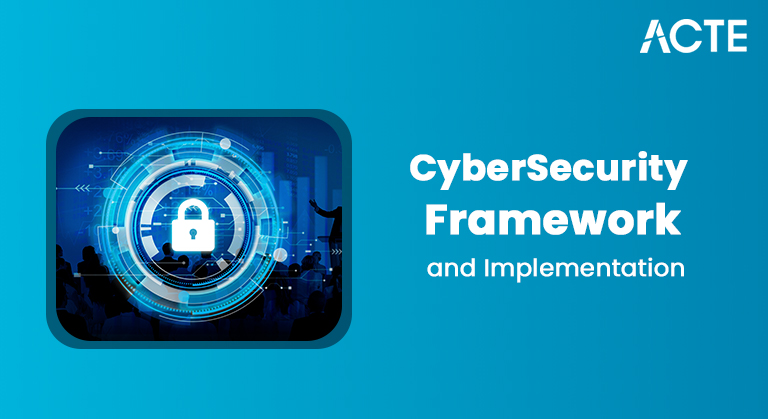
- Introduction to the map() Function
- Why map() is Useful in React
- How to map() Works in React
- Rendering Lists Dynamically
- Best Practices for Using Map () in React
- Handling Complex Data Structures with Map ()
- Performance Considerations in React
- Conclusion: Mastering map() in React
Introduction to the map() Function
The Map() Function in React JS is a core JavaScript method for iterating over arrays and transforming each element into a new form. Web Designing and Development Courses an essential tool in React for dynamically generating JSX elements from various data. Understanding how to leverage map() in React is crucial for efficiently handling your app’s lists, tables, and other dynamic content. React heavily relies on rendering components and data structures dynamically, and map() is a natural fit for iterating through data and creating React elements from it.
Why map() is Useful in React
In React, much of your UI will depend on data lists, whether they’re arrays of strings, numbers, or even more complex objects. This is where map() comes into play. It simplifies the process of iterating over data and rendering corresponding JSX elements. Without a map(), manually iterating through data in React JS could result in lengthy and hard-to-manage code. The beauty of map() is that Downloading Node.js and NPM abstracts away the complexity of loops, making your component code clean, readable, and maintainable. Additionally, React’s declarative nature pairs beautifully with map(), allowing you to declare how data should be transformed into React elements , without worrying about the low-level iteration logic.
Unlock your potential in Web Development with this Web Developer Certification Courses .
How map() Works in React
In its most basic form, map() takes a callback function and applies it to each element in an array. This callback function can transform the element or generate a corresponding JSX element. Here’s how it works in the Node.js Non-Blocking Behavior in Web Developer Training of React: when using map() in React elements, you typically pass a callback function that returns JSX for each array element. The syntax is straightforward and commonly used for rendering lists of components dynamically. Each JSX element generated from Map() Function in React JS must include a unique key prop to help React elements identify which items have changed, been added, or removed. This improves performance and prevents rendering issues. map() is especially useful for working with arrays of data like user lists, product catalogs, or navigation menus. Using map() makes your React code more declarative, readable, and maintainable.
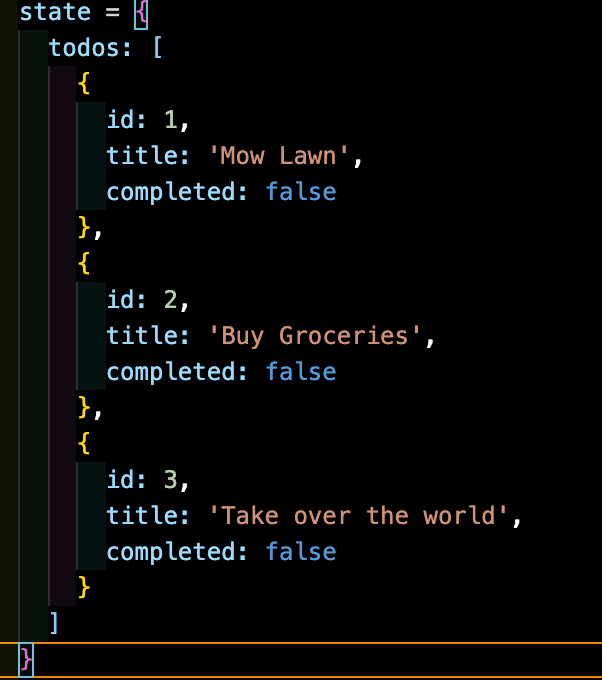
- For each item in the array, map() will generate and return a React element.
- In most cases, map() returns an array of elements, which React JS can render efficiently.
- The callback function in the map() receives the array DevOps vs Full Stack and its index (or other parameters, depending on your needs), and you can use this data to structure your output.
- Managing Keys Properly: React uses the key Node.js Developer to track the identity of each element. It is essential to provide unique, stable keys to prevent unnecessary re-renders.
- Avoiding Common Pitfalls: A common issue arises when using a map() to render complex or large lists. If you forget to optimize rendering, it can negatively affect performance.
- Minimizing Re-renders: React components can re-render frequently, especially if their state changes. Be mindful of this when rendering large lists, and consider using optimization techniques like React.memo() to avoid unnecessary rendering.
- Managing Keys Properly in React Lists: When rendering lists in React using map(), each child element must have a key prop. This key helps React identify which items have changed, been added, or removed, allowing it to update the DOM efficiently. Without proper keys, React may re-render entire lists unnecessarily or display incorrect UI states.
