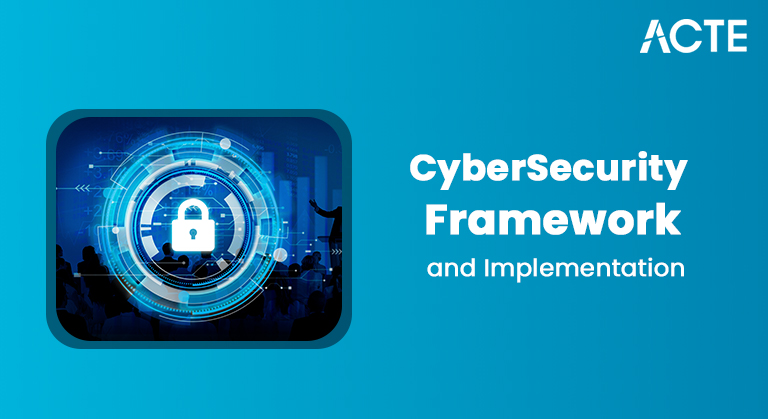
- Introduction to Loops in React
- The Basics of a For Loop in JavaScript
- Using the For Loop in React to Render Lists
- Alternatives to For Loop in React (map Method)
- Best Practices for Using Loops in JSX
- Handling Dynamic Data with For Loops in React
- Performance Considerations when Using Loop
- Common Pitfalls and How to Avoid Them
- Conclusion
Introduction to Loops in React
React is a popular JavaScript library used for building dynamic and interactive user interfaces. One common use case in React is rendering lists of data, such as displaying a list of users, products, or messages. In such cases, loops play a crucial role by allowing developers to efficiently generate multiple elements without manually coding each one. Although traditional loops like for loops are fundamental in JavaScript, React has its own optimized methods for rendering elements. Specifically, you cannot place a for loop directly inside JSX, as JSX expects expressions, not statements. Instead, React encourages the use of the map() method, which is the most common and recommended way to iterate over arrays and return elements for rendering. This method is clean, functional, and integrates well within JSX syntax. If you’re looking to dive deeper into React and other web technologies, our Web Designing & Development Courses can provide the skills you need. Nevertheless, understanding how to use traditional loops like for loops in React is also valuable, particularly when performing logic or processing data before rendering. This flexibility allows developers to write more controlled and optimized React components.
The Basics of a For Loop in JavaScript
A basic for loop in JavaScript looks like this:
- for (let i = 0; i < 5; i++)
- {
- console.log(i);
- // Logs 0, 1, 2, 3, 4
- }
- Initialization: Sets up a variable (e.g., let i = 0) that starts the loop.
- Condition: Determines how long the loop will run (e.g., i < 5).
- Increment: Modifies the loop variable after each iteration (e.g., i++).
- Body: Contains the logic or code that runs on each iteration.
In React, this loop structure can be helpful when preparing data for rendering or performing side effects before returning JSX.
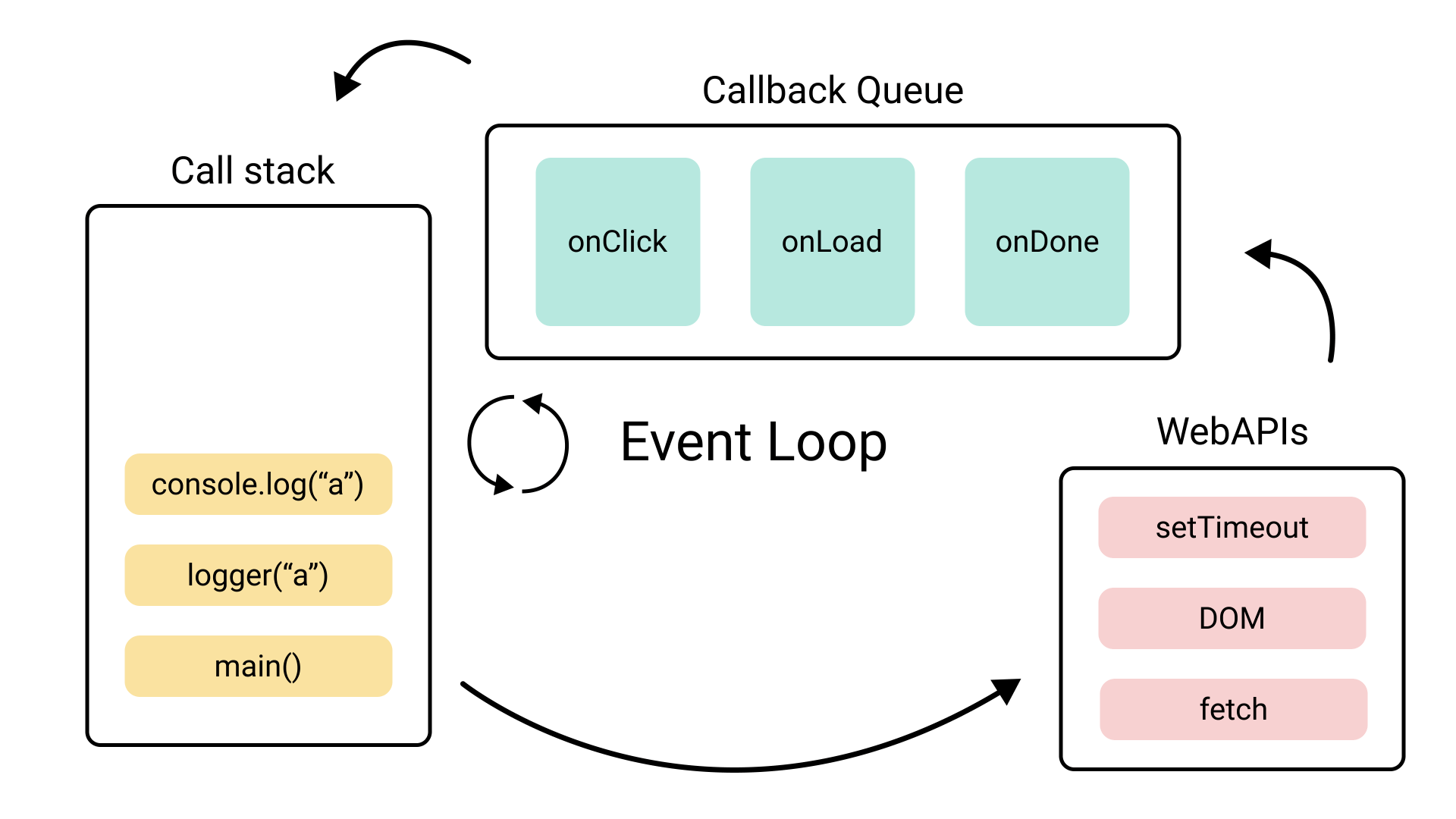
Using the For Loop in React to Render Lists
React components are often responsible for rendering lists of data. By using a for loop in React, you can prepare the data before returning JSX. For example, consider the following scenario where we want to render a list of items in a React component:
In the given React component, the ItemList function is designed to render a list of items passed as a prop. It begins by importing React, which is essential for working with JSX and components. Inside the component, an empty array named listItems is initialized to store the list elements. A traditional for loop is then used to iterate over the items array. During each iteration, a new element is created with a unique key set to the current index (i) and the corresponding item as its content. These elements are pushed into the listItems array. Finally, the component returns a element that contains all the generated list items. This approach demonstrates how a traditional for loop can be used in React to construct JSX elements before returning them within the component’s render output. The component is then exported as the default export for use in other parts of the application. If you’re interested in learning more about React and how to create applications like this, check out our guide on How to Become a Web Developer.
