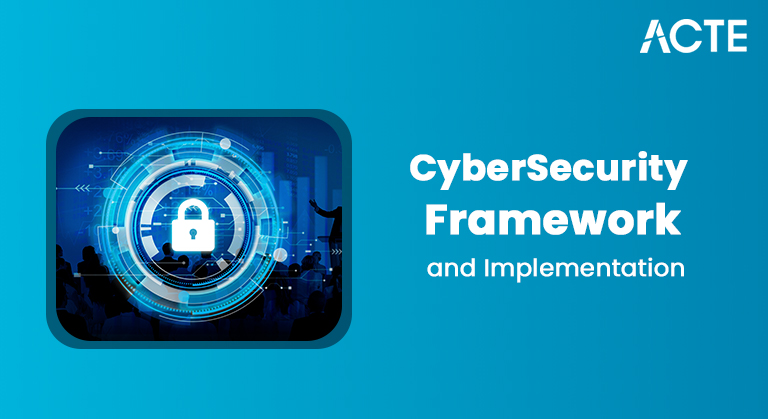
- Introduction to React Native and Barcode/QR Scanning
- Setting Up React Native Environment
- Integrating Barcode/QR Scanning Libraries
- Creating the UI for the Barcode/QR Scanner
- Implementing Barcode/QR Code Detection
- Handling Barcode/QR Data
- Optimizing for Different Devices and Platforms
- Publishing and Maintaining the App
- Conclusion
Introduction to React Native and Barcode/QR Scanning
React Native is a popular framework developed by Facebook for building mobile applications using JavaScript and React. It allows developers to write code once and run it on Android and iOS devices. The framework uses native components to render the application, ensuring a smooth and performant user experience on both platforms. With the increasing demand for mobile apps, React Native has become a go-to solution for building cross-platform apps with a native look and feel. Barcode and QR code scanning functionality is Web Designing and Development Courses for many mobile apps today. From e-commerce platforms and ticketing apps to inventory management systems and contactless payments, barcode and QR code scanning allows users to quickly access data or perform actions by scanning a code. In this guide, we’ll explore how to build a simple React Native app that can scan barcodes and QR codes. We’ll look at setting up the development environment, integrating scanning libraries, designing the user interface, and optimizing the app for different devices.
Setting Up React Native Environment
Before you begin developing a React Native app for barcode and QR scanning, it’s important to first set up your development environment. Follow these steps to get started with React Native:
- Install Node.js: React Native relies on Node.js to run its JavaScript engine and manage project dependencies. You’ll need to install the latest stable version of Node.js from the official website.
- Install React Native CLI: To create a React Native application, you’ll need the what-is-react Native Command Line Interface (CLI) tool. Install this tool globally on your system to manage and generate React Native projects.
- Set Up Android and iOS Environments: For Android development, you’ll need to install Android Studio and configure the Android SDK. For testing on iOS, you must have a Mac with Xcode installed. These tools allow you to run your app on both simulators and physical devices. Install Android Studio and set up an emulator for testing. Install Xcode and set up an iOS simulator for testing (this is only available on macOS). Once these tools are set up, you can create a new React Native project that serves as the foundation for your barcode scanner app.
- Start the Development Server With your project created, navigate to the project folder and launch the React Native development server to begin testing your app on Android or iOS devices.
Learn how to manage and deploy Web Development Services by joining this Web Developer Certification Courses today.
Integrating Barcode/QR Scanning Libraries
ntegrating barcode and QR scanning functionality into a React Native app can be done with several libraries available in the React Native ecosystem. Some of the most popular ones include react-native-camera and react-native-qrcode-scanner, which offer straightforward solutions for barcode and QR code scanning. Another widely used library is react-native-camera-kit, which provides robust support for both barcode and QR scanning. To start, you need to install the necessary package using a library like react-native-camera-kit by running a simple command in your project.