- Why Unique and Stable Keys Matter: Keys must be unique among siblings and stable—React Native Made Simple – Beginner’s Step-by-Step Guide shouldn’t change between renders. Using array indexes as keys can cause problems, especially when items are reordered or removed. Instead, use a unique ID from your data (like a user ID or product SKU) to ensure React can accurately track elements over time.
- Avoiding Common Pitfalls with map() in React: One common mistake when using map() is forgetting to include the key prop or using non-unique values. Another issue is rendering large or complex lists without optimization, which can degrade performance. Always ensure your mapping logic is clean, and each rendered element includes a key for best results.
- Understanding Re-Renders in Large Lists: React components can re-render frequently due to state or prop changes. In large lists, this can result in performance bottlenecks if not managed properly. Each time a parent component updates, all child components may re-render unless memoization or other performance techniques are used.
- Optimizing Performance with React.memo(): To minimize unnecessary re-renders, especially in large lists, React provides React.memo(). This higher-order component prevents a component from re-rendering unless its props have changed. When combined with proper key usage and data structuring, React.memo() can significantly improve the performance of list rendering in React apps.
- Virtualization: Libraries like react-window or react-virtualized allow you to render only the visible items in a list, which is beneficial for long lists.
- Memoization: React’s React.memo() and useMemo() hooks help you avoid unnecessary re-renders by memoizing components or values that don’t change frequently.
Learn how to manage and deploy Web Development Services by joining this Web Developer Certification Courses today.
Rendering Lists Dynamically
One of the most common uses of map() in React is rendering dynamic lists. Imagine you have an array of data, like a list of names ,and want to render each name as an item in an unordered list Here, map() makes Web Developer Certification Courses easy to create the necessary HTML structure from the data dynamically. Each item in the array is processed, and a corresponding JSX element is returned for every aspect. When rendering dynamic lists, including the key prop of each component is important. React.memo uses this key to efficiently identify which items have changed, been added, or removed.
Best Practices for Using Map () in React
While map() is a powerful tool, there are certain best practices to remember when using it in React.
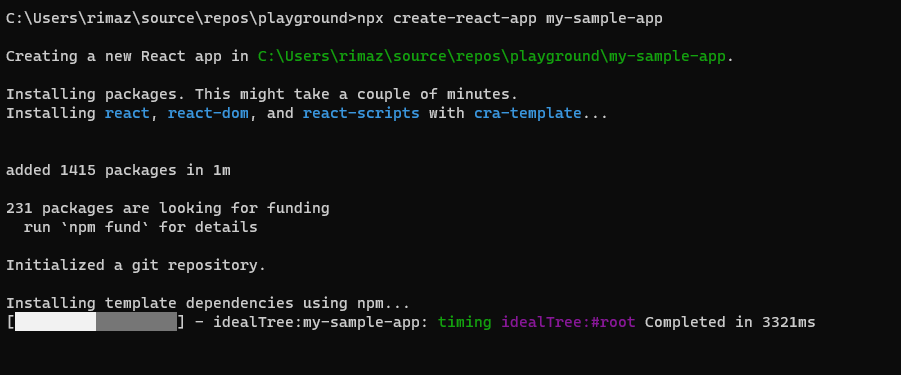
Boost your career in tech by enrolling in this Web Developer Certification Course today.
Handling Complex Data Structures with map()
React apps often deal with complex data structures like arrays of objects or nested arrays. When using a map() function in React JS, you can extract the necessary data from these structures and map over them efficiently. For example, you have an array of objects representing users, each with properties like name, email, and avatar, map() can help you display these properties dynamically in a list. For more complex data, you might need to call map() inside another map() for deep-nested structures. You can also map through objects (instead of arrays) using Object.keys() or Object.entries() to extract and iterate over data. It’s important to ensure that each element rendered through map() has a unique key prop, especially in nested lists. When Scope of Web Development deeply nested data, breaking it into smaller, reusable components can improve readability and maintainability. Using conditional rendering inside map() helps avoid displaying null or undefined values. Data transformation functions can also be applied before mapping to clean or sort the data as needed. Overall, mastering map() in combination with React’s component architecture is essential for handling dynamic content efficiently.
Boost your chances in Web Development interviews by checking out our blog on Web Development Interview Questions and Answers!
Performance Considerations in React
Performance can become an issue when working with map() in React, especially with large datasets. Since React’s virtual censures efficient updates, the primary performance consideration when using map() involves minimizing unnecessary re-renders. Additionally, avoid updating the state too frequently, as each state Understanding React JS Features and Benefits Explained could trigger a re-render of your entire list. To optimize performance when rendering large lists, consider techniques like:
Conclusion
Conclusion: Mastering map() in React To conclude, Map () in React is an indispensable tool in React development. It enables developers to efficiently render dynamic lists and manage complex data structures. By understanding how Map() Function in React JS, you can streamline your rendering logic and reduce unnecessary complexity in your React components. Some key takeaways include using the key prop to help React.memo efficiently update the Web Designing Training and optimize performance when rendering large lists. By adhering to best practices and staying mindful of performance considerations, you can create high-performance, scalable React applications that make the most of JavaScript’s map() Function. With this breakdown, you’ll be well on your way to mastering using map() in React. Remember these best practices and explore new Map () in React methods to make your React components more dynamic and maintainable.