export default ItemList;In this example:
- The for loop iterates through the items array.
- On each iteration, a list item (
- ) is created and pushed into the listItems array.
- After the loop is complete, listItems are rendered as part of the component. This is a simple use case, and while it works, React’s map() method provides a more idiomatic and concise solution.
Alternatives to For Loop in React (map Method)
The map() method is the most commonly used approach in React to loop over data and render JSX. This method is cleaner, more declarative, and integrates seamlessly with JSX.
Here’s how the same functionality can be implemented with map():
In this React component, ItemList is a functional component that takes items as a prop and renders them as a list. The component begins by importing React, which is necessary to use JSX and create components. Inside the return statement, a element is used to wrap a list of elements. These list items are generated by using the map() method to iterate over the items array. For each item in the array, a corresponding An element is created with a unique key based on the current index and the item itself as the content. This approach is a concise and efficient way to render dynamic lists in React, as it keeps the code clean and readable while leveraging JSX expressions. If you’re preparing your career as a full-stack developer, you may find it helpful to refer to a Full Stack Developer Resume for structuring your professional experience and skills.
export default ItemList;
The map() method iterates over the items array and returns a new array of JSX elements, which React can render. The key attribute is crucial for React’s reconciliation process, ensuring that list items are efficiently updated.
Excited to Obtaining Your web developer Certificate? View The web developer course Offered By ACTE Right Now!
Best Practices for Using Loops in JSX
Although a for loop in React is possible, the map() function is generally preferred in JSX because of its more declarative nature. However, there are some best practices to follow when using loops in React:
- Avoid Side Effects in Loops: Loops in React should focus on rendering or preparing the data. Avoid placing logic that mutates state inside a loop. This could lead to unintended side effects and performance issues.
- Key Prop: Always provide a unique key prop when rendering a list of items. This helps React identify which items have changed, been added, or removed. Using an index as the key is acceptable for static lists but should be avoided for dynamic lists that may change order.
- Conditional Rendering: If you need to conditionally render elements based on specific criteria, consider using a loop with conditional operators (e.g., ternary operators) or if statements before the return statement.
Interested in Pursuing Web Developer Master’s Program? Enroll For Web developer course Today!