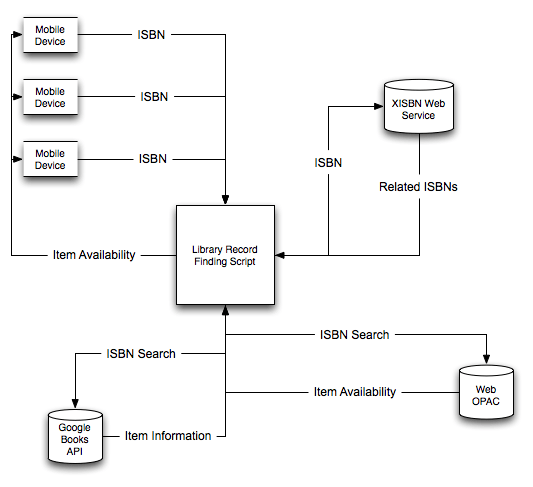
Once the dependencies are installed, for both Android and iOS, you must link the native dependencies of the camera and barcode scanning libraries. With React Native version 0.60 and above, this linking should happen automatically. However, if you’re using an older version of React Native, Web Developer Certification Courses need to link the package manually. On Android, camera permissions need to be declared in the AndroidManifest.xml file, ensuring that the app has access to the device’s camera. You’ll need to include permissions for camera access and autofocus, and possibly request permission during runtime, depending on the platform version. For iOS, you must request camera access permission in the Info.plist file, specifying that the app needs access to the camera for scanning purposes. After configuring these settings, remember to rebuild your app to ensure everything is correctly set up. It’s important to thoroughly test barcode and QR code scanning functionality on both Android and iOS platforms to ensure smooth performance across devices. Additionally, ensure that the camera permissions are handled gracefully to avoid disruptions during scanning. Consider implementing error handling for cases where the user denies camera access. With everything in place, you’ll be ready to integrate barcode and QR code scanning seamlessly into your React Native app.
Boost your career in tech by enrolling in this Web Developer Certification Course today.
Creating the UI for the Barcode/QR Scanner
- Designing the UI: The user interface should include a camera view for scanning barcodes and QR codes. It should also include a result view to display the information extracted from the scan.
- Basic Scanner Screen UI Setup: In App.js, import the necessary components (View, Text, StyleSheet, and CameraKitCameraScreen from react-native-camera-kit).
- Setting Up Camera View: Create a camera view using the CameraKitCameraScreen component. The camera view should fill the entire screen by setting Http Request Methods style to have width: ‘100%’ and height: ‘100%’.
- Configuring Camera Options: Set camera options such as flash mode (auto, on, off), focus mode (on, off), and zoom mode (on, off).
- Handling Barcode/QR Code Scans: Define the onScanCode function to handle the scan result whenever a barcode or QR code is detected. This function receives the scan result and can be used to display or process the scanned data.
- Styling the UI: Apply basic styles to the camera and container using StyleSheet.create(). Ensure that the container uses flex: 1 to take up the full screen and centers the content.
- Final Touch: Ensure that when the app detects a barcode or QR code, the scan result is logged or displayed on the screen. These points guide you through setting up the user interface for the barcode/QR scanner in React Native.
Implementing Barcode/QR Code Detection
The react-native-camera-kit library provides built-in support for barcode and QR code scanning, making it easy to implement detection in your app. The camera view continuously scans for barcodes and QR codes, and once a valid code is detected, the onBarcodeScan event is triggered. The scan result contains essential information, such as the type of code detected (e.g., QR code or barcode) and the data encoded within it (such as a URL, product ID, or text). For instance, you can process the scan result with a simple function like onScanCode, which handles the barcode/QR code data once it’s detected. When a code is scanned, the event passes the data, allowing you to display it or use it in the app, such as showing the URL in a browser or retrieving product details from a database. In the example provided, the scanned data is displayed via an Downloading Node Js and NPM, showing the value of the scanned code. This functionality can be extended to suit your app’s needs, such as redirecting users to a URL or storing the scanned product ID for further processing. By using this built-in feature, you can quickly and effectively implement barcode and QR code detection in your React Native app. Additionally, you can apply custom logic to handle different types of scanned data based on the code’s content.
