don’t need to be re-executed unless certain dependencies have changed. On the other hand, useCallback memoizes the function itself, preventing it from being redefined on every render. This ensures that the function reference remains consistent across renders unless its dependencies change. While useMemo is typically applied when you want to store the result of a computation, useCallback comes into play when you want to avoid re-creating a function, particularly when that function is passed as a prop to child components. Without useCallback, every time a parent component re-renders, the function would be recreated, causing unnecessary re-renders of the child component. Developers working with dynamic rendering patterns, such as using a For Loop in React can also benefit from understanding when to apply these hooks effectively. Therefore, using useCallback helps optimize performance by preventing unnecessary re-renders due to new function references. This is crucial in large applications with many components and complex rendering logic, as it helps ensure smooth and efficient updates without performance degradation.
Excited to Obtaining Your web developer Certificate? View The web developer course Offered By ACTE Right Now!
Common Pitfalls with useMemo
While useMemo is a powerful optimization tool, it is essential to avoid common mistakes that can lead to suboptimal performance or even bugs:
A. Overuse of useMemoIt’s easy to overuse useMemo to optimize every calculation, but this can backfire. React’s default rendering behavior is optimized, and unnecessary use of useMemo can introduce additional complexity without significant performance benefits. Continually measure performance before and after applying the use of a memo.
B. Incorrect Dependency ArraysThe dependency array is critical for the correct operation of useMemo. If you omit dependencies or mistakenly include unnecessary ones, it can cause issues with incorrect memoization. If you leave out dependencies, the memoized value might never get updated, and if you include unnecessary ones, React may unnecessarily recompute values. Understanding how dependency arrays work is especially important when using advanced state management tools if you’re new to such concepts, learning What Is React Redux can provide helpful context for how memoization fits into larger React applications.
Example of Incorrect Usage:const memoizedValue = useMemo(() => computeExpensiveValue(a, b), []); In this example, the dependency array is empty, so computeExpensiveValue will only run once, regardless of any changes to a or b. This can cause bugs, as the memoized value will never be recomputed when a or b changes.
Interested in Pursuing Web Developer Master’s Program? Enroll For Web developer course Today!
Performance Considerations and Best Practices
When using useMemo, it’s essential to follow some best practices to maximize its performance benefits:
A. Measure Before and AfterBefore applying useMemo, measure your application’s performance. Use React’s developer tools, browser DevTools, or profiling libraries like why-did-you-render to understand the performance impact.
B. Avoid Premature OptimizationWhile React can be slow in some cases, it’s essential not to optimize your code prematurely use useMemo only for expensive calculations or large datasets and only when it makes a measurable difference. If you’re experimenting with larger applications or looking for hands-on practice, exploring full stack project ideas can help you understand where and how performance optimizations like useMemo are most effective.
C. Use useMemo for Referential EqualityFor functions and objects passed as props to child components, use useMemo to ensure that the references to those values remain the same between renders. This helps avoid unnecessary re-renders of child components.
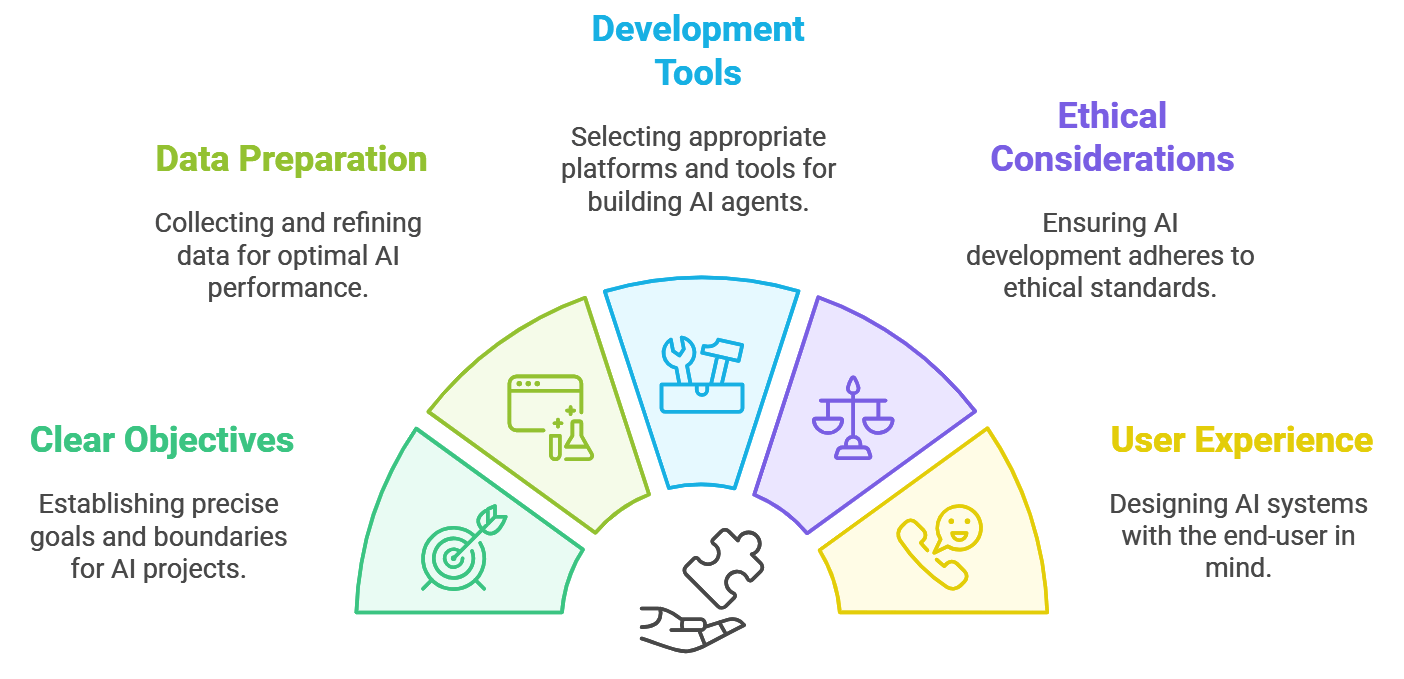
Practical Example: Optimizing Complex Components
Let’s explore a practical example of how a memo is helpful. Suppose you have a large dataset and filter and sort it for rendering. Without useMemo, these operations would be performed on each render, leading to a performance hit.
Without useMemo:Every time the component renders, data.sort() and data.filter() are executed, which could be expensive for large datasets.
With useMemo:Using useMemo, sorting and filtering only happen when data or sorted data changes. This avoids unnecessary recalculations during re-renders, leading to improved performance.
Conclusion
The useMemo hook in React is a valuable tool for optimizing performance, especially in scenarios involving expensive computations or handling large datasets. By memoizing the results of functions, useMemo prevents unnecessary recalculations, recalculating only when the dependencies change. This behavior ensures that React applications can run more efficiently by avoiding repetitive calculations on each render. If you’re aiming to deepen your understanding of performance optimization and front-end skills, our Web Designing Training can help you build a strong foundation. The primary benefit of useMemo is its ability to store function results, which can reduce rendering time, particularly for computations that require significant resources. However, it’s essential to use useMemo judiciously. Misuse or premature optimization can introduce unnecessary complexity and bugs. For example, improper dependency arrays can lead to stale or incorrect data being used. Developers should continuously measure performance before and after using useMemo to assess its impact. Focus on optimizing the most costly operations, rather than applying useMemo to every function or calculation, to achieve noticeable improvements in efficiency. Ultimately, the strategic use of useMemo can help streamline React applications and enhance user experience.