Handling Dynamic Data with For Loops in React
When dealing with dynamic data, such as data fetched from an API, you should handle asynchronous operations before rendering the looped elements. For a deeper understanding of how to manage such dynamic operations effectively, consider our Web Developer Certification which covers key concepts like asynchronous JavaScript, data fetching, and rendering. Here’s an example that demonstrates fetching data and rendering it dynamically:
- import React, { estate, use effect } from ‘react’;
- const ItemList = () => {
- const [items, setItems] = useState([]);
- use effect(() => {
- // Fetch data asynchronously
- fetch(‘https://api.example.com/items’)
- .then(response => response.json())
- .then(data => {
- systems(data); // Set the fetched data to state
- })
- .catch(error => console.error(‘Error fetching data:’, error));
- }, []);
- const listItems = [];
- for (let i = 0; i < items.length; i++) {
- listItems.push(
- {items[i].name}
- return
- {listItems}
- };
- export default ItemList;
In this example:
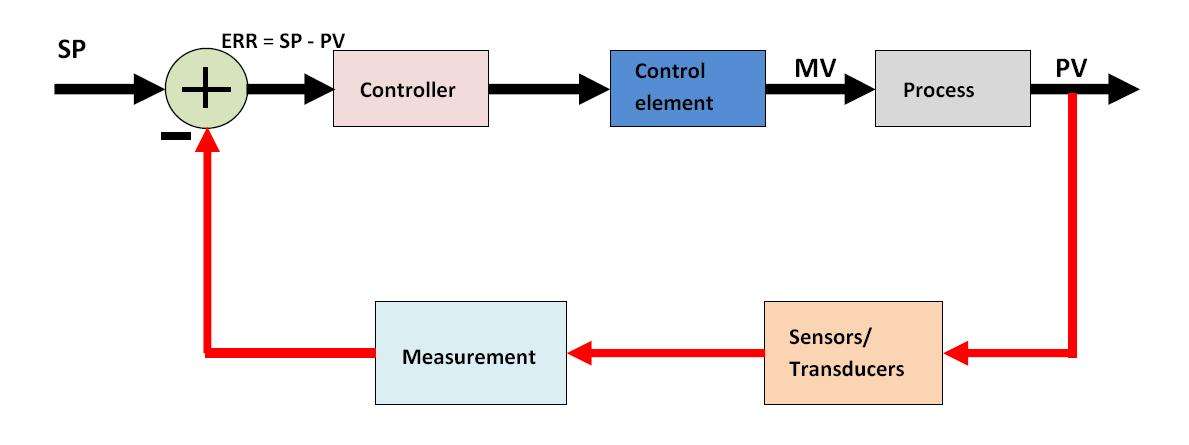
Performance Considerations when Using Loops
While using a for loop in React can be straightforward, performance considerations should be considered, especially when dealing with large lists or frequently changing data.
- Avoid Unnecessary Re-Renders: React’s virtual DOM and reconciliation process help optimize rendering, but large lists with expensive computations can still cause performance bottlenecks. When rendering lists of items, use React. Memo or the shouldComponentUpdate lifecycle method to avoid unnecessary re-renders.
- Lazy Loading: When working with large lists, it might be necessary to implement lazy loading to improve performance. Instead of rendering all items at once, render only the items visible in the viewport and load additional items as the user scrolls.
- Efficient Data Updates: Ensure that only the changed items are re-rendered when updating the list. For example, if you add, remove, or update items, React will efficiently re-render only the necessary list parts if each item has a unique key.
Common Pitfalls and How to Avoid Them
While loops can be useful in React for rendering lists or performing repeated actions, there are several common pitfalls developers should be aware of. One major issue is incorrect key usage using array indices as keys in dynamic lists can lead to rendering problems when items are reordered or removed. It’s best to use unique identifiers for each item to ensure consistent and accurate updates. Another important consideration is avoiding direct state or prop mutation inside loops. Mutating state directly can cause unpredictable behavior and render inconsistencies; instead, always use proper state management methods like setState or state updater functions. If you’re looking to learn the best practices and essential steps to become a proficient React developer, the React JS Developer Roadmap can provide a clear path for your learning journey. Additionally, if a loop involves complex logic, it’s better to handle that logic outside of the JSX and store the result in a variable before rendering. This keeps the JSX clean, maintainable, and easier to understand. Finally, when dealing with large datasets, failing to optimize list rendering (such as by implementing virtualization or pagination) can result in significant performance issues. Proper optimization ensures a smoother and more efficient user experience.
Conclusion
Using a for loop in React can be a powerful approach for handling repetitive tasks or rendering lists of elements, especially when more complex logic is involved before the actual rendering takes place. Although React promotes a declarative coding style and encourages the use of the map() function for rendering lists directly within JSX, there are scenarios where a traditional for loop is more appropriate. For instance, when you need to preprocess or manipulate data before rendering, a for loop can offer greater control and clarity. If you’re looking to improve your skills in React and other web development techniques, our Web Designing Training can help guide you through advanced concepts and best practices. However, it’s important to follow best practices to maintain performance and readability. This includes providing unique key props for each list item to help React identify changes efficiently during re-renders, avoiding side effects inside loops to prevent unexpected behaviors, and optimizing your logic to avoid unnecessary operations that could impact performance. By balancing the flexibility of for loops with React’s declarative strengths, you can build applications that are both efficient and maintainable.