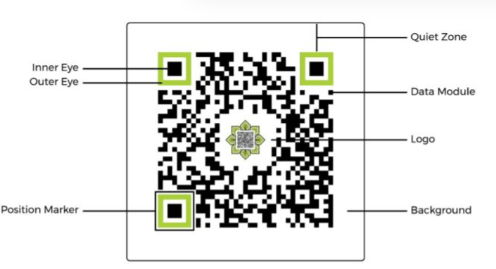
Handling Barcode/QR Data
Once a barcode or QR code is scanned, the app must handle the scanned data appropriately based on the use case, such as a URL, product ID, or text.
- Handling URL Scans: If the scanned data is a URL (e.g., starting with “http://” or “https://”), you can navigate the user to the URL using React Native’s Linking module.
- Example steps: Import the Linking module from React Native. Define an onScanCode function that processes the scan result. Check if the scanned data starts with “http://” or “https://”. Use Linking.openURL() to open the URL in the device’s default browser. Handle errors with .catch() if the URL fails to open.
- Handling Product or Text Data: If the scanned data is not a URL but instead a product ID, text, or other types of data: You can display the information in a modal or alert. Alternatively, save the data in a database for future reference. You can also trigger specific actions based on the React Native and Barcode, like searching for product details or showing a confirmation message.
- Additional Actions: For other types of scanned data, you may: Open a specific screen in the app (e.g., product details or a search page). Log the data for analytics or tracking purposes. Implement features like adding the scanned product to a cart or wishlist.
- Error Handling: Always ensure that proper error handling is in place to handle invalid or unrecognized QR/barcode data, or if there is an issue with opening a URL.
- User Experience: To enhance the user experience, consider providing feedback after a scan, such as displaying a message or a progress bar while the app processes the scan result.
Start your journey in Web Development by enrolling in this Web Developer Certification Courses .
Optimizing for Different Devices and Platforms
It is crucial to ensure a React Native app performs well on Android and iOS. This includes optimizing the camera performance and guaranteeing the UI adapts to different screen sizes.
- Camera Performance Optimization: Optimizing camera performance can improve the scanning experience on both Android and iOS. For instance, limiting the camera resolution to a lower setting can improve performance on lower-end devices. Additionally, ensure that the app checks for camera permissions before trying to use the camera.
- UI Responsiveness: Since React Native supports a variety of device sizes, you should use responsive design principles to ensure the app looks great on different screen sizes. You can use the Dimensions API to get the screen width and height and adjust the camera preview accordingly
Preparing for Web Development interviews? Visit our blog for the best Web Development Interview Questions and Answers!
Publishing and Maintaining the App
Once the app is ready and thoroughly tested, Control Statements in Java time to publish it. The publishing process for React Native apps is similar to that of any other mobile app. Publishing to the Google Play Store For Android, follow these steps: Generate a signed APK or AAB file by running the following command: cd android Upload the generated APK/AAB file to the Google Play Console. Publishing to the Apple App Store: For iOS, you need a macOS machine with Xcode to create an app archive. Afterward, you can upload the app to App Store Connect for submission. Test your app on real devices and ensure it meets Apple and Google’s guidelines. Maintenance: Once the app is published, ensure you monitor user feedback, fix bugs, and release updates as needed. React Native lets you quickly deploy OTA (Over-the-Air) updates using services like CodePush.
Conclusion
Building a barcode and QR scanner app with React Native is straightforward, thanks to powerful libraries like react-native-camera-kit. Following the Web Designing Training outlined in this guide, you can set up the environment, integrate scanning functionality, design a user-friendly interface, and optimize the app for different devices and platforms. With the app published and maintained, you can deliver a seamless scanning experience to users across Android and iOS devices.